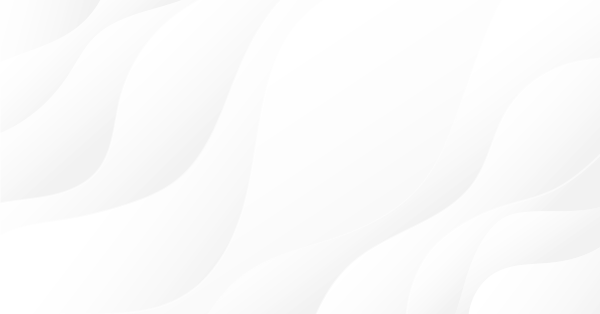
Better Programmer (Part 06): 9 habits that lead to effective coding
Introduction
Before starting to code, there are certain habits that we need to add to our coding that can help us stay focused all the coding time. Most of the time we might find ourselves coding but not realizing that we are not being effective due to various reasons, such as lack of focus, having no plan or having poor plan of execution.
Contents:
- Planning your project before coding
- Make code snippets collection
- Have a routine
- Schedule deep works
- Make small single purpose functions and test them
- Add comments to your future self
- Touch typing habit
- Using the best tools
- Do version control
Disclaimer:
All code fragments and coding examples used in this article are intended for educational purposes only. Some of them have not been carefully tested/debugged. They are used just to make my point clear. If you decide to use any of the code, you will be using it at your own risk.
01: Planning your project before coding
If you ask 100 programmers if they plan for their systems before starting to code, less than ten will tell you that they plan for their work before they start to write code on the keyboard.
This is the major problem that we have to address if we want to code effectively.
Can you jump on the public bus without a clue as to where you are going? Absolutely not.
Before you start typing code on the Keyboard, write down the kind of program you need, the tools, the libraries you might want to use inside, etc.
There is something called work specifications on the Freelance, which is the exact same thing as a work plan that every developer must have before starting to code anything.
You can use free programs such as Microsoft Word or WPS office to plan for your works if you don't have a pen and a notebook or a piece of paper. You can draw diagrams and Illustrations to describe everything you need.
I strongly agree with Charles Kettering when he said that "Problem well stated is half solved".
For example I just want to create a Simple Grid Expert Advisor with Labouchere money management systems in it.
Here is the simple work plan.
Note: All the initial values below can be optimized/are the input variables
Initial Values: Lotsize = 0.01; xgrid = 100; LotIncrement = 0.01;
My strategy | Lotsize Formula By Position Type | Grid Conditions (Strategy but in a more clear way) | Money Managements | Libraries |
---|---|---|---|---|
Condition 01: When there is no open position Open both buy and sell positions(single position for each type) | X = Lotsize + (LotIncrement * Number Of Position By Position Type) Example: BuyLotsize = 0.01 + ( 0.01 * Number Of Buy Positions); | buy condition happens when the number of buy positions is 0 (zero) or when there is already a buy position(s) and Bid price is below Last Buy Position Open Price minus xgrid points | StopLoss = 0 (by default) | Standard Positions Class Library |
Condition 02 (Sell Condition): If the Market price goes up by xgrid Points from the previous sell position open a Sell position with Lotsize equals to previous sell position Lotsize Plus LotIncrement value. | Sell condition happens when the number of sell positions is 0 (zero) or when there is already a sell position(s) and the Ask price is above Last sell position Open price plus xgrid points | TakeProfit = 100 (by defaul) | ||
Condition 03 (Buy condition): If the Market price goes down by xgrid Points from the previous Buy Position Open a buy Position with Lotsize equals to previous Lotsize Plus LotIncrement value. |
Just from the simple work plan, you can see that it is much easier to execute the work rather than starting to code with the vague mindset of figuring things out along the way. Better plan can help you Identify areas that you need to learn or remind your-self just in case you seem to forgot them.
Before starting to code make sure you have a well-explained plan for your work. The clearer the better
02: Make a collection of code snippets
If you find that you are defining functions or classes several times in multiple programs, such as Expert Advisors, indicators, or scripts, you probably need a collection of them so that you can re-use them several times without defining them over and over again. To be able to do this most effectively is through Object-Oriented Programming (OOP).
From the work plan example, we've seen that we need to create two functions in our EA.
- A function to count positions by their type
- A function to provide the last position Open price by position type
These functions are needed in almost every grid EA so we can create an Include(.mqh) file called gridmodule and store those two functions in it that we can include them in our main .mq5 file.
Inside gridmodule.mqh
//+------------------------------------------------------------------+ //| gridmodule.mqh | //| Copyright 2021, Omega Joctan | //| https://www.mql5.com/en/users/omegajoctan | //+------------------------------------------------------------------+ #property copyright "Copyright 2021, Omega Joctan" #property link "https://www.mql5.com/en/users/omegajoctan" //+------------------------------------------------------------------+ //| Libraries | //+------------------------------------------------------------------+ #include <Trade\PositionInfo.mqh> #include <Trade\SymbolInfo.mqh> CSymbolInfo m_symbol; CPositionInfo m_position; //+------------------------------------------------------------------+ //| DLL imports | //+------------------------------------------------------------------+ class CGrid { protected: int MagicNumber; public: CGrid(void); ~CGrid(void); void InitializeModule(int magic) { MagicNumber = magic; } double LastPositionOpenPrice(ENUM_POSITION_TYPE type); int CountPositions(ENUM_POSITION_TYPE type); }; //+------------------------------------------------------------------+ //| Constructor | //+------------------------------------------------------------------+ CGrid::CGrid(void) { } //+------------------------------------------------------------------+ //| Destructor | //+------------------------------------------------------------------+ CGrid :: ~CGrid(void) { } //+------------------------------------------------------------------+ //| Last Position Open Price By Position Type | //+------------------------------------------------------------------+ double CGrid::LastPositionOpenPrice(ENUM_POSITION_TYPE type) { double LastPrice = -1; ulong LastTime = 0; for (int i=PositionsTotal()-1; i>=0; i--) if (m_position.SelectByIndex(i)) if (m_position.Magic() == MagicNumber && m_position.Symbol()==Symbol() && m_position.PositionType()==type) { ulong positionTime = m_position.TimeMsc(); if ( positionTime > LastTime ) //FInd the latest position { LastPrice = m_position.PriceOpen(); LastTime = m_position.TimeMsc(); } } return LastPrice; } //+------------------------------------------------------------------+ //| Count Positions By Type | //+------------------------------------------------------------------+ int CGrid::CountPositions(ENUM_POSITION_TYPE type) { int counter = 0; //variable to store our positions number for (int i=PositionsTotal()-1; i>=0; i--) if (m_position.SelectByIndex(i)) // Select position by its index if (m_position.Magic() == MagicNumber && m_position.Symbol() == Symbol() && m_position.PositionType() == type) { counter++; } return counter; } //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+
There are a lot of benefits that you will obtain by doing so, as it makes it easy to debug your code: debugging has to be done in one place and it gives you less code to work with on your main .mq5 file.
03: Have a routine
Routines can be great to put your mind into the right coding mind-frame that can be great to improve your productivity in general. It is also the best way to help you stay committed and become a consistent day-by-day programmer.
For instance, you can have a routine to code every day from 08:00 am to 11:00 am.
When you stick to the same routine, after several weeks or months you will notice that your mind gets excited to start coding every time you step up on your PC during those hours.
Create a timetable that works best for you.
04: Schedule deep works
In these modern days, it is so easy to get distracted by a lot of things. It is hard to focus nowadays especially when things get tough when coding. Notice that it is only a few moments/hours of the day that we are being hyper-focused to the extent that we become creative.
Often our mind needs a long time of working free from distractions to get to that level. To be able to get to that state we need to schedule for days of long hours of coding non-stop.
It is through long hours of deep work that we can do the majority of the hard work which we may have faced while working on our regular routines. I recommend every coder to plan for certain days that you will work hard and free of distractions.
05: Make single-purpose functions and test them
Avoid having too many operations inside one function. Consider creating another function every time you realize that you have to code for a major operation.
Having too many loops in the Ontick function (or in any other major or minor function) will harm you in no time. It's like pulling out the pin of a hand grenade that you are keeping in your pockets.
Forcing simple algorithms to become complex by forcing all logic to appear in one place is one of the best ways to create bugs that will cost you money and the most valuable resource in your life, Time.
I believe coding has to be fun most of the time, so that we can be able to do it consistently. There is no fun in convoluted algorithms, no matter how experienced and clever is someone who's reading the code. Make sure each function does one operation and on top of that it should have an easy to read and understand what the function is all about function name.
Take a look at the function CountPositions with an argument for position type from the previous example:
int CGrid::CountPositions(ENUM_POSITION_TYPE type) { int counter = 0; //variable to store our positions number for (int i=PositionsTotal()-1; i>=0; i--) if (m_position.SelectByIndex(i)) // Select position by its index if (m_position.Magic() == MagicNumber && m_position.Symbol() == Symbol() && m_position.PositionType() == type) { counter++; } return counter; } //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+
Just by looking at the function name, everybody can tell that the function is all about counting positions by their type, and that's what has been done on the inside of the function, nothing more nothing less.
06: Add comments for your future self
Simple habit of adding comments to your code can be seen as a premature way of coding. But it can be a game-changer to those who know how to use it effectively.
input group "Money management" input double InitialLots = 0.01; //Initial position volume input int EquityUse = 50; // Percentage of equity to be used in all trading operations input group "Average True Range"; input int AtrBarSignal = 1; //Atr value will be calculation taken from this candle
Most of the variables, functions, and code snippets that you write now will be forgotten by you several weeks or even days from now. Without meaningful comments, you will be unfamiliar with the code you write earlier (Just think how stupid is that !!).
Adding comments in MQL5 will allow the IDE MetaEditor to help you remember it easier every time you want to use it.
Why make it harder for MetaEditor to help you? That doesn't make sense to me.
Keep in mind that, you are not alone in this career, so you always want to have the code that can be easily passed and used by other developers on the codebase or something and the only way you are going to do it is by having easy to read and understand code that is made possible by comments.
07: Touch typing habit
Let's not forget that to code effectively we need to become good writers on the keyboard. Every coder must strive to develop and improve their keyboard typing abilities. The best way for you to become good at typing code is to develop a touch typing habit: a habit of striking the correct letters on the keyboard without actually looking at the keyboard.
Most of the time we tend to code while looking at the keyboard and the screen at the same time without realizing that is an inexpert form of typing.
You have to break the hunt-and-peck typing habit and train yourself to type by not looking at the keyboard (looking at the screen only). This is possible through muscle memory typing.
It is a bit harder to develop this habit but once you develop it typing becomes an automated practice.
I was able to develop this habit after several weeks of practice on a free site called keybr.com, which is the best site that I recommend to everybody that wants to develop this habit.
08: Use the best tools
Tools can be a great part to improve your overall productivity as a programmer and I can not stress this enough to say that you need better tools if not the best(both hardware and software).
Always make sure that you are using the best libraries (I recommend MQL5 standard libraries), you also need to have the best sources of information when you are stuck and when you need to learn and try new things out (read this article for more details) and on top of all that you need a well able PC and good internet connection.
09: Do version control
It is always a good habit to have version control for your code so that you can track your progress and be able to go back to previous versions of code anytime you want.
I know two ways to do this. The first one is using Git and the second way is a manual process that involves copying and pasting methods of storing code through a text file.
Using Git
This is a no-quick fix way that I can teach you in few minutes if you are not familiar with Git and Github, I recommend reading the Git documentation.
Through a Text-file
Create a folder for your project, add all the necessary files needed in that project. Then open that folder in your file explorer to view what's in the folder (see the image below from my previous example).
After making a major change to your .mq5 like, adding a new function, you have to create a text file with a name specifying the version of the code and what was added. See the image
You can open a text file alongside the main .mq5 or .mqh file and save them both with one click CTRL+F7 in windows, which will compile all the open files on your editor. After a successful compilation, you can ignore all the errors coming from that text file and close it.
Conclusion
That's it for this article. I hope you have gained something positive to move you one step further to become a better programmer. If you have any pearls of wisdom that you find missing, share them with me and other programmers in the discussion section below. All the code used in this article are attached below.
Thanks for reading, best regards.





- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use