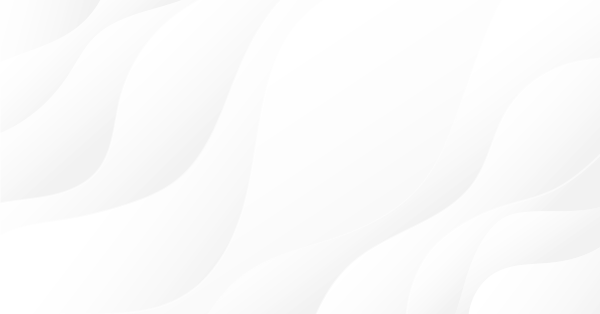
Guia prático do MQL5: Monitoramento de múltiplos períodos de tempo em uma única janela
Introdução
Ao escolher a direção para abertura de uma posição, um gráfico de preço com vários períodos de tempo exibidos no mesmo momento pode ser bastante útil. O terminal do cliente MetaTrader 5 fornece 21 períodos de tempo para análise. Você pode tirar proveito de objetos gráficos especiais, os quais você pode colocar no gráfico existente e definir o símbolo, período de tempo e algumas outras propriedades ali mesmo. Você pode adicionar qualquer número de tais objetos gráficos, no entanto, seria bastante inconveniente e demorado se feito manualmente. Além disso, nem todas as propriedades gráficas podem ser ajustadas no modo manual.
Neste artigo, vamos examinar mais detalhadamente tais objetos gráficos. Para fins ilustrativos, criaremos um indicador com controles (botões), que nos permitirá definir vários objetos gráficos em uma sub-janela ao mesmo tempo. Além disso, os objetos gráficos se encaixarão com precisão na sub-janela e serão ajustados automaticamente quando o gráfico principal ou a janela do terminal forem redimensionados.
Além de botões para adição de objetos gráficos, teremos também botões para ativar/desativar algumas das propriedades do gráfico, incluindo aquelas que só podem ser modificadas por meio de programação.
Desenvolvimento
Você pode adicionar manualmente um objeto gráfico usando o menu Inserir->Objetos->Objetos gráficos->Gráfico. Por exemplo, esta é a forma como os objetos com períodos de tempo H4 e D1 são exibidos no gráfico de 1 hora:
Fig. 1. Objetos gráficos
Pela modificação dos parâmetros dos objetos, você pode gerenciar somente um conjunto limitado de propriedades:
Fig. 2. Propriedades dos objetos gráficos
No entanto, parâmetros como os níveis dos preços de venda e compra, o recuo da borda direita do gráfico, os níveis de negociação, etc. só podem ser apresentados quando devidamente programados.
Assim, começamos o desenvolvimento do indicador. Vamos dizer que o nomeamos de ChartObjects (título provisório do artigo). Usando o assistente do MQL5, crie um modelo para o indicador no MetaEditor. Ao selecionar os manipuladores de eventos do programa do indicador personalizado, opte por aqueles como mostrado na captura de imagem abaixo:
Fig. 3. Manipuladores de evento do indicador
Quando aberto no MetaEditor, o código-fonte modelo será como um resultado parecido com seguinte:
//+------------------------------------------------------------------+ //| ChartObjects.mq5 | //| Copyright 2013, MetaQuotes Software Corp. | //| http://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2013, MetaQuotes Software Corp." #property link "http://www.mql5.com" #property version "1.00" #property indicator_chart_window //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- indicator buffers mapping //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const int begin, const double &price[]) { //--- //--- return value of prev_calculated for next call return(rates_total); } //+------------------------------------------------------------------+ //| TradeTransaction function | //+------------------------------------------------------------------+ void OnTradeTransaction(const MqlTradeTransaction& trans, const MqlTradeRequest& request, const MqlTradeResult& result) { //--- } //+------------------------------------------------------------------+ //| ChartEvent function | //+------------------------------------------------------------------+ void OnChartEvent(const int id, const long &lparam, const double &dparam, const string &sparam) { //--- } //+------------------------------------------------------------------+
Basicamente não precisaremos da função OnCalculate() nesta implementação, mas é impossível compilar o indicador sem a mesma. Além disso, vamos precisar de uma das principais funções - OnDeinit(). Ela monitorará a exclusão do programa a partir do gráfico. Após o processamento primário do modelo, temos o seguinte códigofonte:
//+------------------------------------------------------------------+ //| ChartObjects.mq5 | //| Copyright 2013, MetaQuotes Software Corp. | //| http://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2013, MetaQuotes Software Corp." #property link "http://www.mql5.com" #property version "1.00" //--- #property indicator_chart_window // Indicator is in the main window #property indicator_plots 0 // Zero plotting series //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- Set the short name for the indicator IndicatorSetString(INDICATOR_SHORTNAME,"TimeFramesPanel"); //--- Initialization completed successfully return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Indicator deinitialization | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- If the indicator has been deleted from the chart if(reason==REASON_REMOVE) { } } //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const int begin, const double &price[]) { //--- //--- return value of prev_calculated for next call return(rates_total); } //+------------------------------------------------------------------+ //| ChartEvent function | //+------------------------------------------------------------------+ void OnChartEvent(const int id, const long &lparam, const double &dparam, const string &sparam) { //--- } //+------------------------------------------------------------------+
Agora, precisamos criar um indicador que será utilizado como armazenamento (sub-janela) para objetos gráficos. Ele será basicamente um indicador simbólico. Vamos nomeá-lo de SubWindow. Seu código é fornecido abaixo:
//+------------------------------------------------------------------+ //| SubWindow.mq5 | //| Copyright 2013, MetaQuotes Software Corp. | //| http://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2013, MetaQuotes Software Corp." #property link "http://www.mql5.com" #property version "1.00" //--- #property indicator_chart_window // Indicator is in the subwindow #property indicator_plots 0 // Zero plotting series //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- Set the short name for the indicator IndicatorSetString(INDICATOR_SHORTNAME,"SubWindow"); //--- Initialization completed successfully return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const int begin, const double &price[]) { //--- //--- return value of prev_calculated for next call return(rates_total); } //+------------------------------------------------------------------+
O indicador SubWindow.ex5 será armazenado como um recurso dentro de ChartObjects.ex5 após a compilação. Assim, o desenvolvedor do programa acabará por ser capaz de fornecer ao usuário final apenas um arquivo em vez de dois.
Como já foi descrito no artigo anterior intitulado "guia prático do MQL5: Notificações sonoras para eventos de negociação do MetaTrader 5", arquivos de recursos podem ser incluídos em um programa usando a diretiva #resource. Precisamos adicionar a seguinte sequência de código no início do nosso programa ChartObjects:
//--- Include indicator resource #resource "\\Indicators\\SubWindow.ex5"
Em seguida, usando a diretiva #define, definimos o tamanho das matrizes que serão atribuídas aos controles:
//--- Number of time frame buttons #define TIMEFRAME_BUTTONS 21 //--- Number of buttons for chart object properties #define PROPERTY_BUTTONS 5
E, como de costume, declaramos as variáveis globais no início do programa:
//--- Location of the SubWindow indicator in the resource string subwindow_path ="::Indicators\\SubWindow.ex5"; int subwindow_number =-1; // Subwindow number int subwindow_handle =INVALID_HANDLE; // SubWindow indicator handle string subwindow_shortname ="SubWindow"; // Short name of the indicator //--- int chart_width =0; // Chart width int chart_height =0; // Chart height int chart_scale =0; // Chart scale //--- color cOffButtonFont =clrWhite; // Unclicked button text color color cOffButtonBackground =clrDarkSlateGray; // Unclicked button background color color cOffButtonBorder =clrLightGray; // Unclicked button border color //--- color cOnButtonFont =clrGold; // Clicked button text color color cOnButtonBackground =C'28,47,47'; // Clicked button background color color cOnButtonBorder =clrLightGray; // Clicked button border color
Isto é seguido pela declaração das matrizes para os botões do período de tempo:
//--- Array of object names for time frame buttons string timeframe_button_names[TIMEFRAME_BUTTONS]= { "button_M1","button_M2","button_M3","button_M4","button_M5","button_M6","button_M10", "button_M12","button_M15","button_M20","button_M30","button_H1","button_H2","button_H3", "button_H4","button_H6","button_H8","button_H12","button_D1","button_W1","button_MN" }; //--- Array of text displayed on time frame buttons string timeframe_button_texts[TIMEFRAME_BUTTONS]= { "M1","M2","M3","M4","M5","M6","M10", "M12","M15","M20","M30","H1","H2","H3", "H4","H6","H8","H12","D1","W1","MN" }; //--- Array of time frame button states bool timeframe_button_states[TIMEFRAME_BUTTONS]={false};
Matrizes de botões para controle das propriedades do objeto gráfico:
//--- Array of object names for buttons of chart properties string property_button_names[PROPERTY_BUTTONS]= { "property_button_date","property_button_price", "property_button_ohlc","property_button_askbid", "property_button_trade_levels" }; //--- Array of text displayed on buttons of chart properties string property_button_texts[PROPERTY_BUTTONS]= { "Date","Price","OHLC","Ask / Bid","Trade Levels" }; //--- Array of states for buttons of chart properties bool property_button_states[PROPERTY_BUTTONS]={false}; //--- Array of sizes for buttons of chart properties int property_button_widths[PROPERTY_BUTTONS]= { 66,68,66,100,101 };
E, finalmente, temos uma matriz de nomes de objetos gráficos:
//--- Array of chart object names string chart_object_names[TIMEFRAME_BUTTONS]= { "chart_object_m1","chart_object_m2","chart_object_m3","chart_object_m4","chart_object_m5","chart_object_m6","chart_object_m10", "chart_object_m12","chart_object_m15","chart_object_m20","chart_object_m30","chart_object_h1","chart_object_h2","chart_object_h3", "chart_object_h4","chart_object_h6","chart_object_h8","chart_object_h12","chart_object_d1","chart_object_w1","chart_object_mn" };
Antes de passarmos às funções, que têm a ver com a interação dos objetos gráficos, vamos primeiro escrever as que criam esses objetos no gráfico. Em nosso programa, vamos precisar de dois tipos de objetos gráficos: OBJ_BUTTON e OBJ_CHART.
Os botões serão criados pela função CreateButton():
//+------------------------------------------------------------------+ //| Creating the Button object | //+------------------------------------------------------------------+ void CreateButton(long chart_id, // chart id int window_number, // window number string name, // object name string text, // displayed name ENUM_ANCHOR_POINT anchor, // anchor point ENUM_BASE_CORNER corner, // chart corner string font_name, // font int font_size, // font size color font_color, // font color color background_color, // background color color border_color, // border color int x_size, // width int y_size, // height int x_distance, // X-coordinate int y_distance, // Y-coordinate long z_order) // Z-order { //--- If the object has been created successfully if(ObjectCreate(chart_id,name,OBJ_BUTTON,window_number,0,0)) { // set its properties ObjectSetString(chart_id,name,OBJPROP_TEXT,text); // setting name ObjectSetString(chart_id,name,OBJPROP_FONT,font_name); // setting font ObjectSetInteger(chart_id,name,OBJPROP_COLOR,font_color); // setting font color ObjectSetInteger(chart_id,name,OBJPROP_BGCOLOR,background_color); // setting background color ObjectSetInteger(chart_id,name,OBJPROP_BORDER_COLOR,border_color); // setting border color ObjectSetInteger(chart_id,name,OBJPROP_ANCHOR,anchor); // setting anchor point ObjectSetInteger(chart_id,name,OBJPROP_CORNER,corner); // setting chart corner ObjectSetInteger(chart_id,name,OBJPROP_FONTSIZE,font_size); // setting font size ObjectSetInteger(chart_id,name,OBJPROP_XSIZE,x_size); // setting width ObjectSetInteger(chart_id,name,OBJPROP_YSIZE,y_size); // setting height ObjectSetInteger(chart_id,name,OBJPROP_XDISTANCE,x_distance); // setting X-coordinate ObjectSetInteger(chart_id,name,OBJPROP_YDISTANCE,y_distance); // setting Y-coordinate ObjectSetInteger(chart_id,name,OBJPROP_SELECTABLE,false); // object is not available for selection ObjectSetInteger(chart_id,name,OBJPROP_STATE,false); // button state (clicked/unclicked) ObjectSetInteger(chart_id,name,OBJPROP_ZORDER,z_order); // Z-order for getting the click event ObjectSetString(chart_id,name,OBJPROP_TOOLTIP,"\n"); // no tooltip } }
Por conseguinte, a criação de um gráfico em uma sub-janela será realizada pela função CreateChartInSubwindow():
//+------------------------------------------------------------------+ //| Creating a chart object in a subwindow | //+------------------------------------------------------------------+ void CreateChartInSubwindow(int window_number, // subwindow number int x_distance, // X-coordinate int y_distance, // Y-coordinate int x_size, // width int y_size, // height string name, // object name string symbol, // symbol ENUM_TIMEFRAMES timeframe, // time frame int subchart_scale, // bar scale bool show_dates, // show date scale bool show_prices, // show price scale bool show_ohlc, // show OHLC prices bool show_ask_bid, // show ask/bid levels bool show_levels, // show trade levels string tooltip) // tooltip { //--- If the object has been created successfully if(ObjectCreate(0,name,OBJ_CHART,window_number,0,0)) { //--- Set the properties of the chart object ObjectSetInteger(0,name,OBJPROP_CORNER,CORNER_LEFT_UPPER); // chart corner ObjectSetInteger(0,name,OBJPROP_XDISTANCE,x_distance); // X-coordinate ObjectSetInteger(0,name,OBJPROP_YDISTANCE,y_distance); // Y-coordinate ObjectSetInteger(0,name,OBJPROP_XSIZE,x_size); // width ObjectSetInteger(0,name,OBJPROP_YSIZE,y_size); // height ObjectSetInteger(0,name,OBJPROP_CHART_SCALE,subchart_scale); // bar scale ObjectSetInteger(0,name,OBJPROP_DATE_SCALE,show_dates); // date scale ObjectSetInteger(0,name,OBJPROP_PRICE_SCALE,show_prices); // price scale ObjectSetString(0,name,OBJPROP_SYMBOL,symbol); // symbol ObjectSetInteger(0,name,OBJPROP_PERIOD,timeframe); // time frame ObjectSetString(0,name,OBJPROP_TOOLTIP,tooltip); // tooltip ObjectSetInteger(0,name,OBJPROP_BACK,false); // object in the foreground ObjectSetInteger(0,name,OBJPROP_SELECTABLE,false); // object is not available for selection ObjectSetInteger(0,name,OBJPROP_COLOR,clrWhite); // white color //--- Get the chart object identifier long subchart_id=ObjectGetInteger(0,name,OBJPROP_CHART_ID); //--- Set the special properties of the chart object ChartSetInteger(subchart_id,CHART_SHOW_OHLC,show_ohlc); // OHLC ChartSetInteger(subchart_id,CHART_SHOW_TRADE_LEVELS,show_levels); // trade levels ChartSetInteger(subchart_id,CHART_SHOW_BID_LINE,show_ask_bid); // bid level ChartSetInteger(subchart_id,CHART_SHOW_ASK_LINE,show_ask_bid); // ask level ChartSetInteger(subchart_id,CHART_COLOR_LAST,clrLimeGreen); // color of the level of the last executed deal ChartSetInteger(subchart_id,CHART_COLOR_STOP_LEVEL,clrRed); // color of Stop order levels //--- Refresh the chart object ChartRedraw(subchart_id); } }
No código acima, definimos primeiro as propriedades do gráfico padrão para um objeto gráfico. Depois de obter o identificador do objeto gráfico, as propriedades especiais são definidas. Também é importante atualizar o objeto gráfico usando a função ChartRedraw(), com o identificador do objeto gráfico sendo passado para ele.
Vamos dividir a definição de controles entre duas funções: AddTimeframeButtons() e AddPropertyButtons():
//+------------------------------------------------------------------+ //| Adding time frame buttons | //+------------------------------------------------------------------+ void AddTimeframeButtons() { int x_dist =1; // Indent from the left side of the chart int y_dist =125; // Indent from the bottom of the chart int x_size =28; // Button width int y_size =20; // Button height //--- for(int i=0; i<TIMEFRAME_BUTTONS; i++) { //--- If 7 buttons have already been added to the same row, set the coordinates for the next row if(i%7==0) { x_dist=1; y_dist-=21; } //--- Add a time frame button CreateButton(0,0,timeframe_button_names[i],timeframe_button_texts[i], ANCHOR_LEFT_LOWER,CORNER_LEFT_LOWER,"Arial",8, cOffButtonFont,cOffButtonBackground,cOffButtonBorder, x_size,y_size,x_dist,y_dist,3); //--- Set the X-coordinate for the next button x_dist+=x_size+1; } } //+------------------------------------------------------------------+ //| Adding buttons of chart properties | //+------------------------------------------------------------------+ void AddPropertyButtons() { int x_dist =1; // Indent from the left side of the chart int y_dist =41; // Indent from the bottom of the chart int x_size =66; // Button width int y_size =20; // Button height //--- for(int i=0; i<PROPERTY_BUTTONS; i++) { //--- If the first three buttons have already been added, set the coordinates for the next row if(i==3) { x_dist=1; y_dist-=21; } //--- Add a button CreateButton(0,0,property_button_names[i],property_button_texts[i], ANCHOR_LEFT_LOWER,CORNER_LEFT_LOWER,"Arial",8, cOffButtonFont,cOffButtonBackground,cOffButtonBorder, property_button_widths[i],y_size,x_dist,y_dist,3); //--- Set the X-coordinate for the next button x_dist+=property_button_widths[i]+1; } }
Ao apagar o indicador do gráfico, também devemos excluir os objetos criados pelo programa. Para isso, vamos precisar das seguintes funções auxiliares:
//+------------------------------------------------------------------+ //| Deleting the panel with time frame buttons | //+------------------------------------------------------------------+ void DeleteTimeframeButtons() { for(int i=0; i<TIMEFRAME_BUTTONS; i++) DeleteObjectByName(timeframe_button_names[i]); } //+------------------------------------------------------------------+ //| Deleting the panel with buttons of chart properties | //+------------------------------------------------------------------+ void DeletePropertyButtons() { for(int i=0; i<PROPERTY_BUTTONS; i++) DeleteObjectByName(property_button_names[i]); } //+------------------------------------------------------------------+ //| Deleting objects by name | //+------------------------------------------------------------------+ void DeleteObjectByName(string object_name) { //--- If such object exists if(ObjectFind(ChartID(),object_name)>=0) { //--- Delete it or print the relevant error message if(!ObjectDelete(ChartID(),object_name)) Print("Error ("+IntegerToString(GetLastError())+") when deleting the object!"); } }
Agora, para garantir que o painel esteja definido no gráfico ao carregar o indicador, e que todos os objetos do painel sejam apagados ao excluir o indicador do gráfico, precisamos adicionar as seguintes sequências de código às funções do manipulador OnInit() e OnDeinit():
//+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- Add the panel with time frame buttons to the chart AddTimeframeButtons(); //--- Add the panel with buttons of chart properties to the chart AddPropertyButtons(); //--- Redraw the chart ChartRedraw(); //--- Initialization completed successfully return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Indicator deinitialization | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- If the indicator has been deleted from the chart if(reason==REASON_REMOVE) { //--- Delete buttons DeleteTimeframeButtons(); DeletePropertyButtons(); //--- Redraw the chart ChartRedraw(); } }
Se nós compilarmos o indicador agora e o anexarmos ao gráfico, veremos o painel como mostrado na captura de tela abaixo:
Fig. 4. O painel com os botões
Agora tudo está pronto a fim de começar a criar funções para a interação entre o usuário e o painel. Praticamente todos elas serão chamadas a partir da função principal OnChartEvent(). Neste artigo, vamos considerar dois eventos que serão tratados nesta função:
- CHARTEVENT_OBJECT_CLICK - evento do clique sobre um objeto gráfico.
- CHARTEVENT_CHART_CHANGE - evento de redimensionamento do gráfico ou modificação das propriedades gráficas usando as propriedades da janela de diálogo.
Vamos começar com o evento CHARTEVENT_OBJECT_CLICK. A função ChartEventObjectClick() que estamos prestes a escrever terá todos os argumentos da função OnChartEvent() (para outros eventos criaremos funções semelhantes):
//+------------------------------------------------------------------+ //| Event of the click on a graphical object | //+------------------------------------------------------------------+ bool ChartEventObjectClick(int id, long lparam, double dparam, string sparam) { //--- Click on a graphical object if(id==CHARTEVENT_OBJECT_CLICK) { //--- If a time frame button has been clicked, set/delete 'SubWindow' and a chart object if(ToggleSubwindowAndChartObject(sparam)) return(true); //--- If a button of chart properties has been clicked, set/delete the property in chart objects if(ToggleChartObjectProperty(sparam)) return(true); } //--- return(false); }
O código de função ChartEventObjectClick() é simples. O evento de clique do botão do painel é determinado utilizando o identificador. Então, a lógica de implementação está dividida em duas direções: manipulação do evento de clique nos botões de períodos de tempo ou o evento de clique nos botões de propriedades gráficas. O parâmetro de sequência sparam, que contém o nome do objeto esquerdo, é passado às funções correspondentes ToggleSubwindowAndChartObject() e ToggleChartObjectProperty().
Vamos dar uma olhada no códigofonte dessas funções. Vamos começar com ToggleSubwindowAndChartObject():
//+------------------------------------------------------------------+ //| Setting/deleting SubWindow and a chart object | //+------------------------------------------------------------------+ bool ToggleSubwindowAndChartObject(string clicked_object_name) { //--- Make sure that the click was on the time frame button object if(CheckClickOnTimeframeButton(clicked_object_name)) { //--- Check if the SubWindow exists subwindow_number=ChartWindowFind(0,subwindow_shortname); //--- If the SubWindow does not exist, set it if(subwindow_number<0) { //--- If the SubWindow is set if(AddSubwindow()) { //--- Add chart objects to it AddChartObjectsToSubwindow(clicked_object_name); return(true); } } //--- If the SubWindow exists if(subwindow_number>0) { //--- Add chart objects to it AddChartObjectsToSubwindow(clicked_object_name); return(true); } } //--- return(false); }
Você deve ser capaz de compreender facilmente a lógica da implementação usando os comentários apresentados no código acima. As sequências destacadas apresentam algumas funções personalizadas cujo código pode ser encontrado abaixo.
A função CheckClickOnTimeframeButton() retorna verdadeira se o botão clicado é associado ao painel dos períodos de tempo:
//+------------------------------------------------------------------+ //| Checking if a time frame button has been clicked | //+------------------------------------------------------------------+ bool CheckClickOnTimeframeButton(string clicked_object_name) { //--- Iterate over all time frame buttons and check the names for(int i=0; i<TIMEFRAME_BUTTONS; i++) { //--- Report the match if(clicked_object_name==timeframe_button_names[i]) return(true); } //--- return(false); }
Se o clique em um botão do período de tempo foi confirmado, então verificamos se a SubWindow (sub-janela) está atualmente adicionada ao gráfico principal. Se não, ela é definida usando a função AddSubwindow():
//+------------------------------------------------------------------+ //| Adding a subwindow for chart objects | //+------------------------------------------------------------------+ bool AddSubwindow() { //--- Get the "SubWindow" indicator handle subwindow_handle=iCustom(_Symbol,_Period,subwindow_path); //--- If the handle has been obtained if(subwindow_handle!=INVALID_HANDLE) { //--- Determine the number of windows in the chart for the subwindow number subwindow_number=(int)ChartGetInteger(0,CHART_WINDOWS_TOTAL); //--- Add the SubWindow to the chart if(!ChartIndicatorAdd(0,subwindow_number,subwindow_handle)) Print("Failed to add the SUBWINDOW indicator ! "); //--- The subwindow exists else return(true); } //--- There is no subwindow return(false); }
Em seguida, adicionamos objetos gráficos à sub-janela criada usando a função AddChartObjectsToSubwindow():
//+------------------------------------------------------------------+ //| Adding chart objects to the subwindow | //+------------------------------------------------------------------+ void AddChartObjectsToSubwindow(string clicked_object_name) { ENUM_TIMEFRAMES tf =WRONG_VALUE; // Time frame string object_name =""; // Object name string object_text =""; // Object text int x_distance =0; // X-coordinate int total_charts =0; // Total chart objects int chart_object_width =0; // Chart object width //--- Get the bar scale and SubWindow height/width chart_scale=(int)ChartGetInteger(0,CHART_SCALE); chart_width=(int)ChartGetInteger(0,CHART_WIDTH_IN_PIXELS,subwindow_number); chart_height=(int)ChartGetInteger(0,CHART_HEIGHT_IN_PIXELS,subwindow_number); //--- Get the number of chart objects in the SUBWINDOW total_charts=ObjectsTotal(0,subwindow_number,OBJ_CHART); //--- If there are no chart objects if(total_charts==0) { //--- Check if a time frame button has been clicked if(CheckClickOnTimeframeButton(clicked_object_name)) { //--- Initialize the array of time frame buttons InitializeTimeframeButtonStates(); //--- Get the time frame button text for the chart object tooltip object_text=ObjectGetString(0,clicked_object_name,OBJPROP_TEXT); //--- Get the time frame for the chart object tf=StringToTimeframe(object_text); //--- Set the chart object CreateChartInSubwindow(subwindow_number,0,0,chart_width,chart_height, "chart_object_"+object_text,_Symbol,tf,chart_scale, property_button_states[0],property_button_states[1], property_button_states[2],property_button_states[3], property_button_states[4],object_text); //--- Refresh the chart and exit ChartRedraw(); return; } } //--- If chart objects already exist in the SubWindow if(total_charts>0) { //--- Get the number of clicked time frame buttons and initialize the array of states int pressed_buttons_count=InitializeTimeframeButtonStates(); //--- If there are no clicked buttons, delete the SubWindow if(pressed_buttons_count==0) DeleteSubwindow(); //--- If the clicked buttons exist else { //--- Delete all chart objects from the subwindow ObjectsDeleteAll(0,subwindow_number,OBJ_CHART); //--- Get the width for chart objects chart_object_width=chart_width/pressed_buttons_count; //--- Iterate over all buttons in a loop for(int i=0; i<TIMEFRAME_BUTTONS; i++) { //--- If the button is clicked if(timeframe_button_states[i]) { //--- Get the time frame button text for the chart object tooltip object_text=ObjectGetString(0,timeframe_button_names[i],OBJPROP_TEXT); //--- Get the time frame for the chart object tf=StringToTimeframe(object_text); //--- Set the chart object CreateChartInSubwindow(subwindow_number,x_distance,0,chart_object_width,chart_height, chart_object_names[i],_Symbol,tf,chart_scale, property_button_states[0],property_button_states[1], property_button_states[2],property_button_states[3], property_button_states[4],object_text); //--- Determine the X-coordinate for the next chart object x_distance+=chart_object_width; } } } } //--- Refresh the chart ChartRedraw(); }
Os comentários detalhados fornecidos no código acima devem ajudá-lo a compreender o funcionamento da operação. As funções personalizadas que não nos deparamos antes estão destacadas.
A função InitializeTimeframeButtonStates() retorna o número dos botões de períodos de tempo clicados e inicializa a matriz correspondente dos estados. Ela também define cores, dependendo do estado do botão:
//+------------------------------------------------------------------+ //| Initializing array of time frame button states and | //| returning the number of clicked buttons | //+------------------------------------------------------------------+ int InitializeTimeframeButtonStates() { //--- Counter of the clicked time frame buttons int pressed_buttons_count=0; //--- Iterate over all time frame buttons and count the clicked ones for(int i=0; i<TIMEFRAME_BUTTONS; i++) { //--- If the button is clicked if(ObjectGetInteger(0,timeframe_button_names[i],OBJPROP_STATE)) { //--- Indicate it in the current index of the array timeframe_button_states[i]=true; //--- Set clicked button colors ObjectSetInteger(0,timeframe_button_names[i],OBJPROP_COLOR,cOnButtonFont); ObjectSetInteger(0,timeframe_button_names[i],OBJPROP_BGCOLOR,cOnButtonBackground); //--- Increase the counter by one pressed_buttons_count++; } else { //--- Set unclicked button colors ObjectSetInteger(0,timeframe_button_names[i],OBJPROP_COLOR,cOffButtonFont); ObjectSetInteger(0,timeframe_button_names[i],OBJPROP_BGCOLOR,cOffButtonBackground); //--- Indicate that the button is unclicked timeframe_button_states[i]=false; } } //--- Return the number of clicked buttons return(pressed_buttons_count); }
A função DeleteSubwindow() é muito simples: ela verifica a existência de sub-janela para gráficos e a exclui:
//+------------------------------------------------------------------+ //| Deleting subwindow for chart objects | //+------------------------------------------------------------------+ void DeleteSubwindow() { //--- If the SubWindow exists if((subwindow_number=ChartWindowFind(0,subwindow_shortname))>0) { //--- Delete it if(!ChartIndicatorDelete(0,subwindow_number,subwindow_shortname)) Print("Failed to delete the "+subwindow_shortname+" indicator!"); } }
Agora, devemos olhar as propriedades dos objetos gráficos. Em outras palavras, voltemos à função ChartEventObjectClick() e consideremos a função ToggleChartObjectProperty(). O nome do objeto clicado também é passado para ela.
//+------------------------------------------------------------------+ //| Setting/deleting chart object property | //| depending on the clicked button state | //+------------------------------------------------------------------+ bool ToggleChartObjectProperty(string clicked_object_name) { //--- If the "Date" button is clicked if(clicked_object_name=="property_button_date") { //--- If the button is clicked if(SetButtonColor(clicked_object_name)) ShowDate(true); //--- If the button is unclicked else ShowDate(false); //--- Refresh the chart and exit ChartRedraw(); return(true); } //--- If the "Price" button is clicked if(clicked_object_name=="property_button_price") { //--- If the button is clicked if(SetButtonColor(clicked_object_name)) ShowPrice(true); //--- If the button is unclicked else ShowPrice(false); //--- Refresh the chart and exit ChartRedraw(); return(true); } //--- If the "OHLC" button is clicked if(clicked_object_name=="property_button_ohlc") { //--- If the button is clicked if(SetButtonColor(clicked_object_name)) ShowOHLC(true); //--- If the button is unclicked else ShowOHLC(false); //--- Refresh the chart and exit ChartRedraw(); return(true); } //--- If the "Ask/Bid" button is clicked if(clicked_object_name=="property_button_askbid") { //--- If the button is clicked if(SetButtonColor(clicked_object_name)) ShowAskBid(true); //--- If the button is unclicked else ShowAskBid(false); //--- Refresh the chart and exit ChartRedraw(); return(true); } //--- If the "Trade Levels" button is clicked if(clicked_object_name=="property_button_trade_levels") { //--- If the button is clicked if(SetButtonColor(clicked_object_name)) ShowTradeLevels(true); //--- If the button is unclicked else ShowTradeLevels(false); //--- Refresh the chart and exit ChartRedraw(); return(true); } //--- No matches return(false); }
No código acima, o nome do objeto clicado está em sucessão comparado ao nome do objeto relacionado às propriedades gráficas. Se houver uma combinação, em seguida verificamos se o botão é clicado ou não na função SetButtonColor() e definimos as cores dos botões relevantes.
//+------------------------------------------------------------------+ //| Setting color of button elements depending on the state | //+------------------------------------------------------------------+ bool SetButtonColor(string clicked_object_name) { //--- If the button is clicked if(ObjectGetInteger(0,clicked_object_name,OBJPROP_STATE)) { //--- Set clicked button colors ObjectSetInteger(0,clicked_object_name,OBJPROP_COLOR,cOnButtonFont); ObjectSetInteger(0,clicked_object_name,OBJPROP_BGCOLOR,cOnButtonBackground); return(true); } //--- If the button is unclicked if(!ObjectGetInteger(0,clicked_object_name,OBJPROP_STATE)) { //--- Set unclicked button colors ObjectSetInteger(0,clicked_object_name,OBJPROP_COLOR,cOffButtonFont); ObjectSetInteger(0,clicked_object_name,OBJPROP_BGCOLOR,cOffButtonBackground); return(false); } //--- return(false); }
A função SetButtonColor() retorna o estado do botão. Dependendo desse atributo o programa informa a função relevante, que uma certa propriedade deve ser ativada ou desativada, em todos os objetos gráficos na SubWindow. Há uma função separada escrita para cada propriedade. Os códigos da função correspondentes são fornecidos abaixo:
//+------------------------------------------------------------------+ //| Enabling/disabling dates for all chart objects | //+------------------------------------------------------------------+ void ShowDate(bool state) { int total_charts =0; // Number of objects string chart_name =""; // Chart object name //--- Check if the SubWindow exists // If it exists, then if((subwindow_number=ChartWindowFind(0,subwindow_shortname))>0) { //--- Get the number of chart objects total_charts=ObjectsTotal(0,subwindow_number,OBJ_CHART); //--- Iterate over all chart objects in a loop for(int i=0; i<total_charts; i++) { //--- Get the chart object name chart_name=ObjectName(0,i,subwindow_number,OBJ_CHART); //--- Set the property ObjectSetInteger(0,chart_name,OBJPROP_DATE_SCALE,state); } //--- Set the button state to the relevant index if(state) property_button_states[0]=true; else property_button_states[0]=false; //--- Refresh the chart ChartRedraw(); } } //+------------------------------------------------------------------+ //| Enabling/disabling prices for all chart objects | //+------------------------------------------------------------------+ void ShowPrice(bool state) { int total_charts =0; // Number of objects string chart_name =""; // Chart object name //--- Check if the SubWindow exists // If it exists, then if((subwindow_number=ChartWindowFind(0,subwindow_shortname))>0) { //--- Get the number of chart objects total_charts=ObjectsTotal(0,subwindow_number,OBJ_CHART); //--- Iterate over all chart objects in a loop for(int i=0; i<total_charts; i++) { //--- Get the chart object name chart_name=ObjectName(0,i,subwindow_number,OBJ_CHART); //--- Set the property ObjectSetInteger(0,chart_name,OBJPROP_PRICE_SCALE,state); } //--- Set the button state to the relevant index if(state) property_button_states[1]=true; else property_button_states[1]=false; //--- Refresh the chart ChartRedraw(); } } //+------------------------------------------------------------------+ //| Enabling/disabling OHLC for all chart objects | //+------------------------------------------------------------------+ void ShowOHLC(bool state) { int total_charts =0; // Number of objects long subchart_id =0; // Chart object identifier string chart_name =""; // Chart object name //--- Check if the SubWindow exists // If it exists, then if((subwindow_number=ChartWindowFind(0,subwindow_shortname))>0) { //--- Get the number of chart objects total_charts=ObjectsTotal(0,subwindow_number,OBJ_CHART); //--- Iterate over all chart objects in a loop for(int i=0; i<total_charts; i++) { //--- Get the chart object name chart_name=ObjectName(0,i,subwindow_number,OBJ_CHART); //--- Get the chart object identifier subchart_id=ObjectGetInteger(0,chart_name,OBJPROP_CHART_ID); //--- Set the property ChartSetInteger(subchart_id,CHART_SHOW_OHLC,state); //--- Refresh the chart object ChartRedraw(subchart_id); } //--- Set the button state to the relevant index if(state) property_button_states[2]=true; else property_button_states[2]=false; //--- Refresh the chart ChartRedraw(); } } //+------------------------------------------------------------------+ //| Enabling/disabling Ask/Bid levels for all chart objects | //+------------------------------------------------------------------+ void ShowAskBid(bool state) { int total_charts =0; // Number of objects long subchart_id =0; // Chart object identifier string chart_name =""; // Chart object name //--- Check if the SubWindow exists // If it exists, then if((subwindow_number=ChartWindowFind(0,subwindow_shortname))>0) { //--- Get the number of chart objects total_charts=ObjectsTotal(0,subwindow_number,OBJ_CHART); //--- Iterate over all chart objects in a loop for(int i=0; i<total_charts; i++) { //--- Get the chart object name chart_name=ObjectName(0,i,subwindow_number,OBJ_CHART); //--- Get the chart object identifier subchart_id=ObjectGetInteger(0,chart_name,OBJPROP_CHART_ID); //--- Set the properties ChartSetInteger(subchart_id,CHART_SHOW_ASK_LINE,state); ChartSetInteger(subchart_id,CHART_SHOW_BID_LINE,state); //--- Refresh the chart object ChartRedraw(subchart_id); } //--- Set the button state to the relevant index if(state) property_button_states[3]=true; else property_button_states[3]=false; //--- Refresh the chart ChartRedraw(); } } //+------------------------------------------------------------------+ //| Enabling/disabling trade levels for all chart objects | //+------------------------------------------------------------------+ void ShowTradeLevels(bool state) { int total_charts =0; // Number of objects long subchart_id =0; // Chart object identifier string chart_name =""; // Chart object name //--- Check if the SubWindow exists // If it exists, then if((subwindow_number=ChartWindowFind(0,subwindow_shortname))>0) { //--- Get the number of chart objects total_charts=ObjectsTotal(0,subwindow_number,OBJ_CHART); //--- Iterate over all chart objects in a loop for(int i=0; i<total_charts; i++) { //--- Get the chart object name chart_name=ObjectName(0,i,subwindow_number,OBJ_CHART); //--- Get the chart object identifier subchart_id=ObjectGetInteger(0,chart_name,OBJPROP_CHART_ID); //--- Set the property ChartSetInteger(subchart_id,CHART_SHOW_TRADE_LEVELS,state); //--- Refresh the chart object ChartRedraw(subchart_id); } //--- Set the button state to the relevant index if(state) property_button_states[4]=true; else property_button_states[4]=false; //--- Refresh the chart ChartRedraw(); } }
Agora, todas as funções estão prontas para a interação com o painel. Nós só precisamos adicionar uma sequência de código à função principal OnChartEvent():
//+------------------------------------------------------------------+ //| ChartEvent function | //+------------------------------------------------------------------+ void OnChartEvent(const int id, const long &lparam, const double &dparam, const string &sparam) { //--- The CHARTEVENT_OBJECT_CLICK event if(ChartEventObjectClick(id,lparam,dparam,sparam)) return; }
Se o indicador é compilado e executado no gráfico agora, os objetos gráficos serão adicionados à sub-janela quando os botões dos períodos de tempo relevantes forem clicados. Além disso, se clicarmos em qualquer um dos botões das propriedades, seremos capazes de ver as alterações correspondentes nos objetos gráficos:
Fig. 5. Adicionando objetos gráficos com propriedades especificadas
No entanto, se a janela do gráfico ou a sub-janela é redimensionada, os tamanhos dos objetos gráficos não serão ajustados em conformidade. Então, é hora de ver o evento CHARTEVENT_CHART_CHANGE.
Assim como criamos a função ChartEventObjectClick() para rastrear o evento de "clique sobre um objeto gráfico", vamos agora escrever a função ChartEventChartChange():
//+------------------------------------------------------------------+ //| Event of modifying the chart properties | //+------------------------------------------------------------------+ bool ChartEventChartChange(int id, long lparam, double dparam, string sparam) { //--- Chart has been resized or the chart properties have been modified if(id==CHARTEVENT_CHART_CHANGE) { //--- If the SubWindow has been deleted (or does not exist), while the time frame buttons are clicked, // release all the buttons (reset) if(OnSubwindowDelete()) return(true); //--- Save the height and width values of the main chart and SubWindow, if it exists GetSubwindowWidthAndHeight(); //--- Adjust the sizes of chart objects AdjustChartObjectsSizes(); //--- Refresh the chart and exit ChartRedraw(); return(true); } //--- return(false); }
Se o programa estabeleceu que o tamanho ou propriedades gráficas principais foram modificados, primeiro usamos a função OnSubwindowDelete() para verificar se a SubWindow foi excluída. Se a sub-janela não pode ser encontrada, o painel é zerado.
//+------------------------------------------------------------------+ //| Response to Subwindow deletion | //+------------------------------------------------------------------+ bool OnSubwindowDelete() { //--- if there is no SubWindow if(ChartWindowFind(0,subwindow_shortname)<1) { //--- Reset the panel with time frame buttons AddTimeframeButtons(); ChartRedraw(); return(true); } //--- SubWindow exists return(false); }
Se a sub-janela está exatamente onde deveria, os valores de largura e altura da sub-janela são atribuídos às variáveis globais na função GetSubwindowWidthAndHeight():
//+------------------------------------------------------------------+ //| Saving the SubWindow height and width values | //+------------------------------------------------------------------+ void GetSubwindowWidthAndHeight() { //--- Check if there is a subwindow named SubWindow if((subwindow_number=ChartWindowFind(0,subwindow_shortname))>0) { // Get the subwindow height and width chart_height=(int)ChartGetInteger(0,CHART_HEIGHT_IN_PIXELS,subwindow_number); chart_width=(int)ChartGetInteger(0,CHART_WIDTH_IN_PIXELS,subwindow_number); } }
E, finalmente, os tamanhos dos objetos gráficos são ajustados na função AdjustChartObjectsSizes():
//+------------------------------------------------------------------+ //| Adjusting width of chart objects when modifying the window width | //+------------------------------------------------------------------+ void AdjustChartObjectsSizes() { int x_distance =0; // X-coordinate int total_objects =0; // Number of chart objects int chart_object_width =0; // Chart object width string object_name =""; // Object name ENUM_TIMEFRAMES TF =WRONG_VALUE; // Time frame //--- Get the SubWindow number if((subwindow_number=ChartWindowFind(0,subwindow_shortname))>0) { //--- Get the total number of chart objects total_objects=ObjectsTotal(0,subwindow_number,OBJ_CHART); //--- If there are no objects, delete the subwindow and exit if(total_objects==0) { DeleteSubwindow(); return; } //--- Get the width for chart objects chart_object_width=chart_width/total_objects; //--- Iterate over all chart objects in a loop for(int i=total_objects-1; i>=0; i--) { //--- Get the name object_name=ObjectName(0,i,subwindow_number,OBJ_CHART); //--- Set the chart object width and height ObjectSetInteger(0,object_name,OBJPROP_YSIZE,chart_height); ObjectSetInteger(0,object_name,OBJPROP_XSIZE,chart_object_width); //--- Set the chart object position ObjectSetInteger(0,object_name,OBJPROP_YDISTANCE,0); ObjectSetInteger(0,object_name,OBJPROP_XDISTANCE,x_distance); //--- Set the new X-coordinate for the next chart object x_distance+=chart_object_width; } } }
Para rastrear o evento de modificação do tamanho e das propriedades gráficas principais, a seguinte sequência deve ser adicionada à função OnChartEvent():
Depois de compilar o indicador e anexá-lo ao gráfico, você será capaz de ver que os objetos do gráfico são ajustados ao tamanho da sub-janela cada vez que a janela principal é redimensionada.
Conclusão
Vamos terminar o artigo aqui. Como trabalho de casa, tente implementar essa característica como ajuste de símbolos em objetos gráficos quando o símbolo no gráfico principal é modificado. Você também pode querer ter períodos de tempo nos objetos gráficos definidos em sucessão do menor ao maior (da esquerda para a direita). Esta possibilidade não foi implementada na versão do indicador descrita acima.
Você pode encontrar um vídeo demonstrando a implementação desses recursos na descrição do aplicativo já feito - TF PANEL. Os arquivos do código-fonte estão anexados ao artigo e disponíveis para download.
Traduzido do russo pela MetaQuotes Ltd.
Artigo original: https://www.mql5.com/ru/articles/749





- Aplicativos de negociação gratuitos
- 8 000+ sinais para cópia
- Notícias econômicas para análise dos mercados financeiros
Você concorda com a política do site e com os termos de uso