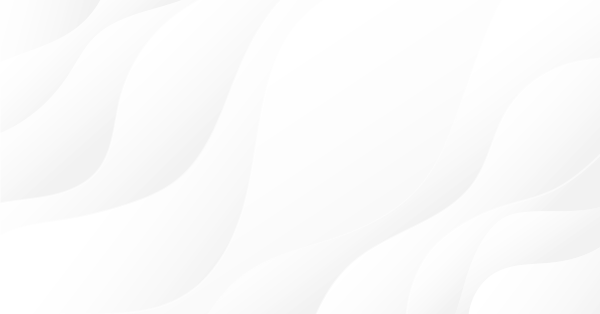
初心者のためのMQL5: Expert Advisorでのテクニカルインディケーター使用ガイド
はじめに
コードで標準テクニカルインディケーターを使わないExpert Advisorやインディケーターは稀です。トレーディングストラテジーの初心者、ベテラン開発者の両方に人気です。インディケーター作成の詳細を理解するのは難しくなく、この本記事が手助けします。内蔵標準テクニカルインディケーターを使用するための関数の使用を考えます。
標準テクニカルインディケーターの関数使用の基本
呼び出し時、 テクニカルインディケーターの各関数は、指定入力パラメーターで作成されたオブジェクト(インディケーターのインスタンス)のハンドラーを作成します。ハンドルの目的はそれをこのオブジェクトを割り当てることです。このインディケーターバッファーからデータを入手しそれを自身の計算に使うことで十分です。以下の例を考えましょう。
double MA[]; // array for the indicator iMA int MA_handle; // handle of the indicator iMA //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iMA MA_handle=iMA(NULL,0,21,0,MODE_EMA,PRICE_CLOSE); return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- filling an array MA[] with current values of iMA //--- Copying 100 elements CopyBuffer(MA_handle,0,0,100,MA); //--- set indexing of elements as series, as in MQL4 ArraySetAsSeries(MA,true); //--- here you can use the data of MA[] as you wish, for example: if(MA[0]>MA[1]) { //--- some operations } }
ご覧のとおり、すべてがかなりシンプルです。この例はトレーダーの間で最も人気なインディケーター iMAの使用方法を説明します。
このインディケーターのハンドルは OnInit()関数内に作成されるべきです。
- 「MQL4のようにインデックスの要素をシリーズとして設定する」というのはどういう意味? 我々の例では時系列として要素インデックスを使いました。つまり、現在のバー (まだ未形成) は常にインデックス[0]を持ちます。以前の (既に形成済)はインデックス [1]などを持ちます。
- なぜインデックスを設定するのか?利便性またアルゴリズムの最適な実行のために使いました。MQL5 言語ではプログラマーにとって便利なインデックス作成が可能です。
- OnInit()関数内にインディケーターを作成する方がいい理由とは?明示的な禁止がないため、もちろんプログラム内どこにでも作成することが可能です。しかし、 それを支持する強い論点があります。: OnInit() 関数はExpert Advisorスタートアップ時に呼ばれ、実行中にパラメーターを変えないインディケーターの初期化を行うことで十分です。それらの入力パラメーターを変える場合、 もう一度それらを例えば OnTick()関数で初期化することが可能です。
プログラミングレッスン
ほぼすべてのExpert Advisorはデモだけでなくリアルアカウントでトレード操作をするようにデザインされています。それがトレードしている時に静かに眠りたければ、すべての潜在的トレード結果を予測するべきです。自動トレーディング システム開発のエキスパートでもイライラするミスをすることがよくあります。そしてこのようなミスの代償はとても大きくなる可能性があります!
例えば、これは 自動トレーディングチャンピオンシップ 2008で起こった話です。参加者の一人のExpert Advisorがデポジットをあるレベルまで増加した後、停止するはずでした。それが完了しました。Expert Advisorが起動しまたトレードを開始しお金を失った時、著者とその動作を見ていた全員が驚きました。
もちろん、ロボットが操作不能になった時に同じミスをしたくありません。 そのため、 標準テクニカルインディケーター使用におけるセーフネットを考えましょう 。
- オブジェクトポインターは初期化ブロック内に作成されます。もしもこれがうまくいかなかったら?この場合、空のレファレンスを取ります。 そのため, MQL5開発者によって提供された妥当性の確認方法を使用しましょう。もしもポインターが未作成の場合、関数によって戻されたハンドルの値は標準定数 INVALID_HANDLE = -1に等しいです。
- このインディケーターからデータを入手する時、 その値をこのインディケーターのバッファーにコピーし、計算に使います。もしもそれが失敗したら?コピーが失敗したら、トレーディングシステムが不適切なトレード シグナルを発生するかもしれません。このような場合、MQL5開発者はコピーされた要素数をすぐに確認する可能性を提供しました。エラーの場合のコピーされたエレメントは-1に等しいです。
それではどのようにコードできるかを考えましょう。
//---- arrays for indicators double MA[]; // array for the indicator iMA //---- handles for indicators int MA_handle; // handle of the indicator iMA //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iMA MA_handle=iMA(NULL,0,21,0,MODE_EMA,PRICE_CLOSE); //--- report if there was an error in object creation if(MA_handle<0) { Print("The creation of iMA has failed: MA_handle=",INVALID_HANDLE); Print("Runtime error = ",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- filling an array MA[] with current values of iMA //--- 100 elements should be written to the array //--- if there was an error, we terminate the execution of program if(CopyBuffer(MA_handle,0,0,100,MA)<=0) return; //--- set indexation of array MA[] as timeseries ArraySetAsSeries(MA,true); //--- here you can use the data of MA[] as you wish if(MA[0]>MA[1]) { //--- some operations } }
コード中の少しの変化で安全性と信頼性が増した事に注意して下さい。しかし、これがすべてではありません。このインディケーター値を配列にコピーする手順は改善されたかもしれないので、書かれた関数を使う必要があります。
//+------------------------------------------------------------------------------+ //| The function copies the values of the indicator,the elements | //| will be indexed depending on the value of the input parameter asSeries | //+------------------------------------------------------------------------------+ bool CopyBufferAsSeries( int handle, // indicator's handle int bufer, // buffer index int start, // start index int number, // number of elements to copy bool asSeries, // if it's true, the elements will be indexed as series double &M[] // target array ) { //--- filling the array M with current values of the indicator if(CopyBuffer(handle,bufer,start,number,M)<=0) return(false); //--- the elements will be indexed as follows: //--- if asSeries=true, it will be indexed as timeseries //--- if asSeries=false, it will be indexed as default ArraySetAsSeries(M,asSeries); //--- return(true); }
CopyBufferAsSeries関数は本記事に添付されているGetIndicatorBuffers.mqhファイル内にあります。その使用には、我々のコードに 指令 includeを追加しなければなりません。フォルダ ..\ MQL5\Include\にそれをコピーする必要があります。このiMAインディケーターを呼び出し、このインディケーターバッファーからのデータを対応配列にコピーする最終的なコードは以下のように見えます。:
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double MA[]; // array for the indicator iMA //---- handles for indicators int MA_handle; // handle for the indicator iMA //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iMA MA_handle=iMA(NULL,0,21,0,MODE_EMA,PRICE_CLOSE); //--- report if there was an error in object creation if(MA_handle<0) { Print("The creation of iMA has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- filling an array MA[] with current values of iMA //--- set indexation of array MA[] as timeseries //--- return if there was an error if(!CopyBufferAsSeries(MA_handle,0,0,100,true,MA)) return; //--- here you can use the data of MA[] as you wish, for example: if(MA[0]>MA[1]) { //--- some operations } }
マルチバッファーインディケーターの特徴
例として、 3つのインディケーターバッファー iAlligatorインディケーターを考えましょう。
- 0 buffer - GATORJAW_LINE
- 1st buffer - GATORTEETH_LINE
- 2nd buffer - GATORLIPS_LINE
ワンコールを使ってこれらのインディケーターバッファーのデータ受信を実行するには、本記事に含まれるファイル内の以下の関数を使用します。
//+------------------------------------------------------------------------------+ //| The function copies the values of the indicator Alligator to three arrays: | //| Jaws[], Teeth[], Lips[] (with elements indexed as timeaseries). | //+------------------------------------------------------------------------------+ bool GetAlligatorBuffers(int Alligator_handle, int start, int number, double &Jaws[], double &Teeth[], double &Lips[], bool asSeries=true // (elements indexed as timeaseries) ) { //--- filling an array Jaws with current values of GATORJAW_LINE if(!CopyBufferAsSeries(Alligator_handle,0,start,number,asSeries,Jaws)) return(false); //--- filling an array Teeth with current values of GATORTEETH_LINE if(!CopyBufferAsSeries(Alligator_handle,1,start,number,asSeries,Teeth)) return(false); //--- filling an array Lisp with current values of GATORLIPS_LINE if(!CopyBufferAsSeries(Alligator_handle,2,start,number,asSeries,Lips)) return(false); //--- return(true); }
それは以下のように使われることができます。
#include <GetIndicatorBuffers.mqh> //---- indicator buffers double Jaws[]; // array for GATORJAW_LINE of iAlligator double Teeth[]; // array for GATORTEETH_LINE of iAlligator double Lips[]; // array for GATORLIPS_LINE of iAlligator //---- handles for indicators int Alligator_handle; // handle of the indicator iAlligator //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iAlligator Alligator_handle=iAlligator(NULL,0,13,8,8,5,5,3,MODE_EMA,PRICE_MEDIAN); //--- report if there was an error in object creation if(Alligator_handle<0) { Print("The creation of iAlligator has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- set indexation of arrays as timeseries //--- fill arrays with current values of Alligator //--- return if there was an error if(!GetAlligatorBuffers(Alligator_handle,0,100,Jaws,Teeth,Lips,true)) return; }
キーポイントを概説したので、すべての標準インディケーターの例を考察する用意ができました。
標準インディケーターの使用例
iAC
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double AC[]; // array for the indicator iAC //---- handles for indicators int AC_handle; // handle of the indicator iAC //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iAC AC_handle=iAC(NULL,0); //--- report if there was an error in object creation if(AC_handle<0) { Print("The creation of iAC has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- filling an array AC[] with current values of iAC //--- set indexation of array AC[] as timeseries //--- return if there was an error if(!CopyBufferAsSeries(AC_handle,0,0,100,true,AC)) return; }
iAD
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double AD[]; // array for the indicator iAD //---- handles for indicators int AD_handle; // handle for the indicator iAD //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iAD AD_handle=iAD(NULL,0,VOLUME_TICK); //--- report if there was an error in object creation if(AD_handle<0) { Print("The creation of iAD has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- filling an array AD[] with current values of iAD //--- set indexation of array AD[] as timeseries //--- return if there was an error if(!CopyBufferAsSeries(AD_handle,0,0,100,true,AD)) return; }
iADX
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double Main[]; // array for MAIN_LINE of iADX double PlusDI[]; // array for PLUSDI_LINE of iADX double MinusDI[]; // array for MINUSDI_LINE of iADX //---- handles for indicators int ADX_handle; // handle of the indicator iADX //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iADX ADX_handle=iADX(NULL,0,14); //--- report if there was an error in object creation if(ADX_handle<0) { Print("The creation of iADX has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- set indexation of arrays as timeseries //--- filling the arrays with current values of all indicator's buffers //--- return if there was an error if(!GetADXBuffers(ADX_handle,0,100,Main,PlusDI,MinusDI,true)) return; }
iADXWilder
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double Main[]; // array for MAIN_LINE of iADXWilder double PlusDI[]; // array for PLUSDI_LINE of iADXWilder double MinusDI[]; // array for MINUSDI_LINE of iADXWilder //---- handles for indicators int ADXWilder_handle; // handle of the indicator iADXWilder //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iADXWilder ADXWilder_handle=iADXWilder(NULL,0,14); //--- report if there was an error in object creation if(ADXWilder_handle<0) { Print("The creation of iADXWilder has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- set indexation of arrays as timeseries //--- filling the arrays with current values of all indicator's buffers //--- return if there was an error if(!GetADXWilderBuffers(ADXWilder_handle,0,100,Main,PlusDI,MinusDI,true)) return; }
iAlligator
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double Jaws[]; // array for GATORJAW_LINE of iAlligator double Teeth[]; // array for GATORTEETH_LINE of iAlligator double Lips[]; // array for GATORLIPS_LINE of iAlligator //---- handles for indicators int Alligator_handle; // handle of the indicator iAlligator //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iAlligator Alligator_handle=iAlligator(NULL,0,13,8,8,5,5,3,MODE_EMA,PRICE_MEDIAN); //--- report if there was an error in object creation if(Alligator_handle<0) { Print("The creation of iAlligator has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- set indexation of arrays as timeseries //--- filling the arrays with current values of all indicator's buffers //--- return if there was an error if(!GetAlligatorBuffers(Alligator_handle,0,100,Jaws,Teeth,Lips,true)) return; }
iAMA
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double AMA[]; // array for the indicator iAMA //---- handles for indicators int AMA_handle; // handle for the indicator iAMA //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iAMA AMA_handle=iAMA(NULL,0,21,5,8,0,PRICE_CLOSE); //--- report if there was an error in object creation if(AMA_handle<0) { Print("The creation of iAMA has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- filling an array AMA[] with current values of iAMA //--- set indexation of array AMA[] as timeseries //--- return if there was an error if(!CopyBufferAsSeries(AMA_handle,0,0,100,true,AMA)) return; }
iAO
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double AO[]; // array for the indicator iAO //---- handles for indicators int AO_handle; // handle of the indicator iAO //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iAO AO_handle=iAO(NULL,0); //--- report if there was an error in object creation if(AO_handle<0) { Print("The creation of iAO has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- filling an array AO[] with current values of iAO //--- set indexation of array AO[] as timeseries //--- return if there was an error if(!CopyBufferAsSeries(AO_handle,0,0,100,true,AO)) return; }
iATR
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double ATR[]; // array for the indicator iATR //---- handles for indicators int ATR_handle; // handle of the indicator iATR //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iATR ATR_handle=iATR(NULL,0,14); //--- report if there was an error in object creation if(ATR_handle<0) { Print("The creation of iATR has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- filling an array ATR[] with current values of iATR //--- set indexation of array ATR[] as timeseries //--- return if there was an error if(!CopyBufferAsSeries(ATR_handle,0,0,100,true,ATR)) return; }
iBearsPower
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double BearsPower[]; // array for the indicator iBearsPower //---- handles for indicators int BearsPower_handle; // handle for the indicator iBearsPower //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iBearsPower BearsPower_handle=iBearsPower(NULL,0,14); //--- report if there was an error in object creation if(BearsPower_handle<0) { Print("The creation of iBearsPower has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- filling an array BearsPower[] with current values of iBearsPower //--- set indexation of array BearsPower[] as timeseries //--- return if there was an error if(!CopyBufferAsSeries(BearsPower_handle,0,0,100,true,BearsPower)) return; }
iBands
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double Base[]; // array for BASE_LINE of iBands double Upper[]; // array for UPPER_BAND of iBands double Lower[]; // array for LOWER_BAND of iBands //---- handles for indicators int Bands_handle; // handle of the indicator iBands //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iBands Bands_handle=iBands(NULL,0,144,0,2,PRICE_CLOSE); //--- report if there was an error in object creation if(Bands_handle<0) { Print("The creation of iBands has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- set indexation of arrays as timeseries //--- filling the arrays with current values of all indicator's buffers //--- return if there was an error if(!GetBandsBuffers(Bands_handle,0,100,Base,Upper,Lower,true)) return; }
iBullsPower
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double BullsPower[]; // array for the indicator iBullsPower //---- handles for indicators int BullsPower_handle; // handle for the indicatoriBullsPower //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iBullsPower BullsPower_handle=iBullsPower(NULL,0,14); //--- report if there was an error in object creation if(BullsPower_handle<0) { Print("The creation of iBullsPower has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- filling an array BullsPower[] with current values of iBullsPower //--- set indexation of array BullsPower[] as timeseries //--- return if there was an error if(!CopyBufferAsSeries(BullsPower_handle,0,0,100,true,BullsPower)) return; }
iCCI
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double CCI[]; // array for the indicator iCCI //---- handles for indicators int CCI_handle; // handle for the indicator iCCI //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iCCI CCI_handle=iCCI(NULL,0,14,PRICE_CLOSE); //--- report if there was an error in object creation if(CCI_handle<0) { Print("The creation of iCCI has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- filling an array CCI[] with current values of iCCI //--- set indexation of array CCI[] as timeseries //--- return if there was an error if(!CopyBufferAsSeries(CCI_handle,0,0,100,true,CCI)) return; }
iChaikin
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double Chaikin[]; // array for the indicator iChaikin //---- handles for indicators int Chaikin_handle; // handle for the indicator iChaikin //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iChaikin Chaikin_handle=iChaikin(NULL,0,8,14,MODE_EMA,VOLUME_TICK); //--- report if there was an error in object creation if(Chaikin_handle<0) { Print("The creation of iChaikin has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- filling an array Chaikin[] with current values of iChaikin //--- set indexation of array AC[] as timeseries //--- return if there was an error if(!CopyBufferAsSeries(Chaikin_handle,0,0,100,true,Chaikin)) return; }
iDEMA
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double DEMA[]; // array for the indicator iDEMA //---- handles for indicators int DEMA_handle; // handle for the indicator iDEMA //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iDEMA DEMA_handle=iDEMA(NULL,0,8,0,PRICE_CLOSE); //--- report if there was an error in object creation if(DEMA_handle<0) { Print("The creation of iDEMA has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- filling an array DEMA[] with current values of iDEMA //--- set indexation of array DEMA[] as timeseries //--- return if there was an error if(!CopyBufferAsSeries(DEMA_handle,0,0,100,true,DEMA)) return; }
iDeMarker
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double DeMarker[]; // array for the indicator iDeMarker //---- handles for indicators int DeMarker_handle; // handle for the indicator iDeMarker //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iDeMarker DeMarker_handle=iDeMarker(NULL,0,21); //--- report if there was an error in object creation if(DeMarker_handle<0) { Print("The creation of iDeMarker has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- filling an array DeMarker[] with current values of iDeMarker //--- set indexation of array DeMarker[] as timeseries //--- return if there was an error if(!CopyBufferAsSeries(DeMarker_handle,0,0,100,true,DeMarker)) return; }
iEnvelopes
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double Upper[]; // array for UPPER_LINE of iEnvelopes double Lower[]; // array for LOWER_LINE of iEnvelopes //---- handles for indicators int Envelopes_handle; // handle of the indicator iEnvelopes //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iEnvelopes Envelopes_handle=iEnvelopes(NULL,0,14,0,MODE_SMA,PRICE_CLOSE,0.1); //--- report if there was an error in object creation if(Envelopes_handle<0) { Print("The creation of iEnvelopes has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- set indexation for arrays as timeseries //--- return if there was an error //--- filling the arrays with current values of iEnvelopes if(!GetEnvelopesBuffers(Envelopes_handle,0,100,Upper,Lower,true)) return; }
iForce
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double Force[]; // array for the indicator iForce //---- handles for indicators int Force_handle; // handle for the indicator iForce //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iForce Force_handle=iForce(NULL,0,21,MODE_SMA,VOLUME_TICK); //--- report if there was an error in object creation if(Force_handle<0) { Print("The creation of iForce has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- filling an array Force[] with current values of iForce //--- set indexation of array Force[] as timeseries //--- return if there was an error if(!CopyBufferAsSeries(Force_handle,0,0,100,true,Force)) return; }
iFractals
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double Upper[]; // array for UPPER_LINE of iFractals double Lower[]; // array for LOWER_LINE of iFractals //---- handles for indicators int Fractals_handle; // handle of the indicator iFractals //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iFractals Fractals_handle=iFractals(NULL,0); //--- report if there was an error in object creation if(Fractals_handle<0) { Print("The creation of iFractals has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- set indexation of arrays as timeseries //--- filling the arrays with current values of all indicator's buffers //--- return if there was an error if(!GetFractalsBuffers(Fractals_handle,0,100,Upper,Lower,true)) return; }
iFrAMA
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double FrAMA[]; // array for the indicator iFrAMA //---- handles for indicators int FrAMA_handle; // handle for the indicator iFrAMA //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iFrAMA FrAMA_handle=iFrAMA(NULL,0,21,0,MODE_SMA); //--- report if there was an error in object creation if(FrAMA_handle<0) { Print("The creation of iFrAMA has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- filling an array FrAMA[] with current values of iFrAMA //--- set indexation of array FrAMA[] as timeseries //--- return if there was an error if(!CopyBufferAsSeries(FrAMA_handle,0,0,100,true,FrAMA)) return; }
iGator
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double Upper[]; // array for UPPER_LINE of iGator double Lower[]; // array for LOWER_LINE of iGator //---- handles for indicators int Gator_handle; // handle of the indicator iGator //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iGator Gator_handle=iGator(NULL,0,13,8,8,5,5,3,MODE_EMA,PRICE_MEDIAN); //--- report if there was an error in object creation if(Gator_handle<0) { Print("The creation of iGator has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- set indexation of arrays as timeseries //--- filling the arrays with current values of all indicator's buffers //--- return if there was an error if(!GetGatorBuffers(Gator_handle,0,100,Upper,Lower,true)) return; }
iIchimoku
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double Tenkansen[]; // array for TENKANSEN_LINE of iIchimoku double Kijunsen[]; // array for KIJUNSEN_LINE of iIchimoku double SenkouspanA[]; // array for SENKOUSPANA_LINE of iIchimoku double SenkouspanB[]; // array for SENKOUSPANB_LINE of iIchimoku double Chinkouspan[]; // array for CHINKOUSPAN_LINE of iIchimoku //---- handles for indicators int Ichimoku_handle; // handle of the indicator iIchimoku //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iIchimoku Ichimoku_handle=iIchimoku(NULL,0,9,26,52); //--- report if there was an error in object creation if(Ichimoku_handle<0) { Print("The creation of iIchimoku has failed: Runtime error =",GetLastError()); //--- forced program terminatio return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- set indexation of arrays as timeseries //--- filling the arrays with current values of all indicator's buffers //--- return if there was an error if(!GetIchimokuBuffers(Ichimoku_handle,0,100, Tenkansen, Kijunsen, SenkouspanA, SenkouspanB, Chinkouspan, true)) return; }
iBWMFI
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double BWMFI[]; // array for the indicator iBWMFI //---- handles for indicators int BWMFI_handle; // handle of the indicato iBWMFI //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iBWMFI BWMFI_handle=iBWMFI(NULL,0,VOLUME_TICK); //--- report if there was an error in object creation if(BWMFI_handle<0) { Print("The creation of iBWMFI has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- filling an array BWMFI[] with current values of iBWMFI //--- set indexation of array BWMFI[] as timeseries //--- return if there was an error if(!CopyBufferAsSeries(BWMFI_handle,0,0,100,true,BWMFI)) return; }
iMomentum
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double Momentum[]; // array for the indicator iMomentum //---- handles for indicators int Momentum_handle; // handle for the indicator iMomentum //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iMomentum Momentum_handle=iMomentum(NULL,0,14,PRICE_CLOSE); //--- report if there was an error in object creation if(Momentum_handle<0) { Print("The creation of iMomentum has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- filling an array Momentum[] with current values of iMomentum //--- set indexation of array Momentum[] as timeseries //--- return if there was an error if(!CopyBufferAsSeries(Momentum_handle,0,0,100,true,Momentum)) return; }
iMFI
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double MFI[]; // array for the indicator iMFI //---- handles for indicators int MFI_handle; // handle of the indicator iMFI //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iMFI MFI_handle=iMFI(NULL,0,14,VOLUME_TICK); //--- report if there was an error in object creation if(MFI_handle<0) { Print("The creation of iMFI has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- filling an array MFI[] with current values of iMFI //--- set indexation of array MFI[] as timeseries //--- return if there was an error if(!CopyBufferAsSeries(MFI_handle,0,0,100,true,MFI)) return; }
iMA
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double MA[]; // array for the indicator iMA //---- handles for indicators int MA_handle; // handle of the indicator iMA //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iMA MA_handle=iMA(NULL,0,21,0,MODE_EMA,PRICE_CLOSE); //--- report if there was an error in object creation if(MA_handle<0) { Print("The creation of iMA has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- filling an array MA[] with current values of iMA //--- set indexation of array MA[] as timeseries //--- return if there was an error if(!CopyBufferAsSeries(MA_handle,0,0,100,true,MA)) return; }
iOsMA
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double OsMA[]; // array for the indicator iOsMA //---- handles for indicators int OsMA_handle; // handle of the indicator iOsMA //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iOsMA OsMA_handle=iOsMA(NULL,0,8,21,14,PRICE_MEDIAN); //--- report if there was an error in object creation if(OsMA_handle<0) { Print("The creation of iOsMA has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- filling an array OsMA[] with current values of iOsMA //--- set indexation of array OsMA[] timeseries //--- return if there was an error if(!CopyBufferAsSeries(OsMA_handle,0,0,100,true,OsMA)) return; }
iMACD
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double Main[]; // array for MAIN_LINE of iMACD double Signal[]; // array for SIGNAL_LINE of iMACD //---- handles for indicators int MACD_handle; // handle of the indicator iMACD //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iMACD MACD_handle=iMACD(NULL,0,12,26,9,PRICE_CLOSE); //--- report if there was an error in object creation if(MACD_handle<0) { Print("The creation of iMACD has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- set indexation of arrays as timeseries //--- filling the arrays with current values of all indicator's buffers //--- return if there was an error if(!GetMACDBuffers(MACD_handle,0,100,Main,Signal,true)) return; }
iOBV
#include <GetIndicatorBuffers.mqh> //---- indicator buffers double OBV[]; // array for the indicator iOBV //---- handles for indicators int OBV_handle; // handle for the indicator iOBV //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iOBV OBV_handle=iOBV(NULL,0,VOLUME_TICK); //--- report if there was an error in object creation if(OBV_handle<0) { Print("The creation of iOBV has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- filling an array OBV[] with current values of iOBV //--- set indexation of array AC[] as timeseries //--- return if there was an error if(!CopyBufferAsSeries(OBV_handle,0,0,100,true,OBV)) return; }
iSAR
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double SAR[]; // array for the indicator iSAR //---- handles for indicators int SAR_handle; // handle of the indicator iSAR //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iSAR SAR_handle=iSAR(NULL,0,0.02,0.2); //--- report if there was an error in object creation if(SAR_handle<0) { Print("The creation of iSAR has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- filling an array SAR[] with current values of iSAR //--- set indexation of array SAR[] as timeseries //--- return if there was an error if(!CopyBufferAsSeries(SAR_handle,0,0,100,true,SAR)) return; }
iRSI
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double RSI[]; // array for the indicator iRSI //---- handles for indicators int RSI_handle; // handle of the indicator iRSI //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iRSI RSI_handle=iRSI(NULL,0,21,PRICE_CLOSE); //--- report if there was an error in object creation if(RSI_handle<0) { Print("The creation of iRSI has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- filling an array RSI[] with current values of iRSI //--- set indexation of array RSI[] as timeserie //--- return if there was an error if(!CopyBufferAsSeries(RSI_handle,0,0,100,true,RSI)) return; }
iRVI
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double Main[]; // array for MAIN_LINE of iRVI double Signal[]; // array for SIGNAL_LINE of iRVI //---- handles for indicators int RVI_handle; // handle of the indicator iRVI //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iRVI RVI_handle=iRVI(NULL,0,14); //--- report if there was an error in object creation if(RVI_handle<0) { Print("The creation of iRVI has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- set indexation of arrays as timeseries //--- filling the arrays with current values of all indicator's buffers //--- return if there was an error if(!GetRVIBuffers(RVI_handle,0,100,Main,Signal,true)) return; }
iStdDev
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double StdDev[]; // array for the indicator iStdDev //---- handles for indicators int StdDev_handle; // handle for the indicator iStdDev //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iStdDev StdDev_handle=iStdDev(NULL,0,20,0,MODE_SMA,PRICE_CLOSE); //--- report if there was an error in object creation if(StdDev_handle<0) { Print("The creation of iStdDev has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- filling an array StdDev[] with current values of iStdDev //--- set indexation of array StdDev[] as timeseries //--- return if there was an error if(!CopyBufferAsSeries(StdDev_handle,0,0,100,true,StdDev)) return; }
iStochastic
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double Main[]; // array for MAIN_LINE of iStochastic double Signal[]; // array for SIGNAL_LINE of iStochastic //---- handles for indicators int Stochastic_handle; // handle of the indicator iStochastic //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iStochastic Stochastic_handle=iStochastic(NULL,0,5,3,3,MODE_SMA,STO_LOWHIGH); //--- report if there was an error in object creation if(Stochastic_handle<0) { Print("The creation of iStochastic has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- set indexation of arrays as timeseries //--- filling the arrays with current values of all indicator's buffers //--- return if there was an error if(!GetStochasticBuffers(Stochastic_handle,0,100,Main,Signal,true)) return; }
iTEMA
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double TEMA[]; // array for the indicator iTEMA //---- handles for indicators int TEMA_handle; // handle of the indicator iTEMA //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iTEMA TEMA_handle=iTEMA(NULL,0,20,0,PRICE_CLOSE); //--- report if there was an error in object creation if(TEMA_handle<0) { Print("The creation of iTEMA has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- filling an array TEMA[] with current values of iTEMA //--- set indexation of array TEMA[] as timeseries //--- return if there was an error if(!CopyBufferAsSeries(TEMA_handle,0,0,100,true,TEMA)) return; }
iTriX
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double TriX[]; // array for the indicator iTriX //---- handles for indicators int TriX_handle; // handle of the indicator iTriX //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iTriX TriX_handle=iTriX(NULL,0,20,PRICE_CLOSE); //--- report if there was an error in object creation if(TriX_handle<0) { Print("The creation of iTriX has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- filling an array TriX[] with current values of iTriX //--- set indexation of array TriX[] as timeseries //--- return if there was an error if(!CopyBufferAsSeries(TriX_handle,0,0,100,true,TriX)) return; }
iWPR
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double WPR[]; // array for the indicator iWPR //---- handles for indicators int WPR_handle; // handle of the indicator iWPR //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iWPR WPR_handle=iWPR(NULL,0,14); //--- report if there was an error in object creation if(WPR_handle<0) { Print("The creation of iWPR has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- filling an array WPR[] with current values of iWPR //--- set indexation of array WPR[] as timeseries //--- return if there was an error if(!CopyBufferAsSeries(WPR_handle,0,0,100,true,WPR)) return; }
iVIDyA
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double VIDyA[]; // array for the indicator iVIDyA //---- handles for indicators int VIDyA_handle; // handle of the indicator iVIDyA //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iVIDyA VIDyA_handle=iVIDyA(NULL,0,14,21,0,PRICE_MEDIAN); //--- report if there was an error in object creation if(VIDyA_handle<0) { Print("The creation of iVIDyA has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- filling an array VIDyA[] with current values of iVIDyA //--- set indexation of array VIDyA[] as timeseries //--- return if there was an error if(!CopyBufferAsSeries(VIDyA_handle,0,0,100,true,VIDyA)) return; }
iVolumes
#include <GetIndicatorBuffers.mqh> //---- arrays for indicators double Volumes[]; // array for the indicator iVolumes //---- handles for indicators int Volumes_handle; // handle of the indicator iVolumes //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- creation of the indicator iVolumes Volumes_handle=iVolumes(NULL,0,VOLUME_TICK); //--- report if there was an error in object creation if(Volumes_handle<0) { Print("The creation of iVolumes has failed: Runtime error =",GetLastError()); //--- forced program termination return(-1); } return(0); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- filling an array Volumes[] with current values of iVolumes //--- set indexation of array Volumes[] as timeseries //--- return if there was an error if(!CopyBufferAsSeries(Volumes_handle,0,0,100,true,Volumes)) return; }
結論
- MQL5のExpert Advisorで標準テクニカルインディケーター を使うことは以前のバージョンのように簡単です。
- あなたのExpert Advisorで安全なプログラミング方法だけを使ってください。すべてのエラーを分析し排除します。 ミスの代償はとても大きいです。あなたのデポジットですから!
MetaQuotes Ltdによってロシア語から翻訳されました。
元の記事: https://www.mql5.com/ru/articles/31





- 無料取引アプリ
- 8千を超えるシグナルをコピー
- 金融ニュースで金融マーケットを探索
ターミナルでは選択できるのですが、コードにどのように書くのかがわかりません。
マニュアルにはHandleが必要と書いてあるのですが、バッファしかありません。
よろしくお願いします。
インジケーターのPRICE_CLOSEを変更する方法を教えてください。
ターミナルでは選択できるのですが、コードにどのように書くのかがわかりません。
マニュアルにはHandleが必要と書いてあるのですが、バッファしかありません。
よろしくお願いします。
https://www.mql5.com/ru/articles/15 の記事を見てください。
https:// www.mql5.com/ru/articles/15
Григорий Муратов #:
Подскажите пожалуйста, как в индикаторе, например в
ターミナルでは選択できるのですが、コードにどう書けばいいのかわかりません。
マニュアルにはHandleが必要と書いてあるのですが、バッファしかありません。
よろしくお願いします。
https://www.mql5.com/ru/docs/indicators/ibands ドキュメントを見ると -- 仕様が書いてあります:
-- ここでPRICE_CLOSEの代わりにカスタムインジケーターのハンドルを書くことができます。
例えば