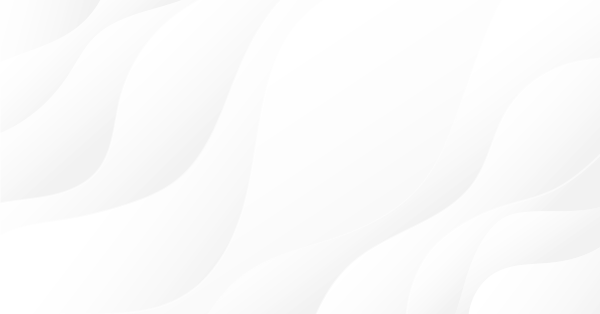
Traslado de MQL4 a MQL5
Introducción
Muchos desarrolladores han acumulado muchos indicadores y estrategias de trading escritas en MQL4. Para usarlos en Metatrader 5, se deben convertir a MQL5. No es fácil reescribir todos los programas para MQL5. Sería mucho más fácil convertirlos si hubiera una referencia de traducción, y aún mejor con ejemplos.
En este artículo me gustaría sugerir mi versión de una guía para migrar de MQL4 a MQL5.
1. Períodos de Gráfico
En MQL5, las constantes de los períodos del gráfico cambian, y se han añadido algunos períodos de tiempo nuevos (M2, M3, M4, M6, M10, M12, H2, H3, H6, H8, H12). Para convertir los períodos de tiempo de MQL4, puede usar la siguiente función:
ENUM_TIMEFRAMES TFMigrate(int tf) { switch(tf) { case 0: return(PERIOD_CURRENT); case 1: return(PERIOD_M1); case 5: return(PERIOD_M5); case 15: return(PERIOD_M15); case 30: return(PERIOD_M30); case 60: return(PERIOD_H1); case 240: return(PERIOD_H4); case 1440: return(PERIOD_D1); case 10080: return(PERIOD_W1); case 43200: return(PERIOD_MN1); case 2: return(PERIOD_M2); case 3: return(PERIOD_M3); case 4: return(PERIOD_M4); case 6: return(PERIOD_M6); case 10: return(PERIOD_M10); case 12: return(PERIOD_M12); case 16385: return(PERIOD_H1); case 16386: return(PERIOD_H2); case 16387: return(PERIOD_H3); case 16388: return(PERIOD_H4); case 16390: return(PERIOD_H6); case 16392: return(PERIOD_H8); case 16396: return(PERIOD_H12); case 16408: return(PERIOD_D1); case 32769: return(PERIOD_W1); case 49153: return(PERIOD_MN1); default: return(PERIOD_CURRENT); } }
Se debe señalar que, en MQL5, los valores numéricos de las constantes del intervalo de gráfico (de H1) no son iguales al número de minutos de una barra (por ejemplo, en MQL5, el valor numérico de la constante PERIOD_H1=16385, pero en MQL4 PERIOD_H1=60). Debe tenerlo en cuenta al convertirlos a MQL5 si los valores numéricos de las constantes de MQL4 se usan en programas MQL4.
Para determinar el número de minutos del período de tiempo especificado en el gráfico, divida el valor devuelto por la función PeriodSeconds por 60.
2. Declarar Constantes
Algunas de las constantes estándar de MQL4 no están en MQL5, y por tanto se deben declarar:
//+------------------------------------------------------------------+ //| InitMQL4.mqh | //| Copyright DC2008 | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "keiji" #property copyright "DC2008" #property link "https://www.mql5.com" //--- Declaration of constants #define OP_BUY 0 //Buy #define OP_SELL 1 //Sell #define OP_BUYLIMIT 2 //Pending order of BUY LIMIT type #define OP_SELLLIMIT 3 //Pending order of SELL LIMIT type #define OP_BUYSTOP 4 //Pending order of BUY STOP type #define OP_SELLSTOP 5 //Pending order of SELL STOP type //--- #define MODE_OPEN 0 #define MODE_CLOSE 3 #define MODE_VOLUME 4 #define MODE_REAL_VOLUME 5 #define MODE_TRADES 0 #define MODE_HISTORY 1 #define SELECT_BY_POS 0 #define SELECT_BY_TICKET 1 //--- #define DOUBLE_VALUE 0 #define FLOAT_VALUE 1 #define LONG_VALUE INT_VALUE //--- #define CHART_BAR 0 #define CHART_CANDLE 1 //--- #define MODE_ASCEND 0 #define MODE_DESCEND 1 //--- #define MODE_LOW 1 #define MODE_HIGH 2 #define MODE_TIME 5 #define MODE_BID 9 #define MODE_ASK 10 #define MODE_POINT 11 #define MODE_DIGITS 12 #define MODE_SPREAD 13 #define MODE_STOPLEVEL 14 #define MODE_LOTSIZE 15 #define MODE_TICKVALUE 16 #define MODE_TICKSIZE 17 #define MODE_SWAPLONG 18 #define MODE_SWAPSHORT 19 #define MODE_STARTING 20 #define MODE_EXPIRATION 21 #define MODE_TRADEALLOWED 22 #define MODE_MINLOT 23 #define MODE_LOTSTEP 24 #define MODE_MAXLOT 25 #define MODE_SWAPTYPE 26 #define MODE_PROFITCALCMODE 27 #define MODE_MARGINCALCMODE 28 #define MODE_MARGININIT 29 #define MODE_MARGINMAINTENANCE 30 #define MODE_MARGINHEDGED 31 #define MODE_MARGINREQUIRED 32 #define MODE_FREEZELEVEL 33 //--- #define EMPTY -1Nota: Las constantes en MQL4 y MQL5 son diferentes, y por tanto es mejor declararlas en un archivo separado initMQ4.mqh para su uso futuro.
3. Variables Predefinidas
MQL4 | MQL5 | Descripción |
---|---|---|
double Ask | MqlTick last_tick; SymbolInfoTick(_Symbol,last_tick); double Ask=last_tick.ask; | Ask El último precio de demanda conocido para el símbolo actual. SymbolInfoTick |
int Bars | int Bars=Bars(_Symbol,_Period); | Bars Número de barras en el gráfico actual. Bars |
double Bid | MqlTick last_tick; SymbolInfoTick(_Symbol,last_tick); double Bid=last_tick.bid; | Bid El último precio de oferta conocido del símbolo actual. SymbolInfoTick |
double Close[] | double Close[]; int count; // number of elements to copy ArraySetAsSeries(Close,true); CopyClose(_Symbol,_Period,0,count,Close); | Close Array de series que contiene los precios de cierre para cada barra del gráfico actual. CopyClose, ArraySetAsSeries |
int Digits | int Digits=_Digits; | Digits Número de dígitos después de la coma decimal para los precios actuales del símbolo. Digits |
double High[] | double High[]; int count; // number of elements to copy ArraySetAsSeries(High,true); CopyHigh(_Symbol,_Period,0,count,High); | High Array de series que contiene los precios más altos de cada barra del gráfico actual. CopyHigh, ArraySetAsSeries |
double Low[] | double Low[]; int count; // number of elements to copy ArraySetAsSeries(Low,true); CopyLow(_Symbol,_Period,0,count,Low); | Low Array de series que contiene los precios más bajos de cada barra del gráfico actual. CopyLow, ArraySetAsSeries |
double Open[] | double Open[]; int count; // number of elements to copy ArraySetAsSeries(Open,true); CopyOpen(_Symbol,_Period,0,count,Open); | Open Array de series que contiene los precios de apertura de cada barra del gráfico actual. CopyOpen, ArraySetAsSeries |
double Point | double Point=_Point; | Point El valor puntual del símbolo actual en la divisa de cuota. _Point |
datetime Time[] | datetime Time[]; int count; // number of elements to copy ArraySetAsSeries(Time,true); CopyTime(_Symbol,_Period,0,count,Time); | Time Array de series que contiene los precios de apertura para cada barra del gráfico actual. Los datos como datetime representan el tiempo, en segundos, que ha pasado desde las 00:00 a.m. de enero de 1970. CopyTime, ArraySetAsSeries |
double Volume[] | long Volume[]; int count; // number of elements to copy ArraySetAsSeries(Volume,true); CopyTickVolume(_Symbol,_Period,0,count,Volume); | Volume Array de series que contiene los volúmenes de ticks de cada barra del gráfico actual. CopyTickVolume, ArraySetAsSeries |
4. Información de Cuenta
MQL4 | MQL5 | Descripción |
---|---|---|
double AccountBalance() | double AccountInfoDouble(ACCOUNT_BALANCE) | AccountBalance Devuelve el valor de saldo de la cuenta actual (la cantidad de dinero en la cuenta). AccountInfoDouble |
double AccountCredit() | double AccountInfoDouble(ACCOUNT_CREDIT) | AccountCredit Devuelve el valor de crédito de la cuenta actual. AccountInfoDouble |
string AccountCompany() | string AccountInfoString(ACCOUNT_COMPANY) | AccountCompany Devuelve el nombre de la correduría de bolsa en la que se registró la cuenta actual. AccountInfoString |
string AccountCurrency() | string AccountInfoString(ACCOUNT_CURRENCY) | AccountCurrency Devuelve el nombre de la cuenta actual. AccountInfoString |
double AccountEquity() | double AccountInfoDouble(ACCOUNT_EQUITY) | AccountEquity Devuelve el valor de beneficio de la cuenta actual. El cálculo de patrimonio depende de la configuración del servidor de trading. AccountInfoDouble |
double AccountFreeMargin() | double AccountInfoDouble(ACCOUNT_FREEMARGIN) | AccountFreeMargin Devuelve el valor de margen libre de la cuenta actual. AccountInfoDouble |
double AccountFreeMarginCheck(string symbol, int cmd, double volume) | - | AccountFreeMarginCheck Devuelve el margen libre que queda después de que la posición especificada se haya abierto al precio actual en la cuenta actual. |
double AccountFreeMarginMode() | - | AccountFreeMarginMode Modo de cálculo de margen libre permitido para posiciones abiertas en la cuenta actual. |
int AccountLeverage() | int AccountInfoInteger(ACCOUNT_LEVERAGE) | AccountLeverage Devuelve el apalancamiento de la cuenta actual. AccountInfoInteger |
double AccountMargin() | double AccountInfoDouble(ACCOUNT_MARGIN) | AccountMargin Devuelve el valor de margen de la cuenta actual. AccountInfoDouble |
string AccountName() | string AccountInfoString(ACCOUNT_NAME) | AccountName Devuelve el nombre de la cuenta. AccountInfoString |
int AccountNumber() | int AccountInfoInteger(ACCOUNT_LOGIN) | AccountNumber Devuelve el número de la cuenta actual. AccountInfoInteger |
double AccountProfit() | double AccountInfoDouble(ACCOUNT_PROFIT) | AccountProfit Devuelve el valor de beneficio de la cuenta actual. AccountInfoDouble |
string AccountServer() | string AccountInfoString(ACCOUNT_SERVER) | AccountServer Devuelve el nombre del servidor conectado. AccountInfoString |
int AccountStopoutLevel() | double AccountInfoDouble(ACCOUNT_MARGIN_SO_SO) | AccountStopoutLevel Devuelve el valor del nivel Stop Out. AccountInfoDouble |
int AccountStopoutMode() | int AccountInfoInteger(ACCOUNT_MARGIN_SO_MODE) | AccountStopoutMode Devuelve el modo de cálculo del nivel Stop Out. AccountInfoInteger |
5. Funciones de Array
MQL4 | MQL5 | Descripción |
---|---|---|
int ArrayBsearch(double array[], double value, int count=WHOLE_ARRAY, int start=0, int direction=MODE_ASCEND) | int ArrayBsearch(double array[], double searched_value ) | ArrayBsearch La función busca un valor especificado en un array numérico unidimensional. ArrayBsearch |
int ArrayCopy(object&dest[], object source[], int start_dest=0, int start_source=0, int count=WHOLE_ARRAY) | int ArrayCopy(void dst_array[], void src_array[], int dst_start=0, int src_start=0, int cnt=WHOLE_ARRAY ) | ArrayCopy Copia un array a otro. Los arrays deben ser del mismo tipo, pero los arrays del tipo double[], int[], datetime[], color[], y bool[] se pueden copiar como arrays del mismo tipo. Devuelve la cantidad de elementos copiados. ArrayCopy |
int ArrayCopyRates(double&dest_array[], string symbol=NULL, int timeframe=0) | - | ArrayCopyRates Copia datos de las barras del gráfico actual al array bidimensional del tipo RateInfo[][6] y devuelve la cantidad de barras copiadas, o -1 si falló. |
int ArrayCopySeries(double&array[], int series_index, string symbol=NULL, int timeframe=0) | int ArrayCopySeriesMQL4(double &array[], int series_index, string symbol=NULL, int tf=0) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); int count=Bars(symbol,timeframe); switch(series_index) { case MODE_OPEN: return(CopyOpen(symbol,timeframe,0,count,array)); case MODE_LOW: return(CopyLow(symbol,timeframe,0,count,array)); case MODE_HIGH: return(CopyHigh(symbol,timeframe,0,count,array)); case MODE_CLOSE: return(CopyClose(symbol,timeframe,0,count,array)); default: return(0); } return(0); } | ArrayCopySeries Copia un array de series de tiempo a un array personalizado y devuelve el número de elementos copiados. CopyOpen, CopyLow, CopyHigh, CopyClose, Bars |
int ArrayDimension( object array[]) | - | ArrayDimension Devuelve el rango del array multidimensional. |
bool ArrayGetAsSeries( object array[]) | bool ArrayGetAsSeries(void array) | ArrayGetAsSeries Devuelve TRUE si un array se organizó como un array de series de tiempo (los elementos del array se indexan del último al primero), de lo contrario devolverá FALSE. ArrayGetAsSeries |
int ArrayInitialize(double &array[], double value) | int ArrayInitializeMQL4(double &array[], double value) { ArrayInitialize(array,value); return(ArraySize(array)); } | ArrayInitialize Configura todos los elementos de un array numérico al mismo valor. Devuelve el número de elementos inicializados. ArrayInitialize, ArraySize |
bool ArrayIsSeries( object array[]) | bool ArrayIsSeries(void array[]) | ArrayIsSeries Devuelve TRUE si el array que se revisa es un array de series de tiempo (Time[],Open[],Close[],High[],Low[], o Volume[]), de lo contrario devolverá FALSE. ArrayIsSeries |
int ArrayMaximum(double array[], int count=WHOLE_ARRAY, int start=0) | int ArrayMaximumMQL4(double &array[], int count=WHOLE_ARRAY, int start=0) { return(ArrayMaximum(array,start,count)); } | ArrayMaximum Busca el elemento con el máximo valor. La función devuelve la posición de este elemento máximo en el array. ArrayMaximum |
int ArrayMinimum(double array[], int count=WHOLE_ARRAY, int start=0) | int ArrayMinimumMQL4(double &array[], int count=WHOLE_ARRAY, int start=0) { return(ArrayMinimum(array,start,count)); } | ArrayMinimum Busca el elemento con el valor mínimo. La función devuelve la posición de este elemento mínimo en el array. ArrayMinimum |
int ArrayRange(object array[], int range_index) | int ArrayRange(void array[], int rank_index ) | ArrayRange Devuelve el número de elementos en la dimensión dada del array. ArrayRange |
int ArrayResize(object &array[], int new_size) | int ArrayResize(void array[], int new_size, int allocated_size=0 ) | ArrayResize Configura un nuevo tamaño para la primera dimensión. ArrayResize |
bool ArraySetAsSeries(double &array[], bool set) | bool ArraySetAsSeries(void array[], bool set ) | ArraySetAsSeries Devuelve el número de elementos en la dimensión dada del array. Puesto que los índices están basados en cero, el tamaño de la dimensión es 1 más grande que el índice mayor. ArraySetAsSeries |
int ArraySize( object array[]) | int ArraySize(void array[]) | ArraySize Devuelve el número de elementos contenidos en el array. ArraySize |
int ArraySort(double &array[], int count=WHOLE_ARRAY, int start=0, int sort_dir=MODE_ASCEND) | int ArraySortMQL4(double &array[], int count=WHOLE_ARRAY, int start=0, int sort_dir=MODE_ASCEND) { switch(sort_dir) { case MODE_ASCEND: ArraySetAsSeries(array,true); case MODE_DESCEND: ArraySetAsSeries(array,false); default: ArraySetAsSeries(array,true); } ArraySort(array); return(0); } | ArraySort Ordena los arrays numéricos por su primera dimensión. Las series de arrays no se pueden ordenar por ArraySort(). ArraySort, ArraySetAsSeries |
6. Revisión
MQL4 | MQL5 | Descripción |
---|---|---|
int GetLastError() | int GetLastError() | GetLastError La función devuelve el último error ocurrido, y después el valor de la variable especial last_error donde el último código de error se guarda pasará a ser cero. GetLastError |
bool IsConnected() | bool TerminalInfoInteger(TERMINAL_CONNECTED) | IsConnected La función devuelve el estado de la principal conexión entre el terminal de cliente y el servidor que realiza la infusión de datos. Devuelve TRUE si la conexión con el servidor se estableció con éxito, de lo contrario devolverá FALSE. TerminalInfoInteger |
bool IsDemo() | bool IsDemoMQL4() { if(AccountInfoInteger(ACCOUNT_TRADE_MODE)==ACCOUNT_TRADE_MODE_DEMO) return(true); else return(false); } | IsDemo DevuelveTRUE si el Experto se ejecuta en una cuenta de prueba, de lo contrario devolverá FALSE. AccountInfoInteger |
bool IsDllsAllowed() | bool TerminalInfoInteger(TERMINAL_DLLS_ALLOWED) | IsDllsAllowed Devuelve TRUE si la función de llamada a DLL se permite para el Asesor Experto, de lo contrario devolverá FALSE. TerminalInfoInteger |
bool IsExpertEnabled() | bool AccountInfoInteger(ACCOUNT_TRADE_EXPERT) | IsExpertEnabled Devuelve TRUE si el uso de Asesores Expertos está activo en el terminal de cliente, de lo contrario devolverá FALSE. AccountInfoInteger |
bool IsLibrariesAllowed() | bool MQLInfoInteger(MQL5_DLLS_ALLOWED) | IsLibrariesAllowed Devuelve TRUE si un Asesor Experto puede llamar a funciones de la biblioteca, de lo contrario devolverá FALSE. MQLInfoInteger |
bool IsOptimization() | bool MQLInfoInteger(MQL5_OPTIMIZATION) | IsOptimization Devuelve TRUE si un Asesor Experto se está ejecutando en el modo de optimización del Probador de Estrategias, de lo contrario devolverá FALSE. MQLInfoInteger |
bool IsStopped() | bool IsStopped() | IsStopped Devuelve TRUE si el programa (un Asesor Experto o un script) ha recibido el comando de detener sus operaciones, de lo contrario devolverá FALSE. IsStopped |
bool IsTesting() | bool MQLInfoInteger(MQL5_TESTING) | IsTesting Devuelve TRUE si un Asesor Experto se está ejecutando en el modo de simulación, de lo contrario devolverá FALSE. MQLInfoInteger |
bool IsTradeAllowed() | bool MQLInfoInteger(MQL5_TRADE_ALLOWED) | IsTradeAllowed Devuelve TRUE si el trading con el Asesor Experto se permite y el canal para trading no está ocupado, de lo contrario devolverá FALSE. MQLInfoInteger |
bool IsTradeContextBusy() | - | IsTradeContextBusy Devuelve TRUE si un canal para trading está ocupado por otro Asesor Experto, de lo contrario devolverá FALSE. |
bool IsVisualMode() | bool MQLInfoInteger(MQL5_VISUAL_MODE) | IsVisualMode Devuelve TRUE si el Asesor Experto se simula con el botón "Visual Mode" ("Modo Visual") activado, de lo contrario devolverá FALSE. MQLInfoInteger |
int UninitializeReason() | int UninitializeReason() | UninitializeReason Devuelve el código para la razón de desinicialización para Asesores Expertos, indicadores personalizados y scripts. UninitializeReason |
7. Terminal de Cliente
MQL4 | MQL5 | Descripción |
---|---|---|
string TerminalCompany() | string TerminalInfoString(TERMINAL_COMPANY) | TerminalCompany Devuelve el nombre de la compañía a la que pertenece el terminal de cliente. TerminalInfoString |
string TerminalName() | string TerminalInfoString(TERMINAL_NAME) | TerminalName Devuelve el nombre el terminal de cliente. TerminalInfoString |
string TerminalPath() | string TerminalInfoString(TERMINAL_PATH) | TerminalPath Devuelve el directorio del que se ejecutó el terminal de cliente. TerminalInfoString |
8. Funciones Comunes
MQL4 | MQL5 | Descripción |
---|---|---|
void Alert(...) | void Alert(argument,...) | Alert Muestra un cuadro de diálogo conteniendo los datos definidos por el usuario. Los parámetros pueden ser de cualquier tipo. Alert |
void Comment(...) | void Comment(argument,...) | Comment La función imprime el comentario definido por el usuario en la esquina superior izquierda del gráfico. Comment |
int GetTickCount() | uint GetTickCount() | GetTickCount La función GetTickCount() recupera el número de milisegundos que han pasado desde que el sistema se inició. GetTickCount |
double MarketInfo(string symbol, int type) | double MarketInfoMQL4(string symbol, int type) { switch(type) { case MODE_LOW: return(SymbolInfoDouble(symbol,SYMBOL_LASTLOW)); case MODE_HIGH: return(SymbolInfoDouble(symbol,SYMBOL_LASTHIGH)); case MODE_TIME: return(SymbolInfoInteger(symbol,SYMBOL_TIME)); case MODE_BID: return(Bid); case MODE_ASK: return(Ask); case MODE_POINT: return(SymbolInfoDouble(symbol,SYMBOL_POINT)); case MODE_DIGITS: return(SymbolInfoInteger(symbol,SYMBOL_DIGITS)); case MODE_SPREAD: return(SymbolInfoInteger(symbol,SYMBOL_SPREAD)); case MODE_STOPLEVEL: return(SymbolInfoInteger(symbol,SYMBOL_TRADE_STOPS_LEVEL)); case MODE_LOTSIZE: return(SymbolInfoDouble(symbol,SYMBOL_TRADE_CONTRACT_SIZE)); case MODE_TICKVALUE: return(SymbolInfoDouble(symbol,SYMBOL_TRADE_TICK_VALUE)); case MODE_TICKSIZE: return(SymbolInfoDouble(symbol,SYMBOL_TRADE_TICK_SIZE)); case MODE_SWAPLONG: return(SymbolInfoDouble(symbol,SYMBOL_SWAP_LONG)); case MODE_SWAPSHORT: return(SymbolInfoDouble(symbol,SYMBOL_SWAP_SHORT)); case MODE_STARTING: return(0); case MODE_EXPIRATION: return(0); case MODE_TRADEALLOWED: return(0); case MODE_MINLOT: return(SymbolInfoDouble(symbol,SYMBOL_VOLUME_MIN)); case MODE_LOTSTEP: return(SymbolInfoDouble(symbol,SYMBOL_VOLUME_STEP)); case MODE_MAXLOT: return(SymbolInfoDouble(symbol,SYMBOL_VOLUME_MAX)); case MODE_SWAPTYPE: return(SymbolInfoInteger(symbol,SYMBOL_SWAP_MODE)); case MODE_PROFITCALCMODE: return(SymbolInfoInteger(symbol,SYMBOL_TRADE_CALC_MODE)); case MODE_MARGINCALCMODE: return(0); case MODE_MARGININIT: return(0); case MODE_MARGINMAINTENANCE: return(0); case MODE_MARGINHEDGED: return(0); case MODE_MARGINREQUIRED: return(0); case MODE_FREEZELEVEL: return(SymbolInfoInteger(symbol,SYMBOL_TRADE_FREEZE_LEVEL)); default: return(0); } return(0); } | MarketInfo Devuelve datos varios sobre seguridad alistados en la ventana Market Watch (Observación de Mercado). SymbolInfoInteger, SymbolInfoDouble, Bid, Ask |
int MessageBox(string text=NULL, string caption=NULL, int flags=EMPTY) | int MessageBox(string text, string caption=NULL, int flags=0) | MessageBox La función MessageBox crea, muestra y opera el cuadro de mensajes. MessageBox |
void PlaySound(string filename) | bool PlaySound(string filename) | PlaySound Función para reproducir un archivo de sonido. PlaySound |
void Print(...) | void Print(argument,...) | Print Imprime un mensaje en el registro del expert. |
bool SendFTP(string filename, string ftp_path=NULL) | bool SendFTP(string filename, string ftp_path=NULL) | SendFTP Envía el archivo al servidor FTP configurado en la pestaña Tools->Options->Publisher (Herramientas->Opciones->Publicador). Si el intento falla, devolverá FALSE. SendFTP |
void SendMail(string subject, string some_text) | bool SendMail(string subject, string some_text) | SendMail Envía un mensaje a la dirección de correo electrónico configurada en la pestaña Tools->Options->EMail (Herramientas->Opciones->Correo electrónico). SendMail |
void Sleep(int milliseconds) | void Sleep(int milliseconds) | Sleep La función Sleep() suspende la ejecución del experto actual dentro del intervalo especificado. Sleep |
9. Funciones de Conversión
MQL4 | MQL5 | Descripción |
---|---|---|
string CharToStr(int char_code) | string CharToString(int char_code) | CharToStr Conversión del código de símbolo en una cadena de caracteres de un carácter. CharToString |
string DoubleToStr(double value, int digits) | string DoubleToString(double value, int digits=8) | DoubleToStr Devuelve una cadena de caracteres de texto con el valor numérico especificado convertido en un formato de precisión especificado. DoubleToString |
double NormalizeDouble(double value, int digits) | double NormalizeDouble(double value, int digits) | NormalizeDouble Redondea el valor de punto flotante a la precisión especificada. Devuelve un valor normalizado de tipo doble NormalizeDouble |
double StrToDouble(string value) | double StringToDouble(string value) | StrToDouble Convierte la representación de la cadena de caracteres de números a tipo doble (formato de doble precisión con punto flotante). StringToDouble |
int StrToInteger(string value) | long StringToInteger(string value) | StrToInteger Convierte la cadena de caracteres con la representación de carácter de valor en un valor de tipo int (número íntegro). StringToInteger |
datetime StrToTime(string value) | datetime StringToTime(string value) | StrToTime Convierte una cadena de caracteres en formato "yyyy.mm.dd hh:mi" al tipo datetime (la cantidad de segundos pasados desde el 1 de enero de 1970). StringToTime |
string TimeToStr(datetime value, int mode=TIME_DATE|TIME_MINUTES) | string TimeToString(datetime value, int mode=TIME_DATE|TIME_MINUTES) | TimeToStr Convierte el valor que contiene el tiempo en segundos que han pasado desde el 1 de enero de 1970 en una cadena de caracteres de formato "yyyy.mm.dd hh:mi". TimeToString |
10. Indicadores Personalizados
MQL4 | MQL5 | Descripción |
---|---|---|
void IndicatorBuffers(int count) | - | IndicatorBuffers Distribuye la memoria para buffers usados para cálculos de indicador personalizados. |
int IndicatorCounted() | int IndicatorCountedMQL4() { if(prev_calculated>0) return(prev_calculated-1); if(prev_calculated==0) return(0); return(0); } | IndicatorCounted La función devuelve la cantidad de barras que no han cambiado después de la última ejecución del indicador. OnCalculate |
void IndicatorDigits(int digits) | bool IndicatorSetInteger(INDICATOR_DIGITS,digits) | IndicatorDigits Configura el formato de precisión (la cuenta de dígitos tras la coma decimal) para visualizar los valores de indicador. IndicatorSetInteger |
void IndicatorShortName(string name) | bool IndicatorSetString(INDICATOR_SHORTNAME,name) | IndicatorShortName Configura el nombre "corto" de un indicador personalizado que se mostrará en la ventana DataWindow y en la subventana del gráfico. IndicatorSetString |
void SetIndexArrow(int index, int code) | bool PlotIndexSetInteger(index,PLOT_ARROW,code) | SetIndexArrow Configura un símbolo de flecha para la línea del indicador del tipo DRAW_ARROW. PlotIndexSetInteger |
bool SetIndexBuffer(int index, double array[]) | bool SetIndexBuffer(index,array,INDICATOR_DATA) | SetIndexBuffer Vincula la variable de array declarada en un nivel global al buffer predefinido del indicador personalizado. SetIndexBuffer |
void SetIndexDrawBegin(int index, int begin) | bool PlotIndexSetInteger(index,PLOT_DRAW_BEGIN,begin) | SetIndexDrawBegin Configura el número de barras (desde el comienzo de los datos) desde las que debe empezar el dibujo de la línea del indicador especificado. PlotIndexSetInteger |
void SetIndexEmptyValue(int index, double value) | bool PlotIndexSetDouble(index,PLOT_EMPTY_VALUE,value) | SetIndexEmptyValue Configura el valor vacío de la línea de dibujo. PlotIndexSetDouble |
void SetIndexLabel(int index, string text) | bool PlotIndexSetString(index,PLOT_LABEL,text) | SetIndexLabel Configura la descripción de la línea de dibujo que se mostrará en la ventana DataWindow y en el tooltip. PlotIndexSetString |
void SetIndexShift(int index, int shift) | bool PlotIndexSetInteger(index,PLOT_SHIFT,shift) | SetIndexShift Configura el offset de la línea de dibujo. PlotIndexSetInteger |
void SetIndexStyle(int index, int type, int style=EMPTY, int width=EMPTY, color clr=CLR_NONE) | void SetIndexStyleMQL4(int index, int type, int style=EMPTY, int width=EMPTY, color clr=CLR_NONE) { if(width>-1) PlotIndexSetInteger(index,PLOT_LINE_WIDTH,width); if(clr!=CLR_NONE) PlotIndexSetInteger(index,PLOT_LINE_COLOR,clr); switch(type) { case 0: PlotIndexSetInteger(index,PLOT_DRAW_TYPE,DRAW_LINE); case 1: PlotIndexSetInteger(index,PLOT_DRAW_TYPE,DRAW_SECTION); case 2: PlotIndexSetInteger(index,PLOT_DRAW_TYPE,DRAW_HISTOGRAM); case 3: PlotIndexSetInteger(index,PLOT_DRAW_TYPE,DRAW_ARROW); case 4: PlotIndexSetInteger(index,PLOT_DRAW_TYPE,DRAW_ZIGZAG); case 12: PlotIndexSetInteger(index,PLOT_DRAW_TYPE,DRAW_NONE); default: PlotIndexSetInteger(index,PLOT_DRAW_TYPE,DRAW_LINE); } switch(style) { case 0: PlotIndexSetInteger(index,PLOT_LINE_STYLE,STYLE_SOLID); case 1: PlotIndexSetInteger(index,PLOT_LINE_STYLE,STYLE_DASH); case 2: PlotIndexSetInteger(index,PLOT_LINE_STYLE,STYLE_DOT); case 3: PlotIndexSetInteger(index,PLOT_LINE_STYLE,STYLE_DASHDOT); case 4: PlotIndexSetInteger(index,PLOT_LINE_STYLE,STYLE_DASHDOTDOT); default: return; } | SetIndexStyle Configura el nuevo tipo, estilo, anchura y color para una línea de indicador especificada. PlotIndexSetInteger |
void SetLevelStyle(int draw_style, int line_width, color clr=CLR_NONE) | void SetLevelStyleMQL4(int draw_style, int line_width, color clr=CLR_NONE) { IndicatorSetInteger(INDICATOR_LEVELWIDTH,line_width); if(clr!=CLR_NONE) IndicatorSetInteger(INDICATOR_LEVELCOLOR,clr); switch(draw_style) { case 0: IndicatorSetInteger(INDICATOR_LEVELSTYLE,STYLE_SOLID); case 1: IndicatorSetInteger(INDICATOR_LEVELSTYLE,STYLE_DASH); case 2: IndicatorSetInteger(INDICATOR_LEVELSTYLE,STYLE_DOT); case 3: IndicatorSetInteger(INDICATOR_LEVELSTYLE,STYLE_DASHDOT); case 4: IndicatorSetInteger(INDICATOR_LEVELSTYLE,STYLE_DASHDOTDOT); default: return; } } | SetLevelStyle La función configura un nuevo estilo, anchura y color de niveles horizontales de indicador que se imprimirán en una ventana separada. IndicatorSetInteger |
void SetLevelValue(int level, double value) | bool IndicatorSetDouble(INDICATOR_LEVELVALUE,level,value) | SetLevelValue La función configura un valor para un nivel horizontal del indicador especificado que se imprimirán en una ventana separada. IndicatorSetDouble |
11. Funciones de Fecha y Hora
MQL4 | MQL5 | Descripción |
---|---|---|
int Day() | int DayMQL4() { MqlDateTime tm; TimeCurrent(tm); return(tm.day); } | Day Devuelve el día del mes actual, es decir, el día del mes de la última fecha del servidor conocida. TimeCurrent, MqlDateTime |
int DayOfWeek() | int DayOfWeekMQL4() { MqlDateTime tm; TimeCurrent(tm); return(tm.day_of_week); } | DayOfWeek Devuelve el día actual de la semana actual basado en 0 (0=domingo,1,2,3,4,5,6) de la última fecha del servidor conocida. TimeCurrent, MqlDateTime |
int DayOfYear() | int DayOfYearMQL4() { MqlDateTime tm; TimeCurrent(tm); return(tm.day_of_year); } | DayOfYear Devuelve el día del año actual (1 significa 1 de enero,..,365(6) significa 31 de diciembre), es decir, el día del año de la última fecha del servidor conocida. TimeCurrent, MqlDateTime |
int Hour() | int HourMQL4() { MqlDateTime tm; TimeCurrent(tm); return(tm.hour); } | Hour Devuelve la hora (0,1,2,..23) de la última fecha del servidor conocida en el momento del inicio del programa (este valor no cambiará durante el tiempo de la ejecución del programa). TimeCurrent, MqlDateTime |
int Minute() | int MinuteMQL4() { MqlDateTime tm; TimeCurrent(tm); return(tm.min); } | Minute Devuelve el minuto actual (0,1,2,..59) de la última hora del servidor conocida en el momento del inicio del programa (este valor no cambiará durante el tiempo de la ejecución del programa). TimeCurrent, MqlDateTime |
int Month() | int MonthMQL4() { MqlDateTime tm; TimeCurrent(tm); return(tm.mon); } | Month Devuelve el mes actual como número (1=enero,2,3,4,5,6,7,8,9,10,11,12), es decir, el número del mes de la última fecha del servidor conocida. TimeCurrent, MqlDateTime |
int Seconds() | int SecondsMQL4() { MqlDateTime tm; TimeCurrent(tm); return(tm.sec); } | Seconds Devuelve la cantidad de segundos pasados desde el comienzo del minuto actual de la última hora del servidor conocida en el momento del inicio del programa (este valor no cambiará durante el tiempo de la ejecución del programa). TimeCurrent, MqlDateTime |
datetime TimeCurrent() | datetime TimeCurrent() | TimeCurrent Devuelve la última fecha del servidor (fecha de entrada de la última cuota) como número de segundos pasados desde las 00:00 del 1 de enero de 1970. TimeCurrent |
int TimeDay(datetime date) | int TimeDayMQL4(datetime date) { MqlDateTime tm; TimeToStruct(date,tm); return(tm.day); } | TimeDay Devuelve el día del mes (1 - 31) para la fecha especificada. TimeToStruct, MqlDateTime |
int TimeDayOfWeek(datetime date) | int TimeDayOfWeekMQL4(datetime date) { MqlDateTime tm; TimeToStruct(date,tm); return(tm.day_of_week); } | TimeDayOfWeek Devuelve el día de la semana basado en 0 (0 significa domingo,1,2,3,4,5,6) para la fecha especificada. TimeToStruct, MqlDateTime |
int TimeDayOfYear(datetime date) | int TimeDayOfYearMQL4(datetime date) { MqlDateTime tm; TimeToStruct(date,tm); return(tm.day_of_year); } | TimeDayOfYear Devuelve el día (1 significa 1 de enero,..,365(6) significa 31 de diciembre) del año para la fecha especificada. TimeToStruct, MqlDateTime |
int TimeHour(datetime time) | int TimeHourMQL4(datetime date) { MqlDateTime tm; TimeToStruct(date,tm); return(tm.hour); } | TimeHour Devuelve la hora para la fecha especificada. TimeToStruct, MqlDateTime |
datetime TimeLocal() | datetime TimeLocal() | TimeLocal Devuelve la hora del ordenador local como número de segundos pasados desde las 00:00 del 1 de enero de 1970. TimeLocal |
int TimeMinute(datetime time) | int TimeMinuteMQL4(datetime date) { MqlDateTime tm; TimeToStruct(date,tm); return(tm.min); } | TimeMinute Devuelve el minuto para la hora especificada. TimeToStruct, MqlDateTime |
int TimeMonth(datetime time) | int TimeMonthMQL4(datetime date) { MqlDateTime tm; TimeToStruct(date,tm); return(tm.mon); } | TimeMonth Devuelve el número de mes para la fecha especificada. TimeToStruct, MqlDateTime |
int TimeSeconds(datetime time) | int TimeSecondsMQL4(datetime date) { MqlDateTime tm; TimeToStruct(date,tm); return(tm.sec); } | TimeSeconds Devuelve la cantidad de segundos pasados desde el principio del minuto para la hora especificada. TimeToStruct, MqlDateTime |
int TimeYear(datetime time) | int TimeYearMQL4(datetime date) { MqlDateTime tm; TimeToStruct(date,tm); return(tm.year); } | TimeYear Devuelve el año para la fecha especificada. El valor devuelto estará entre 1970 y 2037. TimeToStruct, MqlDateTime |
int Year() | int YearMQL4() { MqlDateTime tm; TimeCurrent(tm); return(tm.year); } | Year Devuelve el año actual, es decir, el año de la última fecha del servidor conocida. TimeCurrent, MqlDateTime |
12. Funciones de archivo
MQL4 | MQL5 | Descripción |
---|---|---|
void FileClose(int handle) | void FileClose(int file_handle) | FileClose Cierra un archivo abierto anteriormente con la función FileOpen(). FileClose |
void FileDelete(string filename) | bool FileDelete(string file_name int common_flag=0) | FileDelete Elimina el nombre de archivo especificado. FileDelete |
void FileFlush(int handle) | void FileFlush(int file_handle) | FileFlush Pasa todos los datos almacenados en el buffer de archivo al disco. FileFlush |
bool FileIsEnding(int handle) | bool FileIsEnding(int file_handle) | FileIsEnding Devuelve true lógico si el puntero de archivo se encuentra al final del archivo, de lo contrario devolverá false. FileIsEnding |
bool FileIsLineEnding(int handle) | bool FileIsLineEnding(int file_handle) | FileIsLineEnding Devolverá true lógico para un archivo CSV si el puntero de archivo se encuentra al final de la línea, de lo contrario devolverá false. FileIsLineEnding |
int FileOpen(string filename, int mode, int delimiter=';') | int FileOpen(string ile_name, int pen_flags, short delimiter='\t' uint codepage=CP_ACP) | FileOpen Abre archivo para entrada y/o salida. Devuelve un identificador de archivo para el archivo abierto, o -1 (si la función falló). FileOpen |
int FileOpenHistory(string filename, int mode, int delimiter=';') | - | FileOpenHistory Abre un archivo en el historial actual (terminal_directory\history\server_name) o en sus subcarpetas. Devuelve el identificador de archivo para el archivo abierto. Si la función falla, el valor devuelto será -1. |
int FileReadArray(int handle, object &array[], int start, int count) | uint FileReadArray(int file_handle, void array[], int start_item=0, int items_count=WHOLE_ARRAY) | FileReadArray Lee la cantidad de elementos especificada del archivo binario en el array. FileReadArray |
double FileReadDouble(int handle, int size=DOUBLE_VALUE) | double FileReadDoubleMQL4(int handle, int size=DOUBLE_VALUE) { return(FileReadDouble(handle)); } | FileReadDouble Lee el número de precisión doble con punto flotante de la posición de archivo binario actual. FileReadDouble |
int FileReadInteger(int handle, int size=LONG_VALUE) | int FileReadInteger(int file_handle, int size=INT_VALUE) | FileReadInteger La función lee el número íntegro de la posición de archivo binario actual. FileReadInteger |
double FileReadNumber(int handle) | double FileReadNumber(int file_handle) | FileReadNumber Lee el número de la posición de archivo actual antes del delimitador. Solo para archivos CSV. FileReadNumber |
string FileReadString(int handle, int length=0) | string FileReadString(int file_handle, int size=-1) | FileReadString La función devuelve la línea de la posición actual del archivo. FileReadString |
bool FileSeek(int handle, int offset, int origin) | bool FileSeekMQL4(long handle, int offset, ENUM_FILE_POSITION origin) { FileSeek(handle,offset,origin); return(true); } | FileSeek La función mueve el puntero de archivo a una nueva posición que es un offset, en bytes, desde el principio, el final o la posición actual del archivo. FileSeek |
int FileSize(int handle) | ulong FileSize(int file_handle) | FileSize La función devuelve el tamaño del archivo en bytes. FileSize |
int FileTell(int handle) | ulong FileTell(int file_handle) | FileTell Devuelve la posición actual del puntero de archivo. FileTell |
int FileWrite(int handle,...) | uint FileWrite(int file_handle,...) | FileWrite La función está diseñada para escribir datos en un archivo CSV, con el delimitador insertado automáticamente. FileWrite |
int FileWriteArray(int handle, object array[], int start, int count) | int FileWriteArray(int file_handle, void array[], int start_item=0, int items_count=WHOLE_ARRAY) | FileWriteArray La función escribe el array en un archivo binario. FileWriteArray |
int FileWriteDouble(int handle, double value, int size=DOUBLE_VALUE) | uint FileWriteDouble(int file_handle, double dvalue) | FileWriteDouble La función escribe un valor doble con un puntero flotante en un archivo binario. FileWriteDouble |
int FileWriteInteger(int handle, int value, int size=LONG_VALUE) | uint FileWriteInteger(int file_handle, int ivalue, int size=INT_VALUE) | FileWriteInteger La función escribe el valor íntegro a un archivo binario. FileWriteInteger |
int FileWriteString(int handle, string value, int size) | uint FileWriteString(int file_handle, string svalue, int size=-1) | FileWriteString La función escribe la cadena de caracteres a un archivo binario desde la posición actual del archivo. FileWriteString |
13. Variables Globales
MQL4 | MQL5 | Descripción |
---|---|---|
bool GlobalVariableCheck(string name) | bool GlobalVariableCheck(string name) | GlobalVariableCheck Devuelve TRUE si la variable global existe, de lo contrario devolverá FALSE. GlobalVariableCheck |
bool GlobalVariableDel(string name) | bool GlobalVariableDel(string name) | GlobalVariableDel Elimina la variable global. GlobalVariableDel |
double GlobalVariableGet(string name) | double GlobalVariableGet(string name) | GlobalVariableGet Devuelve el valor de una variable global existente, o 0 si se dio un error. GlobalVariableGet |
string GlobalVariableName(int index) | string GlobalVariableName(int index) | GlobalVariableName La función devuelve el nombre de una variable global por su índice en la lista de variables globales. GlobalVariableName |
datetime GlobalVariableSet(string name, double value) | datetime GlobalVariableSet(string name, double value) | GlobalVariableSet Configura un nuevo valor de la variable global. Si no existe, el sistema crea una nueva variable global. GlobalVariableSet |
bool GlobalVariableSetOnCondition(string name, double value, double check_value) | bool GlobalVariableSetOnCondition(string name, double value, double check_value) | GlobalVariableSetOnCondition Configura el nuevo valor de la variable global si el valor actual es igual al tercer parámetro check_value. GlobalVariableSetOnCondition |
int GlobalVariablesDeleteAll(string prefix_name=NULL) | int GlobalVariablesDeleteAll(string prefix_name=NULL datetime limit_data=0) | GlobalVariablesDeleteAll Elimina variables globales. GlobalVariablesDeleteAll |
int GlobalVariablesTotal() | int GlobalVariablesTotal() | GlobalVariablesTotal La función devuelve el número total de variables globales. GlobalVariablesTotal |
14. Funciones Matemáticas
MQL4 | MQL5 | Descripción |
---|---|---|
double MathAbs(double value) | double MathAbs(double value) | MathAbs Devuelve el valor absoluto (modulus) del valor numérico especificado. MathAbs |
double MathArccos(double x) | double MathArccos(double val) | MathArccos La función MathArccos devuelve el arccoseno de x dentro del margen de 0 a Pi (en radianes). MathArccos |
double MathArcsin(double x) | double MathArcsin(double val) | MathArcsin La función MathArcsin devuelve el arcseno de x dentro del margen de -Pi/2 a Pi/2 radianes. MathArcsin |
double MathArctan(double x) | double MathArctan(double value) | MathArctan La función MathArctan devuelve la arctangente de x. MathArctan |
double MathCeil(double x) | double MathCeil(double val) | MathCeil La función MathCeil devuelve un valor numérico que representa el número íntegro más pequeño que supera o es igual a x. MathCeil |
double MathCos(double value) | double MathCos(double value) | MathCos Devuelve el coseno del ángulo especificado. MathCos |
double MathExp(double d) | double MathExp(double value) | MathExp Devuelve el valor de e elevado a la potencia de d. MathExp |
double MathFloor(double x) | double MathFloor(double val) | MathFloor La función MathFloor devuelve un valor numérico que representa el número íntegro más grande que es menor o igual a x. MathFloor |
double MathLog(double x) | double MathLog(double val) | MathLog La función MathLog devuelve el logaritmo natural de x si tiene éxito. MathLog |
double MathMax(double value1, double value2) | double MathMax(double value1, double value2) | MathMax Devuelve el valor máximo de dos valores numéricos. MathMax |
double MathMin(double value1, double value2) | double MathMin(double value1, double value2) | MathMin Devuelve el valor mínimo de dos valores numéricos. MathMin |
double MathMod(double value1, double value2) | double MathMod(double value1, double value2) | MathMod La función devuelve el recordatorio de punto flotante de la división de dos números. MathMod |
double MathPow(double base, double exponent) | double MathPow(double base, double exponent) | MathPow Devuelve el valor de la expresión base elevada a la potencia especificada (valor de exponente). MathPow |
int MathRand() | int MathRand() | MathRand La función MathRand devuelve un número íntegro pseudoaleatorio dentro del margen de 0 a 32767. MathRand |
double MathRound(double value) | double MathRound(double value) | MathRound Devuelve el valor redondeado al número íntegro más cercano del valor numérico especificado. MathRound |
double MathSin(double value) | double MathSin(double value) | MathSin Devuelve el seno del ángulo especificado. MathSin |
double MathSqrt(double x) | double MathSqrt(double value) | MathSqrt La función MathSqrt devuelve la raíz cuadrada de x. MathSqrt |
void MathSrand(int seed) | void MathSrand(int seed) | MathSrand La función MathSrand() configura el punto de inicio para generar una serie de números íntegros pseudoaleatorios. MathSrand |
double MathTan(double x) | double MathTan(double rad) | MathTan MathTan devuelve la tangente de x. MathTan |
15. Funciones de Objeto
MQL4 | MQL5 | Descripción |
---|---|---|
bool ObjectCreate(string name, int type, int window, datetime time1, double price1, datetime time2=0, double price2=0, datetime time3=0, double price3=0) | bool ObjectCreateMQL4(string name, ENUM_OBJECT type, int window, datetime time1, double price1, datetime time2=0, double price2=0, datetime time3=0, double price3=0) { return(ObjectCreate(0,name,type,window, time1,price1,time2,price2,time3,price3)); } | ObjectCreate Creación de un objeto con el nombre específico, tipo y coordenadas iniciales en la ventana especificada. ObjectCreate |
bool ObjectDelete(string name) | bool ObjectDeleteMQL4(string name) { return(ObjectDelete(0,name)); } | ObjectDelete Elimina el objeto con el nombre especificado. ObjectDelete |
string ObjectDescription(string name) | string ObjectDescriptionMQL4(string name) { return(ObjectGetString(0,name,OBJPROP_TEXT)); } | ObjectDescription Devuelve la descripción del objeto. ObjectGetString |
int ObjectFind(string name) | int ObjectFindMQL4(string name) { return(ObjectFind(0,name)); } | ObjectFind Busca un objeto con el nombre especificado. ObjectFind |
double ObjectGet(string name, int prop_id) | double ObjectGetMQL4(string name, int index) { switch(index) { case OBJPROP_TIME1: return(ObjectGetInteger(0,name,OBJPROP_TIME)); case OBJPROP_PRICE1: return(ObjectGetDouble(0,name,OBJPROP_PRICE)); case OBJPROP_TIME2: return(ObjectGetInteger(0,name,OBJPROP_TIME,1)); case OBJPROP_PRICE2: return(ObjectGetDouble(0,name,OBJPROP_PRICE,1)); case OBJPROP_TIME3: return(ObjectGetInteger(0,name,OBJPROP_TIME,2)); case OBJPROP_PRICE3: return(ObjectGetDouble(0,name,OBJPROP_PRICE,2)); case OBJPROP_COLOR: return(ObjectGetInteger(0,name,OBJPROP_COLOR)); case OBJPROP_STYLE: return(ObjectGetInteger(0,name,OBJPROP_STYLE)); case OBJPROP_WIDTH: return(ObjectGetInteger(0,name,OBJPROP_WIDTH)); case OBJPROP_BACK: return(ObjectGetInteger(0,name,OBJPROP_WIDTH)); case OBJPROP_RAY: return(ObjectGetInteger(0,name,OBJPROP_RAY_RIGHT)); case OBJPROP_ELLIPSE: return(ObjectGetInteger(0,name,OBJPROP_ELLIPSE)); case OBJPROP_SCALE: return(ObjectGetDouble(0,name,OBJPROP_SCALE)); case OBJPROP_ANGLE: return(ObjectGetDouble(0,name,OBJPROP_ANGLE)); case OBJPROP_ARROWCODE: return(ObjectGetInteger(0,name,OBJPROP_ARROWCODE)); case OBJPROP_TIMEFRAMES: return(ObjectGetInteger(0,name,OBJPROP_TIMEFRAMES)); case OBJPROP_DEVIATION: return(ObjectGetDouble(0,name,OBJPROP_DEVIATION)); case OBJPROP_FONTSIZE: return(ObjectGetInteger(0,name,OBJPROP_FONTSIZE)); case OBJPROP_CORNER: return(ObjectGetInteger(0,name,OBJPROP_CORNER)); case OBJPROP_XDISTANCE: return(ObjectGetInteger(0,name,OBJPROP_XDISTANCE)); case OBJPROP_YDISTANCE: return(ObjectGetInteger(0,name,OBJPROP_YDISTANCE)); case OBJPROP_FIBOLEVELS: return(ObjectGetInteger(0,name,OBJPROP_LEVELS)); case OBJPROP_LEVELCOLOR: return(ObjectGetInteger(0,name,OBJPROP_LEVELCOLOR)); case OBJPROP_LEVELSTYLE: return(ObjectGetInteger(0,name,OBJPROP_LEVELSTYLE)); case OBJPROP_LEVELWIDTH: return(ObjectGetInteger(0,name,OBJPROP_LEVELWIDTH)); } } | ObjectGet La función devuelve el valor de la propiedad del objeto especificado. ObjectGetInteger, ObjectGetDouble |
string ObjectGetFiboDescription(string name, int index) | string ObjectGetFiboDescriptionMQL4(string name, int index) { return(ObjectGetString(0,name,OBJPROP_LEVELTEXT,index)); } | ObjectGetFiboDescription La función devuelve la descripción de nivel de un objeto Fibonacci. ObjectGetString |
int ObjectGetShiftByValue(string name, double value) | int ObjectGetShiftByValueMQL4(string name, double value) { ENUM_TIMEFRAMES timeframe=TFMigrate(PERIOD_CURRENT); datetime Arr[]; int shift; MqlRates mql4[]; if(ObjectGetTimeByValue(0,name,value)<0) return(-1); CopyRates(NULL,timeframe,0,1,mql4); if(CopyTime(NULL,timeframe,mql4[0].time, ObjectGetTimeByValue(0,name,value),Arr)>0) return(ArraySize(Arr)-1); else return(-1); } | ObjectGetShiftByValue La función calcula y devuelve el índice de barra (variación relativa a la barra actual) para el precio especificado. MqlRates, ObjectGetTimeByValue, CopyRates, CopyTime, ArraySize |
double ObjectGetValueByShift(string name, int shift) | double ObjectGetValueByShiftMQL4(string name, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(PERIOD_CURRENT); MqlRates mql4[]; CopyRates(NULL,timeframe,shift,1,mql4); return(ObjectGetValueByTime(0,name,mql4[0].time,0)); } | ObjectGetValueByShift La función calcula y devuelve el valor de precio para la barra especificada (variación relativa a la barra actual). MqlRates, CopyRates, ObjectGetValueByTime |
bool ObjectMove(string name, int point, datetime time1, double price1) | bool ObjectMoveMQL4(string name, int point, datetime time1, double price1) { return(ObjectMove(0,name,point,time1,price1)); } | ObjectMove La función mueve una coordinada de objeto en el gráfico. Los objetos pueden tener entre una y tres coordinadas, dependiendo de sus tipos. ObjectMove |
string ObjectName(int index) | string ObjectNameMQL4(int index) { return(ObjectName(0,index)); } | ObjectName La función devuelve el nombre de objeto por su índice en la lista de objetos. ObjectName |
int ObjectsDeleteAll(int window=EMPTY, int type=EMPTY) | int ObjectsDeleteAllMQL4(int window=EMPTY, int type=EMPTY) { return(ObjectsDeleteAll(0,window,type)); } | ObjectsDeleteAll Elimina todos los objetos del tipos especificados en la subventana del gráfico especificada. ObjectsDeleteAll |
bool ObjectSet(string name, int prop_id, double value) | bool ObjectSetMQL4(string name, int index, double value) { switch(index) { case OBJPROP_TIME1: ObjectSetInteger(0,name,OBJPROP_TIME,(int)value);return(true); case OBJPROP_PRICE1: ObjectSetDouble(0,name,OBJPROP_PRICE,value);return(true); case OBJPROP_TIME2: ObjectSetInteger(0,name,OBJPROP_TIME,1,(int)value);return(true); case OBJPROP_PRICE2: ObjectSetDouble(0,name,OBJPROP_PRICE,1,value);return(true); case OBJPROP_TIME3: ObjectSetInteger(0,name,OBJPROP_TIME,2,(int)value);return(true); case OBJPROP_PRICE3: ObjectSetDouble(0,name,OBJPROP_PRICE,2,value);return(true); case OBJPROP_COLOR: ObjectSetInteger(0,name,OBJPROP_COLOR,(int)value);return(true); case OBJPROP_STYLE: ObjectSetInteger(0,name,OBJPROP_STYLE,(int)value);return(true); case OBJPROP_WIDTH: ObjectSetInteger(0,name,OBJPROP_WIDTH,(int)value);return(true); case OBJPROP_BACK: ObjectSetInteger(0,name,OBJPROP_BACK,(int)value);return(true); case OBJPROP_RAY: ObjectSetInteger(0,name,OBJPROP_RAY_RIGHT,(int)value);return(true); case OBJPROP_ELLIPSE: ObjectSetInteger(0,name,OBJPROP_ELLIPSE,(int)value);return(true); case OBJPROP_SCALE: ObjectSetDouble(0,name,OBJPROP_SCALE,value);return(true); case OBJPROP_ANGLE: ObjectSetDouble(0,name,OBJPROP_ANGLE,value);return(true); case OBJPROP_ARROWCODE: ObjectSetInteger(0,name,OBJPROP_ARROWCODE,(int)value);return(true); case OBJPROP_TIMEFRAMES: ObjectSetInteger(0,name,OBJPROP_TIMEFRAMES,(int)value);return(true); case OBJPROP_DEVIATION: ObjectSetDouble(0,name,OBJPROP_DEVIATION,value);return(true); case OBJPROP_FONTSIZE: ObjectSetInteger(0,name,OBJPROP_FONTSIZE,(int)value);return(true); case OBJPROP_CORNER: ObjectSetInteger(0,name,OBJPROP_CORNER,(int)value);return(true); case OBJPROP_XDISTANCE: ObjectSetInteger(0,name,OBJPROP_XDISTANCE,(int)value);return(true); case OBJPROP_YDISTANCE: ObjectSetInteger(0,name,OBJPROP_YDISTANCE,(int)value);return(true); case OBJPROP_FIBOLEVELS: ObjectSetInteger(0,name,OBJPROP_LEVELS,(int)value);return(true); case OBJPROP_LEVELCOLOR: ObjectSetInteger(0,name,OBJPROP_LEVELCOLOR,(int)value);return(true); case OBJPROP_LEVELSTYLE: ObjectSetInteger(0,name,OBJPROP_LEVELSTYLE,(int)value);return(true); case OBJPROP_LEVELWIDTH: ObjectSetInteger(0,name,OBJPROP_LEVELWIDTH,(int)value);return(true); default: return(false); } return(false); } | ObjectSet Cambia el valor de la propiedad de objeto especificada. ObjectSetInteger, ObjectSetDouble |
bool ObjectSetFiboDescription(string name, int index, string text) | bool ObjectSetFiboDescriptionMQL4(string name, int index, string text) { return(ObjectSetString(0,name,OBJPROP_LEVELTEXT,index,text)); } | ObjectSetFiboDescription La función asigna una nueva descripción a un nivel de un objeto Fibonacci. ObjectSetString |
bool ObjectSetText(string name, string text, int font_size, string font_name=NULL, color text_color=CLR_NONE) | bool ObjectSetTextMQL4(string name, string text, int font_size, string font="", color text_color=CLR_NONE) { int tmpObjType=(int)ObjectGetInteger(0,name,OBJPROP_TYPE); if(tmpObjType!=OBJ_LABEL && tmpObjType!=OBJ_TEXT) return(false); if(StringLen(text)>0 && font_size>0) { if(ObjectSetString(0,name,OBJPROP_TEXT,text)==true && ObjectSetInteger(0,name,OBJPROP_FONTSIZE,font_size)==true) { if((StringLen(font)>0) && ObjectSetString(0,name,OBJPROP_FONT,font)==false) return(false); if(text_color>-1 && ObjectSetInteger(0,name,OBJPROP_COLOR,text_color)==false) return(false); return(true); } return(false); } return(false); } | ObjectSetText Cambia la descripción del objeto. ObjectGetInteger, ObjectSetString, ObjectSetInteger StringLen |
int ObjectsTotal(int type=EMPTY) | int ObjectsTotalMQL4(int type=EMPTY, int window=-1) { return(ObjectsTotal(0,window,type)); } | ObjectsTotal Devuelve el número total de objetos del tipo especificado en el gráfico. ObjectsTotal |
int ObjectType(string name) | int ObjectTypeMQL4(string name) { return((int)ObjectGetInteger(0,name,OBJPROP_TYPE)); } | ObjectType La función devuelve el valor del tipo de objeto. ObjectGetInteger |
16. Funciones de Cadena de Caracteres
MQL4 | MQL5 | Descripción |
---|---|---|
string StringConcatenate(...) | int StringConcatenate(string &string_var, void argument1 void argument2 ...) | StringConcatenate Forma una cadena de caracteres de los datos transferidos y la devuelve. StringConcatenate |
int StringFind(string text, string matched_text, int start=0) | int StringFind(string string_value, string match_substring, int start_pos=0) | StringFind Busca una subcadena de caracteres. Devuelve la posición en la cadena de caracteres desde la que comienza la subcadena de caracteres buscada, o -1 si la subcadena de caracteres no se pudo encontrar. StringFind |
int StringGetChar(string text, int pos) | ushort StringGetCharacter(string string_value, int pos) | StringGetChar Devuelve el carácter (código) de la posición especificada en la cadena de caracteres. StringGetCharacter |
int StringLen(string text) | int StringLen(string string_value) | StringLen Devuelve el número de caracteres en una cadena de caracteres. StringLen |
string StringSetChar(string text, int pos, int value) | bool StringSetCharacter(string &string_var, int pos, ushort character) | StringSetChar Devuelve la copia de la cadena de caracteres con un carácter cambiado en la posición especificada. StringSetCharacter |
string StringSubstr(string text, int start, int length=0) | string StringSubstr(string string_value, int start_pos, int length=-1) | StringSubstr Extrae una subcadena de caracteres de la cadena de caracteres de texto empezando en la posición especificada. StringSubstr |
string StringTrimLeft(string text) | int StringTrimLeft(string& string_var) | StringTrimLeft La función corta caracteres de la línea de feed, espacios y sangrados en la parte izquierda de la cadena de caracteres. StringTrimLeft |
string StringTrimRight(string text) | int StringTrimRight(string& string_var) | StringTrimRight La función corta caracteres de la línea de feed, espacios y sangrados en la parte derecha de la cadena de caracteres. StringTrimRight |
17. Indicadores Técnicos
Los principios del uso de los indicadores técnicos en Asesores Expertos se tratan en el artículo MQL5 for Newbies: Guide to Using Technical Indicators in Expert Advisors (MQL5 para Principiantes: Guía de Uso de Indicadores Técnicos en Asesores Expertos). El método usado en este artículo es suficiente para obtener los resultados del cálculo del indicador para el precio especificado. Para usar este método, necesitamos la función auxiliar:
double CopyBufferMQL4(int handle,int index,int shift) { double buf[]; switch(index) { case 0: if(CopyBuffer(handle,0,shift,1,buf)>0) return(buf[0]); break; case 1: if(CopyBuffer(handle,1,shift,1,buf)>0) return(buf[0]); break; case 2: if(CopyBuffer(handle,2,shift,1,buf)>0) return(buf[0]); break; case 3: if(CopyBuffer(handle,3,shift,1,buf)>0) return(buf[0]); break; case 4: if(CopyBuffer(handle,4,shift,1,buf)>0) return(buf[0]); break; default: break; } return(EMPTY_VALUE); }Declaremos las siguientes constantes:
ENUM_MA_METHOD MethodMigrate(int method) { switch(method) { case 0: return(MODE_SMA); case 1: return(MODE_EMA); case 2: return(MODE_SMMA); case 3: return(MODE_LWMA); default: return(MODE_SMA); } } ENUM_APPLIED_PRICE PriceMigrate(int price) { switch(price) { case 1: return(PRICE_CLOSE); case 2: return(PRICE_OPEN); case 3: return(PRICE_HIGH); case 4: return(PRICE_LOW); case 5: return(PRICE_MEDIAN); case 6: return(PRICE_TYPICAL); case 7: return(PRICE_WEIGHTED); default: return(PRICE_CLOSE); } } ENUM_STO_PRICE StoFieldMigrate(int field) { switch(field) { case 0: return(STO_LOWHIGH); case 1: return(STO_CLOSECLOSE); default: return(STO_LOWHIGH); } } //+------------------------------------------------------------------+ enum ALLIGATOR_MODE { MODE_GATORJAW=1, MODE_GATORTEETH, MODE_GATORLIPS }; enum ADX_MODE { MODE_MAIN, MODE_PLUSDI, MODE_MINUSDI }; enum UP_LOW_MODE { MODE_BASE, MODE_UPPER, MODE_LOWER }; enum ICHIMOKU_MODE { MODE_TENKANSEN=1, MODE_KIJUNSEN, MODE_SENKOUSPANA, MODE_SENKOUSPANB, MODE_CHINKOUSPAN }; enum MAIN_SIGNAL_MODE{ MODE_MAIN, MODE_SIGNAL };
MQL4 | MQL5 | Descripción |
---|---|---|
double iAC(string symbol, int timeframe, int shift) | double iACMQL4(string symbol, int tf, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); int handle=iAC(symbol,timeframe); if(handle<0) { Print("The iAC object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iAC Calcula el oscilador Accelerator/Decelerator (Acelerador/Decelerador) de Bill Williams. iAC |
double iAD(string symbol, int timeframe, int shift) | double iADMQL4(string symbol, int tf, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); int handle=(int)iAD(symbol,timeframe,VOLUME_TICK); if(handle<0) { Print("The iAD object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iAD Calcula el indicador Accumulation/Distribution (Acumulación/Distribución) y devuelve su valor. iAD |
double iAlligator(string symbol, int timeframe, int jaw_period, int jaw_shift, int teeth_period, int teeth_shift, int lips_period, int lips_shift, int ma_method, int applied_price, int mode, int shift) | double iAlligatorMQL4(string symbol, int tf, int jaw_period, int jaw_shift, int teeth_period, int teeth_shift, int lips_period, int lips_shift, int method, int price, int mode, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); ENUM_MA_METHOD ma_method=MethodMigrate(method); ENUM_APPLIED_PRICE applied_price=PriceMigrate(price); int handle=iAlligator(symbol,timeframe,jaw_period,jaw_shift, teeth_period,teeth_shift, lips_period,lips_shift, ma_method,applied_price); if(handle<0) { Print("The iAlligator object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,mode-1,shift)); } | iAlligator Calcula el Alligator de Bill Williams y devuelve su valor. iAlligator |
double iADX(string symbol, int timeframe, int period, int applied_price, int mode, int shift) | double iADXMQL4(string symbol, int tf, int period, int price, int mode, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); int handle=iADX(symbol,timeframe,period); if(handle<0) { Print("The iADX object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,mode,shift)); } | iADX Calcula el índice directional Movement (Movimiento direccional) y devuelve su valor. iADX |
double iATR(string symbol, int timeframe, int period, int shift) | double iATRMQL4(string symbol, int tf, int period, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); int handle=iATR(symbol,timeframe,period); if(handle<0) { Print("The iATR object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iATR Calcula el indicador del margen medio verdadero y devuelve su valor. iATR |
double iAO(string symbol, int timeframe, int shift) | double iAOMQL4(string symbol, int tf, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); int handle=iAO(symbol,timeframe); if(handle<0) { Print("The iAO object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iAO Calcula el Awesome Oscillator (Gran Oscilador) de Bill Williams y devuelve su valor. iAO |
double iBearsPower(string symbol, int timeframe, int period, int applied_price, int shift) | double iBearsPowerMQL4(string symbol, int tf, int period, int price, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); int handle=iBearsPower(symbol,timeframe,period); if(handle<0) { Print("The iBearsPower object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iBearsPower Calcula el indicador Bears Power y devuelve su valor. iBearsPower |
double iBands(string symbol, int timeframe, int period, int deviation, int bands_shift, int applied_price, int mode, int shift) | double iBandsMQL4(string symbol, int tf, int period, double deviation, int bands_shift, int method, int mode, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); ENUM_MA_METHOD ma_method=MethodMigrate(method); int handle=iBands(symbol,timeframe,period, bands_shift,deviation,ma_method); if(handle<0) { Print("The iBands object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,mode,shift)); } | iBands Calcula el indicador Bollinger Bands y devuelve su valor. iBands |
double iBandsOnArray(double array[], int total, int period, int deviation, int bands_shift, int mode, int shift) | - | iBandsOnArray El cálculo del indicador Bollinger Bands se lleva a cabo con datos almacenados en un array numérico. |
double iBullsPower(string symbol, int timeframe, int period, int applied_price, int shift) | double iBullsPowerMQL4(string symbol, int tf, int period, int price, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); int handle=iBullsPower(symbol,timeframe,period); if(handle<0) { Print("The iBullsPower object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iBullsPower Calcula el indicador Bulls Power y devuelve su valor. iBullsPower |
double iCCI(string symbol, int timeframe, int period, int applied_price, int shift) | double iCCIMQL4(string symbol, int tf, int period, int price, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); ENUM_APPLIED_PRICE applied_price=PriceMigrate(price); int handle=iCCI(symbol,timeframe,period,price); if(handle<0) { Print("The iCCI object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iCCI Calcula el índice de Commodity Channel y devuelve su valor. iCCI |
double iCCIOnArray(double array[], int total, int period, int shift) | - | iCCIOnArray El cálculo del índice Commodity Channel se lleva a cabo con datos almacenados en un array numérico. |
double iCustom(string symbol, int timeframe, string name, ..., int mode, int shift) | int iCustom(string symbol, ENUM_TIMEFRAMES period, string name ...) | iCustom Calcula el indicador personalizado especificado y devuelve su valor. iCustom |
double iDeMarker(string symbol, int timeframe, int period, int shift) | double iDeMarkerMQL4(string symbol, int tf, int period, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); int handle=iDeMarker(symbol,timeframe,period); if(handle<0) { Print("The iDeMarker object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iDeMarker Calcula el indicador DeMarker y devuelve su valor. iDeMarker |
double iEnvelopes(string symbol, int timeframe, int ma_period, int ma_method, int ma_shift, int applied_price, double deviation, int mode, int shift) | double EnvelopesMQL4(string symbol, int tf, int ma_period, int method, int ma_shift, int price, double deviation, int mode, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); ENUM_MA_METHOD ma_method=MethodMigrate(method); ENUM_APPLIED_PRICE applied_price=PriceMigrate(price); int handle=iEnvelopes(symbol,timeframe, ma_period,ma_shift,ma_method, applied_price,deviation); if(handle<0) { Print("The iEnvelopes object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,mode-1,shift)); } | iEnvelopes Calcula el indicador Envelopes y devuelve su valor. iEnvelopes |
double iEnvelopesOnArray(double array[], int total, int ma_period, int ma_method, int ma_shift, double deviation, int mode, int shift) | - | iEnvelopesOnArray El cálculo del indicador Envelopes se lleva a cabo con datos almacenados en un array numérico. |
double iForce(string symbol, int timeframe, int period, int ma_method, int applied_price, int shift) | double iForceMQL4(string symbol, int tf, int period, int method, int price, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); ENUM_MA_METHOD ma_method=MethodMigrate(method); int handle=iForce(symbol,timeframe,period,ma_method,VOLUME_TICK); if(handle<0) { Print("The iForce object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iForce Calcula el índice Force y devuelve su valor. iForce |
double iFractals(string symbol, int timeframe, int mode, int shift) | double iFractalsMQL4(string symbol, int tf, int mode, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); int handle=iFractals(symbol,timeframe); if(handle<0) { Print("The iFractals object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,mode-1,shift)); } | iFractals Calcula el Fractals (Fractales) y devuelve su valor. iFractals |
double iGator(string symbol, int timeframe, int jaw_period, int jaw_shift, int teeth_period, int teeth_shift, int lips_period, int lips_shift, int ma_method, int applied_price, int mode, int shift) | double iGatorMQL4(string symbol, int tf, int jaw_period, int jaw_shift, int teeth_period, int teeth_shift, int lips_period, int lips_shift, int method, int price, int mode, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); ENUM_MA_METHOD ma_method=MethodMigrate(method); ENUM_APPLIED_PRICE applied_price=PriceMigrate(price); int handle=iGator(symbol,timeframe,jaw_period,jaw_shift, teeth_period,teeth_shift, lips_period,lips_shift, ma_method,applied_price); if(handle<0) { Print("The iGator object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,mode-1,shift)); } | iGator Calcula el oscilador Gator. iGator |
double iIchimoku(string symbol, int timeframe, int tenkan_sen, int kijun_sen, int senkou_span_b, int mode, int shift) | double iIchimokuMQL4(string symbol, int tf, int tenkan_sen, int kijun_sen, int senkou_span_b, int mode, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); int handle=iIchimoku(symbol,timeframe, tenkan_sen,kijun_sen,senkou_span_b); if(handle<0) { Print("The iIchimoku object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,mode-1,shift)); } | iIchimoku Calcula el Ichimoku Kinko Hyo y devuelve su valor. iIchimoku |
double iBWMFI(string symbol, int timeframe, int shift) | double iBWMFIMQL4(string symbol, int tf, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); int handle=(int)iBWMFI(symbol,timeframe,VOLUME_TICK); if(handle<0) { Print("The iBWMFI object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iBWMFI Calcula el índice Market Facilitation de Bill Williams y devuelve su valor. iBWMFI |
double iMomentum(string symbol, int timeframe, int period, int applied_price, int shift) | double iMomentumMQL4(string symbol, int tf, int period, int price, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); ENUM_APPLIED_PRICE applied_price=PriceMigrate(price); int handle=iMomentum(symbol,timeframe,period,applied_price); if(handle<0) { Print("The iMomentum object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iMomentum Calcula el indicador Momentum y devuelve su valor. iMomentum |
double iMomentumOnArray(double array[], int total, int period, int shift) | - | iMomentumOnArray El cálculo del indicador Momentum se lleva a cabo con datos almacenados en un array numérico. |
double iMFI(string symbol, int timeframe, int period, int shift) | double iMFIMQL4(string symbol, int tf, int period, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); int handle=(int)iMFI(symbol,timeframe,period,VOLUME_TICK); if(handle<0) { Print("The iMFI object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iMFI Calcula el índice Money flow y devuelve su valor. iMFI |
double iMA(string symbol, int timeframe, int period, int ma_shift, int ma_method, int applied_price, int shift) | double iMAMQL4(string symbol, int tf, int period, int ma_shift, int method, int price, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); ENUM_MA_METHOD ma_method=MethodMigrate(method); ENUM_APPLIED_PRICE applied_price=PriceMigrate(price); int handle=iMA(symbol,timeframe,period,ma_shift, ma_method,applied_price); if(handle<0) { Print("The iMA object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iMA Calcula el indicador Moving Average (Media Móvil) y devuelve su valor. iMA |
double iMAOnArray(double array[], int total, int period, int ma_shift, int ma_method, int shift) | double iMAOnArrayMQL4(double &array[], int total, int period, int ma_shift, int ma_method, int shift) { double buf[],arr[]; if(total==0) total=ArraySize(array); if(total>0 && total<=period) return(0); if(shift>total-period-ma_shift) return(0); switch(ma_method) { case MODE_SMA : { total=ArrayCopy(arr,array,0,shift+ma_shift,period); if(ArrayResize(buf,total)<0) return(0); double sum=0; int i,pos=total-1; for(i=1;i<period;i++,pos--) sum+=arr[pos]; while(pos>=0) { sum+=arr[pos]; buf[pos]=sum/period; sum-=arr[pos+period-1]; pos--; } return(buf[0]); } case MODE_EMA : { if(ArrayResize(buf,total)<0) return(0); double pr=2.0/(period+1); int pos=total-2; while(pos>=0) { if(pos==total-2) buf[pos+1]=array[pos+1]; buf[pos]=array[pos]*pr+buf[pos+1]*(1-pr); pos--; } return(buf[shift+ma_shift]); } case MODE_SMMA : { if(ArrayResize(buf,total)<0) return(0); double sum=0; int i,k,pos; pos=total-period; while(pos>=0) { if(pos==total-period) { for(i=0,k=pos;i<period;i++,k++) { sum+=array[k]; buf[k]=0; } } else sum=buf[pos+1]*(period-1)+array[pos]; buf[pos]=sum/period; pos--; } return(buf[shift+ma_shift]); } case MODE_LWMA : { if(ArrayResize(buf,total)<0) return(0); double sum=0.0,lsum=0.0; double price; int i,weight=0,pos=total-1; for(i=1;i<=period;i++,pos--) { price=array[pos]; sum+=price*i; lsum+=price; weight+=i; } pos++; i=pos+period; while(pos>=0) { buf[pos]=sum/weight; if(pos==0) break; pos--; i--; price=array[pos]; sum=sum-lsum+price*period; lsum-=array[i]; lsum+=price; } return(buf[shift+ma_shift]); } default: return(0); } return(0); } | |
double iOsMA(string symbol, int timeframe, int fast_ema_period, int slow_ema_period, int signal_period, int applied_price, int shift) | double iOsMAMQL4(string symbol, int tf, int fast_ema_period, int slow_ema_period, int signal_period, int price, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); ENUM_APPLIED_PRICE applied_price=PriceMigrate(price); int handle=iOsMA(symbol,timeframe, fast_ema_period,slow_ema_period, signal_period,applied_price); if(handle<0) { Print("The iOsMA object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iOsMA Calcula la Media Móvil del Oscilador y devuelve su valor. iOsMA |
double iMACD(string symbol, int timeframe, int fast_ema_period, int slow_ema_period, int signal_period, int applied_price, int mode, int shift) | double iMACDMQL4(string symbol, int tf, int fast_ema_period, int slow_ema_period, int signal_period, int price, int mode, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); ENUM_APPLIED_PRICE applied_price=PriceMigrate(price); int handle=iMACD(symbol,timeframe, fast_ema_period,slow_ema_period, signal_period,applied_price); if(handle<0) { Print("The iMACD object is not created: Error ",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,mode,shift)); } | iMACD Calcula la convergencia/divergencia de Medias Móviles y devuelve su valor. iMACD |
double iOBV(string symbol, int timeframe, int applied_price, int shift) | double iOBVMQL4(string symbol, int tf, int price, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); int handle=iOBV(symbol,timeframe,VOLUME_TICK); if(handle<0) { Print("The iOBV object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iOBV Calcula el indicador On Balance Volume (Volumen en Saldo) y devuelve su valor. iOBV |
double iSAR(string symbol, int timeframe, double step, double maximum, int shift) | double iSARMQL4(string symbol, int tf, double step, double maximum, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); int handle=iSAR(symbol,timeframe,step,maximum); if(handle<0) { Print("The iSAR object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iSAR Calcula el sistema Parabolic Stop and Reverse (Detención Parabólica e Inversa) y devuelve su valor. iSAR |
double iRSI(string symbol, int timeframe, int period, int applied_price, int shift) | double iRSIMQL4(string symbol, int tf, int period, int price, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); ENUM_APPLIED_PRICE applied_price=PriceMigrate(price); int handle=iRSI(symbol,timeframe,period,applied_price); if(handle<0) { Print("The iRSI object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iRSI Calcula el índice Relative Strength (Fuerza Relativa) y devuelve su valor. iRSI |
double iRSIOnArray(double array[], int total, int period, int shift) | - | iRSIOnArray El cálculo del índice Relative Strength se lleva a cabo con datos almacenados en un array numérico. |
double iRVI(string symbol, int timeframe, int period, int mode, int shift) | double iRVIMQL4(string symbol, int tf, int period, int mode, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); int handle=iRVI(symbol,timeframe,period); if(handle<0) { Print("The iRVI object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,mode,shift)); } | iRVI Calcula el índice Relative Vigor (Vigor Relativo) y devuelve su valor. iRVI |
double iStdDev(string symbol, int timeframe, int ma_period, int ma_shift, int ma_method, int applied_price, int shift) | double iStdDevMQL4(string symbol, int tf, int ma_period, int ma_shift, int method, int price, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); ENUM_MA_METHOD ma_method=MethodMigrate(method); ENUM_APPLIED_PRICE applied_price=PriceMigrate(price); int handle=iStdDev(symbol,timeframe,ma_period,ma_shift, ma_method,applied_price); if(handle<0) { Print("The iStdDev object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iStdDev Calcula el indicador Standard Deviation (Desviación Estándar) y devuelve su valor. iStdDev |
double iStdDevOnArray(double array[], int total, int ma_period, int ma_shift, int ma_method, int shift) | - | iStdDevOnArray El cálculo del indicador Standard Deviation se lleva a cabo con datos almacenados en un array numérico. |
double iStochastic(string symbol, int timeframe, int%Kperiod, int%Dperiod, int slowing, int method, int price_field, int mode, int shift) | double iStochasticMQL4(string symbol, int tf, int Kperiod, int Dperiod, int slowing, int method, int field, int mode, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); ENUM_MA_METHOD ma_method=MethodMigrate(method); ENUM_STO_PRICE price_field=StoFieldMigrate(field); int handle=iStochastic(symbol,timeframe,Kperiod,Dperiod, slowing,ma_method,price_field); if(handle<0) { Print("The iStochastic object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,mode,shift)); } | iStochastic Calcula el Stochastic Oscillator (Oscilador Estocástico) y devuelve su valor. iStochastic |
double iWPR(string symbol, int timeframe, int period, int shift) | double iWPRMQL4(string symbol, int tf, int period, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); int handle=iWPR(symbol,timeframe,period); if(handle<0) { Print("The iWPR object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iWPR Calcula el indicador Percent Range (Margen de Porcentaje) de Larry William y devuelve su valor. iWPR |
18. Acceso a Series de Tiempo
MQL4 | MQL5 | Descripción |
---|---|---|
int iBars(string symbol, int timeframe) | int iBarsMQL4(string symbol,int tf) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); return(Bars(symbol,timeframe)); } | int iBarsMQL4(string symbol,int tf) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); return(Bars(symbol,timeframe)); }Bars Devuelve el número de barras en el gráfico especificado. Bars |
int iBarShift(string symbol, int timeframe, datetime time, bool exact=false | int iBarShiftMQL4(string symbol, int tf, datetime time, bool exact=false) { if(time<0) return(-1); ENUM_TIMEFRAMES timeframe=TFMigrate(tf); datetime Arr[],time1; CopyTime(symbol,timeframe,0,1,Arr); time1=Arr[0]; if(CopyTime(symbol,timeframe,time,time1,Arr)>0) { if(ArraySize(Arr)>2) return(ArraySize(Arr)-1); if(time<time1) return(1); else return(0); } else return(-1); } | iBarShift Busca una barra por su momento de apertura. CopyTime, ArraySize |
double iClose(string symbol, int timeframe, int shift) | double iCloseMQL4(string symbol,int tf,int index){ if(index < 0) return(-1); double Arr[]; ENUM_TIMEFRAMES timeframe=TFMigrate(tf); if(CopyClose(symbol,timeframe, index, 1, Arr)>0) return(Arr[0]); else return(-1); } | Close Devuelve el valor de Close (Cierre) para la barra del símbolo indicado con intervalo de tiempo y variación. Si el historial local está vacío (no se ha cargado), la función devuelve 0. CopyRates, MqlRates |
double iHigh(string symbol, int timeframe, int shift) | double iHighMQL4(string symbol,int tf,int index) { if(index < 0) return(-1); double Arr[]; ENUM_TIMEFRAMES timeframe=TFMigrate(tf); if(CopyHigh(symbol,timeframe, index, 1, Arr)>0) return(Arr[0]); else return(-1); } | High Devuelve el valor de High (Alto) para la barra del símbolo indicado con intervalo de tiempo y variación. Si el historial local está vacío (no se ha cargado), la función devuelve 0. CopyRates, MqlRates |
int iHighest(string symbol, int timeframe, int type, int count=WHOLE_ARRAY, int start=0) | int iHighestMQL4(string symbol, int tf, int type, int count=WHOLE_ARRAY, int start=0) { if(start<0) return(-1); ENUM_TIMEFRAMES timeframe=TFMigrate(tf); if(count<=0) count=Bars(symbol,timeframe); if(type<=MODE_OPEN) { double Open[]; ArraySetAsSeries(Open,true); CopyOpen(symbol,timeframe,start,count,Open); return(ArrayMaximum(Open,0,count)+start); } if(type==MODE_LOW) { double Low[]; ArraySetAsSeries(Low,true); CopyLow(symbol,timeframe,start,count,Low); return(ArrayMaximum(Low,0,count)+start); } if(type==MODE_HIGH) { double High[]; ArraySetAsSeries(High,true); CopyHigh(symbol,timeframe,start,count,High); return(ArrayMaximum(High,0,count)+start); } if(type==MODE_CLOSE) { double Close[]; ArraySetAsSeries(Close,true); CopyClose(symbol,timeframe,start,count,Close); return(ArrayMaximum(Close,0,count)+start); } if(type==MODE_VOLUME) { long Volume[]; ArraySetAsSeries(Volume,true); CopyTickVolume(symbol,timeframe,start,count,Volume); return(ArrayMaximum(Volume,0,count)+start); } if(type>=MODE_TIME) { datetime Time[]; ArraySetAsSeries(Time,true); CopyTime(symbol,timeframe,start,count,Time); return(ArrayMaximum(Time,0,count)+start); //--- } return(0); } | iHighest Devuelve la variación del valor máximo valor sobre un número específico de períodos, dependiendo del tipo. CopyOpen, CopyLow, CopyHigh, CopyClose, CopyTickVolume, CopyTime, ArrayMaximum |
double iLow(string symbol, int timeframe, int shift) | double iLowMQL4(string symbol,int tf,int index) { if(index < 0) return(-1); double Arr[]; ENUM_TIMEFRAMES timeframe=TFMigrate(tf); if(CopyLow(symbol,timeframe, index, 1, Arr)>0) return(Arr[0]); else return(-1); } | iLow Devuelve el valor Low (Bajo) para la barra del símbolo indicado con intervalo de tiempo y variación. Si el historial local está vacío (no se ha cargado), la función devuelve 0. CopyRates, MqlRates |
int iLowest(string symbol, int timeframe, int type, int count=WHOLE_ARRAY, int start=0) | int iLowestMQL4(string symbol, int tf, int type, int count=WHOLE_ARRAY, int start=0) { if(start<0) return(-1); ENUM_TIMEFRAMES timeframe=TFMigrate(tf); if(count<=0) count=Bars(symbol,timeframe); if(type<=MODE_OPEN) { double Open[]; ArraySetAsSeries(Open,true); CopyOpen(symbol,timeframe,start,count,Open); return(ArrayMinimum(Open,0,count)+start); } if(type==MODE_LOW) { double Low[]; ArraySetAsSeries(Low,true); CopyLow(symbol,timeframe,start,count,Low); return(ArrayMinimum(Low,0,count)+start); } if(type==MODE_HIGH) { double High[]; ArraySetAsSeries(High,true); CopyHigh(symbol,timeframe,start,count,High); return(ArrayMinimum(High,0,count)+start); } if(type==MODE_CLOSE) { double Close[]; ArraySetAsSeries(Close,true); CopyClose(symbol,timeframe,start,count,Close); return(ArrayMinimum(Close,0,count)+start); } if(type==MODE_VOLUME) { long Volume[]; ArraySetAsSeries(Volume,true); CopyTickVolume(symbol,timeframe,start,count,Volume); return(ArrayMinimum(Volume,0,count)+start); } if(type>=MODE_TIME) { datetime Time[]; ArraySetAsSeries(Time,true); CopyTime(symbol,timeframe,start,count,Time); return(ArrayMinimum(Time,0,count)+start); } //--- return(0); } | iLowest Devuelve la variación del valor más bajo sobre un número de períodos específico, dependiendo del tipo. CopyOpen, CopyLow, CopyHigh, CopyClose, CopyTickVolume, CopyTime, ArrayMinimum |
double iOpen(string symbol, int timeframe, int shift) | double iOpenMQL4(string symbol,int tf,int index) { if(index < 0) return(-1); double Arr[]; ENUM_TIMEFRAMES timeframe=TFMigrate(tf); if(CopyOpen(symbol,timeframe, index, 1, Arr)>0) return(Arr[0]); else return(-1); } | iOpen Devuelve el valor Open (Apertura) para la barra del símbolo indicado con intervalo de tiempo y variación. Si el historial local está vacío (no se ha cargado), la función devuelve 0. CopyRates, MqlRates |
datetime iTime(string symbol, int timeframe, int shift) | datetime iTimeMQL4(string symbol,int tf,int index) { if(index < 0) return(-1); ENUM_TIMEFRAMES timeframe=TFMigrate(tf); datetime Arr[]; if(CopyTime(symbol, timeframe, index, 1, Arr)>0) return(Arr[0]); else return(-1); } | iTime Devuelve el valor Time (Tiempo) para la barra del símbolo indicado con intervalo de tiempo y variación. Si el historial local está vacío (no se ha cargado), la función devuelve 0. CopyRates, MqlRates |
double iVolume(string symbol, int timeframe, int shift) | int iVolumeMQL4(string symbol,int tf,int index) { if(index < 0) return(-1); long Arr[]; ENUM_TIMEFRAMES timeframe=TFMigrate(tf); if(CopyTickVolume(symbol, timeframe, index, 1, Arr)>0) return(Arr[0]); else return(-1); } | iVolume Devuelve el valor de Tick Volume (Volumen de Ticks) para la barra del símbolo indicado con intervalo de tiempo y variación. Si el historial local está vacío (no se ha cargado), la función devuelve 0. CopyRates, MqlRates |
19. Operaciones de Gráfico
MQL4 | MQL5 | Descripción |
---|---|---|
void HideTestIndicators(bool hide) | - | HideTestIndicators La función configura una flag que oculta indicadores llamados por el Asesor Experto. |
int Period() | ENUM_TIMEFRAMES Period() | Period Devuelve la cantidad de minutos que determinan el períodos usado (intervalo de gráfico). Period |
bool RefreshRates() | - | RefreshRates Actualiza los datos en variables predefinidas y arrays de series. |
string Symbol() | string Symbol() | Symbol Devuelve una cadena de caracteres de texto con el nombre del instrumento financiero actual. Symbol |
int WindowBarsPerChart() | int ChartGetInteger(0,CHART_VISIBLE_BARS,0) | WindowBarsPerChart La función devuelve la cantidad de barras visibles en el gráfico. ChartGetInteger |
string WindowExpertName() | string MQLInfoString(MQL_PROGRAM_NAME) | WindowExpertName Devuelve el nombre del experto ejecutado, script, indicador personalizado o biblioteca, dependiendo del programa MQL4 del que se haya llamado esta función. MQLInfoString |
int WindowFind(string name) | int WindowFindMQL4(string name) { int window=-1; if((ENUM_PROGRAM_TYPE)MQLInfoInteger(MQL5_PROGRAM_TYPE)==PROGRAM_INDICATOR) { window=ChartWindowFind(); } else { window=ChartWindowFind(0,name); if(window==-1) Print(__FUNCTION__+"(): Error = ",GetLastError()); } return(window); } | WindowFind Si se encontró un indicador con este nombre, la función devuelve la ventana índice que contiene este indicador especificado. De lo contrario, devolverá -1. ChartWindowFind, MQLInfoInteger |
int WindowFirstVisibleBar() | int ChartGetInteger(0,CHART_FIRST_VISIBLE_BAR,0) | WindowFirstVisibleBar La función devuelve la primera barra visible en la ventana de gráfico actual. ChartGetInteger |
int WindowHandle(string symbol, int timeframe) | int WindowHandleMQL4(string symbol, int tf) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); long currChart,prevChart=ChartFirst(); int i=0,limit=100; while(i<limit) { currChart=ChartNext(prevChart); if(currChart<0) break; if(ChartSymbol(currChart)==symbol && ChartPeriod(currChart)==timeframe) return((int)currChart); prevChart=currChart; i++; } return(0); } | WindowHandle Devuelve el identificador de la ventana de sistema del gráfico especificado. ChartFirst, ChartNext, ChartSymbol, ChartPeriod |
bool WindowIsVisible(int index) | bool ChartGetInteger(0,CHART_WINDOW_IS_VISIBLE,index) | WindowIsVisible Devuelve TRUE si la subventana del gráfico está visible, de lo contrario devolverá FALSE. ChartGetInteger |
int WindowOnDropped() | int ChartWindowOnDropped() | WindowOnDropped Devuelve la ventana índice en la que se colocó el Experto, indicador personalizado o script. ChartWindowOnDropped |
double WindowPriceMax(int index=0) | double ChartGetDouble(0,CHART_PRICE_MAX,index) | WindowPriceMax Devuelve el valor máximo de la escala vertical de la subventana especificada del gráfico actual (0-ventana de gráfico principal; las subventanas del indicador se numeran a partir de 1). ChartGetDouble |
double WindowPriceMin(int index=0) | double ChartGetDouble(0,CHART_PRICE_MIN,index) | WindowPriceMin Devuelve el valor mínimo de la escala vertical de la subventana especificada del gráfico actual (0-ventana de gráfico principal; las subventanas del indicador se numeran a partir de 1). ChartGetDouble |
double WindowPriceOnDropped() | double ChartPriceOnDropped() | WindowPriceOnDropped Devuelve la parte del precio del gráfico en la que se colocó el Experto o script. ChartPriceOnDropped |
void WindowRedraw() | void ChartRedraw(0) | WindowRedraw Redibuja el gráfico actual de manera forzada. ChartRedraw |
bool WindowScreenShot(string filename, int size_x, int size_y, int start_bar=-1, int chart_scale=-1, int chart_mode=-1) | bool WindowScreenShotMQL4(string filename, int size_x, int size_y, int start_bar=-1, int chart_scale=-1, int chart_mode=-1) { if(chart_scale>0 && chart_scale<=5) ChartSetInteger(0,CHART_SCALE,chart_scale); switch(chart_mode) { case 0: ChartSetInteger(0,CHART_MODE,CHART_BARS); case 1: ChartSetInteger(0,CHART_MODE,CHART_CANDLES); case 2: ChartSetInteger(0,CHART_MODE,CHART_LINE); } if(start_bar<0) return(ChartScreenShot(0,filename,size_x,size_y,ALIGN_RIGHT)); else return(ChartScreenShot(0,filename,size_x,size_y,ALIGN_LEFT)); } | WindowScreenShot Guarda la captura de pantalla del gráfico como archivo GIF. ChartSetInteger, ChartScreenShot |
datetime WindowTimeOnDropped() | datetime ChartTimeOnDropped() | WindowTimeOnDropped Devuelve la parte del tiempo del gráfico en la que se colocó el Experto o script. ChartTimeOnDropped |
int WindowsTotal() | int ChartGetInteger(0,CHART_WINDOWS_TOTAL) | WindowsTotal Devuelve el número de ventanas de indicador en el gráfico (incluyendo el gráfico principal). ChartGetInteger |
int WindowXOnDropped() | int ChartXOnDropped() | WindowXOnDropped Devuelve el valor en el eje X en píxeles para el punto del área de la venta de cliente en el que se colocó el Experto o script. ChartXOnDropped |
int WindowYOnDropped() | int ChartYOnDropped() | WindowYOnDropped Devuelve el valor en el eje Y en píxeles para el punto del área de la venta de cliente en el que se colocó el Experto o script. ChartYOnDropped |
Conclusión
- No hemos tratado las funciones de trading porque el concepto en MQL5 es diferente, ¡y se debería usar el original! Es posible convertirlas, pero la lógica de trading cambiaría. En otras palabras, no tiene sentido convertirlas.
- La conversión de programas de un lenguaje a otro siempre está asociado con la pérdida de funcionalidad y eficiencia. Por tanto, use esta guía para una búsqueda rápida de las funciones análogas.
- En el futuro pienso desarrollar un emulador de MQL4 que le permitirá ejecutar su programa MQL4 en el terminal de Cliente MetaTrader 5.
Créditos: keiji, A. Williams.
Traducción del ruso hecha por MetaQuotes Ltd.
Artículo original: https://www.mql5.com/ru/articles/81





- Aplicaciones de trading gratuitas
- 8 000+ señales para copiar
- Noticias económicas para analizar los mercados financieros
Usted acepta la política del sitio web y las condiciones de uso