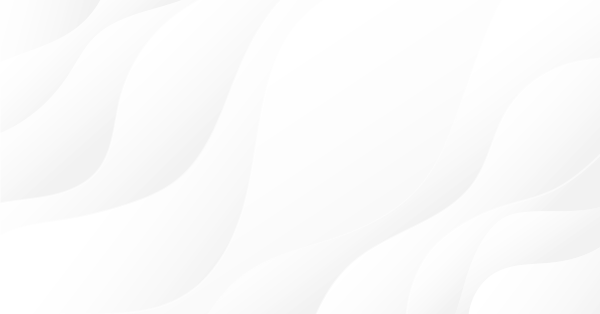
Connection of Expert Advisor with ICQ in MQL5
Introduction
ICQ is a centralized service of instant exchange of text messages, with an offline mode, which uses the OSCAR protocol. For a trader, ICQ can serve as a terminal, which displays timely information, as well as a control panel. This article will demonstrate an example of how to implement an ICQ client, with a minimum set of functions, within an Expert Advisor.
The draft of the IcqMod project, containing an open original code, was used and processed as a basis for the article. Protocol of exchange with the ICQ server is implemented in the DLL module icq_mql5.dll. It is written in C++ and makes uses the only Windows library winsock2. A compiled modules and a source code for Visual Studio 2005 are attached to this article.
Distinguishing features and limitations of the implementation of this client:
- the maximum number of simultaneously working clients is theoretically unlimited.
- the maximum size of an incoming messages - 150 characters. The reception of longer messages is not supported.
- Unicode support.
- supports only a direct connection. Connection made through a proxy server (HTTP / SOCK4 / SOCK5) is not supported.
- offline messages do not get processed.
Description of the library functions
Descriptions of constants and functions of the dll module are located in the executable file icq_mql5.mqh.
The function ICQConnect is used to connect to the server:
uint ICQConnect (ICQ_CLIENT & cl, // Variable for storing data about the connection string host, // Server name, such as login.icq.com ushort port, // Server port, eg 5190 string login, // Account Number (UIN) string pass // Account password for)
Description of the return value through ICQConnect:
Constant's Name |
Value |
Description |
---|---|---|
ICQ_CONNECT_STATUS_OK |
0xFFFFFFFF | Connection established |
ICQ_CONNECT_STATUS_RECV_ERROR |
0xFFFFFFFE |
Reading data error |
ICQ_CONNECT_STATUS_SEND_ERR |
0xFFFFFFFD |
Sending data error |
ICQ_CONNECT_STATUS_CONNECT_ERROR |
0xFFFFFFFC |
server connection error |
ICQ_CONNECT_STATUS_AUTH_ERROR | 0xFFFFFFFB | Authorization error: incorrect password or exceeded the limit of connections |
Structure for storing data about the connection:
struct ICQ_CLIENT ( uchar status; // connection status code ushort sequence; // sequence meter uint sock; // socket number )
In practice, in order to perform an analysis of the connection status in this structure, we use the variable status, which can assume the following values:
Constant's Name |
Value |
Description |
---|---|---|
ICQ_CLIENT_STATUS_CONNECTED | 0x01 | A connection to the server is established |
ICQ_CLIENT_STATUS_DISCONNECTED |
0x02 | Connection to server failed |
Frequent attempts to connect to the server can lead to a temporary block of access to your account. Thus it is necessary to wait out a time interval between connection attempts to the server.
Recommended timeout is 20-30 seconds.
The function ICQClose serves to terminate the connection to the server:
void ICQClose ( ICQ_CLIENT & cl // Variable for storing connection data)
The ICQSendMsg function is used to send text messages:
uint ICQSendMsg ( ICQ_CLIENT & cl, // Variable to store data about the connection. string uin, // Account number of the recipient string msg // Message)
The return value equals to 0x01, if the message is sent successful, and equals 0x00 if there was a sending error.
The ICQReadMsg function checks for incoming messages:
uint ICQReadMsg ( ICQ_CLIENT & cl, // Variable for storing connection data string & Uin, // Account number of the sender string & Msg, // Message uint & Len // Number of received symbols in the message)
The return value equals to 0x01 if there is an incoming message and equals to 0x00 if there is no message.
COscarClient class
For the convenience of working with ICQ in an object-oriented environment of MQL5, the COscarClient class was developed. Aside from the basic functions described above, it contains a mechanism, which, after a specific interval of time, automatically reconnects to the server (when autocon = true). Description of the class is included in the attached file icq_mql5.mqh and is given below:
//+------------------------------------------------------------------+ class COscarClient //+------------------------------------------------------------------+ { private: ICQ_CLIENT client; // storing connection data uint connect; // flag of status connection datetime timesave; // the time of last connection to the server datetime time_in; // the time of last reading of messages public: string uin; // buffer for the storage of the uin of the sender for a received message string msg; // buffer for the storage of text for a received message uint len; // the number of symbols in the received message string login; // number of the sender's account (UIN) string password; // password for UIN string server; // name of the server uint port; // network port uint timeout; // timeout tasks (in seconds) between attempts to reconnect to the server bool autocon; // automatic connection resume COscarClient(); // constructor for initialization of variable classes bool Connect(void); // establishment of a connection with a server void Disconnect(void); // breaking a connection with a server bool SendMessage(string UIN, string msg); // sending a message bool ReadMessage(string &UIN, string &msg, uint &len); // receiving a message };
Expert Advisor on the COscarClient bases
The minimum Expert Advisor code needed to work with ICQ using the class COscarClient is located in the icq_demo.mq5 file and below:
#include <icq_mql5.mqh> COscarClient client; //+------------------------------------------------------------------+ int OnInit() //+------------------------------------------------------------------+ { printf("Start ICQ Client"); client.login = "641848065"; //<- login client.password = "password"; //<- password client.server = "login.icq.com"; client.port = 5190; client.Connect(); return(0); } //+------------------------------------------------------------------+ void OnDeinit(const int reason) //+------------------------------------------------------------------+ { client.Disconnect(); printf("Stop ICQ Client"); } //+------------------------------------------------------------------+ void OnTick() //+------------------------------------------------------------------+ { string text; static datetime time_out; MqlTick last_tick; // reading the messages while(client.ReadMessage(client.uin,client.msg,client.len)) printf("Receive: %s, %s, %u", client.uin, client.msg, client.len); // transmission of quotes every 30 seconds if((TimeCurrent()-time_out)>=30) { time_out = TimeCurrent(); SymbolInfoTick(Symbol(), last_tick); text = Symbol()+" BID:"+DoubleToString(last_tick.bid, Digits())+ " ASK:"+DoubleToString(last_tick.ask, Digits()); if (client.SendMessage("266690424", //<- number of the recipient text)) //<- message text printf("Send: " + text); } } //+------------------------------------------------------------------+
Figure 1. serves as a demonstration of the work of the Expert Advisor, which allows for the exchange of text messages with the ICQ client.
Figure 1. Text messaging between MetaTrader 5 and ICQ2Go
Capacity building
Let's complicate the task by bringing it closer to practical application. For example, we need to manage the work of our Expert Advisor, and obtain the necessary information remotely, using a mobile phone or another PC connected to the Internet. To do this, we describe a set of commands for controlling the future Expert Advisor. Also, let's complement the advisor with a parsing function to decode the incoming commands.
The format, common to all commands, will be as following:
[? |!] [command] [parameter] [value] ,
where? - A symbol of command reading; ! - A symbol of a operation writing.
A list of commands given in the table below:
help | reading | Display of reference of the syntax and the list of commands |
info | reading | Display of data of the account summary |
symb | reading | Market price for given currency pair |
ords | reading/writing | Managing of open orders |
param | reading/writing | managing Expert Advisor's parameters |
close | record | Termination of the Expert Advisor's work and the closure of the terminal |
shdwn | record | Shutdown of PC |
The Expert Advisor, which implements the processing of this set of commands, is located in the file icq_power.mq5 .
Figure 2 shows a clear demonstration of the work of the Expert Advisor. Commands are received from the CCP with an installed ICQ client (Figure 2a), as well as through the WAP server http://wap.ebuddy.com which implements the work with ICQ (Figure 2b). The second option is preferable for those who do not wish to deal with searching for, installing, and configuring software for ICQ on their mobile phone.
Figure 2. Working with an advisor via the ICQ client for Pocket PC (Figure 2a), as well as through the wap.ebuddy.com wap site (Figure 2b).
Visual ICQ component
This section will briefly consider an example of a script icq_visual.mq5 which implements a component, the visual appearance of which is shown in Figure 3.
Figure 3. Visual ICQ component
The form of the component resembles a Windows window and is built from arrays of control elements, such as buttons, text boxes, and text labels.
For convenience, an integrated control element for storing a list of accounts and contacts is implemented in the form. Values are selected from the list through the use of appropriate navigation buttons.
To create a window in the style of ICQ 6.5, we can replace the buttons with image tags. Figure 4 shows the visual appearance of the component, implemented in the icq_visual_skin.mq5 script. For those who wish to create their own component design, it is sufficient enough to develop and replace the skin.bmp file, which is responsible for the appearance of the window.
Figure 4. Color design of the visual ICQ component
Conclusion
This article demonstrates one of the easiest ways to implement an ICQ client for MetaTrader 5 by using the means of an embedded programming language.
Translated from Russian by MetaQuotes Ltd.
Original article: https://www.mql5.com/ru/articles/64




Andrei, are there any plans for a similar library for Skype?
Doesn't this one fit?
Andrei, are there any plans for a similar library for Skype?
unfortunately the shop has been closed.
Skype doesn't support sending messages with dll anymore
Unfortunately, the shop has been shut down.
Skype no longer supports dll messaging.