MetaTrader 5 Platform build 3390: Float in OpenCL and in mathematical functions, Activation and Loss methods for machine learning
The tester and meta editor features is still not working for Mac M1 and M2 if it is a fresh install. Old version works fine.
MT5 will freeze when trying to launch Meta Editor for few minutes then nothing happens. Running tester will also lead to a core stating "busy" then nothing happens.
This started in version 3300+ and is persisting.
There have been many similar complaints here:
https://www.mql5.com/en/forum/428028
https://www.mql5.com/en/forum/428080
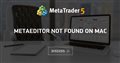
- 2022.07.03
- www.mql5.com
I have an issue with StringFormat/PrintFormat with version 3360 as I described in
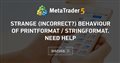
- 2022.07.17
- www.mql5.com
In Build 3366:
Same issue as described in https://www.mql5.com/en/forum/428829
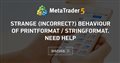
- 2022.07.17
- www.mql5.com
In Build 3366:
Same issue as described in https://www.mql5.com/en/forum/428829
The same in Build 3367.
Probably never! After decades, they still have not learned their lesson about how to properly handle financial data.
lol. Cursory google search shows this https://github.com/vpiotr/decimal_for_cpp for example. It's bsd licensed so they could just add it to their C++ flavor if they do not want to do the heavy lifting.
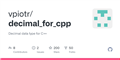
- vpiotr
- github.com
Please correct the specifications of XAUUSD & XAGUSD (and provide hist. data for XAGUSD ).
Here are the results of calculating the optimal lot size if sl, risk an free margin are given: Gold and Silver with problems while EURUSD ok:
MetaQuotes demo account (b. 3369 Beta channel) 2022.07.25 17:43:42.645 test_MM-functions (EURUSD,M1) XAUUSD in: 1717.65000 sl:1717.15000 diff:0.50000 delta:10.00000 delta:*diff:5.00000 tVal:0.10000 tSz:0.01000 2022.07.25 17:43:42.645 test_MM-functions (EURUSD,M1) XAGUSD in: 18.45100 sl:18.40100 diff:0.05000 delta:500.00000 delta:*diff:25.00000 tVal:0.50000 tSz:0.00100 2022.07.25 17:43:42.645 test_MM-functions (EURUSD,M1) EURUSD in: 1.02185 sl:1.02235 diff:0.00050 delta:97861.72139 delta:*diff:48.93086 tVal:0.97862 tSz:0.00001 2022.07.25 17:43:42.645 test_MM-functions (EURUSD,M1) Set: Risk: 5.0 %, dSL: 50 Com/Lot: 2.00 Free: 9983.40 EUR: XAUUSD BU in: 1717.65000 sl: 1717.15000 lotsC: 71.3100 (-3489.26) lotsB: 10.2000 (-499.09) 2022.07.25 17:43:42.645 test_MM-functions (EURUSD,M1) Set: Risk: 5.0 %, dSL: 50 Com/Lot: 2.00 Free: 9983.40 EUR: XAGUSD BU in: 18.45100 sl: 18.40100 lotsC: 18.4900 (-4523.66) lotsB: 2.0400 (-499.09) 2022.07.25 17:43:42.645 test_MM-functions (EURUSD,M1) Set: Risk: 5.0 %, dSL: 50 Com/Lot: 2.00 Free: 9983.40 EUR: EURUSD SE in: 1.02185 sl: 1.02235 lotsC: 9.8000 (-479.29) lotsB: 10.2100 (-499.34) RoboForex Demo account (b. 3320 release channel) 2022.07.25 17:46:22.772 test_MM-functions (EURUSD,D1) XAUUSD in: 1717.10000 sl:1717.15000 diff:0.05000 delta:100.00000 delta:*diff:5.00000 tVal:0.10000 tSz:0.00100 2022.07.25 17:46:22.772 test_MM-functions (EURUSD,D1) XAGUSD in: 18.43400 sl:18.43350 diff:0.00050 delta:5000.00000 delta:*diff:2.50000 tVal:0.05000 tSz:0.00001 2022.07.25 17:46:22.772 test_MM-functions (EURUSD,D1) EURUSD in: 1.02186 sl:1.02236 diff:0.00050 delta:100000.00000 delta:*diff:50.00000 tVal:1.00000 tSz:0.00001 2022.07.25 17:46:22.773 test_MM-functions (EURUSD,D1) Set: Risk: 5.0 %, dSL: 50 Com/Lot: 2.00 Free: 5348.69 USD: XAUUSD SE in: 1717.10000 sl: 1717.15000 lotsC: 38.2000 (-191.00) lotsB: 53.4900 (-267.45) 2022.07.25 17:46:22.773 test_MM-functions (EURUSD,D1) Set: Risk: 5.0 %, dSL: 50 Com/Lot: 2.00 Free: 5348.69 USD: XAGUSD BU in: 18.43400 sl: 18.43350 lotsC: 59.4300 (-148.57) lotsB: 106.9700 (-267.42) 2022.07.25 17:46:22.773 test_MM-functions (EURUSD,D1) Set: Risk: 5.0 %, dSL: 50 Com/Lot: 2.00 Free: 5348.69 USD: EURUSD SE in: 1.02186 sl: 1.02236 lotsC: 5.1400 (-257.00) lotsB: 5.3500 (-267.50)
Lot size of 71.31 and 18.49 are way to high. I attached the script to compare an alternative version of calculation. Thanks B. Flotterer: https://www.mql5.com/de/forum/394471/page1408#comment_41003325.
Here are the specs for Gold of RoboForex and MQ:
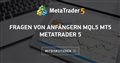
- 2022.07.20
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
The MetaTrader 5 platform update will be released on Thursday, August 4, 2022. The update will feature the following changes:
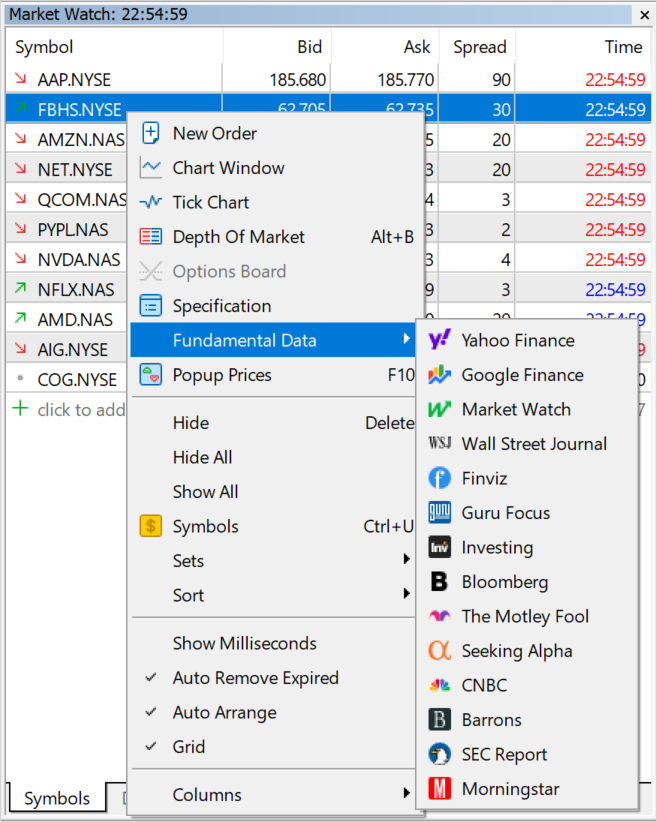
About 7,000 securities and more than 2,000 ETFs are listed on the global exchange market. Furthermore, exchanges provide futures and other derivatives. The MetaTrader 5 platform offers access to a huge database of exchange instruments. To access the relevant fundamental data, users can switch to the selected aggregator's website in one click directly from the Market Watch. For convenience, the platform includes a selection of information sources for each financial instrument.We continue expanding algorithmic trading and machine learning capabilities in the MetaTrader 5 platform. Previously, we have added new data types: matrices and vectors, which eliminate the need to use arrays for data processing. More than 70 methods have been added to MQL5 for operations with these data types. The new methods enable linear algebra and statistics calculations in a single operation. Multiplication, transformation and systems of equations can be implemented easily, without extra coding. The latest update includes mathematical functions.
Mathematical functions were originally designed to perform relevant operations on scalar values. From this build and onward, most of the functions can be applied to matrices and vectors. These include MathAbs, MathArccos, MathArcsin, MathArctan, MathCeil, MathCos, MathExp, MathFloor, MathLog, MathLog10, MathMod, MathPow, MathRound, MathSin, MathSqrt, MathTan, MathExpm1, MathLog1p, MathArccosh, MathArcsinh, MathArctanh, MathCosh, MathSinh, and MathTanh. Such operations imply element-wise handling of matrices and vectors. Example:
For MathMod and MathPow, the second element can be either a scalar or a matrix/vector of the appropriate size.
The following example shows how to calculate the standard deviation by applying math functions to a vector.
MQL5: Improved mathematical functions for operations with the float type. The newly implemented possibility to apply mathematical functions to 'float' matrix and vectors has enabled an improvement in mathematical functions applied to 'float' scalars. Previously, these function parameters were unconditionally cast to the 'double' type, then the corresponding implementation of the mathematical function was called, and the result was cast back to the 'float' type. Now the operations are implemented without extra type casting.
The following example shows the difference in the mathematical sine calculations:
The neural network activation function determines how the weighted input signal sum is converted into a node output signal at the network level. The selection of the activation function has a big impact on the neural network performance. Different parts of the model can use different activation functions. In addition to all known functions, MQL5 also offers derivatives. Derivative functions enable fast calculation of adjustments based on the error received in learning.
The loss function evaluates the quality of model predictions. The model construction targets the minimization of the function value at each stage. The approach depends on the specific dataset. Also, the loss function can depend on weight and offset. The loss function is one-dimensional and is not a vector since it provides a general evaluation of the neural network.
To set the mandatory use of GPUs with double support for specific tasks, use the CL_USE_GPU_DOUBLE_ONLY in the CLContextCreate call.
volume=-1 && volume_real=2 — volume_real=2 will be used,
volume=3 && volume_real=0 — volume=3 will be used.
Increased-precision volume MqlBookInfo.volume_real has a higher priority than MqlBookInfo.volume. Therefore, if both values are specified and are valid, volume_real will be used.
If any of the Market Depth elements is described incorrectly, the system will discard the transferred state completely.
The update will be available through the Live Update system.