Thanks for the new functionality.
I consider the good help on the new functionality as the key to success in mastering it. I really miss examples of the work in the help itself.
Please also pay attention to the following deficiencies which were found:
1) The description of the DatabaseExecute function is not true, but copied fromDatabasePrepare.
2) Incomplete description of the first parameter ofDatabaseRead function:intdatabase,// database handle obtained in DatabaseOpen;
SinceDatabasePrepare provides more complete information:Creates a query handle, which can then be executed with DatabaseRead().
3) DoDatabaseTransactionXXX functions really generate the given GetLastError() error lists or do they perform a "follow-up error from a previous failure"?
4) No information is provided for DatabaseTransactionXXX functions about handling nested transactions.
5) There is a misprint in theDatabaseColumnName function parameter description (it must be "to get the field name")
string&name// reference to the variable for getting thetable name
Great news, Renat! One question has arisen.
Can a .sqlite file be included in the ME project structure?
If so, how will the .ex5 program behave when the .sqlite file size is increased? in an already compiled .ex5 program
Most likely we will allow resources to be included and these files will be automatically extracted to disk the first time the programme is started.
That is, there will be no swelling of the base inside ex5. The file can only be handled on disk.
The bases can be kept either on disk or in memory only, using the DATABASE_OPEN_MEMORY flag.
Do I understand correctly that this is the official mechanism of data exchange between MT5 terminals (instead of killing SSD files) and between programs inside the terminal (instead of resources)?
Thanks for the new functionality.
I think a good help for the new functionality is the key to success in mastering it. I really miss the examples of how to work in the help itself.
Please also pay attention to the following disadvantages which I found:
1) The description of the DatabaseExecute function is not true, but copied from DatabasePrepare.
2) Incomplete description of the first parameter ofDatabaseRead function:intdatabase, // database handle obtained in DatabaseOpen;
Since DatabasePrepare provides more complete information: s creates a query handle, which can then be executed with DatabaseRead().
3) DoDatabaseTransactionXXX functions really generate the given error lists GetLastError() or do they perform "follow-up error from a previous failure"?
4) No information is provided for DatabaseTransactionXXX functions about handling nested transactions.
5) There is a misprint in the DatabaseColumnName function parameter description (it must be "to get the field name")
string&name// reference to the variable for getting thetable name
The help has already been partially updated, have a look again. This is still the first version of the help and it will be updated.
There are no nested transactions in SQLite, so no need to try to do them.
Examples are presented in the topic, there's nothing complicated there at all. We'll do an article and a wrapper class in the standard library later on.
Do I understand correctly that this is an official mechanism of data exchange between MT5 terminals (instead of SSD killing files) and between programs inside the terminal (instead of resources)?
Stop spreading blatant nonsense about "killing SSDs" from incompetent users.
No, these are file bases - they can be exchanged, but it's risky to access them simultaneously from different experts due to potentially monopoly access when bases are open at the same time.
Open "in-memory-only by DATABASE_OPEN_MEMORY flag" databases are only available to a specific program and are not shared with anyone.
Database applications:
- Storing of settings and states of MQL5 programs
- Mass data storage
- Using externally prepared data
. For example, exported data from Metatrader to SQLite, in Python calculated this data using ready-to-use math packages and put the result also in SQlite format.
The database editor in ME, well that would be very handy, thank you.
No, these are file bases - they can be exchanged, but it is risky to access them simultaneously from different EAs due to potentially monopoly access when bases are open at the same time.
Python has a library calledSqlite3Worker for thread-safe I/O when working with a database from multiple threads.
Perhaps it makes sense to think about porting the implementation to mql, to allow asynchronous work with the database of multiple Expert Advisors.
Well, or borrow the idea and implement your own asynchronous I/O.
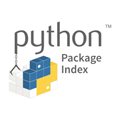
Python hasSqlite3Worker library, for thread-safe input/output, when working with the database from multiple threads.
Perhaps there is a point to consider porting implementation to mql, to allow asynchronous work with the database of several Expert Advisors.
Well, or borrow the idea and implement your own asynchronous I/O.
Have you seen the performance table above? It's often faster in MQL5 than in C++.
We have multithreading, of course, and everything is correct.
The question is about something else - what happens if different programs/processes independently access the same database file. Not a single program (MQL5), but several independent programs that do not know about each other and do not use the same database handle.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
In the 2265 build we have implemented the regular database functions based on SQLite 3.30.1:
As we are maximally focused on performance, here are the results of LLVM 9.0.0 vs MQL5 tests. Time in milliseconds, the less the better:Databases can be held either on disk or in memory only with the DATABASE_OPEN_MEMORY flag. Wrapping massive inserts/changes in DatabaseTransactionBegin/Commit/Rollback transactions speeds up operations hundreds of times.
The speed in MQL5 is absolutely the same as in native C++ with one of the best compilers. A suite of benchmarks for replaying is attached.
We have also implemented a unique DatabaseReadBind function that allows you to read records directly into the structure, which simplifies and speeds up bulk operations.
Here is a simple example: