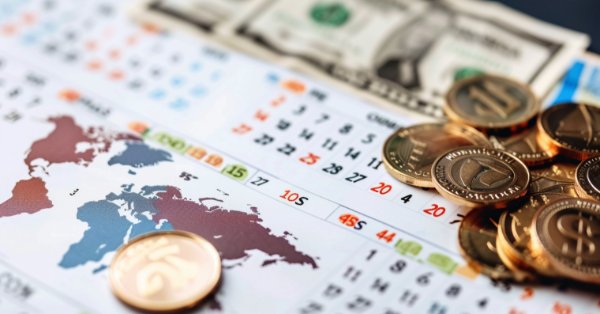
让新闻交易轻松上手(第二部分):风险管理
概述
简单回顾让新闻交易轻松上手系列的前一篇文章。在第一部分中,我们回顾了夏令时(DST)的概念,以及不同国家在不同财政年度将时区提前或推后一小时的各种版本。这将改变使用夏令时的相关经纪公司的交易时间表。文章讨论了创建数据库的原因及好处。创建数据库是为了存储来自MQL5经济日历的新闻事件,并随后更改事件时间数据,以反映经纪公司夏令时的时间表,从而在之后进行准确地回测。在项目文件中,包含一个SQL脚本,该脚本将MQL5日历中所有不同国家的所有特殊事件导出为Excel格式。
然而,在本文中,我们将对第一部分中先前的代码进行一些修改。首先,通过对现有代码和即将编写的新代码实现继承,改进了先前的新闻/日历数据库,使其变得更加实用和方便。此外,我们将解决风险管理问题,并为具有不同风险等级或偏好的用户推荐不同的风险概况以供选择。
继承
什么是继承?
继承是面向对象编程(OOP)中的一个基本概念,它允许一个新类(也称为子类或派生类)从现有类(也称为父类或基类)中继承属性和行为(字段和方法)。这种机制提供了一种通过扩展或修改现有类的行为来创建新类的方法,从而提升了代码的复用性,并创建了更具逻辑性和层次结构的类体系。
继承的目的是什么?
继承能使现有代码被复用。通过从基类继承,子类可以使用现有的方法和字段,而无需重新编写。这减少了冗余,并使代码更易于维护。继承有助于将代码组织成层次结构,使其更容易理解和管理。可以根据共享属性和行为对类进行分组,从而实现构建代码库的简洁性和逻辑性。继承与多态性密切相关,多态性允许不同类的对象被视为共同基类的对象。这对于实现灵活性和可扩展性的代码特别有用。例如,只要不同的类的对象继承自同一个基类,用一个函数就可以对它们进行操作,从而实现动态方法的绑定,也让代码变得更通用。
继承允许扩展现有类的功能。子类可以添加新的方法和字段,或者覆盖现有的方法和字段,以引入特定的行为,而无需修改原始类。这推进了开/闭原则,即类对扩展开放,对修改关闭。继承支持封装,允许子类访问父类受保护的和公共的成员,同时保持实现细节的私有性。这确保了接口和工具之间的清晰分离,增强了模块化,并降低了意外交互的风险。
什么是访问修饰符?
访问修饰符是面向对象编程语言中的关键字,用于设置类、方法/函数和其他成员的访问权限。它们控制这些元素在代码不同部分中的可见性和可访问性,从而强制实现封装并保护数据的完整性。
访问修饰符的类型
- 公有
- 私密
- 受保护
1. 公有
目的:使类、函数或变量可以用于其他类或程序。
2. 私有
目的:限制对类成员的访问,从而保护数据的完整性。
3. 受保护
目的:允许子类继承和访问成员,同时仍然限制对类对象的访问。
与MQL5相关的继承示例
我们将首先为示例创建一个UML类图例,以可视化类及其关系和属性。
类UnitedStates和Switzerland从类 NewsData单向继承:
class NewsData { private://Properties are only accessible from this class string Country;//Private variable struct EventDetails//Private structure { int EventID; string EventName; datetime EventDate; }; protected: //-- Protected Array Only accessible from this class and its children EventDetails News[]; //-- Proctected virtual void Function(to be expanded on via child classes) virtual void SetNews(); //-- Protected Function Only accessible from this class and its children void SetCountry(string myCountry) {Country=myCountry;} public: void GetNews()//Public function to display 'News' array details { PrintFormat("+---------- %s ----------+",Country); for(uint i=0;i<News.Size();i++) { Print("ID: ",News[i].EventID," Name: ",News[i].EventName," Date: ",News[i].EventDate); } } NewsData(void) {}//Class constructor ~NewsData(void) {ArrayFree(News);}//Class destructor }; //+------------------------------------------------------------------+ //|(Subclass/Child) for 'NewsData' | //+------------------------------------------------------------------+ class UnitedStates:private NewsData //private inheritance from NewsData, //'UnitedStates' class's objects and children //will not have access to 'NewsData' class's properties { private: virtual void SetNews()//private Function only Accessible in 'UnitedStates' class { ArrayResize(News,News.Size()+1,News.Size()+2); News[News.Size()-1].EventID = 1; News[News.Size()-1].EventName = "NFP(Non-Farm Payrolls)"; News[News.Size()-1].EventDate = D'2024.01.03 14:00:00'; } public: void myNews()//public Function accessible via class's object { SetCountry("United States");//Calling function from 'NewsData' GetNews();//Calling Function from private inherited class 'NewsData' } UnitedStates(void) {SetNews();}//Class constructor }; //+------------------------------------------------------------------+ //|(Subclass/Child) for 'NewsData' | //+------------------------------------------------------------------+ class Switzerland: public NewsData //public inheritance from NewsData { public: virtual void SetNews()//Public Function to set News data { ArrayResize(News,News.Size()+1,News.Size()+2);//Adjusting News structure array's size News[News.Size()-1].EventID = 0;//Setting event id to '0' News[News.Size()-1].EventName = "Interest Rate Decision";//Assigning event name News[News.Size()-1].EventDate = D'2024.01.06 10:00:00';//Assigning event date } Switzerland(void) {SetCountry("Switerland"); SetNews();}//Class construct };
在此例中:
将NewsData作为父类/基类/超类,任何私有声明仅可通过该类访问。类的对象和子类将无法访问私有声明。而类及其子类均可访问受保护声明。类、其子类以及对象均可访问所有公有声明。
新闻数据的辅助功能表:
类的属性 | 类 | 子类 | 对象 |
---|---|---|---|
变量:Country(Private) | ✔ | ✘ | ✘ |
结构体:EventDetails(Private) | ✔ | ✘ | ✘ |
变量:News(Protected) | ✔ | ✔ | ✘ |
函数:SetNews(Protected) | ✔ | ✔ | ✘ |
函数:SetCountry(Protected) | ✔ | ✔ | ✘ |
函数:GetNews(Public) | ✔ | ✔ | ✔ |
构造函数:NewsData(Public) | ✔ | ✘ | ✘ |
析构函数:~NewsData(Public) | ✔ | ✘ | ✘ |
class NewsData { private://Properties are only accessible from this class string Country;//Private variable struct EventDetails//Private structure { int EventID; string EventName; datetime EventDate; }; protected: //-- Protected Array Only accessible from this class and its children EventDetails News[]; //-- Proctected virtual void Function(to be expanded on via child classes) virtual void SetNews(); //-- Protected Function Only accessible from this class and its children void SetCountry(string myCountry) {Country=myCountry;} public: void GetNews()//Public function to display 'News' array details { PrintFormat("+---------- %s ----------+",Country); for(uint i=0;i<News.Size();i++) { Print("ID: ",News[i].EventID," Name: ",News[i].EventName," Date: ",News[i].EventDate); } } NewsData(void) {}//Class constructor ~NewsData(void) {ArrayFree(News);}//Class destructor };
NewsData对象的可见属性:
NewsData的GetNews函数结果:
继承是用剩下的两个类实现的:
关于UnitedStates类(作为子/附属/派生类),它私有地继承了父类NewsData。
这意味着UnitedStates(子)类可以访问父类NewsData中的受保护性和公共属性,但UnitedStates类的子类及其对象将无法访问父类NewsData的任何属性。 如果继承的Access Modifier是受保护性的,那么UnitedStates类的子类将能够访问父类NewsData的受保护性和公共属性,但UnitedStates类的对象将无法访问父类的任何属性。
UnitedStates类的可访问性表格:
类的属性 | 类 | 子类 | 对象 |
---|---|---|---|
继承(私有)变量:Country(Private) | ✘ | ✘ | ✘ |
继承(私有)结构体:EventDetails(Private) | ✘ | ✘ | ✘ |
继承(私有)变量:News(Protected) | ✔ | ✘ | ✘ |
继承(私有)函数:SetNews(Protected) | ✔ | ✘ | ✘ |
继承(私有)函数:SetCountry(Protected) | ✔ | ✘ | ✘ |
继承(私有)函数:GetNews(Public) | ✔ | ✘ | ✘ |
继承(私有)结构体:NewsData(Public) | ✘ | ✘ | ✘ |
继承(私有)结构体:~NewsData(Public) | ✘ | ✘ | ✘ |
函数:SetNews(Private) | ✔ | ✘ | ✘ |
函数:myNews(Public) | ✔ | ✔ | ✔ |
结构体:UnitedStates(Public) | ✔ | ✘ | ✘ |
class UnitedStates:private NewsData //private inheritance from NewsData, //'UnitedStates' class's objects and children //will not have access to 'NewsData' class's properties { private: virtual void SetNews()//private Function only Accessible in 'UnitedStates' class { ArrayResize(News,News.Size()+1,News.Size()+2); News[News.Size()-1].EventID = 1; News[News.Size()-1].EventName = "NFP(Non-Farm Payrolls)"; News[News.Size()-1].EventDate = D'2024.01.03 14:00:00'; } public: void myNews()//public Function accessible via class's object { SetCountry("United States");//Calling function from 'NewsData' GetNews();//Calling Function from private inherited class 'NewsData' } UnitedStates(void) {SetNews();}//Class constructor };
UnitedStates对象的可见属性:
尝试访问从NewsData类私有继承的GetNews函数时会出现编译错误,这阻止了UnitedStates对象访问该函数。
在(子/附属/派生)类Switzerland中。
继承的访问修饰符是public。这使得子类(Switzerland)的子类能够访问父类(NewsData)的公有属性和受保护性,而Switzerland类的对象只能访问所有相关类的公有属性。
Switzerland的可访问性表格:
类的属性 | 类 | 子类 | 对象 |
---|---|---|---|
继承(公有)变量:Country(Private) | ✘ | ✘ | ✘ |
继承(公有)结构体:EventDetails(Private) | ✘ | ✘ | ✘ |
继承(公有)变量:News(Protected) | ✔ | ✔ | ✘ |
继承(公有)函数:SetNews(Protected) | ✔ | ✔ | ✘ |
继承(公有)函数:SetCountry(Protected) | ✔ | ✔ | ✘ |
继承(公有)函数:GetNews(Public) | ✔ | ✔ | ✔ |
继承(公有)结构体:NewsData(Public) | ✘ | ✘ | ✘ |
继承(公有)析构函数:~NewsData(Public) | ✘ | ✘ | ✘ |
函数:SetNews(Public) | ✔ | ✔ | ✔ |
构造函数:Switzerland(Public) | ✔ | ✘ | ✘ |
class Switzerland: public NewsData //public inheritance from NewsData { public: virtual void SetNews()//Public Function to set News data { ArrayResize(News,News.Size()+1,News.Size()+2);//Adjusting News structure array's size News[News.Size()-1].EventID = 0;//Setting event id to '0' News[News.Size()-1].EventName = "Interest Rate Decision";//Assigning event name News[News.Size()-1].EventDate = D'2024.01.06 10:00:00';//Assigning event date } Switzerland(void) {SetCountry("Switerland"); SetNews();}//Class construct };
Switzerland对象的可见属性:
结果:
夏令时课程
在让新闻交易变得容易(第一部分)中:
UML类图例
项目文件:
在先前的代码中,我们有三个夏令时类,即:
- CDaylightSavings_AU
- CDaylightSavings_UK
- CDaylightSavings_US
在第二部分中:
UML类图例
项目文件:
我们将为夏令时设置以下类,即:
- CDaylightSavings
- CDaylightSavings_AU
- CDaylightSavings_UK
- CDaylightSavings_US
为什么创建另一个夏令时类?
在先前的类代码中,各个类之间存在大量重复,这基本上是为列表中的不同值重复编写了相同的代码。为了避免这种在相似类之间的重复代码,我们将所有共性提取到一个单独的类中,并让不同的夏令时类继承这些共同特征。
什么是虚函数?
在面向对象编程(OOP)中,虚函数是基类中的一个成员函数,您可以在派生类中对其进行重写。当函数被声明为虚函数时,它实现了多态性,允许派生类完成该函数的特定实现,可以通过基类指针或引用来调用。
目的?
- 多态性:虚函数允许动态(运行时)多态性。这意味着执行方式是在运行时根据被引用对象的实际类型来确定的,而不是根据引用或指针的类型。
- 灵活性:它们通过允许派生类修改或扩展基类行为,使代码更加灵活和可重用。
- 解耦:虚函数有助于代码解耦,通过将接口与实现分离,使得更改实现更加容易,而不会影响到使用基类接口的代码。
CDaylightSavings类
在该类中,我们将之前代码的所有共同属性放入一个类中,并将声明几个虚函数来初始化各自夏令时计划的不同时间表。
CDaylightSavings类单一继承自CObject类。
CDaylightSavings类包含了以下类成员:
- CArrayObj
- CTimeManagement
//+------------------------------------------------------------------+ //| NewsTrading | //| Copyright 2024, MetaQuotes Ltd. | //| https://www.mql5.com/en/users/kaaiblo | //+------------------------------------------------------------------+ #include <Object.mqh> #include <Arrays\ArrayObj.mqh> #include "../TimeManagement.mqh" //+------------------------------------------------------------------+ //|DaylightSavings class | //+------------------------------------------------------------------+ class CDaylightSavings: public CObject { protected: CTimeManagement Time; CDaylightSavings(datetime startdate,datetime enddate); CObject *List() { return savings;}//Gets the list of Daylightsavings time datetime StartDate; datetime EndDate; CArrayObj *savings; CArrayObj *getSavings; CDaylightSavings *dayLight; virtual void SetDaylightSavings_UK();//Initialize UK Daylight Savings Dates into List virtual void SetDaylightSavings_US();//Initialize US Daylight Savings Dates into List virtual void SetDaylightSavings_AU();//Initialize AU Daylight Savings Dates into List public: CDaylightSavings(void); ~CDaylightSavings(void); bool isDaylightSavings(datetime Date);//This function checks if a given date falls within Daylight Savings Time. bool DaylightSavings(int Year,datetime &startDate,datetime &endDate);//Check if DaylightSavings Dates are available for a certain Year string adjustDaylightSavings(datetime EventDate);//Will adjust the date's timezone depending on DaylightSavings }; //+------------------------------------------------------------------+ //|Constructor | //+------------------------------------------------------------------+ CDaylightSavings::CDaylightSavings(void) { } //+------------------------------------------------------------------+ //|Initialize variables | //+------------------------------------------------------------------+ CDaylightSavings::CDaylightSavings(datetime startdate,datetime enddate) { StartDate = startdate;//Assign class's global variable StartDate value from parameter variable startdate EndDate = enddate;//Assign class's global variable EndDate value from parameter variable enddate } //+------------------------------------------------------------------+ //|checks if a given date falls within Daylight Savings Time | //+------------------------------------------------------------------+ bool CDaylightSavings::isDaylightSavings(datetime Date) { // Initialize a list to store daylight savings periods. getSavings = List(); // Iterate through all the periods in the list. for(int i=0; i<getSavings.Total(); i++) { // Access the current daylight savings period. dayLight = getSavings.At(i); // Check if the given date is within the current daylight savings period. if(Time.DateIsInRange(dayLight.StartDate,dayLight.EndDate,Date)) { // If yes, return true indicating it is daylight savings time. return true; } } // If no period matches, return false indicating it is not daylight savings time. return false; } //+------------------------------------------------------------------+ //|Check if DaylightSavings Dates are available for a certain Year | //+------------------------------------------------------------------+ bool CDaylightSavings::DaylightSavings(int Year,datetime &startDate,datetime &endDate) { // Initialize a list to store daylight savings periods. getSavings = List(); bool startDateDetected=false,endDateDetected=false; // Iterate through all the periods in the list. for(int i=0; i<getSavings.Total(); i++) { dayLight = getSavings.At(i); if(Year==Time.ReturnYear(dayLight.StartDate))//Check if a certain year's date is available within the DaylightSavings start dates in the List { startDate = dayLight.StartDate; startDateDetected = true; } if(Year==Time.ReturnYear(dayLight.EndDate))//Check if a certain year's date is available within the DaylightSavings end dates in the List { endDate = dayLight.EndDate; endDateDetected = true; } if(startDateDetected&&endDateDetected)//Check if both DaylightSavings start and end dates are found for a certain Year { return true; } } startDate = D'1970.01.01 00:00:00';//Set a default start date if no DaylightSaving date is found endDate = D'1970.01.01 00:00:00';//Set a default end date if no DaylightSaving date is found return false; } //+------------------------------------------------------------------+ //|Will adjust the date's timezone depending on DaylightSavings | //+------------------------------------------------------------------+ string CDaylightSavings::adjustDaylightSavings(datetime EventDate) { if(isDaylightSavings(TimeTradeServer()))//Check if the current tradeserver time is already within the DaylightSavings Period { if(isDaylightSavings(EventDate))//Checks if the event time is during daylight savings { return TimeToString(EventDate);//normal event time } else { return TimeToString((datetime)(EventDate-Time.HoursS()));//event time minus an hour for DST } } else { if(isDaylightSavings(EventDate))//Checks if the event time is during daylight savings { return TimeToString((datetime)(Time.HoursS()+EventDate));//event time plus an hour for DST } else { return TimeToString(EventDate);//normal event time } } } //+------------------------------------------------------------------+ //|Destructor | //+------------------------------------------------------------------+ CDaylightSavings::~CDaylightSavings(void) { delete savings;//Delete CArrayObj Pointer delete dayLight;//Delete CDaylightSavings Pointer delete getSavings;//Delete CArrayObj Pointer } //+------------------------------------------------------------------+
CDaylightSavings_AU类
在该类中,我们扩展了虚函数SetDaylightSavings_AU,并继续添加澳大利亚的夏令时计划。
澳大利亚的夏令时日期在这里。
CDaylightSavings_AU类多层级继承自其他类:
- CDaylightSavings
- CObject
CDaylightSavings_AU类分层级继承自其他类:
- CDaylightSavings
- CArrayObj
- CTimeManagement
//+------------------------------------------------------------------+ //| NewsTrading | //| Copyright 2024, MetaQuotes Ltd. | //| https://www.mql5.com/en/users/kaaiblo | //+------------------------------------------------------------------+ #include "DaylightSavings.mqh" //+------------------------------------------------------------------+ //|DaylightSavings_AU class | //+------------------------------------------------------------------+ class CDaylightSavings_AU: public CDaylightSavings { public: CDaylightSavings_AU(void); }; //+------------------------------------------------------------------+ //|Set Daylight Savings Schedule for Australia | //+------------------------------------------------------------------+ void CDaylightSavings::SetDaylightSavings_AU() { savings = new CArrayObj(); //Daylight savings dates to readjust dates in the database for accurate testing in the strategy tester savings.Add(new CDaylightSavings(D'2006.10.29 03:00:00',D'2007.03.25 02:00:00')); savings.Add(new CDaylightSavings(D'2007.10.28 03:00:00',D'2008.04.06 02:00:00')); savings.Add(new CDaylightSavings(D'2008.10.05 03:00:00',D'2009.04.05 02:00:00')); savings.Add(new CDaylightSavings(D'2009.10.04 03:00:00',D'2010.04.04 02:00:00')); savings.Add(new CDaylightSavings(D'2010.10.03 03:00:00',D'2011.04.03 02:00:00')); savings.Add(new CDaylightSavings(D'2011.10.02 03:00:00',D'2012.04.01 02:00:00')); savings.Add(new CDaylightSavings(D'2012.10.07 03:00:00',D'2013.04.07 02:00:00')); savings.Add(new CDaylightSavings(D'2013.10.06 03:00:00',D'2014.04.06 02:00:00')); savings.Add(new CDaylightSavings(D'2014.10.05 03:00:00',D'2015.04.05 02:00:00')); savings.Add(new CDaylightSavings(D'2015.10.04 03:00:00',D'2016.04.03 02:00:00')); savings.Add(new CDaylightSavings(D'2016.10.02 03:00:00',D'2017.04.02 02:00:00')); savings.Add(new CDaylightSavings(D'2017.10.01 03:00:00',D'2018.04.01 02:00:00')); savings.Add(new CDaylightSavings(D'2018.10.07 03:00:00',D'2019.04.07 02:00:00')); savings.Add(new CDaylightSavings(D'2019.10.06 03:00:00',D'2020.04.05 02:00:00')); savings.Add(new CDaylightSavings(D'2020.10.04 03:00:00',D'2021.04.04 02:00:00')); savings.Add(new CDaylightSavings(D'2021.10.03 03:00:00',D'2022.04.03 02:00:00')); savings.Add(new CDaylightSavings(D'2022.10.02 03:00:00',D'2023.04.02 02:00:00')); savings.Add(new CDaylightSavings(D'2023.10.01 03:00:00',D'2024.04.07 02:00:00')); savings.Add(new CDaylightSavings(D'2024.10.06 03:00:00',D'2025.04.06 02:00:00')); savings.Add(new CDaylightSavings(D'2025.10.05 03:00:00',D'2026.04.05 02:00:00')); savings.Add(new CDaylightSavings(D'2026.10.04 03:00:00',D'2027.04.04 02:00:00')); savings.Add(new CDaylightSavings(D'2027.10.03 03:00:00',D'2028.04.02 02:00:00')); savings.Add(new CDaylightSavings(D'2028.10.01 03:00:00',D'2029.04.01 02:00:00')); } //+------------------------------------------------------------------+ //|Constructor | //+------------------------------------------------------------------+ CDaylightSavings_AU::CDaylightSavings_AU(void) { SetDaylightSavings_AU(); } //+------------------------------------------------------------------+
CDaylightSavings_UK类
在该类中,我们扩展了虚空SetDaylightSavings_UK,并继续添加欧洲的夏令时计划。
英国的夏令时日期在这里。
CDaylightSavings_UK类多层级继承自其他类:
- CDaylightSavings
- CObject
CDaylightSavings_UK类分层级继承自其他类:
- CDaylightSavings
- CArrayObj
- CTimeManagement
//+------------------------------------------------------------------+ //| NewsTrading | //| Copyright 2024, MetaQuotes Ltd. | //| https://www.mql5.com/en/users/kaaiblo | //+------------------------------------------------------------------+ #include "DaylightSavings.mqh" //+------------------------------------------------------------------+ //|DaylightSavings_UK class | //+------------------------------------------------------------------+ class CDaylightSavings_UK: public CDaylightSavings { public: CDaylightSavings_UK(void); }; //+------------------------------------------------------------------+ //|Set Daylight Savings Schedule for Europe | //+------------------------------------------------------------------+ void CDaylightSavings::SetDaylightSavings_UK() { savings = new CArrayObj(); //Daylight savings dates to readjust dates in the database for accurate testing in the strategy tester savings.Add(new CDaylightSavings(D'2007.03.25 02:00:00',D'2007.10.28 01:00:00')); savings.Add(new CDaylightSavings(D'2008.03.30 02:00:00',D'2008.10.26 01:00:00')); savings.Add(new CDaylightSavings(D'2009.03.29 02:00:00',D'2009.10.25 01:00:00')); savings.Add(new CDaylightSavings(D'2010.03.28 02:00:00',D'2010.10.31 01:00:00')); savings.Add(new CDaylightSavings(D'2011.03.27 02:00:00',D'2011.10.30 01:00:00')); savings.Add(new CDaylightSavings(D'2012.03.25 02:00:00',D'2012.10.28 01:00:00')); savings.Add(new CDaylightSavings(D'2013.03.31 02:00:00',D'2013.10.27 01:00:00')); savings.Add(new CDaylightSavings(D'2014.03.30 02:00:00',D'2014.10.26 01:00:00')); savings.Add(new CDaylightSavings(D'2015.03.29 02:00:00',D'2015.10.25 01:00:00')); savings.Add(new CDaylightSavings(D'2016.03.27 02:00:00',D'2016.10.30 01:00:00')); savings.Add(new CDaylightSavings(D'2017.03.26 02:00:00',D'2017.10.29 01:00:00')); savings.Add(new CDaylightSavings(D'2018.03.25 02:00:00',D'2018.10.28 01:00:00')); savings.Add(new CDaylightSavings(D'2019.03.31 02:00:00',D'2019.10.27 01:00:00')); savings.Add(new CDaylightSavings(D'2020.03.29 02:00:00',D'2020.10.25 01:00:00')); savings.Add(new CDaylightSavings(D'2021.03.28 02:00:00',D'2021.10.31 01:00:00')); savings.Add(new CDaylightSavings(D'2022.03.27 02:00:00',D'2022.10.30 01:00:00')); savings.Add(new CDaylightSavings(D'2023.03.26 02:00:00',D'2023.10.29 01:00:00')); savings.Add(new CDaylightSavings(D'2024.03.31 02:00:00',D'2024.10.27 01:00:00')); savings.Add(new CDaylightSavings(D'2025.03.30 02:00:00',D'2025.10.26 01:00:00')); savings.Add(new CDaylightSavings(D'2026.03.29 02:00:00',D'2026.10.25 01:00:00')); savings.Add(new CDaylightSavings(D'2027.03.28 02:00:00',D'2027.10.31 01:00:00')); savings.Add(new CDaylightSavings(D'2028.03.26 02:00:00',D'2028.10.29 01:00:00')); savings.Add(new CDaylightSavings(D'2029.03.25 02:00:00',D'2029.10.28 01:00:00')); } //+------------------------------------------------------------------+ //|Constructor | //+------------------------------------------------------------------+ CDaylightSavings_UK::CDaylightSavings_UK(void) { SetDaylightSavings_UK(); } //+------------------------------------------------------------------+
CDaylightSavings_US类
在这节课中,我们将对虚空函数SetDaylightSavings_US进行扩展,并添加美国的夏令时时间表。
美国的夏令时日期在这里。
CDaylightSavings_US类多层级继承自其他类。
- CDaylightSavings
- CObject
CDaylightSavings_US类分层级继承自其他类:
- CDaylightSavings
- CArrayObj
- CTimeManagement
//+------------------------------------------------------------------+ //| NewsTrading | //| Copyright 2024, MetaQuotes Ltd. | //| https://www.mql5.com/en/users/kaaiblo | //+------------------------------------------------------------------+ #include "DaylightSavings.mqh" //+------------------------------------------------------------------+ //|DaylightSavings_US Class | //+------------------------------------------------------------------+ class CDaylightSavings_US: public CDaylightSavings { public: CDaylightSavings_US(void); }; //+------------------------------------------------------------------+ //|Set Daylight Savings Schedule for the United States | //+------------------------------------------------------------------+ void CDaylightSavings::SetDaylightSavings_US() { savings = new CArrayObj(); //Daylight savings dates to readjust dates in the database for accurate testing in the strategy tester savings.Add(new CDaylightSavings(D'2007.03.11 03:00:00',D'2007.11.04 01:00:00')); savings.Add(new CDaylightSavings(D'2008.03.09 03:00:00',D'2008.11.02 01:00:00')); savings.Add(new CDaylightSavings(D'2009.03.08 03:00:00',D'2009.11.01 01:00:00')); savings.Add(new CDaylightSavings(D'2010.03.14 03:00:00',D'2010.11.07 01:00:00')); savings.Add(new CDaylightSavings(D'2011.03.13 03:00:00',D'2011.11.06 01:00:00')); savings.Add(new CDaylightSavings(D'2012.03.11 03:00:00',D'2012.11.04 01:00:00')); savings.Add(new CDaylightSavings(D'2013.03.10 03:00:00',D'2013.11.03 01:00:00')); savings.Add(new CDaylightSavings(D'2014.03.09 03:00:00',D'2014.11.02 01:00:00')); savings.Add(new CDaylightSavings(D'2015.03.08 03:00:00',D'2015.11.01 01:00:00')); savings.Add(new CDaylightSavings(D'2016.03.13 03:00:00',D'2016.11.06 01:00:00')); savings.Add(new CDaylightSavings(D'2017.03.12 03:00:00',D'2017.11.05 01:00:00')); savings.Add(new CDaylightSavings(D'2018.03.11 03:00:00',D'2018.11.04 01:00:00')); savings.Add(new CDaylightSavings(D'2019.03.10 03:00:00',D'2019.11.03 01:00:00')); savings.Add(new CDaylightSavings(D'2020.03.08 03:00:00',D'2020.11.01 01:00:00')); savings.Add(new CDaylightSavings(D'2021.03.14 03:00:00',D'2021.11.07 01:00:00')); savings.Add(new CDaylightSavings(D'2022.03.13 03:00:00',D'2022.11.06 01:00:00')); savings.Add(new CDaylightSavings(D'2023.03.12 03:00:00',D'2023.11.05 01:00:00')); savings.Add(new CDaylightSavings(D'2024.03.10 03:00:00',D'2024.11.03 01:00:00')); savings.Add(new CDaylightSavings(D'2025.03.09 03:00:00',D'2025.11.02 01:00:00')); savings.Add(new CDaylightSavings(D'2026.03.08 03:00:00',D'2026.11.01 01:00:00')); savings.Add(new CDaylightSavings(D'2027.03.14 03:00:00',D'2027.11.07 01:00:00')); savings.Add(new CDaylightSavings(D'2028.03.12 03:00:00',D'2028.11.05 01:00:00')); savings.Add(new CDaylightSavings(D'2029.03.11 03:00:00',D'2029.11.04 01:00:00')); } //+------------------------------------------------------------------+ //|Constructor | //+------------------------------------------------------------------+ CDaylightSavings_US::CDaylightSavings_US(void) { SetDaylightSavings_US(); } //+------------------------------------------------------------------+
有关交易品种属性的类
在该类中,我们将设置一个交易品种,从中检索数据。这个类将为我们提供一个简便快捷的方法,以便在其他类中获取交易品种的属性,从而消除代码中的冗余。
我们将选择一组属性来检索,列表可以扩展,但当前列表如下:
- 买入价
- 卖出价
- 合约规模
- 最小交易量
- 最大交易量
- 交易量步长
- 交易量限制
- 点差
- 最小止损水平
- 冻结水平
- 交易品种的时间
- 交易品种的标准化价格
- 交易品种的报价精度
- 交易品种的价格最小变动点值
- 交易品种的交易模式
- 交易品种的累计订单量
- 交易品种的累计头寸量
- 交易品种的货币基准
- 交易品种的盈利
- 交易品种的保证金
- 交易品种的自定义状态
- 交易品种的背景颜色
CSymbolProperties类包含了来自CSymbolInfo类的成员或信息。
//+------------------------------------------------------------------+ //| NewsTrading | //| Copyright 2024, MetaQuotes Ltd. | //| https://www.mql5.com/en/users/kaaiblo | //+------------------------------------------------------------------+ #include <Trade/SymbolInfo.mqh> //+------------------------------------------------------------------+ //|SymbolProperties class | //+------------------------------------------------------------------+ class CSymbolProperties { private: double ASK;//Store Ask Price double BID;//Store Bid Price double LOTSMIN;//Store Minimum Lotsize double LOTSMAX;//Store Maximum Lotsize double LOTSSTEP;//Store Lotsize Step double LOTSLIMIT;//Store Lotsize Limit(Maximum sum of Volume) long SPREAD;//Store Spread value long STOPLEVEL;//Store Stop level long FREEZELEVEL;//Store Freeze level long TIME;//Store time long DIGITS;//Store Digits double POINT;//Store Point double ORDERSVOLUME;//Store Orders volume double POSITIONSVOLUME;//Store Positions volume long CUSTOM;//Store if Symbol is Custom long BACKGROUND_CLR;//Store Symbol's background color protected: CSymbolInfo CSymbol;//Creating class CSymbolInfo's Object bool SetSymbolName(string SYMBOL) { //-- If Symbol's name was successfully set. if(!CSymbol.Name((SYMBOL==NULL)?Symbol():SYMBOL)) { Print("Invalid Symbol: ",SYMBOL); return false; } return true; } //-- Retrieve Symbol's name string GetSymbolName() { return CSymbol.Name(); } public: CSymbolProperties(void);//Constructor double Ask(string SYMBOL=NULL);//Retrieve Ask Price double Bid(string SYMBOL=NULL);//Retrieve Bid Price double ContractSize(string SYMBOL=NULL);//Retrieve Contract Size double LotsMin(string SYMBOL=NULL);//Retrieve Min Volume double LotsMax(string SYMBOL=NULL);//Retrieve Max Volume double LotsStep(string SYMBOL=NULL);//Retrieve Volume Step double LotsLimit(string SYMBOL=NULL);//Retrieve Volume Limit int Spread(string SYMBOL=NULL);//Retrieve Spread int StopLevel(string SYMBOL=NULL);//Retrieve Stop Level int FreezeLevel(string SYMBOL=NULL);//Retrieve Freeze Level datetime Time(string SYMBOL=NULL);//Retrieve Symbol's Time //-- Normalize Price double NormalizePrice(const double price,string SYMBOL=NULL); int Digits(string SYMBOL=NULL);//Retrieve Symbol's Digits double Point(string SYMBOL=NULL);//Retrieve Symbol's Point ENUM_SYMBOL_TRADE_MODE TradeMode(string SYMBOL=NULL);//Retrieve Symbol's Trade Mode double OrdersVolume(string SYMBOL=NULL);//Retrieve Symbol's Orders Volume double PositionsVolume(string SYMBOL=NULL);//Retrieve Symbol's Positions Volume string CurrencyBase(string SYMBOL=NULL);//Retrieve Symbol's Currency Base string CurrencyProfit(string SYMBOL=NULL);//Retrieve Symbol's Currency Profit string CurrencyMargin(string SYMBOL=NULL);//Retrieve Symbol's Currency Margin bool Custom(string SYMBOL=NULL);//Retrieve Symbol's Custom status color SymbolBackground(string SYMBOL=NULL,bool allow_black=false);//Retrieve Symbol's Background color }; //+------------------------------------------------------------------+ //|Constructor | //+------------------------------------------------------------------+ //Initializing Variables CSymbolProperties::CSymbolProperties(void):ASK(0.0),BID(0.0), LOTSMIN(0.0),LOTSMAX(0.0), LOTSSTEP(0.0),LOTSLIMIT(0.0),DIGITS(0), SPREAD(0),STOPLEVEL(0),ORDERSVOLUME(0.0), FREEZELEVEL(0),TIME(0),POINT(0.0),POSITIONSVOLUME(0.0), CUSTOM(0),BACKGROUND_CLR(0) { } //+------------------------------------------------------------------+ //|Retrieve Ask Price | //+------------------------------------------------------------------+ double CSymbolProperties::Ask(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.InfoDouble(SYMBOL_ASK,ASK)) { return ASK; } } Print("Unable to retrieve Symbol's Ask Price"); return 0.0; } //+------------------------------------------------------------------+ //|Retrieve Bid Price | //+------------------------------------------------------------------+ double CSymbolProperties::Bid(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.InfoDouble(SYMBOL_BID,BID)) { return BID; } } Print("Unable to retrieve Symbol's Bid Price"); return 0.0; } //+------------------------------------------------------------------+ //|Retrieve Contract Size | //+------------------------------------------------------------------+ double CSymbolProperties::ContractSize(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.Refresh()) { return CSymbol.ContractSize(); } } Print("Unable to retrieve Symbol's Contract size"); return 0.0; } //+------------------------------------------------------------------+ //|Retrieve Min Volume | //+------------------------------------------------------------------+ double CSymbolProperties::LotsMin(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.InfoDouble(SYMBOL_VOLUME_MIN,LOTSMIN)) { return LOTSMIN; } } Print("Unable to retrieve Symbol's LotsMin"); return 0.0; } //+------------------------------------------------------------------+ //|Retrieve Max Volume | //+------------------------------------------------------------------+ double CSymbolProperties::LotsMax(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.InfoDouble(SYMBOL_VOLUME_MAX,LOTSMAX)) { return LOTSMAX; } } Print("Unable to retrieve Symbol's LotsMax"); return 0.0; } //+------------------------------------------------------------------+ //|Retrieve Volume Step | //+------------------------------------------------------------------+ double CSymbolProperties::LotsStep(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.InfoDouble(SYMBOL_VOLUME_STEP,LOTSSTEP)) { return LOTSSTEP; } } Print("Unable to retrieve Symbol's LotsStep"); return 0.0; } //+------------------------------------------------------------------+ //|Retrieve Volume Limit | //+------------------------------------------------------------------+ double CSymbolProperties::LotsLimit(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.InfoDouble(SYMBOL_VOLUME_LIMIT,LOTSLIMIT)) { return LOTSLIMIT; } } Print("Unable to retrieve Symbol's LotsLimit"); return 0.0; } //+------------------------------------------------------------------+ //|Retrieve Spread | //+------------------------------------------------------------------+ int CSymbolProperties::Spread(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.InfoInteger(SYMBOL_SPREAD,SPREAD)) { return int(SPREAD); } } Print("Unable to retrieve Symbol's Spread"); return 0; } //+------------------------------------------------------------------+ //|Retrieve Stop Level | //+------------------------------------------------------------------+ int CSymbolProperties::StopLevel(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.InfoInteger(SYMBOL_TRADE_STOPS_LEVEL,STOPLEVEL)) { return int(STOPLEVEL); } } Print("Unable to retrieve Symbol's StopLevel"); return 0; } //+------------------------------------------------------------------+ //|Retrieve Freeze Level | //+------------------------------------------------------------------+ int CSymbolProperties::FreezeLevel(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.InfoInteger(SYMBOL_TRADE_FREEZE_LEVEL,FREEZELEVEL)) { return int(FREEZELEVEL); } } Print("Unable to retrieve Symbol's FreezeLevel"); return 0; } //+------------------------------------------------------------------+ //|Retrieve Symbol's Time | //+------------------------------------------------------------------+ datetime CSymbolProperties::Time(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.InfoInteger(SYMBOL_TIME,TIME)) { return datetime(TIME); } } Print("Unable to retrieve Symbol's Time"); TIME=0; return datetime(TIME); } //+------------------------------------------------------------------+ //|Normalize Price | //+------------------------------------------------------------------+ double CSymbolProperties::NormalizePrice(const double price,string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.Refresh()&&CSymbol.RefreshRates()) { return CSymbol.NormalizePrice(price); } } Print("Unable to Normalize Symbol's Price"); return price; } //+------------------------------------------------------------------+ //|Retrieve Symbol's Digits | //+------------------------------------------------------------------+ int CSymbolProperties::Digits(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.InfoInteger(SYMBOL_DIGITS,DIGITS)) { return int(DIGITS); } } Print("Unable to retrieve Symbol's Digits"); return 0; } //+------------------------------------------------------------------+ //|Retrieve Symbol's Point | //+------------------------------------------------------------------+ double CSymbolProperties::Point(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.InfoDouble(SYMBOL_POINT,POINT)) { return POINT; } } Print("Unable to retrieve Symbol's Point"); return 0.0; } //+------------------------------------------------------------------+ //|Retrieve Symbol's Trade Mode | //+------------------------------------------------------------------+ ENUM_SYMBOL_TRADE_MODE CSymbolProperties::TradeMode(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.Refresh()) { return CSymbol.TradeMode(); } } Print("Unable to retrieve Symbol's TradeMode"); return SYMBOL_TRADE_MODE_DISABLED; } //+------------------------------------------------------------------+ //|Retrieve Symbol's Orders Volume | //+------------------------------------------------------------------+ double CSymbolProperties::OrdersVolume(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { for(int i=0; i<OrdersTotal(); i++) { if(OrderSelect(OrderGetTicket(i))) { if(OrderGetString(ORDER_SYMBOL)==GetSymbolName()) { ORDERSVOLUME+=OrderGetDouble(ORDER_VOLUME_CURRENT); } } } } else { Print("Unable to retrieve Symbol's OrdersVolume"); return 0.0; } return ORDERSVOLUME; } //+------------------------------------------------------------------+ //|Retrieve Symbol's Positions Volume | //+------------------------------------------------------------------+ double CSymbolProperties::PositionsVolume(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { for(int i=0; i<PositionsTotal(); i++) { if(PositionGetTicket(i)>0) { if(PositionGetString(POSITION_SYMBOL)==GetSymbolName()) { POSITIONSVOLUME+=PositionGetDouble(POSITION_VOLUME); } } } } else { Print("Unable to retrieve Symbol's PositionsVolume"); return 0.0; } return POSITIONSVOLUME; } //+------------------------------------------------------------------+ //|Retrieve Symbol's Currency Base | //+------------------------------------------------------------------+ string CSymbolProperties::CurrencyBase(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.Refresh()) { return CSymbol.CurrencyBase(); } } Print("Unable to retrieve Symbol's CurrencyBase"); return ""; } //+------------------------------------------------------------------+ //|Retrieve Symbol's Currency Profit | //+------------------------------------------------------------------+ string CSymbolProperties::CurrencyProfit(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.Refresh()) { return CSymbol.CurrencyProfit(); } } Print("Unable to retrieve Symbol's CurrencyProfit"); return ""; } //+------------------------------------------------------------------+ //|Retrieve Symbol's Currency Margin | //+------------------------------------------------------------------+ string CSymbolProperties::CurrencyMargin(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.Refresh()) { return CSymbol.CurrencyMargin(); } } Print("Unable to retrieve Symbol's CurrencyMargin"); return ""; } //+------------------------------------------------------------------+ //|Retrieve Symbol's Custom status | //+------------------------------------------------------------------+ bool CSymbolProperties::Custom(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.InfoInteger(SYMBOL_CUSTOM,CUSTOM)) { return bool(CUSTOM); } } Print("Unable to retrieve if Symbol is Custom"); return false; } //+------------------------------------------------------------------+ //|Retrieve Symbol's Background color | //+------------------------------------------------------------------+ color CSymbolProperties::SymbolBackground(string SYMBOL=NULL,bool allow_black=false) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.InfoInteger(SYMBOL_BACKGROUND_COLOR,BACKGROUND_CLR)) { /*Avoid any Symbol black background color */ BACKGROUND_CLR = ((ColorToString(color(BACKGROUND_CLR))=="0,0,0"|| color(BACKGROUND_CLR)==clrBlack)&&!allow_black)? long(StringToColor("236,236,236")):BACKGROUND_CLR; return color(BACKGROUND_CLR); } } Print("Unable to retrieve Symbol's Background color"); return color(StringToColor("236,236,236"));//Retrieve a lightish gray color } //+------------------------------------------------------------------+
在以下类的构造函数中,我们初始化了之前声明的变量,例如双精度浮点变量ASK,并且由于我们尚未获得该交易品种的买入价(Ask)价格,因此我们将ASK的值赋为0.0。
//Initializing Variables CSymbolProperties::CSymbolProperties(void):ASK(0.0),BID(0.0), LOTSMIN(0.0),LOTSMAX(0.0), LOTSSTEP(0.0),LOTSLIMIT(0.0),DIGITS(0), SPREAD(0),STOPLEVEL(0),ORDERSVOLUME(0.0), FREEZELEVEL(0),TIME(0),POINT(0.0),POSITIONSVOLUME(0.0), CUSTOM(0),BACKGROUND_CLR(0) { }
在以下的代码中,我们按照步骤顺序最终检索出该交易品种的买入价。
1.首先,我们有一个可选参数,可以决定是否在变量SYMBOL中输入或编辑交易品种名称。
2. 然后,我们将交易品种名称设置为参数值。如果参数值仍然是默认值NULL,我们将假定我们想要获取当前图表交易品种的属性——即Symbol()。
3. 如果我们无法找到交易品种名称,我们将打印一条错误信息,通知用户无法检索到该交易品种的买入价价格,并返回0.0。
4. 一旦我们可以设置交易品种名称,就会检索该特定交易品种的买入价价格,并返回该值。
double CSymbolProperties::Ask(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL)) { if(CSymbol.InfoDouble(SYMBOL_ASK,ASK)) { return ASK; } } Print("Unable to retrieve Symbol's Ask Price"); return 0.0; }
bool SetSymbolName(string SYMBOL)
{
//-- If Symbol's name was successfully set.
if(!CSymbol.Name((SYMBOL==NULL)?Symbol():SYMBOL))
{
Print("Invalid Symbol: ",SYMBOL);
return false;
}
return true;
}
以下函数将根据SYMBOL变量检索交易品种的卖出价(Bid)价格。
double CSymbolProperties::Bid(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.InfoDouble(SYMBOL_BID,BID)) { return BID; } } Print("Unable to retrieve Symbol's Bid Price"); return 0.0; }
以下函数将检索交易品种的合约规模。交易品种的合约规模对交易者有影响,因为较大的合约规模会增加个人交易的风险。而较小的合约规模则会降低个人交易的风险。
double CSymbolProperties::ContractSize(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.Refresh()) { return CSymbol.ContractSize(); } } Print("Unable to retrieve Symbol's Contract size"); return 0.0; }
以下函数将检索交易品种所允许的最小交易手数/交易量。这意味着交易者不能以低于最小手数的交易量开设仓位。
double CSymbolProperties::LotsMin(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.InfoDouble(SYMBOL_VOLUME_MIN,LOTSMIN)) { return LOTSMIN; } } Print("Unable to retrieve Symbol's LotsMin"); return 0.0; }
以下函数将检索交易品类允许的最大手数/交易量。这意味着交易者将无法开设手数/交易量高于此最大值的头寸,但他们可以根据经纪商的交易量限制和账户订单限制,开设多个头寸,这些头寸的手数/交易量总和可能高于最大值。

double CSymbolProperties::LotsMax(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.InfoDouble(SYMBOL_VOLUME_MAX,LOTSMAX)) { return LOTSMAX; } } Print("Unable to retrieve Symbol's LotsMax"); return 0.0; }
以下函数将检索交易品种的交易量/手数步长。这意味着手数应该按照此步长的间隔来选择。例如,如果交易量步长是1,那么交易者无法选择1.5这样的手数/交易量。

double CSymbolProperties::LotsStep(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.InfoDouble(SYMBOL_VOLUME_STEP,LOTSSTEP)) { return LOTSSTEP; } } Print("Unable to retrieve Symbol's LotsStep"); return 0.0; }
以下函数将检索交易品种的交易量/手数限制。这是指在针对该特定交易品种对交易者账户实施限制之前,允许设置的交易量/手数总和。

double CSymbolProperties::LotsLimit(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.InfoDouble(SYMBOL_VOLUME_LIMIT,LOTSLIMIT)) { return LOTSLIMIT; } } Print("Unable to retrieve Symbol's LotsLimit"); return 0.0; }
以下函数将检索交易品种的点差。点差对交易者有影响,因为交易品种的点差越高,交易者的盈利就越少。根据点差的大小,一个策略可能盈利也可能不盈利。当然,有很多不同的因素可能导致策略不盈利,但点差可能是一个重要因素。点差本质上是经纪商的一种收入来源,你可以把它看作是经纪商对该交易品种收取的“税”。
int CSymbolProperties::Spread(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.InfoInteger(SYMBOL_SPREAD,SPREAD)) { return int(SPREAD); } } Print("Unable to retrieve Symbol's Spread"); return 0; }
以下函数将检索交易品种的止损水平限制。这是指开盘价与止损价或止盈价之间的最小差距限制,以及当前买入价(Ask)或卖出价(Bid)与开盘订单价格之间的最小差距限制。
int CSymbolProperties::StopLevel(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.InfoInteger(SYMBOL_TRADE_STOPS_LEVEL,STOPLEVEL)) { return int(STOPLEVEL); } } Print("Unable to retrieve Symbol's StopLevel"); return 0; }
以下函数将检索交易品种的冻结水平。这是价格需要从开盘价开始变动的最小差距,以便允许交易关闭(即允许以盈利或亏损的方式关闭特定交易)。
int CSymbolProperties::FreezeLevel(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.InfoInteger(SYMBOL_TRADE_FREEZE_LEVEL,FREEZELEVEL)) { return int(FREEZELEVEL); } } Print("Unable to retrieve Symbol's FreezeLevel"); return 0; }
以下函数将检索交易品种的时间。
datetime CSymbolProperties::Time(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.InfoInteger(SYMBOL_TIME,TIME)) { return datetime(TIME); } } Print("Unable to retrieve Symbol's Time"); TIME=0; return datetime(TIME); }
以下函数将尝试对特定交易品种的价格进行标准化处理。
例如,如果EURUSD的买入价是1.07735,而您尝试以1.077351的价格水平开设买入交易。您可能会收到一个错误提示,比如“无效价格”,因为价格精度的小数位数超过了允许值,例如只允许5位小数。该函数会将具有6位小数的价格转换为5位小数,从而对价格进行标准化处理。
double CSymbolProperties::NormalizePrice(const double price,string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.Refresh()&&CSymbol.RefreshRates()) { return CSymbol.NormalizePrice(price); } } Print("Unable to Normalize Symbol's Price"); return price; }
以下函数将检索交易品种的报价精度。这里的精度指的是交易品种报价的小数位数。
int CSymbolProperties::Digits(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.InfoInteger(SYMBOL_DIGITS,DIGITS)) { return int(DIGITS); } } Print("Unable to retrieve Symbol's Digits"); return 0; }
以下函数将检索交易品种的最小变动点值。
double CSymbolProperties::Point(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.InfoDouble(SYMBOL_POINT,POINT)) { return POINT; } } Print("Unable to retrieve Symbol's Point"); return 0.0; }
以下函数将检索交易品种的交易模式。
ENUM_SYMBOL_TRADE_MODE CSymbolProperties::TradeMode(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.Refresh()) { return CSymbol.TradeMode(); } } Print("Unable to retrieve Symbol's TradeMode"); return SYMBOL_TRADE_MODE_DISABLED; }
以下函数将检索该交易品种的订单量/手数的总和。
double CSymbolProperties::OrdersVolume(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { for(int i=0; i<OrdersTotal(); i++) { if(OrderSelect(OrderGetTicket(i))) { if(OrderGetString(ORDER_SYMBOL)==GetSymbolName()) { ORDERSVOLUME+=OrderGetDouble(ORDER_VOLUME_CURRENT); } } } } else { Print("Unable to retrieve Symbol's OrdersVolume"); return 0.0; } return ORDERSVOLUME; }
以下函数将检索交易品种的累计头寸量/手数之和。
double CSymbolProperties::PositionsVolume(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { for(int i=0; i<PositionsTotal(); i++) { if(PositionGetTicket(i)>0) { if(PositionGetString(POSITION_SYMBOL)==GetSymbolName()) { POSITIONSVOLUME+=PositionGetDouble(POSITION_VOLUME); } } } } else { Print("Unable to retrieve Symbol's PositionsVolume"); return 0.0; } return POSITIONSVOLUME; }
以下函数将检索交易品种的货币基准。
string CSymbolProperties::CurrencyBase(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.Refresh()) { return CSymbol.CurrencyBase(); } } Print("Unable to retrieve Symbol's CurrencyBase"); return ""; }
以下函数将检索交易品种的盈利。
string CSymbolProperties::CurrencyProfit(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.Refresh()) { return CSymbol.CurrencyProfit(); } } Print("Unable to retrieve Symbol's CurrencyProfit"); return ""; }
以下函数将检索交易品种的保证金。
string CSymbolProperties::CurrencyMargin(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.Refresh()) { return CSymbol.CurrencyMargin(); } } Print("Unable to retrieve Symbol's CurrencyMargin"); return ""; }
以下函数将检索一个布尔值,用于标识是否为自定义的交易品种。
bool CSymbolProperties::Custom(string SYMBOL=NULL) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.InfoInteger(SYMBOL_CUSTOM,CUSTOM)) { return bool(CUSTOM); } } Print("Unable to retrieve if Symbol is Custom"); return false; }
以下函数将检索交易品种的背景颜色。它有一个可选参数allow_black,该参数默认为false。这是因为我们稍后将使用交易品种的背景颜色来设置图表的背景颜色,而我们不希望获取黑色,因为图表的其他元素也是黑色的。如果我们允许黑色,基于我们预期的图表格式,这将导致容易分散注意力且难以阅读。
以下示例是一个使用我们稍后将要建立的新图表格式,其背景为黑色。
color CSymbolProperties::SymbolBackground(string SYMBOL=NULL,bool allow_black=false) { if(SetSymbolName(SYMBOL))//Set Symbol { if(CSymbol.InfoInteger(SYMBOL_BACKGROUND_COLOR,BACKGROUND_CLR)) { /*Avoid any Symbol black background color */ BACKGROUND_CLR = ((ColorToString(color(BACKGROUND_CLR))=="0,0,0"|| color(BACKGROUND_CLR)==clrBlack)&&!allow_black)? long(StringToColor("236,236,236")):BACKGROUND_CLR; return color(BACKGROUND_CLR); } } Print("Unable to retrieve Symbol's Background color"); return color(StringToColor("236,236,236"));//Retrieve a lightish gray color }
时间管理类
在该类种,我将重点介绍为这个类的功能新增的函数。这个类的目的是对时间数据进行操作或交互。
//+------------------------------------------------------------------+ //| NewsTrading | //| Copyright 2024, MetaQuotes Ltd. | //| https://www.mql5.com/en/users/kaaiblo | //+------------------------------------------------------------------+ //+------------------------------------------------------------------+ //|TimeManagement class | //+------------------------------------------------------------------+ class CTimeManagement { private: MqlDateTime today;//private variable MqlDateTime timeFormat;//private variable public: //-- Checks if a date is within two other dates bool DateIsInRange(datetime FirstTime,datetime SecondTime,datetime compareTime); //-- Check if two dates(Start&End) are within CompareStart & CompareEnd bool DateIsInRange(datetime Start,datetime End,datetime CompareStart,datetime CompareEnd); bool DateisToday(datetime TimeRepresented);//Checks if a date is within the current day int SecondsS(int multiple=1);//Returns seconds int MinutesS(int multiple=1);//Returns Minutes in seconds int HoursS(int multiple=1);//Returns Hours in seconds int DaysS(int multiple=1);//Returns Days in seconds int WeeksS(int multiple=1);//Returns Weeks in seconds int MonthsS(int multiple=1);//Returns Months in seconds int YearsS(int multiple=1);//Returns Years in seconds int ReturnYear(datetime time);//Returns the Year for a specific date int ReturnMonth(datetime time);//Returns the Month for a specific date int ReturnDay(datetime time);//Returns the Day for a specific date //-- Will return a datetime type of a date with an subtraction offset in seconds datetime TimeMinusOffset(datetime standardtime,int timeoffset); //-- Will return a datetime type of a date with an addition offset in seconds datetime TimePlusOffset(datetime standardtime,int timeoffset); }; //+------------------------------------------------------------------+ //|Checks if a date is within two other dates | //+------------------------------------------------------------------+ bool CTimeManagement::DateIsInRange(datetime FirstTime,datetime SecondTime,datetime compareTime) { return(FirstTime<=compareTime&&SecondTime>compareTime); } //+------------------------------------------------------------------+ //|Check if two dates(Start&End) are within CompareStart & CompareEnd| //+------------------------------------------------------------------+ bool CTimeManagement::DateIsInRange(datetime Start,datetime End,datetime CompareStart,datetime CompareEnd) { return(Start<=CompareStart&&CompareEnd<End); } //+------------------------------------------------------------------+ //|Checks if a date is within the current day | //+------------------------------------------------------------------+ bool CTimeManagement::DateisToday(datetime TimeRepresented) { MqlDateTime TiM; TimeToStruct(TimeRepresented,TiM); TimeCurrent(today); return(TiM.year==today.year&&TiM.mon==today.mon&&TiM.day==today.day); } //+------------------------------------------------------------------+ //|Returns seconds | //+------------------------------------------------------------------+ int CTimeManagement::SecondsS(int multiple=1) { return (1*multiple); } //+------------------------------------------------------------------+ //|Returns Minutes in seconds | //+------------------------------------------------------------------+ int CTimeManagement::MinutesS(int multiple=1) { return (SecondsS(60)*multiple); } //+------------------------------------------------------------------+ //|Returns Hours in seconds | //+------------------------------------------------------------------+ int CTimeManagement::HoursS(int multiple=1) { return (MinutesS(60)*multiple); } //+------------------------------------------------------------------+ //|Returns Days in seconds | //+------------------------------------------------------------------+ int CTimeManagement::DaysS(int multiple=1) { return (HoursS(24)*multiple); } //+------------------------------------------------------------------+ //|Returns Weeks in seconds | //+------------------------------------------------------------------+ int CTimeManagement::WeeksS(int multiple=1) { return (DaysS(7)*multiple); } //+------------------------------------------------------------------+ //|Returns Months in seconds | //+------------------------------------------------------------------+ int CTimeManagement::MonthsS(int multiple=1) { return (WeeksS(4)*multiple); } //+------------------------------------------------------------------+ //|Returns Years in seconds | //+------------------------------------------------------------------+ int CTimeManagement::YearsS(int multiple=1) { return (MonthsS(12)*multiple); } //+------------------------------------------------------------------+ //|Returns the Year for a specific date | //+------------------------------------------------------------------+ int CTimeManagement::ReturnYear(datetime time) { TimeToStruct(time,timeFormat); return timeFormat.year; } //+------------------------------------------------------------------+ //|Returns the Month for a specific date | //+------------------------------------------------------------------+ int CTimeManagement::ReturnMonth(datetime time) { TimeToStruct(time,timeFormat); return timeFormat.mon; } //+------------------------------------------------------------------+ //|Returns the Day for a specific date | //+------------------------------------------------------------------+ int CTimeManagement::ReturnDay(datetime time) { TimeToStruct(time,timeFormat); return timeFormat.day; } //+------------------------------------------------------------------+ //|Will return a datetime type of a date with an subtraction offset | //|in seconds | //+------------------------------------------------------------------+ datetime CTimeManagement::TimeMinusOffset(datetime standardtime,int timeoffset) { standardtime-=timeoffset; return standardtime; } //+------------------------------------------------------------------+ //|Will return a datetime type of a date with an addition offset | //|in seconds | //+------------------------------------------------------------------+ datetime CTimeManagement::TimePlusOffset(datetime standardtime,int timeoffset) { standardtime+=timeoffset; return standardtime; } //+------------------------------------------------------------------+
图表属性类
图表属性类的目的是在更改图表布局之前存储图表的配置。一旦EA从图表中移除,该类的析构函数将恢复图表配置的状态,即恢复到我们对图表做出更改之前的状态。
更改图表并不是EA必需的功能,但拥有一个不仅仅是绿色和黑色(默认图表布局)的图表不仅在视觉上会更加吸引人,并且在交易发生后,也更容易看清图表价格和交易水平。
CChartProperties类单一继承自 CSymbolProperties类。
CChartProperties类具有层次化的继承关系,其继承自以下类:
- CSymbolProperties
- CSymbolInfo
//+------------------------------------------------------------------+ //| NewsTrading | //| Copyright 2024, MetaQuotes Ltd. | //| https://www.mql5.com/en/users/kaaiblo | //+------------------------------------------------------------------+ #include "SymbolProperties.mqh" //+------------------------------------------------------------------+ //|ChartProperties class | //+------------------------------------------------------------------+ class CChartProperties : public CSymbolProperties { private: struct ChartFormat { ulong CHART_MODE;//Chart Candle Mode ulong CHART_COLOR_BACKGROUND;//Chart Background Color ulong CHART_COLOR_FOREGROUND;//Chart Foreground Color ulong CHART_COLOR_CHART_LINE;//Chart Line Color ulong CHART_COLOR_CANDLE_BEAR;//Chart Bear Candle Color ulong CHART_COLOR_CHART_DOWN;//Chart Down Candle Color ulong CHART_COLOR_CANDLE_BULL;//Chart Bull Candle Color ulong CHART_COLOR_CHART_UP;//Chart Up Candle Color ulong CHART_COLOR_ASK;//Chart Ask Color ulong CHART_COLOR_BID;//Chart Bid Color ulong CHART_COLOR_STOP_LEVEL;//Chart Stoplevel Color ulong CHART_SHOW_PERIOD_SEP;//Chart Show Period Separator ulong CHART_SCALE;//Chart Scale ulong CHART_FOREGROUND;//Chart Show Foreground ulong CHART_SHOW_ASK_LINE;//Chart Show Ask Line ulong CHART_SHOW_BID_LINE;//Chart Show Bid Line ulong CHART_SHOW_TRADE_LEVELS;//Chart Show Trade Levels ulong CHART_SHOW_OHLC;//Chart Show Open-High-Low-Close ulong CHART_SHOW_GRID;//Chart Show Grid ulong CHART_SHOW_VOLUMES;//Chart Show Volumes ulong CHART_AUTOSCROLL;//Chart Auto Scroll double CHART_SHIFT_SIZE;//Chart Shift Size ulong CHART_SHIFT;//Chart Shift ulong CHART_SHOW_ONE_CLICK;//Chart One Click Trading }; ulong ChartConfig[65];//Array To Store Chart Properties void ChartSet();//Apply Chart format void ChartConfigure();//Set Chart Values ChartFormat Chart;//Variable of type ChartFormat public: CChartProperties(void);//Constructor ~CChartProperties(void);//Destructor void ChartRefresh() {ChartConfigure();} }; //+------------------------------------------------------------------+ //|Constructor | //+------------------------------------------------------------------+ CChartProperties::CChartProperties(void)//Class Constructor { for(int i=0;i<65;i++)//Iterating through ENUM_CHART_PROPERTY_INTEGER Elements { ChartGetInteger(0,(ENUM_CHART_PROPERTY_INTEGER)i,0,ChartConfig[i]);//Storing Chart values into ChartConfig array } ChartConfigure(); } //+------------------------------------------------------------------+ //|Destructor | //+------------------------------------------------------------------+ CChartProperties::~CChartProperties(void) { for(int i=0;i<65;i++)//Iterating through ENUM_CHART_PROPERTY_INTEGER Elements { ChartSetInteger(0,(ENUM_CHART_PROPERTY_INTEGER)i,0,ChartConfig[i]);//Restoring Chart values from ChartConfig array } } //+------------------------------------------------------------------+ //|Set Chart Properties | //+------------------------------------------------------------------+ void CChartProperties::ChartSet() { ChartSetInteger(0,CHART_MODE,Chart.CHART_MODE);//Set Chart Candle Mode ChartSetInteger(0,CHART_COLOR_BACKGROUND,Chart.CHART_COLOR_BACKGROUND);//Set Chart Background Color ChartSetInteger(0,CHART_COLOR_FOREGROUND,Chart.CHART_COLOR_FOREGROUND);//Set Chart Foreground Color ChartSetInteger(0,CHART_COLOR_CHART_LINE,Chart.CHART_COLOR_CHART_LINE);//Set Chart Line Color ChartSetInteger(0,CHART_COLOR_CANDLE_BEAR,Chart.CHART_COLOR_CANDLE_BEAR);//Set Chart Bear Candle Color ChartSetInteger(0,CHART_COLOR_CHART_DOWN,Chart.CHART_COLOR_CHART_DOWN);//Set Chart Down Candle Color ChartSetInteger(0,CHART_COLOR_CANDLE_BULL,Chart.CHART_COLOR_CANDLE_BULL);//Set Chart Bull Candle Color ChartSetInteger(0,CHART_COLOR_CHART_UP,Chart.CHART_COLOR_CHART_UP);//Set Chart Up Candle Color ChartSetInteger(0,CHART_COLOR_ASK,Chart.CHART_COLOR_ASK);//Set Chart Ask Color ChartSetInteger(0,CHART_COLOR_BID,Chart.CHART_COLOR_BID);//Set Chart Bid Color ChartSetInteger(0,CHART_COLOR_STOP_LEVEL,Chart.CHART_COLOR_STOP_LEVEL);//Set Chart Stop Level Color ChartSetInteger(0,CHART_FOREGROUND,Chart.CHART_FOREGROUND);//Set if Chart is in Foreground Visibility ChartSetInteger(0,CHART_SHOW_ASK_LINE,Chart.CHART_SHOW_ASK_LINE);//Set Chart Ask Line Visibility ChartSetInteger(0,CHART_SHOW_BID_LINE,Chart.CHART_SHOW_BID_LINE);//Set Chart Bid Line Visibility ChartSetInteger(0,CHART_SHOW_PERIOD_SEP,Chart.CHART_SHOW_PERIOD_SEP);//Set Chart Period Separator Visibility ChartSetInteger(0,CHART_SHOW_TRADE_LEVELS,Chart.CHART_SHOW_TRADE_LEVELS);//Set Chart Trade Levels Visibility ChartSetInteger(0,CHART_SHOW_OHLC,Chart.CHART_SHOW_OHLC);//Set Chart Open-High-Low-Close Visibility ChartSetInteger(0,CHART_SHOW_GRID,Chart.CHART_SHOW_GRID);//Set Chart Grid Visibility ChartSetInteger(0,CHART_SHOW_VOLUMES,Chart.CHART_SHOW_VOLUMES);//Set Chart Volumes Visibility ChartSetInteger(0,CHART_SCALE,Chart.CHART_SCALE);//Set Chart Scale Value ChartSetInteger(0,CHART_AUTOSCROLL,Chart.CHART_AUTOSCROLL);//Set Chart Auto Scroll Option ChartSetDouble(0,CHART_SHIFT_SIZE,Chart.CHART_SHIFT_SIZE);//Set Chart Shift Size Value ChartSetInteger(0,CHART_SHIFT,Chart.CHART_SHIFT);//Set Chart Shift Option ChartSetInteger(0,CHART_SHOW_ONE_CLICK,Chart.CHART_SHOW_ONE_CLICK);//Set Chart One Click Trading } //+------------------------------------------------------------------+ //|Initialize Chart Properties | //+------------------------------------------------------------------+ void CChartProperties::ChartConfigure(void) { Chart.CHART_MODE=(ulong)CHART_CANDLES;//Assigning Chart Mode of CHART_CANDLES Chart.CHART_COLOR_BACKGROUND=ulong(SymbolBackground());//Assigning Chart Background Color of Symbol's Background color Chart.CHART_COLOR_FOREGROUND=(ulong)clrBlack;//Assigning Chart Foreground Color of clrBalck(Black color) Chart.CHART_COLOR_CHART_LINE=(ulong)clrBlack;//Assigning Chart Line Color of clrBlack(Black color) Chart.CHART_COLOR_CANDLE_BEAR=(ulong)clrBlack;//Assigning Chart Bear Candle Color of clrBlack(Black color) Chart.CHART_COLOR_CHART_DOWN=(ulong)clrBlack;//Assigning Chart Down Candle Color of clrBlack(Black color) Chart.CHART_COLOR_CANDLE_BULL=(ulong)clrWhite;//Assigning Chart Bull Candle Color of clrWhite(White color) Chart.CHART_COLOR_CHART_UP=(ulong)clrBlack;//Assigning Chart Up Candle Color of clrBlack(Black color) Chart.CHART_COLOR_ASK=(ulong)clrBlack;//Assigning Chart Ask Color of clrBlack(Black color) Chart.CHART_COLOR_BID=(ulong)clrBlack;//Assigning Chart Bid Color of clrBlack(Black color) Chart.CHART_COLOR_STOP_LEVEL=(ulong)clrBlack;//Assigning Chart Stop Level Color of clrBlack(Black color) Chart.CHART_FOREGROUND=(ulong)false;//Assigning Chart Foreground Boolean Value of 'false' Chart.CHART_SHOW_ASK_LINE=(ulong)true;//Assigning Chart Ask Line Boolean Value of 'true' Chart.CHART_SHOW_BID_LINE=(ulong)true;//Assigning Chart Bid Line Boolean Value of 'true' Chart.CHART_SHOW_PERIOD_SEP=(ulong)true;//Assigning Chart Period Separator Boolean Value of 'true' Chart.CHART_SHOW_TRADE_LEVELS=(ulong)true;//Assigning Chart Trade Levels Boolean Value of 'true' Chart.CHART_SHOW_OHLC=(ulong)false;//Assigning Chart Open-High-Low-Close Boolean Value of 'false' Chart.CHART_SHOW_GRID=(ulong)false;//Assigning Chart Grid Boolean Value of 'false' Chart.CHART_SHOW_VOLUMES=(ulong)false;//Assigning Chart Volumes Boolean Value of 'false' Chart.CHART_SCALE=(ulong)3;//Assigning Chart Scale Boolean Value of '3' Chart.CHART_AUTOSCROLL=(ulong)true;//Assigning Chart Auto Scroll Boolean Value of 'true' Chart.CHART_SHIFT_SIZE=30;//Assigning Chart Shift Size Value of '30' Chart.CHART_SHIFT=(ulong)true;//Assigning Chart Shift Boolean Value of 'true' Chart.CHART_SHOW_ONE_CLICK=ulong(false);//Assigning Chart One Click Trading a value of 'false' ChartSet();//Calling Function to set chart format } //+------------------------------------------------------------------+
在我们声明的ChartFormat结构中,我们存储了将在当前EA所在的图表上进行更改的不同图表变量。
struct ChartFormat { ulong CHART_MODE;//Chart Candle Mode ulong CHART_COLOR_BACKGROUND;//Chart Background Color ulong CHART_COLOR_FOREGROUND;//Chart Foreground Color ulong CHART_COLOR_CHART_LINE;//Chart Line Color ulong CHART_COLOR_CANDLE_BEAR;//Chart Bear Candle Color ulong CHART_COLOR_CHART_DOWN;//Chart Down Candle Color ulong CHART_COLOR_CANDLE_BULL;//Chart Bull Candle Color ulong CHART_COLOR_CHART_UP;//Chart Up Candle Color ulong CHART_COLOR_ASK;//Chart Ask Color ulong CHART_COLOR_BID;//Chart Bid Color ulong CHART_COLOR_STOP_LEVEL;//Chart Stoplevel Color ulong CHART_SHOW_PERIOD_SEP;//Chart Show Period Separator ulong CHART_SCALE;//Chart Scale ulong CHART_FOREGROUND;//Chart Show Foreground ulong CHART_SHOW_ASK_LINE;//Chart Show Ask Line ulong CHART_SHOW_BID_LINE;//Chart Show Bid Line ulong CHART_SHOW_TRADE_LEVELS;//Chart Show Trade Levels ulong CHART_SHOW_OHLC;//Chart Show Open-High-Low-Close ulong CHART_SHOW_GRID;//Chart Show Grid ulong CHART_SHOW_VOLUMES;//Chart Show Volumes ulong CHART_AUTOSCROLL;//Chart Auto Scroll double CHART_SHIFT_SIZE;//Chart Shift Size ulong CHART_SHIFT;//Chart Shift ulong CHART_SHOW_ONE_CLICK;//Chart One Click Trading };
在更改图表之前,ChartConfig数组将存储所有图表属性。
ulong ChartConfig[65];//Array To Store Chart Properties
在Function SetBackground中,我们得到当前交易品种的MarketWatch背景颜色:
并将当前图表的背景颜色设置为该颜色:
在类的构造函数中,我们获取整型类型的所有图表属性,并将其存储到ChartConfig数组中。
CChartProperties::CChartProperties(void)//Class Constructor { for(int i=0;i<65;i++)//Iterating through ENUM_CHART_PROPERTY_INTEGER Elements { ChartGetInteger(0,(ENUM_CHART_PROPERTY_INTEGER)i,0,ChartConfig[i]);//Storing Chart values into ChartConfig array } ChartConfigure(); }
我们还在函数ChartConfigure中初始化了一个名为Chart的变量,该变量是之前提到的ChartFormat结构体类型,并为其赋予适当的值,以便按照我们的喜好自定义图表。
void CChartProperties::ChartConfigure(void) { Chart.CHART_MODE=(ulong)CHART_CANDLES;//Assigning Chart Mode of CHART_CANDLES Chart.CHART_COLOR_BACKGROUND=ulong(SymbolBackground());//Assigning Chart Background Color of Symbol's Background color Chart.CHART_COLOR_FOREGROUND=(ulong)clrBlack;//Assigning Chart Foreground Color of clrBalck(Black color) Chart.CHART_COLOR_CHART_LINE=(ulong)clrBlack;//Assigning Chart Line Color of clrBlack(Black color) Chart.CHART_COLOR_CANDLE_BEAR=(ulong)clrBlack;//Assigning Chart Bear Candle Color of clrBlack(Black color) Chart.CHART_COLOR_CHART_DOWN=(ulong)clrBlack;//Assigning Chart Down Candle Color of clrBlack(Black color) Chart.CHART_COLOR_CANDLE_BULL=(ulong)clrWhite;//Assigning Chart Bull Candle Color of clrWhite(White color) Chart.CHART_COLOR_CHART_UP=(ulong)clrBlack;//Assigning Chart Up Candle Color of clrBlack(Black color) Chart.CHART_COLOR_ASK=(ulong)clrBlack;//Assigning Chart Ask Color of clrBlack(Black color) Chart.CHART_COLOR_BID=(ulong)clrBlack;//Assigning Chart Bid Color of clrBlack(Black color) Chart.CHART_COLOR_STOP_LEVEL=(ulong)clrBlack;//Assigning Chart Stop Level Color of clrBlack(Black color) Chart.CHART_FOREGROUND=(ulong)false;//Assigning Chart Foreground Boolean Value of 'false' Chart.CHART_SHOW_ASK_LINE=(ulong)true;//Assigning Chart Ask Line Boolean Value of 'true' Chart.CHART_SHOW_BID_LINE=(ulong)true;//Assigning Chart Bid Line Boolean Value of 'true' Chart.CHART_SHOW_PERIOD_SEP=(ulong)true;//Assigning Chart Period Separator Boolean Value of 'true' Chart.CHART_SHOW_TRADE_LEVELS=(ulong)true;//Assigning Chart Trade Levels Boolean Value of 'true' Chart.CHART_SHOW_OHLC=(ulong)false;//Assigning Chart Open-High-Low-Close Boolean Value of 'false' Chart.CHART_SHOW_GRID=(ulong)false;//Assigning Chart Grid Boolean Value of 'false' Chart.CHART_SHOW_VOLUMES=(ulong)false;//Assigning Chart Volumes Boolean Value of 'false' Chart.CHART_SCALE=(ulong)3;//Assigning Chart Scale Boolean Value of '3' Chart.CHART_AUTOSCROLL=(ulong)true;//Assigning Chart Auto Scroll Boolean Value of 'true' Chart.CHART_SHIFT_SIZE=30;//Assigning Chart Shift Size Value of '30' Chart.CHART_SHIFT=(ulong)true;//Assigning Chart Shift Boolean Value of 'true' Chart.CHART_SHOW_ONE_CLICK=ulong(false);//Assigning Chart One Click Trading a value of 'false' ChartSet();//Calling Function to set chart format }
在ChartSet函数中,将会从结构类型为ChartFormat的变量Chart中设置所选图表属性的值。
void CChartProperties::ChartSet() { ChartSetInteger(0,CHART_MODE,Chart.CHART_MODE);//Set Chart Candle Mode ChartSetInteger(0,CHART_COLOR_BACKGROUND,Chart.CHART_COLOR_BACKGROUND);//Set Chart Background Color ChartSetInteger(0,CHART_COLOR_FOREGROUND,Chart.CHART_COLOR_FOREGROUND);//Set Chart Foreground Color ChartSetInteger(0,CHART_COLOR_CHART_LINE,Chart.CHART_COLOR_CHART_LINE);//Set Chart Line Color ChartSetInteger(0,CHART_COLOR_CANDLE_BEAR,Chart.CHART_COLOR_CANDLE_BEAR);//Set Chart Bear Candle Color ChartSetInteger(0,CHART_COLOR_CHART_DOWN,Chart.CHART_COLOR_CHART_DOWN);//Set Chart Down Candle Color ChartSetInteger(0,CHART_COLOR_CANDLE_BULL,Chart.CHART_COLOR_CANDLE_BULL);//Set Chart Bull Candle Color ChartSetInteger(0,CHART_COLOR_CHART_UP,Chart.CHART_COLOR_CHART_UP);//Set Chart Up Candle Color ChartSetInteger(0,CHART_COLOR_ASK,Chart.CHART_COLOR_ASK);//Set Chart Ask Color ChartSetInteger(0,CHART_COLOR_BID,Chart.CHART_COLOR_BID);//Set Chart Bid Color ChartSetInteger(0,CHART_COLOR_STOP_LEVEL,Chart.CHART_COLOR_STOP_LEVEL);//Set Chart Stop Level Color ChartSetInteger(0,CHART_FOREGROUND,Chart.CHART_FOREGROUND);//Set if Chart is in Foreground Visibility ChartSetInteger(0,CHART_SHOW_ASK_LINE,Chart.CHART_SHOW_ASK_LINE);//Set Chart Ask Line Visibility ChartSetInteger(0,CHART_SHOW_BID_LINE,Chart.CHART_SHOW_BID_LINE);//Set Chart Bid Line Visibility ChartSetInteger(0,CHART_SHOW_PERIOD_SEP,Chart.CHART_SHOW_PERIOD_SEP);//Set Chart Period Separator Visibility ChartSetInteger(0,CHART_SHOW_TRADE_LEVELS,Chart.CHART_SHOW_TRADE_LEVELS);//Set Chart Trade Levels Visibility ChartSetInteger(0,CHART_SHOW_OHLC,Chart.CHART_SHOW_OHLC);//Set Chart Open-High-Low-Close Visibility ChartSetInteger(0,CHART_SHOW_GRID,Chart.CHART_SHOW_GRID);//Set Chart Grid Visibility ChartSetInteger(0,CHART_SHOW_VOLUMES,Chart.CHART_SHOW_VOLUMES);//Set Chart Volumes Visibility ChartSetInteger(0,CHART_SCALE,Chart.CHART_SCALE);//Set Chart Scale Value ChartSetInteger(0,CHART_AUTOSCROLL,Chart.CHART_AUTOSCROLL);//Set Chart Auto Scroll Option ChartSetDouble(0,CHART_SHIFT_SIZE,Chart.CHART_SHIFT_SIZE);//Set Chart Shift Size Value ChartSetInteger(0,CHART_SHIFT,Chart.CHART_SHIFT);//Set Chart Shift Option ChartSetInteger(0,CHART_SHOW_ONE_CLICK,Chart.CHART_SHOW_ONE_CLICK);//Set Chart One Click Trading }
在析构函数中,我们将恢复上一个图表的整数值。
CChartProperties::~CChartProperties(void) { for(int i=0;i<65;i++)//Iterating through ENUM_CHART_PROPERTY_INTEGER Elements { ChartSetInteger(0,(ENUM_CHART_PROPERTY_INTEGER)i,0,ChartConfig[i]);//Restoring Chart values from ChartConfig array } }
K线属性类
CCandleProperties类是从多个类中多层级继承而来:
- CChartProperties
- CSymbolProperties
CCandleProperties 类包含了来自CTimeManagement类的属性。
CCandleProperties类是从多个类中分层级继承而来:
- CSymbolProperties
- CSymbolInfo
//+------------------------------------------------------------------+ //| NewsTrading | //| Copyright 2024, MetaQuotes Ltd. | //| https://www.mql5.com/en/users/kaaiblo | //+------------------------------------------------------------------+ #include "TimeManagement.mqh" #include "ChartProperties.mqh" //+------------------------------------------------------------------+ //|CandleProperties class | //+------------------------------------------------------------------+ class CCandleProperties : public CChartProperties { private: CTimeManagement Time; public: double Open(int CandleIndex,ENUM_TIMEFRAMES Period=PERIOD_CURRENT,string SYMBOL=NULL);//Retrieve Candle Open-Price double Close(int CandleIndex,ENUM_TIMEFRAMES Period=PERIOD_CURRENT,string SYMBOL=NULL);//Retrieve Candle Close-Price double High(int CandleIndex,ENUM_TIMEFRAMES Period=PERIOD_CURRENT,string SYMBOL=NULL);//Retrieve Candle High-Price double Low(int CandleIndex,ENUM_TIMEFRAMES Period=PERIOD_CURRENT,string SYMBOL=NULL);//Retrieve Candle Low-Price bool IsLargerThanPreviousAndNext(datetime CandleTime,int Offset,string SYMBOL);//Determine if one candle is larger than two others }; //+------------------------------------------------------------------+ //|Retrieve Candle Open-Price | //+------------------------------------------------------------------+ double CCandleProperties::Open(int CandleIndex,ENUM_TIMEFRAMES Period=PERIOD_CURRENT,string SYMBOL=NULL) { return (SetSymbolName(SYMBOL))?iOpen(GetSymbolName(),Period,CandleIndex):0;//return candle open price } //+------------------------------------------------------------------+ //|Retrieve Candle Close-Price | //+------------------------------------------------------------------+ double CCandleProperties::Close(int CandleIndex,ENUM_TIMEFRAMES Period=PERIOD_CURRENT,string SYMBOL=NULL) { return (SetSymbolName(SYMBOL))?iClose(GetSymbolName(),Period,CandleIndex):0;//return candle close price } //+------------------------------------------------------------------+ //|Retrieve Candle High-Price | //+------------------------------------------------------------------+ double CCandleProperties::High(int CandleIndex,ENUM_TIMEFRAMES Period=PERIOD_CURRENT,string SYMBOL=NULL) { return (SetSymbolName(SYMBOL))?iHigh(GetSymbolName(),Period,CandleIndex):0;//return candle high price } //+------------------------------------------------------------------+ //|Retrieve Candle Low-Price | //+------------------------------------------------------------------+ double CCandleProperties::Low(int CandleIndex,ENUM_TIMEFRAMES Period=PERIOD_CURRENT,string SYMBOL=NULL) { return (SetSymbolName(SYMBOL))?iLow(GetSymbolName(),Period,CandleIndex):0;//return candle low price } //+------------------------------------------------------------------+ //|Determine if one candle is larger than two others | //+------------------------------------------------------------------+ bool CCandleProperties::IsLargerThanPreviousAndNext(datetime CandleTime,int Offset,string SYMBOL) { int CandleIndex = iBarShift(SYMBOL,PERIOD_M15,CandleTime);//Assign candle index of candletime //--Assign candle index of candletime minus time offset int CandleIndexMinusOffset = iBarShift(SYMBOL,PERIOD_M15,Time.TimeMinusOffset(CandleTime,Offset)); //--Assign candle index of candletime plus time offset int CandleIndexPlusOffset = iBarShift(SYMBOL,PERIOD_M15,Time.TimePlusOffset(CandleTime,Offset)); //--Assign height of M15 candletime in pips double CandleHeight = High(CandleIndex,PERIOD_M15,SYMBOL)-Low(CandleIndex,PERIOD_M15,SYMBOL); //--Assign height of M15 candletime minus offset in Pips double CandleHeightMinusOffset = High(CandleIndexMinusOffset,PERIOD_M15,SYMBOL)-Low(CandleIndexMinusOffset,PERIOD_M15,SYMBOL); //--Assign height of M15 candletime plus offset in Pips double CandleHeightPlusOffset = High(CandleIndexPlusOffset,PERIOD_M15,SYMBOL)-Low(CandleIndexPlusOffset,PERIOD_M15,SYMBOL); //--Determine if candletime height is greater than candletime height minus offset and candletime height plus offset if(CandleHeight>CandleHeightMinusOffset&&CandleHeight>CandleHeightPlusOffset) { return true;//Candletime is likely when the news event occured } return false;//Candletime is unlikely when the real news data was released } //+------------------------------------------------------------------+
对象属性类
该类将负责创建和删除图表对象。
CObjectProperties 类是从多个类中多层级继承而来:
- CChartProperties
- CSymbolProperties
- CSymbolProperties
- CSymbolInfo
//+------------------------------------------------------------------+ //| NewsTrading | //| Copyright 2024, MetaQuotes Ltd. | //| https://www.mql5.com/en/users/kaaiblo | //+------------------------------------------------------------------+ #include "ChartProperties.mqh" //+------------------------------------------------------------------+ //|ObjectProperties class | //+------------------------------------------------------------------+ class CObjectProperties:public CChartProperties { private: //Simple chart objects structure struct ObjStruct { long ChartId; string Name; } Objects[];//ObjStruct variable array //-- Add chart object to Objects array void AddObj(long chart_id,string name) { ArrayResize(Objects,Objects.Size()+1,Objects.Size()+2); Objects[Objects.Size()-1].ChartId=chart_id; Objects[Objects.Size()-1].Name=name; } public: CObjectProperties(void) {}//Class constructor //-- Create Rectangle chart object void Square(long chart_ID,string name,int x_coord,int y_coord,int width,int height,ENUM_ANCHOR_POINT Anchor); //-- Create text chart object void TextObj(long chartID,string name,string text,int x_coord,int y_coord, ENUM_BASE_CORNER Corner=CORNER_LEFT_UPPER,int fontsize=10); //-- Create Event object void EventObj(long chartID,string name,string description,datetime eventdate); //-- Class destructor removes all chart objects created previously ~CObjectProperties(void) { for(uint i=0;i<Objects.Size();i++) { ObjectDelete(Objects[i].ChartId,Objects[i].Name); } } }; //+------------------------------------------------------------------+ //|Create Rectangle chart object | //+------------------------------------------------------------------+ void CObjectProperties::Square(long chart_ID,string name,int x_coord,int y_coord,int width,int height,ENUM_ANCHOR_POINT Anchor) { const int sub_window=0; // subwindow index const int x=x_coord; // X coordinate const int y=y_coord; // Y coordinate const color back_clr=clrBlack; // background color const ENUM_BORDER_TYPE border=BORDER_SUNKEN; // border type const color clr=clrRed; // flat border color (Flat) const ENUM_LINE_STYLE style=STYLE_SOLID; // flat border style const int line_width=0; // flat border width const bool back=false; // in the background const bool selection=false; // highlight to move const bool hidden=true; // hidden in the object list ObjectDelete(chart_ID,name);//Delete previous object with the same name and chart id if(ObjectCreate(chart_ID,name,OBJ_RECTANGLE_LABEL,sub_window,0,0))//create rectangle object label { AddObj(chart_ID,name);//Add object to array ObjectSetInteger(chart_ID,name,OBJPROP_XDISTANCE,x);//Set x Distance/coordinate ObjectSetInteger(chart_ID,name,OBJPROP_YDISTANCE,y);//Set y Distance/coordinate ObjectSetInteger(chart_ID,name,OBJPROP_XSIZE,width);//Set object's width/x-size ObjectSetInteger(chart_ID,name,OBJPROP_YSIZE,height);//Set object's height/y-size ObjectSetInteger(chart_ID,name,OBJPROP_BGCOLOR,back_clr);//Set object's background color ObjectSetInteger(chart_ID,name,OBJPROP_BORDER_TYPE,border);//Set object's border type ObjectSetInteger(chart_ID,name,OBJPROP_ANCHOR,Anchor);//Set objects anchor point ObjectSetInteger(chart_ID,name,OBJPROP_COLOR,clr);//Set object's color ObjectSetInteger(chart_ID,name,OBJPROP_STYLE,style);//Set object's style ObjectSetInteger(chart_ID,name,OBJPROP_WIDTH,line_width);//Set object's flat border width ObjectSetInteger(chart_ID,name,OBJPROP_BACK,back);//Set if object is in foreground or not ObjectSetInteger(chart_ID,name,OBJPROP_SELECTABLE,selection);//Set if object is selectable/dragable ObjectSetInteger(chart_ID,name,OBJPROP_SELECTED,selection);//Set if object is Selected ObjectSetInteger(chart_ID,name,OBJPROP_HIDDEN,hidden);//Set if object is hidden in object list ChartRedraw(chart_ID); } else { Print("Failed to create object: ",name); } } //+------------------------------------------------------------------+ //|Create text chart object | //+------------------------------------------------------------------+ void CObjectProperties::TextObj(long chartID,string name,string text,int x_coord,int y_coord, ENUM_BASE_CORNER Corner=CORNER_LEFT_UPPER,int fontsize=10) { ObjectDelete(chartID,name);//Delete previous object with the same name and chart id if(ObjectCreate(chartID,name,OBJ_LABEL,0,0,0))//Create object label { AddObj(chartID,name);//Add object to array ObjectSetInteger(chartID,name,OBJPROP_XDISTANCE,x_coord);//Set x Distance/coordinate ObjectSetInteger(chartID,name,OBJPROP_YDISTANCE,y_coord);//Set y Distance/coordinate ObjectSetInteger(chartID,name,OBJPROP_CORNER,Corner);//Set object's corner anchor ObjectSetString(chartID,name,OBJPROP_TEXT,text);//Set object's text ObjectSetInteger(chartID,name,OBJPROP_COLOR,SymbolBackground());//Set object's color ObjectSetInteger(chartID,name,OBJPROP_FONTSIZE,fontsize);//Set object's font-size } else { Print("Failed to create object: ",name); } } //+------------------------------------------------------------------+ //|Create Event object | //+------------------------------------------------------------------+ void CObjectProperties::EventObj(long chartID,string name,string description,datetime eventdate) { ObjectDelete(chartID,name);//Delete previous object with the same name and chart id if(ObjectCreate(chartID,name,OBJ_EVENT,0,eventdate,0))//Create object event { AddObj(chartID,name);//Add object to array ObjectSetString(chartID,name,OBJPROP_TEXT,description);//Set object's text ObjectSetInteger(chartID,name,OBJPROP_COLOR,clrBlack);//Set object's color } else { Print("Failed to create object: ",name); } } //+------------------------------------------------------------------
数组变量Objects将存储在CObjectProperties类中创建的所有图表对象。
struct ObjStruct { long ChartId; string Name; } Objects[];//ObjStruct variable array
函数AddObj将图表对象的图表id和对象名称添加到Objects数组中。
//-- Add chart object to Objects array void AddObj(long chart_id,string name) { ArrayResize(Objects,Objects.Size()+1,Objects.Size()+2); Objects[Objects.Size()-1].ChartId=chart_id; Objects[Objects.Size()-1].Name=name; }
函数Square的目的是创建一个具有特定属性的Rectangle对象,该对象允许自定义。
void CObjectProperties::Square(long chart_ID,string name,int x_coord,int y_coord,int width,int height,ENUM_ANCHOR_POINT Anchor) { const int sub_window=0; // subwindow index const int x=x_coord; // X coordinate const int y=y_coord; // Y coordinate const color back_clr=clrBlack; // background color const ENUM_BORDER_TYPE border=BORDER_SUNKEN; // border type const color clr=clrRed; // flat border color (Flat) const ENUM_LINE_STYLE style=STYLE_SOLID; // flat border style const int line_width=0; // flat border width const bool back=false; // in the background const bool selection=false; // highlight to move const bool hidden=true; // hidden in the object list ObjectDelete(chart_ID,name);//Delete previous object with the same name and chart id if(ObjectCreate(chart_ID,name,OBJ_RECTANGLE_LABEL,sub_window,0,0))//create rectangle object label { AddObj(chart_ID,name);//Add object to array ObjectSetInteger(chart_ID,name,OBJPROP_XDISTANCE,x);//Set x Distance/coordinate ObjectSetInteger(chart_ID,name,OBJPROP_YDISTANCE,y);//Set y Distance/coordinate ObjectSetInteger(chart_ID,name,OBJPROP_XSIZE,width);//Set object's width/x-size ObjectSetInteger(chart_ID,name,OBJPROP_YSIZE,height);//Set object's height/y-size ObjectSetInteger(chart_ID,name,OBJPROP_BGCOLOR,back_clr);//Set object's background color ObjectSetInteger(chart_ID,name,OBJPROP_BORDER_TYPE,border);//Set object's border type ObjectSetInteger(chart_ID,name,OBJPROP_ANCHOR,Anchor);//Set objects anchor point ObjectSetInteger(chart_ID,name,OBJPROP_COLOR,clr);//Set object's color ObjectSetInteger(chart_ID,name,OBJPROP_STYLE,style);//Set object's style ObjectSetInteger(chart_ID,name,OBJPROP_WIDTH,line_width);//Set object's flat border width ObjectSetInteger(chart_ID,name,OBJPROP_BACK,back);//Set if object is in foreground or not ObjectSetInteger(chart_ID,name,OBJPROP_SELECTABLE,selection);//Set if object is selectable/dragable ObjectSetInteger(chart_ID,name,OBJPROP_SELECTED,selection);//Set if object is Selected ObjectSetInteger(chart_ID,name,OBJPROP_HIDDEN,hidden);//Set if object is hidden in object list ChartRedraw(chart_ID); } else { Print("Failed to create object: ",name); } }
函数TextObj将在图表上创建文本对象。
void CObjectProperties::TextObj(long chartID,string name,string text,int x_coord,int y_coord, ENUM_BASE_CORNER Corner=CORNER_LEFT_UPPER,int fontsize=10) { ObjectDelete(chartID,name);//Delete previous object with the same name and chart id if(ObjectCreate(chartID,name,OBJ_LABEL,0,0,0))//Create object label { AddObj(chartID,name);//Add object to array ObjectSetInteger(chartID,name,OBJPROP_XDISTANCE,x_coord);//Set x Distance/coordinate ObjectSetInteger(chartID,name,OBJPROP_YDISTANCE,y_coord);//Set y Distance/coordinate ObjectSetInteger(chartID,name,OBJPROP_CORNER,Corner);//Set object's corner anchor ObjectSetString(chartID,name,OBJPROP_TEXT,text);//Set object's text ObjectSetInteger(chartID,name,OBJPROP_COLOR,SymbolBackground());//Set object's color ObjectSetInteger(chartID,name,OBJPROP_FONTSIZE,fontsize);//Set object's font-size } else { Print("Failed to create object: ",name); } }
函数EventObj将在图表上创建事件对象,以显示已经发生或将要发生的经济事件。
void CObjectProperties::EventObj(long chartID,string name,string description,datetime eventdate) { ObjectDelete(chartID,name);//Delete previous object with the same name and chart id if(ObjectCreate(chartID,name,OBJ_EVENT,0,eventdate,0))//Create object event { AddObj(chartID,name);//Add object to array ObjectSetString(chartID,name,OBJPROP_TEXT,description);//Set object's text ObjectSetInteger(chartID,name,OBJPROP_COLOR,clrBlack);//Set object's color } else { Print("Failed to create object: ",name); } }
新闻类
第一部分中的日历表:
在我们之前的数据库(第一部分)中,文件体积非常庞大,将所有新闻数据存入数据库的速度非常慢。这是因为新闻数据的存储效率低下。导致文件体积庞大和性能低下的最主要原因是,大量重复存储了相同的数据。
表格
- Data_AU
- Data_None
- Data_UK
- Data_US
存储相同的新闻数据,但时间数据有所不同。
新设计:
第二部分日历内容:
因此,在新的数据库设计中,我们将包含以下内容:
- AutoDST表
- Calendar_AU视图
- Calendar_NONE视图
- Calendar_UK视图
- Calendar_US视图
- MQL5日历表
- 记录表
- TimeSchedule表
- Only One_AutoDST触发器
- OnlyOne_Record触发器
我们将规范化之前的数据库表,这些表分别是Data_AU、Data_None、Data_UK和Data_US。
数据库规范化是数据库设计中的一个过程,用于将数据库组织成表和列,以最大限度地减少冗余和依赖。其主要目标是消除冗余数据(例如,在多个表中存储相同的数据)并确保数据依赖关系合理(只在一个表中存储相关数据)。这个过程会产生一系列更容易维护的表,并减少数据异常的可能性。
我们还将创建触发器,以确保在AutoDST表和Record表中仅存储一条记录。此外,我们还将为每个夏令时计划创建视图,以显示最后更新日的新闻事件,在理想情况下,这样可以方便地查看哪些新闻事件是最新的,而无需在包含数千条记录的表上反复运行查询。
那么触发器又是什么?
SQLite中的触发器是一种特殊的存储过程,它会在特定表上发生某些事件时自动执行一组指定的操作。这些事件可以是向表中插入、更新或删除行。
什么是视图?
SQLite中的视图是一个基于SELECT查询结果集的虚拟表。与表不同,视图不物理地存储数据。相反,它提供了一种方式,以特定的结构或格式展示一个或多个表中的数据,这通常可以简化复杂的查询并增强数据安全。
在我们开始创建新表并添加至已经是大容量的数据库中。我们需要一种方法来知道哪些表可以删除,哪些需要保留。一个简单的解决方案是检查我们之前数据库中已存在的每张表,这包括Data_AU和其他表。但我们不能仅仅根据记忆中的信息硬编码要删除的表,我们的程序需要自行找到不再需要的表。为了实现这一点,我们需要检查数据库中存在的表,并遍历我们要删除的表,同时跳过我们要保留的表。
在SQLite中,有一个名为SQLITE_MASTER/SQLITE_SCHEMA的表,它存储数据库的元数据,包括数据库中所有对象的信息以及用于定义这些对象的SQL。它是SQLite中最重要的系统目录。以下查询用于获取数据库中的所有信息。
SELECT * FROM SQLITE_MASTER;
数据库输出
type name tbl_name rootpage sql table Data_None Data_None 2 CREATE TABLE Data_None(ID INT NOT NULL,EVENTID INT NOT NULL,COUNTRY STRING NOT NULL,EVENTNAME STRING NOT NULL,EVENTTYPE STRING NOT NULL,EVENTIMPORTANCE STRING NOT NULL,EVENTDATE STRING NOT NULL,EVENTCURRENCY STRING NOT NULL,EVENTCODE STRING NOT NULL,EVENTSECTOR STRING NOT NULL,EVENTFORECAST STRING NOT NULL,EVENTPREVALUE STRING NOT NULL,EVENTIMPACT STRING NOT NULL,EVENTFREQUENCY STRING NOT NULL,PRIMARY KEY(ID)) index sqlite_autoindex_Data_None_1 Data_None 3 table Data_US Data_US 4 CREATE TABLE Data_US(ID INT NOT NULL,EVENTID INT NOT NULL,COUNTRY STRING NOT NULL,EVENTNAME STRING NOT NULL,EVENTTYPE STRING NOT NULL,EVENTIMPORTANCE STRING NOT NULL,EVENTDATE STRING NOT NULL,EVENTCURRENCY STRING NOT NULL,EVENTCODE STRING NOT NULL,EVENTSECTOR STRING NOT NULL,EVENTFORECAST STRING NOT NULL,EVENTPREVALUE STRING NOT NULL,EVENTIMPACT STRING NOT NULL,EVENTFREQUENCY STRING NOT NULL,PRIMARY KEY(ID)) index sqlite_autoindex_Data_US_1 Data_US 5 table Data_UK Data_UK 6 CREATE TABLE Data_UK(ID INT NOT NULL,EVENTID INT NOT NULL,COUNTRY STRING NOT NULL,EVENTNAME STRING NOT NULL,EVENTTYPE STRING NOT NULL,EVENTIMPORTANCE STRING NOT NULL,EVENTDATE STRING NOT NULL,EVENTCURRENCY STRING NOT NULL,EVENTCODE STRING NOT NULL,EVENTSECTOR STRING NOT NULL,EVENTFORECAST STRING NOT NULL,EVENTPREVALUE STRING NOT NULL,EVENTIMPACT STRING NOT NULL,EVENTFREQUENCY STRING NOT NULL,PRIMARY KEY(ID)) index sqlite_autoindex_Data_UK_1 Data_UK 7 table Data_AU Data_AU 8 CREATE TABLE Data_AU(ID INT NOT NULL,EVENTID INT NOT NULL,COUNTRY STRING NOT NULL,EVENTNAME STRING NOT NULL,EVENTTYPE STRING NOT NULL,EVENTIMPORTANCE STRING NOT NULL,EVENTDATE STRING NOT NULL,EVENTCURRENCY STRING NOT NULL,EVENTCODE STRING NOT NULL,EVENTSECTOR STRING NOT NULL,EVENTFORECAST STRING NOT NULL,EVENTPREVALUE STRING NOT NULL,EVENTIMPACT STRING NOT NULL,EVENTFREQUENCY STRING NOT NULL,PRIMARY KEY(ID)) index sqlite_autoindex_Data_AU_1 Data_AU 9 table Records Records 38774 CREATE TABLE Records(RECORDEDTIME INT NOT NULL) table AutoDST AutoDST 38775 CREATE TABLE AutoDST(DST STRING NOT NULL)
从数据库输出中可以看出,我们之前没有创建过索引。
什么是索引
在SQLite中,索引是一种数据库对象,它通过从表中更快地检索记录来提高查询操作的性能。索引通过创建一个排序的数据结构(通常是B树)来特别帮助加快搜索、排序和连接操作的速度,这种数据结构允许数据库引擎更快地定位到行。
如果我们之前没有创建过索引,那么为什么创建索引?
在SQLite中,当一张表有主键时,会为该表自动创建一个索引。
在我们之前的数据库输出中,我们已经获得了数据库中的所有对象和元数据,现在我们可以找到那些不再需要的表并将它们删除。为了实现这一点,我们将创建一个包含所需表及其SQL语句的数组,以便我们可以将它们与数据库输出中的表进行比较,从而删除那些不匹配的表。
CNews是从多个类中多层级继承而来:
- CCandleProperties
- CChartProperties
- CSymbolProperties
CNews包含来自其它类的内容:
- CDaylightSavings_UK
- CDaylightSavings_US
- CDaylightSavings_AU
CNews包含来自头文件CommonVariables.mqh的内容
CNews是从多个类中分层级继承而来:
- CSymbolProperties
- CSymbolInfo
- CCandleProperties
- CTimeManagement
//+------------------------------------------------------------------+ //| NewsTrading | //| Copyright 2024, MetaQuotes Ltd. | //| https://www.mql5.com/en/users/kaaiblo | //+------------------------------------------------------------------+ #include "CommonVariables.mqh" #include "DayLightSavings/DaylightSavings_UK.mqh" #include "DayLightSavings/DaylightSavings_US.mqh" #include "DayLightSavings/DaylightSavings_AU.mqh" #include "CandleProperties.mqh" //+------------------------------------------------------------------+ //|News class | //+------------------------------------------------------------------+ class CNews : private CCandleProperties { //Private Declarations Only accessable by this class/header file private: //-- To keep track of what is in our database enum CalendarComponents { AutoDST_Table,//AutoDST Table CalendarAU_View,//View for DST_AU CalendarNONE_View,//View for DST_NONE CalendarUK_View,//View for DST_UK CalendarUS_View,//View for DST_US Record_Table,// Record Table TimeSchedule_Table,//TimeSchedule Table MQL5Calendar_Table,//MQL5Calendar Table AutoDST_Trigger,//Table Trigger for AutoDST Record_Trigger//Table Trigger for Record }; //-- structure to retrieve all the objects in the database struct SQLiteMaster { string type;//will store object's type string name;//will store object's name string tbl_name;//will store table name int rootpage;//will store rootpage string sql;//Will store the sql create statement } DBContents[];//Array of type SQLiteMaster //-- MQL5CalendarContents inherits from SQLiteMaster structure struct MQL5CalendarContents:SQLiteMaster { CalendarComponents Content; string insert;//Will store the sql insert statement } CalendarContents[10];//Array to Store objects in our database CTimeManagement Time;//TimeManagement Object declaration CDaylightSavings_UK Savings_UK;//DaylightSavings Object for the UK and EU CDaylightSavings_US Savings_US;//DaylightSavings Object for the US CDaylightSavings_AU Savings_AU;//DaylightSavings Object for the AU bool AutoDetectDST(DST_type &dstType);//Function will determine Broker DST DST_type DSTType;//variable of DST_type enumeration declared in the CommonVariables class/header file bool InsertIntoTables(int db,Calendar &Evalues[]);//Function for inserting Economic Data in to a database's table void CreateAutoDST(int db);//Function for creating and inserting Recommend DST for the Broker into a table bool CreateCalendarTable(int db,bool &tableExists);//Function for creating a table in a database bool CreateTimeTable(int db,bool &tableExists);//Function for creating a table in a database void CreateCalendarViews(int db);//Function for creating a view in a database void CreateRecordTable(int db);//Creates a table to store the record of when last the Calendar database was updated/created bool UpdateRecords();//Checks if the main Calendar database needs an update or not void EconomicDetails(Calendar &NewsTime[]);//Gets values from the MQL5 economic Calendar string DropRequest;//Variable for dropping tables in the database //-- Function for retrieving the MQL5CalendarContents structure for the enumartion type CalendarComponents MQL5CalendarContents CalendarStruct(CalendarComponents Content) { MQL5CalendarContents Calendar; for(uint i=0;i<CalendarContents.Size();i++) { if(CalendarContents[i].Content==Content) { return CalendarContents[i]; } } return Calendar; } //Public declarations accessable via a class's Object public: CNews(void); ~CNews(void);//Deletes a text file created when the Calendar database is being worked on void CreateEconomicDatabase();//Creates the Calendar database for a specific Broker datetime GetLatestNewsDate();//Gets the lastest/newest date in the Calendar database }; //+------------------------------------------------------------------+ //|Constructor | //+------------------------------------------------------------------+ CNews::CNews(void):DropRequest("PRAGMA foreign_keys = OFF; " "PRAGMA secure_delete = ON; " "Drop %s IF EXISTS %s; " "Vacuum; " "PRAGMA foreign_keys = ON;")//Sql drop statement { //-- initializing properties for the AutoDST table CalendarContents[0].Content = AutoDST_Table; CalendarContents[0].name = "AutoDST"; CalendarContents[0].sql = "CREATE TABLE AutoDST(DST TEXT NOT NULL DEFAULT 'DST_NONE')STRICT;"; CalendarContents[0].tbl_name = "AutoDST"; CalendarContents[0].type = "table"; CalendarContents[0].insert = "INSERT INTO 'AutoDST'(DST) VALUES ('%s');"; string views[] = {"UK","US","AU","NONE"}; string view_sql = "CREATE VIEW IF NOT EXISTS Calendar_%s " "AS " "SELECT C.Eventid,C.Eventname,C.Country,T.DST_%s as Time,C.EventCurrency,C.Eventcode from MQL5Calendar C,Record R " "Inner join TimeSchedule T on C.ID=T.ID " "Where DATE(REPLACE(T.DST_%s,'.','-'))=R.Date " "Order by T.DST_%s Asc;"; //-- Sql statements for creating the table views for(uint i=1;i<=views.Size();i++) { CalendarContents[i].Content = (CalendarComponents)i; CalendarContents[i].name = StringFormat("Calendar_%s",views[i-1]); CalendarContents[i].sql = StringFormat(view_sql,views[i-1],views[i-1],views[i-1],views[i-1]); CalendarContents[i].tbl_name = StringFormat("Calendar_%s",views[i-1]); CalendarContents[i].type = "view"; } //-- initializing properties for the Record table CalendarContents[5].Content = Record_Table; CalendarContents[5].name = "Record"; CalendarContents[5].sql = "CREATE TABLE Record(Date TEXT NOT NULL)STRICT;"; CalendarContents[5].tbl_name="Record"; CalendarContents[5].type = "table"; CalendarContents[5].insert = "INSERT INTO 'Record'(Date) VALUES (Date(REPLACE('%s','.','-')));"; //-- initializing properties for the TimeSchedule table CalendarContents[6].Content = TimeSchedule_Table; CalendarContents[6].name = "TimeSchedule"; CalendarContents[6].sql = "CREATE TABLE TimeSchedule(ID INT NOT NULL,DST_UK TEXT NOT NULL,DST_US TEXT NOT NULL," "DST_AU TEXT NOT NULL,DST_NONE TEXT NOT NULL,FOREIGN KEY (ID) REFERENCES MQL5Calendar (ID))STRICT;"; CalendarContents[6].tbl_name="TimeSchedule"; CalendarContents[6].type = "table"; CalendarContents[6].insert = "INSERT INTO 'TimeSchedule'(ID,DST_UK,DST_US,DST_AU,DST_NONE) " "VALUES (%d,'%s','%s', '%s', '%s');"; //-- initializing properties for the MQL5Calendar table CalendarContents[7].Content = MQL5Calendar_Table; CalendarContents[7].name = "MQL5Calendar"; CalendarContents[7].sql = "CREATE TABLE MQL5Calendar(ID INT NOT NULL,EVENTID INT NOT NULL,COUNTRY TEXT NOT NULL," "EVENTNAME TEXT NOT NULL,EVENTTYPE TEXT NOT NULL,EVENTIMPORTANCE TEXT NOT NULL," "EVENTCURRENCY TEXT NOT NULL,EVENTCODE TEXT NOT NULL,EVENTSECTOR TEXT NOT NULL," "EVENTFORECAST TEXT NOT NULL,EVENTPREVALUE TEXT NOT NULL,EVENTIMPACT TEXT NOT NULL," "EVENTFREQUENCY TEXT NOT NULL,PRIMARY KEY(ID))STRICT;"; CalendarContents[7].tbl_name="MQL5Calendar"; CalendarContents[7].type = "table"; CalendarContents[7].insert = "INSERT INTO 'MQL5Calendar'(ID,EVENTID,COUNTRY,EVENTNAME,EVENTTYPE,EVENTIMPORTANCE,EVENTCURRENCY,EVENTCODE," "EVENTSECTOR,EVENTFORECAST,EVENTPREVALUE,EVENTIMPACT,EVENTFREQUENCY) " "VALUES (%d,%d,'%s','%s','%s','%s','%s','%s','%s','%s','%s','%s','%s');"; //-- Sql statement for creating the AutoDST table's trigger CalendarContents[8].Content = AutoDST_Trigger; CalendarContents[8].name = "OnlyOne_AutoDST"; CalendarContents[8].sql = "CREATE TRIGGER IF NOT EXISTS OnlyOne_AutoDST " "BEFORE INSERT ON AutoDST " "BEGIN " "Delete from AutoDST; " "END;"; CalendarContents[8].tbl_name="AutoDST"; CalendarContents[8].type = "trigger"; //-- Sql statement for creating the Record table's trigger CalendarContents[9].Content = Record_Trigger; CalendarContents[9].name = "OnlyOne_Record"; CalendarContents[9].sql = "CREATE TRIGGER IF NOT EXISTS OnlyOne_Record " "BEFORE INSERT ON Record " "BEGIN " "Delete from Record; " "END;"; CalendarContents[9].tbl_name="Record"; CalendarContents[9].type = "trigger"; } //+------------------------------------------------------------------+ //|Destructor | //+------------------------------------------------------------------+ CNews::~CNews(void) { if(FileIsExist(NEWS_TEXT_FILE,FILE_COMMON))//Check if the news database open text file exists { FileDelete(NEWS_TEXT_FILE,FILE_COMMON); } } //+------------------------------------------------------------------+ //|Gets values from the MQL5 economic Calendar | //+------------------------------------------------------------------+ void CNews::EconomicDetails(Calendar &NewsTime[]) { int Size=0;//to keep track of the size of the events in the NewsTime array MqlCalendarCountry countries[]; string Country_code=""; for(int i=0,count=CalendarCountries(countries); i<count; i++) { MqlCalendarValue values[]; datetime date_from=0;//Get date from the beginning datetime date_to=(datetime)(Time.MonthsS()+iTime(Symbol(),PERIOD_D1,0));//Date of the next month from the current day if(CalendarValueHistory(values,date_from,date_to,countries[i].code)) { for(int x=0; x<(int)ArraySize(values); x++) { MqlCalendarEvent event; ulong event_id=values[x].event_id;//Get the event id if(CalendarEventById(event_id,event)) { ArrayResize(NewsTime,Size+1,Size+2);//Readjust the size of the array to +1 of the array size StringReplace(event.name,"'","");//Removing or replacing single quotes(') from event name with an empty string NewsTime[Size].CountryName = countries[i].name;//storing the country's name from the specific event NewsTime[Size].EventName = event.name;//storing the event's name NewsTime[Size].EventType = EnumToString(event.type);//storing the event type from (ENUM_CALENDAR_EVENT_TYPE) to a string //-- storing the event importance from (ENUM_CALENDAR_EVENT_IMPORTANCE) to a string NewsTime[Size].EventImportance = EnumToString(event.importance); NewsTime[Size].EventId = event.id;//storing the event id NewsTime[Size].EventDate = TimeToString(values[x].time);//storing normal event time NewsTime[Size].EventCurrency = countries[i].currency;//storing event currency NewsTime[Size].EventCode = countries[i].code;//storing event code NewsTime[Size].EventSector = EnumToString(event.sector);//storing event sector from (ENUM_CALENDAR_EVENT_SECTOR) to a string if(values[x].HasForecastValue())//Checks if the event has a forecast value { NewsTime[Size].EventForecast = (string)values[x].forecast_value;//storing the forecast value into a string } else { NewsTime[Size].EventForecast = "None";//storing 'None' as the forecast value } if(values[x].HasPreviousValue())//Checks if the event has a previous value { NewsTime[Size].EventPreval = (string)values[x].prev_value;//storing the previous value into a string } else { NewsTime[Size].EventPreval = "None";//storing 'None' as the previous value } //-- storing the event impact from (ENUM_CALENDAR_EVENT_IMPACT) to a string NewsTime[Size].EventImpact = EnumToString(values[x].impact_type); //-- storing the event frequency from (ENUM_CALENDAR_EVENT_FREQUENCY) to a string NewsTime[Size].EventFrequency = EnumToString(event.frequency); Size++;//incrementing the Calendar array NewsTime } } } } } //+------------------------------------------------------------------+ //|Checks if the main Calendar database needs an update or not | //+------------------------------------------------------------------+ bool CNews::UpdateRecords() { //initialize variable to true bool perform_update=true; //--- open/create //-- try to open database Calendar int db=DatabaseOpen(NEWS_DATABASE_FILE, DATABASE_OPEN_READWRITE | DATABASE_OPEN_CREATE| DATABASE_OPEN_COMMON); if(db==INVALID_HANDLE)//Checks if the database was able to be opened { //if opening the database failed if(!FileIsExist(NEWS_DATABASE_FILE,FILE_COMMON))//Checks if the database Calendar exists in the common folder { return perform_update;//Returns true when the database was failed to be opened and the file doesn't exist in the common folder } } int MasterRequest = DatabasePrepare(db,"select * from sqlite_master where type<>'index';"); if(MasterRequest==INVALID_HANDLE) { Print("DB: ",NEWS_DATABASE_FILE, " request failed with code ", GetLastError()); } else { SQLiteMaster ReadContents; //Assigning values from the sql query into DBContents array for(int i=0; DatabaseReadBind(MasterRequest,ReadContents); i++) { ArrayResize(DBContents,i+1,i+2); DBContents[i].type = ReadContents.type; DBContents[i].name = ReadContents.name; DBContents[i].tbl_name = ReadContents.tbl_name; DBContents[i].rootpage = ReadContents.rootpage; /*Check if the end of the sql string has a character ';' if not add this character to the string*/ DBContents[i].sql = (StringFind(ReadContents.sql,";",StringLen(ReadContents.sql)-1)== (StringLen(ReadContents.sql)-1))?ReadContents.sql:ReadContents.sql+";";; } uint contents_exists = 0; for(uint i=0;i<DBContents.Size();i++) { bool isCalendarContents = false; for(uint x=0;x<CalendarContents.Size();x++) { /*Store Sql query from CalendarContents without string ' IF NOT EXISTS'*/ string CalendarSql=CalendarContents[x].sql; StringReplace(CalendarSql," IF NOT EXISTS",""); //-- Check if the Db object is in our list if(DBContents[i].name==CalendarContents[x].name&& (DBContents[i].sql==CalendarSql|| DBContents[i].sql==CalendarContents[x].sql)&& CalendarContents[x].type==DBContents[i].type&& CalendarContents[x].tbl_name==DBContents[i].tbl_name) { contents_exists++; isCalendarContents = true; } } if(!isCalendarContents) { //-- Print DBcontent's name if it does not match with CalendarContents PrintFormat("DBContent: %s is not needed!",DBContents[i].name); //-- We will drop the table if it is not neccessary DatabaseExecute(db,StringFormat(DropRequest,DBContents[i].type,DBContents[i].name)); Print("Attempting To Clean Database..."); } } /*If not all the CalendarContents exist in the Calendar Database before an update */ if(contents_exists!=CalendarContents.Size()) { return perform_update; } } if(!DatabaseTableExists(db,CalendarStruct(Record_Table).name))//If the database table 'Record' doesn't exist { DatabaseClose(db); return perform_update; } //-- Sql query to determine the lastest or maximum date recorded /* If the last recorded date data in the 'Record' table is not equal to the current day, perform an update! */ string request_text=StringFormat("SELECT Date FROM %s where Date=Date(REPLACE('%s','.','-'))", CalendarStruct(Record_Table).name,TimeToString(TimeTradeServer())); int request=DatabasePrepare(db,request_text);//Creates a handle of a request, which can then be executed using DatabaseRead() if(request==INVALID_HANDLE)//Checks if the request failed to be completed { Print("DB: ",NEWS_DATABASE_FILE, " request failed with code ", GetLastError()); DatabaseClose(db); return perform_update; } if(DatabaseRead(request))//Will be true if there are results from the sql query/request { DatabaseFinalize(request);//Removes a request created in DatabasePrepare() DatabaseClose(db);//Closes the database perform_update=false; return perform_update; } else { DatabaseFinalize(request);//Removes a request created in DatabasePrepare() DatabaseClose(db);//Closes the database return perform_update; } } //+------------------------------------------------------------------+ //|Creates the Calendar database for a specific Broker | //+------------------------------------------------------------------+ void CNews::CreateEconomicDatabase() { if(FileIsExist(NEWS_DATABASE_FILE,FILE_COMMON))//Check if the database exists { if(!UpdateRecords())//Check if the database is up to date { return;//will terminate execution of the rest of the code below } } if(FileIsExist(NEWS_TEXT_FILE,FILE_COMMON))//Check if the database is open { return;//will terminate execution of the rest of the code below } Calendar Evalues[];//Creating a Calendar array variable bool failed=false,tableExists=false; int file=INVALID_HANDLE; //--- open/create the database 'Calendar' //-- will try to open/create in the common folder int db=DatabaseOpen(NEWS_DATABASE_FILE, DATABASE_OPEN_READWRITE | DATABASE_OPEN_CREATE| DATABASE_OPEN_COMMON); if(db==INVALID_HANDLE)//Checks if the database 'Calendar' failed to open/create { Print("DB: ",NEWS_DATABASE_FILE, " open failed with code ", GetLastError()); return;//will terminate execution of the rest of the code below } else { //-- try to create a text file 'NewsDatabaseOpen' in common folder file=FileOpen(NEWS_TEXT_FILE,FILE_WRITE|FILE_ANSI|FILE_TXT|FILE_COMMON); if(file==INVALID_HANDLE) { DatabaseClose(db);//Closes the database 'Calendar' if the News text file failed to be created return;//will terminate execution of the rest of the code below } } DatabaseTransactionBegin(db);//Starts transaction execution Print("Please wait..."); //-- attempt to create the MQL5Calendar and TimeSchedule tables if(!CreateCalendarTable(db,tableExists)||!CreateTimeTable(db,tableExists)) { FileClose(file);//Closing the file 'NewsDatabaseOpen.txt' FileDelete(NEWS_TEXT_FILE,FILE_COMMON);//Deleting the file 'NewsDatabaseOpen.txt' return;//will terminate execution of the rest of the code below } EconomicDetails(Evalues);//Retrieving the data from the Economic Calendar if(tableExists)//Checks if there is an existing table within the Calendar Database { //if there is an existing table we will notify the user that we are updating the table. PrintFormat("Updating %s",NEWS_DATABASE_FILE); } else { //if there isn't an existing table we will notify the user that we about to create one PrintFormat("Creating %s",NEWS_DATABASE_FILE); } //-- attempt to insert economic event data into the calendar tables if(!InsertIntoTables(db,Evalues)) { //-- Will assign true if inserting economic vaules failed in the MQL5Calendar and TimeSchedule tables failed=true; } if(failed) { //--- roll back all transactions and unlock the database DatabaseTransactionRollback(db); PrintFormat("%s: DatabaseExecute() failed with code %d", __FUNCTION__, GetLastError()); FileClose(file);//Close the text file 'NEWS_TEXT_FILE' FileDelete(NEWS_TEXT_FILE,FILE_COMMON);//Delete the text file, as we are reverted/rolled-back the database ArrayRemove(Evalues,0,WHOLE_ARRAY);//Removes the values in the array } else { CreateCalendarViews(db); CreateRecordTable(db);//Will create the 'Record' table and insert the current time CreateAutoDST(db);//Will create the 'AutoDST' table and insert the broker's DST schedule FileClose(file);//Close the text file 'NEWS_TEXT_FILE' FileDelete(NEWS_TEXT_FILE,FILE_COMMON);//Delete the text file, as we are about to close the database ArrayRemove(Evalues,0,WHOLE_ARRAY);//Removes the values in the array if(tableExists) { //Let the user/trader know that the database was updated PrintFormat("%s Updated",NEWS_DATABASE_FILE); } else { //Let the user/trader know that the database was created PrintFormat("%s Created",NEWS_DATABASE_FILE); } } //--- all transactions have been performed successfully - record changes and unlock the database DatabaseTransactionCommit(db); DatabaseClose(db);//Close the database } //+------------------------------------------------------------------+ //|Function for creating a table in a database | //+------------------------------------------------------------------+ bool CNews::CreateCalendarTable(int db,bool &tableExists) { //-- Checks if a table 'MQL5Calendar' exists if(DatabaseTableExists(db,CalendarStruct(MQL5Calendar_Table).name)) { tableExists=true;//Assigns true to tableExists variable //-- Checks if a table 'TimeSchedule' exists in the database 'Calendar' if(DatabaseTableExists(db,CalendarStruct(TimeSchedule_Table).name)) { //-- We will drop the table if the table already exists if(!DatabaseExecute(db,StringFormat("Drop Table %s",CalendarStruct(TimeSchedule_Table).name))) { //If the table failed to be dropped/deleted PrintFormat("Failed to drop table %s with code %d",CalendarStruct(TimeSchedule_Table).name,GetLastError()); DatabaseClose(db);//Close the database return false;//will terminate execution of the rest of the code below and return false, when the table cannot be dropped } } //--We will drop the table if the table already exists if(!DatabaseExecute(db,StringFormat("Drop Table %s",CalendarStruct(MQL5Calendar_Table).name))) { //If the table failed to be dropped/deleted PrintFormat("Failed to drop table %s with code %d",CalendarStruct(MQL5Calendar_Table).name,GetLastError()); DatabaseClose(db);//Close the database return false;//will terminate execution of the rest of the code below and return false, when the table cannot be dropped } } //-- If the database table 'MQL5Calendar' doesn't exist if(!DatabaseTableExists(db,CalendarStruct(MQL5Calendar_Table).name)) { //--- create the table 'MQL5Calendar' if(!DatabaseExecute(db,CalendarStruct(MQL5Calendar_Table).sql))//Checks if the table was successfully created { Print("DB: create the Calendar table failed with code ", GetLastError()); DatabaseClose(db);//Close the database return false;//Function returns false if creating the table failed } } return true;//Function returns true if creating the table was successful } //+------------------------------------------------------------------+ //|Function for creating a table in a database | //+------------------------------------------------------------------+ bool CNews::CreateTimeTable(int db,bool &tableExists) { //-- If the database table 'TimeSchedule' doesn't exist if(!DatabaseTableExists(db,CalendarStruct(TimeSchedule_Table).name)) { //--- create the table 'TimeSchedule' if(!DatabaseExecute(db,CalendarStruct(TimeSchedule_Table).sql))//Checks if the table was successfully created { Print("DB: create the Calendar table failed with code ", GetLastError()); DatabaseClose(db);//Close the database return false;//Function returns false if creating the table failed } } return true;//Function returns true if creating the table was successful } //+------------------------------------------------------------------+ //|Function for creating views in a database | //+------------------------------------------------------------------+ void CNews::CreateCalendarViews(int db) { for(uint i=1;i<=4;i++) { if(!DatabaseExecute(db,CalendarStruct((CalendarComponents)i).sql))//Checks if the view was successfully created { Print("DB: create the Calendar view failed with code ", GetLastError()); } } } //+------------------------------------------------------------------+ //|Function for inserting Economic Data in to a database's table | //+------------------------------------------------------------------+ bool CNews::InsertIntoTables(int db,Calendar &Evalues[]) { for(uint i=0; i<Evalues.Size(); i++)//Looping through all the Economic Events { string request_insert_into_calendar = StringFormat(CalendarStruct(MQL5Calendar_Table).insert, i, Evalues[i].EventId, Evalues[i].CountryName, Evalues[i].EventName, Evalues[i].EventType, Evalues[i].EventImportance, Evalues[i].EventCurrency, Evalues[i].EventCode, Evalues[i].EventSector, Evalues[i].EventForecast, Evalues[i].EventPreval, Evalues[i].EventImpact, Evalues[i].EventFrequency);//Inserting all the columns for each event record if(DatabaseExecute(db,request_insert_into_calendar))//Check if insert query into calendar was successful { string request_insert_into_time = StringFormat(CalendarStruct(TimeSchedule_Table).insert, i, //-- Economic EventDate adjusted for UK DST(Daylight Savings Time) Savings_UK.adjustDaylightSavings(StringToTime(Evalues[i].EventDate)), //-- Economic EventDate adjusted for US DST(Daylight Savings Time) Savings_US.adjustDaylightSavings(StringToTime(Evalues[i].EventDate)), //-- Economic EventDate adjusted for AU DST(Daylight Savings Time) Savings_AU.adjustDaylightSavings(StringToTime(Evalues[i].EventDate)), Evalues[i].EventDate//normal Economic EventDate );//Inserting all the columns for each event record if(!DatabaseExecute(db,request_insert_into_time)) { Print(GetLastError()); //-- Will print the sql query to check for any errors or possible defaults in the query/request Print(request_insert_into_time); return false;//Will end the loop and return false, as values failed to be inserted into the table } } else { Print(GetLastError()); //-- Will print the sql query to check for any errors or possible defaults in the query/request Print(request_insert_into_calendar); return false;//Will end the loop and return false, as values failed to be inserted into the table } } return true;//Will return true, all values were inserted into the table successfully } //+------------------------------------------------------------------+ //|Creates a table to store the record of when last the Calendar | //|database was updated/created | //+------------------------------------------------------------------+ void CNews::CreateRecordTable(int db) { bool failed=false; if(!DatabaseTableExists(db,CalendarStruct(Record_Table).name))//Checks if the table 'Record' exists in the databse 'Calendar' { //--- create the table if(!DatabaseExecute(db,CalendarStruct(Record_Table).sql))//Will attempt to create the table 'Record' { Print("DB: create the Records table failed with code ", GetLastError()); DatabaseClose(db);//Close the database return;//Exits the function if creating the table failed } else//If Table was created Successfully then Create Trigger { DatabaseExecute(db,CalendarStruct(Record_Trigger).sql); } } else { DatabaseExecute(db,CalendarStruct(Record_Trigger).sql); } //Sql query/request to insert the current time into the 'Date' column in the table 'Record' string request_text=StringFormat(CalendarStruct(Record_Table).insert,TimeToString(TimeTradeServer())); if(!DatabaseExecute(db, request_text))//Will attempt to run this sql request/query { Print(GetLastError()); PrintFormat(CalendarStruct(Record_Table).insert,TimeToString(TimeTradeServer())); failed=true;//assign true if the request failed } if(failed) { //--- roll back all transactions and unlock the database DatabaseTransactionRollback(db); PrintFormat("%s: DatabaseExecute() failed with code %d", __FUNCTION__, GetLastError()); } } //+------------------------------------------------------------------+ //|Function for creating and inserting Recommend DST for the Broker | //|into a table | //+------------------------------------------------------------------+ void CNews::CreateAutoDST(int db) { bool failed=false;//boolean variable if(!AutoDetectDST(DSTType))//Check if AutoDetectDST went through all the right procedures { return;//will terminate execution of the rest of the code below } if(!DatabaseTableExists(db,CalendarStruct(AutoDST_Table).name))//Checks if the table 'AutoDST' exists in the databse 'Calendar' { //--- create the table AutoDST if(!DatabaseExecute(db,CalendarStruct(AutoDST_Table).sql))//Will attempt to create the table 'AutoDST' { Print("DB: create the AutoDST table failed with code ", GetLastError()); DatabaseClose(db);//Close the database return;//Exits the function if creating the table failed } else//If Table was created Successfully then Create Trigger { DatabaseExecute(db,CalendarStruct(AutoDST_Trigger).sql); } } else { //Create trigger if AutoDST table exists DatabaseExecute(db,CalendarStruct(AutoDST_Trigger).sql); } //Sql query/request to insert the recommend DST for the Broker using the DSTType variable to determine which string data to insert string request_text=StringFormat(CalendarStruct(AutoDST_Table).insert,EnumToString(DSTType)); if(!DatabaseExecute(db, request_text))//Will attempt to run this sql request/query { Print(GetLastError()); PrintFormat(CalendarStruct(AutoDST_Table).insert,EnumToString(DSTType));//Will print the sql query if failed failed=true;//assign true if the request failed } if(failed) { //--- roll back all transactions and unlock the database DatabaseTransactionRollback(db); PrintFormat("%s: DatabaseExecute() failed with code %d", __FUNCTION__, GetLastError()); } } //+------------------------------------------------------------------+ //|Gets the latest/newest date in the Calendar database | //+------------------------------------------------------------------+ datetime CNews::GetLatestNewsDate() { //--- open the database 'Calendar' in the common folder int db=DatabaseOpen(NEWS_DATABASE_FILE, DATABASE_OPEN_READONLY|DATABASE_OPEN_COMMON); if(db==INVALID_HANDLE)//Checks if 'Calendar' failed to be opened { if(!FileIsExist(NEWS_DATABASE_FILE,FILE_COMMON))//Checks if 'Calendar' database exists { Print("Could not find Database!"); return 0;//Will return the earliest date which is 1970.01.01 00:00:00 } } string latest_record="1970.01.01";//string variable with the first/earliest possible date in MQL5 //Sql query to determine the lastest or maximum recorded time from which the database was updated. string request_text="SELECT REPLACE(Date,'-','.') FROM 'Record'"; int request=DatabasePrepare(db,request_text); if(request==INVALID_HANDLE) { Print("DB: ",NEWS_DATABASE_FILE, " request failed with code ", GetLastError()); DatabaseClose(db);//Close Database return 0; } if(DatabaseRead(request))//Will read the one record in the 'Record' table { //-- Will assign the first column(column 0) value to the variable 'latest_record' if(!DatabaseColumnText(request,0,latest_record)) { Print("DatabaseRead() failed with code ", GetLastError()); DatabaseFinalize(request);//Finalize request DatabaseClose(db);//Closes the database 'Calendar' return D'1970.01.01';//Will end the for loop and will return the earliest date which is 1970.01.01 00:00:00 } } DatabaseFinalize(request); DatabaseClose(db);//Closes the database 'Calendar' return (datetime)latest_record;//Returns the string latest_record converted to datetime } //+------------------------------------------------------------------+ //|Function will determine Broker DST | //+------------------------------------------------------------------+ bool CNews::AutoDetectDST(DST_type &dstType) { MqlCalendarValue values[];//Single array of MqlCalendarValue type string eventtime[];//Single string array variable to store NFP(Nonfarm Payrolls) dates for the 'United States' from the previous year //-- Will store the previous year into an integer int lastyear = Time.ReturnYear(Time.TimeMinusOffset(iTime(Symbol(),PERIOD_CURRENT,0),Time.YearsS())); //-- Will store the start date for the previous year datetime lastyearstart = StringToTime(StringFormat("%s.01.01 00:00:00",(string)lastyear)); //-- Will store the end date for the previous year datetime lastyearend = StringToTime(StringFormat("%s.12.31 23:59:59",(string)lastyear)); //-- Getting last year's calendar values for CountryCode = 'US' if(CalendarValueHistory(values,lastyearstart,lastyearend,"US")) { for(int x=0; x<(int)ArraySize(values); x++) { if(values[x].event_id==840030016)//Get only NFP Event Dates { ArrayResize(eventtime,eventtime.Size()+1,eventtime.Size()+2);//Increasing the size of eventtime array by 1 eventtime[eventtime.Size()-1] = TimeToString(values[x].time);//Storing the dates in an array of type string } } } //-- datetime variables to store the broker's timezone shift(change) datetime ShiftStart=D'1970.01.01 00:00:00',ShiftEnd=D'1970.01.01 00:00:00'; string EURUSD="";//String variables declarations for working with EURUSD bool EurusdIsFound=false;//Boolean variables declarations for working with EURUSD for(int i=0;i<SymbolsTotal(true);i++)//Will loop through all the Symbols inside the Market Watch { string SymName = SymbolName(i,true);//Assign the Symbol Name of index 'i' from the list of Symbols inside the Market Watch //-- Check if the Symbol outside the Market Watch has a SYMBOL_CURRENCY_BASE of EUR //-- and a SYMBOL_CURRENCY_PROFIT of USD, and this Symbol is not a Custom Symbol(Is not from the broker) if(((CurrencyBase(SymName)=="EUR"&&CurrencyProfit(SymName)=="USD")|| (StringFind(SymName,"EUR")>-1&&CurrencyProfit(SymName)=="USD"))&&!Custom(SymName)) { EURUSD = SymName;//Assigning the name of the EURUSD Symbol found inside the Market Watch EurusdIsFound = true;//EURUSD Symbol was found in the Trading Terminal for your Broker break;//Will end the for loop } } if(!EurusdIsFound)//Check if EURUSD Symbol was already Found in the Market Watch { for(int i=0; i<SymbolsTotal(false); i++)//Will loop through all the available Symbols outside the Market Watch { string SymName = SymbolName(i,false);//Assign the Symbol Name of index 'i' from the list of Symbols outside the Market Watch //-- Check if the Symbol outside the Market Watch has a SYMBOL_CURRENCY_BASE of EUR //-- and a SYMBOL_CURRENCY_PROFIT of USD, and this Symbol is not a Custom Symbol(Is not from the broker) if(((CurrencyBase(SymName)=="EUR"&&CurrencyProfit(SymName)=="USD")|| (StringFind(SymName,"EUR")>-1&&CurrencyProfit(SymName)=="USD"))&&!Custom(SymName)) { EURUSD = SymName;//Assigning the name of the EURUSD Symbol found outside the Market Watch EurusdIsFound = true;//EURUSD Symbol was found in the Trading Terminal for your Broker break;//Will end the for loop } } } if(!EurusdIsFound)//Check if EURUSD Symbol was Found in the Trading Terminal for your Broker { Print("Cannot Find EURUSD!"); Print("Cannot Create Database!"); Print("Server DST Cannot be Detected!"); dstType = DST_NONE;//Assigning enumeration value DST_NONE, Broker has no DST(Daylight Savings Time) return false;//Returning False, Broker's DST schedule was not found } struct DST { bool result; datetime date; } previousresult,currentresult; bool timeIsShifted;//Boolean variable declaration will be used to determine if the broker changes it's timezone for(uint i=0;i<eventtime.Size();i++) { //-- Store the result of if the eventdate is the larger candlestick currentresult.result = IsLargerThanPreviousAndNext((datetime)eventtime[i],Time.HoursS(),EURUSD); currentresult.date = (datetime)eventtime[i];//Store the eventdate from eventtime[i] //-- Check if there is a difference between the previous result and the current result timeIsShifted = ((currentresult.result!=previousresult.result&&i>0)?true:false); //-- Check if the Larger candle has shifted from the previous event date to the current event date in eventtime[i] array if(timeIsShifted) { if(ShiftStart==D'1970.01.01 00:00:00')//Check if the ShiftStart variable has not been assigned a relevant value yet { ShiftStart=currentresult.date;//Store the eventdate for when the timeshift began } ShiftEnd=previousresult.date;//Store the eventdate timeshift } previousresult.result = currentresult.result;//Store the previous result of if the eventdate is the larger candlestick previousresult.date = currentresult.date;//Store the eventdate from eventtime[i] } //-- Check if the ShiftStart variable has not been assigned a relevant value and the eventdates are more than zero if(ShiftStart==D'1970.01.01 00:00:00'&&eventtime.Size()>0) { Print("Broker ServerTime unchanged!"); dstType = DST_NONE;//Assigning enumeration value DST_NONE, Broker has no DST(Daylight Savings Time) return true;//Returning True, Broker's DST schedule was found successfully } datetime DaylightStart,DaylightEnd;//Datetime variables declarations for start and end dates for DaylightSavings if(Savings_AU.DaylightSavings(lastyear,DaylightStart,DaylightEnd)) { if(Time.DateIsInRange(DaylightStart,DaylightEnd,ShiftStart,ShiftEnd)) { Print("Broker ServerTime Adjusted For AU DST"); dstType = DST_AU;//Assigning enumeration value AU_DST, Broker has AU DST(Daylight Savings Time) return true;//Returning True, Broker's DST schedule was found successfully } } else { Print("Something went wrong!"); Print("Cannot Find Daylight-Savings Date For AU"); Print("Year: %d Cannot Be Found!",lastyear); dstType = DST_NONE;//Assigning enumeration value DST_NONE, Broker has no DST(Daylight Savings Time) return false;//Returning False, Broker's DST schedule was not found } if(Savings_UK.DaylightSavings(lastyear,DaylightStart,DaylightEnd)) { if(Time.DateIsInRange(DaylightStart,DaylightEnd,ShiftStart,ShiftEnd)) { Print("Broker ServerTime Adjusted For UK DST"); dstType = DST_UK;//Assigning enumeration value UK_DST, Broker has UK/EU DST(Daylight Savings Time) return true;//Returning True, Broker's DST schedule was found successfully } } else { Print("Something went wrong!"); Print("Cannot Find Daylight-Savings Date For UK"); Print("Year: %d Cannot Be Found!",lastyear); dstType = DST_NONE;//Assigning enumeration value DST_NONE, Broker has no DST(Daylight Savings Time) return false;//Returning False, Broker's DST schedule was not found } if(Savings_US.DaylightSavings(lastyear,DaylightStart,DaylightEnd)) { if(Time.DateIsInRange(DaylightStart,DaylightEnd,ShiftStart,ShiftEnd)) { Print("Broker ServerTime Adjusted For US DST"); dstType = DST_US;//Assigning enumeration value US_DST, Broker has US DST(Daylight Savings Time) return true;//Returning True, Broker's DST schedule was found successfully } } else { Print("Something went wrong!"); Print("Cannot Find Daylight-Savings Date For US"); Print("Year: %d Cannot Be Found!",lastyear); dstType = DST_NONE;//Assigning enumeration value DST_NONE, Broker has no DST(Daylight Savings Time) return false;//Returning False, Broker's DST schedule was not found } Print("Cannot Detect Broker ServerTime Configuration!"); dstType = DST_NONE;//Assigning enumeration value DST_NONE, Broker has no DST(Daylight Savings Time) return false;//Returning False, Broker's DST schedule was not found } //+------------------------------------------------------------------+
我们将为所需的数据库设计中的每个组件分配一个枚举值,作为一种身份标识。
enum CalendarComponents { AutoDST_Table,//AutoDST Table CalendarAU_View,//View for DST_AU CalendarNONE_View,//View for DST_NONE CalendarUK_View,//View for DST_UK CalendarUS_View,//View for DST_US Record_Table,// Record Table TimeSchedule_Table,//TimeSchedule Table MQL5Calendar_Table,//MQL5Calendar Table AutoDST_Trigger,//Table Trigger for AutoDST Record_Trigger//Table Trigger for Record };
SQLiteMaster表用于存储当前数据库对象的属性,例如类型、名称等。因此,我们可以跟踪DBContents 数组中的所有对象。
//-- structure to retrieve all the objects in the database struct SQLiteMaster { string type;//will store object type string name;//will store object's name string tbl_name;//will store table name int rootpage;//will store rootpage string sql;//Will store the sql create statement } DBContents[];//Array of type SQLiteMaster
在MQL5CalendarContents结构中,我们将存储附加属性,即Content和insert变量。
我们的字符串变量insert将用于存储我们的SQL对象的SQLinsertion语句。而CalendarComponents变量Content将用于存储我们SQL对象的枚举值,作为一种身份标识,一旦所有对象的属性都存储在CalendarContents结构数组中,我们就可以知道哪个SQL对象对应哪个。
//-- MQL5CalendarContents inherits from SQLiteMaster structure struct MQL5CalendarContents:SQLiteMaster { CalendarComponents Content; string insert;//Will store the sql insert statement } CalendarContents[10];//Array to Store objects in our database
在 Content参数等于CalendarContents数组结构中变量 Content 的枚举值时,CalendarStruct函数将返回结构MQL5CalendarContents的值。
//-- Function for retrieving the MQL5CalendarContents structure for the enumartion type CalendarComponents MQL5CalendarContents CalendarStruct(CalendarComponents Content) { MQL5CalendarContents Calendar; for(uint i=0;i<CalendarContents.Size();i++) { if(CalendarContents[i].Content==Content) { return CalendarContents[i]; } } return Calendar; }
字符串变量DropRequest将负责删除我们不再需要或不想要的数据库对象。在SQL查询中,我们使用了PRAGMA语句。
什么是PRAGMA语句?
SQLite中的PRAGMA语句是一种特殊命令,用于修改SQLite库的操作或查询数据库引擎的内部状态。PRAGMA不是标准SQL的一部分,而是SQLite特有的。它们提供了一种方式来控制各种环境设置和数据库行为。
PRAGMA语句有什么用?
- 配置:PRAGMA语句允许您配置数据库环境,例如启用或禁用外键约束、设置日志模式或调整内存使用参数。
- 诊断:它们可用于检索有关数据库的信息,如检查数据库的完整性、获取当前设置或查看SQLite引擎的状态。
- 优化:PRAGMA有助于通过调整缓存大小、锁定模式和同步设置等参数来优化数据库性能。
- 维护:它们对于重建索引、分析表和管理自动真空设置等维护任务非常有用。
在第一个PRAGMA语句中,我们禁用任何外键约束,这些约束会阻止我们删除任何具有外键约束的表。
在我们的第二个PRAGMA语句中,我们启用了secure_delete,它控制删除的内容是否在从数据库文件中删除之前被清零。在这种情况下,如果这样做不会增加I/O量,数据库将用0覆盖删除的内容。
在我们的第三个语句中,我们将删除存在的SQL对象。然后,我们将使用Vacuum命令,该命令将重建数据库文件,将其重新打包为占用最小磁盘空间的格式。此过程有助于优化数据库性能并回收未使用的空间。
最后,我们将重新启用外键约束。
CNews::CNews(void):DropRequest("PRAGMA foreign_keys = OFF; " "PRAGMA secure_delete = ON; " "Drop %s IF EXISTS %s; " "Vacuum; " "PRAGMA foreign_keys = ON;")//Sql drop statement
我们将会把AutoDST表的属性存储到CalendarContents数组的第一个索引中。这里,我们在Content变量中分配了一个枚举值,即AutoDST_Table。
然后,我们为name、table name、type和insert赋值。在创建表的SQL语句中,我们为列'DST'设置默认值为'DST_NONE',并以'STRICT'关键字结束语句。
关键字STRICT强制要求插入到列中的数据必须与该列声明的类型匹配。这提供了存储数据类型的更高可预测性和一致性。
而没有STRICT关键字时,基本上任何数据类型都可以插入到表中,对于声明列的数据类型,仅仅作为推荐使用而非强制。
//-- initializing properties for the AutoDST table CalendarContents[0].Content = AutoDST_Table; CalendarContents[0].name = "AutoDST"; CalendarContents[0].sql = "CREATE TABLE AutoDST(DST TEXT NOT NULL DEFAULT 'DST_NONE')STRICT;"; CalendarContents[0].tbl_name = "AutoDST"; CalendarContents[0].type = "table"; CalendarContents[0].insert = "INSERT INTO 'AutoDST'(DST) VALUES ('%s');";
现在,我们将为UK、US、AU和NONE初始化日历视图的属性。
在view_sql变量中,我们存储用于创建单个视图的SQL语句。在我们的SQL语句中,我们从MQL5Calendar表中选择Eventid、Eventname、Country、EventCurrency和Eventcode。
ID EVENTID COUNTRY EVENTNAME EVENTTYPE EVENTIMPORTANCE EVENTCURRENCY EVENTCODE EVENTSECTOR EVENTFORECAST EVENTPREVALUE EVENTIMPACT EVENTFREQUENCY 18742 999020002 European Union Eurogroup Meeting CALENDAR_TYPE_EVENT CALENDAR_IMPORTANCE_MODERATE EUR EU CALENDAR_SECTOR_GOVERNMENT None None CALENDAR_IMPACT_NA CALENDAR_FREQUENCY_NONE 18746 999010020 European Union ECB Executive Board Member Lane Speech CALENDAR_TYPE_EVENT CALENDAR_IMPORTANCE_MODERATE EUR EU CALENDAR_SECTOR_MONEY None None CALENDAR_IMPACT_NA CALENDAR_FREQUENCY_NONE 34896 392010004 Japan Coincident Index CALENDAR_TYPE_INDICATOR CALENDAR_IMPORTANCE_LOW JPY JP CALENDAR_SECTOR_BUSINESS 113900000 113900000 CALENDAR_IMPACT_NEGATIVE CALENDAR_FREQUENCY_MONTH 34897 392010005 Japan Leading Index CALENDAR_TYPE_INDICATOR CALENDAR_IMPORTANCE_LOW JPY JP CALENDAR_SECTOR_BUSINESS 111400000 111400000 CALENDAR_IMPACT_POSITIVE CALENDAR_FREQUENCY_MONTH 34898 392010011 Japan Coincident Index m/m CALENDAR_TYPE_INDICATOR CALENDAR_IMPORTANCE_LOW JPY JP CALENDAR_SECTOR_BUSINESS None 2400000 CALENDAR_IMPACT_NA CALENDAR_FREQUENCY_MONTH 34899 392010012 Japan Leading Index m/m CALENDAR_TYPE_INDICATOR CALENDAR_IMPORTANCE_LOW JPY JP CALENDAR_SECTOR_BUSINESS None -700000 CALENDAR_IMPACT_NA CALENDAR_FREQUENCY_MONTH 55462 156010014 China Industrial Profit YTD y/y CALENDAR_TYPE_INDICATOR CALENDAR_IMPORTANCE_LOW CNY CN CALENDAR_SECTOR_BUSINESS None 4300000 CALENDAR_IMPACT_NA CALENDAR_FREQUENCY_MONTH 72568 276030001 Germany Ifo Business Expectations CALENDAR_TYPE_INDICATOR CALENDAR_IMPORTANCE_MODERATE EUR DE CALENDAR_SECTOR_BUSINESS 92000000 89900000 CALENDAR_IMPACT_NA CALENDAR_FREQUENCY_MONTH 72569 276030002 Germany Ifo Current Business Situation CALENDAR_TYPE_INDICATOR CALENDAR_IMPORTANCE_MODERATE EUR DE CALENDAR_SECTOR_BUSINESS 88800000 88900000 CALENDAR_IMPACT_NA CALENDAR_FREQUENCY_MONTH 72570 276030003 Germany Ifo Business Climate CALENDAR_TYPE_INDICATOR CALENDAR_IMPORTANCE_HIGH EUR DE CALENDAR_SECTOR_BUSINESS 89900000 89400000 CALENDAR_IMPACT_NA CALENDAR_FREQUENCY_MONTH 72571 276050007 Germany Bbk Executive Board Member Mauderer Speech CALENDAR_TYPE_EVENT CALENDAR_IMPORTANCE_MODERATE EUR DE CALENDAR_SECTOR_MONEY None None CALENDAR_IMPACT_NA CALENDAR_FREQUENCY_NONE 78850 250020001 France 3-Month BTF Auction CALENDAR_TYPE_INDICATOR CALENDAR_IMPORTANCE_LOW EUR FR CALENDAR_SECTOR_MARKET None 3746000 CALENDAR_IMPACT_NA CALENDAR_FREQUENCY_NONE 78851 250020002 France 6-Month BTF Auction CALENDAR_TYPE_INDICATOR CALENDAR_IMPORTANCE_LOW EUR FR CALENDAR_SECTOR_MARKET None 3657000 CALENDAR_IMPACT_NA CALENDAR_FREQUENCY_NONE 78852 250020003 France 12-Month BTF Auction CALENDAR_TYPE_INDICATOR CALENDAR_IMPORTANCE_LOW EUR FR CALENDAR_SECTOR_MARKET None 3467000 CALENDAR_IMPACT_NA CALENDAR_FREQUENCY_NONE 84771 76020007 Brazil BCB Bank Lending m/m CALENDAR_TYPE_INDICATOR CALENDAR_IMPORTANCE_LOW BRL BR CALENDAR_SECTOR_MONEY 400000 1200000 CALENDAR_IMPACT_NA CALENDAR_FREQUENCY_MONTH 84772 76020001 Brazil BCB Focus Market Report CALENDAR_TYPE_EVENT CALENDAR_IMPORTANCE_MODERATE BRL BR CALENDAR_SECTOR_MONEY None None CALENDAR_IMPACT_NA CALENDAR_FREQUENCY_NONE 94938 344020004 Hong Kong Exports y/y CALENDAR_TYPE_INDICATOR CALENDAR_IMPORTANCE_LOW HKD HK CALENDAR_SECTOR_TRADE 18100000 4700000 CALENDAR_IMPACT_NA CALENDAR_FREQUENCY_MONTH 94939 344020005 Hong Kong Imports y/y CALENDAR_TYPE_INDICATOR CALENDAR_IMPORTANCE_LOW HKD HK CALENDAR_SECTOR_TRADE 15000000 5300000 CALENDAR_IMPACT_NA CALENDAR_FREQUENCY_MONTH 94940 344020006 Hong Kong Trade Balance CALENDAR_TYPE_INDICATOR CALENDAR_IMPORTANCE_MODERATE HKD HK CALENDAR_SECTOR_TRADE -29054000 -45000000 CALENDAR_IMPACT_NA CALENDAR_FREQUENCY_MONTH 102731 578020001 Norway Unemployment Rate CALENDAR_TYPE_INDICATOR CALENDAR_IMPORTANCE_MODERATE NOK NO CALENDAR_SECTOR_JOBS 3700000 4000000 CALENDAR_IMPACT_NA CALENDAR_FREQUENCY_MONTH 102732 578020020 Norway General Public Domestic Loan Debt y/y CALENDAR_TYPE_INDICATOR CALENDAR_IMPORTANCE_LOW NOK NO CALENDAR_SECTOR_MONEY 3300000 3500000 CALENDAR_IMPACT_NA CALENDAR_FREQUENCY_MONTH 147163 840031004 United States Memorial Day CALENDAR_TYPE_HOLIDAY CALENDAR_IMPORTANCE_NONE USD US CALENDAR_SECTOR_HOLIDAYS None None CALENDAR_IMPACT_NA CALENDAR_FREQUENCY_NONE 162245 826090005 United Kingdom Spring Bank Holiday CALENDAR_TYPE_HOLIDAY CALENDAR_IMPORTANCE_NONE GBP GB CALENDAR_SECTOR_HOLIDAYS None None CALENDAR_IMPACT_NA CALENDAR_FREQUENCY_NONE
然后,我们从TimeSchedule中为相应的时间表选择DST列。
ID DST_UK DST_US DST_AU DST_NONE 18742 2024.05.27 02:00 2024.05.27 02:00 2024.05.27 02:00 2024.05.27 02:00 18746 2024.05.27 14:00 2024.05.27 14:00 2024.05.27 14:00 2024.05.27 14:00 34896 2024.05.27 07:00 2024.05.27 07:00 2024.05.27 07:00 2024.05.27 07:00 34897 2024.05.27 07:00 2024.05.27 07:00 2024.05.27 07:00 2024.05.27 07:00 34898 2024.05.27 07:00 2024.05.27 07:00 2024.05.27 07:00 2024.05.27 07:00 34899 2024.05.27 07:00 2024.05.27 07:00 2024.05.27 07:00 2024.05.27 07:00 55462 2024.05.27 03:30 2024.05.27 03:30 2024.05.27 03:30 2024.05.27 03:30 72568 2024.05.27 10:30 2024.05.27 10:30 2024.05.27 10:30 2024.05.27 10:30 72569 2024.05.27 10:30 2024.05.27 10:30 2024.05.27 10:30 2024.05.27 10:30 72570 2024.05.27 10:30 2024.05.27 10:30 2024.05.27 10:30 2024.05.27 10:30 72571 2024.05.27 15:30 2024.05.27 15:30 2024.05.27 15:30 2024.05.27 15:30 78850 2024.05.27 14:50 2024.05.27 14:50 2024.05.27 14:50 2024.05.27 14:50 78851 2024.05.27 14:50 2024.05.27 14:50 2024.05.27 14:50 2024.05.27 14:50 78852 2024.05.27 14:50 2024.05.27 14:50 2024.05.27 14:50 2024.05.27 14:50 84771 2024.05.27 13:30 2024.05.27 13:30 2024.05.27 13:30 2024.05.27 13:30 84772 2024.05.27 13:30 2024.05.27 13:30 2024.05.27 13:30 2024.05.27 13:30 94938 2024.05.27 10:30 2024.05.27 10:30 2024.05.27 10:30 2024.05.27 10:30 94939 2024.05.27 10:30 2024.05.27 10:30 2024.05.27 10:30 2024.05.27 10:30 94940 2024.05.27 10:30 2024.05.27 10:30 2024.05.27 10:30 2024.05.27 10:30 102731 2024.05.27 08:00 2024.05.27 08:00 2024.05.27 08:00 2024.05.27 08:00 102732 2024.05.27 08:00 2024.05.27 08:00 2024.05.27 08:00 2024.05.27 08:00 147163 2024.05.27 02:00 2024.05.27 02:00 2024.05.27 02:00 2024.05.27 02:00 162245 2024.05.27 02:00 2024.05.27 02:00 2024.05.27 02:00 2024.05.27 02:00
并将两个表MQL5Calendar和TimeSchedule连接到同一ID上。
我们使用Record表中的Date过滤此列表。
Date 27-05-2024
一旦我们从查询到结果,将根据TimeSchedule的相应DST时间按升序排序。
string views[] = {"UK","US","AU","NONE"}; string view_sql = "CREATE VIEW IF NOT EXISTS Calendar_%s " "AS " "SELECT C.Eventid,C.Eventname,C.Country,T.DST_%s as Time,C.EventCurrency,C.Eventcode from MQL5Calendar C,Record R " "Inner join TimeSchedule T on C.ID=T.ID " "Where DATE(REPLACE(T.DST_%s,'.','-'))=R.Date " "Order by T.DST_%s Asc;"; //-- Sql statements for creating the table views for(uint i=1;i<=views.Size();i++) { CalendarContents[i].Content = (CalendarComponents)i; CalendarContents[i].name = StringFormat("Calendar_%s",views[i-1]); CalendarContents[i].sql = StringFormat(view_sql,views[i-1],views[i-1],views[i-1],views[i-1]); CalendarContents[i].tbl_name = StringFormat("Calendar_%s",views[i-1]); CalendarContents[i].type = "view"; }
我们将查看其中一个视图,看看查询结果如何:
SELECT * FROM 'Calendar_UK';
输出:
EVENTID EVENTNAME COUNTRY Time EVENTCURRENCY EVENTCODE 999020002 Eurogroup Meeting European Union 2024.05.27 02:00 EUR EU 840031004 Memorial Day United States 2024.05.27 02:00 USD US 826090005 Spring Bank Holiday United Kingdom 2024.05.27 02:00 GBP GB 156010014 Industrial Profit YTD y/y China 2024.05.27 03:30 CNY CN 392010004 Coincident Index Japan 2024.05.27 07:00 JPY JP 392010005 Leading Index Japan 2024.05.27 07:00 JPY JP 392010011 Coincident Index m/m Japan 2024.05.27 07:00 JPY JP 392010012 Leading Index m/m Japan 2024.05.27 07:00 JPY JP 578020001 Unemployment Rate Norway 2024.05.27 08:00 NOK NO 578020020 General Public Domestic Loan Debt y/y Norway 2024.05.27 08:00 NOK NO 276030001 Ifo Business Expectations Germany 2024.05.27 10:30 EUR DE 276030002 Ifo Current Business Situation Germany 2024.05.27 10:30 EUR DE 276030003 Ifo Business Climate Germany 2024.05.27 10:30 EUR DE 344020004 Exports y/y Hong Kong 2024.05.27 10:30 HKD HK 344020005 Imports y/y Hong Kong 2024.05.27 10:30 HKD HK 344020006 Trade Balance Hong Kong 2024.05.27 10:30 HKD HK 76020007 BCB Bank Lending m/m Brazil 2024.05.27 13:30 BRL BR 76020001 BCB Focus Market Report Brazil 2024.05.27 13:30 BRL BR 999010020 ECB Executive Board Member Lane Speech European Union 2024.05.27 14:00 EUR EU 250020001 3-Month BTF Auction France 2024.05.27 14:50 EUR FR 250020002 6-Month BTF Auction France 2024.05.27 14:50 EUR FR 250020003 12-Month BTF Auction France 2024.05.27 14:50 EUR FR 276050007 Bbk Executive Board Member Mauderer Speech Germany 2024.05.27 15:30 EUR DE
我们有一个新表,名为Record,这个表将替代我们之前的Records表,因为现在我们将只存储一条记录。我们的表将有一个数据类型为TEXT的列,列名为'Date',不要将其与SQLite中的Date函数混淆。
//-- initializing properties for the Record table CalendarContents[5].Content = Record_Table; CalendarContents[5].name = "Record"; CalendarContents[5].sql = "CREATE TABLE Record(Date TEXT NOT NULL)STRICT;"; CalendarContents[5].tbl_name="Record"; CalendarContents[5].type = "table"; CalendarContents[5].insert = "INSERT INTO 'Record'(Date) VALUES (Date(REPLACE('%s','.','-')));";
我们的TimeSchedule表将存储所有单个事件的时间数据,并将使用外键标识'ID'来将该表(创建关系)与MQL5Calendar表关联起来。
//-- initializing properties for the TimeSchedule table CalendarContents[6].Content = TimeSchedule_Table; CalendarContents[6].name = "TimeSchedule"; CalendarContents[6].sql = "CREATE TABLE TimeSchedule(ID INT NOT NULL,DST_UK TEXT NOT NULL,DST_US TEXT NOT NULL," "DST_AU TEXT NOT NULL,DST_NONE TEXT NOT NULL,FOREIGN KEY (ID) REFERENCES MQL5Calendar (ID))STRICT;"; CalendarContents[6].tbl_name="TimeSchedule"; CalendarContents[6].type = "table"; CalendarContents[6].insert = "INSERT INTO 'TimeSchedule'(ID,DST_UK,DST_US,DST_AU,DST_NONE) " "VALUES (%d,'%s','%s', '%s', '%s');";
MQL5日历表有一个名为“ID”的主键,该主键对于表中的每个新闻事件记录都是唯一的。
//-- initializing properties for the MQL5Calendar table CalendarContents[7].Content = MQL5Calendar_Table; CalendarContents[7].name = "MQL5Calendar"; CalendarContents[7].sql = "CREATE TABLE MQL5Calendar(ID INT NOT NULL,EVENTID INT NOT NULL,COUNTRY TEXT NOT NULL," "EVENTNAME TEXT NOT NULL,EVENTTYPE TEXT NOT NULL,EVENTIMPORTANCE TEXT NOT NULL," "EVENTCURRENCY TEXT NOT NULL,EVENTCODE TEXT NOT NULL,EVENTSECTOR TEXT NOT NULL," "EVENTFORECAST TEXT NOT NULL,EVENTPREVALUE TEXT NOT NULL,EVENTIMPACT TEXT NOT NULL," "EVENTFREQUENCY TEXT NOT NULL,PRIMARY KEY(ID))STRICT;"; CalendarContents[7].tbl_name="MQL5Calendar"; CalendarContents[7].type = "table"; CalendarContents[7].insert = "INSERT INTO 'MQL5Calendar'(ID,EVENTID,COUNTRY,EVENTNAME,EVENTTYPE,EVENTIMPORTANCE,EVENTCURRENCY,EVENTCODE," "EVENTSECTOR,EVENTFORECAST,EVENTPREVALUE,EVENTIMPACT,EVENTFREQUENCY) " "VALUES (%d,%d,'%s','%s','%s','%s','%s','%s','%s','%s','%s','%s','%s');";
我们将新建一个名为OnlyOne_AutoDST的触发器。该触发器的触发条件是,我们尝试向AutoDST表中插入一个值时,并且在插入新记录之前删除AutoDST中的所有记录。
//-- Sql statement for creating the AutoDST table's trigger CalendarContents[8].Content = AutoDST_Trigger; CalendarContents[8].name = "OnlyOne_AutoDST"; CalendarContents[8].sql = "CREATE TRIGGER IF NOT EXISTS OnlyOne_AutoDST " "BEFORE INSERT ON AutoDST " "BEGIN " "Delete from AutoDST; " "END;"; CalendarContents[8].tbl_name="AutoDST"; CalendarContents[8].type = "trigger";
Only One_Record也是如此,但该触发器与Record表有关。
//-- Sql statement for creating the Record table's trigger CalendarContents[9].Content = Record_Trigger; CalendarContents[9].name = "OnlyOne_Record"; CalendarContents[9].sql = "CREATE TRIGGER IF NOT EXISTS OnlyOne_Record " "BEFORE INSERT ON Record " "BEGIN " "Delete from Record; " "END;"; CalendarContents[9].tbl_name="Record"; CalendarContents[9].type = "trigger";
当前,在我们的UpdateRecords函数中,我们将确定日历数据库是否需要更新。
与第一部分相比,此函数的主要更改如下:
1. 我们将使用SQL查询语句“select * from sqlite_master where type<>'index' ;”读取数据库中所有不是索引的对象。
2. 我们将把所有对象的属性存储到数组DBContents中,如果SQL语句末尾没有分号,我们将添加一个分号。
3. 我们将比较数据库中找到的对象和我们在数组CalendarContents中初始化的对象。我们会从CalendarContents的SQL语句中移除' IF NOT EXISTS'。
4. 当我们在DBContents和CalendarContents之间找不到匹配项时,我们将删除DBcontents索引中的对象。
5. 如果SQL对象的匹配数量不等于CalendarContents的大小,我们将执行更新操作。
bool CNews::UpdateRecords() { //initialize variable to true bool perform_update=true; //--- open/create //-- try to open database Calendar int db=DatabaseOpen(NEWS_DATABASE_FILE, DATABASE_OPEN_READWRITE | DATABASE_OPEN_CREATE| DATABASE_OPEN_COMMON); if(db==INVALID_HANDLE)//Checks if the database was able to be opened { //if opening the database failed if(!FileIsExist(NEWS_DATABASE_FILE,FILE_COMMON))//Checks if the database Calendar exists in the common folder { return perform_update;//Returns true when the database was failed to be opened and the file doesn't exist in the common folder } } int MasterRequest = DatabasePrepare(db,"select * from sqlite_master where type<>'index';"); if(MasterRequest==INVALID_HANDLE) { Print("DB: ",NEWS_DATABASE_FILE, " request failed with code ", GetLastError()); } else { SQLiteMaster ReadContents; //Assigning values from the sql query into DBContents array for(int i=0; DatabaseReadBind(MasterRequest,ReadContents); i++) { ArrayResize(DBContents,i+1,i+2); DBContents[i].type = ReadContents.type; DBContents[i].name = ReadContents.name; DBContents[i].tbl_name = ReadContents.tbl_name; DBContents[i].rootpage = ReadContents.rootpage; /*Check if the end of the sql string has a character ';' if not add this character to the string*/ DBContents[i].sql = (StringFind(ReadContents.sql,";",StringLen(ReadContents.sql)-1)== (StringLen(ReadContents.sql)-1))?ReadContents.sql:ReadContents.sql+";";; } uint contents_exists = 0; for(uint i=0;i<DBContents.Size();i++) { bool isCalendarContents = false; for(uint x=0;x<CalendarContents.Size();x++) { /*Store Sql query from CalendarContents without string ' IF NOT EXISTS'*/ string CalendarSql=CalendarContents[x].sql; StringReplace(CalendarSql," IF NOT EXISTS",""); //-- Check if the Db object is in our list if(DBContents[i].name==CalendarContents[x].name&& (DBContents[i].sql==CalendarSql|| DBContents[i].sql==CalendarContents[x].sql)&& CalendarContents[x].type==DBContents[i].type&& CalendarContents[x].tbl_name==DBContents[i].tbl_name) { contents_exists++; isCalendarContents = true; } } if(!isCalendarContents) { //-- Print DBcontent's name if it does not match with CalendarContents PrintFormat("DBContent: %s is not needed!",DBContents[i].name); //-- We will drop the table if it is not neccessary DatabaseExecute(db,StringFormat(DropRequest,DBContents[i].type,DBContents[i].name)); Print("Attempting To Clean Database..."); } } /*If not all the CalendarContents exist in the Calendar Database before an update */ if(contents_exists!=CalendarContents.Size()) { return perform_update; } } if(!DatabaseTableExists(db,CalendarStruct(Record_Table).name))//If the database table 'Record' doesn't exist { DatabaseClose(db); return perform_update; } //-- Sql query to determine the lastest or maximum date recorded /* If the last recorded date data in the 'Record' table is not equal to the current day, perform an update! */ string request_text=StringFormat("SELECT Date FROM %s where Date=Date(REPLACE('%s','.','-'))", CalendarStruct(Record_Table).name,TimeToString(TimeTradeServer())); int request=DatabasePrepare(db,request_text);//Creates a handle of a request, which can then be executed using DatabaseRead() if(request==INVALID_HANDLE)//Checks if the request failed to be completed { Print("DB: ",NEWS_DATABASE_FILE, " request failed with code ", GetLastError()); DatabaseClose(db); return perform_update; } if(DatabaseRead(request))//Will be true if there are results from the sql query/request { DatabaseFinalize(request);//Removes a request created in DatabasePrepare() DatabaseClose(db);//Closes the database perform_update=false; return perform_update; } else { DatabaseFinalize(request);//Removes a request created in DatabasePrepare() DatabaseClose(db);//Closes the database return perform_update; } }
在CreateCalendarTable函数中,我们将检查MQL5Calendar表是否已存在于日历数据库中,同时还会检查TimeSchedule表是否已存在,并尝试在它们存在的情况下删除每张表。由于TimeSchedule表依赖于MQL5Calendar表,因此我们需要先删除TimeSchedule表之后再删除MQL5Calendar表。
一旦MQL5Calendar表不存在,我们将创建该表。
bool CNews::CreateCalendarTable(int db,bool &tableExists) { //-- Checks if a table 'MQL5Calendar' exists if(DatabaseTableExists(db,CalendarStruct(MQL5Calendar_Table).name)) { tableExists=true;//Assigns true to tableExists variable //-- Checks if a table 'TimeSchedule' exists in the database 'Calendar' if(DatabaseTableExists(db,CalendarStruct(TimeSchedule_Table).name)) { //-- We will drop the table if the table already exists if(!DatabaseExecute(db,StringFormat("Drop Table %s",CalendarStruct(TimeSchedule_Table).name))) { //If the table failed to be dropped/deleted PrintFormat("Failed to drop table %s with code %d",CalendarStruct(TimeSchedule_Table).name,GetLastError()); DatabaseClose(db);//Close the database return false;//will terminate execution of the rest of the code below and return false, when the table cannot be dropped } } //--We will drop the table if the table already exists if(!DatabaseExecute(db,StringFormat("Drop Table %s",CalendarStruct(MQL5Calendar_Table).name))) { //If the table failed to be dropped/deleted PrintFormat("Failed to drop table %s with code %d",CalendarStruct(MQL5Calendar_Table).name,GetLastError()); DatabaseClose(db);//Close the database return false;//will terminate execution of the rest of the code below and return false, when the table cannot be dropped } } //-- If the database table 'MQL5Calendar' doesn't exist if(!DatabaseTableExists(db,CalendarStruct(MQL5Calendar_Table).name)) { //--- create the table 'MQL5Calendar' if(!DatabaseExecute(db,CalendarStruct(MQL5Calendar_Table).sql))//Checks if the table was successfully created { Print("DB: create the Calendar table failed with code ", GetLastError()); DatabaseClose(db);//Close the database return false;//Function returns false if creating the table failed } } return true;//Function returns true if creating the table was successful }
在函数CreateTimeTable中,我们验证该表是否存在于Calendar数据库中,如果不存在,则创建它。
bool CNews::CreateTimeTable(int db,bool &tableExists) { //-- If the database table 'TimeSchedule' doesn't exist if(!DatabaseTableExists(db,CalendarStruct(TimeSchedule_Table).name)) { //--- create the table 'TimeSchedule' if(!DatabaseExecute(db,CalendarStruct(TimeSchedule_Table).sql))//Checks if the table was successfully created { Print("DB: create the Calendar table failed with code ", GetLastError()); DatabaseClose(db);//Close the database return false;//Function returns false if creating the table failed } } return true;//Function returns true if creating the table was successful }
在CreateCalendarViews函数中,我们使用CalendarComponents创建所有视图,以查找每个视图的身份标识(枚举值),并据此创建每个视图。
void CNews::CreateCalendarViews(int db) { for(uint i=1;i<=4;i++) { if(!DatabaseExecute(db,CalendarStruct((CalendarComponents)i).sql))//Checks if the view was successfully created { Print("DB: create the Calendar view failed with code ", GetLastError()); } } }
在InsertIntoTables函数中,我们将把Evalues数组中的每条记录分别插入到MQL5Calendar表和TimeSchedule表中。对于TimeSchedule表中的事件日期,将会根据不同的夏令时安排进行相应的调整。
bool CNews::InsertIntoTables(int db,Calendar &Evalues[]) { for(uint i=0; i<Evalues.Size(); i++)//Looping through all the Economic Events { string request_insert_into_calendar = StringFormat(CalendarStruct(MQL5Calendar_Table).insert, i, Evalues[i].EventId, Evalues[i].CountryName, Evalues[i].EventName, Evalues[i].EventType, Evalues[i].EventImportance, Evalues[i].EventCurrency, Evalues[i].EventCode, Evalues[i].EventSector, Evalues[i].EventForecast, Evalues[i].EventPreval, Evalues[i].EventImpact, Evalues[i].EventFrequency);//Inserting all the columns for each event record if(DatabaseExecute(db,request_insert_into_calendar))//Check if insert query into calendar was successful { string request_insert_into_time = StringFormat(CalendarStruct(TimeSchedule_Table).insert, i, //-- Economic EventDate adjusted for UK DST(Daylight Savings Time) Savings_UK.adjustDaylightSavings(StringToTime(Evalues[i].EventDate)), //-- Economic EventDate adjusted for US DST(Daylight Savings Time) Savings_US.adjustDaylightSavings(StringToTime(Evalues[i].EventDate)), //-- Economic EventDate adjusted for AU DST(Daylight Savings Time) Savings_AU.adjustDaylightSavings(StringToTime(Evalues[i].EventDate)), Evalues[i].EventDate//normal Economic EventDate );//Inserting all the columns for each event record if(!DatabaseExecute(db,request_insert_into_time)) { Print(GetLastError()); //-- Will print the sql query to check for any errors or possible defaults in the query/request Print(request_insert_into_time); return false;//Will end the loop and return false, as values failed to be inserted into the table } } else { Print(GetLastError()); //-- Will print the sql query to check for any errors or possible defaults in the query/request Print(request_insert_into_calendar); return false;//Will end the loop and return false, as values failed to be inserted into the table } } return true;//Will return true, all values were inserted into the table successfully }
在CreateRecordTable函数中,我们首先检查Record表是否已经存在,如果不存在,则创建该表。一旦Record表创建完成,我们接着会为该表新建一个触发器。随后,我们会将当前服务器日期插入到表中。
为什么使用TimeTradeServer而不是TimeCurrent?
如果我们使用TimeCurrent,那么得到的时间数据将是当前交易品种的时间数据,而每个交易品种的时间数据可能会随着每个新报价的到达而更新。当交易品种的交易时间不同,或者当前图表对应的交易品种已关闭(即不再接收新的报价)时,这可能会引发问题。因为一旦关闭,TimeCurrent可能会返回一个比实际日期落后一天或更多天的日期。而TimeTradeServer则不受交易品种类型的影响,始终保持更新。
void CNews::CreateRecordTable(int db) { bool failed=false; if(!DatabaseTableExists(db,CalendarStruct(Record_Table).name))//Checks if the table 'Record' exists in the databse 'Calendar' { //--- create the table if(!DatabaseExecute(db,CalendarStruct(Record_Table).sql))//Will attempt to create the table 'Record' { Print("DB: create the Records table failed with code ", GetLastError()); DatabaseClose(db);//Close the database return;//Exits the function if creating the table failed } else//If Table was created Successfully then Create Trigger { DatabaseExecute(db,CalendarStruct(Record_Trigger).sql); } } else { DatabaseExecute(db,CalendarStruct(Record_Trigger).sql); } //Sql query/request to insert the current time into the 'Date' column in the table 'Record' string request_text=StringFormat(CalendarStruct(Record_Table).insert,TimeToString(TimeTradeServer())); if(!DatabaseExecute(db, request_text))//Will attempt to run this sql request/query { Print(GetLastError()); PrintFormat(CalendarStruct(Record_Table).insert,TimeToString(TimeTradeServer())); failed=true;//assign true if the request failed } if(failed) { //--- roll back all transactions and unlock the database DatabaseTransactionRollback(db); PrintFormat("%s: DatabaseExecute() failed with code %d", __FUNCTION__, GetLastError()); } }在CreateAutoDST函数中,我们首先检查经纪人的夏令时时间表。如果成功获取到夏令时时间表,接下来我们会检查日历数据库中是否存在AutoDST表。如果AutoDST表不存在,则会新建该表。一旦AutoDST表存在,我们就会为其创建触发器,并尝试将夏令时时间表从枚举类型转换为字符串后插入表中。
void CNews::CreateAutoDST(int db) { bool failed=false;//boolean variable if(!AutoDetectDST(DSTType))//Check if AutoDetectDST went through all the right procedures { return;//will terminate execution of the rest of the code below } if(!DatabaseTableExists(db,CalendarStruct(AutoDST_Table).name))//Checks if the table 'AutoDST' exists in the databse 'Calendar' { //--- create the table AutoDST if(!DatabaseExecute(db,CalendarStruct(AutoDST_Table).sql))//Will attempt to create the table 'AutoDST' { Print("DB: create the AutoDST table failed with code ", GetLastError()); DatabaseClose(db);//Close the database return;//Exits the function if creating the table failed } else//If Table was created Successfully then Create Trigger { DatabaseExecute(db,CalendarStruct(AutoDST_Trigger).sql); } } else { //Create trigger if AutoDST table exists DatabaseExecute(db,CalendarStruct(AutoDST_Trigger).sql); } //Sql query/request to insert the recommend DST for the Broker using the DSTType variable to determine which string data to insert string request_text=StringFormat(CalendarStruct(AutoDST_Table).insert,EnumToString(DSTType)); if(!DatabaseExecute(db, request_text))//Will attempt to run this sql request/query { Print(GetLastError()); PrintFormat(CalendarStruct(AutoDST_Table).insert,EnumToString(DSTType));//Will print the sql query if failed failed=true;//assign true if the request failed } if(failed) { //--- roll back all transactions and unlock the database DatabaseTransactionRollback(db); PrintFormat("%s: DatabaseExecute() failed with code %d", __FUNCTION__, GetLastError()); } }
风险管理类
风险管理是成功交易的关键组成部分,其主要目标是保护交易资金。没有资金,交易者就无法继续进行交易。实施限制损失的策略可以确保交易者在市场中存活得更久,从而提供更多从挫折中恢复并实现整体盈利的机会。在本例中,我们将为用户提供不同的风险等级供其选择,并找到最适合的选项。
免责声明:在风险管理的上下文中,我将交替使用手数、手数大小和交易量这三个术语。请将它们视为同一概念。
风险等级列表
- 最小手数
- 最大手数
- 账户余额百分比
- 可用保证金百分比
- 各账户余额的风险金额
- 各可用保证金的风险金额
- 各账户余额的手数
- 各可用保证金的手数
- 自定义手数
- 风险百分比
最小手数:
在此风险选项中,我们将使用当前交易品种允许的最小手数。
最大手数:
在此风险选项中,我们将使用当前交易品种允许的最大手数。
账户余额百分比:
在此风险选项中,我们首先将确定风险金额。
amount_of_risk = Balance*Percent;
我们设置Percent = 5%并且账户余额是10000。
amount_of_risk = 10000*(5/100); amount_of_risk = 500;
然后,我们需要一个开盘价和一个收盘价来计算在使用最小手数进行特定交易时的最小风险。
OrderCalcProfit(ORDER_TYPE,Symbol(),Minimum_lotsize,OpenPrice,ClosePrice,Minimum_risk);
一旦确定了最小风险(Minimum_risk),我们将使用以下公式来计算达到特定风险金额(amount_of_risk)所需的手数(Lot-size)
required_lotsize = (amount_of_risk/Minimum_risk)*Minimum_lotsize;
我们让Minimum_risk = 100并且Minimum_lotsize = 0.1;
required_lotsize = (500/100)*0.1; required_lotsize = 5*0.1; required_lotsize = 0.5;
可用保证金百分比:
这一风险选项与账户余额百分比相似。但是,当交易者账户中有未平仓交易时,这一风险选项的优势就体现出来了。
因为只要账户余额相同,无论是否有未平仓交易,账户余额百分比的风险都保持不变。而可用保证金百分比的风险则会随着未平仓交易盈亏的波动而变化。这为当前账户状况提供了更准确的风险计算。
各账户余额的风险金额:
在这一风险选项中,我们首先需要获得风险金额(除数)与账户余额(被除数)之间的商。
risk = Balance/Risk_in_Amount;
然后,我们将设置Balance=10000,Risk_in_Amount=800;
在本例中,对于交易者账户余额中的每10000美元,我们基本上希望承担每笔交易800美元的风险。
risk = 10000/800; risk = 12.5;
然后,我们将风险除以实际的账户余额,得到我风险金额。
amount_of_risk = AccountBalance/risk;
我们设置AccountBalance = 5000;
amount_of_risk = 5000/12.5; amount_of_risk = 400;
现在我们知道交易者希望在这笔特定交易中承担400美元的风险。
各可用保证金的风险金额:
这一风险选项与各账户余额的风险金额相似,我们再举一个例子来说明。
risk = FreeMargin/Risk_in_Amount;
我们设置FreeMargin=150,Risk_in_Mounter=1;
在本例中,我们将为每150美元的可用保证金承担1美元的风险。
risk = 150/1; risk = 150; amount_of_risk = AccountFreeMargin/risk; //-- Let AccountFreeMargin = 750 amount_of_risk = 750/150; amount_of_risk = 5;
在确定了风险金额后,我们将计算满足5美元风险要求的该笔特定交易所需的手数。
各账户余额的手数:
在这一风险选项中,交易者将提供他们希望为特定账户余额所承担风险的手数。
required_lotsize = (AccountBalance/Balance)*lotsize;
其中,AccountBalance指的是交易者的实际账户余额,Balance和lotsize是交易者提供的输入值。
我们设置AccountBalance = 10,000,Balance = 350并且lotsize = 0.01;
在本例中,交易者希望为账户余额中的每350美元承担0.01手的风险。
required_lotsize = (10000/350)*0.01; required_lotsize = 0.285;
所需的手数(required_lotsize)是0.285,但实际值可能是一个更长的数字。假设我们想要交易的具体交易品种的交易量步长为0.01。当交易量步长为0.01时,尝试以0.285手进行交易将会导致错误。
为了防止这种情况,我们将对手数进行规范化处理,即根据交易量步长来格式化手数。
required_lotsize = Volume_Step*MathFloor(0.285/Volume_Step); requred_lotsize = 0.01*MathFloor(0.285/0.01); required_lotsize = 0.01*MathFloor(28.5); required_lotsize = 0.01*28; required_lotsize = 0.28;
各可用保证金的手数:
这一风险选项与各账户余额的手数类似,我们将再举一个例子来说明。
required_lotsize = (AccountFreeMargin/FreeMargin)*lotsize;
我们设置AccountFreeMargin = 134,560,FreeMargin = 1622并且lot-size = 0.0056
在这种情况下:
required_lotsize = (134560/1622)*0.0056; required_lotsize = 0.464;
假设交易量步长为0.02。
我们将根据交易量步长对手数(required_lotsize)进行规范化处理。
//-- normalize for Volume Step required_lotsize = Volume_Step*MathFloor(0.464/Volume_Step); requred_lotsize = 0.02*MathFloor(0.464/0.02); required_lotsize = 0.02*MathFloor(23.2); required_lotsize = 0.02*23; required_lotsize = 0.46;
自定义手数:
在这个风险选项中,我们将使用交易者提供的手数输入。
风险百分比:
在这个风险选项中,我们将使用可用保证金和特定交易品种的保证金要求来计算当前交易品种的最大允许风险百分比。
CRiskManagement类从多个类继承而来,形成了多级继承结构:
- CSymbolProperties
- CChartProperties
CRiskManagement类包含了CAccountInfo类的某些功能或属性。
CRiskManagement类从多个类继承了层次化的结构和功能:
- CSymbolProperties
- CSymbolInfo
//+------------------------------------------------------------------+ //| NewsTrading | //| Copyright 2024, MetaQuotes Ltd. | //| https://www.mql5.com/en/users/kaaiblo | //+------------------------------------------------------------------+ #include "ChartProperties.mqh" #include <Trade/AccountInfo.mqh> CAccountInfo Account; //-- Enumeration declaration for Risk options enum RiskOptions { MINIMUM_LOT,//MINIMUM LOTSIZE MAXIMUM_LOT,//MAXIMUM LOTSIZE PERCENTAGE_OF_BALANCE,//PERCENTAGE OF BALANCE PERCENTAGE_OF_FREEMARGIN,//PERCENTAGE OF FREE-MARGIN AMOUNT_PER_BALANCE,//AMOUNT PER BALANCE AMOUNT_PER_FREEMARGIN,//AMOUNT PER FREE-MARGIN LOTSIZE_PER_BALANCE,//LOTSIZE PER BALANCE LOTSIZE_PER_FREEMARGIN,//LOTSIZE PER FREE-MARGIN CUSTOM_LOT,//CUSTOM LOTSIZE PERCENTAGE_OF_MAXRISK//PERCENTAGE OF MAX-RISK } RiskProfileOption;//variable for Risk options //-- Enumeration declaration for Risk floor enum RiskFloor { RiskFloorMin,//MINIMUM LOTSIZE RiskFloorMax,//MAX-RISK RiskFloorNone//NONE } RiskFloorOption;//variable for Risk floor //-- Enumeration declaration for Risk ceiling(Maximum allowable risk in terms of lot-size) enum RiskCeil { RiskCeilMax,//MAX LOTSIZE RiskCeilMax2,//MAX LOTSIZE(x2) RiskCeilMax3,//MAX LOTSIZE(x3) RiskCeilMax4,//MAX LOTSIZE(x4) RiskCeilMax5,//MAX LOTSIZE(x5) } RiskCeilOption;//variable for Risk ceiling //-- Structure declaration for Risk options (AMOUNT PER BALANCE and AMOUNT PER FREE-MARGIN) struct RISK_AMOUNT { double RiskAmountBoF;//store Balance or Free-Margin double RiskAmount;//store risk amount } Risk_Profile_2;//variable for Risk options (AMOUNT PER BALANCE and AMOUNT PER FREE-MARGIN) //-- Structure declaration for Risk options (LOTSIZE PER BALANCE and LOTSIZE PER FREE-MARGIN) struct RISK_LOT { double RiskLotBoF;//store Balance or Free-Margin double RiskLot;//store lot-size } Risk_Profile_3;//variable for Risk options (LOTSIZE PER BALANCE and LOTSIZE PER FREE-MARGIN) double RiskFloorPercentage;//variable for RiskFloorMax double Risk_Profile_1;//variable for Risk options (PERCENTAGE OF BALANCE and PERCENTAGE OF FREE-MARGIN) double Risk_Profile_4;//variable for Risk option (CUSTOM LOTSIZE) double Risk_Profile_5;//variable for Risk option (PERCENTAGE OF MAX-RISK) //+------------------------------------------------------------------+ //|RiskManagement class | //+------------------------------------------------------------------+ class CRiskManagement : public CChartProperties { private: double Medium;//variable to store actual Account (Balance or Free-Margin) double RiskAmount,MinimumAmount; double Lots;//variable to store Lot-size to open trade const double max_percent;//variable to store percentage for Maximum risk //-- enumeration for dealing with account balance/free-margin enum RiskMedium { BALANCE, MARGIN }; //-- calculations for Risk options (PERCENTAGE OF BALANCE and PERCENTAGE OF FREE-MARGIN) double RiskProfile1(const RiskMedium R_Medium); //-- calculations for Risk options (AMOUNT PER BALANCE and AMOUNT PER FREE-MARGIN) double RiskProfile2(const RiskMedium R_Medium); //-- calculations for Risk options (LOTSIZE PER BALANCE and LOTSIZE PER FREE-MARGIN) double RiskProfile3(const RiskMedium R_Medium); //-- calculations for Maximum allowable Risk double MaxRisk(const double percent); //-- Store Trade's Open-price double OpenPrice; //-- Store Trade's Close-price double ClosePrice; //-- Store Ordertype between (ORDER_TYPE_BUY or ORDER_TYPE_SELL) for risk calaculations ENUM_ORDER_TYPE ORDERTYPE; //-- Set Medium variable value void SetMedium(const RiskMedium R_Medium) {Medium = (R_Medium==BALANCE)?Account.Balance():Account.FreeMargin();} //-- Get Minimum Risk for a Trade using Minimum Lot-size bool GetMinimumRisk() { return OrderCalcProfit(ORDERTYPE,Symbol(),LotsMin(),OpenPrice,ClosePrice,MinimumAmount); } //-- Retrieve Risk amount based on Risk inputs double GetRisk(double Amount) { if(!GetMinimumRisk()||Amount==0) return 0.0; return ((Amount/MinimumAmount)*LotsMin()); } protected: //-- Application of Lot-size limits void ValidateLotsize(double &Lotsize); //-- Set ORDERTYPE variable to (ORDER_TYPE_BUY or ORDER_TYPE_SELL) respectively void SetOrderType(ENUM_ORDER_TYPE Type) { if(Type==ORDER_TYPE_BUY||Type==ORDER_TYPE_BUY_LIMIT||Type==ORDER_TYPE_BUY_STOP) { ORDERTYPE = ORDER_TYPE_BUY; } else if(Type==ORDER_TYPE_SELL||Type==ORDER_TYPE_SELL_LIMIT||Type==ORDER_TYPE_SELL_STOP) { ORDERTYPE = ORDER_TYPE_SELL; } } public: CRiskManagement();//Class's constructor //-- Retrieve user's Risk option string GetRiskOption() { switch(RiskProfileOption) { case MINIMUM_LOT://MINIMUM LOTSIZE - Risk Option return "MINIMUM LOTSIZE"; break; case MAXIMUM_LOT://MAXIMUM LOTSIZE - Risk Option return "MAXIMUM LOTSIZE"; break; case PERCENTAGE_OF_BALANCE://PERCENTAGE OF BALANCE - Risk Option return "PERCENTAGE OF BALANCE"; break; case PERCENTAGE_OF_FREEMARGIN://PERCENTAGE OF FREE-MARGIN - Risk Option return "PERCENTAGE OF FREE-MARGIN"; break; case AMOUNT_PER_BALANCE://AMOUNT PER BALANCE - Risk Option return "AMOUNT PER BALANCE"; break; case AMOUNT_PER_FREEMARGIN://AMOUNT PER FREE-MARGIN - Risk Option return "AMOUNT PER FREE-MARGIN"; break; case LOTSIZE_PER_BALANCE://LOTSIZE PER BALANCE - Risk Option return "LOTSIZE PER BALANCE"; break; case LOTSIZE_PER_FREEMARGIN://LOTSIZE PER FREE-MARGIN - Risk Option return "LOTSIZE PER FREE-MARGIN"; break; case CUSTOM_LOT://CUSTOM LOTSIZE - Risk Option return "CUSTOM LOTSIZE"; break; case PERCENTAGE_OF_MAXRISK://PERCENTAGE OF MAX-RISK - Risk Option return "PERCENTAGE OF MAX-RISK"; break; default: return ""; break; } } //-- Retrieve user's Risk Floor Option string GetRiskFloor() { switch(RiskFloorOption) { case RiskFloorMin://MINIMUM LOTSIZE for Risk floor options return "MINIMUM LOTSIZE"; break; case RiskFloorMax://MAX-RISK for Risk floor options return "MAX-RISK"; break; case RiskFloorNone://NONE for Risk floor options return "NONE"; break; default: return ""; break; } } //-- Retrieve user's Risk Ceiling option string GetRiskCeil() { switch(RiskCeilOption) { case RiskCeilMax://MAX LOTSIZE for Risk ceiling options return "MAX LOTSIZE"; break; case RiskCeilMax2://MAX LOTSIZE(x2) for Risk ceiling options return "MAX LOTSIZE(x2)"; break; case RiskCeilMax3://MAX LOTSIZE(x3) for Risk ceiling options return "MAX LOTSIZE(x3)"; break; case RiskCeilMax4://MAX LOTSIZE(x4) for Risk ceiling options return "MAX LOTSIZE(x4)"; break; case RiskCeilMax5://MAX LOTSIZE(x5) for Risk ceiling options return "MAX LOTSIZE(x5)"; break; default: return ""; break; } } double Volume();//Get risk in Volume //Apply fixes to lot-size where applicable void NormalizeLotsize(double &Lotsize); }; //+------------------------------------------------------------------+ //|Constructor | //+------------------------------------------------------------------+ //Initialize values CRiskManagement::CRiskManagement(void):Lots(0.0),max_percent(100), ORDERTYPE(ORDER_TYPE_BUY),OpenPrice(Ask()), ClosePrice(NormalizePrice(Ask()+Ask()*0.01)) { } //+------------------------------------------------------------------+ //|Get risk in Volume | //+------------------------------------------------------------------+ double CRiskManagement::Volume() { switch(RiskProfileOption) { case MINIMUM_LOT://MINIMUM LOTSIZE - Risk Option return LotsMin(); break; case MAXIMUM_LOT://MAXIMUM LOTSIZE - Risk Option Lots = LotsMax(); break; case PERCENTAGE_OF_BALANCE://PERCENTAGE OF BALANCE - Risk Option Lots = RiskProfile1(BALANCE); break; case PERCENTAGE_OF_FREEMARGIN://PERCENTAGE OF FREE-MARGIN - Risk Option Lots = RiskProfile1(MARGIN); break; case AMOUNT_PER_BALANCE://AMOUNT PER BALANCE - Risk Option Lots = RiskProfile2(BALANCE); break; case AMOUNT_PER_FREEMARGIN://AMOUNT PER FREE-MARGIN - Risk Option Lots = RiskProfile2(MARGIN); break; case LOTSIZE_PER_BALANCE://LOTSIZE PER BALANCE - Risk Option Lots = RiskProfile3(BALANCE); break; case LOTSIZE_PER_FREEMARGIN://LOTSIZE PER FREE-MARGIN - Risk Option Lots = RiskProfile3(MARGIN); break; case CUSTOM_LOT://CUSTOM LOTSIZE - Risk Option Lots = Risk_Profile_4; break; case PERCENTAGE_OF_MAXRISK://PERCENTAGE OF MAX-RISK - Risk Option Lots = MaxRisk(Risk_Profile_5); break; default: Lots = 0.0; break; } ValidateLotsize(Lots);//Check/Adjust Lotsize Limits NormalizeLotsize(Lots);//Normalize Lotsize return Lots; } //+------------------------------------------------------------------+ //|calculations for Risk options | //|(PERCENTAGE OF BALANCE and PERCENTAGE OF FREE-MARGIN) | //+------------------------------------------------------------------+ //-- calculations for Risk options (PERCENTAGE OF BALANCE and PERCENTAGE OF FREE-MARGIN) double CRiskManagement::RiskProfile1(const RiskMedium R_Medium) { SetMedium(R_Medium); RiskAmount = Medium*(Risk_Profile_1/100); return GetRisk(RiskAmount); } //+------------------------------------------------------------------+ //|calculations for Risk options | //|(AMOUNT PER BALANCE and AMOUNT PER FREE-MARGIN) | //+------------------------------------------------------------------+ //-- calculations for Risk options (AMOUNT PER BALANCE and AMOUNT PER FREE-MARGIN) double CRiskManagement::RiskProfile2(const RiskMedium R_Medium) { SetMedium(R_Medium); double risk = (Risk_Profile_2.RiskAmountBoF/Risk_Profile_2.RiskAmount); risk = (risk<1)?1:risk; if(Medium<=0) return 0.0; RiskAmount = Medium/risk; return GetRisk(RiskAmount); } //+------------------------------------------------------------------+ //|calculations for Risk options | //|(LOTSIZE PER BALANCE and LOTSIZE PER FREE-MARGIN) | //+------------------------------------------------------------------+ //-- calculations for Risk options (LOTSIZE PER BALANCE and LOTSIZE PER FREE-MARGIN) double CRiskManagement::RiskProfile3(const RiskMedium R_Medium) { SetMedium(R_Medium); return (Medium>0)?((Medium/Risk_Profile_3.RiskLotBoF)*Risk_Profile_3.RiskLot):0.0; } //+------------------------------------------------------------------+ //|calculations for Maximum allowable Risk | //+------------------------------------------------------------------+ //-- calculations for Maximum allowable Risk double CRiskManagement::MaxRisk(const double percent) { double margin=0.0,max_risk=0.0; //--- checks if(percent<0.01 || percent>100) { Print(__FUNCTION__," invalid parameters"); return(0.0); } //--- calculate margin requirements for 1 lot if(!OrderCalcMargin(ORDERTYPE,Symbol(),1.0,OpenPrice,margin) || margin<0.0) { Print(__FUNCTION__," margin calculation failed"); return(0.0); } //--- calculate maximum volume max_risk=Account.FreeMargin()*(percent/100.0)/margin; //--- return volume return(max_risk); } //+------------------------------------------------------------------+ //|Apply fixes to lot-size where applicable | //+------------------------------------------------------------------+ void CRiskManagement::NormalizeLotsize(double &Lotsize) { if(Lotsize<=0.0) return; //-- Check if the is a Volume limit for the current Symbol if(LotsLimit()>0.0) { if((Lots+PositionsVolume()+OrdersVolume())>LotsLimit()) { //-- calculation of available lotsize remaining double remaining_avail_lots = (LotsLimit()-(PositionsVolume()+OrdersVolume())); if(remaining_avail_lots>=LotsMin()) { if(RiskFloorOption==RiskFloorMin)//Check if Risk floor option is MINIMUM LOTSIZE { Print("Warning: Volume Limit Reached, minimum Lotsize selected."); Lotsize = LotsMin(); } else if(RiskFloorOption==RiskFloorMax)//Check if Risk floor option is MAX-RISK { Print("Warning: Volume Limit Reached, Lotsize Reduced."); Lotsize = ((remaining_avail_lots*(RiskFloorPercentage/100))>LotsMin())? (remaining_avail_lots*(RiskFloorPercentage/100)):LotsMin(); } } else { Print("Volume Limit Reached!"); Lotsize=0.0; return; } } } //Check if there is a valid Volume Step for the current Symbol if(LotsStep()>0.0) Lotsize=LotsStep()*MathFloor(Lotsize/LotsStep()); } //+------------------------------------------------------------------+ //|Application of Lot-size limits | //+------------------------------------------------------------------+ void CRiskManagement::ValidateLotsize(double &Lotsize) { switch(RiskFloorOption) { case RiskFloorMin://MINIMUM LOTSIZE for Risk floor options //-- Check if lot-size is not less than Minimum lot or more than maximum allowable risk if(Lotsize<LotsMin()||Lotsize>MaxRisk(max_percent)) { Lotsize=LotsMin(); } break; case RiskFloorMax://MAX-RISK for Risk floor options //-- Check if lot-size is more the maximum allowable risk if(Lotsize>MaxRisk(max_percent)) { Lotsize=(MaxRisk(RiskFloorPercentage)>LotsMin())?MaxRisk(RiskFloorPercentage):LotsMin(); } else if(Lotsize<LotsMin())//Check if lot-size is less than Minimum lot { Lotsize=LotsMin(); } break; case RiskFloorNone://NONE for Risk floor options //Check if lot-size is less than Minimum lot if(Lotsize<LotsMin()) { Lotsize=0.0; } break; default: Lotsize=0.0; break; } switch(RiskCeilOption) { case RiskCeilMax://MAX LOTSIZE for Risk ceiling options //Check if lot-size is more than Maximum lot if(Lotsize>LotsMax()) Lotsize=LotsMax(); break; case RiskCeilMax2://MAX LOTSIZE(x2) for Risk ceiling options //Check if lot-size is more than Maximum lot times two if(Lotsize>(LotsMax()*2)) Lotsize=(LotsMax()*2); break; case RiskCeilMax3://MAX LOTSIZE(x3) for Risk ceiling options //Check if lot-size is more than Maximum lot times three if(Lotsize>(LotsMax()*3)) Lotsize=(LotsMax()*3); break; case RiskCeilMax4://MAX LOTSIZE(x4) for Risk ceiling options //Check if lot-size is more than Maximum lot times four if(Lotsize>(LotsMax()*4)) Lotsize=(LotsMax()*4); break; case RiskCeilMax5://MAX LOTSIZE(x5) for Risk ceiling options //Check if lot-size is more than Maximum lot times five if(Lotsize>(LotsMax()*5)) Lotsize=(LotsMax()*5); break; default: break; } } //+------------------------------------------------------------------+
类型为枚举RiskFloor的变量RiskFloorOption将存储用户/交易者的的风险偏好选项,该选项将作为EA的输入。
//-- Enumeration declaration for Risk options enum RiskOptions { MINIMUM_LOT,//MINIMUM LOTSIZE MAXIMUM_LOT,//MAXIMUM LOTSIZE PERCENTAGE_OF_BALANCE,//PERCENTAGE OF BALANCE PERCENTAGE_OF_FREEMARGIN,//PERCENTAGE OF FREE-MARGIN AMOUNT_PER_BALANCE,//AMOUNT PER BALANCE AMOUNT_PER_FREEMARGIN,//AMOUNT PER FREE-MARGIN LOTSIZE_PER_BALANCE,//LOTSIZE PER BALANCE LOTSIZE_PER_FREEMARGIN,//LOTSIZE PER FREE-MARGIN CUSTOM_LOT,//CUSTOM LOTSIZE PERCENTAGE_OF_MAXRISK//PERCENTAGE OF MAX-RISK } RiskProfileOption;//variable for Risk options
类型为枚举RiskFloor的变量RiskFloorOption将存储用户/交易者的最小风险偏好,该偏好将作为EA的输入。
//-- Enumeration declaration for Risk floor enum RiskFloor { RiskFloorMin,//MINIMUM LOTSIZE RiskFloorMax,//MAX-RISK RiskFloorNone//NONE } RiskFloorOption;//variable for Risk floor
类型为枚举 RiskCeil 的变量RiskCeilOption将存储用户/交易者的最大风险偏好,该偏好将作为EA的输入信息。
//-- Enumeration declaration for Risk ceiling(Maximum allowable risk in terms of lot-size) enum RiskCeil { RiskCeilMax,//MAX LOTSIZE RiskCeilMax2,//MAX LOTSIZE(x2) RiskCeilMax3,//MAX LOTSIZE(x3) RiskCeilMax4,//MAX LOTSIZE(x4) RiskCeilMax5,//MAX LOTSIZE(x5) } RiskCeilOption;//variable for Risk ceiling
用户/交易者的(账户余额或可用保证金)将存储在双精度浮点变量 RiskAmountBoF 中,而另一个双精度浮点变量 RiskAmount 将用于存储风险金额。Risk_Profile_2 将用于存储风险配置文件的“各余额金额”和“各可用保证金金额”属性。
//-- Structure declaration for Risk options (AMOUNT PER BALANCE and AMOUNT PER FREE-MARGIN) struct RISK_AMOUNT { double RiskAmountBoF;//store Balance or Free-Margin double RiskAmount;//store risk amount } Risk_Profile_2;//variable for Risk options (AMOUNT PER BALANCE and AMOUNT PER FREE-MARGIN)
类型为结构体 RISK_LOT的变量 Risk_Profile_3将用于存储风险配置文件的“各余额手数”和“各可用保证金手数”属性。
//-- Structure declaration for Risk options (LOTSIZE PER BALANCE and LOTSIZE PER FREE-MARGIN) struct RISK_LOT { double RiskLotBoF;//store Balance or Free-Margin double RiskLot;//store lot-size } Risk_Profile_3;//variable for Risk options (LOTSIZE PER BALANCE and LOTSIZE PER FREE-MARGIN)
变量RiskFloorPercentage将存储Riskfloor选项中的RiskFloorMax最大风险百分比。
double RiskFloorPercentage;//variable for RiskFloorMax
变量Risk_Profile_1将存储风险选项的百分比,即账户百分比或可用保证金百分比。
double Risk_Profile_1;//variable for Risk options (PERCENTAGE OF BALANCE and PERCENTAGE OF FREE-MARGIN)
变量Risk_Profile_4将存储风险选项CUSTOM LOTSIZE即自定义手数值。
double Risk_Profile_4;//variable for Risk option (CUSTOM LOTSIZE)
变量Risk_Profile_5将存储风险选项PERCENTAGE OF MAX-RISK即最大风险百分比。
double Risk_Profile_5;//variable for Risk option (PERCENTAGE OF MAX-RISK)
在函数 GetRiskOption中,我们将以字符串数据类型检索用户/交易者的风险选项。
//-- Retrieve user's Risk option string GetRiskOption() { switch(RiskProfileOption) { case MINIMUM_LOT://MINIMUM LOTSIZE - Risk Option return "MINIMUM LOTSIZE"; break; case MAXIMUM_LOT://MAXIMUM LOTSIZE - Risk Option return "MAXIMUM LOTSIZE"; break; case PERCENTAGE_OF_BALANCE://PERCENTAGE OF BALANCE - Risk Option return "PERCENTAGE OF BALANCE"; break; case PERCENTAGE_OF_FREEMARGIN://PERCENTAGE OF FREE-MARGIN - Risk Option return "PERCENTAGE OF FREE-MARGIN"; break; case AMOUNT_PER_BALANCE://AMOUNT PER BALANCE - Risk Option return "AMOUNT PER BALANCE"; break; case AMOUNT_PER_FREEMARGIN://AMOUNT PER FREE-MARGIN - Risk Option return "AMOUNT PER FREE-MARGIN"; break; case LOTSIZE_PER_BALANCE://LOTSIZE PER BALANCE - Risk Option return "LOTSIZE PER BALANCE"; break; case LOTSIZE_PER_FREEMARGIN://LOTSIZE PER FREE-MARGIN - Risk Option return "LOTSIZE PER FREE-MARGIN"; break; case CUSTOM_LOT://CUSTOM LOTSIZE - Risk Option return "CUSTOM LOTSIZE"; break; case PERCENTAGE_OF_MAXRISK://PERCENTAGE OF MAX-RISK - Risk Option return "PERCENTAGE OF MAX-RISK"; break; default: return ""; break; } }
在函数GetRiskFloor中,我们将以字符串数据类型检索用户/交易者的风险下限选项。
//-- Retrieve user's Risk Floor Option string GetRiskFloor() { switch(RiskFloorOption) { case RiskFloorMin://MINIMUM LOTSIZE for Risk floor options return "MINIMUM LOTSIZE"; break; case RiskFloorMax://MAX-RISK for Risk floor options return "MAX-RISK"; break; case RiskFloorNone://NONE for Risk floor options return "NONE"; break; default: return ""; break; } }
在函数GetRiskCeil中,我们将以字符串数据类型检索用户/交易者的风险上限选项。
//-- Retrieve user's Risk Ceiling option string GetRiskCeil() { switch(RiskCeilOption) { case RiskCeilMax://MAX LOTSIZE for Risk ceiling options return "MAX LOTSIZE"; break; case RiskCeilMax2://MAX LOTSIZE(x2) for Risk ceiling options return "MAX LOTSIZE(x2)"; break; case RiskCeilMax3://MAX LOTSIZE(x3) for Risk ceiling options return "MAX LOTSIZE(x3)"; break; case RiskCeilMax4://MAX LOTSIZE(x4) for Risk ceiling options return "MAX LOTSIZE(x4)"; break; case RiskCeilMax5://MAX LOTSIZE(x5) for Risk ceiling options return "MAX LOTSIZE(x5)"; break; default: return ""; break; } }
在风险管理类的构造函数中,我们将使用默认值初始化之前声明的变量。变量ORDERTYPE的默认值是ORDER_TYPE_BUY,因此对于需要订单类型来计算风险的风险选项,该变量将设置订单类型,并用于模拟开仓交易的风险计算。默认的开仓价格将存储在变量OpenPrice中,并且该价格将是我们ORDERTYPE交易品种的买入价(Ask price)。默认的平仓价格将是在报价基础上上浮1%的价格,并存储在变量ClosePrice中。
//Initialize values CRiskManagement::CRiskManagement(void):Lots(0.0),max_percent(100), ORDERTYPE(ORDER_TYPE_BUY),OpenPrice(Ask()), ClosePrice(NormalizePrice(Ask()+Ask()*0.01)) { }
该函数 Volume将根据用户/交易者的风险配置选项来获取手数,并根据用户/交易者所选的风险上限(Risk Ceiling)和风险下限(Risk Floor)选项来调整从所选风险配置中获取的手数大小。
之后,手数将被标准化,以便可以使用特定的手数实际开仓交易。
double CRiskManagement::Volume() { switch(RiskProfileOption) { case MINIMUM_LOT://MINIMUM LOTSIZE - Risk Option return LotsMin(); break; case MAXIMUM_LOT://MAXIMUM LOTSIZE - Risk Option Lots = LotsMax(); break; case PERCENTAGE_OF_BALANCE://PERCENTAGE OF BALANCE - Risk Option Lots = RiskProfile1(BALANCE); break; case PERCENTAGE_OF_FREEMARGIN://PERCENTAGE OF FREE-MARGIN - Risk Option Lots = RiskProfile1(MARGIN); break; case AMOUNT_PER_BALANCE://AMOUNT PER BALANCE - Risk Option Lots = RiskProfile2(BALANCE); break; case AMOUNT_PER_FREEMARGIN://AMOUNT PER FREE-MARGIN - Risk Option Lots = RiskProfile2(MARGIN); break; case LOTSIZE_PER_BALANCE://LOTSIZE PER BALANCE - Risk Option Lots = RiskProfile3(BALANCE); break; case LOTSIZE_PER_FREEMARGIN://LOTSIZE PER FREE-MARGIN - Risk Option Lots = RiskProfile3(MARGIN); break; case CUSTOM_LOT://CUSTOM LOTSIZE - Risk Option Lots = Risk_Profile_4; break; case PERCENTAGE_OF_MAXRISK://PERCENTAGE OF MAX-RISK - Risk Option Lots = MaxRisk(Risk_Profile_5); break; default: Lots = 0.0; break; } ValidateLotsize(Lots);//Check/Adjust Lotsize Limits NormalizeLotsize(Lots);//Normalize Lotsize return Lots; }
函数SetMedium将根据枚举变量R_Medium的值,将双精度变量Medium赋值为用户/交易者的账户余额或账户可用保证金。
//-- Set Medium variable value void SetMedium(const RiskMedium R_Medium) {Medium = (R_Medium==BALANCE)?Account.Balance():Account.FreeMargin();}
函数GetMinimumRisk将计算具有最小手数的特定交易相对应的最小风险,并将该值赋给变量MinimumAmount。
//-- Get Minimum Risk for a Trade using Minimum Lot-size bool GetMinimumRisk() { return OrderCalcProfit(ORDERTYPE,Symbol(),LotsMin(),OpenPrice,ClosePrice,MinimumAmount); }
函数GetRisk将根据参数变量Amount(指定的风险金额)来获取所需的手数。当MinimumAmount(最小风险金额)确定后,Amount将被除以MinimumAmount,得到一个商,然后将这个商与minimum lot-size(最小手数)相乘,从而得到对应Amount所需的手数。
//-- Retrieve Risk amount based on Risk inputs double GetRisk(double Amount) { if(!GetMinimumRisk()||Amount==0) return 0.0; return ((Amount/MinimumAmount)*LotsMin()); }
在函数RiskProfile1中,我们计算并返回针对风险选项“账户余额百分比”或“可用保证金百分比”的手数大小。
//-- calculations for Risk options (PERCENTAGE OF BALANCE and PERCENTAGE OF FREE-MARGIN) double CRiskManagement::RiskProfile1(const RiskMedium R_Medium) { SetMedium(R_Medium); RiskAmount = Medium*(Risk_Profile_1/100); return GetRisk(RiskAmount); }
在函数RiskProfile2中,我们计算并返回基于风险选项“按余额的金额”或“按可用保证金的金额”的手数大小。
//-- calculations for Risk options (AMOUNT PER BALANCE and AMOUNT PER FREE-MARGIN) double CRiskManagement::RiskProfile2(const RiskMedium R_Medium) { SetMedium(R_Medium); double risk = (Risk_Profile_2.RiskAmountBoF/Risk_Profile_2.RiskAmount); risk = (risk<1)?1:risk; if(Medium<=0) return 0.0; RiskAmount = Medium/risk; return GetRisk(RiskAmount); }
在函数RiskProfile3中,我们计算并返回基于风险选项“按余额的手数大小”或“按可用保证金的手数大小”的相应值。
//-- calculations for Risk options (LOTSIZE PER BALANCE and LOTSIZE PER FREE-MARGIN) double CRiskManagement::RiskProfile3(const RiskMedium R_Medium) { SetMedium(R_Medium); return (Medium>0)?((Medium/Risk_Profile_3.RiskLotBoF)*Risk_Profile_3.RiskLot):0.0; }
在函数ValidateLotsize中,对通过索引传递的变量Lotsize进行了调整。
在第一个Switch表达式RiskFloorOption中:
- 在RiskFloorMin的情况下:我们检查变量Lotsize是否超出了其限制范围,如果是,则将其设置为当前交易品种的最小手数。我们检查下限,即变量值是否小于最小手数。上限是当Lotsize变量超过可能承担的最大风险时。
- 在RiskFloorMax的情况下:我们首先检查Lotsize变量是否超过了可能承担的最大风险,如果超过,则进一步检查所需的最大最小风险是否大于最小手数,如果大于,则将该交易最大期望下的最小风险值赋给Lotsize,否则赋值为最小手数。如果Lotsize最初小于可能承担的最大风险且小于最小手数,则我们将其赋值为最小手数。
void CRiskManagement::ValidateLotsize(double &Lotsize) { switch(RiskFloorOption) { case RiskFloorMin://MINIMUM LOTSIZE for Risk floor options //-- Check if lot-size is not less than Minimum lot or more than maximum allowable risk if(Lotsize<LotsMin()||Lotsize>MaxRisk(max_percent)) { Lotsize=LotsMin(); } break; case RiskFloorMax://MAX-RISK for Risk floor options //-- Check if lot-size is more the maximum allowable risk if(Lotsize>MaxRisk(max_percent)) { Lotsize=(MaxRisk(RiskFloorPercentage)>LotsMin())?MaxRisk(RiskFloorPercentage):LotsMin(); } else if(Lotsize<LotsMin())//Check if lot-size is less than Minimum lot { Lotsize=LotsMin(); } break; case RiskFloorNone://NONE for Risk floor options //Check if lot-size is less than Minimum lot if(Lotsize<LotsMin()) { Lotsize=0.0; } break; default: Lotsize=0.0; break; } switch(RiskCeilOption) { case RiskCeilMax://MAX LOTSIZE for Risk ceiling options //Check if lot-size is more than Maximum lot if(Lotsize>LotsMax()) Lotsize=LotsMax(); break; case RiskCeilMax2://MAX LOTSIZE(x2) for Risk ceiling options //Check if lot-size is more than Maximum lot times two if(Lotsize>(LotsMax()*2)) Lotsize=(LotsMax()*2); break; case RiskCeilMax3://MAX LOTSIZE(x3) for Risk ceiling options //Check if lot-size is more than Maximum lot times three if(Lotsize>(LotsMax()*3)) Lotsize=(LotsMax()*3); break; case RiskCeilMax4://MAX LOTSIZE(x4) for Risk ceiling options //Check if lot-size is more than Maximum lot times four if(Lotsize>(LotsMax()*4)) Lotsize=(LotsMax()*4); break; case RiskCeilMax5://MAX LOTSIZE(x5) for Risk ceiling options //Check if lot-size is more than Maximum lot times five if(Lotsize>(LotsMax()*5)) Lotsize=(LotsMax()*5); break; default: break; } }
在函数NormalizeLotsize中,其目的是检查手数大小是否在交易品种的交易量限制内,并且手数大小是否符合交易量步长。
如果手数大小违反了交易品种的交易量限制,我们则计算达到交易量限制之前剩余可用的手数。之后,我们检查剩余的手数大小是否大于或等于当前交易品种的最小手数。
- RiskFloorMin:我们将Lotsize变量设置为最小手数大小。
- RiskFloorMax:如果剩余手数大小的RiskFloorPercentage(风险下限百分比)大于最小手数,我们将Lotsize变量设置为这个值。如果剩余手数大小的RiskFloorPercentage小于或等于最小手数,我们将Lotsize变量设置为最小手数。
void CRiskManagement::NormalizeLotsize(double &Lotsize) { if(Lotsize<=0.0) return; //-- Check if the is a Volume limit for the current Symbol if(LotsLimit()>0.0) { if((Lots+PositionsVolume()+OrdersVolume())>LotsLimit()) { //-- calculation of available lotsize remaining double remaining_avail_lots = (LotsLimit()-(PositionsVolume()+OrdersVolume())); if(remaining_avail_lots>=LotsMin()) { if(RiskFloorOption==RiskFloorMin)//Check if Risk floor option is MINIMUM LOTSIZE { Print("Warning: Volume Limit Reached, minimum Lotsize selected."); Lotsize = LotsMin(); } else if(RiskFloorOption==RiskFloorMax)//Check if Risk floor option is MAX-RISK { Print("Warning: Volume Limit Reached, Lotsize Reduced."); Lotsize = ((remaining_avail_lots*(RiskFloorPercentage/100))>LotsMin())? (remaining_avail_lots*(RiskFloorPercentage/100)):LotsMin(); } } else { Print("Volume Limit Reached!"); Lotsize=0.0; return; } } } //Check if there is a valid Volume Step for the current Symbol if(LotsStep()>0.0) Lotsize=LotsStep()*MathFloor(Lotsize/LotsStep()); }
通用图形类
通用图形类将展示当前交易品种的一般属性以及交易者设置的一些风险选项。
CommonGraphics 类从多个级别的类继承而来:
- CObjectProperties
- CChartProperties
- CSymbolProperties
CommonGraphics包含来自CRiskManagement类的某些功能或属性。
CommonGraphics类从多个类继承了层次化的结构和功能:
- CSymbolProperties
- CSymbolInfo
//+------------------------------------------------------------------+ //| NewsTrading | //| Copyright 2024, MetaQuotes Ltd. | //| https://www.mql5.com/en/users/kaaiblo | //+------------------------------------------------------------------+ #include "ObjectProperties.mqh" #include "RiskManagement.mqh" //+------------------------------------------------------------------+ //|CommonGraphics class | //+------------------------------------------------------------------+ class CCommonGraphics:CObjectProperties { private: CRiskManagement CRisk;//Risk management class object public: CCommonGraphics(void);//class constructor ~CCommonGraphics(void) {}//class destructor void GraphicsRefresh();//will create the chart objects }; //+------------------------------------------------------------------+ //|Constructor | //+------------------------------------------------------------------+ CCommonGraphics::CCommonGraphics(void) { GraphicsRefresh();//calling GraphicsRefresh function } //+------------------------------------------------------------------+ //|Specify Chart Objects | //+------------------------------------------------------------------+ void CCommonGraphics::GraphicsRefresh() { //-- Will create the rectangle object Square(0,"Symbol Properties",2,20,330,183,ANCHOR_LEFT_UPPER); //-- Will create the text object for the Symbol's name TextObj(0,"Symbol Name",Symbol(),5,23); //-- Will create the text object for the contract size TextObj(0,"Symbol Contract Size","Contract Size: "+string(ContractSize()),5,40,CORNER_LEFT_UPPER,9); //-- Will create the text object for the Symbol's Minimum lotsize TextObj(0,"Symbol MinLot","Minimum Lot: "+string(LotsMin()),5,60,CORNER_LEFT_UPPER,9); //-- Will create the text object for the Symbol's Maximum lotsize TextObj(0,"Symbol MaxLot","Max Lot: "+string(LotsMax()),5,80,CORNER_LEFT_UPPER,9); //-- Will create the text object for the Symbol's Volume Step TextObj(0,"Symbol Volume Step","Volume Step: "+string(LotsStep()),5,100,CORNER_LEFT_UPPER,9); //-- Will create the text object for the Symbol's Volume Limit TextObj(0,"Symbol Volume Limit","Volume Limit: "+string(LotsLimit()),5,120,CORNER_LEFT_UPPER,9); //-- Will create the text object for the trader's Risk Option TextObj(0,"Risk Option","Risk Option: "+CRisk.GetRiskOption(),5,140,CORNER_LEFT_UPPER,9); //-- Will create the text object for the trader's Risk Floor TextObj(0,"Risk Floor","Risk Floor: "+CRisk.GetRiskFloor(),5,160,CORNER_LEFT_UPPER,9); //-- Will create the text object for the trader's Risk Ceiling TextObj(0,"Risk Ceil","Risk Ceiling: "+CRisk.GetRiskCeil(),5,180,CORNER_LEFT_UPPER,9); } //+------------------------------------------------------------------+
在函数GraphicsFresfresh中,我们设置了图形图表对象的属性。
void CCommonGraphics::GraphicsRefresh() { //-- Will create the rectangle object Square(0,"Symbol Properties",2,20,330,183,ANCHOR_LEFT_UPPER); //-- Will create the text object for the Symbol's name TextObj(0,"Symbol Name",Symbol(),5,23); //-- Will create the text object for the contract size TextObj(0,"Symbol Contract Size","Contract Size: "+string(ContractSize()),5,40,CORNER_LEFT_UPPER,9); //-- Will create the text object for the Symbol's Minimum lotsize TextObj(0,"Symbol MinLot","Minimum Lot: "+string(LotsMin()),5,60,CORNER_LEFT_UPPER,9); //-- Will create the text object for the Symbol's Maximum lotsize TextObj(0,"Symbol MaxLot","Max Lot: "+string(LotsMax()),5,80,CORNER_LEFT_UPPER,9); //-- Will create the text object for the Symbol's Volume Step TextObj(0,"Symbol Volume Step","Volume Step: "+string(LotsStep()),5,100,CORNER_LEFT_UPPER,9); //-- Will create the text object for the Symbol's Volume Limit TextObj(0,"Symbol Volume Limit","Volume Limit: "+string(LotsLimit()),5,120,CORNER_LEFT_UPPER,9); //-- Will create the text object for the trader's Risk Option TextObj(0,"Risk Option","Risk Option: "+CRisk.GetRiskOption(),5,140,CORNER_LEFT_UPPER,9); //-- Will create the text object for the trader's Risk Floor TextObj(0,"Risk Floor","Risk Floor: "+CRisk.GetRiskFloor(),5,160,CORNER_LEFT_UPPER,9); //-- Will create the text object for the trader's Risk Ceiling TextObj(0,"Risk Ceil","Risk Ceiling: "+CRisk.GetRiskCeil(),5,180,CORNER_LEFT_UPPER,9); }
EA:
到此,我们将再次不进行任何交易。//+------------------------------------------------------------------+ //| NewsTrading | //| Copyright 2024, MetaQuotes Ltd. | //| https://www.mql5.com/en/users/kaaiblo | //+------------------------------------------------------------------+ //--- width and height of the canvas (used for drawing) #define IMG_WIDTH 200 #define IMG_HEIGHT 100 //--- enable to set color format ENUM_COLOR_FORMAT clr_format=COLOR_FORMAT_XRGB_NOALPHA; //--- drawing array (buffer) uint ExtImg[IMG_WIDTH*IMG_HEIGHT]; #include "News.mqh" CNews NewsObject;//Class CNews Object 'NewsObject' #include "TimeManagement.mqh" CTimeManagement CTM;//Class CTimeManagement Object 'CTM' #include "WorkingWithFolders.mqh" CFolders Folder();//Calling Class's Constructor #include "ChartProperties.mqh" CChartProperties CChart;//Class CChartProperties Object 'CChart' #include "RiskManagement.mqh" CRiskManagement CRisk;//Class CRiskManagement Object 'CRisk' #include "CommonGraphics.mqh" CCommonGraphics CGraphics();//Calling Class's Constructor enum iSeparator { Delimiter//__________________________ }; sinput group "+--------| RISK MANAGEMENT |--------+"; input RiskOptions RISK_Type=MINIMUM_LOT;//SELECT RISK OPTION input RiskFloor RISK_Mini=RiskFloorMin;//RISK FLOOR input double RISK_Mini_Percent=75;//MAX-RISK [100<-->0.01]% input RiskCeil RISK_Maxi=RiskCeilMax;//RISK CEILING sinput iSeparator iRisk_1=Delimiter;//__________________________ sinput iSeparator iRisk_1L=Delimiter;//PERCENTAGE OF [BALANCE | FREE-MARGIN] input double Risk_1_PERCENTAGE=3;//[100<-->0.01]% sinput iSeparator iRisk_2=Delimiter;//__________________________ sinput iSeparator iRisk_2L=Delimiter;//AMOUNT PER [BALANCE | FREE-MARGIN] input double Risk_2_VALUE=1000;//[BALANCE | FREE-MARGIN] input double Risk_2_AMOUNT=10;//EACH AMOUNT sinput iSeparator iRisk_3=Delimiter;//__________________________ sinput iSeparator iRisk_3L=Delimiter;//LOTSIZE PER [BALANCE | FREE-MARGIN] input double Risk_3_VALUE=1000;//[BALANCE | FREE-MARGIN] input double Risk_3_LOTSIZE=0.1;//EACH LOTS(VOLUME) sinput iSeparator iRisk_4=Delimiter;//__________________________ sinput iSeparator iRisk_4L=Delimiter;//CUSTOM LOTSIZE input double Risk_4_LOTSIZE=0.01;//LOTS(VOLUME) sinput iSeparator iRisk_5=Delimiter;//__________________________ sinput iSeparator iRisk_5L=Delimiter;//PERCENTAGE OF MAX-RISK input double Risk_5_PERCENTAGE=1;//[100<-->0.01]% //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- //Initializing CRiskManagement variable for Risk options RiskProfileOption = RISK_Type; //Initializing CRiskManagement variable for Risk floor RiskFloorOption = RISK_Mini; //Initializing CRiskManagement variable for RiskFloorMax RiskFloorPercentage = (RISK_Mini_Percent>100)?100: (RISK_Mini_Percent<0.01)?0.01:RISK_Mini_Percent;//Percentage cannot be more than 100% or less than 0.01% //Initializing CRiskManagement variable for Risk ceiling RiskCeilOption = RISK_Maxi; //Initializing CRiskManagement variable for Risk options (PERCENTAGE OF BALANCE and PERCENTAGE OF FREE-MARGIN) Risk_Profile_1 = (Risk_1_PERCENTAGE>100)?100: (Risk_1_PERCENTAGE<0.01)?0.01:Risk_1_PERCENTAGE;//Percentage cannot be more than 100% or less than 0.01% //Initializing CRiskManagement variables for Risk options (AMOUNT PER BALANCE and AMOUNT PER FREE-MARGIN) Risk_Profile_2.RiskAmountBoF = Risk_2_VALUE; Risk_Profile_2.RiskAmount = Risk_2_AMOUNT; //Initializing CRiskManagement variables for Risk options (LOTSIZE PER BALANCE and LOTSIZE PER FREE-MARGIN) Risk_Profile_3.RiskLotBoF = Risk_3_VALUE; Risk_Profile_3.RiskLot = Risk_3_LOTSIZE; //Initializing CRiskManagement variable for Risk option (CUSTOM LOTSIZE) Risk_Profile_4 = Risk_4_LOTSIZE; //Initializing CRiskManagement variable for Risk option (PERCENTAGE OF MAX-RISK) Risk_Profile_5 = (Risk_5_PERCENTAGE>100)?100: (Risk_5_PERCENTAGE<0.01)?0.01:Risk_5_PERCENTAGE;//Percentage cannot be more than 100% or less than 0.01% CChart.ChartRefresh();//Load chart configurations CGraphics.GraphicsRefresh();//-- Create/Re-create chart objects if(!MQLInfoInteger(MQL_TESTER))//Checks whether the program is in the strategy tester { //--- create OBJ_BITMAP_LABEL object for drawing ObjectCreate(0,"STATUS",OBJ_BITMAP_LABEL,0,0,0); ObjectSetInteger(0,"STATUS",OBJPROP_XDISTANCE,5); ObjectSetInteger(0,"STATUS",OBJPROP_YDISTANCE,22); //--- specify the name of the graphical resource ObjectSetString(0,"STATUS",OBJPROP_BMPFILE,"::PROGRESS"); uint w,h; // variables for receiving text string sizes uint x,y; // variables for calculation of the current coordinates of text string anchor points /* In the Do while loop below, the code will check if the terminal is connected to the internet. If the the program is stopped the loop will break, if the program is not stopped and the terminal is connected to the internet the function CreateEconomicDatabase will be called from the News.mqh header file's object called NewsObject and the loop will break once called. */ bool done=false; do { //--- clear the drawing buffer array ArrayFill(ExtImg,0,IMG_WIDTH*IMG_HEIGHT,0); if(!TerminalInfoInteger(TERMINAL_CONNECTED)) { //-- integer dots used as a loading animation static int dots=0; //--- set the font TextSetFont("Arial",-150,FW_EXTRABOLD,0); TextGetSize("Waiting",w,h);//get text width and height values //--- calculate the coordinates of the 'Waiting' text x=10;//horizontal alignment y=IMG_HEIGHT/2-(h/2);//alignment for the text to be centered vertically //--- output the 'Waiting' text to ExtImg[] buffer TextOut("Waiting",x,y,TA_LEFT|TA_TOP,ExtImg,IMG_WIDTH,IMG_HEIGHT,ColorToARGB(CChart.SymbolBackground()),clr_format); //--- calculate the coordinates for the dots after the 'Waiting' text x=w+13;//horizontal alignment y=IMG_HEIGHT/2-(h/2);//alignment for the text to be centered vertically TextSetFont("Arial",-160,FW_EXTRABOLD,0); //--- output of dots to ExtImg[] buffer TextOut(StringSubstr("...",0,dots),x,y,TA_LEFT|TA_TOP,ExtImg,IMG_WIDTH,IMG_HEIGHT,ColorToARGB(CChart.SymbolBackground()),clr_format); //--- update the graphical resource ResourceCreate("::PROGRESS",ExtImg,IMG_WIDTH,IMG_HEIGHT,0,0,IMG_WIDTH,clr_format); //--- force chart update ChartRedraw(); dots=(dots==3)?0:dots+1; //-- Notify user that program is waiting for connection Print("Waiting for connection..."); Sleep(500); continue; } else { //--- set the font TextSetFont("Arial",-120,FW_EXTRABOLD,0); TextGetSize("Getting Ready",w,h);//get text width and height values x=20;//horizontal alignment y=IMG_HEIGHT/2-(h/2);//alignment for the text to be centered vertically //--- output the text 'Getting Ready...' to ExtImg[] buffer TextOut("Getting Ready...",x,y,TA_LEFT|TA_TOP,ExtImg,IMG_WIDTH,IMG_HEIGHT,ColorToARGB(CChart.SymbolBackground()),clr_format); //--- update the graphical resource ResourceCreate("::PROGRESS",ExtImg,IMG_WIDTH,IMG_HEIGHT,0,0,IMG_WIDTH,clr_format); //--- force chart update ChartRedraw(); //-- Notify user that connection is successful Print("Connection Successful!"); NewsObject.CreateEconomicDatabase();//calling the database create function done=true; } } while(!done&&!IsStopped()); //-- Delete chart object ObjectDelete(0,"STATUS"); //-- force chart to update ChartRedraw(); } else { //Checks whether the database file exists if(!FileIsExist(NEWS_DATABASE_FILE,FILE_COMMON)) { Print("Necessary Files Do not Exist!"); Print("Run Program outside of the Strategy Tester"); Print("Necessary Files Should be Created First"); return(INIT_FAILED); } else { //Checks whether the lastest database date includes the time and date being tested datetime latestdate = CTM.TimePlusOffset(NewsObject.GetLatestNewsDate(),CTM.DaysS());//Day after the lastest recorded time in the database if(latestdate<TimeCurrent()) { Print("Necessary Files outdated!"); Print("To Update Files: Run Program outside of the Strategy Tester"); } Print("Database Dates End at: ",latestdate); PrintFormat("Dates after %s will not be available for backtest",TimeToString(latestdate)); } } //-- the volume calculations and the risk type set by the trader Print("Lots: ",CRisk.Volume()," || Risk type: ",CRisk.GetRiskOption()); //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- } //+------------------------------------------------------------------+
一旦所有内容都编译完成,我们将进行一些步骤,这些步骤将在EA被放置在图表上后开始进行。
一旦您决定好要打开哪个交易品种的图表窗口,在附加EA之前,您的图表可能与上面的图表类似。
现在我们将附加EA。
我们可以首先配置风险管理输入变量,我将先保留其默认值。
如果这是您首次运行NewsTrading2.00版本,且之前未在您的交易商平台上运行过NewsTrading1.00版本,且通用文件夹中不存在日历数据库。
你的图表将以这种方式显示,但图表颜色可能因交易商而异。
正如下面的消息所示,将根据设置中选择的风险选项显示手数。
如果终端无法建立连接。
当首次运行NewsTrading2.00版EA,且日历数据库是从NewsTrading1.00版EA创建的。
NewsTrading2.00将删除NewsTrading1.00中的所有表格
结论
在本文中,我们探讨了继承的工作原理,并通过一个示例进行了说明。我们创建了一个新的“夏令时”类,作为不同夏令时安排类的父类。我们创建了一个“交易品种属性”类,用于从所有继承自该类的子类中检索交易品种属性。我们还创建了一个“图表属性”类,用于自定义图表。我们比较了不同的SQLite方法,并介绍了一种提高数据库整体效率的简单方法。我们新建了一个“对象属性”类,用于创建图表对象并删除它们,我们还新建了一个“风险管理”类,以满足不同风险偏好的交易者的需求。最后,我们创建了最后一个名为“通用图形”的类,用于将交易品种属性显示在图表上,并同时显示交易者的风险选项。在下一篇文章让新闻交易轻松上手(第三部分):执行交易,我们将最终开始开仓交易,我已经迫不及待地在一个合理的时间范围内来完成它!
本文由MetaQuotes Ltd译自英文
原文地址: https://www.mql5.com/en/articles/14912


