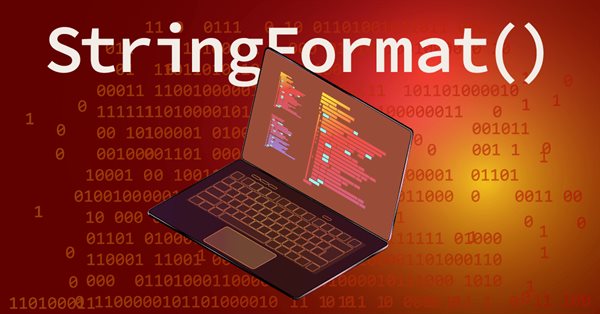
StringFormat():レビューと既成の例
内容
- はじめに
- StringFormat()の概要
- 文字列の書式設定
- 銘柄のプロパティの書式付き出力
- 銘柄の整数プロパティの受信と表示
- データ遅延
- 経済部門
- 業種
- カスタム銘柄
- 気配値表示の背景色
- バーを構築するための価格
- 銘柄の存在
- 気配値表示での選択
- 気配値表示での表示
- 現在のセッションの取引数
- 買い注文数
- 売り注文数
- 最終取引量
- 1日の最大ボリューム
- 1日の最小ボリューム
- 最終クォート時刻
- 最終クォート時刻(ミリ秒単位)
- 桁数
- スプレッド
- 浮動スプレッド
- 市場深度の要求数
- 契約価格計算モード
- 注文執行タイプ
- 取引開始日
- 取引終了日
- 停止レベル
- フリーズレベル
- 取引実行モード
- スワップ計算モデル
- トリプルスワップ発生日
- ヘッジ証拠金計算モード
- 注文の期限切れモードのフラグ
- 注文フィルモードのフラグ
- 許可された注文タイプのフラグ
- ストップロスとテイクプロフィットの有効期間
- オプションタイプ
- 銘柄の実数プロパティの受信と表示
- 買値
- 当日の最高買値
- 当日の最低買値
- 売値
- 当日の最高売値
- 当日の最低売値
- 最終価格
- 当日の最高最終価格
- 当日最終価格最安値
- 最終取引量
- 1日の最大ボリューム
- 1日の最小ボリューム
- オプション行使価格
- ポイント
- ティック価格
- 勝ちポジションのティック価格
- 負けポジションのティック価格
- 最低価格変更
- 約定サイズ
- 未収利息
- 額面金額
- 流動性レート
- 最低ボリューム
- 最高ボリューム
- ボリューム変化ステップ
- 一方向のポジションと注文の最大ボリューム
- 買いスワップ
- 売りスワップ
- スワップ発生率
- 月曜日から火曜日へのスワップ発生率
- 火曜日から水曜日へのスワップ発生率
- 水曜日から木曜日へのスワップ発生率
- 木曜日から金曜日へのスワップ発生率
- 金曜日から土曜日へのスワップ発生率
- 土曜日から日曜日へのスワップ発生率
- 当初証拠金
- 維持証拠金
- 当日の取引量
- 現在のセッション取引高
- ポジションのボリューム
- 買い注文のボリューム
- 売り注文のボリューム
- セッション始値
- セッション終値
- セッション平均加重価格
- 現在のセッションの決済価格
- 1セッションの最低価格
- 1セッションの最高価格
- カバーポジション1ロットの契約サイズ
- 現在価格変動率
- 価格変動率(%)
- 理論オプション価格
- オプション/ワラントデルタ
- オプション/ワラントセータ
- オプション/ワラントガンマ
- オプション/ワラントベガ
- オプション/ワラントロー
- オプション/ワラントオメガ
- 銘柄の文字列プロパティの受信と表示
- 原資産名
- 商品カテゴリまたは部門
- 金融商品の国
- 経済部門
- 経済分野
- 基準通貨
- 利益通貨
- 担保通貨
- クォート元
- 銘柄の説明
- 取引所名
- カスタム銘柄方程式
- ISINシステム名
- ホームページアドレス
- 銘柄ツリー内のパス
- すべての銘柄データを操作ログに出力する関数
- 結論
はじめに
PrintFormat()を使用して構造化されフォーマットされた情報をログする場合、単に指定されたフォーマットに従ってデータをフォーマットし、ターミナルの操作ログに表示します。そのようなフォーマットされた情報は、その後使用することができなくなります。これを再び表示するには、再びPrintFormat()を使って書式設定する必要があります。一度目的の型にフォーマットしたデータを再利用できるのはいいことです。実際に、そのような可能性があります。StringFormat()関数です。単にデータを操作ログに表示する PrintFormat() とは異なり、この関数はフォーマットされた文字列を返します。これにより、この文字列をプログラム内でさらに使用することができます。つまり、必要な形式にフォーマットされたデータは失われません。この方がずっと便利です。フォーマットされた文字列を変数や配列に保存し、繰り返し使用することができます。より柔軟で便利です。
今回の記事は、教育的というよりは、既製の関数テンプレートに重点を置いた拡張リファレンス資料です。
StringFormat()のまとめ
StringFormat()関数は、受け取ったパラメータをフォーマットし、フォーマットされた文字列を返します。文字列の書式設定規則は、PrintFormat()関数とまったく同じです。これらは、記事「PrintFormat()を調べてすぐ使える例を適用する」で詳しく検討しました。テキストと書式指定子を含む文字列が最初に渡され、その後に、文字列中の指定子を置き換えるために必要なデータがカンマ区切りで渡されます。各指定子は、厳密な順序で独自のデータセットを持たなければなりません。
この関数は、一度フォーマットされた文字列をプログラム内で再利用できるようにします。ここでは、操作ログに銘柄のプロパティを表示するためのテンプレートを作成する際に、この関数を使用します。最初の関数は文字列をフォーマットして返し、2番目の関数は結果の文字列を操作ログに表示します。
文字列の書式設定
文字列の書式設定ルールに慣れるには、PrintFormat()に関する記事のすべての指定子に関するセクションをご覧ください。データサイズを示す指定子(h | l | ll | I32 | I64)の動作を明確にするために、異なるサイズのデータの出力の違いを明確に示す次の関数を使うことができます。
//+------------------------------------------------------------------+ //| Script program start function | //+------------------------------------------------------------------+ void OnStart() { //--- long x = (long)0xFFFFFFFF * 1000; Print("\nDEC: ", typename(x)," x=",x,"\n---------"); Print("%hu: ", StringFormat("%hu",x)); Print("%u: ", StringFormat("%u",x)); Print("%I64u: ",StringFormat("%I64u",x)); Print("%llu: ", StringFormat("%llu",x)); Print("\nHEX: ", typename(x)," x=",StringFormat("%llx",x),"\n---------"); Print("%hx: ", StringFormat("%hx",x)); Print("%x: ", StringFormat("%x",x)); Print("%I64x: ",StringFormat("%I64x",x)); Print("%llx: ", StringFormat("%llx",x)); }
結果:
2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) DEC: long x=4294967295000 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) --------- 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) %hu: 64536 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) %u: 4294966296 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) %I64u: 4294967295000 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) %llu: 4294967295000 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) HEX: long x=3e7fffffc18 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) --------- 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) %hx: fc18 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) %x: fffffc18 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) %I64x: 3e7fffffc18 2023.07.12 17:48:12.920 PrinFormat (EURUSD,H1) %llx: 3e7fffffc18
これはおそらく、PrintFormat()に関する記事でカバーしきれなかった唯一の指定子でしょう。
銘柄プロパティのフォーマットされた出力
この記事では、各銘柄プロパティに対して2つの関数を書く必要があります。最初の関数は、文字列を表示に必要な形式に整形し、2番目の関数は、最初の関数が受け取った文字列を操作ログに表示します。PrintFormat()の記事で採用した文字列書式設定の原則に従うため、左端からの文字列インデントとヘッダーフィールドの幅も各関数に渡します。こうすることで、口座と銘柄のプロパティを記録する際の書式設定を統一することができます。
ミリ秒単位で時刻を取得するために、前回のPrintFormat()で説明した関数に基づいて、Date Time.Msc形式の時刻を文字列で返す関数を書いてみましょう。
//+------------------------------------------------------------------+ //| Accept a date in ms, return time in Date Time.Msc format | //+------------------------------------------------------------------+ string TimeMSC(const long time_msc) { return StringFormat("%s.%.3hu",string((datetime)time_msc / 1000),time_msc % 1000); /* Sample output: 2023.07.13 09:31:58.177 */ }
先に作成した関数とは異なり、ここでは日付と時刻だけをミリ秒単位で文字列にして返します。残りの書式設定は、この時間フォーマットでデータを表示する関数でおこなわれます。
それでは、整数、実数、文字列の銘柄プロパティの順に説明します。関数は、「銘柄のプロパティ」セクションで設定された順序で設定されます。
銘柄の整数プロパティの受信と表示 ENUM_SYMBOL_INFO_INTEGER
データ遅延(SYMBOL_SUBSCRIPTION_DELAY)
銘柄データは遅延して到着します。このプロパティは、気配値表示で選択された(SYMBOL_SELECT = true)銘柄に対してのみ要求できます。他の銘柄に対しては、ERR_MARKET_NOT_SELECTED (4302)エラーが発生します。
プロパティの文字列値をヘッダとともに返します。フォーマットされた文字列に対して、ヘッダーフィールドの左マージンと幅を指定することができます。
//+------------------------------------------------------------------+ //| Return the flag | //} of symbol data latency as a string | //+------------------------------------------------------------------+ string SymbolSubscriptionDelay(const string symbol,const uint header_width=0,const uint indent=0) { //--- Create a variable to store the error text //string error_str; //--- If symbol does not exist, return the error text if(!SymbolInfoInteger(symbol,SYMBOL_EXIST)) return StringFormat("%s: Error. Symbol '%s' is not exist",__FUNCTION__,symbol); //--- If a symbol is not selected in MarketWatch, try to select it if(!SymbolInfoInteger(symbol,SYMBOL_SELECT)) { //--- If unable to select a symbol in MarketWatch, return the error text if(!SymbolSelect(symbol,true)) return StringFormat("%s: Failed to select '%s' symbol in MarketWatch. Error %lu",__FUNCTION__,symbol,GetLastError()); } //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Subscription Delay:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,(bool)SymbolInfoInteger(symbol,SYMBOL_SUBSCRIPTION_DELAY) ? "Yes" : "No"); /* Sample output: Subscription Delay: No */ }
ここでは、データを要求する前に、まずそのような銘柄の存在を確認し、確認が成功すれば、気配値表示ウィンドウでその銘柄が選択されます。そのような銘柄がないか、選択できなかった場合、この関数はエラーテキストを返します。
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the flag | //| of the symbol data latency in the journal | //+------------------------------------------------------------------+ void SymbolSubscriptionDelayPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSubscriptionDelay(symbol,header_width,indent)); }
経済部門(SYMBOL_SECTOR)
銘柄が属する経済部門。
//+------------------------------------------------------------------+ //| Return the economic sector as a string | //| a symbol belongs to | //+------------------------------------------------------------------+ string SymbolSector(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get the value of the economic sector ENUM_SYMBOL_SECTOR symbol_sector=(ENUM_SYMBOL_SECTOR)SymbolInfoInteger(symbol,SYMBOL_SECTOR); //--- "Cut out" the sector name from the string obtained from enum string sector=StringSubstr(EnumToString(symbol_sector),7); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(sector.Lower()) sector.SetChar(0,ushort(sector.GetChar(0)-0x20)); //--- Replace all underscore characters with space in the resulting line StringReplace(sector,"_"," "); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Sector:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,sector); /* Sample output: Sector: Currency */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the Economy sector the symbol belongs to in the journal | //+------------------------------------------------------------------+ void SymbolSectorPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSector(symbol,header_width,indent)); }
業種(SYMBOL_INDUSTRY)
銘柄が属する産業または経済部門。
//+------------------------------------------------------------------+ //| Return the industry type or economic sector as a string | //| the symbol belongs to | //+------------------------------------------------------------------+ string SymbolIndustry(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get industry type value ENUM_SYMBOL_INDUSTRY symbol_industry=(ENUM_SYMBOL_INDUSTRY)SymbolInfoInteger(symbol,SYMBOL_INDUSTRY); //--- "Cut out" the industry type from the string obtained from enum string industry=StringSubstr(EnumToString(symbol_industry),9); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(industry.Lower()) industry.SetChar(0,ushort(industry.GetChar(0)-0x20)); //--- Replace all underscore characters with space in the resulting line StringReplace(industry,"_"," "); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Industry:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,industry); /* Sample output: Industry: Undefined */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the industry type or economic sector | //| the symbol belongs to | //+------------------------------------------------------------------+ void SymbolIndustryPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolIndustry(symbol,header_width,indent)); }
カスタム銘柄(SYMBOL_CUSTOM)
銘柄がカスタムであることを示すフラグで、他の気配値表示の銘柄や外部データソースを基に人工的に作成されたもの。
//+------------------------------------------------------------------+ //| Return the flag | //| of a custom symbol | //+------------------------------------------------------------------+ string SymbolCustom(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Custom symbol:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,(bool)SymbolInfoInteger(symbol,SYMBOL_CUSTOM) ? "Yes" : "No"); /* Sample output: Custom symbol: No */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the flag | //| of a custom symbol | //+------------------------------------------------------------------+ void SymbolCustomPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolCustom(symbol,header_width,indent)); }
気配値表示の背景色(SYMBOL_BACKGROUND_COLOR)
気配値表示での銘柄の背景色。
//+------------------------------------------------------------------+ //| Return the background color | //| used to highlight the Market Watch symbol as a string | //+------------------------------------------------------------------+ string SymbolBackgroundColor(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Background color:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the symbol background color in Market Watch color back_color=(color)SymbolInfoInteger(symbol,SYMBOL_BACKGROUND_COLOR); //--- Return the property value with a header having the required width and indentation //--- If a default color is set for a symbol (0xFF000000), return 'Default', otherwise - return its string description return StringFormat("%*s%-*s%-s",indent,"",w,header,back_color==0xFF000000 ? "Default" : ColorToString(back_color,true)); /* Sample output: Background color: Default */ }
背景色は完全に透明な色に設定でき、ColorToString()は黒(0, 0, 0)を返します。しかし、そうではありません。これはいわゆるデフォルトの色(0xFF000000 - CLR_DEFAULT)です。RGBはゼロで黒に相当しますが、完全に透明です。デフォルトの色にはアルファチャンネルがあり、背景を完全に透明(0xFF000000)に設定します。したがって、ColorToString()を使って色の名前を表示する前に、CLR_DEFAULTと等しいかどうかを確認します。
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Displays the background color | //| used to highlight the Market Watch symbol as a string | //+------------------------------------------------------------------+ void SymbolBackgroundColorPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolBackgroundColor(symbol,header_width,indent)); }
バーを構築するための価格(SYMBOL_CHART_MODE)
バーの生成に使用される価格タイプ(BidまたはLast)。
//+------------------------------------------------------------------+ //| Return the price type for building bars as a string | //+------------------------------------------------------------------+ string SymbolChartMode(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get price type used for generating bars ENUM_SYMBOL_CHART_MODE symbol_chart_mode=(ENUM_SYMBOL_CHART_MODE)SymbolInfoInteger(symbol,SYMBOL_CHART_MODE); //--- "Cut out" price type from the string obtained from enum string chart_mode=StringSubstr(EnumToString(symbol_chart_mode),18); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(chart_mode.Lower()) chart_mode.SetChar(0,ushort(chart_mode.GetChar(0)-0x20)); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Chart mode:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,chart_mode); /* Sample output: Chart mode: Bid */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Log the price type for building bars | //+------------------------------------------------------------------+ void SymbolChartModePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolChartMode(symbol,header_width,indent)); }
銘柄の存在(SYMBOL_EXIST)
この名前の銘柄が存在することを示すフラグ。
//+------------------------------------------------------------------+ //| Return the flag | //| indicating that a symbol with this name exists | //+------------------------------------------------------------------+ string SymbolExists(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Exists:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,(bool)SymbolInfoInteger(symbol,SYMBOL_EXIST) ? "Yes" : "No"); /* Sample output: Exists: Yes */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the flag | //| indicating that a symbol with this name exists | //+------------------------------------------------------------------+ void SymbolExistsPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolExists(symbol,header_width,indent)); }
気配値表示での選択(SYMBOL_SELECT)
気配値表示で銘柄が選択されていることを示すフラグ。
//+------------------------------------------------------------------+ //| Return the flag | //| indicating that the symbol is selected in Market Watch | //+------------------------------------------------------------------+ string SymbolSelected(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Selected:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,(bool)SymbolInfoInteger(symbol,SYMBOL_SELECT) ? "Yes" : "No"); /* Sample output: Selected: Yes */ }
操作ログ内の最初の関数の戻り値の表示
//+--------------------------------------------------------------------------------+ //| Display the flag, that the symbol is selected in Market Watch, in the journal | //+--------------------------------------------------------------------------------+ void SymbolSelectedPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSelected(symbol,header_width,indent)); }
気配値表示での表示(SYMBOL_VISIBLE)
銘柄が気配値表示に表示されていることを示すフラグ。
一部の銘柄(通常、預託通貨での必要証拠金と利益を計算するために必要なクロスレート)は自動的に選択されますが、気配値表示には表示されない場合があります。このような銘柄は、表示されるように明示的に選択されなければなりません。
//+------------------------------------------------------------------+ //| Return the flag | //| indicating that the symbol is visible in Market Watch | //+------------------------------------------------------------------+ string SymbolVisible(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Visible:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,(bool)SymbolInfoInteger(symbol,SYMBOL_VISIBLE) ? "Yes" : "No"); /* Sample output: Visible: Yes */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the flag | //| indicating that the symbol is visible in Market Watch | //+------------------------------------------------------------------+ void SymbolVisiblePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolVisible(symbol,header_width,indent)); }
現在のセッションの取引数(SYMBOL_SESSION_DEALS)
//+------------------------------------------------------------------+ //| Return the number of deals in the current session as a string | //+------------------------------------------------------------------+ string SymbolSessionDeals(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session deals:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-llu",indent,"",w,header,SymbolInfoInteger(symbol,SYMBOL_SESSION_DEALS)); /* Sample output: Session deals: 0 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the number of deals in the current session in the journal| //+------------------------------------------------------------------+ void SymbolSessionDealsPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionDeals(symbol,header_width,indent)); }
買い注文数(SYMBOL_SESSION_BUY_ORDERS)
現時点での買い注文の総数。
//+------------------------------------------------------------------+ //| Return the total number | //| of current buy orders as a string | //+------------------------------------------------------------------+ string SymbolSessionBuyOrders(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session Buy orders:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-llu",indent,"",w,header,SymbolInfoInteger(symbol,SYMBOL_SESSION_BUY_ORDERS)); /* Sample output: Session Buy orders: 0 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the total number of Buy orders at the moment | //+------------------------------------------------------------------+ void SymbolSessionBuyOrdersPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionBuyOrders(symbol,header_width,indent)); }
売り注文数(SYMBOL_SESSION_SELL_ORDERS)
現時点での売り注文の総数。
//+------------------------------------------------------------------+ //| Return the total number | //| of current sell orders as a string | //+------------------------------------------------------------------+ string SymbolSessionSellOrders(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session Sell orders:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-llu",indent,"",w,header,SymbolInfoInteger(symbol,SYMBOL_SESSION_BUY_ORDERS)); /* Sample output: Session Sell orders: 0 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the total number of Sell orders at the moment | //+------------------------------------------------------------------+ void SymbolSessionSellOrdersPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionSellOrders(symbol,header_width,indent)); }
直近の取引量(SYMBOL_VOLUME)
取引量 - 直近の取引量。
//+------------------------------------------------------------------+ //| Return the last trade volume as a string | //+------------------------------------------------------------------+ string SymbolVolume(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Volume:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-llu",indent,"",w,header,SymbolInfoInteger(symbol,SYMBOL_VOLUME)); /* Sample output: Volume: 0 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the last trade volume in the journal | //+------------------------------------------------------------------+ void SymbolVolumePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolVolume(symbol,header_width,indent)); }
1日の最大ボリューム(SYMBOL_VOLUMEHIGH)
//+------------------------------------------------------------------+ //| Return the maximum volume per day as a string | //+------------------------------------------------------------------+ string SymbolVolumeHigh(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Volume high:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-llu",indent,"",w,header,SymbolInfoInteger(symbol,SYMBOL_VOLUMEHIGH)); /* Sample output: Volume high: 0 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the maximum volume per day in the journal | //+------------------------------------------------------------------+ void SymbolVolumeHighPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolVolumeHigh(symbol,header_width,indent)); }
1日の最小ボリューム(SYMBOL_VOLUMELOW)
//+------------------------------------------------------------------+ //| Return the minimum volume per day as a string | //+------------------------------------------------------------------+ string SymbolVolumeLow(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Volume low:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-llu",indent,"",w,header,SymbolInfoInteger(symbol,SYMBOL_VOLUMELOW)); /* Sample output: Volume low: 0 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the minimum volume per day in the journal | //+------------------------------------------------------------------+ void SymbolVolumeLowPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolVolumeLow(symbol,header_width,indent)); }
最終クォート時刻(SYMBOL_TIME)
//+------------------------------------------------------------------+ //| Return the last quote time as a string | //+------------------------------------------------------------------+ string SymbolTime(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Time:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,(string)(datetime)SymbolInfoInteger(symbol,SYMBOL_TIME)); /* Sample output: Time: 2023.07.13 21:05:12 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the last quote time in the journal | //+------------------------------------------------------------------+ void SymbolTimePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTime(symbol,header_width,indent)); }
最終クォート時刻(ミリ秒単位) (SYMBOL_TIME_MSC)
1970.01.01からのミリ秒単位での最後のクォートの時間。
//+------------------------------------------------------------------+ //| Return the last quote time as a string in milliseconds | //+------------------------------------------------------------------+ string SymbolTimeMSC(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Time msc:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,TimeMSC(SymbolInfoInteger(symbol,SYMBOL_TIME_MSC))); /* Sample output: Time msc: 2023.07.13 21:09:24.327 */ }
ここでは、冒頭で設定したTimeMSC()関数を使用して、ミリ秒を含む日付時刻文字列を取得しています。
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the last quote time in milliseconds in the journal | //+------------------------------------------------------------------+ void SymbolTimeMSCPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTimeMSC(symbol,header_width,indent)); }
桁数(SYMBOL_DIGITS)
小数点以下の桁数。
//+------------------------------------------------------------------+ //| Return the number of decimal places as a string | //+------------------------------------------------------------------+ string SymbolDigits(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Digits:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-lu",indent,"",w,header,SymbolInfoInteger(symbol,SYMBOL_DIGITS)); /* Sample output: Digits: 5 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the number of decimal places in the journal | //+------------------------------------------------------------------+ void SymbolDigitsPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolDigits(symbol,header_width,indent)); }
スプレッド(SYMBOL_SPREAD)
ポイント単位のスプレッド値。
//+------------------------------------------------------------------+ //| Return the spread size in points as a string | //+------------------------------------------------------------------+ string SymbolSpread(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Spread:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-lu",indent,"",w,header,SymbolInfoInteger(symbol,SYMBOL_SPREAD)); /* Sample output: Spread: 7 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the spread size in points in the journal | //+------------------------------------------------------------------+ void SymbolSpreadPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSpread(symbol,header_width,indent)); }
浮動スプレッド(SYMBOL_SPREAD_FLOAT)
浮動スプレッドの表示
//+------------------------------------------------------------------+ //| Return the floating spread flag as a string | //+------------------------------------------------------------------+ string SymbolSpreadFloat(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Floating Spread:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,(bool)SymbolInfoInteger(symbol,SYMBOL_SPREAD_FLOAT) ? "Yes" : "No"); /* Sample output: Spread float: Yes */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the floating spread flag in the journal | //+------------------------------------------------------------------+ void SymbolSpreadFloatPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSpreadFloat(symbol,header_width,indent)); }
市場深度の要求数(SYMBOL_TICKS_BOOKDEPTH)
市場深度に表示される要求の最大数。要求キューを持たない商品の場合、値は0になります。
//+------------------------------------------------------------------+ //| Return the maximum number of | //| displayed market depth requests in the journal | //+------------------------------------------------------------------+ string SymbolTicksBookDepth(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Ticks book depth:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-lu",indent,"",w,header,SymbolInfoInteger(symbol,SYMBOL_TICKS_BOOKDEPTH)); /* Sample output: Ticks book depth: 10 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the maximum number of | //| displayed market depth requests in the journal | //+------------------------------------------------------------------+ void SymbolTicksBookDepthPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTicksBookDepth(symbol,header_width,indent)); }
契約価格計算モード(SYMBOL_TRADE_CALC_MODE)
//+------------------------------------------------------------------+ //| Return the contract price calculation mode as a string | //+------------------------------------------------------------------+ string SymbolTradeCalcMode(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get the contract price calculation mode value ENUM_SYMBOL_CALC_MODE symbol_trade_calc_mode=(ENUM_SYMBOL_CALC_MODE)SymbolInfoInteger(symbol,SYMBOL_TRADE_CALC_MODE); //--- "Cut out" the contract price calculation mode from the string obtained from enum string trade_calc_mode=StringSubstr(EnumToString(symbol_trade_calc_mode),17); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(trade_calc_mode.Lower()) trade_calc_mode.SetChar(0,ushort(trade_calc_mode.GetChar(0)-0x20)); //--- Replace all underscore characters with space in the resulting line StringReplace(trade_calc_mode,"_"," "); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Trade calculation mode:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,trade_calc_mode); /* Sample output: Trade calculation mode: Forex */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the contract price calculation mode in the journal | //+------------------------------------------------------------------+ void SymbolTradeCalcModePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeCalcMode(symbol,header_width,indent)); }
注文執行タイプ(SYMBOL_TRADE_MODE)
//+------------------------------------------------------------------+ //| Return the order execution type as a string | //+------------------------------------------------------------------+ string SymbolTradeMode(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get the contract price calculation mode value ENUM_SYMBOL_TRADE_MODE symbol_trade_mode=(ENUM_SYMBOL_TRADE_MODE)SymbolInfoInteger(symbol,SYMBOL_TRADE_MODE); //--- "Cut out" the contract price calculation mode from the string obtained from enum string trade_mode=StringSubstr(EnumToString(symbol_trade_mode),18); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(trade_mode.Lower()) trade_mode.SetChar(0,ushort(trade_mode.GetChar(0)-0x20)); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Trade mode:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,trade_mode); /* Sample output: Trade mode: Full */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the order execution type in the journal | //+------------------------------------------------------------------+ void SymbolTradeModePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeMode(symbol,header_width,indent)); }
取引開始日(SYMBOL_START_TIME)
銘柄の取引開始日(通常、先物に使用されます)。
//+------------------------------------------------------------------+ //| Return the trading start date for an instrument as a string | //+------------------------------------------------------------------+ string SymbolStartTime(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Start time:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the property value and define its description long time=SymbolInfoInteger(symbol,SYMBOL_START_TIME); string descr=(time==0 ? " (Not used)" : ""); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s%s",indent,"",w,header,(string)(datetime)time,descr); /* Sample output: Start time: 1970.01.01 00:00:00 (Not used) */ }
値が指定されていない(ゼロに等しい)場合は、日付1970.01.01 00:00:00として表示されます。これは少し混乱を招くかもしれません。したがって、その場合は日時の値の後に「Not used」と表示されます。
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the trading start date for an instrument in the journal | //+------------------------------------------------------------------+ void SymbolSymbolStartTimePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolStartTime(symbol,header_width,indent)); }
取引終了日
銘柄の取引終了日(通常、先物に使用されます)。
//+------------------------------------------------------------------+ //| Return the trading end date for an instrument as a string | //+------------------------------------------------------------------+ string SymbolExpirationTime(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Expiration time:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the property value and define its description long time=SymbolInfoInteger(symbol,SYMBOL_EXPIRATION_TIME); string descr=(time==0 ? " (Not used)" : ""); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s%s",indent,"",w,header,(string)(datetime)time,descr); /* Sample output: Expiration time: 1970.01.01 00:00:00 (Not used) */ }
値が指定されていない(ゼロに等しい)場合は、日付と時刻の値の後に「Not used」と表示されます。
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the trading end date for an instrument in the journal | //+------------------------------------------------------------------+ void SymbolSymbolExpirationTimePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolExpirationTime(symbol,header_width,indent)); }
ストップレベル(SYMBOL_TRADE_STOPS_LEVEL)
逆指値注文を設定するための、現在の終値からの最小距離。
//+-------------------------------------------------------------------------+ //| Return the minimum indentation | //| from the current close price in points to place Stop orders as a string | //+-------------------------------------------------------------------------+ string SymbolTradeStopsLevel(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Stops level:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the property value and define its description int stops_level=(int)SymbolInfoInteger(symbol,SYMBOL_TRADE_STOPS_LEVEL); string descr=(stops_level==0 ? " (By Spread)" : ""); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-lu%s",indent,"",w,header,stops_level,descr); /* Sample output: Stops level: 0 (By Spread) */ }
値がゼロの場合、これはストップの設定レベルがゼロであること、またはそれがないことを意味するものではありません。つまり、ストップレベルはスプレッドの値に依存します。通常、2 * スプレッドであるため、「By Spread」という説明項目が表示されます。
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the minimum indentation in points from the previous | //| close price for setting Stop orders in the journal | //+------------------------------------------------------------------+ void SymbolTradeStopsLevelPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeStopsLevel(symbol,header_width,indent)); }
フリーズレベル(SYMBOL_TRADE_FREEZE_LEVEL)
取引操作のための凍結距離(ポイント単位)。
//+------------------------------------------------------------------+ //| Return the distance of freezing | //| trading operations in points as a string | //+------------------------------------------------------------------+ string SymbolTradeFreezeLevel(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Freeze level:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the property value and define its description int freeze_level=(int)SymbolInfoInteger(symbol,SYMBOL_TRADE_FREEZE_LEVEL); string descr=(freeze_level==0 ? " (Not used)" : ""); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-lu%s",indent,"",w,header,freeze_level,descr); /* Sample output: Freeze level: 0 (Not used) */ }
値がゼロの場合、これはフリーズレベルが使用されていないことを意味します。
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------------------+ //| Display the distance of freezing trading operations in points in the journal | //+------------------------------------------------------------------------------+ void SymbolTradeFreezeLevelPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeFreezeLevel(symbol,header_width,indent)); }
取引執行モード(SYMBOL_TRADE_EXEMODE)
//+------------------------------------------------------------------+ //| Return the trade execution mode as a string | //+------------------------------------------------------------------+ string SymbolTradeExeMode(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get the contract price calculation mode value ENUM_SYMBOL_TRADE_EXECUTION symbol_trade_exemode=(ENUM_SYMBOL_TRADE_EXECUTION)SymbolInfoInteger(symbol,SYMBOL_TRADE_EXEMODE); //--- "Cut out" the contract price calculation mode from the string obtained from enum string trade_exemode=StringSubstr(EnumToString(symbol_trade_exemode),23); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(trade_exemode.Lower()) trade_exemode.SetChar(0,ushort(trade_exemode.GetChar(0)-0x20)); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Trade Execution mode:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,trade_exemode); /* Sample output: Trade Execution mode: Instant */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the trade execution mode in the journal | //+------------------------------------------------------------------+ void SymbolTradeExeModePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeExeMode(symbol,header_width,indent)); }
スワップ計算モデル(SYMBOL_SWAP_MODE)
//+------------------------------------------------------------------+ //| Return the swap calculation model as a string | //+------------------------------------------------------------------+ string SymbolSwapMode(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get the value of the swap calculation model ENUM_SYMBOL_SWAP_MODE symbol_swap_mode=(ENUM_SYMBOL_SWAP_MODE)SymbolInfoInteger(symbol,SYMBOL_SWAP_MODE); //--- "Cut out" the swap calculation model from the string obtained from enum string swap_mode=StringSubstr(EnumToString(symbol_swap_mode),17); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(swap_mode.Lower()) swap_mode.SetChar(0,ushort(swap_mode.GetChar(0)-0x20)); //--- Replace all underscore characters with space in the resulting line StringReplace(swap_mode,"_"," "); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Swap mode:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,swap_mode); /* Sample output: Swap mode: Points */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the swap calculation model to the journal | //+------------------------------------------------------------------+ void SymbolSwapModePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapMode(symbol,header_width,indent)); }
トリプルスワップ発生日(SYMBOL_SWAP_ROLLOVER3DAYS)
トリプルスワップを計算する曜日。
//+--------------------------------------------------------------------+ //| Return the day of the week for calculating triple swap as a string | //+--------------------------------------------------------------------+ string SymbolSwapRollover3Days(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get the value of the swap calculation model ENUM_DAY_OF_WEEK symbol_swap_rollover3days=(ENUM_DAY_OF_WEEK)SymbolInfoInteger(symbol,SYMBOL_SWAP_ROLLOVER3DAYS); //--- Convert enum into a string with the name of the day string swap_rollover3days=EnumToString(symbol_swap_rollover3days); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(swap_rollover3days.Lower()) swap_rollover3days.SetChar(0,ushort(swap_rollover3days.GetChar(0)-0x20)); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Swap Rollover 3 days:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,swap_rollover3days); /* Sample output: Swap Rollover 3 days: Wednesday */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------------+ //| Display the day of the week for calculating triple swap in the journal | //+------------------------------------------------------------------------+ void SymbolSwapRollover3DaysPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapRollover3Days(symbol,header_width,indent)); }
ヘッジ証拠金計算モード(SYMBOL_MARGIN_HEDGED_USE_LEG)
大きい方の足(買いまたは売り)を使ってヘッジ証拠金を計算します。
//+------------------------------------------------------------------+ //| Return the calculation mode | //| of hedged margin using the larger leg as a string | //+------------------------------------------------------------------+ string SymbolMarginHedgedUseLeg(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Margin hedged use leg:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,(bool)SymbolInfoInteger(symbol,SYMBOL_MARGIN_HEDGED_USE_LEG) ? "Yes" : "No"); /* Sample output: Margin hedged use leg: No */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the mode for calculating the hedged | //| margin using the larger leg in the journal | //+------------------------------------------------------------------+ void SymbolMarginHedgedUseLegPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolMarginHedgedUseLeg(symbol,header_width,indent)); }
注文の期限切れモードのフラグ(SYMBOL_EXPIRATION_MODE)
銘柄に対してSYMBOL_EXPIRATION_SPECIFIEDフラグが指定されている場合、未決注文を送信する際に、この未決注文がどの時点まで有効かを具体的に示すことができます。
//+------------------------------------------------------------------+ //| Return the flags of allowed order expiration modes as a string | //+------------------------------------------------------------------+ string SymbolExpirationMode(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get order expiration modes int exp_mode=(int)SymbolInfoInteger(symbol,SYMBOL_EXPIRATION_MODE); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Expiration mode:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Parse mode flags into components string flags=""; if((exp_mode&SYMBOL_EXPIRATION_GTC)==SYMBOL_EXPIRATION_GTC) flags+=(flags.Length()>0 ? "|" : "")+"GTC"; if((exp_mode&SYMBOL_EXPIRATION_DAY)==SYMBOL_EXPIRATION_DAY) flags+=(flags.Length()>0 ? "|" : "")+"DAY"; if((exp_mode&SYMBOL_EXPIRATION_SPECIFIED)==SYMBOL_EXPIRATION_SPECIFIED) flags+=(flags.Length()>0 ? "|" : "")+"SPECIFIED"; if((exp_mode&SYMBOL_EXPIRATION_SPECIFIED_DAY)==SYMBOL_EXPIRATION_SPECIFIED_DAY) flags+=(flags.Length()>0 ? "|" : "")+"SPECIFIED_DAY"; //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,flags); /* Sample output: Expiration mode: GTC|DAY|SPECIFIED */ }
操作ログ内の最初の関数の戻り値の表示
//+--------------------------------------------------------------------+ //| Display the flags of allowed order expiration modes in the journal | //+--------------------------------------------------------------------+ void SymbolExpirationModePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolExpirationMode(symbol,header_width,indent)); }
注文フィルモードのフラグ(SYMBOL_FILLING_MODE)
許容される注文フィルモードのフラグ。
注文を送信する際、注文に設定されたボリュームのフィルポリシーを指定することができます。フラグの組み合わせにより、各商品に複数のモードを設定できます。フラグの組み合わせは、論理和(|)演算で表されます(例:SYMBOL_FILLING_FOK|SYMBOL_FILLING_IOC)。ORDER_FILLING_RETURNフィルタイプは、「市場執行」(SYMBOL_TRADE_EXECUTION_MARKET)を除くすべての執行モードで有効です。//+------------------------------------------------------------------+ //| Return the flags of allowed order filling modes as a string | //+------------------------------------------------------------------+ string SymbolFillingMode(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get order filling modes int fill_mode=(int)SymbolInfoInteger(symbol,SYMBOL_FILLING_MODE); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Filling mode:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Parse mode flags into components string flags=""; if((fill_mode&ORDER_FILLING_FOK)==ORDER_FILLING_FOK) flags+=(flags.Length()>0 ? "|" : "")+"FOK"; if((fill_mode&ORDER_FILLING_IOC)==ORDER_FILLING_IOC) flags+=(flags.Length()>0 ? "|" : "")+"IOC"; if((fill_mode&ORDER_FILLING_BOC)==ORDER_FILLING_BOC) flags+=(flags.Length()>0 ? "|" : "")+"BOC"; if(SymbolInfoInteger(symbol,SYMBOL_TRADE_EXEMODE)!=SYMBOL_TRADE_EXECUTION_MARKET) flags+=(flags.Length()>0 ? "|" : "")+"RETURN"; //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,flags); /* Sample output: Filling mode: FOK|IOC|RETURN */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the flags of allowed order filling modes in the journal | //+------------------------------------------------------------------+ void SymbolFillingModePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolFillingMode(symbol,header_width,indent)); }
許可される注文タイプのフラグ(SYMBOL_ORDER_MODE)
//+------------------------------------------------------------------+ //| Return flags of allowed order types as a string | //+------------------------------------------------------------------+ string SymbolOrderMode(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get order types int order_mode=(int)SymbolInfoInteger(symbol,SYMBOL_ORDER_MODE); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Order mode:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Parse type flags into components string flags=""; if((order_mode&SYMBOL_ORDER_MARKET)==SYMBOL_ORDER_MARKET) flags+=(flags.Length()>0 ? "|" : "")+"MARKET"; if((order_mode&SYMBOL_ORDER_LIMIT)==SYMBOL_ORDER_LIMIT) flags+=(flags.Length()>0 ? "|" : "")+"LIMIT"; if((order_mode&SYMBOL_ORDER_STOP)==SYMBOL_ORDER_STOP) flags+=(flags.Length()>0 ? "|" : "")+"STOP"; if((order_mode&SYMBOL_ORDER_STOP_LIMIT )==SYMBOL_ORDER_STOP_LIMIT ) flags+=(flags.Length()>0 ? "|" : "")+"STOP_LIMIT"; if((order_mode&SYMBOL_ORDER_SL)==SYMBOL_ORDER_SL) flags+=(flags.Length()>0 ? "|" : "")+"SL"; if((order_mode&SYMBOL_ORDER_TP)==SYMBOL_ORDER_TP) flags+=(flags.Length()>0 ? "|" : "")+"TP"; if((order_mode&SYMBOL_ORDER_CLOSEBY)==SYMBOL_ORDER_CLOSEBY) flags+=(flags.Length()>0 ? "|" : "")+"CLOSEBY"; //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,flags); /* Sample output: Order mode: MARKET|LIMIT|STOP|STOP_LIMIT|SL|TP|CLOSEBY */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the flags of allowed order types in the journal | //+------------------------------------------------------------------+ void SymbolOrderModePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolOrderMode(symbol,header_width,indent)); }
ストップロスとテイクプロフィットの有効期間(SYMBOL_ORDER_GTC_MODE)
SYMBOL_EXPIRATION_MODE=SYMBOL_EXPIRATION_GTC(キャンセルされるまで有効)の場合、StopLoss注文とTakeProfit注文の有効期限。
//+------------------------------------------------------------------+ //| Return StopLoss and TakeProfit validity periods as a string | //+------------------------------------------------------------------+ string SymbolOrderGTCMode(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get the value of the validity period of pending, StopLoss and TakeProfit orders ENUM_SYMBOL_ORDER_GTC_MODE symbol_order_gtc_mode=(ENUM_SYMBOL_ORDER_GTC_MODE)SymbolInfoInteger(symbol,SYMBOL_ORDER_GTC_MODE); //--- Set the validity period description as 'GTC' string gtc_mode="GTC"; //--- If the validity period is not GTC if(symbol_order_gtc_mode!=SYMBOL_ORDERS_GTC) { //--- "Cut out" pending, StopLoss and TakeProfit order validity periods from the string obtained from enum StringSubstr(EnumToString(symbol_order_gtc_mode),14); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(gtc_mode.Lower()) gtc_mode.SetChar(0,ushort(gtc_mode.GetChar(0)-0x20)); //--- Replace all underscore characters with space in the resulting line StringReplace(gtc_mode,"_"," "); } //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Order GTC mode:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,gtc_mode); /* Sample output: Order GTC mode: GTC */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display StopLoss and TakeProfit validity periods in the journal | //+------------------------------------------------------------------+ void SymbolOrderGTCModePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolOrderGTCMode(symbol,header_width,indent)); }
オプションタイプ(SYMBOL_OPTION_MODE)
//+------------------------------------------------------------------+ //| Return option type as a string | //+------------------------------------------------------------------+ string SymbolOptionMode(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get the value of the option type model ENUM_SYMBOL_OPTION_MODE symbol_option_mode=(ENUM_SYMBOL_OPTION_MODE)SymbolInfoInteger(symbol,SYMBOL_OPTION_MODE); //--- "Cut out" the option type from the string obtained from enum string option_mode=StringSubstr(EnumToString(symbol_option_mode),19); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(option_mode.Lower()) option_mode.SetChar(0,ushort(option_mode.GetChar(0)-0x20)); //--- Replace all underscore characters with space in the resulting line StringReplace(option_mode,"_"," "); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Option mode:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,option_mode); /* Sample output: Option mode: European */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the option type in the journal | //+------------------------------------------------------------------+ void SymbolOptionModePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolOptionMode(symbol,header_width,indent)); }
オプション権(SYMBOL_OPTION_RIGHT)
//+------------------------------------------------------------------+ //| Return the option right as a string | //+------------------------------------------------------------------+ string SymbolOptionRight(const string symbol,const uint header_width=0,const uint indent=0) { //--- Get the option right value ENUM_SYMBOL_OPTION_RIGHT symbol_option_right=(ENUM_SYMBOL_OPTION_RIGHT)SymbolInfoInteger(symbol,SYMBOL_OPTION_RIGHT); //--- "Cut out" the option right from the string obtained from enum string option_right=StringSubstr(EnumToString(symbol_option_right),20); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(option_right.Lower()) option_right.SetChar(0,ushort(option_right.GetChar(0)-0x20)); //--- Replace all underscore characters with space in the resulting line StringReplace(option_right,"_"," "); //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Option right:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-s",indent,"",w,header,option_right); /* Sample output: Option right: Call */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the option right in the journal | //+------------------------------------------------------------------+ void SymbolOptionRightPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolOptionRight(symbol,header_width,indent)); }
銘柄の実数プロパティの受信と表示 ENUM_SYMBOL_INFO_DOUBLE
買値(SYMBOL_BID)
買値 - 商品を売ることができる最良の価格。
//+------------------------------------------------------------------+ //| Return the Bid price as a string | //+------------------------------------------------------------------+ string SymbolBid(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Bid:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_BID)); /* Sample output: Bid: 1.31017 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the Bid price in the journal | //+------------------------------------------------------------------+ void SymbolBidPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolBid(symbol,header_width,indent)); }
当日の最高買値(SYMBOL_BIDHIGH)
//+------------------------------------------------------------------+ //| Return the maximum Bid per day as a string | //+------------------------------------------------------------------+ string SymbolBidHigh(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Bid High:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_BIDHIGH)); /* Sample output: Bid High: 1.31422 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the maximum Bid per day in the journal | //+------------------------------------------------------------------+ void SymbolBidHighPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolBidHigh(symbol,header_width,indent)); }
当日の最低買値(SYMBOL_BIDLOW)
//+------------------------------------------------------------------+ //| Return the minimum Bid per day as a string | //+------------------------------------------------------------------+ string SymbolBidLow(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Bid Low:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_BIDLOW)); /* Sample output: Bid Low: 1.30934 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the minimum Bid per day in the journal | //+------------------------------------------------------------------+ void SymbolBidLowPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolBidLow(symbol,header_width,indent)); }
売値(SYMBOL_ASK)
売値 - その商品を買うことができる最良の価格
//+------------------------------------------------------------------+ //| Return the Ask price as a string | //+------------------------------------------------------------------+ string SymbolAsk(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Ask:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_ASK)); /* Sample output: Ask: 1.31060 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the Ask price in the journal | //+------------------------------------------------------------------+ void SymbolAskPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolAsk(symbol,header_width,indent)); }
当日の最高売値(SYMBOL_ASKHIGH)
//+------------------------------------------------------------------+ //| Return the maximum Ask per day as a string | //+------------------------------------------------------------------+ string SymbolAskHigh(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Ask High:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_ASKHIGH)); /* Sample output: Ask High: 1.31427 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the maximum Ask per day in the journal | //+------------------------------------------------------------------+ void SymbolAskHighPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolAskHigh(symbol,header_width,indent)); }
当日の最低売値(SYMBOL_ASKLOW)
//+------------------------------------------------------------------+ //| Return the minimum Ask per day as a string | //+------------------------------------------------------------------+ string SymbolAskLow(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Ask Low:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_ASKLOW)); /* Sample output: Ask Low: 1.30938 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the minimum Ask per day in the journal | //+------------------------------------------------------------------+ void SymbolAskLowPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolAskLow(symbol,header_width,indent)); }
最終価格(SYMBOL_LAST)
最後の取引がおこなわれた価格。
//+------------------------------------------------------------------+ //| Return the Last price as a string | //+------------------------------------------------------------------+ string SymbolLast(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Last:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_LAST)); /* Sample output: Last: 0.00000 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the Last price in the journal | //+------------------------------------------------------------------+ void SymbolLastPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolLast(symbol,header_width,indent)); }
当日の最高最終価格(SYMBOL_LASTHIGH)
//+------------------------------------------------------------------+ //| Return the maximum Last per day as a string | //+------------------------------------------------------------------+ string SymbolLastHigh(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Last High:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_LASTHIGH)); /* Sample output: Last High: 0.00000 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the maximum Last per day in the journal | //+------------------------------------------------------------------+ void SymbolLastHighPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolLastHigh(symbol,header_width,indent)); }
当日の最低最終価格(SYMBOL_LASTLOW)
//+------------------------------------------------------------------+ //| Return the minimum Last per day as a string | //+------------------------------------------------------------------+ string SymbolLastLow(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Last Low:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_LASTLOW)); /* Sample output: Last Low: 0.00000 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the minimum Last per day in the journal | //+------------------------------------------------------------------+ void SymbolLastLowPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolLastLow(symbol,header_width,indent)); }
最終取引量(SYMBOL_VOLUME_REAL)
最終約定取引量
//+------------------------------------------------------------------+ //| Return the last executed trade volume as a string | //+------------------------------------------------------------------+ string SymbolVolumeReal(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Volume real:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_VOLUME_REAL)); /* Sample output: Volume real: 0.00 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the last executed trade volume in the journal | //+------------------------------------------------------------------+ void SymbolVolumeRealPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolVolumeReal(symbol,header_width,indent)); }
1日の最大ボリューム(SYMBOL_VOLUMEHIGH_REAL)
//+------------------------------------------------------------------+ //| Return the maximum volume per day as a string | //+------------------------------------------------------------------+ string SymbolVolumeHighReal(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Volume High real:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_VOLUMEHIGH_REAL)); /* Sample output: Volume High real: 0.00 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the maximum volume per day in the journal | //+------------------------------------------------------------------+ void SymbolVolumeHighRealPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolVolumeHighReal(symbol,header_width,indent)); }
1日の最小ボリューム(SYMBOL_VOLUMELOW_REAL)
//+------------------------------------------------------------------+ //| Return the minimum volume per day as a string | //+------------------------------------------------------------------+ string SymbolVolumeLowReal(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Volume Low real:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_VOLUMELOW_REAL)); /* Sample output: Volume Low real: 0.00 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the minimum volume per day in the journal | //+------------------------------------------------------------------+ void SymbolVolumeLowRealPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolVolumeLowReal(symbol,header_width,indent)); }
オプション執行価格(SYMBOL_OPTION_STRIKE)
オプション行使価格。これは、オプションの買い手が原資産を買い(コールオプション)または売り(プットオプション)、オプションの売り手は、それに応じて、原資産の対応する量を売却または購入する義務を負うことができる価格です。
//+------------------------------------------------------------------+ //| Return the strike price as a string | //+------------------------------------------------------------------+ string SymbolOptionStrike(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Option strike:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_OPTION_STRIKE)); /* Sample output: Option strike: 0.00000 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the option strike price in the journal | //+------------------------------------------------------------------+ void SymbolOptionStrikePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolOptionStrike(symbol,header_width,indent)); }
ポイント(SYMBOL_POINT)
ポイント。
//+------------------------------------------------------------------+ //| Return the point value as a string | //+------------------------------------------------------------------+ string SymbolPoint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Point:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_POINT)); /* Sample output: Point: 0.00001 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the point value in the journal | //+------------------------------------------------------------------+ void SymbolPointPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPoint(symbol,header_width,indent)); }
ティック価格(SYMBOL_TRADE_TICK_VALUE)
SYMBOL_TRADE_TICK_VALUE_PROFIT - 勝ちポジションの計算ティック値。
//+------------------------------------------------------------------+ //| Return the tick price as a string | //+------------------------------------------------------------------+ string SymbolTradeTickValue(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Tick value:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_TRADE_TICK_VALUE)); /* Sample output: Tick value: 1.00000 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the tick price in the journal | //+------------------------------------------------------------------+ void SymbolTradeTickValuePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeTickValue(symbol,header_width,indent)); }
勝ちポジションのティック価格(SYMBOL_TRADE_TICK_VALUE_PROFIT)
勝ちポジションの計算されたティック値。
//+------------------------------------------------------------------+ //| Return the calculated tick price as a string | //| for a profitable position | //+------------------------------------------------------------------+ string SymbolTradeTickValueProfit(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Tick value profit:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_TRADE_TICK_VALUE_PROFIT)); /* Sample output: Tick value profit: 1.00000 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the calculated tick price in the journal | //| for a profitable position | //+------------------------------------------------------------------+ void SymbolTradeTickValueProfitPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeTickValueProfit(symbol,header_width,indent)); }
負けポジションのティック価格(SYMBOL_TRADE_TICK_VALUE_LOSS)
負けポジションの計算されたティック値を。
//+------------------------------------------------------------------+ //| Return the calculated tick price as a string | //| for a losing position | //+------------------------------------------------------------------+ string SymbolTradeTickValueLoss(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Tick value loss:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_TRADE_TICK_VALUE_LOSS)); /* Sample output: Tick value loss: 1.00000 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the calculated tick price in the journal | //| for a losing position | //+------------------------------------------------------------------+ void SymbolTradeTickValueLossPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeTickValueLoss(symbol,header_width,indent)); }
最小価格変動(SYMBOL_TRADE_TICK_SIZE)
//+------------------------------------------------------------------+ //| Return the minimum price change as a string | //+------------------------------------------------------------------+ string SymbolTradeTickSize(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Tick size:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_TRADE_TICK_SIZE)); /* Sample output: Tick size: 0.00001 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the minimum price change in the journal | //+------------------------------------------------------------------+ void SymbolTradeTickSizePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeTickSize(symbol,header_width,indent)); }
約定サイズ(SYMBOL_TRADE_CONTRACT_SIZE)
//+------------------------------------------------------------------+ //| Return the trading contract size as a string | //+------------------------------------------------------------------+ string SymbolTradeContractSize(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Contract size:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f %s",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_TRADE_CONTRACT_SIZE),SymbolInfoString(symbol,SYMBOL_CURRENCY_BASE)); /* Sample output: Contract size: 100000.00 GBP */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the trading contract size in the journal | //+------------------------------------------------------------------+ void SymbolTradeContractSizePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeContractSize(symbol,header_width,indent)); }
未収利息(SYMBOL_TRADE_ACCRUED_INTEREST)
未収利息 - 累積クーポン利息(クーポン債発行または最後のクーポン利息支払いからの日数に比例して計算されるクーポン利息の一部)。
//+------------------------------------------------------------------+ //| Return accrued interest as a string | //+------------------------------------------------------------------+ string SymbolTradeAccruedInterest(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Accrued interest:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_TRADE_ACCRUED_INTEREST)); /* Sample output: Accrued interest: 0.00 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display accrued interest in the journal | //+------------------------------------------------------------------+ void SymbolTradeAccruedInterestPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeAccruedInterest(symbol,header_width,indent)); }
額面金額(SYMBOL_TRADE_FACE_VALUE)
額面金額 - 発行体によって設定される債券の当初価値。
//+------------------------------------------------------------------+ //| Return the face value as a string | //+------------------------------------------------------------------+ string SymbolTradeFaceValue(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Face value:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_TRADE_FACE_VALUE)); /* Sample output: Face value: 0.00 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the face value in the journal | //+------------------------------------------------------------------+ void SymbolTradeFaceValuePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeFaceValue(symbol,header_width,indent)); }
流動性率(SYMBOL_TRADE_LIQUIDITY_RATE)
流動性率とは、証拠金に使用できる資産の割合です。
//+------------------------------------------------------------------+ //| Return the liquidity rate as a string | //+------------------------------------------------------------------+ string SymbolTradeLiquidityRate(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Liquidity rate:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_TRADE_LIQUIDITY_RATE)); /* Sample output: Liquidity rate: 0.00 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the rate liquidity in the journal | //+------------------------------------------------------------------+ void SymbolTradeLiquidityRatePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolTradeLiquidityRate(symbol,header_width,indent)); }
最小ボリューム(SYMBOL_VOLUME_MIN)
取引実行のための最小取引量。
//+------------------------------------------------------------------+ //| Return the minimum volume as a string | //+------------------------------------------------------------------+ string SymbolVolumeMin(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Volume min:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places in the lot value int dg=(int)ceil(fabs(log10(SymbolInfoDouble(symbol,SYMBOL_VOLUME_STEP)))); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_VOLUME_MIN)); /* Sample output: Volume min: 0.01 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the minimum volume in the journal | //+------------------------------------------------------------------+ void SymbolVolumeMinPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolVolumeMin(symbol,header_width,indent)); }
最大ボリューム(SYMBOL_VOLUME_MAX)
取引実行のための最大取引量。
//+------------------------------------------------------------------+ //| Return the maximum volume as a string | //+------------------------------------------------------------------+ string SymbolVolumeMax(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Volume max:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places in the lot value int dg=(int)ceil(fabs(log10(SymbolInfoDouble(symbol,SYMBOL_VOLUME_STEP)))); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_VOLUME_MAX)); /* Sample output: Volume max: 500.00 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the maximum volume in the journal | //+------------------------------------------------------------------+ void SymbolVolumeMaxPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolVolumeMax(symbol,header_width,indent)); }
ボリューム変更ステップ(SYMBOL_VOLUME_STEP)
取引実行のための最小限の数量変更ステップ。
//+------------------------------------------------------------------+ //| Return the minimum volume change step as a string | //+------------------------------------------------------------------+ string SymbolVolumeStep(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Volume step:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places in the lot value int dg=(int)ceil(fabs(log10(SymbolInfoDouble(symbol,SYMBOL_VOLUME_STEP)))); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_VOLUME_STEP)); /* Sample output: Volume step: 0.01 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the minimum volume change step in the journal | //+------------------------------------------------------------------+ void SymbolVolumeStepPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolVolumeStep(symbol,header_width,indent)); }
一方向のポジションと注文の最大ボリューム(SYMBOL_VOLUME_LIMIT)
この銘柄のポジションと一方向(買いまたは売り)の未決注文の最大許容合計ボリューム。たとえば、上限が5ロットの場合、5ロットのポジションがあって5ロットの売り指値注文もおこなうことができます。ただし、この場合、買い指値注文を出すことはできません(一方向の総量が指値を超えるため)。また、5ロットを超える売り指値注文を出すことはできません。
//+----------------------------------------------------------------------------+ //| Return the maximum acceptable | //| total volume of open position and pending orders for a symbol as a string | //+----------------------------------------------------------------------------+ string SymbolVolumeLimit(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Volume limit:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places in the lot value int dg=(int)ceil(fabs(log10(SymbolInfoDouble(symbol,SYMBOL_VOLUME_STEP)))); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_VOLUME_LIMIT)); /* Sample output: Volume limit: 0.00 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------------------+ //| Display the maximum acceptable | //| total volume of open position and pending orders for a symbol in the journal | //+------------------------------------------------------------------------------+ void SymbolVolumeLimitPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolVolumeLimit(symbol,header_width,indent)); }
買いスワップ(SYMBOL_SWAP_LONG)
//+------------------------------------------------------------------+ //| Return the Buy swap value as a string | //+------------------------------------------------------------------+ string SymbolSwapLong(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Swap long:"; uint w=(header_width==0 ? header.Length()+1 : header_width-1); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%- .2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_SWAP_LONG)); /* Sample output: Swap long: -0.20 */ }
プロパティの値は負になることがあるので、負のプロパティ値を1文字左にずらし、マイナス記号がリストの残りのプロパティ値の列の左に来るようにする必要があります。これを実現するには、渡されたフィールドの幅を1減らし、フォーマット指定子の中にスペースを入れます。これは、負でない値の場合、文字列を1文字分右にシフトします。このように、フィールド幅を1つ減らし、一方、負でない値には1つのスペースが追加され、フィールド幅を1文字減らすことで平準化します。
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the Buy swap value in the journal | //+------------------------------------------------------------------+ void SymbolSwapLongPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapLong(symbol,header_width,indent)); }
売りスワップ(SYMBOL_SWAP_SHORT)
//+------------------------------------------------------------------+ //| Return the Sell swap value as a string | //+------------------------------------------------------------------+ string SymbolSwapShort(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Swap short:"; uint w=(header_width==0 ? header.Length()+1 : header_width-1); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%- .2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_SWAP_SHORT)); /* Sample output: Swap short: -2.20 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the Sell swap value in the journal | //+------------------------------------------------------------------+ void SymbolSwapShortPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapShort(symbol,header_width,indent)); }
スワップ発生率(SYMBOL_SWAP_SUNDAY)
日曜日から翌日にロールオーバーされたオーバーナイトポジションのスワップ計算比率(SYMBOL_SWAP_LONGまたはSYMBOL_SWAP_SHORT)。以下の値がサポートされています。
0 - スワップはチャージされない
1 - シングルスワップ
3 - トリプルスワップ
//+------------------------------------------------------------------+ //| Return the swap accrual ratio as a string | //| when swapping a position from Sunday to Monday | //+------------------------------------------------------------------+ string SymbolSwapSunday(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Swap sunday:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the ratio and define its description string double swap_coeff=SymbolInfoDouble(symbol,SYMBOL_SWAP_SUNDAY); string coeff_descr=(swap_coeff==1 ? "single swap" : swap_coeff==3 ? "triple swap" : "no swap is charged"); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%.0f (%s)",indent,"",w,header,swap_coeff,coeff_descr); /* Sample output: Swap sunday: 0 (no swap is charged) */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the swap accrual ratio in the journal | //| when swapping a position from Sunday to Monday | //+------------------------------------------------------------------+ void SymbolSwapSundayPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapSunday(symbol,header_width,indent)); }
月曜日から火曜日へのスワップ発生率(SYMBOL_SWAP_MONDAY)
月曜日から火曜日にロールオーバーされたオーバーナイトポジションのスワップ計算比率(SYMBOL_SWAP_LONGまたはSYMBOL_SWAP_SHORT)。
//+------------------------------------------------------------------+ //| Return the swap accrual ratio as a string | //| when swapping a position from Monday to Tuesday | //+------------------------------------------------------------------+ string SymbolSwapMonday(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Swap monday:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the ratio and define its description string double swap_coeff=SymbolInfoDouble(symbol,SYMBOL_SWAP_MONDAY); string coeff_descr=(swap_coeff==1 ? "single swap" : swap_coeff==3 ? "triple swap" : "no swap is charged"); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%.0f (%s)",indent,"",w,header,swap_coeff,coeff_descr); /* Sample output: Swap monday: 1 (single swap) */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the swap accrual ratio in the journal | //| when swapping a position from Monday to Tuesday | //+------------------------------------------------------------------+ void SymbolSwapMondayPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapMonday(symbol,header_width,indent)); }
火曜日から水曜日へのスワップ発生率(SYMBOL_SWAP_TUESDAY)
火曜日から水曜日にロールオーバーされたオーバーナイトポジションのスワップ計算比率(SYMBOL_SWAP_LONG または SYMBOL_SWAP_SHORT
//+------------------------------------------------------------------+ //| Return the swap accrual ratio as a string | //| when swapping a position from Tuesday to Wednesday | //+------------------------------------------------------------------+ string SymbolSwapTuesday(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Swap Tuesday:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the ratio and define its description string double swap_coeff=SymbolInfoDouble(symbol,SYMBOL_SWAP_TUESDAY); string coeff_descr=(swap_coeff==1 ? "single swap" : swap_coeff==3 ? "triple swap" : "no swap is charged"); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%.0f (%s)",indent,"",w,header,swap_coeff,coeff_descr); /* Sample output: Swap Tuesday: 1 (single swap) */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the swap accrual ratio in the journal | //| when swapping a position from Tuesday to Wednesday | //+------------------------------------------------------------------+ void SymbolSwapTuesdayPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapTuesday(symbol,header_width,indent)); }
水曜日から木曜日へのスワップ発生率(SYMBOL_SWAP_WEDNESDAY)
水曜日から木曜日にロールオーバーされたオーバーナイトポジションのスワップ計算比率(SYMBOL_SWAP_LONG または SYMBOL_SWAP_SHORT
//+------------------------------------------------------------------+ //| Return the swap accrual ratio as a string | //| when swapping a position from Wednesday to Thursday | //+------------------------------------------------------------------+ string SymbolSwapWednesday(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Swap Wednesday:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the ratio and define its description string double swap_coeff=SymbolInfoDouble(symbol,SYMBOL_SWAP_WEDNESDAY); string coeff_descr=(swap_coeff==1 ? "single swap" : swap_coeff==3 ? "triple swap" : "no swap is charged"); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%.0f (%s)",indent,"",w,header,swap_coeff,coeff_descr); /* Sample output: Swap Wednesday: 3 (triple swap) */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the swap accrual ratio in the journal | //| when swapping a position from Wednesday to Thursday | //+------------------------------------------------------------------+ void SymbolSwapWednesdayPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapWednesday(symbol,header_width,indent)); }
木曜日から金曜日へのスワップ発生率(SYMBOL_SWAP_THURSDAY)
木曜日から金曜日にロールオーバーされたオーバーナイトポジションのスワップ計算比率(SYMBOL_SWAP_LONGまたはSYMBOL_SWAP_SHORT)。
//+------------------------------------------------------------------+ //| Return the swap accrual ratio as a string | //| when swapping a position from Thursday to Friday | //+------------------------------------------------------------------+ string SymbolSwapThursday(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Swap Thursday:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the ratio and define its description string double swap_coeff=SymbolInfoDouble(symbol,SYMBOL_SWAP_THURSDAY); string coeff_descr=(swap_coeff==1 ? "single swap" : swap_coeff==3 ? "triple swap" : "no swap is charged"); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%.0f (%s)",indent,"",w,header,swap_coeff,coeff_descr); /* Sample output: Swap Thursday: 1 (single swap) */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the swap accrual ratio in the journal | //| when swapping a position from Thursday to Friday | //+------------------------------------------------------------------+ void SymbolSwapThursdayPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapThursday(symbol,header_width,indent)); }
金曜日から土曜日へのスワップ発生率(SYMBOL_SWAP_FRIDAY)
金曜日から土曜日へロールオーバーされたオーバーナイトポジションのスワップ計算比率(SYMBOL_SWAP_LONGまたはSYMBOL_SWAP_SHORT)。
//+------------------------------------------------------------------+ //| Return the swap accrual ratio as a string | //| when swapping a position from Friday to Saturday | //+------------------------------------------------------------------+ string SymbolSwapFriday(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Swap Friday:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the ratio and define its description string double swap_coeff=SymbolInfoDouble(symbol,SYMBOL_SWAP_FRIDAY); string coeff_descr=(swap_coeff==1 ? "single swap" : swap_coeff==3 ? "triple swap" : "no swap is charged"); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%.0f (%s)",indent,"",w,header,swap_coeff,coeff_descr); /* Sample output: Swap Friday: 1 (single swap) */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the swap accrual ratio in the journal | //| when swapping a position from Friday to Saturday | //+------------------------------------------------------------------+ void SymbolSwapFridayPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapFriday(symbol,header_width,indent)); }
土曜日から日曜日へのスワップ発生率(SYMBOL_SWAP_SATURDAY)
土曜日から日曜日へロールオーバーされたオーバーナイトポジションのスワップ計算比率(SYMBOL_SWAP_LONGまたはSYMBOL_SWAP_SHORT)。
//+------------------------------------------------------------------+ //| Return the swap accrual ratio as a string | //| when swapping a position from Saturday to Sunday | //+------------------------------------------------------------------+ string SymbolSwapSaturday(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Swap Saturday:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the ratio and define its description string double swap_coeff=SymbolInfoDouble(symbol,SYMBOL_SWAP_SATURDAY); string coeff_descr=(swap_coeff==1 ? "single swap" : swap_coeff==3 ? "triple swap" : "no swap is charged"); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%.0f (%s)",indent,"",w,header,swap_coeff,coeff_descr); /* Sample output: Swap Saturday: 0 (no swap is charged) */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the swap accrual ratio in the journal | //| when swapping a position from Saturday to Sunday | //+------------------------------------------------------------------+ void SymbolSwapSaturdayPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapSaturday(symbol,header_width,indent)); }
合計で、各曜日のスワップ発生係数を表示するための7 * 2個の関数が完成しましたが、指定した曜日のスワップ比率を表す文字列を返す普遍的な関数を1つ作り、最初の関数が返した結果を操作ログに表示する関数を1つ作ることができます。付け加えましょう。
//+------------------------------------------------------------------+ //| Return the swap accrual ratio as a string | //| for a specified day of the week | //+------------------------------------------------------------------+ string SymbolSwapByDay(const string symbol,const ENUM_DAY_OF_WEEK day_week,const uint header_width=0,const uint indent=0) { //--- Get a text description of a day of the week string day=EnumToString(day_week); //--- Convert all obtained symbols to lower case and replace the first letter from small to capital if(day.Lower()) day.SetChar(0,ushort(day.GetChar(0)-0x20)); //--- Create the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header=StringFormat("Swap %s:",day); uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Declare the property that needs to be requested in SymbolInfoDouble() int prop=SYMBOL_SWAP_SUNDAY; //--- Depending on the day of the week, indicate the requested property switch(day_week) { case MONDAY : prop=SYMBOL_SWAP_MONDAY; break; case TUESDAY : prop=SYMBOL_SWAP_TUESDAY; break; case WEDNESDAY : prop=SYMBOL_SWAP_WEDNESDAY; break; case THURSDAY : prop=SYMBOL_SWAP_THURSDAY; break; case FRIDAY : prop=SYMBOL_SWAP_FRIDAY; break; case SATURDAY : prop=SYMBOL_SWAP_SATURDAY; break; default/*SUNDAY*/ : prop=SYMBOL_SWAP_SUNDAY; break; } //--- Get the ratio by a selected property and define its description string double swap_coeff=SymbolInfoDouble(symbol,(ENUM_SYMBOL_INFO_DOUBLE)prop); string coeff_descr=(swap_coeff==1 ? "single swap" : swap_coeff==3 ? "triple swap" : "no swap is charged"); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%.0f (%s)",indent,"",w,header,swap_coeff,coeff_descr); /* Sample output: Swap Sunday: 0 (no swap is charged) */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the swap accrual ratio in the journal | //| for a specified day of the week | //+------------------------------------------------------------------+ void SymbolSwapByDayPrint(const string symbol,const ENUM_DAY_OF_WEEK day_week,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSwapByDay(symbol,day_week,header_width,indent)); }
当初証拠金(SYMBOL_MARGIN_INITIAL)
当初証拠金 - ポジション(1ロット)を建てるために必要な証拠金通貨の金額。市場参入時に顧客の資金をチェックする際に使用します。SymbolInfoMarginRate()関数は、注文の種類と方向によって課金される証拠金の金額に関する情報を取得するために使用されます。
//+------------------------------------------------------------------+ //| Return the initial margin as a string | //+------------------------------------------------------------------+ string SymbolMarginInitial(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Margin initial:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f %s",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_MARGIN_INITIAL),SymbolInfoString(symbol,SYMBOL_CURRENCY_MARGIN)); /* Sample output: Margin initial: 0.00 GBP */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the initial margin in the journal | //+------------------------------------------------------------------+ void SymbolMarginInitialPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolMarginInitial(symbol,header_width,indent)); }
維持証拠金(SYMBOL_MARGIN_MAINTENANCE)
機器の維持証拠金。指定された場合、1ロットから差し引かれた、商品の証拠金通貨での証拠金サイズを示します。これは、顧客の口座状況の変化を確認する際に、顧客の資金を確認するのに使用されます。維持証拠金が0の場合、当初証拠金が使用されます。SymbolInfoMarginRate()関数は、注文の種類と方向によって課金される証拠金の金額に関する情報を取得するために使用されます。
//+------------------------------------------------------------------+ //| Return the maintenance margin for an instrument as a string | //+------------------------------------------------------------------+ string SymbolMarginMaintenance(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Margin maintenance:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f %s",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_MARGIN_MAINTENANCE),SymbolInfoString(symbol,SYMBOL_CURRENCY_MARGIN)); /* Sample output: Margin maintenance: 0.00 GBP */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the maintenance margin for an instrument in the journal | //+------------------------------------------------------------------+ void SymbolMarginMaintenancePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolMarginMaintenance(symbol,header_width,indent)); }
現在のセッションの取引量(SYMBOL_SESSION_VOLUME)
現在のセッションの総取引量。
//+------------------------------------------------------------------------+ //| Returns the total volume of trades in the current session as a string | //+------------------------------------------------------------------------+ string SymbolSessionVolume(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session volume:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_SESSION_VOLUME)); /* Sample output: Session volume: 0.00 */ }
操作ログ内の最初の関数の戻り値の表示
//+---------------------------------------------------------------------------+ //| Display the total volume of trades in the current session in the journal | //+---------------------------------------------------------------------------+ void SymbolSessionVolumePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionVolume(symbol,header_width,indent)); }
現在のセッション取引高(SYMBOL_SESSION_TURNOVER)
現在のセッションの総取引高。
//+------------------------------------------------------------------+ //| Return the total turnover in the current session as a string | //+------------------------------------------------------------------+ string SymbolSessionTurnover(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session turnover:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_SESSION_TURNOVER)); /* Sample output: Session turnover: 0.00 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------------+ //| Displays the total turnover during the current session in the journal | //+------------------------------------------------------------------------+ void SymbolSessionTurnoverPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionTurnover(symbol,header_width,indent)); }
ポジションのボリューム(SYMBOL_SESSION_INTEREST)
開かれているポジションのボリュームの合計。
//+------------------------------------------------------------------+ //| Return the total volume of open positions as a string | //+------------------------------------------------------------------+ string SymbolSessionInterest(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session interest:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_SESSION_INTEREST)); /* Sample output: Session interest: 0.00 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the total volume of open positions in the journal | //+------------------------------------------------------------------+ void SymbolSessionInterestPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionInterest(symbol,header_width,indent)); }
買い注文のボリューム(SYMBOL_SESSION_BUY_ORDERS_VOLUME)
現時点での買い注文のボリュームの合計。
//+------------------------------------------------------------------+ //| Return the current total volume of buy orders | //| as a string | //+------------------------------------------------------------------+ string SymbolSessionBuyOrdersVolume(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session Buy orders volume:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_SESSION_BUY_ORDERS_VOLUME)); /* Sample output: Session Buy orders volume: 0.00 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the total volume of Buy orders at the moment | //+------------------------------------------------------------------+ void SymbolSessionBuyOrdersVolumePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionBuyOrdersVolume(symbol,header_width,indent)); }
売り注文のボリューム(SYMBOL_SESSION_SELL_ORDERS_VOLUME)
現時点での売り注文のボリュームの合計。
//+------------------------------------------------------------------+ //| Return the current total volume of sell orders | //| as a string | //+------------------------------------------------------------------+ string SymbolSessionSellOrdersVolume(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session Sell orders volume:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_SESSION_SELL_ORDERS_VOLUME)); /* Sample output: Session Sell orders volume: 0.00 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the total volume of Sell orders at the moment | //+------------------------------------------------------------------+ void SymbolSessionSellOrdersVolumePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionSellOrdersVolume(symbol,header_width,indent)); }
セッション始値(SYMBOL_SESSION_OPEN)
//+------------------------------------------------------------------+ //| Return the session open price as a string | //+------------------------------------------------------------------+ string SymbolSessionOpen(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session Open:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_SESSION_OPEN)); /* Sample output: Session Open: 1.31314 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the session open price in the journal | //+------------------------------------------------------------------+ void SymbolSessionOpenPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionOpen(symbol,header_width,indent)); }
セッション終値(SYMBOL_SESSION_CLOSE)
//+------------------------------------------------------------------+ //| Return the session close price as a string | //+------------------------------------------------------------------+ string SymbolSessionClose(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session Close:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_SESSION_CLOSE)); /* Sample output: Session Close: 1.31349 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the session close price in the journal | //+------------------------------------------------------------------+ void SymbolSessionClosePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionClose(symbol,header_width,indent)); }
セッション平均加重価格(SYMBOL_SESSION_AW)
//+------------------------------------------------------------------+ //| Return the session average weighted price as a string | //+------------------------------------------------------------------+ string SymbolSessionAW(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session AW:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_SESSION_AW)); /* Sample output: Session AW: 0.00000 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the weighted average session price in the journal | //+------------------------------------------------------------------+ void SymbolSessionAWPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionAW(symbol,header_width,indent)); }
現在のセッションの決済価格(SYMBOL_SESSION_PRICE_SETTLEMENT)
//+------------------------------------------------------------------+ //| Return the settlement price of the current session as a string | //+------------------------------------------------------------------+ string SymbolSessionPriceSettlement(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session price settlement:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_SESSION_PRICE_SETTLEMENT)); /* Sample output: Session price settlement: 0.00000 */ }
操作ログ内の最初の関数の戻り値の表示
//+--------------------------------------------------------------------+ //| Display the settlement price of the current session in the journal | //+--------------------------------------------------------------------+ void SymbolSessionPriceSettlementPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionPriceSettlement(symbol,header_width,indent)); }
1セッションの最低価格(SYMBOL_SESSION_PRICE_LIMIT_MIN)
セッションの最低許容価格。
//+------------------------------------------------------------------+ //| Return the minimum acceptable | //| price value per session as a string | //+------------------------------------------------------------------+ string SymbolSessionPriceLimitMin(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session price limit min:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_SESSION_PRICE_LIMIT_MIN)); /* Sample output: Session price limit min: 0.00000 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Displays the minimum acceptable | //| price value per session in the journal | //+------------------------------------------------------------------+ void SymbolSessionPriceLimitMinPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionPriceLimitMin(symbol,header_width,indent)); }
1セッションの最高価格(SYMBOL_SESSION_PRICE_LIMIT_MAX)
セッションの最大許容価格。
//+------------------------------------------------------------------+ //| Return the maximum acceptable | //| price value per session as a string | //+------------------------------------------------------------------+ string SymbolSessionPriceLimitMax(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Session price limit max:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_SESSION_PRICE_LIMIT_MAX)); /* Sample output: Session price limit max: 0.00000 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the maximum acceptable | //| price value per session in the journal | //+------------------------------------------------------------------+ void SymbolSessionPriceLimitMaxPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSessionPriceLimitMax(symbol,header_width,indent)); }
カバーポジション1ロットの契約サイズ(SYMBOL_MARGIN_HEDGED)
1ロットのヘッジポジション(1つの銘柄で反対方向のポジション)の約定または証拠金の大きさ。ヘッジされたポジションについては、2つの証拠金計算方法が可能です。計算方法はブローカーが決定します。
基本的な計算
- 銘柄に当初証拠金(SYMBOL_MARGIN_INITIAL)が指定されている場合、ヘッジ証拠金は絶対値(金額)で指定されます。
- 当初証拠金が設定されていない(0に等しい)場合、証拠金計算に使用される契約サイズは SYMBOL_MARGIN_HEDGEDで指定されます。証拠金は、取引銘柄タイプ(SYMBOL_TRADE_CALC_MODE)に対応する方程式を使用して計算されます。
最大ポジションに基づく計算:
- SYMBOL_MARGIN_HEDGED値は無視されます。
- 銘柄のすべての売りポジションとすべての買いポジションの取引量が計算されます。
- 各方向について、加重平均建値と預託通貨への加重平均転換レートが計算されます。
- 次に、銘柄タイプ(SYMBOL_TRADE_CALC_MODE)に対応する方程式を使用して、短い足と長い足の証拠金を計算します。
- その中で最も大きな値が最終的な値として使われます。
//+------------------------------------------------------------------+ //| Return the contract or margin size as a string | //| for a single lot of covered positions | //+------------------------------------------------------------------+ string SymbolMarginHedged(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Margin hedged:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_MARGIN_HEDGED)); /* Sample output: Margin hedged: 100000.00 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the contract or margin size in the journal | //| for a single lot of covered positions | //+------------------------------------------------------------------+ void SymbolMarginHedgedPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolMarginHedged(symbol,header_width,indent)); }
現在価格の変動率(SYMBOL_PRICE_CHANGE)
前取引日終値に対する現在価格の変化率。
//+------------------------------------------------------------------+ //| Return the change of the current price relative to | //| the end of the previous trading day in percentage as a string | //+------------------------------------------------------------------+ string SymbolPriceChange(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Price change:"; uint w=(header_width==0 ? header.Length()+1 : header_width-1); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%- .2f %%",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_PRICE_CHANGE)); /* Sample output: Price change: -0.28 % */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display in the journal | //| the end of the previous trading day in percentage as a string | //+------------------------------------------------------------------+ void SymbolPriceChangePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPriceChange(symbol,header_width,indent)); }
価格変動率(%)(SYMBOL_PRICE_VOLATILITY)
//+------------------------------------------------------------------+ //| Return the price volatility in percentage as a string | //+------------------------------------------------------------------+ string SymbolPriceVolatility(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Price volatility:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.2f %%",indent,"",w,header,SymbolInfoDouble(symbol,SYMBOL_PRICE_VOLATILITY)); /* Sample output: Price volatility: 0.00 % */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the price volatility in percentage in the journal | //+------------------------------------------------------------------+ void SymbolPriceVolatilityPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPriceVolatility(symbol,header_width,indent)); }
理論オプション価格(SYMBOL_PRICE_THEORETICAL)
//+------------------------------------------------------------------+ //| Return the theoretical price of an option as a string | //+------------------------------------------------------------------+ string SymbolPriceTheoretical(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Price theoretical:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_PRICE_THEORETICAL)); /* Sample output: Price theoretical: 0.00000 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the theoretical price of an option in the journal | //+------------------------------------------------------------------+ void SymbolPriceTheoreticalPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPriceTheoretical(symbol,header_width,indent)); }
オプション/ワラントのデルタ(SYMBOL_PRICE_DELTA)
オプション/ワラントのデルタ。原資産価格が1変化したときに、オプション価格が変化する値を示します。
//+------------------------------------------------------------------+ //| Return as the option/warrant delta as a string | //+------------------------------------------------------------------+ string SymbolPriceDelta(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Price delta:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_PRICE_DELTA)); /* Sample output: Price delta: 0.00000 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the option/warrant delta in the journal | //+------------------------------------------------------------------+ void SymbolPriceDeltaPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPriceDelta(symbol,header_width,indent)); }
オプション/ワラントのシータ(SYMBOL_PRICE_THETA)
オプション/ワラントのθ。オプション価格が、一時的な破綻によって、つまり満期日が近づいたときに、毎日失われるポイント数。
//+------------------------------------------------------------------+ //| Return as the option/warrant theta as a string | //+------------------------------------------------------------------+ string SymbolPriceTheta(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Price theta:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_PRICE_THETA)); /* Sample output: Price theta: 0.00000 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the option/warrant theta in the journal | //+------------------------------------------------------------------+ void SymbolPriceThetaPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPriceTheta(symbol,header_width,indent)); }
オプション/ワラントガンマ(SYMBOL_PRICE_GAMMA)
オプション/ワラントガンマ。SYMBOL_PRICE_GAMMA - オプション/ワラントのガンマ。
//+------------------------------------------------------------------+ //| Return as the option/warrant gamma as a string | //+------------------------------------------------------------------+ string SymbolPriceGamma(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Price gamma:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_PRICE_GAMMA)); /* Sample output: Price gamma: 0.00000 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the option/warrant gamma in the journal | //+------------------------------------------------------------------+ void SymbolPriceGammaPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPriceGamma(symbol,header_width,indent)); }
オプション/ワラントベガ(SYMBOL_PRICE_VEGA)
オプション/ワラントベガボラティリティが1%変化したときにオプション価格が何ポイント変化するかを示します。
//+------------------------------------------------------------------+ //| Return as the option/warrant vega as a string | //+------------------------------------------------------------------+ string SymbolPriceVega(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Price vega:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_PRICE_VEGA)); /* Sample output: Price vega: 0.00000 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the option/warrant vega in the journal | //+------------------------------------------------------------------+ void SymbolPriceVegaPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPriceVega(symbol,header_width,indent)); }
オプション/ワラントロー(SYMBOL_PRICE_RHO)
オプション/ワラントロー。金利が1%変化した場合の理論オプション価格の感応度を反映。
//+------------------------------------------------------------------+ //| Return as the option/warrant rho as a string | //+------------------------------------------------------------------+ string SymbolPriceRho(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Price rho:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_PRICE_RHO)); /* Sample output: Price rho: 0.00000 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the option/warrant rho in the journal | //+------------------------------------------------------------------+ void SymbolPriceRhoPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPriceRho(symbol,header_width,indent)); }
オプション/ワラントオメガ(SYMBOL_PRICE_OMEGA)
オプション/ワラントオメガ。オプションの弾力性 - 原資産価格の変化率によるオプション価格の相対的な変化率。
//+------------------------------------------------------------------+ //| Return as the option/warrant omega as a string | //+------------------------------------------------------------------+ string SymbolPriceOmega(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Price omega:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_PRICE_OMEGA)); /* Sample output: Price omega: 0.00000 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the option/warrant omega in the journal | //+------------------------------------------------------------------+ void SymbolPriceOmegaPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPriceOmega(symbol,header_width,indent)); }
オプション/ワラント感度 (SYMBOL_PRICE_SENSITIVITY)
オプション/ワラントの感度 オプションの価格が1ポイント変化するために、原資産の価格が何ポイント変化すべきかを示します。
//+------------------------------------------------------------------+ //| Return the option/warrant sensitivity as a string | //+------------------------------------------------------------------+ string SymbolPriceSensitivity(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Price sensitivity:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the number of decimal places int dg=(int)SymbolInfoInteger(symbol,SYMBOL_DIGITS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s%-.*f",indent,"",w,header,dg,SymbolInfoDouble(symbol,SYMBOL_PRICE_SENSITIVITY)); /* Sample output: Price sensitivity: 0.00000 */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the option/warrant sensitivity in the journal | //+------------------------------------------------------------------+ void SymbolPriceSensitivityPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPriceSensitivity(symbol,header_width,indent)); }
銘柄の文字列プロパティの受信と表示
原資産名 (SYMBOL_BASIS)
デリバティブ銘柄の裏付けとなる資産の名称。
//+------------------------------------------------------------------+ //| Return the base asset name for a derivative instrument | //+------------------------------------------------------------------+ string SymbolBasis(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Basis:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_BASIS); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Basis: '' (Not specified) */ }
パラメータが指定されず、空の文字列を返す場合は、空のパラメータの隣に「Not specified」を追加します。
操作ログ内の最初の関数の戻り値の表示
//+----------------------------------------------------------------------+ //| Return the base asset name for a derivative instrument in the journal| //+----------------------------------------------------------------------+ void SymbolBasisPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolBasis(symbol,header_width,indent)); }
商品カテゴリまたは部門(SYMBOL_CATEGORY)
金融商品が属するカテゴリまたは部門の名称。
//+------------------------------------------------------------------+ //| Return the name of the financial instrument category or sector | //+------------------------------------------------------------------+ string SymbolCategory(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Category:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_CATEGORY); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Category: '' (Not specified) */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the name of the financial instrument category or sector | //| in the journal | //+------------------------------------------------------------------+ void SymbolCategoryPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolCategory(symbol,header_width,indent)); }
金融商品の国 (SYMBOL_COUNTRY)
取引商品が属する国。
//+------------------------------------------------------------------+ //| Return the country the trading instrument belongs to | //+------------------------------------------------------------------+ string SymbolCountry(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Country:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_COUNTRY); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Country: '' (Not specified) */ }
操作ログ内の最初の関数の戻り値の表示
//+----------------------------------------------------------------------+ //| Display the country the trading instrument belongs to in the journal | //+----------------------------------------------------------------------+ void SymbolCountryPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolCountry(symbol,header_width,indent)); }
経済部門(SYMBOL_SECTOR_NAME)
取引商品が属する経済部門。
//+------------------------------------------------------------------+ //| Return the economic sector | //| the financial instrument belongs to | //+------------------------------------------------------------------+ string SymbolSectorName(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Sector name:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_SECTOR_NAME); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Sector name: 'Currency' */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display in the journal the economic sector | //| the financial instrument belongs to | //+------------------------------------------------------------------+ void SymbolSectorNamePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolSectorName(symbol,header_width,indent)); }
経済分野(SYMBOL_INDUSTRY_NAME)
金融商品が属する業種。
//+------------------------------------------------------------------+ //| Return the economy or industry branch | //| the financial instrument belongs to | //+------------------------------------------------------------------+ string SymbolIndustryName(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Industry name:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_INDUSTRY_NAME); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Industry name: 'Undefined' */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display in the journal the economy or industry branch | //| the financial instrument belongs to | //+------------------------------------------------------------------+ void SymbolIndustryNamePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolIndustryName(symbol,header_width,indent)); }
基準通貨 (SYMBOL_CURRENCY_BASE)
計器の基準通貨。
//+------------------------------------------------------------------+ //| Return the instrument base currency | //+------------------------------------------------------------------+ string SymbolCurrencyBase(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Currency base:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_CURRENCY_BASE); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Currency base: 'GBP' */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the instrument base currency in the journal | //+------------------------------------------------------------------+ void SymbolCurrencyBasePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolCurrencyBase(symbol,header_width,indent)); }
利益通貨(SYMBOL_CURRENCY_PROFIT)
//+------------------------------------------------------------------+ //| Return the profit currency | //+------------------------------------------------------------------+ string SymbolCurrencyProfit(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Currency profit:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_CURRENCY_PROFIT); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Currency profit: 'USD' */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the profit currency in the journal | //+------------------------------------------------------------------+ void SymbolCurrencyProfitPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolCurrencyProfit(symbol,header_width,indent)); }
担保通貨(SYMBOL_CURRENCY_MARGIN)
証拠金通貨。
//+------------------------------------------------------------------+ //| Return margin currency | //+------------------------------------------------------------------+ string SymbolCurrencyMargin(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Currency margin:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_CURRENCY_MARGIN); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Currency margin: 'GBP' */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the margin currency in the journal | //+------------------------------------------------------------------+ void SymbolCurrencyMarginPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolCurrencyMargin(symbol,header_width,indent)); }
クォート元(SYMBOL_BANK)
現在のクォート元。
//+------------------------------------------------------------------+ //| Return the current quote source | //+------------------------------------------------------------------+ string SymbolBank(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Bank:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_BANK); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Bank: '' (Not specified) */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the current quote source in the journal | //+------------------------------------------------------------------+ void SymbolBankPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolBank(symbol,header_width,indent)); }
銘柄の説明(SYMBOL_DESCRIPTION)
銘柄文字列の説明。
//+------------------------------------------------------------------+ //| Return the symbol string description | //+------------------------------------------------------------------+ string SymbolDescription(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Description:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_DESCRIPTION); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Description: 'Pound Sterling vs US Dollar' */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the symbol string description in the journal | //+------------------------------------------------------------------+ void SymbolDescriptionPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolDescription(symbol,header_width,indent)); }
取引所名(SYMBOL_EXCHANGE)
証券が取引されている取引所名。
//+------------------------------------------------------------------+ //| Return the name of an exchange | //| a symbol is traded on | //+------------------------------------------------------------------+ string SymbolExchange(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Exchange:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_EXCHANGE); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Exchange: '' (Not specified) */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display in the journal the name of an exchange | //| a symbol is traded on | //+------------------------------------------------------------------+ void SymbolExchangePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolExchange(symbol,header_width,indent)); }
カスタム記号式(SYMBOL_FORMULA)
カスタム銘柄の価格設定に使用される方程式。計算式に含まれる金融商品名が数字で始まる場合、または特殊文字(「"」、「."」、「-」、「&」、「#」など)を含む場合は、その名前を引用符で囲みます。
- 合成銘柄:"@ESU19"/EURCAD
- カレンダーのスプレッド:"Si-9.13"-"Si-6.13"
- ユーロ指数:34.38805726 * pow(EURUSD,0.3155) * pow(EURGBP,0.3056) * pow(EURJPY,0.1891) * pow(EURCHF,0.1113) * pow(EURSEK,0.0785)
//+------------------------------------------------------------------+ //| Return a formula for constructing a custom symbol price | //+------------------------------------------------------------------+ string SymbolFormula(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Formula:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_FORMULA); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Formula: '' (Not specified) */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the equation for constructing | //| the custom symbol price in the journal | //+------------------------------------------------------------------+ void SymbolFormulaPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolFormula(symbol,header_width,indent)); }
ISINシステム名(SYMBOL_ISIN)
国際証券識別番号システム(ISIN)における取引記号の名称。国際証券識別コードは、証券を一意に識別する12桁の英数字コードです。この銘柄プロパティが利用可能かどうかは、取引サーバー側で定義されます。
//+------------------------------------------------------------------+ //| Return the trading symbol name in the | //| ISIN system | //+------------------------------------------------------------------+ string SymbolISIN(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="ISIN:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_ISIN); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: ISIN: '' (Not specified) */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display in the journal the trading symbol name in the | //| ISIN system | //+------------------------------------------------------------------+ void SymbolISINPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolISIN(symbol,header_width,indent)); }
Webページのアドレス(SYMBOL_PAGE)
銘柄情報を含むWebページのアドレス。このアドレスは、ターミナルで銘柄のプロパティを表示する際にリンクとして表示されます。
//+------------------------------------------------------------------+ //| Return the address of a web page with a symbol data | //+------------------------------------------------------------------+ string SymbolPage(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Page:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_PAGE); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Page: 'https://www.mql5.com/ja/quotes/currencies/gbpusd' */ }
操作ログ内の最初の関数の戻り値の表示
//+--------------------------------------------------------------------+ //| Display the address of a web page with a symbol data in the journal| //+--------------------------------------------------------------------+ void SymbolPagePrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPage(symbol,header_width,indent)); }
銘柄ツリー内のパス(SYMBOL_PATH)
//+------------------------------------------------------------------+ //| Return the path in the symbol tree | //+------------------------------------------------------------------+ string SymbolPath(const string symbol,const uint header_width=0,const uint indent=0) { //--- Define the header text and the width of the header field //--- If the header width is passed to the function equal to zero, then the width will be the size of the header line + 1 string header="Path:"; uint w=(header_width==0 ? header.Length()+1 : header_width); //--- Get the string parameter value string param=SymbolInfoString(symbol,SYMBOL_PATH); //--- Return the property value with a header having the required width and indentation return StringFormat("%*s%-*s'%-s'%-s",indent,"",w,header,param,param=="" ? " (Not specified)" : ""); /* Sample output: Path: 'Forex\GBPUSD' */ }
操作ログ内の最初の関数の戻り値の表示
//+------------------------------------------------------------------+ //| Display the path in the symbol tree in the journal | //+------------------------------------------------------------------+ void SymbolPathPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Print the property value in the log with a header with the required width and indentation Print(SymbolPath(symbol,header_width,indent)); }
上記で紹介した関数は、論理的には互いに同一です。それぞれを「そのまま」使って操作ログに出力することも、必要な銘柄のプロパティを文字列として取得することもできます。デフォルトでは、文字列のインデントはゼロで、ヘッダーフィールドの幅はヘッダーテキストの幅と等しくなります。操作ログのデータフィールドを整列させるために、銘柄プロパティのグループを表示する必要がある場合、これらは必須です。そのような関数を実装してみましょう。
すべての銘柄データを操作ログに出力する関数
作成した関数を基に、銘柄のすべてのプロパティをENUM_SYMBOL_INFO_INTEGER、ENUM_SYMBOL_INFO_DOUBLE、
ENUM_SYMBOL_INFO_STRING列挙型に現れる順番でログに記録する関数を作成します。
//+------------------------------------------------------------------+ //| Send all symbol properties to the journal | //+------------------------------------------------------------------+ void SymbolInfoPrint(const string symbol,const uint header_width=0,const uint indent=0) { //--- Display descriptions of integer properties according to their location in ENUM_SYMBOL_INFO_INTEGER Print("SymbolInfoInteger properties:"); SymbolSubscriptionDelayPrint(symbol,header_width,indent); SymbolSectorPrint(symbol,header_width,indent); SymbolIndustryPrint(symbol,header_width,indent); SymbolCustomPrint(symbol,header_width,indent); SymbolBackgroundColorPrint(symbol,header_width,indent); SymbolChartModePrint(symbol,header_width,indent); SymbolExistsPrint(symbol,header_width,indent); SymbolSelectedPrint(symbol,header_width,indent); SymbolVisiblePrint(symbol,header_width,indent); SymbolSessionDealsPrint(symbol,header_width,indent); SymbolSessionBuyOrdersPrint(symbol,header_width,indent); SymbolSessionSellOrdersPrint(symbol,header_width,indent); SymbolVolumePrint(symbol,header_width,indent); SymbolVolumeHighPrint(symbol,header_width,indent); SymbolVolumeLowPrint(symbol,header_width,indent); SymbolTimePrint(symbol,header_width,indent); SymbolTimeMSCPrint(symbol,header_width,indent); SymbolDigitsPrint(symbol,header_width,indent); SymbolSpreadPrint(symbol,header_width,indent); SymbolSpreadFloatPrint(symbol,header_width,indent); SymbolTicksBookDepthPrint(symbol,header_width,indent); SymbolTradeCalcModePrint(symbol,header_width,indent); SymbolTradeModePrint(symbol,header_width,indent); SymbolSymbolStartTimePrint(symbol,header_width,indent); SymbolSymbolExpirationTimePrint(symbol,header_width,indent); SymbolTradeStopsLevelPrint(symbol,header_width,indent); SymbolTradeFreezeLevelPrint(symbol,header_width,indent); SymbolTradeExeModePrint(symbol,header_width,indent); SymbolSwapModePrint(symbol,header_width,indent); SymbolSwapRollover3DaysPrint(symbol,header_width,indent); SymbolMarginHedgedUseLegPrint(symbol,header_width,indent); SymbolExpirationModePrint(symbol,header_width,indent); SymbolFillingModePrint(symbol,header_width,indent); SymbolOrderModePrint(symbol,header_width,indent); SymbolOrderGTCModePrint(symbol,header_width,indent); SymbolOptionModePrint(symbol,header_width,indent); SymbolOptionRightPrint(symbol,header_width,indent); //--- Display descriptions of real properties according to their location in ENUM_SYMBOL_INFO_DOUBLE Print("SymbolInfoDouble properties:"); SymbolBidPrint(symbol,header_width,indent); SymbolBidHighPrint(symbol,header_width,indent); SymbolBidLowPrint(symbol,header_width,indent); SymbolAskPrint(symbol,header_width,indent); SymbolAskHighPrint(symbol,header_width,indent); SymbolAskLowPrint(symbol,header_width,indent); SymbolLastPrint(symbol,header_width,indent); SymbolLastHighPrint(symbol,header_width,indent); SymbolLastLowPrint(symbol,header_width,indent); SymbolVolumeRealPrint(symbol,header_width,indent); SymbolVolumeHighRealPrint(symbol,header_width,indent); SymbolVolumeLowRealPrint(symbol,header_width,indent); SymbolOptionStrikePrint(symbol,header_width,indent); SymbolPointPrint(symbol,header_width,indent); SymbolTradeTickValuePrint(symbol,header_width,indent); SymbolTradeTickValueProfitPrint(symbol,header_width,indent); SymbolTradeTickValueLossPrint(symbol,header_width,indent); SymbolTradeTickSizePrint(symbol,header_width,indent); SymbolTradeContractSizePrint(symbol,header_width,indent); SymbolTradeAccruedInterestPrint(symbol,header_width,indent); SymbolTradeFaceValuePrint(symbol,header_width,indent); SymbolTradeLiquidityRatePrint(symbol,header_width,indent); SymbolVolumeMinPrint(symbol,header_width,indent); SymbolVolumeMaxPrint(symbol,header_width,indent); SymbolVolumeStepPrint(symbol,header_width,indent); SymbolVolumeLimitPrint(symbol,header_width,indent); SymbolSwapLongPrint(symbol,header_width,indent); SymbolSwapShortPrint(symbol,header_width,indent); SymbolSwapByDayPrint(symbol,SUNDAY,header_width,indent); SymbolSwapByDayPrint(symbol,MONDAY,header_width,indent); SymbolSwapByDayPrint(symbol,TUESDAY,header_width,indent); SymbolSwapByDayPrint(symbol,WEDNESDAY,header_width,indent); SymbolSwapByDayPrint(symbol,THURSDAY,header_width,indent); SymbolSwapByDayPrint(symbol,FRIDAY,header_width,indent); SymbolSwapByDayPrint(symbol,SATURDAY,header_width,indent); SymbolMarginInitialPrint(symbol,header_width,indent); SymbolMarginMaintenancePrint(symbol,header_width,indent); SymbolSessionVolumePrint(symbol,header_width,indent); SymbolSessionTurnoverPrint(symbol,header_width,indent); SymbolSessionInterestPrint(symbol,header_width,indent); SymbolSessionBuyOrdersVolumePrint(symbol,header_width,indent); SymbolSessionSellOrdersVolumePrint(symbol,header_width,indent); SymbolSessionOpenPrint(symbol,header_width,indent); SymbolSessionClosePrint(symbol,header_width,indent); SymbolSessionAWPrint(symbol,header_width,indent); SymbolSessionPriceSettlementPrint(symbol,header_width,indent); SymbolSessionPriceLimitMinPrint(symbol,header_width,indent); SymbolSessionPriceLimitMaxPrint(symbol,header_width,indent); SymbolMarginHedgedPrint(symbol,header_width,indent); SymbolPriceChangePrint(symbol,header_width,indent); SymbolPriceVolatilityPrint(symbol,header_width,indent); SymbolPriceTheoreticalPrint(symbol,header_width,indent); SymbolPriceDeltaPrint(symbol,header_width,indent); SymbolPriceThetaPrint(symbol,header_width,indent); SymbolPriceGammaPrint(symbol,header_width,indent); SymbolPriceVegaPrint(symbol,header_width,indent); SymbolPriceRhoPrint(symbol,header_width,indent); SymbolPriceOmegaPrint(symbol,header_width,indent); SymbolPriceSensitivityPrint(symbol,header_width,indent); //--- Display descriptions of string properties according to their location in ENUM_SYMBOL_INFO_STRING Print("SymbolInfoString properties:"); SymbolBasisPrint(symbol,header_width,indent); SymbolCategoryPrint(symbol,header_width,indent); SymbolCountryPrint(symbol,header_width,indent); SymbolSectorNamePrint(symbol,header_width,indent); SymbolIndustryNamePrint(symbol,header_width,indent); SymbolCurrencyBasePrint(symbol,header_width,indent); SymbolCurrencyProfitPrint(symbol,header_width,indent); SymbolCurrencyMarginPrint(symbol,header_width,indent); SymbolBankPrint(symbol,header_width,indent); SymbolDescriptionPrint(symbol,header_width,indent); SymbolExchangePrint(symbol,header_width,indent); SymbolFormulaPrint(symbol,header_width,indent); SymbolISINPrint(symbol,header_width,indent); SymbolPagePrint(symbol,header_width,indent); SymbolPathPrint(symbol,header_width,indent); } //+------------------------------------------------------------------+
ここでは、すべての銘柄のプロパティが操作ログに一列に表示されるだけです。プロパティの型の前には、integer、real、stringといった型を示すヘッダーが付きます。
以下は、ヘッダーフィールド幅28文字、インデント2文字のスクリプトから関数を呼び出した結果です。
void OnStart() { //--- SymbolInfoPrint(Symbol(),28,2); /* Sample output: SymbolInfoInteger properties: Subscription Delay: No Sector: Currency Industry: Undefined Custom symbol: No Background color: Default Chart mode: Bid Exists: Yes Selected: Yes Visible: Yes Session deals: 0 Session Buy orders: 0 Session Sell orders: 0 Volume: 0 Volume high: 0 Volume low: 0 Time: 2023.07.14 22:07:01 Time msc: 2023.07.14 22:07:01.706 Digits: 5 Spread: 5 Spread float: Yes Ticks book depth: 10 Trade calculation mode: Forex Trade mode: Full Start time: 1970.01.01 00:00:00 (Not used) Expiration time: 1970.01.01 00:00:00 (Not used) Stops level: 0 (By Spread) Freeze level: 0 (Not used) Trade Execution mode: Instant Swap mode: Points Swap Rollover 3 days: Wednesday Margin hedged use leg: No Expiration mode: GTC|DAY|SPECIFIED Filling mode: FOK|IOC|RETURN Order mode: MARKET|LIMIT|STOP|STOP_LIMIT|SL|TP|CLOSEBY Order GTC mode: GTC Option mode: European Option right: Call SymbolInfoDouble properties: Bid: 1.30979 Bid High: 1.31422 Bid Low: 1.30934 Ask: 1.30984 Ask High: 1.31427 Ask Low: 1.30938 Last: 0.00000 Last High: 0.00000 Last Low: 0.00000 Volume real: 0.00 Volume High real: 0.00 Volume Low real: 0.00 Option strike: 0.00000 Point: 0.00001 Tick value: 1.00000 Tick value profit: 1.00000 Tick value loss: 1.00000 Tick size: 0.00001 Contract size: 100000.00 GBP Accrued interest: 0.00 Face value: 0.00 Liquidity rate: 0.00 Volume min: 0.01 Volume max: 500.00 Volume step: 0.01 Volume limit: 0.00 Swap long: -0.20 Swap short: -2.20 Swap Sunday: 0 (no swap is charged) Swap Monday: 1 (single swap) Swap Tuesday: 1 (single swap) Swap Wednesday: 3 (triple swap) Swap Thursday: 1 (single swap) Swap Friday: 1 (single swap) Swap Saturday: 0 (no swap is charged) Margin initial: 0.00 GBP Margin maintenance: 0.00 GBP Session volume: 0.00 Session turnover: 0.00 Session interest: 0.00 Session Buy orders volume: 0.00 Session Sell orders volume: 0.00 Session Open: 1.31314 Session Close: 1.31349 Session AW: 0.00000 Session price settlement: 0.00000 Session price limit min: 0.00000 Session price limit max: 0.00000 Margin hedged: 100000.00 Price change: -0.28 % Price volatility: 0.00 % Price theoretical: 0.00000 Price delta: 0.00000 Price theta: 0.00000 Price gamma: 0.00000 Price vega: 0.00000 Price rho: 0.00000 Price omega: 0.00000 Price sensitivity: 0.00000 SymbolInfoString properties: Basis: '' (Not specified) Category: '' (Not specified) Country: '' (Not specified) Sector name: 'Currency' Industry name: 'Undefined' Currency base: 'GBP' Currency profit: 'USD' Currency margin: 'GBP' Bank: '' (Not specified) Description: 'Pound Sterling vs US Dollar' Exchange: '' (Not specified) Formula: '' (Not specified) ISIN: '' (Not specified) Page: 'https://www.mql5.com/ja/quotes/currencies/gbpusd' Path: 'Forex\GBPUSD' */ }
ご覧の通り、すべてのデータは表形式で整然と並べられています。負の値は左にシフトしているため、全体から目立つことはありません。
これは、上記の関数の使い方の一例です。ご自分のプログラムで「そのまま」使用したり、銘柄のプロパティのいくつかのグループを表示したり、ビジョンやニーズに合わせて変更したりすることができます。
結論
フォーマットされた文字列を使用して銘柄と口座のプロパティを表示する関数について検討しました。次のステップは、MQL5で実装されたいくつかの構造体を記録することです。
MetaQuotes Ltdによってロシア語から翻訳されました。
元の記事: https://www.mql5.com/ru/articles/12953





- 無料取引アプリ
- 8千を超えるシグナルをコピー
- 金融ニュースで金融マーケットを探索