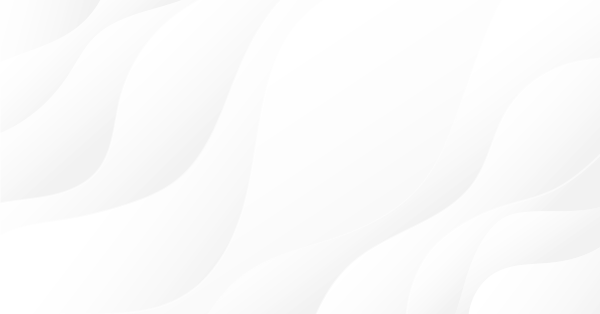
Migrazione da MQL4 a MQL5
Introduzione
Molti sviluppatori hanno accumulato molti indicatori e strategie di trading scritte in MQL4. Per usarli in Metatrader 5, dovrebbero essere convertiti in MQL5. Non è così facile riscrivere tutti i programmi in MQL5. Sarebbe molto più facile convertirli, se ci fosse un riferimento di traduzione e meglio con esempi.
In questo articolo, vorrei suggerire la mia versione di una guida per migrare da MQL4 a MQL5.
1. Periodi del Grafico
In MQL5, le costanti del periodo del grafico sono cambiate e sono stati aggiunti alcuni nuovi periodi di tempo (M2, M3, M4, M6, M10, M12, H2, H3, H6, H8, H12). Per convertire i periodi di tempo MQL4, è possibile utilizzare la seguente funzione:
ENUM_TIMEFRAMES TFMigrate(int tf) { switch(tf) { case 0: return(PERIOD_CURRENT); case 1: return(PERIOD_M1); case 5: return(PERIOD_M5); case 15: return(PERIOD_M15); case 30: return(PERIOD_M30); case 60: return(PERIOD_H1); case 240: return(PERIOD_H4); case 1440: return(PERIOD_D1); case 10080: return(PERIOD_W1); case 43200: return(PERIOD_MN1); case 2: return(PERIOD_M2); case 3: return(PERIOD_M3); case 4: return(PERIOD_M4); case 6: return(PERIOD_M6); case 10: return(PERIOD_M10); case 12: return(PERIOD_M12); case 16385: return(PERIOD_H1); case 16386: return(PERIOD_H2); case 16387: return(PERIOD_H3); case 16388: return(PERIOD_H4); case 16390: return(PERIOD_H6); case 16392: return(PERIOD_H8); case 16396: return(PERIOD_H12); case 16408: return(PERIOD_D1); case 32769: return(PERIOD_W1); case 49153: return(PERIOD_MN1); default: return(PERIOD_CURRENT); } }
Da notare che in MQL5 i valori numerici delle costanti temporali del grafico (da H1) non sono uguali al numero di minuti di una barra (ad esempio, in MQL5, il valore numerico della costante PERIOD_H1=16385, ma in MQL4 PERIOD_H1 =60). Dovresti tenerne conto durante la conversione in MQL5, se i valori numerici delle costanti MQL4 vengono utilizzati nei programmi MQL4.
Per determinare il numero di minuti del periodo di tempo specificato del grafico, dividere il valore restituito dalla funzione PeriodSeconds per 60.
2. Dichiarazione delle Costanti
Alcune delle costanti MQL4 standard sono assenti in MQL5, quindi dovrebbero essere dichiarate:
//+------------------------------------------------------------------+ //| InitMQL4.mqh | //| Copyright DC2008 | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "keiji" #property copyright "DC2008" #property link "https://www.mql5.com" //--- Declaration of constants #define OP_BUY 0 //Buy #define OP_SELL 1 //Sell #define OP_BUYLIMIT 2 //Pending order of BUY LIMIT type #define OP_SELLLIMIT 3 //Pending order of SELL LIMIT type #define OP_BUYSTOP 4 //Pending order of BUY STOP type #define OP_SELLSTOP 5 //Pending order of SELL STOP type //--- #define MODE_OPEN 0 #define MODE_CLOSE 3 #define MODE_VOLUME 4 #define MODE_REAL_VOLUME 5 #define MODE_TRADES 0 #define MODE_HISTORY 1 #define SELECT_BY_POS 0 #define SELECT_BY_TICKET 1 //--- #define DOUBLE_VALUE 0 #define FLOAT_VALUE 1 #define LONG_VALUE INT_VALUE //--- #define CHART_BAR 0 #define CHART_CANDLE 1 //--- #define MODE_ASCEND 0 #define MODE_DESCEND 1 //--- #define MODE_LOW 1 #define MODE_HIGH 2 #define MODE_TIME 5 #define MODE_BID 9 #define MODE_ASK 10 #define MODE_POINT 11 #define MODE_DIGITS 12 #define MODE_SPREAD 13 #define MODE_STOPLEVEL 14 #define MODE_LOTSIZE 15 #define MODE_TICKVALUE 16 #define MODE_TICKSIZE 17 #define MODE_SWAPLONG 18 #define MODE_SWAPSHORT 19 #define MODE_STARTING 20 #define MODE_EXPIRATION 21 #define MODE_TRADEALLOWED 22 #define MODE_MINLOT 23 #define MODE_LOTSTEP 24 #define MODE_MAXLOT 25 #define MODE_SWAPTYPE 26 #define MODE_PROFITCALCMODE 27 #define MODE_MARGINCALCMODE 28 #define MODE_MARGININIT 29 #define MODE_MARGINMAINTENANCE 30 #define MODE_MARGINHEDGED 31 #define MODE_MARGINREQUIRED 32 #define MODE_FREEZELEVEL 33 //--- #define EMPTY -1Nota: Le costanti in MQL4 e MQL5 differiscono, quindi è meglio dichiararle in un file separato initMQ4.mqh per un ulteriore utilizzo.
3. Variabili Predefinite
MQL4 | MQL5 | Descrizione |
---|---|---|
double Ask | MqlTick last_tick; SymbolInfoTick(_Symbol,last_tick); double Ask=last_tick.ask; | Ask L'ultimo prezzo richiesto noto per il simbolo corrente. SymbolInfoTick |
int Bars | int Bars=Bars(_Symbol,_Period); | Bars Numero di barre nel grafico corrente. Bars |
double Bid | MqlTick last_tick; SymbolInfoTick(_Symbol,last_tick); double Bid=last_tick.bid; | Bid L'ultimo prezzo bid noto del simbolo corrente. SymbolInfoTick |
double Close[] | double Close[]; int count; // number of elements to copy ArraySetAsSeries(Close,true); CopyClose(_Symbol,_Period,0,count,Close); | Close Array di serie che contiene i prezzi di chiusura per ogni barra del grafico corrente. CopyClose, ArraySetAsSeries |
int Digits | int Digits=_Digits; | Digits Numero di cifre dopo la virgola per i prezzi dei simboli correnti. _Digits |
double High[] | double High[]; int count; // number of elements to copy ArraySetAsSeries(High,true); CopyHigh(_Symbol,_Period,0,count,High); | High Array di serie che contiene i prezzi più alti di ogni barra del grafico corrente. CopyHigh, ArraySetAsSeries |
double Low[] | double Low[]; int count; // number of elements to copy ArraySetAsSeries(Low,true); CopyLow(_Symbol,_Period,0,count,Low); | Low Array di serie che contiene i prezzi più bassi di ogni barra del grafico corrente. CopyLow, ArraySetAsSeries |
double Open[] | double Open[]; int count; // number of elements to copy ArraySetAsSeries(Open,true); CopyOpen(_Symbol,_Period,0,count,Open); | Open Array di serie che contiene i prezzi di apertura di ciascuna barra del grafico corrente. CopyOpen, ArraySetAsSeries |
double Point | double Point=_Point; | Point Il valore del punto simbolo corrente nella valuta di quotazione. _Point |
datetime Time[] | datetime Time[]; int count; // number of elements to copy ArraySetAsSeries(Time,true); CopyTime(_Symbol,_Period,0,count,Time); | Time Array di serie che contiene il tempo di apertura di ciascuna barra del grafico corrente. Dati come datetime rappresentano il tempo, in secondi, trascorso dalle 00:00 del 1 gennaio 1970. CopyTime, ArraySetAsSeries |
double Volume[] | long Volume[]; int count; // number of elements to copy ArraySetAsSeries(Volume,true); CopyTickVolume(_Symbol,_Period,0,count,Volume); | Volume Array di serie che contiene i volumi di tick di ciascuna barra del grafico corrente. CopyTickVolume, ArraySetAsSeries |
4. Informazioni dell’Account
MQL4 | MQL5 | Descrizione |
---|---|---|
double AccountBalance() | double AccountInfoDouble(ACCOUNT_BALANCE) | AccountBalance Restituisce il valore del saldo del conto corrente (la somma di denaro sul conto). AccountInfoDouble |
double AccountCredit() | double AccountInfoDouble(ACCOUNT_CREDIT) | AccountCredit Restituisce il valore del credito del conto corrente. AccountInfoDouble |
string AccountCompany() | string AccountInfoString(ACCOUNT_COMPANY) | AccountCompany Restituisce il nome della società di intermediazione in cui è stato registrato il conto corrente. AccountInfoString |
string AccountCurrency() | string AccountInfoString(ACCOUNT_CURRENCY) | AccountCurrency Restituisce il nome della valuta del conto corrente. AccountInfoString |
double AccountEquity() | double AccountInfoDouble(ACCOUNT_EQUITY) | AccountEquity Restituisce il valore del capitale del conto corrente. Il calcolo del capitale dipende dalle impostazioni del server di trading. AccountInfoDouble |
double AccountFreeMargin() | double AccountInfoDouble(ACCOUNT_FREEMARGIN) | AccountFreeMargin Restituisce il valore del margine libero del conto corrente. AccountInfoDouble |
double AccountFreeMarginCheck(string symbol, int cmd, double volume) | - | AccountFreeMarginCheck Restituisce il margine libero che rimane dopo l'apertura della posizione specificata al prezzo corrente sul conto corrente. |
double AccountFreeMarginMode() | - | AccountFreeMarginMode Modalità di calcolo del margine libero consentito per l'apertura di posizioni sul conto corrente. |
int AccountLeverage() | int AccountInfoInteger(ACCOUNT_LEVERAGE) | AccountLeverage Restituisce la leva del conto corrente. AccountInfoInteger |
double AccountMargin() | double AccountInfoDouble(ACCOUNT_MARGIN) | AccountMargin Restituisce il valore del margine del conto corrente. AccountInfoDouble |
string AccountName() | string AccountInfoString(ACCOUNT_NAME) | AccountName Restituisce il nome del conto corrente. AccountInfoString |
int AccountNumber() | int AccountInfoInteger(ACCOUNT_LOGIN) | AccountNumber Restituisce il numero del conto corrente. AccountInfoInteger |
double AccountProfit() | double AccountInfoDouble(ACCOUNT_PROFIT) | AccountProfit Restituisce il valore del profitto del conto corrente. AccountInfoDouble |
string AccountServer() | string AccountInfoString(ACCOUNT_SERVER) | AccountServer Restituisce il nome del server connesso. AccountInfoString |
int AccountStopoutLevel() | double AccountInfoDouble(ACCOUNT_MARGIN_SO_SO) | AccountStopoutLevel Restituisce il valore del livello Stop Out. AccountInfoDouble |
int AccountStopoutMode() | int AccountInfoInteger(ACCOUNT_MARGIN_SO_MODE) | AccountStopoutMode Restituisce la modalità di calcolo per il livello Stop Out. AccountInfoInteger |
5. Funzioni di Array
MQL4 | MQL5 | Descrizione |
---|---|---|
int ArrayBsearch(double array[], double value, int count=WHOLE_ARRAY, int start=0, int direction=MODE_ASCEND) | int ArrayBsearch(double array[], double searched_value ) | ArrayBsearch La funzione fa la ricerca per un valore specificato in un array numerico uni-dimensionale. ArrayBsearch |
int ArrayCopy(object&dest[], object source[], int start_dest=0, int start_source=0, int count=WHOLE_ARRAY) | int ArrayCopy(void dst_array[], void src_array[], int dst_start=0, int src_start=0, int cnt=WHOLE_ARRAY ) | ArrayCopy Copia un array in un altro. Gli array devono essere dello stesso tipo, ma gli array di tipo double[], int[], datetime[], color[] e bool[] possono essere copiati come array dello stesso tipo. Restituisce la quantità di elementi copiati. ArrayCopy |
int ArrayCopyRates(double&dest_array[], string symbol=NULL, int timeframe=0) | - | ArrayCopyRates Copia i dati delle barre del grafico correnti nell’array bidimensionale di tipo RateInfo[][6] e restituisce l'importo delle barre copiate o -1 in caso di errore. |
int ArrayCopySeries(double&array[], int series_index, string symbol=NULL, int timeframe=0) | int ArrayCopySeriesMQL4(double &array[], int series_index, string symbol=NULL, int tf=0) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); int count=Bars(symbol,timeframe); switch(series_index) { case MODE_OPEN: return(CopyOpen(symbol,timeframe,0,count,array)); case MODE_LOW: return(CopyLow(symbol,timeframe,0,count,array)); case MODE_HIGH: return(CopyHigh(symbol,timeframe,0,count,array)); case MODE_CLOSE: return(CopyClose(symbol,timeframe,0,count,array)); default: return(0); } return(0); } | ArrayCopySeries Copia un array di serie temporali in un array personalizzato e restituisce il conteggio degli elementi copiati. CopyOpen, CopyLow, CopyHigh, CopyClose, Bars |
int ArrayDimension( object array[]) | - | ArrayDimension Restituisce il livello dell'array multidimensionale. |
bool ArrayGetAsSeries( object array[]) | bool ArrayGetAsSeries(void array) | ArrayGetAsSeries Restituisce TRUE se un array è organizzato come array di serie temporali (gli elementi dell'array sono indicizzati dall'ultimo al primo), altrimenti restituisce FALSE. ArrayGetAsSeries |
int ArrayInitialize(double &array[], double value) | int ArrayInitializeMQL4(double &array[], double value) { ArrayInitialize(array,value); return(ArraySize(array)); } | ArrayInitialize Imposta tutti gli elementi di un array numerico sullo stesso valore. Restituisce il conteggio degli elementi inizializzati. ArrayInitialize, ArraySize |
bool ArrayIsSeries( object array[]) | bool ArrayIsSeries(void array[]) | ArrayIsSeries Restituisce TRUE se l'array sotto controllo è un array di serie temporali (Time[],Open[],Close[],High[],Low[] o Volume[]), altrimenti restituisce FALSE. ArrayIsSeries |
int ArrayMaximum(double array[], int count=WHOLE_ARRAY, int start=0) | int ArrayMaximumMQL4(double &array[], int count=WHOLE_ARRAY, int start=0) { return(ArrayMaximum(array,start,count)); } | ArrayMaximum Cerca l'elemento con il valore massimo. La funzione restituisce la posizione di questo elemento massimo nell'array. ArrayMaximum |
int ArrayMinimum(double array[], int count=WHOLE_ARRAY, int start=0) | int ArrayMinimumMQL4(double &array[], int count=WHOLE_ARRAY, int start=0) { return(ArrayMinimum(array,start,count)); } | ArrayMinimum Cerca l'elemento con il valore minimo. La funzione restituisce la posizione di questo elemento minimo nell'array. ArrayMinimum |
int ArrayRange(object array[], int range_index) | int ArrayRange(void array[], int rank_index ) | ArrayRange Restituisce il conteggio degli elementi nella dimensione data dell'array. ArrayRange |
int ArrayResize(object &array[], int new_size) | int ArrayResize(void array[], int new_size, int allocated_size=0 ) | ArrayResize Imposta una nuova grandezza per la prima dimensione. ArrayResize |
bool ArraySetAsSeries(double &array[], bool set) | bool ArraySetAsSeries(void array[], bool set ) | ArraySetAsSeries Restituisce il conteggio degli elementi nella dimensione data dell'array. Poiché gli indici sono in base zero, la grandezza della dimensione è maggiore di 1 rispetto all'indice più grande. ArraySetAsSeries |
int ArraySize( object array[]) | int ArraySize(void array[]) | ArraySize Restituisce il conteggio degli elementi contenuti nell'array. ArraySize |
int ArraySort(double &array[], int count=WHOLE_ARRAY, int start=0, int sort_dir=MODE_ASCEND) | int ArraySortMQL4(double &array[], int count=WHOLE_ARRAY, int start=0, int sort_dir=MODE_ASCEND) { switch(sort_dir) { case MODE_ASCEND: ArraySetAsSeries(array,true); case MODE_DESCEND: ArraySetAsSeries(array,false); default: ArraySetAsSeries(array,true); } ArraySort(array); return(0); } | ArraySort Ordina gli array numerici in base alla prima dimensione. Gli array di serie non possono essere ordinati da ArraySort(). ArraySort, ArraySetAsSeries |
6. Checkup
MQL4 | MQL5 | Descrizione |
---|---|---|
int GetLastError() | int GetLastError() | GetLastError La funzione restituisce l'ultimo errore verificatosi, quindi il valore della variabile speciale last_error in cui è memorizzato l'ultimo codice di errore verrà azzerato. GetLastError |
bool IsConnected() | bool TerminalInfoInteger(TERMINAL_CONNECTED) | La funzione restituisce lo stato della connessione principale tra il client terminale e il server che esegue il trasferimento dei dati. Restituisce TRUE se la connessione al server è stata stabilita con successo, altrimenti restituisce FALSE. TerminalInfoInteger |
bool IsDemo() | bool IsDemoMQL4() { if(AccountInfoInteger(ACCOUNT_TRADE_MODE)==ACCOUNT_TRADE_MODE_DEMO) return(true); else return(false); } | IsDemo Restituisce TRUE se l'expert viene eseguito su un account demo, altrimenti restituisce FALSE. AccountInfoInteger |
bool IsDllsAllowed() | bool TerminalInfoInteger(TERMINAL_DLLS_ALLOWED) | IsDllsAllowed Restituisce TRUE se la chiamata della DLL della funzione è consentita per l’Expert Advisor, altrimenti restituisce FALSE. TerminalInfoInteger |
bool IsExpertEnabled() | bool AccountInfoInteger(ACCOUNT_TRADE_EXPERT) | IsExpertEnabled Restituisce TRUE se l'uso dell’Expert Advisor è abilitato nel client terminale, altrimenti restituisce FALSE. AccountInfoInteger |
bool IsLibrariesAllowed() | bool MQLInfoInteger(MQL5_DLLS_ALLOWED) | IsLibrariesAllowed Restituisce TRUE se un Expert Advisor può chiamare la funzione libreria, altrimenti restituisce FALSE. MQLInfoInteger |
bool IsOptimization() | bool MQLInfoInteger(MQL5_OPTIMIZATION) | IsOptimization Restituisce TRUE se un Expert Advisor è in esecuzione nella modalità di ottimizzazione dello strategy tester, altrimenti restituisce FALSE. MQLInfoInteger |
bool IsStopped() | bool IsStopped() | IsStopped Restituisce TRUE se al programma (un Expert Advisor o uno script) è stato ordinato di interrompere il suo funzionamento, altrimenti restituisce FALSE. IsStopped |
bool IsTesting() | bool MQLInfoInteger(MQL5_TESTING) | IsTesting Restituisce TRUE se un Expert Advisor è in esecuzione in modalità di test, altrimenti restituisce FALSE. MQLInfoInteger |
bool IsTradeAllowed() | bool MQLInfoInteger(MQL5_TRADE_ALLOWED) | IsTradeAllowed Restituisce TRUE se il trading da parte dell’Expert Advisor è consentito e un thread per il trading non è occupato, altrimenti restituisce FALSE. MQLInfoInteger |
bool IsTradeContextBusy() | - | IsTradeContextBusy Restituisce TRUE se un thread per il trading è occupato da un altro Expert Advisor, altrimenti restituisce FALSE. |
bool IsVisualMode() | bool MQLInfoInteger(MQL5_VISUAL_MODE) | IsVisualMode Restituisce TRUE se l'Expert Advisor viene testato con il pulsante "Visual Mode" selezionato, altrimenti restituisce FALSE. MQLInfoInteger |
int UninitializeReason() | int UninitializeReason() | UninitializeReason Restituisce il codice del motivo di non inizializzazione per l’Expert Advisor, indicatori personalizzati e script. UninitializeReason |
7. Client Terminal
MQL4 | MQL5 | Descrizione |
---|---|---|
string TerminalCompany() | string TerminalInfoString(TERMINAL_COMPANY) | TerminalCompany Restituisce il nome della società proprietaria del client terminal. TerminalInfoString |
string TerminalName() | string TerminalInfoString(TERMINAL_NAME) | TerminalName Restituisce il nome del client terminale. TerminalInfoString |
string TerminalPath() | string TerminalInfoString(TERMINAL_PATH) | TerminalPath Restituisce la directory da cui è stato avviato il client terminal. TerminalInfoString |
8. Funzioni Comuni
MQL4 | MQL5 | Descrizione |
---|---|---|
void Alert(...) | void Alert(argument,...) | Alert Visualizza una finestra di dialogo contenente i dati definiti dall'utente. I parametri possono essere di qualsiasi tipo. Alert |
void Comment(...) | void Comment(argument,...) | Comment La funzione emette il commento definito dall'utente nell'angolo in alto a sinistra del grafico. Commento |
int GetTickCount() | uint GetTickCount() | GetTickCount La funzione GetTickCount() restituisce il numero di millisecondi trascorsi dall'inizio l'avvio del sistema. GetTickCount |
double MarketInfo(string symbol, int type) | double MarketInfoMQL4(string symbol, int type) { switch(type) { case MODE_LOW: return(SymbolInfoDouble(symbol,SYMBOL_LASTLOW)); case MODE_HIGH: return(SymbolInfoDouble(symbol,SYMBOL_LASTHIGH)); case MODE_TIME: return(SymbolInfoInteger(symbol,SYMBOL_TIME)); case MODE_BID: return(Bid); case MODE_ASK: return(Ask); case MODE_POINT: return(SymbolInfoDouble(symbol,SYMBOL_POINT)); case MODE_DIGITS: return(SymbolInfoInteger(symbol,SYMBOL_DIGITS)); case MODE_SPREAD: return(SymbolInfoInteger(symbol,SYMBOL_SPREAD)); case MODE_STOPLEVEL: return(SymbolInfoInteger(symbol,SYMBOL_TRADE_STOPS_LEVEL)); case MODE_LOTSIZE: return(SymbolInfoDouble(symbol,SYMBOL_TRADE_CONTRACT_SIZE)); case MODE_TICKVALUE: return(SymbolInfoDouble(symbol,SYMBOL_TRADE_TICK_VALUE)); case MODE_TICKSIZE: return(SymbolInfoDouble(symbol,SYMBOL_TRADE_TICK_SIZE)); case MODE_SWAPLONG: return(SymbolInfoDouble(symbol,SYMBOL_SWAP_LONG)); case MODE_SWAPSHORT: return(SymbolInfoDouble(symbol,SYMBOL_SWAP_SHORT)); case MODE_STARTING: return(0); case MODE_EXPIRATION: return(0); case MODE_TRADEALLOWED: return(0); case MODE_MINLOT: return(SymbolInfoDouble(symbol,SYMBOL_VOLUME_MIN)); case MODE_LOTSTEP: return(SymbolInfoDouble(symbol,SYMBOL_VOLUME_STEP)); case MODE_MAXLOT: return(SymbolInfoDouble(symbol,SYMBOL_VOLUME_MAX)); case MODE_SWAPTYPE: return(SymbolInfoInteger(symbol,SYMBOL_SWAP_MODE)); case MODE_PROFITCALCMODE: return(SymbolInfoInteger(symbol,SYMBOL_TRADE_CALC_MODE)); case MODE_MARGINCALCMODE: return(0); case MODE_MARGININIT: return(0); case MODE_MARGINMAINTENANCE: return(0); case MODE_MARGINHEDGED: return(0); case MODE_MARGINREQUIRED: return(0); case MODE_FREEZELEVEL: return(SymbolInfoInteger(symbol,SYMBOL_TRADE_FREEZE_LEVEL)); default: return(0); } return(0); } | MarketInfo Restituisce vari dati sui titoli elencati nella finestra Market Watch. SymbolInfoInteger, SymbolInfoDouble, Bid, Ask |
int MessageBox(string text=NULL, string caption=NULL, int flags=EMPTY) | int MessageBox(string text, string caption=NULL, int flags=0) | MessageBox La funzione MessageBox crea, visualizza e gestisce la finestra di messaggio. MessageBox |
void PlaySound(string filename) | bool PlaySound(string filename) | PlaySound La funzione riproduce un file audio. PlaySound |
void Print(...) | void Print(argument,...) | Print Stampa un messaggio nel registro degli esperti. |
bool SendFTP(string filename, string ftp_path=NULL) | bool SendFTP(string filename, string ftp_path=NULL) | SendFTP Invia il file al server FTP impostato nel tab Tools->Options->Publisher. Se il tentativo fallisce, restituisce FALSE. SendFTP |
void SendMail(string subject, string some_text) | bool SendMail(string subject, string some_text) | SendMail Invia un messaggio all'e-mail impostata nel tab Tools->Options->EMail SendMail |
void Sleep(int milliseconds) | void Sleep(int milliseconds) | Sleep La funzione Sleep() sospende l'esecuzione dell'expert corrente entro l'intervallo specificato. Sleep |
9. Funzioni di Conversione
MQL4 | MQL5 | Descrizione |
---|---|---|
string CharToStr(int char_code) | string CharToString(int char_code) | CharToStr Conversione di un codice di simboli in una stringa di un carattere CharToString |
string DoubleToStr(double value, int digits) | string DoubleToString(double value, int digits=8) | DoubleToStr Restituisce una stringa di testo con il valore numerico specificato convertito in un formato di precisione specificato. DoubleToString |
double NormalizeDouble(double value, int digits) | double NormalizeDouble(double value, int digits) | NormalizeDouble Arrotonda il valore in virgola mobile alla precisione data. Restituisce il valore normalizzato di tipo double. NormalizeDouble |
double StrToDouble(string value) | double StringToDouble(string value) | StrToDouble Converte la rappresentazione di stringa del numero in un tipo doppio (formato a precisione doppia con virgola mobile). StringToDouble |
int StrToInteger(string value) | long StringToInteger(string value) | StrToInteger Converte la stringa contenente la rappresentazione del carattere del valore in un valore di tipo int (intero). StringToInteger |
datetime StrToTime(string value) | datetime StringToTime(string value) | StrToTime Converte la stringa nel formato "aaaa.mm.gg hh:mi" nel tipo datetime (la quantità di secondi trascorsi dal 1° gennaio 1970). StringToTime |
string TimeToStr(datetime value, int mode=TIME_DATE|TIME_MINUTES) | string TimeToString(datetime value, int mode=TIME_DATE|TIME_MINUTES) | TimeToStr Converte il valore contenente il tempo in secondi trascorsi dal 1 gennaio 1970 in una stringa di formato "aaaa.mm.gg hh:mi". TimeToString |
10. Indicatori Personalizzati
MQL4 | MQL5 | Descrizione |
---|---|---|
void IndicatorBuffers(int count) | - | IndicatorBuffers Alloca memoria per i buffer utilizzati per i calcoli degli indicatori personalizzati. |
int IndicatorCounted() | int IndicatorCountedMQL4() { if(prev_calculated>0) return(prev_calculated-1); if(prev_calculated==0) return(0); return(0); } | IndicatorCounted La funzione restituisce la quantità di barre non modificate dopo l'ultimo avvio dell'indicatore. OnCalculate |
void IndicatorDigits(int digits) | bool IndicatorSetInteger(INDICATOR_DIGITS,digits) | IndicatorDigits Imposta il formato di precisione (il conteggio delle cifre dopo il punto decimale) per visualizzare i valori dell'indicatore. IndicatorSetInteger |
void IndicatorShortName(string name) | bool IndicatorSetString(INDICATOR_SHORTNAME,name) | IndicatorShortName Imposta il nome "breve" di un indicatore personalizzato da mostrare nella Finestra dei Dati e nella sottofinestra del grafico. IndicatorSetString |
void SetIndexArrow(int index, int code) | bool PlotIndexSetInteger(index,PLOT_ARROW,code) | SetIndexArrow Imposta un simbolo di freccia per la linea degli indicatori di tipo DRAW_ARROW. PlotIndexSetInteger |
bool SetIndexBuffer(int index, double array[]) | bool SetIndexBuffer(index,array,INDICATOR_DATA) | SetIndexBuffer Associa la variabile array dichiarata a livello globale al buffer predefinito dell'indicatore personalizzato. SetIndexBuffer |
void SetIndexDrawBegin(int index, int begin) | bool PlotIndexSetInteger(index,PLOT_DRAW_BEGIN,begin) | SetIndexDrawBegin Imposta il numero della barra (dall'inizio dei dati) da cui deve iniziare il disegno della data linea dell'indicatore. PlotIndexSetInteger |
void SetIndexEmptyValue(int index, double value) | bool PlotIndexSetDouble(index,PLOT_EMPTY_VALUE,value) | SetIndexEmptyValue Imposta il valore vuoto della linea di disegno. PlotIndexSetDouble |
void SetIndexLabel(int index, string text) | bool PlotIndexSetString(index,PLOT_LABEL,text) | SetIndexLabel Imposta la descrizione della linea di disegno da mostrare nella Finestra dei Dati e nel tooltip. PlotIndexSetString |
void SetIndexShift(int index, int shift) | bool PlotIndexSetInteger(index,PLOT_SHIFT,shift) | SetIndexShift Imposta l'offset per la linea di disegno. PlotIndexSetInteger |
void SetIndexStyle(int index, int type, int style=EMPTY, int width=EMPTY, color clr=CLR_NONE) | void SetIndexStyleMQL4(int index, int type, int style=EMPTY, int width=EMPTY, color clr=CLR_NONE) { if(width>-1) PlotIndexSetInteger(index,PLOT_LINE_WIDTH,width); if(clr!=CLR_NONE) PlotIndexSetInteger(index,PLOT_LINE_COLOR,clr); switch(type) { case 0: PlotIndexSetInteger(index,PLOT_DRAW_TYPE,DRAW_LINE); case 1: PlotIndexSetInteger(index,PLOT_DRAW_TYPE,DRAW_SECTION); case 2: PlotIndexSetInteger(index,PLOT_DRAW_TYPE,DRAW_HISTOGRAM); case 3: PlotIndexSetInteger(index,PLOT_DRAW_TYPE,DRAW_ARROW); case 4: PlotIndexSetInteger(index,PLOT_DRAW_TYPE,DRAW_ZIGZAG); case 12: PlotIndexSetInteger(index,PLOT_DRAW_TYPE,DRAW_NONE); default: PlotIndexSetInteger(index,PLOT_DRAW_TYPE,DRAW_LINE); } switch(style) { case 0: PlotIndexSetInteger(index,PLOT_LINE_STYLE,STYLE_SOLID); case 1: PlotIndexSetInteger(index,PLOT_LINE_STYLE,STYLE_DASH); case 2: PlotIndexSetInteger(index,PLOT_LINE_STYLE,STYLE_DOT); case 3: PlotIndexSetInteger(index,PLOT_LINE_STYLE,STYLE_DASHDOT); case 4: PlotIndexSetInteger(index,PLOT_LINE_STYLE,STYLE_DASHDOTDOT); default: return; } | SetIndexStyle Imposta il nuovo tipo, stile, larghezza e colore per una determinata linea dell'indicatore. PlotIndexSetInteger |
void SetLevelStyle(int draw_style, int line_width, color clr=CLR_NONE) | void SetLevelStyleMQL4(int draw_style, int line_width, color clr=CLR_NONE) { IndicatorSetInteger(INDICATOR_LEVELWIDTH,line_width); if(clr!=CLR_NONE) IndicatorSetInteger(INDICATOR_LEVELCOLOR,clr); switch(draw_style) { case 0: IndicatorSetInteger(INDICATOR_LEVELSTYLE,STYLE_SOLID); case 1: IndicatorSetInteger(INDICATOR_LEVELSTYLE,STYLE_DASH); case 2: IndicatorSetInteger(INDICATOR_LEVELSTYLE,STYLE_DOT); case 3: IndicatorSetInteger(INDICATOR_LEVELSTYLE,STYLE_DASHDOT); case 4: IndicatorSetInteger(INDICATOR_LEVELSTYLE,STYLE_DASHDOTDOT); default: return; } } | SetLevelStyle La funzione imposta un nuovo stile, larghezza e colore dei livelli orizzontali dell'indicatore da visualizzare in una finestra separata. IndicatorSetInteger |
void SetLevelValue(int level, double value) | bool IndicatorSetDouble(INDICATOR_LEVELVALUE,level,value) | SetLevelValue La funzione imposta un valore per un dato livello orizzontale dell'indicatore da visualizzare in una finestra separata. IndicatorSetDouble |
11. Funzioni di Data e Ora
MQL4 | MQL5 | Descrizione |
---|---|---|
int Day() | int DayMQL4() { MqlDateTime tm; TimeCurrent(tm); return(tm.day); } | Day Restituisce il giorno del mese corrente, ovvero il giorno del mese dell'ultima ora nota del server. TimeCurrent, MqlDateTime |
int DayOfWeek() | int DayOfWeekMQL4() { MqlDateTime tm; TimeCurrent(tm); return(tm.day_of_week); } | DayOfWeek Restituisce il giorno della settimana in base zero corrente (0-domenica,1,2,3,4,5,6) dell'ultima ora nota del server. TimeCurrent, MqlDateTime |
int DayOfYear() | int DayOfYearMQL4() { MqlDateTime tm; TimeCurrent(tm); return(tm.day_of_year); } | DayOfYear Restituisce il giorno dell'anno corrente (1 significa 1 gennaio,..,365(6) corrisponde al 31 dicembre), ovvero il giorno dell'anno dell'ultima ora nota del server. TimeCurrent, MqlDateTime |
int Hour() | int HourMQL4() { MqlDateTime tm; TimeCurrent(tm); return(tm.hour); } | Hour Restituisce l'ora (0,1,2,..23) dell'ultima ora nota del server al momento dell'avvio del programma (questo valore non cambierà entro l'ora dell'esecuzione del programma). TimeCurrent, MqlDateTime |
int Minute() | int MinuteMQL4() { MqlDateTime tm; TimeCurrent(tm); return(tm.min); } | Minute Restituisce il minuto corrente (0,1,2,..59) dell'ultimo tempo noto del server al momento dell'avvio del programma (questo valore non cambierà entro il tempo di esecuzione del programma). TimeCurrent, MqlDateTime |
int Month() | int MonthMQL4() { MqlDateTime tm; TimeCurrent(tm); return(tm.mon); } | Month Restituisce il mese corrente come numero (1-gennaio,2,3,4,5,6,7,8,9,10,11,12), ovvero il numero del mese dell'ultima ora nota del server. TimeCurrent, MqlDateTime |
int Seconds() | int SecondsMQL4() { MqlDateTime tm; TimeCurrent(tm); return(tm.sec); } | Seconds Restituisce il numero di secondi trascorsi dall'inizio del minuto corrente dell'ultima ora nota del server al momento dell'avvio del programma (questo valore non cambierà entro il tempo di esecuzione del programma). TimeCurrent, MqlDateTime |
datetime TimeCurrent() | datetime TimeCurrent() | TimeCurrent Restituisce l'ultima ora nota del server (ora di ricezione dell'ultima citazione) come numero di secondi trascorsi dalle 00:00 del 1 gennaio 1970. TimeCurrent |
int TimeDay(datetime date) | int TimeDayMQL4(datetime date) { MqlDateTime tm; TimeToStruct(date,tm); return(tm.day); } | TimeDay Restituisce il giorno del mese (1 - 31) per la data specificata. TimeToStruct, MqlDateTime |
int TimeDayOfWeek(datetime date) | int TimeDayOfWeekMQL4(datetime date) { MqlDateTime tm; TimeToStruct(date,tm); return(tm.day_of_week); } | TimeDayOfWeek Restituisce il giorno della settimana in base zero (0 significa domenica,1,2,3,4,5,6) per la data specificata. TimeToStruct, MqlDateTime |
int TimeDayOfYear(datetime date) | int TimeDayOfYearMQL4(datetime date) { MqlDateTime tm; TimeToStruct(date,tm); return(tm.day_of_year); } | TimeDayOfYear Restituisce il giorno (1 significa 1 gennaio,..,365(6) corrisponde al 31 dicembre) dell'anno per la data specificata. TimeToStruct, MqlDateTime |
int TimeHour(datetime time) | int TimeHourMQL4(datetime date) { MqlDateTime tm; TimeToStruct(date,tm); return(tm.hour); } | TimeHour Restituisce l'ora per il tempo specificato. TimeToStruct, MqlDateTime |
datetime TimeLocal() | datetime TimeLocal() | TimeLocal Restituisce l'ora del computer locale come numero di secondi trascorsi dalle 00:00 del 1 gennaio 1970. TimeLocal |
int TimeMinute(datetime time) | int TimeMinuteMQL4(datetime date) { MqlDateTime tm; TimeToStruct(date,tm); return(tm.min); } | TimeMinute Restituisce il minuto per il tempo specificato. TimeToStruct, MqlDateTime |
int TimeMonth(datetime time) | int TimeMonthMQL4(datetime date) { MqlDateTime tm; TimeToStruct(date,tm); return(tm.mon); } | TimeMonth Restituisce il numero del mese per il tempo specificato. TimeToStruct, MqlDateTime |
int TimeSeconds(datetime time) | int TimeSecondsMQL4(datetime date) { MqlDateTime tm; TimeToStruct(date,tm); return(tm.sec); } | TimeSeconds Restituisce la quantità di secondi trascorsi dall'inizio del minuto per il tempo specificato. TimeToStruct, MqlDateTime |
int TimeYear(datetime time) | int TimeYearMQL4(datetime date) { MqlDateTime tm; TimeToStruct(date,tm); return(tm.year); } | TimeYear Restituisce l'anno per la data specificata. Il valore restituito può essere compreso tra 1970 e 2037. TimeToStruct, MqlDateTime |
int Year() | int YearMQL4() { MqlDateTime tm; TimeCurrent(tm); return(tm.year); } | Year Restituisce l'anno corrente, ovvero l'anno dell'ultima ora nota del server. TimeCurrent, MqlDateTime |
12. Funzioni dei File
MQL4 | MQL5 | Descrizione |
---|---|---|
void FileClose(int handle) | void FileClose(int file_handle) | FileClose Chiude il file precedentemente aperto dalla funzione FileOpen(). FileClose |
void FileDelete(string filename) | bool FileDelete(string file_name int common_flag=0) | FileDelete Rimuove il nome del file specificato. FileDelete |
void FileFlush(int handle) | void FileFlush(int file_handle) | FileFlush Scarica tutti i dati memorizzati nel buffer del file sul disco. FileFlush |
bool FileIsEnding(int handle) | bool FileIsEnding(int file_handle) | FileIsEnding Restituisce il vero logico se il puntatore del file si trova alla fine del file, altrimenti restituisce “falso”. FileIsEnding |
bool FileIsLineEnding(int handle) | bool FileIsLineEnding(int file_handle) | FileIsLineEnding Per il file CSV restituisce il vero logico se il puntatore del file si trova alla fine della riga, altrimenti restituisce “falso”. FileIsLineEnding |
int FileOpen(string filename, int mode, int delimiter=';') | int FileOpen(string ile_name, int pen_flags, short delimiter='\t' uint codepage=CP_ACP) | FileOpen Apre il file per l'input e/o l'output. Restituisce un handle di file per il file aperto o -1 (se la funzione non riesce). FileOpen |
int FileOpenHistory(string filename, int mode, int delimiter=';') | - | FileOpenHistory Apre il file nella directory della cronologia corrente (directory_terminal\history\nome_server) o nelle sue sottocartelle. Restituisce l'handle del file per il file aperto. Se la funzione fallisce, il valore restituito è -1. |
int FileReadArray(int handle, object &array[], int start, int count) | uint FileReadArray(int file_handle, void array[], int start_item=0, int items_count=WHOLE_ARRAY) | FileReadArray Legge la quantità specificata di elementi dal file binario nell'array. FileReadArray |
double FileReadDouble(int handle, int size=DOUBLE_VALUE) | double FileReadDoubleMQL4(int handle, int size=DOUBLE_VALUE) { return(FileReadDouble(handle)); } | FileReadDouble Legge il numero a precisione doppia con virgola mobile dalla posizione del file binario corrente. FileReadDouble |
int FileReadInteger(int handle, int size=LONG_VALUE) | int FileReadInteger(int file_handle, int size=INT_VALUE) | FileReadInteger La funzione legge l'intero dalla posizione del file binario corrente. FileReadInteger |
double FileReadNumber(int handle) | double FileReadNumber(int file_handle) | FileReadNumber Legge il numero dalla posizione del file corrente prima del delimitatore. Solo per file CSV. FileReadNumber |
string FileReadString(int handle, int length=0) | string FileReadString(int file_handle, int size=-1) | FileReadString Функция читает строку с текущей позиции файла. FileReadString |
bool FileSeek(int handle, int offset, int origin) | bool FileSeekMQL4(long handle, int offset, ENUM_FILE_POSITION origin) { FileSeek(handle,offset,origin); return(true); } | FileSeek La funzione sposta il puntatore del file in una nuova posizione che è un offset, in byte, dall'inizio, dalla fine o dalla posizione del file corrente. FileSeek |
int FileSize(int handle) | ulong FileSize(int file_handle) | FileSize La funzione restituisce la dimensione del file in byte. FileSize |
int FileTell(int handle) | ulong FileTell(int file_handle) | FileTell Restituisce la posizione corrente del puntatore del file. FileTell |
int FileWrite(int handle,...) | uint FileWrite(int file_handle,...) | FileWrite La funzione è intesa per la scrittura di dati in un file CSV, il delimitatore viene inserito automaticamente a meno che non è uguale a 0. FileWrite |
int FileWriteArray(int handle, object array[], int start, int count) | int FileWriteArray(int file_handle, void array[], int start_item=0, int items_count=WHOLE_ARRAY) | FileWriteArray La funzione scrive l'array in un file binario. FileWriteArray |
int FileWriteDouble(int handle, double value, int size=DOUBLE_VALUE) | uint FileWriteDouble(int file_handle, double dvalue) | FileWriteDouble La funzione scrive un valore double con virgola mobile in un file binario. FileWriteDouble |
int FileWriteInteger(int handle, int value, int size=LONG_VALUE) | uint FileWriteInteger(int file_handle, int ivalue, int size=INT_VALUE) | FileWriteInteger La funzione scrive il valore intero in un file binario. FileWriteInteger |
int FileWriteString(int handle, string value, int size) | uint FileWriteString(int file_handle, string svalue, int size=-1) | FileWriteString La funzione scrive la stringa in un file binario dalla posizione del file corrente. FileWriteString |
13. Variabili Globali
MQL4 | MQL5 | Descrizione |
---|---|---|
bool GlobalVariableCheck(string name) | bool GlobalVariableCheck(string name) | GlobalVariableCheck Restituisce TRUE se la variabile globale esiste, altrimenti restituisce FALSE. GlobalVariableCheck |
bool GlobalVariableDel(string name) | bool GlobalVariableDel(string name) | GlobalVariableDel Elimina la variabile globale. GlobalVariableDel |
double GlobalVariableGet(string name) | double GlobalVariableGet(string name) | GlobalVariableGet Restituisce il valore di una variabile globale esistente o 0 se si verifica un errore. GlobalVariableGet |
string GlobalVariableName(int index) | string GlobalVariableName(int index) | GlobalVariableName Restituisce il nome di una variabile globale dal suo indice nell'elenco delle variabili globali GlobalVariableName |
datetime GlobalVariableSet(string name, double value) | datetime GlobalVariableSet(string name, double value) | GlobalVariableSet Imposta il valore della variabile globale. Se la variabile non esiste, il sistema crea una nuova variabile globale. GlobalVariableSet |
bool GlobalVariableSetOnCondition(string name, double value, double check_value) | bool GlobalVariableSetOnCondition(string name, double value, double check_value) | GlobalVariableSetOnCondition Imposta il nuovo valore della variabile globale esistente se il valore corrente è uguale al terzo parametro check_value. GlobalVariableSetOnCondition |
int GlobalVariablesDeleteAll(string prefix_name=NULL) | int GlobalVariablesDeleteAll(string prefix_name=NULL datetime limit_data=0) | GlobalVariablesDeleteAll Elimina le variabili globali. GlobalVariablesDeleteAll |
int GlobalVariablesTotal() | int GlobalVariablesTotal() | GlobalVariablesTotal La funzione restituisce il conteggio totale delle variabili globali. GlobalVariablesTotal |
14. Funzioni Matematiche
MQL4 | MQL5 | Descrizione |
---|---|---|
double MathAbs(double value) | double MathAbs(double value) | MathAbs Restituisce il valore assoluto (modulo) del valore numerico specificato MathAbs |
double MathArccos(double x) | double MathArccos(double val) | MathArccos La funzione MathArccos restituisce l'arcocoseno di x nell'intervallo da 0 a Pi (in radianti). MathArccos |
double MathArcsin(double x) | double MathArcsin(double val) | MathArcsin La funzione MathArcsin restituisce l'arcoseno di x nell'intervallo da -Pi/2 a Pi/2 radianti. MathArcsin |
double MathArctan(double x) | double MathArctan(double value) | MathArctan Il MathArctan restituisce l'arcotangente di x. MathArctan |
double MathCeil(double x) | double MathCeil(double val) | MathCeil La funzione MathCeil restituisce un valore numerico che rappresenta l'intero più piccolo che supera o è uguale a x. MathCeil |
double MathCos(double value) | double MathCos(double value) | MathCos Restituisce il coseno dell'angolo specificato. MathCos |
double MathExp(double d) | double MathExp(double value) | MathExp La funzione restituisce il valore di e elevato alla potenza di d. MathExp |
double MathFloor(double x) | double MathFloor(double val) | MathFloor La funzione MathFloor restituisce un valore numerico che rappresenta il più grande numero intero che è minore o uguale a x. MathFloor |
double MathLog(double x) | double MathLog(double val) | MathLog La funzione MathLog restituisce il logaritmo naturale di x se ha esito positivo. MathLog |
double MathMax(double value1, double value2) | double MathMax(double value1, double value2) | MathMax Restituisce il valore massimo dei due valori numerici MathMax |
double MathMin(double value1, double value2) | double MathMin(double value1, double value2) | MathMin Restituisce il valore minimo dei due valori numerici MathMin |
double MathMod(double value1, double value2) | double MathMod(double value1, double value2) | MathMod La funzione restituisce il resto della divisione reale di due numeri. MathMod |
double MathPow(double base, double exponent) | double MathPow(double base, double exponent) | MathPow Restituisce il valore dell'espressione di base elevato alla potenza specificata (valore dell'esponente). MathPow |
int MathRand() | int MathRand() | MathRand Restituisce un numero intero pseudocasuale compreso tra 0 e 32767. MathRand |
double MathRound(double value) | double MathRound(double value) | MathRound La funzione restituisce un valore arrotondato al numero intero più vicino al valore numerico specificato. MathRound |
double MathSin(double value) | double MathSin(double value) | MathSin Restituisce il seno di un angolo specificato. MathSin |
double MathSqrt(double x) | double MathSqrt(double value) | MathSqrt La funzione MathSqrt restituisce la radice quadrata di x. MathSqrt |
void MathSrand(int seed) | void MathSrand(int seed) | MathSrand La funzione MathSrand() imposta il punto di partenza per generare una serie di numeri interi pseudocasuali. MathSrand |
double MathTan(double x) | double MathTan(double rad) | MathTan MathTan restituisce la tangente di x. MathTan |
15. Funzioni dell’Oggetto
MQL4 | MQL5 | Descrizione |
---|---|---|
bool ObjectCreate(string name, int type, int window, datetime time1, double price1, datetime time2=0, double price2=0, datetime time3=0, double price3=0) | bool ObjectCreateMQL4(string name, ENUM_OBJECT type, int window, datetime time1, double price1, datetime time2=0, double price2=0, datetime time3=0, double price3=0) { return(ObjectCreate(0,name,type,window, time1,price1,time2,price2,time3,price3)); } | ObjectCreate Creazione di un oggetto con il nome, il tipo e le coordinate iniziali specificati nella finestra specificata. ObjectCreate |
bool ObjectDelete(string name) | bool ObjectDeleteMQL4(string name) { return(ObjectDelete(0,name)); } | ObjectDelete Elimina l'oggetto con il nome specificato. ObjectDelete |
string ObjectDescription(string name) | string ObjectDescriptionMQL4(string name) { return(ObjectGetString(0,name,OBJPROP_TEXT)); } | ObjectDescription Restituire la descrizione dell'oggetto. ObjectGetString |
int ObjectFind(string name) | int ObjectFindMQL4(string name) { return(ObjectFind(0,name)); } | ObjectFind Cerca un oggetto con il nome specificato. ObjectFind |
double ObjectGet(string name, int prop_id) | double ObjectGetMQL4(string name, int index) { switch(index) { case OBJPROP_TIME1: return(ObjectGetInteger(0,name,OBJPROP_TIME)); case OBJPROP_PRICE1: return(ObjectGetDouble(0,name,OBJPROP_PRICE)); case OBJPROP_TIME2: return(ObjectGetInteger(0,name,OBJPROP_TIME,1)); case OBJPROP_PRICE2: return(ObjectGetDouble(0,name,OBJPROP_PRICE,1)); case OBJPROP_TIME3: return(ObjectGetInteger(0,name,OBJPROP_TIME,2)); case OBJPROP_PRICE3: return(ObjectGetDouble(0,name,OBJPROP_PRICE,2)); case OBJPROP_COLOR: return(ObjectGetInteger(0,name,OBJPROP_COLOR)); case OBJPROP_STYLE: return(ObjectGetInteger(0,name,OBJPROP_STYLE)); case OBJPROP_WIDTH: return(ObjectGetInteger(0,name,OBJPROP_WIDTH)); case OBJPROP_BACK: return(ObjectGetInteger(0,name,OBJPROP_WIDTH)); case OBJPROP_RAY: return(ObjectGetInteger(0,name,OBJPROP_RAY_RIGHT)); case OBJPROP_ELLIPSE: return(ObjectGetInteger(0,name,OBJPROP_ELLIPSE)); case OBJPROP_SCALE: return(ObjectGetDouble(0,name,OBJPROP_SCALE)); case OBJPROP_ANGLE: return(ObjectGetDouble(0,name,OBJPROP_ANGLE)); case OBJPROP_ARROWCODE: return(ObjectGetInteger(0,name,OBJPROP_ARROWCODE)); case OBJPROP_TIMEFRAMES: return(ObjectGetInteger(0,name,OBJPROP_TIMEFRAMES)); case OBJPROP_DEVIATION: return(ObjectGetDouble(0,name,OBJPROP_DEVIATION)); case OBJPROP_FONTSIZE: return(ObjectGetInteger(0,name,OBJPROP_FONTSIZE)); case OBJPROP_CORNER: return(ObjectGetInteger(0,name,OBJPROP_CORNER)); case OBJPROP_XDISTANCE: return(ObjectGetInteger(0,name,OBJPROP_XDISTANCE)); case OBJPROP_YDISTANCE: return(ObjectGetInteger(0,name,OBJPROP_YDISTANCE)); case OBJPROP_FIBOLEVELS: return(ObjectGetInteger(0,name,OBJPROP_LEVELS)); case OBJPROP_LEVELCOLOR: return(ObjectGetInteger(0,name,OBJPROP_LEVELCOLOR)); case OBJPROP_LEVELSTYLE: return(ObjectGetInteger(0,name,OBJPROP_LEVELSTYLE)); case OBJPROP_LEVELWIDTH: return(ObjectGetInteger(0,name,OBJPROP_LEVELWIDTH)); } } | ObjectGet La funzione restituisce il valore della proprietà dell'oggetto specificata. ObjectGetInteger, ObjectGetDouble |
string ObjectGetFiboDescription(string name, int index) | string ObjectGetFiboDescriptionMQL4(string name, int index) { return(ObjectGetString(0,name,OBJPROP_LEVELTEXT,index)); } | ObjectGetFiboDescription La funzione restituisce la descrizione del livello di un oggetto Fibonacci. ObjectGetString |
int ObjectGetShiftByValue(string name, double value) | int ObjectGetShiftByValueMQL4(string name, double value) { ENUM_TIMEFRAMES timeframe=TFMigrate(PERIOD_CURRENT); datetime Arr[]; int shift; MqlRates mql4[]; if(ObjectGetTimeByValue(0,name,value)<0) return(-1); CopyRates(NULL,timeframe,0,1,mql4); if(CopyTime(NULL,timeframe,mql4[0].time, ObjectGetTimeByValue(0,name,value),Arr)>0) return(ArraySize(Arr)-1); else return(-1); } | ObjectGetShiftByValue La funzione calcola e restituisce l'indice della barra (spostamento relativo alla barra corrente) per il prezzo indicato. MqlRates, ObjectGetTimeByValue, CopyRates, CopyTime, ArraySize |
double ObjectGetValueByShift(string name, int shift) | double ObjectGetValueByShiftMQL4(string name, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(PERIOD_CURRENT); MqlRates mql4[]; CopyRates(NULL,timeframe,shift,1,mql4); return(ObjectGetValueByTime(0,name,mql4[0].time,0)); } | ObjectGetValueByShift La funzione calcola e restituisce il valore del prezzo per la barra specificata (spostamento relativo alla barra corrente). MqlRates, CopyRates, ObjectGetValueByTime |
bool ObjectMove(string name, int point, datetime time1, double price1) | bool ObjectMoveMQL4(string name, int point, datetime time1, double price1) { return(ObjectMove(0,name,point,time1,price1)); } | ObjectMove La funzione sposta una coordinata dell’oggetto nel grafico. Gli oggetti possono avere da una a tre coordinate a seconda del loro tipo. ObjectMove |
string ObjectName(int index) | string ObjectNameMQL4(int index) { return(ObjectName(0,index)); } | ObjectName La funzione restituisce il nome dell'oggetto in base al suo indice nell'elenco degli oggetti. ObjectName |
int ObjectsDeleteAll(int window=EMPTY, int type=EMPTY) | int ObjectsDeleteAllMQL4(int window=EMPTY, int type=EMPTY) { return(ObjectsDeleteAll(0,window,type)); } | ObjectsDeleteAll Rimuove tutti gli oggetti del tipo specificato e nella sottofinestra specificata del grafico. ObjectsDeleteAll |
bool ObjectSet(string name, int prop_id, double value) | bool ObjectSetMQL4(string name, int index, double value) { switch(index) { case OBJPROP_TIME1: ObjectSetInteger(0,name,OBJPROP_TIME,(int)value);return(true); case OBJPROP_PRICE1: ObjectSetDouble(0,name,OBJPROP_PRICE,value);return(true); case OBJPROP_TIME2: ObjectSetInteger(0,name,OBJPROP_TIME,1,(int)value);return(true); case OBJPROP_PRICE2: ObjectSetDouble(0,name,OBJPROP_PRICE,1,value);return(true); case OBJPROP_TIME3: ObjectSetInteger(0,name,OBJPROP_TIME,2,(int)value);return(true); case OBJPROP_PRICE3: ObjectSetDouble(0,name,OBJPROP_PRICE,2,value);return(true); case OBJPROP_COLOR: ObjectSetInteger(0,name,OBJPROP_COLOR,(int)value);return(true); case OBJPROP_STYLE: ObjectSetInteger(0,name,OBJPROP_STYLE,(int)value);return(true); case OBJPROP_WIDTH: ObjectSetInteger(0,name,OBJPROP_WIDTH,(int)value);return(true); case OBJPROP_BACK: ObjectSetInteger(0,name,OBJPROP_BACK,(int)value);return(true); case OBJPROP_RAY: ObjectSetInteger(0,name,OBJPROP_RAY_RIGHT,(int)value);return(true); case OBJPROP_ELLIPSE: ObjectSetInteger(0,name,OBJPROP_ELLIPSE,(int)value);return(true); case OBJPROP_SCALE: ObjectSetDouble(0,name,OBJPROP_SCALE,value);return(true); case OBJPROP_ANGLE: ObjectSetDouble(0,name,OBJPROP_ANGLE,value);return(true); case OBJPROP_ARROWCODE: ObjectSetInteger(0,name,OBJPROP_ARROWCODE,(int)value);return(true); case OBJPROP_TIMEFRAMES: ObjectSetInteger(0,name,OBJPROP_TIMEFRAMES,(int)value);return(true); case OBJPROP_DEVIATION: ObjectSetDouble(0,name,OBJPROP_DEVIATION,value);return(true); case OBJPROP_FONTSIZE: ObjectSetInteger(0,name,OBJPROP_FONTSIZE,(int)value);return(true); case OBJPROP_CORNER: ObjectSetInteger(0,name,OBJPROP_CORNER,(int)value);return(true); case OBJPROP_XDISTANCE: ObjectSetInteger(0,name,OBJPROP_XDISTANCE,(int)value);return(true); case OBJPROP_YDISTANCE: ObjectSetInteger(0,name,OBJPROP_YDISTANCE,(int)value);return(true); case OBJPROP_FIBOLEVELS: ObjectSetInteger(0,name,OBJPROP_LEVELS,(int)value);return(true); case OBJPROP_LEVELCOLOR: ObjectSetInteger(0,name,OBJPROP_LEVELCOLOR,(int)value);return(true); case OBJPROP_LEVELSTYLE: ObjectSetInteger(0,name,OBJPROP_LEVELSTYLE,(int)value);return(true); case OBJPROP_LEVELWIDTH: ObjectSetInteger(0,name,OBJPROP_LEVELWIDTH,(int)value);return(true); default: return(false); } return(false); } | ObjectSet Modifica il valore della proprietà dell'oggetto specificata. ObjectSetInteger, ObjectSetDouble |
bool ObjectSetFiboDescription(string name, int index, string text) | bool ObjectSetFiboDescriptionMQL4(string name, int index, string text) { return(ObjectSetString(0,name,OBJPROP_LEVELTEXT,index,text)); } | ObjectSetFiboDescription La funzione assegna una nuova descrizione a un livello di un oggetto Fibonacci. ObjectSetString |
bool ObjectSetText(string name, string text, int font_size, string font_name=NULL, color text_color=CLR_NONE) | bool ObjectSetTextMQL4(string name, string text, int font_size, string font="", color text_color=CLR_NONE) { int tmpObjType=(int)ObjectGetInteger(0,name,OBJPROP_TYPE); if(tmpObjType!=OBJ_LABEL && tmpObjType!=OBJ_TEXT) return(false); if(StringLen(text)>0 && font_size>0) { if(ObjectSetString(0,name,OBJPROP_TEXT,text)==true && ObjectSetInteger(0,name,OBJPROP_FONTSIZE,font_size)==true) { if((StringLen(font)>0) && ObjectSetString(0,name,OBJPROP_FONT,font)==false) return(false); if(text_color>-1 && ObjectSetInteger(0,name,OBJPROP_COLOR,text_color)==false) return(false); return(true); } return(false); } return(false); } | ObjectSetText Modifica la descrizione dell'oggetto. ObjectGetInteger, ObjectSetString, ObjectSetInteger StringLen |
int ObjectsTotal(int type=EMPTY) | int ObjectsTotalMQL4(int type=EMPTY, int window=-1) { return(ObjectsTotal(0,window,type)); } | ObjectsTotal Restituisce la quantità totale di oggetti del tipo specificato nel grafico. ObjectsTotal |
int ObjectType(string name) | int ObjectTypeMQL4(string name) { return((int)ObjectGetInteger(0,name,OBJPROP_TYPE)); } | ObjectType La funzione restituisce il puntatore all'oggetto. ObjectGetInteger |
16. Funzioni della Stringa
MQL4 | MQL5 | Descrizione |
---|---|---|
string StringConcatenate(...) | int StringConcatenate(string &string_var, void argument1 void argument2 ...) | StringConcatenate Forma una stringa dei dati passati e la restituisce. StringConcatenate |
int StringFind(string text, string matched_text, int start=0) | int StringFind(string string_value, string match_substring, int start_pos=0) | StringFind Cerca una sottostringa. Restituisce la posizione nella stringa da cui inizia la sottostringa cercata o -1 se la sottostringa non è stata trovata. StringFind |
int StringGetChar(string text, int pos) | ushort StringGetCharacter(string string_value, int pos) | StringGetChar Restituisce il carattere (codice) dalla posizione specificata nella stringa. StringGetCharacter |
int StringLen(string text) | int StringLen(string string_value) | StringLen Restituisce il conteggio dei caratteri in una stringa. StringLen |
string StringSetChar(string text, int pos, int value) | bool StringSetCharacter(string &string_var, int pos, ushort character) | StringSetChar Restituisce la copia della stringa con il carattere modificato nella posizione specificata. StringSetCharacter |
string StringSubstr(string text, int start, int length=0) | string StringSubstr(string string_value, int start_pos, int length=-1) | StringSubstr Estrae una sottostringa da una stringa di testo a partire dalla posizione specificata. StringSubstr |
string StringTrimLeft(string text) | int StringTrimLeft(string& string_var) | StringTrimLeft La funzione taglia i caratteri di avanzamento riga, gli spazi e le tabulazioni nella parte sinistra della stringa. StringTrimLeft |
string StringTrimRight(string text) | int StringTrimRight(string& string_var) | StringTrimRight La funzione taglia i caratteri di avanzamento riga, gli spazi e le tabulazioni nella parte destra della stringa. StringTrimRight |
17. Indicatori Tecnici
I principi di utilizzo degli indicatori tecnici negli Expert Advisor vengono trattati nell'articolo MQL5per Principianti: Guida all'Utilizzo degli Indicatori Tecnici negli Expert Advisor. Il metodo utilizzato in questo riferimento è sufficiente per ottenere i risultati del calcolo dell'indicatore per il prezzo specificato. Per utilizzare questo metodo, abbiamo bisogno della funzione ausiliaria:
double CopyBufferMQL4(int handle,int index,int shift) { double buf[]; switch(index) { case 0: if(CopyBuffer(handle,0,shift,1,buf)>0) return(buf[0]); break; case 1: if(CopyBuffer(handle,1,shift,1,buf)>0) return(buf[0]); break; case 2: if(CopyBuffer(handle,2,shift,1,buf)>0) return(buf[0]); break; case 3: if(CopyBuffer(handle,3,shift,1,buf)>0) return(buf[0]); break; case 4: if(CopyBuffer(handle,4,shift,1,buf)>0) return(buf[0]); break; default: break; } return(EMPTY_VALUE); }dichiariamo le seguenti costanti:
ENUM_MA_METHOD MethodMigrate(int method) { switch(method) { case 0: return(MODE_SMA); case 1: return(MODE_EMA); case 2: return(MODE_SMMA); case 3: return(MODE_LWMA); default: return(MODE_SMA); } } ENUM_APPLIED_PRICE PriceMigrate(int price) { switch(price) { case 1: return(PRICE_CLOSE); case 2: return(PRICE_OPEN); case 3: return(PRICE_HIGH); case 4: return(PRICE_LOW); case 5: return(PRICE_MEDIAN); case 6: return(PRICE_TYPICAL); case 7: return(PRICE_WEIGHTED); default: return(PRICE_CLOSE); } } ENUM_STO_PRICE StoFieldMigrate(int field) { switch(field) { case 0: return(STO_LOWHIGH); case 1: return(STO_CLOSECLOSE); default: return(STO_LOWHIGH); } } //+------------------------------------------------------------------+ enum ALLIGATOR_MODE { MODE_GATORJAW=1, MODE_GATORTEETH, MODE_GATORLIPS }; enum ADX_MODE { MODE_MAIN, MODE_PLUSDI, MODE_MINUSDI }; enum UP_LOW_MODE { MODE_BASE, MODE_UPPER, MODE_LOWER }; enum ICHIMOKU_MODE { MODE_TENKANSEN=1, MODE_KIJUNSEN, MODE_SENKOUSPANA, MODE_SENKOUSPANB, MODE_CHINKOUSPAN }; enum MAIN_SIGNAL_MODE{ MODE_MAIN, MODE_SIGNAL };
MQL4 | MQL5 | Descrizione: |
---|---|---|
double iAC(string symbol, int timeframe, int shift) | double iACMQL4(string symbol, int tf, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); int handle=iAC(symbol,timeframe); if(handle<0) { Print("The iAC object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iAC Calcola l'oscillatore Accelerator/Decelerator di Bill Williams. iAC |
double iAD(string symbol, int timeframe, int shift) | double iADMQL4(string symbol, int tf, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); int handle=(int)iAD(symbol,timeframe,VOLUME_TICK); if(handle<0) { Print("The iAD object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iAD Calcola l'indicatore Accumulo/Distribuzione e ne restituisce il valore. iAD |
double iAlligator(string symbol, int timeframe, int jaw_period, int jaw_shift, int teeth_period, int teeth_shift, int lips_period, int lips_shift, int ma_method, int applied_price, int mode, int shift) | double iAlligatorMQL4(string symbol, int tf, int jaw_period, int jaw_shift, int teeth_period, int teeth_shift, int lips_period, int lips_shift, int method, int price, int mode, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); ENUM_MA_METHOD ma_method=MethodMigrate(method); ENUM_APPLIED_PRICE applied_price=PriceMigrate(price); int handle=iAlligator(symbol,timeframe,jaw_period,jaw_shift, teeth_period,teeth_shift, lips_period,lips_shift, ma_method,applied_price); if(handle<0) { Print("The iAlligator object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,mode-1,shift)); } | iAlligator Calcola l'Alligatore di Bill Williams e ne restituisce il valore. iAlligator |
double iADX(string symbol, int timeframe, int period, int applied_price, int mode, int shift) | double iADXMQL4(string symbol, int tf, int period, int price, int mode, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); int handle=iADX(symbol,timeframe,period); if(handle<0) { Print("The iADX object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,mode,shift)); } | iADX Calcola l'indice direzionale del movimento e ne restituisce il valore. iADX |
double iATR(string symbol, int timeframe, int period, int shift) | double iATRMQL4(string symbol, int tf, int period, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); int handle=iATR(symbol,timeframe,period); if(handle<0) { Print("The iATR object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iATR Calcola l'indicatore dell'intervallo vero medio e ne restituisce il valore. iATR |
double iAO(string symbol, int timeframe, int shift) | double iAOMQL4(string symbol, int tf, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); int handle=iAO(symbol,timeframe); if(handle<0) { Print("The iAO object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iAO Calcola l'oscillatore Awesome di Bill Williams e ne restituisce il valore. iAO |
double iBearsPower(string symbol, int timeframe, int period, int applied_price, int shift) | double iBearsPowerMQL4(string symbol, int tf, int period, int price, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); int handle=iBearsPower(symbol,timeframe,period); if(handle<0) { Print("The iBearsPower object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iBearsPower Calcola l'indicatore Bears Power e ne restituisce il valore. iBearsPower |
double iBands(string symbol, int timeframe, int period, int deviation, int bands_shift, int applied_price, int mode, int shift) | double iBandsMQL4(string symbol, int tf, int period, double deviation, int bands_shift, int method, int mode, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); ENUM_MA_METHOD ma_method=MethodMigrate(method); int handle=iBands(symbol,timeframe,period, bands_shift,deviation,ma_method); if(handle<0) { Print("The iBands object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,mode,shift)); } | iBands Calcola l'indicatore delle bande di Bollinger e ne restituisce il valore. iBands |
double iBandsOnArray(double array[], int total, int period, int deviation, int bands_shift, int mode, int shift) | - | iBandsOnArray Calcolo dell'indicatore Bande di Bollinger su dati memorizzati in un array numerico. |
double iBullsPower(string symbol, int timeframe, int period, int applied_price, int shift) | double iBullsPowerMQL4(string symbol, int tf, int period, int price, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); int handle=iBullsPower(symbol,timeframe,period); if(handle<0) { Print("The iBullsPower object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iBullsPower Calcola l'indicatore Bulls Power e restituisce il suo valore. iBullsPower |
double iCCI(string symbol, int timeframe, int period, int applied_price, int shift) | double iCCIMQL4(string symbol, int tf, int period, int price, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); ENUM_APPLIED_PRICE applied_price=PriceMigrate(price); int handle=iCCI(symbol,timeframe,period,price); if(handle<0) { Print("The iCCI object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iCCI Calcola l'indice del canale Commodity e ne restituisce il valore. iCCI |
double iCCIOnArray(double array[], int total, int period, int shift) | - | iCCIOnArray Calcolo del Commodity Channel Index sui dati archiviati in un array numerico. |
double iCustom(string symbol, int timeframe, string name, ..., int mode, int shift) | int iCustom(string symbol, ENUM_TIMEFRAMES period, string name ...) | iCustom Calcola l'indicatore personalizzato specificato e ne restituisce il valore. iCustom |
double iDeMarker(string symbol, int timeframe, int period, int shift) | double iDeMarkerMQL4(string symbol, int tf, int period, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); int handle=iDeMarker(symbol,timeframe,period); if(handle<0) { Print("The iDeMarker object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iDeMarker Calcola l'indicatore DeMarker e ne restituisce il valore. iDeMarker |
double iEnvelopes(string symbol, int timeframe, int ma_period, int ma_method, int ma_shift, int applied_price, double deviation, int mode, int shift) | double EnvelopesMQL4(string symbol, int tf, int ma_period, int method, int ma_shift, int price, double deviation, int mode, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); ENUM_MA_METHOD ma_method=MethodMigrate(method); ENUM_APPLIED_PRICE applied_price=PriceMigrate(price); int handle=iEnvelopes(symbol,timeframe, ma_period,ma_shift,ma_method, applied_price,deviation); if(handle<0) { Print("The iEnvelopes object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,mode-1,shift)); } | iEnvelopes Calcola l'indicatore Envelopes e ne restituisce il valore. iEnvelopes |
double iEnvelopesOnArray(double array[], int total, int ma_period, int ma_method, int ma_shift, double deviation, int mode, int shift) | - | iEnvelopesOnArray Calcolo dell'indicatore Envelopes su dati archiviati in un array numerico. |
double iForce(string symbol, int timeframe, int period, int ma_method, int applied_price, int shift) | double iForceMQL4(string symbol, int tf, int period, int method, int price, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); ENUM_MA_METHOD ma_method=MethodMigrate(method); int handle=iForce(symbol,timeframe,period,ma_method,VOLUME_TICK); if(handle<0) { Print("The iForce object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iForce Calcola l'indice Force e ne restituisce il valore. iForce |
double iFractals(string symbol, int timeframe, int mode, int shift) | double iFractalsMQL4(string symbol, int tf, int mode, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); int handle=iFractals(symbol,timeframe); if(handle<0) { Print("The iFractals object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,mode-1,shift)); } | iFractals Calcola i Frattali e ne restituisce il valore. iFractals |
double iGator(string symbol, int timeframe, int jaw_period, int jaw_shift, int teeth_period, int teeth_shift, int lips_period, int lips_shift, int ma_method, int applied_price, int mode, int shift) | double iGatorMQL4(string symbol, int tf, int jaw_period, int jaw_shift, int teeth_period, int teeth_shift, int lips_period, int lips_shift, int method, int price, int mode, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); ENUM_MA_METHOD ma_method=MethodMigrate(method); ENUM_APPLIED_PRICE applied_price=PriceMigrate(price); int handle=iGator(symbol,timeframe,jaw_period,jaw_shift, teeth_period,teeth_shift, lips_period,lips_shift, ma_method,applied_price); if(handle<0) { Print("The iGator object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,mode-1,shift)); } | iGator Calcolo dell'oscillatore Gator. iGator |
double iIchimoku(string symbol, int timeframe, int tenkan_sen, int kijun_sen, int senkou_span_b, int mode, int shift) | double iIchimokuMQL4(string symbol, int tf, int tenkan_sen, int kijun_sen, int senkou_span_b, int mode, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); int handle=iIchimoku(symbol,timeframe, tenkan_sen,kijun_sen,senkou_span_b); if(handle<0) { Print("The iIchimoku object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,mode-1,shift)); } | iIchimoku Calcola l'Ichimoku Kinko Hyo e ne restituisce il valore. iIchimoku |
double iBWMFI(string symbol, int timeframe, int shift) | double iBWMFIMQL4(string symbol, int tf, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); int handle=(int)iBWMFI(symbol,timeframe,VOLUME_TICK); if(handle<0) { Print("The iBWMFI object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iBWMFI Calcola l'indice di Facilitazione del Mercato di Bill Williams e ne restituisce il valore. iBWMFI |
double iMomentum(string symbol, int timeframe, int period, int applied_price, int shift) | double iMomentumMQL4(string symbol, int tf, int period, int price, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); ENUM_APPLIED_PRICE applied_price=PriceMigrate(price); int handle=iMomentum(symbol,timeframe,period,applied_price); if(handle<0) { Print("The iMomentum object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iMomentum Calcola l'indicatore Momentum e ne restituisce il valore. iMomentum |
double iMomentumOnArray(double array[], int total, int period, int shift) | - | iMomentumOnArray Calcolo dell'indicatore Momentum sui dati memorizzati in un array numerico. |
double iMFI(string symbol, int timeframe, int period, int shift) | double iMFIMQL4(string symbol, int tf, int period, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); int handle=(int)iMFI(symbol,timeframe,period,VOLUME_TICK); if(handle<0) { Print("The iMFI object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iMFI Calcola l'indice del flusso di Denaro e ne restituisce il valore. iMFI |
double iMA(string symbol, int timeframe, int period, int ma_shift, int ma_method, int applied_price, int shift) | double iMAMQL4(string symbol, int tf, int period, int ma_shift, int method, int price, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); ENUM_MA_METHOD ma_method=MethodMigrate(method); ENUM_APPLIED_PRICE applied_price=PriceMigrate(price); int handle=iMA(symbol,timeframe,period,ma_shift, ma_method,applied_price); if(handle<0) { Print("The iMA object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iMA Calcola l'indicatore della Media Mobile e ne restituisce il valore. iMA |
double iMAOnArray(double array[], int total, int period, int ma_shift, int ma_method, int shift) | double iMAOnArrayMQL4(double &array[], int total, int period, int ma_shift, int ma_method, int shift) { double buf[],arr[]; if(total==0) total=ArraySize(array); if(total>0 && total<=period) return(0); if(shift>total-period-ma_shift) return(0); switch(ma_method) { case MODE_SMA : { total=ArrayCopy(arr,array,0,shift+ma_shift,period); if(ArrayResize(buf,total)<0) return(0); double sum=0; int i,pos=total-1; for(i=1;i<period;i++,pos--) sum+=arr[pos]; while(pos>=0) { sum+=arr[pos]; buf[pos]=sum/period; sum-=arr[pos+period-1]; pos--; } return(buf[0]); } case MODE_EMA : { if(ArrayResize(buf,total)<0) return(0); double pr=2.0/(period+1); int pos=total-2; while(pos>=0) { if(pos==total-2) buf[pos+1]=array[pos+1]; buf[pos]=array[pos]*pr+buf[pos+1]*(1-pr); pos--; } return(buf[shift+ma_shift]); } case MODE_SMMA : { if(ArrayResize(buf,total)<0) return(0); double sum=0; int i,k,pos; pos=total-period; while(pos>=0) { if(pos==total-period) { for(i=0,k=pos;i<period;i++,k++) { sum+=array[k]; buf[k]=0; } } else sum=buf[pos+1]*(period-1)+array[pos]; buf[pos]=sum/period; pos--; } return(buf[shift+ma_shift]); } case MODE_LWMA : { if(ArrayResize(buf,total)<0) return(0); double sum=0.0,lsum=0.0; double price; int i,weight=0,pos=total-1; for(i=1;i<=period;i++,pos--) { price=array[pos]; sum+=price*i; lsum+=price; weight+=i; } pos++; i=pos+period; while(pos>=0) { buf[pos]=sum/weight; if(pos==0) break; pos--; i--; price=array[pos]; sum=sum-lsum+price*period; lsum-=array[i]; lsum+=price; } return(buf[shift+ma_shift]); } default: return(0); } return(0); } | |
double iOsMA(string symbol, int timeframe, int fast_ema_period, int slow_ema_period, int signal_period, int applied_price, int shift) | double iOsMAMQL4(string symbol, int tf, int fast_ema_period, int slow_ema_period, int signal_period, int price, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); ENUM_APPLIED_PRICE applied_price=PriceMigrate(price); int handle=iOsMA(symbol,timeframe, fast_ema_period,slow_ema_period, signal_period,applied_price); if(handle<0) { Print("The iOsMA object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iOsMA Calcola la Media Mobile dell'Oscillatore e ne restituisce il valore. iOsMA |
double iMACD(string symbol, int timeframe, int fast_ema_period, int slow_ema_period, int signal_period, int applied_price, int mode, int shift) | double iMACDMQL4(string symbol, int tf, int fast_ema_period, int slow_ema_period, int signal_period, int price, int mode, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); ENUM_APPLIED_PRICE applied_price=PriceMigrate(price); int handle=iMACD(symbol,timeframe, fast_ema_period,slow_ema_period, signal_period,applied_price); if(handle<0) { Print("The iMACD object is not created: Error ",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,mode,shift)); } | iMACD Calcola la convergenza/divergenza delle Medie Mobili e ne restituisce il valore. iMACD |
double iOBV(string symbol, int timeframe, int applied_price, int shift) | double iOBVMQL4(string symbol, int tf, int price, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); int handle=iOBV(symbol,timeframe,VOLUME_TICK); if(handle<0) { Print("The iOBV object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iOBV Calcola l'indicatore On Balance Volume e ne restituisce il valore. iOBV |
double iSAR(string symbol, int timeframe, double step, double maximum, int shift) | double iSARMQL4(string symbol, int tf, double step, double maximum, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); int handle=iSAR(symbol,timeframe,step,maximum); if(handle<0) { Print("The iSAR object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iSAR Calcola il sistema Parabolic Stop e Reverse e ne restituisce il valore. iSAR |
double iRSI(string symbol, int timeframe, int period, int applied_price, int shift) | double iRSIMQL4(string symbol, int tf, int period, int price, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); ENUM_APPLIED_PRICE applied_price=PriceMigrate(price); int handle=iRSI(symbol,timeframe,period,applied_price); if(handle<0) { Print("The iRSI object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iRSI Calcola il Relative strength index e ne restituisce il valore. iRSI |
double iRSIOnArray(double array[], int total, int period, int shift) | - | iRSIOnArray Calcolo del Relative Strength Index sui dati memorizzati in un array numerico. |
double iRVI(string symbol, int timeframe, int period, int mode, int shift) | double iRVIMQL4(string symbol, int tf, int period, int mode, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); int handle=iRVI(symbol,timeframe,period); if(handle<0) { Print("The iRVI object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,mode,shift)); } | iRVI Calcola il Relative Vigor index e ne restituisce il valore. iRVI |
double iStdDev(string symbol, int timeframe, int ma_period, int ma_shift, int ma_method, int applied_price, int shift) | double iStdDevMQL4(string symbol, int tf, int ma_period, int ma_shift, int method, int price, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); ENUM_MA_METHOD ma_method=MethodMigrate(method); ENUM_APPLIED_PRICE applied_price=PriceMigrate(price); int handle=iStdDev(symbol,timeframe,ma_period,ma_shift, ma_method,applied_price); if(handle<0) { Print("The iStdDev object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iStdDev Calcola l'indicatore Deviazione Standard e ne restituisce il valore. iStdDev |
double iStdDevOnArray(double array[], int total, int ma_period, int ma_shift, int ma_method, int shift) | - | iStdDevOnArray Calcolo dell'indicatore Deviazione Standard sui dati memorizzati in un array numerico. |
double iStochastic(string symbol, int timeframe, int%Kperiod, int%Dperiod, int slowing, int method, int price_field, int mode, int shift) | double iStochasticMQL4(string symbol, int tf, int Kperiod, int Dperiod, int slowing, int method, int field, int mode, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); ENUM_MA_METHOD ma_method=MethodMigrate(method); ENUM_STO_PRICE price_field=StoFieldMigrate(field); int handle=iStochastic(symbol,timeframe,Kperiod,Dperiod, slowing,ma_method,price_field); if(handle<0) { Print("The iStochastic object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,mode,shift)); } | iStochastic Calcola l'oscillatore Stocastico e ne restituisce il valore. iStochastic |
double iWPR(string symbol, int timeframe, int period, int shift) | double iWPRMQL4(string symbol, int tf, int period, int shift) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); int handle=iWPR(symbol,timeframe,period); if(handle<0) { Print("The iWPR object is not created: Error",GetLastError()); return(-1); } else return(CopyBufferMQL4(handle,0,shift)); } | iWPR Calcola l'indicatore dell'intervallo percentuale di Larry William e ne restituisce il valore. iWPR |
18. Accesso alle Serie Temporali
MQL4 | MQL5 | Descrizione |
---|---|---|
int iBars(string symbol, int timeframe) | int iBarsMQL4(string symbol,int tf) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); return(Bars(symbol,timeframe)); } | int iBarsMQL4(string symbol,int tf) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); return(Bars(symbol,timeframe)); }Bars Restituisce il numero di barre nel grafico specificato. Bars |
int iBarShift(string symbol, int timeframe, datetime time, bool exact=false | int iBarShiftMQL4(string symbol, int tf, datetime time, bool exact=false) { if(time<0) return(-1); ENUM_TIMEFRAMES timeframe=TFMigrate(tf); datetime Arr[],time1; CopyTime(symbol,timeframe,0,1,Arr); time1=Arr[0]; if(CopyTime(symbol,timeframe,time,time1,Arr)>0) { if(ArraySize(Arr)>2) return(ArraySize(Arr)-1); if(time<time1) return(1); else return(0); } else return(-1); } | iBarShift Ricerca barra per orario di apertura. CopyTime, ArraySize |
double iClose(string symbol, int timeframe, int shift) | double iCloseMQL4(string symbol,int tf,int index){ if(index < 0) return(-1); double Arr[]; ENUM_TIMEFRAMES timeframe=TFMigrate(tf); if(CopyClose(symbol,timeframe, index, 1, Arr)>0) return(Arr[0]); else return(-1); } | Close Restituisce il valore Close per la barra del simbolo indicato con timeframe e shift. Se la cronologia locale è vuota (non caricata), la funzione restituisce 0. CopyRates, MqlRates |
double iHigh(string symbol, int timeframe, int shift) | double iHighMQL4(string symbol,int tf,int index) { if(index < 0) return(-1); double Arr[]; ENUM_TIMEFRAMES timeframe=TFMigrate(tf); if(CopyHigh(symbol,timeframe, index, 1, Arr)>0) return(Arr[0]); else return(-1); } | High Restituisce il valore High per la barra del simbolo indicato con timeframe e shift. Se la cronologia locale è vuota (non caricata), la funzione restituisce 0. CopyRates, MqlRates |
int iHighest(string symbol, int timeframe, int type, int count=WHOLE_ARRAY, int start=0) | int iHighestMQL4(string symbol, int tf, int type, int count=WHOLE_ARRAY, int start=0) { if(start<0) return(-1); ENUM_TIMEFRAMES timeframe=TFMigrate(tf); if(count<=0) count=Bars(symbol,timeframe); if(type<=MODE_OPEN) { double Open[]; ArraySetAsSeries(Open,true); CopyOpen(symbol,timeframe,start,count,Open); return(ArrayMaximum(Open,0,count)+start); } if(type==MODE_LOW) { double Low[]; ArraySetAsSeries(Low,true); CopyLow(symbol,timeframe,start,count,Low); return(ArrayMaximum(Low,0,count)+start); } if(type==MODE_HIGH) { double High[]; ArraySetAsSeries(High,true); CopyHigh(symbol,timeframe,start,count,High); return(ArrayMaximum(High,0,count)+start); } if(type==MODE_CLOSE) { double Close[]; ArraySetAsSeries(Close,true); CopyClose(symbol,timeframe,start,count,Close); return(ArrayMaximum(Close,0,count)+start); } if(type==MODE_VOLUME) { long Volume[]; ArraySetAsSeries(Volume,true); CopyTickVolume(symbol,timeframe,start,count,Volume); return(ArrayMaximum(Volume,0,count)+start); } if(type>=MODE_TIME) { datetime Time[]; ArraySetAsSeries(Time,true); CopyTime(symbol,timeframe,start,count,Time); return(ArrayMaximum(Time,0,count)+start); //--- } return(0); } | iHighest Restituisce lo spostamento del valore massimo su un numero specifico di periodi a seconda del tipo. CopyOpen, CopyLow, CopyHigh, CopyClose, CopyTickVolume, CopyTime, ArrayMaximum |
double iLow(string symbol, int timeframe, int shift) | double iLowMQL4(string symbol,int tf,int index) { if(index < 0) return(-1); double Arr[]; ENUM_TIMEFRAMES timeframe=TFMigrate(tf); if(CopyLow(symbol,timeframe, index, 1, Arr)>0) return(Arr[0]); else return(-1); } | iLow Restituisce il valore basso per la barra del simbolo indicato con intervallo e spostamento. Se la cronologia locale è vuota (non caricata), la funzione restituisce 0. CopyRates, MqlRates |
int iLowest(string symbol, int timeframe, int type, int count=WHOLE_ARRAY, int start=0) | int iLowestMQL4(string symbol, int tf, int type, int count=WHOLE_ARRAY, int start=0) { if(start<0) return(-1); ENUM_TIMEFRAMES timeframe=TFMigrate(tf); if(count<=0) count=Bars(symbol,timeframe); if(type<=MODE_OPEN) { double Open[]; ArraySetAsSeries(Open,true); CopyOpen(symbol,timeframe,start,count,Open); return(ArrayMinimum(Open,0,count)+start); } if(type==MODE_LOW) { double Low[]; ArraySetAsSeries(Low,true); CopyLow(symbol,timeframe,start,count,Low); return(ArrayMinimum(Low,0,count)+start); } if(type==MODE_HIGH) { double High[]; ArraySetAsSeries(High,true); CopyHigh(symbol,timeframe,start,count,High); return(ArrayMinimum(High,0,count)+start); } if(type==MODE_CLOSE) { double Close[]; ArraySetAsSeries(Close,true); CopyClose(symbol,timeframe,start,count,Close); return(ArrayMinimum(Close,0,count)+start); } if(type==MODE_VOLUME) { long Volume[]; ArraySetAsSeries(Volume,true); CopyTickVolume(symbol,timeframe,start,count,Volume); return(ArrayMinimum(Volume,0,count)+start); } if(type>=MODE_TIME) { datetime Time[]; ArraySetAsSeries(Time,true); CopyTime(symbol,timeframe,start,count,Time); return(ArrayMinimum(Time,0,count)+start); } //--- return(0); } | iLowest Restituisce lo spostamento del valore più basso su un numero specifico di periodi a seconda del tipo. CopyOpen, CopyLow, CopyHigh, CopyClose, CopyTickVolume, CopyTime, ArrayMinimum |
double iOpen(string symbol, int timeframe, int shift) | double iOpenMQL4(string symbol,int tf,int index) { if(index < 0) return(-1); double Arr[]; ENUM_TIMEFRAMES timeframe=TFMigrate(tf); if(CopyOpen(symbol,timeframe, index, 1, Arr)>0) return(Arr[0]); else return(-1); } | iOpen Restituisce il valore Open per la barra del simbolo indicato con intervallo e simbolo. Se la cronologia locale è vuota (non caricata), la funzione restituisce 0. CopyRates, MqlRates |
datetime iTime(string symbol, int timeframe, int shift) | datetime iTimeMQL4(string symbol,int tf,int index) { if(index < 0) return(-1); ENUM_TIMEFRAMES timeframe=TFMigrate(tf); datetime Arr[]; if(CopyTime(symbol, timeframe, index, 1, Arr)>0) return(Arr[0]); else return(-1); } | iTime Restituisce il valore del tempo per la barra del simbolo indicato con intervallo e spostamento. Se la cronologia locale è vuota (non caricata), la funzione restituisce 0. CopyRates, MqlRates |
double iVolume(string symbol, int timeframe, int shift) | int iVolumeMQL4(string symbol,int tf,int index) { if(index < 0) return(-1); long Arr[]; ENUM_TIMEFRAMES timeframe=TFMigrate(tf); if(CopyTickVolume(symbol, timeframe, index, 1, Arr)>0) return(Arr[0]); else return(-1); } | iVolume Restituisce il valore Tick Volume per la barra del simbolo indicato con intervallo e spostamento. Se la cronologia locale è vuota (non caricata), la funzione restituisce 0. CopyRates, MqlRates |
19. Operazioni col Grafico
MQL4 | MQL5 | Descrizione |
---|---|---|
void HideTestIndicators(bool hide) | - | HideTestIndicators La funzione imposta un flag che nasconde gli indicatori richiamati dall'Expert Advisor. |
int Period() | ENUM_TIMEFRAMES Period() | Period Restituisce la quantità di minuti che determinano il periodo utilizzato (intervallo di tempo del grafico). Periodo |
bool RefreshRates() | - | RefreshRates Aggiornamento dei dati in variabili predefinite e array di serie. |
string Symbol() | string Symbol() | Symbol Restituisce una stringa di testo con il nome dello strumento finanziario corrente. Symbol |
int WindowBarsPerChart() | int ChartGetInteger(0,CHART_VISIBLE_BARS,0) | WindowBarsPerChart La funzione restituisce la quantità di barre visibili sul grafico. ChartGetInteger |
string WindowExpertName() | string MQLInfoString(MQL5_PROGRAM_NAME) | WindowExpertName Restituisce il nome dell'expert eseguito, dello script, dell'indicatore personalizzato o della libreria, a seconda del programma MQL4 da cui è stata chiamata questa funzione. MQLInfoString |
int WindowFind(string name) | int WindowFindMQL4(string name) { int window=-1; if((ENUM_PROGRAM_TYPE)MQLInfoInteger(MQL5_PROGRAM_TYPE)==PROGRAM_INDICATOR) { window=ChartWindowFind(); } else { window=ChartWindowFind(0,name); if(window==-1) Print(__FUNCTION__+"(): Error = ",GetLastError()); } return(window); } | WindowFind Se è stato trovato un indicatore con nome, la funzione restituisce l'indice della finestra contenente questo indicatore specificato, altrimenti restituisce -1. ChartWindowFind, MQLInfoInteger |
int WindowFirstVisibleBar() | int ChartGetInteger(0,CHART_FIRST_VISIBLE_BAR,0) | WindowFirstVisibleBar La funzione restituisce il primo numero di barra visibile nella finestra del grafico corrente. ChartGetInteger |
int WindowHandle(string symbol, int timeframe) | int WindowHandleMQL4(string symbol, int tf) { ENUM_TIMEFRAMES timeframe=TFMigrate(tf); long currChart,prevChart=ChartFirst(); int i=0,limit=100; while(i<limit) { currChart=ChartNext(prevChart); if(currChart<0) break; if(ChartSymbol(currChart)==symbol && ChartPeriod(currChart)==timeframe) return((int)currChart); prevChart=currChart; i++; } return(0); } | WindowHandle Restituisce l'handle della finestra di sistema del grafico specificato. ChartFirst, ChartNext, ChartSymbol, ChartPeriod |
bool WindowIsVisible(int index) | bool ChartGetInteger(0,CHART_WINDOW_IS_VISIBLE,index) | WindowIsVisible Restituisce TRUE se la sottofinestra del grafico è visibile, altrimenti restituisce FALSE. ChartGetInteger |
int WindowOnDropped() | int ChartWindowOnDropped() | WindowOnDropped Restituisce l'indice della finestra in cui è stato rilasciato l'expert, l'indicatore personalizzato o lo script. ChartWindowOnDropped |
double WindowPriceMax(int index=0) | double ChartGetDouble(0,CHART_PRICE_MAX,index) | WindowPriceMax Restituisce il valore massimo della scala verticale della sottofinestra specificata del grafico corrente (finestra del grafico 0-principale, le sottofinestre degli indicatori sono numerate a partire da 1). ChartGetDouble |
double WindowPriceMin(int index=0) | double ChartGetDouble(0,CHART_PRICE_MIN,index) | WindowPriceMin Restituisce il valore minimo della scala verticale della sottofinestra specificata del grafico corrente (finestra del grafico 0-principale, le sottofinestre degli indicatori sono numerate a partire da 1). ChartGetDouble |
double WindowPriceOnDropped() | double ChartPriceOnDropped() | WindowPriceOnDropped Restituisce la parte del prezzo del punto del grafico in cui è stato rilasciato l'expert o lo script. ChartPriceOnDropped |
void WindowRedraw() | void ChartRedraw(0) | WindowRedraw Ridisegna forzatamente il grafico corrente. ChartRedraw |
bool WindowScreenShot(string filename, int size_x, int size_y, int start_bar=-1, int chart_scale=-1, int chart_mode=-1) | bool WindowScreenShotMQL4(string filename, int size_x, int size_y, int start_bar=-1, int chart_scale=-1, int chart_mode=-1) { if(chart_scale>0 && chart_scale<=5) ChartSetInteger(0,CHART_SCALE,chart_scale); switch(chart_mode) { case 0: ChartSetInteger(0,CHART_MODE,CHART_BARS); case 1: ChartSetInteger(0,CHART_MODE,CHART_CANDLES); case 2: ChartSetInteger(0,CHART_MODE,CHART_LINE); } if(start_bar<0) return(ChartScreenShot(0,filename,size_x,size_y,ALIGN_RIGHT)); else return(ChartScreenShot(0,filename,size_x,size_y,ALIGN_LEFT)); } | WindowScreenShot Salva la schermata del grafico corrente come file GIF. ChartSetInteger, ChartScreenShot |
datetime WindowTimeOnDropped() | datetime ChartTimeOnDropped() | WindowTimeOnDropped Restituisce la parte temporale del punto del grafico in cui è stato rilasciato l'expert o lo script. ChartTimeOnDropped |
int WindowsTotal() | int ChartGetInteger(0,CHART_WINDOWS_TOTAL) | WindowsTotal Restituisce il conteggio delle finestre degli indicatori sul grafico (incluso il grafico principale). ChartGetInteger |
int WindowXOnDropped() | int ChartXOnDropped() | WindowXOnDropped Restituisce il valore in pixel sull'asse X per il punto dell'area client della finestra del grafico in cui è stato rilasciato l'expert o lo script. ChartXOnDropped |
int WindowYOnDropped() | int ChartYOnDropped() | WindowYOnDropped Restituisce il valore in pixel sull'asse Y per il punto dell'area client della finestra del grafico in cui è stato rilasciato l'expert o lo script. ChartYOnDropped |
Conclusione
- Non abbiamo considerato le funzioni di trading, perché in MQL5 il concetto è diverso e dovrebbe essere usato l'originale! È possibile convertirli, ma la logica di trading dovrebbe essere cambiata. In altre parole, non ha senso convertirli.
- La conversione dei programmi da un linguaggio all'altro è sempre associata alla perdita di funzionalità e prestazioni. Utilizza, quindi, questa guida per la ricerca rapida delle funzioni analoghe.
- Ho in programma di sviluppare l'emulatore MQL4, che ti consentirà di eseguire i tuoi programmi MQL4 nel nuovo client terminal MetaTrader 5.
Ringraziamenti: keiji, A. Williams.
Tradotto dal russo da MetaQuotes Ltd.
Articolo originale: https://www.mql5.com/ru/articles/81





- App di trading gratuite
- Oltre 8.000 segnali per il copy trading
- Notizie economiche per esplorare i mercati finanziari
Accetti la politica del sito e le condizioni d’uso