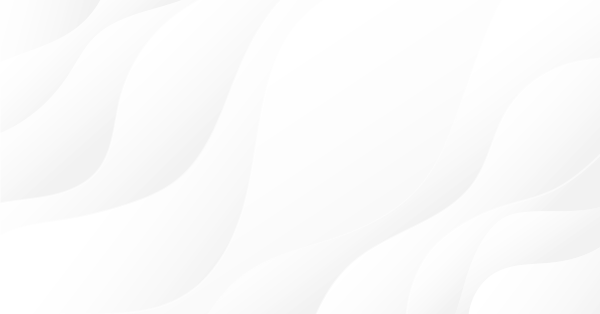
MQL5クックブック - MQL5での未決注文の取り扱いとマルチカレンシーエキスパートアドバイザー
はじめに
今回は、指値売り、逆指値売りなど未決注文に基づくトレーディングアルゴリズムを持つマルチカレンシーエキスパートアドバイザーを作成していきます。作成するものは、デイトレード・テストのために設計されています。この記事は、以下を紹介します:
- 特定の時間半位におけるトレーディングトレーディングの最初と最後の時間を設定する機能を作成しましょう。例えば、ヨーロピアンやアメリカのトレーディングセッション時間になります。エキスパートアドバイザーのパラメーターを最適化する際、最も適した時間の半位を見つけることができます。
- 未決注文の実行・修正・削除
- トレードイベントの処理;最後のポジションが利取りや損切りにて閉じられたかのチェック、各シンボルにおける取引の履歴の管理
エキスパートアドバイザーの開発
MQL5クックブック:マルチカレンシーエキスパートアドバイザー - シンプルで素早いアプローチという記事のコードをテンプレートとして使用していきます。その本質的な構造は同じですが、いくつかの重要な変更を紹介します。エキスパートアドバイザーは、デイトレーディングのため設計されていますが、このモードは、必要があればスイッチがオフになるようになっています。そのような場合、未決注文はもしポジションがクローズされた場合、すぐさま行われます(もしくは新しいバーイベント時)
エキスパートアドバイザーの外部パラメーターから始めましょう。まずは、Enums.mqhのファイルのENUM_HOURS列挙型を作成します。この列挙型において識別子の数は、24に等しいです。
//--- Hours Enumeration enum ENUM_HOURS { h00 = 0, // 00 : 00 h01 = 1, // 01 : 00 h02 = 2, // 02 : 00 h03 = 3, // 03 : 00 h04 = 4, // 04 : 00 h05 = 5, // 05 : 00 h06 = 6, // 06 : 00 h07 = 7, // 07 : 00 h08 = 8, // 08 : 00 h09 = 9, // 09 : 00 h10 = 10, // 10 : 00 h11 = 11, // 11 : 00 h12 = 12, // 12 : 00 h13 = 13, // 13 : 00 h14 = 14, // 14 : 00 h15 = 15, // 15 : 00 h16 = 16, // 16 : 00 h17 = 17, // 17 : 00 h18 = 18, // 18 : 00 h19 = 19, // 19 : 00 h20 = 20, // 20 : 00 h21 = 21, // 21 : 00 h22 = 22, // 22 : 00 h23 = 23 // 23 : 00 };
外部パラメーターのリストにて、時間半位におけるトレーディングに関連した4つのパラメーターを作成します。
- TradeInTimeRange - enabling/disabling the mode. すでに述べられている通り、エキスパートアドバイザーの作業を特定の期間だけではなく、継続モードの時間において可能にしましょう。
- StartTrade - トレーディングセッション開始時間サーバー時間がこの値に等しければ、TradeInTimeRangeモードがオンの状態でエキスパートアドバイザーは未決注文を行っていたでしょう。
- StopOpenOrders - 未決注文の終了時間サーバー時間がこの値に等しければ、エキスパートアドバイザーはポジションがクローズされた場合、未決注文を停止させます。
- EndTrade - トレーディングセッション終了時間サーバーの時間がこの値に等しければ、エキスパートアドバイザーはトレーディングを終了します。特定のシンボルにおいてのオープンポジションはクローズされ、未決注文は削除されます。
外部パラメーターのリストは以下のようになっています。提示されている例は、二つのシンボルにおけるものです。PendingOrderパラメーターにて、現在の価格からの距離を設定しました。
//--- External parameters of the Expert Advisor sinput long MagicNumber = 777; // Magic number sinput int Deviation = 10; // Slippage //--- sinput string delimeter_00=""; // -------------------------------- sinput string Symbol_01 ="EURUSD"; // Symbol 1 input bool TradeInTimeRange_01 =true; // | Trading in a time range input ENUM_HOURS StartTrade_01 = h10; // | The hour of the beginning of a trading session input ENUM_HOURS StopOpenOrders_01 = h17; // | The hour of the end of placing orders input ENUM_HOURS EndTrade_01 = h22; // | The hour of the end of a trading session input double PendingOrder_01 = 50; // | Pending order input double TakeProfit_01 = 100; // | Take Profit input double StopLoss_01 = 50; // | Stop Loss input double TrailingStop_01 = 10; // | Trailing Stop input bool Reverse_01 = true; // | Position reversal input double Lot_01 = 0.1; // | Lot //--- sinput string delimeter_01=""; // -------------------------------- sinput string Symbol_02 ="AUDUSD"; // Symbol 2 input bool TradeInTimeRange_02 =true; // | Trading in a time range input ENUM_HOURS StartTrade_02 = h10; // | The hour of the beginning of a trading session input ENUM_HOURS StopOpenOrders_02 = h17; // | The hour of the end of placing orders input ENUM_HOURS EndTrade_02 = h22; // | The hour of the end of a trading session input double PendingOrder_02 = 50; // | Pending order input double TakeProfit_02 = 100; // | Take Profit input double StopLoss_02 = 50; // | Stop Loss input double TrailingStop_02 = 10; // | Trailing Stop input bool Reverse_02 = true; // | Position reversal input double Lot_02 = 0.1; // | Lot
また、一致する変更が外部パラメーターの値にて格納されるだろう配列のリストにて行われる必要があります:
//--- Arrays for storing external parameters string Symbols[NUMBER_OF_SYMBOLS]; // Symbol bool TradeInTimeRange[NUMBER_OF_SYMBOLS]; // Trading in a time range ENUM_HOURS StartTrade[NUMBER_OF_SYMBOLS]; // The hour of the beginning of a trading session ENUM_HOURS StopOpenOrders[NUMBER_OF_SYMBOLS]; // The hour of the end of placing orders ENUM_HOURS EndTrade[NUMBER_OF_SYMBOLS]; // The hour of the end of a trading session double PendingOrder[NUMBER_OF_SYMBOLS]; // Pending order double TakeProfit[NUMBER_OF_SYMBOLS]; // Take Profit double StopLoss[NUMBER_OF_SYMBOLS]; // Stop Loss double TrailingStop[NUMBER_OF_SYMBOLS]; // Trailing Stop bool Reverse[NUMBER_OF_SYMBOLS]; // Position Reversal double Lot[NUMBER_OF_SYMBOLS]; // Lot
それでは、リバースモードにてそれを調整していきます(Reverseパラメーター値はtrueでした) 反対の未決注文が削除され、新しい注文が発行されます。価格レベルを蹴た場合に行うような未決注文の量の変更はできません。したがって、削除し必要な量の未決注文を行わなければなりません。
さらに、もしリバースモードがオンの状態で、トレール注文レベルが同時に設定されていれば、未決注文は価格に従います。もし損切りが行われれば、その価格値が計算され、未決注文に基づいて明記されます。
そのグローバルスコープにて、未決注文のコメントのための二つのString型変数を作成します。
//--- Pending order comments string comment_top_order ="top_order"; string comment_bottom_order ="bottom_order";
エキスパートアドバイザーのローディング中のOnInit()の関数での初期化にて、外部パラメーターの正誤のチェックを行います。その評価の基準は以下の通りです。TradeInTimeRangeモードがオンであれば、トレードセッションの開始時間は未決注文の終了時間よりも1時間前以下にならなければなりません。未決注文の終了時間はトレードセッション終了時間よりも1時間前以下である必要があります。そのチェックを行うCheckInputParameters()関数を作成しましょう:
//+------------------------------------------------------------------+ //| Checks external parameters | //+------------------------------------------------------------------+ bool CheckInputParameters() { //--- Loop through the specified symbols for(int s=0; s<NUMBER_OF_SYMBOLS; s++) { //--- If there is no symbol and the TradeInTimeRange mode is disabled, move on to the following symbol. if(Symbols[s]=="" || !TradeInTimeRange[s]) continue; //--- Check the accuracy of the start and the end of a trade session time if(StartTrade[s]>=EndTrade[s]) { Print(Symbols[s], ": The hour of the beginning of a trade session("+IntegerToString(StartTrade[s])+") " "must be less than the hour of the end of a trade session"("+IntegerToString(EndTrade[s])+")!"); return(false); } //--- A trading session is to start no later that one hour before the hour of placing pending orders. // Pending orders are to be placed no later than one hour before the hour of the end of a trading session. if(StopOpenOrders[s]>=EndTrade[s] || StopOpenOrders[s]<=StartTrade[s]) { Print(Symbols[s], ": The hour of the end of placing orders ("+IntegerToString(StopOpenOrders[s])+") " "is to be less than the hour of the end ("+IntegerToString(EndTrade[s])+") and " "greater than the hour of the beginning of a trading session ("+IntegerToString(StartTrade[s])+")!"); return(false); } } //--- Parameters are correct return(true); }
このパターンを実装するために、トレードや未決注文のための特定の時間半位にとどまるためのチェックを実行する関数が必要です。これらの IsInTradeTimeRange()やIsInOpenOrdersTimeRange()関数の名前を付けます。これらは同様な働きをし、唯一の違いはチェックにおける上限のみです。これらの関数が使用される部分を見てみましょう。
//+------------------------------------------------------------------+ //| Checks if we are within the time range for trade | //+------------------------------------------------------------------+ bool IsInTradeTimeRange(int symbol_number) { //--- If TradeInTimeRange mode is enabled if(TradeInTimeRange[symbol_number]) { //--- Structure of the date and time MqlDateTime last_date; //--- Get the last value of the date and time data set TimeTradeServer(last_date); //--- Outside of the allowed time range if(last_date.hour<StartTrade[symbol_number] || last_date.hour>=EndTrade[symbol_number]) return(false); } //--- Within the allowed time range return(true); } //+------------------------------------------------------------------+ //| Checks if we are within the time range for placing orders | //+------------------------------------------------------------------+ bool IsInOpenOrdersTimeRange(int symbol_number) { //--- If the TradeInTimeRange mode if enabled if(TradeInTimeRange[symbol_number]) { //--- Structure of the date and time MqlDateTime last_date; //--- Get the last value of the date and time data set TimeTradeServer(last_date); //--- Outside the allowed time range if(last_date.hour<StartTrade[symbol_number] || last_date.hour>=StopOpenOrders[symbol_number]) return(false); } //--- Within the allowed time range return(true); }
以前の記事で、ポジションやシンボルのプロパティや取引履歴を取得する関数を紹介しました。この記事では、未決注文のプロパティを取得する類似した関数が必要です。Enums.mqhファイルに、未決注文の属性を持つ列挙型を作成します。
//--- Enumeration of the properties of a pending order enum ENUM_ORDER_PROPERTIES { O_SYMBOL = 0, O_MAGIC = 1, O_COMMENT = 2, O_PRICE_OPEN = 3, O_PRICE_CURRENT = 4, O_PRICE_STOPLIMIT = 5, O_VOLUME_INITIAL = 6, O_VOLUME_CURRENT = 7, O_SL = 8, O_TP = 9, O_TIME_SETUP = 10, O_TIME_EXPIRATION = 11, O_TIME_SETUP_MSC = 12, O_TYPE_TIME = 13, O_TYPE = 14, O_ALL = 15 };
TradeFunctions.mqhファイルにて、未決注文の属性を持つストラクチャーを作成し、インスタンスを生成する必要があります。
//-- Properties of a pending order struct pending_order_properties { string symbol; // Symbol long magic; // Magic number string comment; // Comment double price_open; // Price specified in the order double price_current; // Current price of the order symbol double price_stoplimit; // Limit order price for the Stop Limit order double volume_initial; // Initial order volume double volume_current; // Current order volume double sl; // Stop Loss level double tp; // Take Profit level datetime time_setup; // Order placement time datetime time_expiration; // Order expiration time datetime time_setup_msc; // The time of placing an order for execution in milliseconds since 01.01.1970 datetime type_time; // Order lifetime ENUM_ORDER_TYPE type; // Position type }; //--- Variable of the order features pending_order_properties ord;
未決注文の属性を取得するために、GetPendingOrderProperties()関数を作成します。未決注文が選択された後、注文の属性を取得するための関数を使用できます。この方法は以下に記載されています。
//+------------------------------------------------------------------+ //| Retrieves the properties of the previously selected pending order| //+------------------------------------------------------------------+ void GetPendingOrderProperties(ENUM_ORDER_PROPERTIES order_property) { switch(order_property) { case O_SYMBOL : ord.symbol=OrderGetString(ORDER_SYMBOL); break; case O_MAGIC : ord.magic=OrderGetInteger(ORDER_MAGIC); break; case O_COMMENT : ord.comment=OrderGetString(ORDER_COMMENT); break; case O_PRICE_OPEN : ord.price_open=OrderGetDouble(ORDER_PRICE_OPEN); break; case O_PRICE_CURRENT : ord.price_current=OrderGetDouble(ORDER_PRICE_CURRENT); break; case O_PRICE_STOPLIMIT : ord.price_stoplimit=OrderGetDouble(ORDER_PRICE_STOPLIMIT); break; case O_VOLUME_INITIAL : ord.volume_initial=OrderGetDouble(ORDER_VOLUME_INITIAL); break; case O_VOLUME_CURRENT : ord.volume_current=OrderGetDouble(ORDER_VOLUME_CURRENT); break; case O_SL : ord.sl=OrderGetDouble(ORDER_SL); break; case O_TP : ord.tp=OrderGetDouble(ORDER_TP); break; case O_TIME_SETUP : ord.time_setup=(datetime)OrderGetInteger(ORDER_TIME_SETUP); break; case O_TIME_EXPIRATION : ord.time_expiration=(datetime)OrderGetInteger(ORDER_TIME_EXPIRATION); break; case O_TIME_SETUP_MSC : ord.time_setup_msc=(datetime)OrderGetInteger(ORDER_TIME_SETUP_MSC); break; case O_TYPE_TIME : ord.type_time=(datetime)OrderGetInteger(ORDER_TYPE_TIME); break; case O_TYPE : ord.type=(ENUM_ORDER_TYPE)OrderGetInteger(ORDER_TYPE); break; case O_ALL : ord.symbol=OrderGetString(ORDER_SYMBOL); ord.magic=OrderGetInteger(ORDER_MAGIC); ord.comment=OrderGetString(ORDER_COMMENT); ord.price_open=OrderGetDouble(ORDER_PRICE_OPEN); ord.price_current=OrderGetDouble(ORDER_PRICE_CURRENT); ord.price_stoplimit=OrderGetDouble(ORDER_PRICE_STOPLIMIT); ord.volume_initial=OrderGetDouble(ORDER_VOLUME_INITIAL); ord.volume_current=OrderGetDouble(ORDER_VOLUME_CURRENT); ord.sl=OrderGetDouble(ORDER_SL); ord.tp=OrderGetDouble(ORDER_TP); ord.time_setup=(datetime)OrderGetInteger(ORDER_TIME_SETUP); ord.time_expiration=(datetime)OrderGetInteger(ORDER_TIME_EXPIRATION); ord.time_setup_msc=(datetime)OrderGetInteger(ORDER_TIME_SETUP_MSC); ord.type_time=(datetime)OrderGetInteger(ORDER_TYPE_TIME); ord.type=(ENUM_ORDER_TYPE)OrderGetInteger(ORDER_TYPE); break; //--- default: Print("Retrieved feature of the pending order was not taken into account in the enumeration "); return; } }
それでは、未決注文の修正や削除などのための基礎的な関数を作成していきましょう。SetPendingOrder()関数は、未決注文を登録します。もし未決注文の登録に失敗すれば、その関数はエラーコードとその詳細にてJournalに報告します。
//+------------------------------------------------------------------+ //| Places a pending order | //+------------------------------------------------------------------+ void SetPendingOrder(int symbol_number, // Symbol number ENUM_ORDER_TYPE order_type, // Order type double lot, // Volume double stoplimit_price, // Level of the StopLimit order double price, // Price double sl, // Stop Loss double tp, // Take Profit ENUM_ORDER_TYPE_TIME type_time, // Order Expiration string comment) // Comment //--- Set magic number in the trade structure trade.SetExpertMagicNumber(MagicNumber); //--- If a pending order failed to be placed, print an error message if(!trade.OrderOpen(Symbols[symbol_number], order_type,lot,stoplimit_price,price,sl,tp,type_time,0,comment)) Print("Error when placing a pending order: ",GetLastError()," - ",ErrorDescription(GetLastError())); }
ModifyPendingOrder()関数は未決注文を修正しました。注文の価格だけではなく、その量を変更し、関数の最後のパラメーターとして渡すことができるようにアレンジしていきましょう。もし渡された量の値が0より大きければ、未決注文が削除される必要があり、必要な量の値の新しい注文が行われます。どのケースにおいても、シンプルに価格の値を変更することで既存の注文を修正します。
//+------------------------------------------------------------------+ //| Modifies a pending order | //+------------------------------------------------------------------+ void ModifyPendingOrder(int symbol_number, //Symbol number ulong ticket, // Order ticket ENUM_ORDER_TYPE type, // Order type double price, // Order price double sl, // Stop Loss of the order double tp, // Take Profit of the order ENUM_ORDER_TYPE_TIME type_time, // Order expiration datetime time_expiration, // Order expiration time double stoplimit_price, // Price string comment, // Comment double volume) // Volume { //--- If the passed volume value is non-zero, delete the order and place it again if(volume>0) { //--- If the order failed to be deleted, exit if(!DeletePendingOrder(ticket)) return; //--- Place a pending order SetPendingOrder(symbol_number,type,volume,0,price,sl,tp,type_time,comment); //--- Adjust Stop Loss of position as related to the order CorrectStopLossByOrder(symbol_number,price,type); } //--- If the passed volume value is zero, modify the order else { //--- If the pending order failed to be modified, print a relevant message if(!trade.OrderModify(ticket,price,sl,tp,type_time,time_expiration,stoplimit_price)) Print("Error when modifying the pending order price: ", GetLastError()," - ",ErrorDescription(GetLastError())); //--- Otherwise adjust Stop Loss of position as related to the order else CorrectStopLossByOrder(symbol_number,price,type); } }
その上記のコードにてDeletePendingOrder()とCorrectStopLossByOrder()の二つの新しい関数がハイライト表示されています。最初の関数は未決注文を削除し、2番目の関数は未決注文に関連したポジションの損切りを調整します。
//+------------------------------------------------------------------+ //| Deletes a pending order | //+------------------------------------------------------------------+ bool DeletePendingOrder(ulong ticket) { //--- If a pending order failed to get deleted, print a relevant message if(!trade.OrderDelete(ticket)) { Print("Error when deleting a pending order: ",GetLastError()," - ",ErrorDescription(GetLastError())); return(false); } //--- return(true); } //+------------------------------------------------------------------+ //| Modifies StopLoss of the position as related to the pending order| //+------------------------------------------------------------------+ void CorrectStopLossByOrder(int symbol_number, // Symbol number double price, // Order Price ENUM_ORDER_TYPE type) // Order Type { //--- If Stop Loss disabled, exit if(StopLoss[symbol_number]==0) return; //--- If Stop Loss enabled double new_sl=0.0; // New Stop Loss value //--- Get a Point value GetSymbolProperties(symbol_number,S_POINT); //--- Number of decimal places GetSymbolProperties(symbol_number,S_DIGITS); //--- Get Take Profit of position GetPositionProperties(symbol_number,P_TP); //--- Calculate as related to the order type switch(type) { case ORDER_TYPE_BUY_STOP : new_sl=NormalizeDouble(price+CorrectValueBySymbolDigits(StopLoss[symbol_number]*symb.point),symb.digits); break; case ORDER_TYPE_SELL_STOP : new_sl=NormalizeDouble(price-CorrectValueBySymbolDigits(StopLoss[symbol_number]*symb.point),symb.digits); break; } //--- Modify the position if(!trade.PositionModify(Symbols[symbol_number],new_sl,pos.tp)) Print("Error when modifying position: ",GetLastError()," - ",ErrorDescription(GetLastError())); }
未決注文を登録する前に同じコメントを持つ未決注文がすでに存在しているかをチェックする必要があります。この記事の最初に言及されている通り、「top_order」というコメントの付いた指値注文と、「bottom_order」というコメントの付いた逆指値注文を登録します。そのようなチェックを促進させる CheckPendingOrderByComment()という関数を作成します:
//+------------------------------------------------------------------+ //| Checks existence of a pending order by a comment | //+------------------------------------------------------------------+ bool CheckPendingOrderByComment(int symbol_number,string comment) { int total_orders =0; // Total number of pending orders string order_symbol =""; // Order Symbol string order_comment =""; // Order Comment //--- Get the total number of pending orders total_orders=OrdersTotal(); //--- Loop through the total orders for(int i=total_orders-1; i>=0; i--) { //---Select the order by the ticket if(OrderGetTicket(i)>0) { //--- Get the symbol name order_symbol=OrderGetString(ORDER_SYMBOL); //--- If the symbols are equal if(order_symbol==Symbols[symbol_number]) { //--- Get the order comment order_comment=OrderGetString(ORDER_COMMENT); //--- If the comments are equal if(order_comment==comment) return(true); } } } //--- Order with a specified comment not found return(false); }
その上記のコードは、注文の合計数がOrdersTotal()関数を用いて取得できるということを示しています。しかし、特定のシンボルにおいて未決注文の合計数を取得するためにユーザーにより定義された関数を作成していきます。それをOrdersTotalBySymbol()と名付けます:
//+------------------------------------------------------------------+ //| Returns the total number of orders for the specified symbol | //+------------------------------------------------------------------+ int OrdersTotalBySymbol(string symbol) { int count =0; // Order counter int total_orders =0; // Total number of pending orders //--- Get the total number of pending orders total_orders=OrdersTotal(); //--- Loop through the total number of orders for(int i=total_orders-1; i>=0; i--) { //--- If an order has been selected if(OrderGetTicket(i)>0) { //--- Get the order symbol GetOrderProperties(O_SYMBOL); //--- If the order symbol and the specified symbol are equal if(ord.symbol==symbol) //--- Increase the counter count++; } } //--- Return the total number of orders return(count); }
未決注文を登録する前に必要であれば損切りや利取りと同様その価格を計算しなければなりません。もしリバースモードがオンであれば、トレーリング注文レベルの再計算と変更のための個別のユーザーにより定義された関数が必要です。
未決注文を計算するためにCalculatePendingOrder()関数を作成しましょう:
//+------------------------------------------------------------------+ //| Calculates the pending order level(price) | //+------------------------------------------------------------------+ double CalculatePendingOrder(int symbol_number,ENUM_ORDER_TYPE order_type) { //--- For the calculated pending order value double price=0.0; //--- If the value for SELL STOP order is to be calculated if(order_type==ORDER_TYPE_SELL_STOP) { //--- Calculate level price=NormalizeDouble(symb.bid-CorrectValueBySymbolDigits(PendingOrder[symbol_number]*symb.point),symb.digits); //--- Return calculated value if it is less than the lower limit of Stops level // If the value is equal or greater, return the adjusted value return(price<symb.down_level ? price : symb.down_level-symb.offset); } //--- If the value for BUY STOP order is to be calculated if(order_type==ORDER_TYPE_BUY_STOP) { //--- Calculate level price=NormalizeDouble(symb.ask+CorrectValueBySymbolDigits(PendingOrder[symbol_number]*symb.point),symb.digits); //--- Return the calculated value if it is greater than the upper limit of Stops level // If the value is equal or less, return the adjusted value return(price>symb.up_level ? price : symb.up_level+symb.offset); } //--- return(0.0); }
以下は未決注文における損切りと利取りレベルの計算のための関数コードです。
//+------------------------------------------------------------------+ //| Calculates Stop Loss level for a pending order | //+------------------------------------------------------------------+ double CalculatePendingOrderStopLoss(int symbol_number,ENUM_ORDER_TYPE order_type,double price) { //--- If Stop Loss is required if(StopLoss[symbol_number]>0) { double sl =0.0; // For the Stop Loss calculated value double up_level =0.0; // Upper limit of Stop Levels double down_level =0.0; // Lower limit of Stop Levels //--- If the value for BUY STOP order is to be calculated if(order_type==ORDER_TYPE_BUY_STOP) { //--- Define lower threshold down_level=NormalizeDouble(price-symb.stops_level*symb.point,symb.digits); //--- Calculate level sl=NormalizeDouble(price-CorrectValueBySymbolDigits(StopLoss[symbol_number]*symb.point),symb.digits); //--- Return the calculated value if it is less than the lower limit of Stop level // If the value is equal or greater, return the adjusted value return(sl<down_level ? sl : NormalizeDouble(down_level-symb.offset,symb.digits)); } //--- If the value for the SELL STOP order is to be calculated if(order_type==ORDER_TYPE_SELL_STOP) { //--- Define the upper threshold up_level=NormalizeDouble(price+symb.stops_level*symb.point,symb.digits); //--- Calculate the level sl=NormalizeDouble(price+CorrectValueBySymbolDigits(StopLoss[symbol_number]*symb.point),symb.digits); //--- Return the calculated value if it is greater than the upper limit of the Stops level // If the value is less or equal, return the adjusted value. return(sl>up_level ? sl : NormalizeDouble(up_level+symb.offset,symb.digits)); } } //--- return(0.0); } //+------------------------------------------------------------------+ //| Calculates the Take Profit level for a pending order | //+------------------------------------------------------------------+ double CalculatePendingOrderTakeProfit(int symbol_number,ENUM_ORDER_TYPE order_type,double price) { //--- If Take Profit is required if(TakeProfit[symbol_number]>0) { double tp =0.0; // For the calculated Take Profit value double up_level =0.0; // Upper limit of Stop Levels double down_level =0.0; // Lower limit of Stop Levels //--- If the value for SELL STOP order is to be calculated if(order_type==ORDER_TYPE_SELL_STOP) { //--- Define lower threshold down_level=NormalizeDouble(price-symb.stops_level*symb.point,symb.digits); //--- Calculate the level tp=NormalizeDouble(price-CorrectValueBySymbolDigits(TakeProfit[symbol_number]*symb.point),symb.digits); //--- Return the calculated value if it is less than the below limit of the Stops level // If the value is greater or equal, return the adjusted value return(tp<down_level ? tp : NormalizeDouble(down_level-symb.offset,symb.digits)); } //--- If the value for the BUY STOP order is to be calculated if(order_type==ORDER_TYPE_BUY_STOP) { //--- Define the upper threshold up_level=NormalizeDouble(price+symb.stops_level*symb.point,symb.digits); //--- Calculate the level tp=NormalizeDouble(price+CorrectValueBySymbolDigits(TakeProfit[symbol_number]*symb.point),symb.digits); //--- Return the calculated value if it is greater than the upper limit of the Stops level // If the value is less or equal, return the adjusted value return(tp>up_level ? tp : NormalizeDouble(up_level+symb.offset,symb.digits)); } } //--- return(0.0); }
リバースされた未決注文の損切りレベルを計算し、引き上げるために以下のCalculateReverseOrderTrailingStop()とModifyPendingOrderTrailingStop()関数を作成します。その関数のコードが以下にあります。
CalculateReverseOrderTrailingStop()関数のコード:
//+----------------------------------------------------------------------------+ //| Calculates the Trailing Stop level for the reversed order | //+----------------------------------------------------------------------------+ double CalculateReverseOrderTrailingStop(int symbol_number,ENUM_POSITION_TYPE position_type) { //--- Variables for calculation double level =0.0; double buy_point =low[symbol_number].value[1]; // Low value for Buy double sell_point =high[symbol_number].value[1]; // High value for Sell //--- Calculate the level for the BUY position if(position_type==POSITION_TYPE_BUY) { //--- Bar's low minus the specified number of points level=NormalizeDouble(buy_point-CorrectValueBySymbolDigits(PendingOrder[symbol_number]*symb.point),symb.digits); //--- If the calculated level is lower than the lower limit of the Stops level, // the calculation is complete, return the current value of the level if(level<symb.down_level) return(level); //--- If it is not lower, try to calculate based on the bid price else { level=NormalizeDouble(symb.bid-CorrectValueBySymbolDigits(PendingOrder[symbol_number]*symb.point),symb.digits); //--- If the calculated level is lower than the limit, return the current value of the level // otherwise set the nearest possible value return(level<symb.down_level ? level : symb.down_level-symb.offset); } } //--- Calculate the level for the SELL position if(position_type==POSITION_TYPE_SELL) { // Bar's high plus the specified number of points level=NormalizeDouble(sell_point+CorrectValueBySymbolDigits(PendingOrder[symbol_number]*symb.point),symb.digits); //--- If the calculated level is higher than the upper limit of the Stops level, // then the calculation is complete, return the current value of the level if(level>symb.up_level) return(level); //--- If it is not higher, try to calculate based on the ask price else { level=NormalizeDouble(symb.ask+CorrectValueBySymbolDigits(PendingOrder[symbol_number]*symb.point),symb.digits); //--- If the calculated level is higher than the limit, return the current value of the level // Otherwise set the nearest possible value return(level>symb.up_level ? level : symb.up_level+symb.offset); } } //--- return(0.0); }
ModifyPendingOrderTrailingStop()関数のコード:
//+------------------------------------------------------------------+ //| Modifying the Trailing Stop level for a pending order | //+------------------------------------------------------------------+ void ModifyPendingOrderTrailingStop(int symbol_number) { //--- Exit, if the reverse position mode is disabled and Trailing Stop is not set if(!Reverse[symbol_number] || TrailingStop[symbol_number]==0) return; //--- double new_level =0.0; // For calculating a new level for a pending order bool condition =false; // For checking the modification condition int total_orders =0; // Total number of pending orders ulong order_ticket =0; // Order ticket string opposite_order_comment =""; // Opposite order comment ENUM_ORDER_TYPE opposite_order_type =WRONG_VALUE; // Order type //--- Get the flag of presence/absence of a position pos.exists=PositionSelect(Symbols[symbol_number]); //--- If a position is absent if(!pos.exists) return; //--- Get a total number of pending orders total_orders=OrdersTotal(); //--- Get the symbol properties GetSymbolProperties(symbol_number,S_ALL); //--- Get the position properties GetPositionProperties(symbol_number,P_ALL); //--- Get the level for Stop Loss new_level=CalculateReverseOrderTrailingStop(symbol_number,pos.type); //--- Loop through the orders from the last to the first one for(int i=total_orders-1; i>=0; i--) { //--- If the order selected if((order_ticket=OrderGetTicket(i))>0) { //--- Get the order symbol GetPendingOrderProperties(O_SYMBOL); //--- Get the order comment GetPendingOrderProperties(O_COMMENT); //--- Get the order price GetPendingOrderProperties(O_PRICE_OPEN); //--- Depending on the position type, check the relevant condition for the Trailing Stop modification switch(pos.type) { case POSITION_TYPE_BUY : //---If the new order value is greater than the current value plus set step then condition fulfilled condition=new_level>ord.price_open+CorrectValueBySymbolDigits(TrailingStop[symbol_number]*symb.point); //--- Define the type and comment of the reversed pending order for check. opposite_order_type =ORDER_TYPE_SELL_STOP; opposite_order_comment =comment_bottom_order; break; case POSITION_TYPE_SELL : //--- If the new value for the order if less than the current value minus a set step then condition fulfilled condition=new_level<ord.price_open-CorrectValueBySymbolDigits(TrailingStop[symbol_number]*symb.point); //--- Define the type and comment of the reversed pending order for check opposite_order_type =ORDER_TYPE_BUY_STOP; opposite_order_comment =comment_top_order; break; } //--- If condition fulfilled, the order symbol and positions are equal // and order comment and the reversed order comment are equal if(condition && ord.symbol==Symbols[symbol_number] && ord.comment==opposite_order_comment) { double sl=0.0; // Stop Loss double tp=0.0; // Take Profit //--- Get Take Profit and Stop Loss levels sl=CalculatePendingOrderStopLoss(symbol_number,opposite_order_type,new_level); tp=CalculatePendingOrderTakeProfit(symbol_number,opposite_order_type,new_level); //--- Modify order ModifyPendingOrder(symbol_number,order_ticket,opposite_order_type,new_level,sl,tp, ORDER_TIME_GTC,ord.time_expiration,ord.price_stoplimit,ord.comment,0); return; } } } }
時々、ポジションが損切りか利取りでクローズされたかを知るために必要です。このケースにおいてはそれが必要になってきます。したがって、このイベントを最後の取引のコメントで特定する関数を作成しましょう。特定のシンボルにおける最後の取引のコメントを取得するためGetLastDealComment()という名前の個別の関数を作成します:
//+------------------------------------------------------------------+ //| Returns a the last deal comment for a specified symbol | //+------------------------------------------------------------------+ string GetLastDealComment(int symbol_number) { int total_deals =0; // Total number of deals in the selected history string deal_symbol =""; // Deal symbol string deal_comment =""; // Deal comment //--- If the deals history retrieved if(HistorySelect(0,TimeCurrent())) { //--- Receive the number of deals in the retrieved list total_deals=HistoryDealsTotal(); //--- Loop though the total number of deals in the retrieved list from the last deal to the first one. for(int i=total_deals-1; i>=0; i--) { //--- Receive the deal comment deal_comment=HistoryDealGetString(HistoryDealGetTicket(i),DEAL_COMMENT); //--- Receive the deal symbol deal_symbol=HistoryDealGetString(HistoryDealGetTicket(i),DEAL_SYMBOL); //--- If the deal symbol and the current symbol are equal, stop the loop if(deal_symbol==Symbols[symbol_number]) break; } } //--- return(deal_comment); }
特定のシンボルにおける最後のポジションのクロージングの理由を決定する関数を作成しやすくなりました。 IsClosedByTakeProfit()とIsClosedByStopLoss()の関数のコードは以下です:
//+------------------------------------------------------------------+ //| Returns the reason for closing position at Take Profit | //+------------------------------------------------------------------+ bool IsClosedByTakeProfit(int symbol_number) { string last_comment=""; //--- Get the last deal comment for the specified symbol last_comment=GetLastDealComment(symbol_number); //--- If the comment contain a string "tp" if(StringFind(last_comment,"tp",0)>-1) return(true); //--- If the comment does not contain a string "tp" return(false); } //+------------------------------------------------------------------+ //| Returns the reason for closing position at Stop Loss | //+------------------------------------------------------------------+ bool IsClosedByStopLoss(int symbol_number) { string last_comment=""; //--- Get the last deal comment for the specified symbol last_comment=GetLastDealComment(symbol_number); //--- If the comment contains the string "sl" if(StringFind(last_comment,"sl",0)>-1) return(true); //--- If the comment does not contain the string "sl" return(false); }
履歴の最後の取引が特定のシンボルにおける取引であるかを判断するためのチェックを実行します。メモリの最後の取引チケットを保管したいと思います。そのために、グローバルスコープ上に配列を追加します。
//--- Array for checking the ticket of the last deal for each symbol. ulong last_deal_ticket[NUMBER_OF_SYMBOLS];
最後の取引のチケットをチェックする関数IsLastDealTicket()は以下のようになります:
//+------------------------------------------------------------------+ //| Returns the event of the last deal for the specified symbol | //+------------------------------------------------------------------+ bool IsLastDealTicket(int symbol_number) { int total_deals =0; // Total number of deals in the selected history list string deal_symbol =""; // Deal symbol ulong deal_ticket =0; // Deal ticket //--- If the deal history was received if(HistorySelect(0,TimeCurrent())) { //--- Get the total number of deals in the received list total_deals=HistoryDealsTotal(); //--- Loop through the total number of deals from the last deal to the first one for(int i=total_deals-1; i>=0; i--) { //--- Get deal ticket deal_ticket=HistoryDealGetTicket(i); //--- Get deal symbol deal_symbol=HistoryDealGetString(deal_ticket,DEAL_SYMBOL); //--- If deal symbol and the current one are equal, stop the loop if(deal_symbol==Symbols[symbol_number]) { //--- If the tickets are equal, exit if(deal_ticket==last_deal_ticket[symbol_number]) return(false); //--- If the tickets are not equal report it else { //--- Save the last deal ticket last_deal_ticket[symbol_number]=deal_ticket; return(true); } } } } //--- return(false); }
もし現在の時間が特定のトレード範囲の外であれば、そのポジションは損失、利益のどちらが出ていてもクローズさせられます。ポジションのクローズのためのClosePosition()関数を作成しましょう:
//+------------------------------------------------------------------+ //| Closes position | //+------------------------------------------------------------------+ void ClosePosition(int symbol_number) { //--- Check if position exists pos.exists=PositionSelect(Symbols[symbol_number]); //--- If there is no position, exit if(!pos.exists) return; //--- Set the slippage value in points trade.SetDeviationInPoints(CorrectValueBySymbolDigits(Deviation)); //--- If the position was not closed, print the relevant message if(!trade.PositionClose(Symbols[symbol_number])) Print("Error when closing position: ",GetLastError()," - ",ErrorDescription(GetLastError())); }
ポジションがトレードの時間範囲外でクローズされた際、すべての未決注文は削除されなければなりません。作成する予定のDeleteAllPendingOrders()関数は特定のシンボルにおける未決注文を全て削除します。
//+------------------------------------------------------------------+ //| Deletes all pending orders | //+------------------------------------------------------------------+ void DeleteAllPendingOrders(int symbol_number) { int total_orders =0; // Total number of pending orders ulong order_ticket =0; // Order ticket //--- Get the total number of pending orders total_orders=OrdersTotal(); //--- Loop through the total number of pending orders for(int i=total_orders-1; i>=0; i--) { //--- If the order selected if((order_ticket=OrderGetTicket(i))>0) { //--- Get the order symbol GetOrderProperties(O_SYMBOL); //--- If the order symbol and the current symbol are equal if(ord.symbol==Symbols[symbol_number]) //--- Delete the order DeletePendingOrder(order_ticket); } } }
ストラクチャースキームにおいて必要な全ての関数が揃いました。重要な変更のあったTradingBlock()と未決注文を管理する新しいManagePendingOrders()関数を見てみましょう。未決注文に関する現在の状況への完全な管理がその中で実行されます。
TradingBlock()関数は以下のようになります:
//+------------------------------------------------------------------+ //| Trade block | //+------------------------------------------------------------------+ void TradingBlock(int symbol_number) { double tp=0.0; // Take Profit double sl=0.0; // Stop Loss double lot=0.0; // Volume for position calculation in case of reversed position double order_price=0.0; // Price for placing the order ENUM_ORDER_TYPE order_type=WRONG_VALUE; // Order type for opening position //--- If outside of the time range for placing pending orders if(!IsInOpenOrdersTimeRange(symbol_number)) return; //--- Find out if there is an open position for the symbol pos.exists=PositionSelect(Symbols[symbol_number]); //--- If there is no position if(!pos.exists) { //--- Get symbol properties GetSymbolProperties(symbol_number,S_ALL); //--- Adjust the volume lot=CalculateLot(symbol_number,Lot[symbol_number]); //--- If there is no upper pending order if(!CheckPendingOrderByComment(symbol_number,comment_top_order)) { //--- Get the price for placing a pending order order_price=CalculatePendingOrder(symbol_number,ORDER_TYPE_BUY_STOP); //--- Get Take Profit and Stop Loss levels sl=CalculatePendingOrderStopLoss(symbol_number,ORDER_TYPE_BUY_STOP,order_price); tp=CalculatePendingOrderTakeProfit(symbol_number,ORDER_TYPE_BUY_STOP,order_price); //--- Place a pending order SetPendingOrder(symbol_number,ORDER_TYPE_BUY_STOP,lot,0,order_price,sl,tp,ORDER_TIME_GTC,comment_top_order); } //--- If there is no lower pending order if(!CheckPendingOrderByComment(symbol_number,comment_bottom_order)) { //--- Get the price for placing the pending order order_price=CalculatePendingOrder(symbol_number,ORDER_TYPE_SELL_STOP); //--- Get Take Profit and Stop Loss levels sl=CalculatePendingOrderStopLoss(symbol_number,ORDER_TYPE_SELL_STOP,order_price); tp=CalculatePendingOrderTakeProfit(symbol_number,ORDER_TYPE_SELL_STOP,order_price); //--- Place a pending order SetPendingOrder(symbol_number,ORDER_TYPE_SELL_STOP,lot,0,order_price,sl,tp,ORDER_TIME_GTC,comment_bottom_order); } } }
未決注文を管理するためのManagePendingOrders()関数コード:
//+------------------------------------------------------------------+ //| Manages pending orders | //+------------------------------------------------------------------+ void ManagePendingOrders() { //--- Loop through the total number of symbols for(int s=0; s<NUMBER_OF_SYMBOLS; s++) { //--- If trading this symbol is forbidden, go to the following one if(Symbols[s]=="") continue; //--- Find out if there is an open position for the symbol pos.exists=PositionSelect(Symbols[s]); //--- If there is no position if(!pos.exists) { //--- If the last deal on current symbol and // position was exited on Take Profit or Stop Loss if(IsLastDealTicket(s) && (IsClosedByStopLoss(s) || IsClosedByTakeProfit(s))) //--- Delete all pending orders for the symbol DeleteAllPendingOrders(s); //--- Go to the following symbol continue; } //--- If there is a position ulong order_ticket =0; // Order ticket int total_orders =0; // Total number of pending orders int symbol_total_orders =0; // Number of pending orders for the specified symbol string opposite_order_comment =""; // Opposite order comment ENUM_ORDER_TYPE opposite_order_type =WRONG_VALUE; // Order type //--- Get the total number of pending orders total_orders=OrdersTotal(); //--- Get the total number of pending orders for the specified symbol symbol_total_orders=OrdersTotalBySymbol(Symbols[s]); //--- Get symbol properties GetSymbolProperties(s,S_ASK); GetSymbolProperties(s,S_BID); //--- Get the comment for the selected position GetPositionProperties(s,P_COMMENT); //--- If the position comment belongs to the upper order, // then the lower order is to be deleted, modified/placed if(pos.comment==comment_top_order) { opposite_order_type =ORDER_TYPE_SELL_STOP; opposite_order_comment =comment_bottom_order; } //--- If the position comment belongs to the lower order, // then the upper order is to be deleted/modified/placed if(pos.comment==comment_bottom_order) { opposite_order_type =ORDER_TYPE_BUY_STOP; opposite_order_comment =comment_top_order; } //--- If there are no pending orders for the specified symbol if(symbol_total_orders==0) { //--- If the position reversal is enabled, place a reversed order if(Reverse[s]) { double tp=0.0; // Take Profit double sl=0.0; // Stop Loss double lot=0.0; // Volume for position calculation in case of reversed positio double order_price=0.0; // Price for placing the order //--- Get the price for placing a pending order order_price=CalculatePendingOrder(s,opposite_order_type); //---Get Take Profit и Stop Loss levels sl=CalculatePendingOrderStopLoss(s,opposite_order_type,order_price); tp=CalculatePendingOrderTakeProfit(s,opposite_order_type,order_price); //--- Calculate double volume lot=CalculateLot(s,pos.volume*2); //--- Place the pending order SetPendingOrder(s,opposite_order_type,lot,0,order_price,sl,tp,ORDER_TIME_GTC,opposite_order_comment); //--- Adjust Stop Loss as related to the order CorrectStopLossByOrder(s,order_price,opposite_order_type); } return; } //--- If there are pending orders for this symbol, then depending on the circumstances delete or // modify the reversed order if(symbol_total_orders>0) { //--- Loop through the total number of orders from the last one to the first one for(int i=total_orders-1; i>=0; i--) { //--- If the order chosen if((order_ticket=OrderGetTicket(i))>0) { //--- Get the order symbol GetPendingOrderProperties(O_SYMBOL); //--- Get the order comment GetPendingOrderProperties(O_COMMENT); //--- If order symbol and position symbol are equal, // and order comment and the reversed order comment are equal if(ord.symbol==Symbols[s] && ord.comment==opposite_order_comment) { //--- If position reversal is disabled if(!Reverse[s]) //--- Delete order DeletePendingOrder(order_ticket); //--- If position reversal is enabled else { double lot=0.0; //--- Get the current order properties GetPendingOrderProperties(O_ALL); //--- Get the current position volume GetPositionProperties(s,P_VOLUME); //--- If the order has been modified already, exit the loop. if(ord.volume_initial>pos.volume) break; //--- Calculate double volume lot=CalculateLot(s,pos.volume*2); //--- Modify (delete and place again) the order ModifyPendingOrder(s,order_ticket,opposite_order_type, ord.price_open,ord.sl,ord.tp, ORDER_TIME_GTC,ord.time_expiration, ord.price_stoplimit,opposite_order_comment,lot); } } } } } } }
メインプログラムファイルの微々たる調整を行う必要があります。トレードイベントハンドラOnTrade()を追加します。未決注文に関連した現在の状況の評価はこの関数にて実行されます。
//+------------------------------------------------------------------+ //| Processing of trade events | //+------------------------------------------------------------------+ void OnTrade() { //--- Check the state of pending orders ManagePendingOrders(); }
ManagePendingOrders()は、ユーザーイベントハンドラOnChartEvent()にて使用されます:
//+------------------------------------------------------------------+ //| User events and chart events handler | //+------------------------------------------------------------------+ void OnChartEvent(const int id, // Event identifier const long &lparam, // Parameter of long event type const double &dparam, // Parameter of double event type const string &sparam) // Parameter of string event type { //--- If it is a user event if(id>=CHARTEVENT_CUSTOM) { //--- Exit, if trade is prohibited if(CheckTradingPermission()>0) return; //--- If it is a tick event if(lparam==CHARTEVENT_TICK) { //--- Check the state of pending orders ManagePendingOrders(); //--- Check signals and trade according to them CheckSignalsAndTrade(); return; } } }
CheckSignalsAndTrade()関数でも同様にいくつかの変更があります。この記事にて紹介された新しい機能が以下のコードにてハイライト表示されています。
//+------------------------------------------------------------------+ //| Checks signals and trades based on New Bar event | //+------------------------------------------------------------------+ void CheckSignalsAndTrade() { //--- Loop through all specified signals for(int s=0; s<NUMBER_OF_SYMBOLS; s++) { //--- If trading this symbol is prohibited, exit if(Symbols[s]=="") continue; //--- If the bar is not new, move on to the following symbol if(!CheckNewBar(s)) continue; //--- If there is a new bar else { //--- If outside the time range if(!IsInTradeTimeRange(s)) { //--- Close position ClosePosition(s); //--- Delete all pending orders DeleteAllPendingOrders(s); //--- Move on to the following symbol continue; } //--- Get bars data GetBarsData(s); //--- Check conditions and trade TradingBlock(s); //--- If position reversal if enabled if(Reverse[s]) //--- Pull up Stop Loss for pending order ModifyPendingOrderTrailingStop(s); //--- If position reversal is disabled else //--- Pull up Stop Loss ModifyTrailingStop(s); } }
すべての準備が完了し、マルチカレンシーエキスパートアドバイザーのパラメーターを最適化できます。以下のストラテジーテスターをセットアップしましょう。
図1 - パラメーターの最適化におけるテスターの設定
まず、通貨ペアのEURUSD、そしてAUDUSDにおけるパラメーターを最適化します。EURUSDの最適化のためにどのパラメーターを選択するかについて以下のスクリーンショットに示されています:
図2 - マルチカレンシーエキスパートアドバイザーの最適化のためのパラメーターの設定
通貨ペアEURUSDのパラメーターの最適化の後、同じパラメーターがAUDUSDに関して最適化されなければなりません。以下がともにテストされた両シンボルにおける結果です。結果は最大のリカバリー要因によって選択されました。両方のシンボルにおいて、ロット値は1に設定されました。
図3 - 二つのシンボルにおけるテスト結果
結論
以上になります。準備のできた関数でトレードでの意思決定のアイディア醸成に集中できます。TradingBlock()とManagePendingOrders()関数にて変更が実装されます。MQL5を最近学習し始めた方には、より多くのシンボルを追加する練習とトレードのアルゴリズムのスキーマを変更することをお勧めします。
MetaQuotes Ltdによってロシア語から翻訳されました。
元の記事: https://www.mql5.com/ru/articles/755





- 無料取引アプリ
- 8千を超えるシグナルをコピー
- 金融ニュースで金融マーケットを探索
オットー ...今なら使える :-)
これでトレードができる。
素晴らしい反応だ。ありがとう!
私はただ、記事の著者が気をつけるべきだと指摘したかっただけです。
あなたがすべきことは
そして、MQL5xxxのゴミをすべて捨てれば、うまくいくだろう;)素晴らしい反応だ。ありがとう!
私はただ、記事の著者が気をつけるべきだと指摘したかっただけです。
う~ん......そうですね。
そして、私はそれを表現してみました。
そのようなことは、誰もそれについて何も言わなくても、他の場所でも気づくものです :-)
うーん......そうだね。
そして、それを表現してみた。
誰も何も言わなくても、他の場所でそれに気づくんだ。)
僕の意図は、MarketOrdersをPendigOrdersにプログラムし直すことだった。
どなたか使ってください。
これは有用なEAではなく、単なる計算方法の例です。テスターでは動作しています。
また、これは私の本当のプログラミング・スタイルではありませんが、非常にシンプルにしてあります。