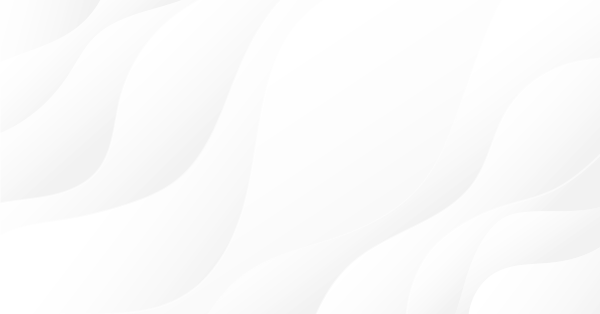
MQL5 Cookbook: Using Different Print Modes
Introduction
This is the first article of the MQL5 Cookbook series. I will start with simple examples to allow those who are taking their first steps in programming to gradually become familiar with the new language. I remember my first efforts at designing and programming trading systems which I may say was quite difficult, given the fact that it was the first programming language in my life. Until then I used to think that I was not made for this and would hardly ever be able to understand it.
However, it turned out to be easier than I thought and it only took me a few months before I could develop a fairly complex program. You can find out more about it in the article called "Limitless Opportunities with MetaTrader 5 and MQL5".
Here, I will try to guide the beginning developers of Expert Advisors through the programming process, building up from the simple to the complex. I am not going to copy here any information available under Help on the MetaEditor 5 menu so prepare to have to press F1 quite often. At the same time this article series will provide you with a lot of examples, ready made functions and schemes that can be used in your developments both in the original and customized way.
So let us start. In this article, we will create a simple script that prints some of the symbol properties in various modes. When developing a program, especially early in the learning process, there will often be situations where the program does not respond as expected. If this happens, you will need to check values of certain variables involved in calculations. In our case we will take a close look at three methods where we will use Print(), Comment() and Alert() functions. And you will afterwards be able to decide for yourself which method is more convenient to you.
MQL5 Wizard
My study of MQL5 started with scripts. It is pretty easy and fast. When the script is loaded, it executes the function you created and is then deleted from the chart. This allows you to experiment on the go and see in what direction to move.
If you have not yet installed the MetaTrader 5 trading terminal, you can install it right now. After installation, start the terminal and open MetaEditor 5 by pressing F4. This button can be used to quickly switch between the MetaTrader 5 trading terminal and MetaEditor 5. You can also start MetaEditor 5 by clicking on the appropriate button in the terminal toolbar. Be sure to study the program interface really well using Help (F1) in the trading terminal and MetaEditor 5 as we may only briefly touch upon certain issues, if at all.
Start MetaEditor 5 and press Ctrl+N or the New button below the main menu on the MetaEditor panel. An MQL5 Wizard window will open where you will be able to select the type of the program you want to create. In our case, we select Script and press Next:
Fig. 1. MQL5 Wizard - Script
You will then need to enter the script name (file name). By default, scripts are created under Metatrader 5\MQL5\Scripts\file_name.mq5. In that directory, you can also create other folders to group your files by their purpose.
Fig. 2. MQL5 Wizard - File Name
At this point, you can also add input parameters, if necessary. When everything is set and ready, click Finish. A new document will be created using the template and you will be able to proceed with the script:
//+------------------------------------------------------------------+ //| PrintModes.mq5 | //| Copyright 2012, MetaQuotes Software Corp. | //| http://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2012, MetaQuotes Software Corp." #property link "http://www.mql5.com" #property version "1.00" //+------------------------------------------------------------------+ //| Script program start function | //+------------------------------------------------------------------+ void OnStart() { //--- } //+------------------------------------------------------------------+
Writing a Code
Everything that follows a double slash is a comment and does not affect the program execution. It is always advisable to provide detailed comments to your code as it makes it considerably easier to understand the program logic, especially after a long break.
All that goes after #property in the first three lines of code above has to do with program properties. More information on each function and property can be found in MQL5 Reference. To quickly view the description of any given function, you only need to double-click on the function in order to select it and press F1. The Help window will open providing you with a description of the selected function.
It is followed by the main script function - OnStart() which is the one that contains all other functions and calculations.
We need to allow for the possibility to select a method for printing the required information before the program execution. It means that we should have an external parameter that will enable us to select the necessary mode from the drop-down list. Further, we need to specify another property (#property) that will be responsible for opening a window with external parameters of the script prior to program execution. This property is script_show_inputs. We will add it below all other properties that we already have in the code:
#property copyright "Copyright 2012, MetaQuotes Software Corp." #property link "http://www.mql5.com" #property version "1.00" #property script_show_inputs //---
To have the drop-down list in the external parameters, we need to create enumeration of all modes. Let us insert this code after the program properties:
// ENUMERATION enum ENUM_PRINT_MODE { PRINT = 0, COMMENT = 1, ALERT = 2 }; //---
This is followed by the only external parameter in this script - PrintMode:
// INPUT PARAMETERS input ENUM_PRINT_MODE printMode=PRINT; // Print mode
Input parameters are as a rule located at the beginning of the program, with the input modifier that defines the external parameter being placed before the variable type. If we run the script now, a program window will open and we will be able to select a print mode from the drop-down list of the Print Mode parameter:
Fig. 3. Window of the script parameters
Then we create variables and assign to them some values (in our case, we will print some symbol data) to further print them using the mode specified by the user in the external parameter. For this purpose, we need to arrange a separate user function - PrintSymbolProperties() - that will be called in the main OnStart() function.
To create the function, we simply need to insert the code as shown below:
//+------------------------------------------------------------------+ //| PRINTING SYMBOL PROPERTIES | //+------------------------------------------------------------------+ void PrintSymbolProperties() { }
The type of the value to be returned should be specified before the function name. Alternatively, if the function does not return anything, as in our case, we should put void. Further, we need to write the remaining code in the PrintSymbolProperties() function body, between the braces. Let us first create the variables:
string symb_symbol = ""; // Symbol int symb_digits = 0; // Number of decimal places int symb_spread = 0; // Difference between the ask price and bid price (spread) int symb_stoplevel = 0; // Stop levels double symb_ask = 0.0; // Ask price double symb_bid = 0.0; // Bid price
Variable type depends on the type of data that will be assigned to it. Now let us assign values to these variables. To get symbol properties, MQL5 provides special functions for every data type.
symb_symbol =Symbol(); symb_digits =(int)SymbolInfoInteger(_Symbol,SYMBOL_DIGITS); symb_spread =(int)SymbolInfoInteger(_Symbol,SYMBOL_SPREAD); symb_stoplevel =(int)SymbolInfoInteger(_Symbol,SYMBOL_TRADE_STOPS_LEVEL); symb_ask =SymbolInfoDouble(_Symbol,SYMBOL_ASK); symb_bid =SymbolInfoDouble(_Symbol,SYMBOL_BID); //---
To ensure adequate learning of the material, use MQL5 Reference to study each and every function and parameters they pass. All symbol properties there are displayed in a table which you will often have to refer to.
Now that the variables have values assigned to them, we only need to write an appropriate code for every print mode. This is what it looks like:
//--- // If it says to print to the journal if(printMode==PRINT) { Print("Symbol: ",symb_symbol,"\n", "Digits: ",symb_digits,"\n", "Spread: ",symb_spread,"\n", "Stops Level: ",symb_stoplevel,"\n", "Ask: ",symb_ask,"\n", "Bid: ",symb_bid ); } //--- // If it says to print to the chart if(printMode==COMMENT) { int mb_res=-1; // Variable with the option selected in the dialog box //--- Comment("Symbol: ",symb_symbol,"\n", "Digits: ",symb_digits,"\n", "Spread: ",symb_spread,"\n", "Stops Level: ",symb_stoplevel,"\n", "Ask: ",symb_ask,"\n", "Bid: ",symb_bid ); //--- // Open a dialog box mb_res=MessageBox("Do you want to delete comments from the chart?",NULL,MB_YESNO|MB_ICONQUESTION); //--- // If "Yes" is clicked, remove the comments from the chart if(mb_res==IDYES) { Comment(""); } //--- return; } //--- // If it says to print to the alert window if(printMode==ALERT) { Alert("Symbol: "+symb_symbol+"\n", "Digits: "+IntegerToString(symb_digits)+"\n", "Spread: "+IntegerToString(symb_spread)+"\n", "Stops Level: "+IntegerToString(symb_stoplevel)+"\n", "Ask: "+DoubleToString(symb_ask,_Digits)+"\n", "Bid: "+DoubleToString(symb_bid,_Digits) ); } //---
If you select PRINT for the external parameter of the Expert Advisor, the information will be printed to the journal of Expert Advisors (Toolbox - Experts tab):
Fig. 4. Toolbox - Experts tab
If you select COMMENT, the information will be displayed in the top left corner of the chart. After the information appears in the chart, the MessageBox() function will open a dialog box that will prompt you to delete comments from the chart:
Fig. 5. Comments in the top left corner of the chart
If you opt for ALERT, after running the script an alert window containing requested information or a simple user message will appear. In this case, as in case with the PRINT option, the information is also printed to the journal but it is additionally accompanied by a sound notification.
Fig. 6. Alert window
Below is the entire script code:
//+------------------------------------------------------------------+ //| PrintModes.mq5 | //| Copyright 2012, MetaQuotes Software Corp. | //| http://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2012, MetaQuotes Software Corp." #property link "http://tol64.blogspot.com" #property description "email: hello.tol64@gmail.com" #property version "1.00" #property script_show_inputs //--- //--- ENUMERATION enum ENUM_PRINT_MODE { PRINT = 0, COMMENT = 1, ALERT = 2 }; //--- // INPUT PARAMETERS input ENUM_PRINT_MODE printMode=PRINT; // Print mode //--- //+------------------------------------------------------------------+ //| MAIN FUNCTION | //+------------------------------------------------------------------+ void OnStart() { PrintSymbolProperties(); } //+------------------------------------------------------------------+ //| PRINTING SYMBOL PROPERTIES | //+------------------------------------------------------------------+ void PrintSymbolProperties() { string symb_symbol = ""; // Symbol int symb_digits = 0; // Number of decimal places int symb_spread = 0; // Difference between the ask price and bid price (spread) int symb_stoplevel = 0; // Stop levels double symb_ask = 0.0; // Ask price double symb_bid = 0.0; // Bid price //--- symb_symbol =Symbol(); symb_digits =(int)SymbolInfoInteger(_Symbol,SYMBOL_DIGITS); symb_spread =(int)SymbolInfoInteger(_Symbol,SYMBOL_SPREAD); symb_stoplevel =(int)SymbolInfoInteger(_Symbol,SYMBOL_TRADE_STOPS_LEVEL); symb_ask =SymbolInfoDouble(_Symbol,SYMBOL_ASK); symb_bid =SymbolInfoDouble(_Symbol,SYMBOL_BID); //--- // If it says to print to the journal if(printMode==PRINT) { Print("Symbol: ",symb_symbol,"\n", "Digits: ",symb_digits,"\n", "Spread: ",symb_spread,"\n", "Stops Level: ",symb_stoplevel,"\n", "Ask: ",symb_ask,"\n", "Bid: ",symb_bid ); } //--- // If it says to print to the chart if(printMode==COMMENT) { int mb_res=-1; // Variable with the option selected in the dialog box //--- Comment("Symbol: ",symb_symbol,"\n", "Digits: ",symb_digits,"\n", "Spread: ",symb_spread,"\n", "Stops Level: ",symb_stoplevel,"\n", "Ask: ",symb_ask,"\n", "Bid: ",symb_bid ); //--- // Open a dialog box mb_res=MessageBox("Do you want to delete comments from the chart?",NULL,MB_YESNO|MB_ICONQUESTION); //--- // If "Yes" is clicked, remove the comments from the chart if(mb_res==IDYES) { Comment(""); } //--- return; } //--- // If it says to print to the alert window if(printMode==ALERT) { Alert("Symbol: "+symb_symbol+"\n", "Digits: "+IntegerToString(symb_digits)+"\n", "Spread: "+IntegerToString(symb_spread)+"\n", "Stops Level: "+IntegerToString(symb_stoplevel)+"\n", "Ask: "+DoubleToString(symb_ask,_Digits)+"\n", "Bid: "+DoubleToString(symb_bid,_Digits) ); } } //+------------------------------------------------------------------+
Conclusion
We will be ending this article here. In addition to the methods described above, you can also write data to a file. It can be a plain text file or even a HTML report nicely formatted using CSS. But these methods are much more complicated for beginners and the ones provided above should be enough for a start.
Translated from Russian by MetaQuotes Ltd.
Original article: https://www.mql5.com/ru/articles/638






- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use