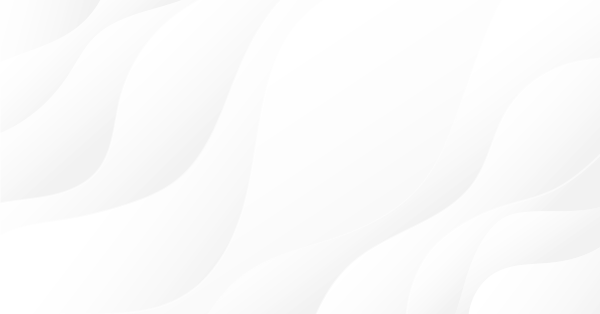
Alert and Comment for External Indicators (Part Two)
Introduction
The first article "Alert and Comment for external indicators" dealt with the method of getting data from indicators that use Wingdings symbols displayed in a chart as a source of information.
Here, we will see how we can get values from indicator buffers and use them to inform the user of certain indicator events.
Whereas there is a group of operators that are used to get the required characteristics when reading the parameters of graphical plotting, parameters of indicator buffers can only be set. Here, we cannot get, let's say, color. All we can obtain are price and time characteristics. As an example, we will take an indicator that is based on the principle of color change when displaying information in the indicator.
One of the options of storing information in indicator buffers of such indicators is arranged so that two indicator buffers are used to display one line. Each of the buffers is assigned a static color. Information is output in such a way that only one of the two buffers is involved in displaying information at any point of time. The active buffer has parameter values greater than zero. The parameter value in the inactive buffer is zero.
So, we have the values that can be analyzed to output information on the changes in the indicator.
Indicator Buffer Data Output
The MQL resources allow us to input/output data to eight indicator buffers.
Therefore we will analyze all eight of them.
Conditions to be applied in the analysis are as follows: When the indicator value changes from zero to a significant one, including the reverse change, i.e. from a significant value to zero, the user must be notified. We take the value of the first changed indicator buffer and check values of all buffers one by one starting with the first bar or any other bar as specified by the user in the external variable.
extern int StartBar=1; // Start bar
As an example, let's take the Slope Trend_mtf indicator that outputs information as follows:
Figure 1
Once detected, the change is registered by the indicator and the operation of the indicator stops.
There are several MQL4 features that can be used to output data:
- Alert windows;
- comments in the indicator window;
- playing a sound file.
A subprogram that outputs data is as follows.
//+------------------------------------------------------------------+ //| AlertSignal_v1 indicator Subfunction | //+------------------------------------------------------------------+ void AlertComment (string text) { if (SignalAlert == true) Alert (text); if (SignalComment == true) Comment (text); if (SignalPlay == true) PlaySound(Signal); }
We add the necessary variables to be controlled at user's discretion.
Let's also provide for the possibility to output a user comment specified by the user.
extern int StartBar=1; // Start bar extern string indicator = "Insert Indicator Name"; // Custom indicator extern bool VLine = true; // Vertical line output switch extern bool SignalAlert=false; // Alert window output switch extern bool SignalComment=true; // Comment output switch extern bool SignalPlay= false; // Sound play switch extern string Signal = "alert.wav"; // Custom sound file extern string UserText = "direction changed"; // User comment
where:
StartBar
The variable indicating the calculation start:
indicator
Here, we enter the name of the indicator for which we want to get data.
This is followed by a series of logical parameters that can be used by the user to handle the output of the relevant program parameters.
VLine
Indicates whether to display (true) or not to display (false) a vertical line at the point of change in the indicator parameters.
SignalAlert
Indicates whether to display (true) or not to display (false) the information window when there is a change in the indicator parameters.
SignalComment
Indicates whether to output (true) or not to output (false) data in the chart when there is a change in the indicator parameters.
SignalPlay
Indicates whether to play (true) or not to play (false) a sound file when there is a change in the indicator parameters.
Signal
Here, we enter the name of the sound file to be played when there is a change in the indicator parameters.
UserText
Here, we enter the text that we want to see in the chart when there is a change in the indicator parameters if we don't like the standard text.
Similarly, you can add a parameter for sending the relevant information to your e-mail.
Now we only need to read the indicator data and handle it using our settings.
The handling block code is as follows:
for(int ii=StartBar;ii<ExtBars;ii++) for(int i=0;i<8;i++) { First =iCustom(NULL,0,indicator,i,ii); Second=iCustom(NULL,0,indicator,i,ii+1); if((Second==0 && First>0) || (Second>0 && First==0)) { text=StringConcatenate(Str+" - On ",ii+1," bar ",Symbol()," - ",indicator," "); AlertComment(text+UserText); if(VLine==true) ObjectCreate(STRING_ID+"VL_"+i,0,0,Time[ii],Bid); return; } }
After implementing the specified approach and setting the relevant parameter to display the vertical line, the chart will look as follows:
Figure 2
Conclusion
Despite the numerous comments on the imperfections of MQL4, this language offers ample features that can be widely used in programming various trading and information applications. In addition, it supports intuitive learning and gives you access to a huge library of ready made applications.
The complete code of the information indicator is provided below:
//+------------------------------------------------------------------+ //| AlertSignal_v1.mq4 | //| Copyright © 2009, WWW.FIBOOK.RU | //| http://fibook.ru | //+------------------------------------------------------------------+ #property copyright "Copyright © 2009, FIBOOK.RU" #property link "http://fibook.ru" #define Str "http://fibook.ru" #define STRING_ID "AlertSignal_" #property indicator_chart_window extern int StartBar=1; // Start bar extern string indicator="Insert Indicator Name"; // Custom indicator extern bool VLine=true; // Vertical line output switch extern bool SignalAlert=false; // Alert window output switch extern bool SignalComment=true; // Comment output switch extern bool SignalPlay=false; // Sound play switch extern string Signal="alert.wav"; // Custom sound file extern string UserText="direction changed"; // User comment IndicatorShortName("AlertSignal_v1 "); //+------------------------------------------------------------------+ //| AlertSignal_v1 indicator deinitialization function | //+------------------------------------------------------------------+ int deinit() { //---- for(int i=0;i<8;i++) ObjectDelete(STRING_ID+"VL_"+i); //---- return(0); } //+------------------------------------------------------------------+ //| AlertSignal_v1 indicator iteration function | //+------------------------------------------------------------------+ int start() { string text; int ExtBars=150; // Number of the last bars for calculation double First; // Internal variable double Second; // Internal variable int per; // Internal variable if(indicator=="Insert Indicator Name") { Alert(indicator); return; } if(per==Time[0]) return; // Adjust once in a period per=Time[0]; //---- for(int ii=StartBar;ii<ExtBars;ii++) for(int i=0;i<8;i++) { First =iCustom(NULL,0,indicator,i,ii); Second=iCustom(NULL,0,indicator,i,ii+1); if((Second==0 && First>0) || (Second>0 && First==0)) { text=StringConcatenate(Str+" - On ",ii+1," bar ",Symbol()," - ",indicator," "); AlertComment(text+UserText); if(VLine==true) ObjectCreate(STRING_ID+"VL_"+i,0,0,Time[ii],Bid); return; } } //---- return(0); } //+------------------------------------------------------------------+ //| AlertSignal_v1 indicator Subfunction | //+------------------------------------------------------------------+ void AlertComment(string text) { if(SignalAlert==true) Alert(text); if(SignalComment==true) Comment(text); if(SignalPlay==true) PlaySound(Signal); } //+------------------------------------------------------------------+
Translated from Russian by MetaQuotes Ltd.
Original article: https://www.mql5.com/ru/articles/1372





- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use