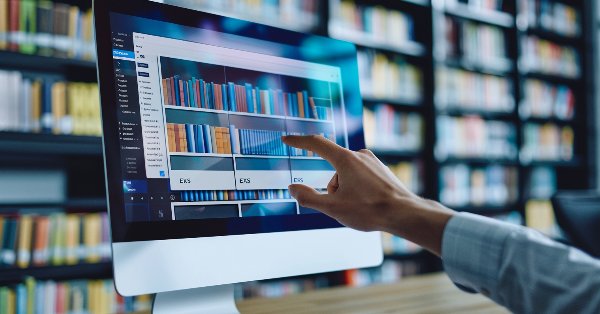
MQL5 Trading Toolkit (Part 6): Expanding the History Management EX5 Library with the Last Filled Pending Order Functions
Introduction
Accessing the details of the most recently filled pending order is particularly valuable in scenarios where your trading logic depends on the type of the last filled pending order. For instance, you can leverage this data to refine your trading strategies based on whether the most recent filled order was a Buy Limit, Sell Stop, Buy Stop, Sell Limit, Buy Stop Limit, or Sell Stop Limit. Understanding the type of order can provide insights into market conditions and inform adjustments to your approach, such as adapting entry or exit points.
This information is also crucial for gathering and analyzing historical trading data to optimize your trading systems or gathering data on how fast your broker fills or executes pending orders when they are triggered and activated. By examining details like slippage, the time elapsed from order placement to execution, and the conditions under which the order was filled, you can identify patterns and areas for improvement in your strategy. Additionally, this data allows you to monitor execution quality, ensuring that your orders are being filled as expected and helping you address potential inefficiencies in your trading operations. Such detailed analysis can enhance decision-making and lead to more effective and robust trading strategies over time.
This history management EX5 library simplifies the process of retrieving details and properties of the most recently filled pending order. By invoking a single function, you can access this data without the additional effort of specifying a period for the trade history search—the EX5 library seamlessly manages that for you. All you need to do is provide the relevant history-querying function with a variable to store the specific pending order property as input. The function will then save the retrieved details into the referenced variable you supply.
If the function successfully retrieves the requested data, it returns true, indicating success. If the specified pending order data is unavailable, it returns false. This streamlined approach eliminates unnecessary complexity, allowing you to focus on analyzing or integrating the retrieved data into your trading strategies, ensuring both efficiency and accuracy in your trading operations.
To get started, we will open the HistoryManager.mq5 file from the previous article where we created the position history retrieval functions and begin by creating the GetLastFilledPendingOrderData() function. Make sure you have downloaded the HistoryManager.mq5 source file, which you will find attached at the end of the previous article. We will continue adding new code below the LastClosedPositionDuration() function, which is where we left off previously.
Get Last Filled Pending Order Data Function
The GetLastFilledPendingOrderData() function is responsible for retrieving the properties of the last filled pending order from the available trading history. It systematically checks the history data for any pending orders that have been filled, and if such an order is found, it saves the relevant data in the referenced getLastFilledPendingOrderData variable.
This function works in tandem with the FetchHistoryByCriteria() function to fetch the necessary trading history data. It ensures that only the filled pending orders are considered and updates the provided structure with the data of the last filled pending order.
Let us begin by defining the GetLastFilledPendingOrderData() function signature. Since this function is marked as export, it is accessible to any MQL5 source code file that imports the compiled EX5 library. The function references the getLastFilledPendingOrderData variable, where the retrieved data will be stored.
bool GetLastFilledPendingOrderData(PendingOrderData &getLastFilledPendingOrderData) export { //-- Function logic will be implemented here }
Next, we will use the FetchHistoryByCriteria() function and pass the GET_PENDING_ORDERS_HISTORY_DATA constant as the function parameter to ensure we have the required pending order history data. If no data is found, we will print a message and return false.
if(!FetchHistoryByCriteria(GET_PENDING_ORDERS_HISTORY_DATA)) { Print(__FUNCTION__, ": No trading history available. Last filled pending order can't be retrieved."); return(false); }
If we successfully retrieve the data, we proceed to find the last filled pending order. We get the total number of pending orders from the GetTotalDataInfoSize() function, then loop through the pendingOrderInfo array to find a pending order that has been filled. Once found, we assign its data to the getLastFilledPendingOrderData structure. Finally, the function returns true if a filled pending order is found and its data is saved. If no filled pending order is found, the function will exit and return false.
int totalPendingOrderInfo = GetTotalDataInfoSize(GET_PENDING_ORDERS_HISTORY_DATA); for(int x = 0; x < totalPendingOrderInfo; x++) { if(pendingOrderInfo[x].state == ORDER_STATE_FILLED) { getLastFilledPendingOrderData = pendingOrderInfo[x]; break; } } return(true);
Here is the full implementation of the GetLastFilledPendingOrderData() function with all the code segments in their correct sequence.
bool GetLastFilledPendingOrderData(PendingOrderData &getLastFilledPendingOrderData) export { if(!FetchHistoryByCriteria(GET_PENDING_ORDERS_HISTORY_DATA)) { Print(__FUNCTION__, ": No trading history available. Last filled pending order can't be retrieved."); return(false); } //-- Save the last filled pending order data in the referenced getLastFilledPendingOrderData variable int totalPendingOrderInfo = GetTotalDataInfoSize(GET_PENDING_ORDERS_HISTORY_DATA); for(int x = 0; x < totalPendingOrderInfo; x++) { if(pendingOrderInfo[x].state == ORDER_STATE_FILLED) { getLastFilledPendingOrderData = pendingOrderInfo[x]; break; } } return(true); }
Last Filled Pending Order Type Function
The LastFilledPendingOrderType() function is responsible for determining the type of the most recently filled pending order. It stores this type in the referenced variable lastFilledPendingOrderType. This capability is essential for applications that need to analyze order execution types or track the performance of specific order categories.
Let us begin by defining the function signature. Since this function is marked as export, it will be accessible for use in any MQL5 source file that imports the EX5 library. The function accepts the referenced lastFilledPendingOrderType variable as an input, where the type of the last filled pending order will be stored.
bool LastFilledPendingOrderType(ENUM_ORDER_TYPE &lastFilledPendingOrderType) export { //-- Function logic will be implemented here }
We will start by declaring a variable of type PendingOrderData named lastFilledPendingOrderInfo. This variable will temporarily store the details of the most recently filled pending order.
PendingOrderData lastFilledPendingOrderInfo;
Next, we will use the GetLastFilledPendingOrderData() function to retrieve the details of the last filled pending order. If the operation is successful, we will extract the type field from the lastFilledPendingOrderInfo structure, store it in the referenced lastFilledPendingOrderType variable, and return true. If the retrieval fails, we will skip updating the referenced variable and return false to indicate that no data was found.
if(GetLastFilledPendingOrderData(lastFilledPendingOrderInfo)) { lastFilledPendingOrderType = lastFilledPendingOrderInfo.type; return(true); } return(false);
Here is the full implementation of the LastFilledPendingOrderType() function.
bool LastFilledPendingOrderType(ENUM_ORDER_TYPE &lastFilledPendingOrderType) export { PendingOrderData lastFilledPendingOrderInfo; if(GetLastFilledPendingOrderData(lastFilledPendingOrderInfo)) { lastFilledPendingOrderType = lastFilledPendingOrderInfo.type; return(true); } return(false); }
Last Filled Pending Order Symbol Function
The LastFilledPendingOrderSymbol() function retrieves the symbol of the most recently filled pending order and stores it in the referenced lastFilledPendingOrderSymbol variable. It accepts the referenced lastFilledPendingOrderSymbol variable as input to store the symbol.
First, we declare a PendingOrderData variable, lastFilledPendingOrderInfo, to temporarily hold the details of the last filled pending order. We then use the GetLastFilledPendingOrderData() function to retrieve the order details. If successful, we extract the symbol from lastFilledPendingOrderInfo, store it in lastFilledPendingOrderSymbol, and return true. If the retrieval fails, we return false without updating the referenced variable.
Here’s the implementation of the LastFilledPendingOrderSymbol() function.
bool LastFilledPendingOrderSymbol(string &lastFilledPendingOrderSymbol) export { PendingOrderData lastFilledPendingOrderInfo; if(GetLastFilledPendingOrderData(lastFilledPendingOrderInfo)) { lastFilledPendingOrderSymbol = lastFilledPendingOrderInfo.symbol; return(true); } return(false); }
Last Filled Pending Order Ticket Function
The LastFilledPendingOrderTicket() function retrieves the ticket number of the most recently filled pending order and stores it in the referenced lastFilledPendingOrderTicket variable. It accepts the lastFilledPendingOrderTicket variable as input, where the ticket number will be saved.
We start by defining a PendingOrderData variable named lastFilledPendingOrderInfo, which serves as a temporary container for the details of the most recently filled pending order. Next, we call the GetLastFilledPendingOrderData() function to fetch the order information. Upon successful retrieval, we extract the ticket from lastFilledPendingOrderInfo, save it into the lastFilledPendingOrderTicket variable, and indicate success by returning true. If the retrieval is unsuccessful, the function returns false, leaving the referenced variable unchanged.
Here’s the implementation of the LastFilledPendingOrderTicket() function.
bool LastFilledPendingOrderTicket(ulong &lastFilledPendingOrderTicket) export { PendingOrderData lastFilledPendingOrderInfo; if(GetLastFilledPendingOrderData(lastFilledPendingOrderInfo)) { lastFilledPendingOrderTicket = lastFilledPendingOrderInfo.ticket; return(true); } return(false); }
Last Filled Pending Order Price Open Function
The LastFilledPendingOrderPriceOpen() function retrieves the opening price of the most recently filled pending order and stores it in the referenced lastFilledPendingOrderPriceOpen variable. It accepts the lastFilledPendingOrderPriceOpen variable as input to store the opening price.
First, we declare a PendingOrderData variable, lastFilledPendingOrderInfo, to temporarily hold the details of the last filled pending order. We then use the GetLastFilledPendingOrderData() function to retrieve the order details. If successful, we extract the priceOpen from lastFilledPendingOrderInfo, store it in lastFilledPendingOrderPriceOpen, and return true. If the retrieval fails, we return false without updating the referenced variable.
Here’s the implementation of the LastFilledPendingOrderPriceOpen() function.
bool LastFilledPendingOrderPriceOpen(double &lastFilledPendingOrderPriceOpen) export { PendingOrderData lastFilledPendingOrderInfo; if(GetLastFilledPendingOrderData(lastFilledPendingOrderInfo)) { lastFilledPendingOrderPriceOpen = lastFilledPendingOrderInfo.priceOpen; return(true); } return(false); }
Last Filled Pending Order Stop Loss Price Function
The LastFilledPendingOrderSlPrice() function retrieves the stop loss price of the most recently filled pending order and stores it in the referenced lastFilledPendingOrderSlPrice variable. It accepts the lastFilledPendingOrderSlPrice variable as input to store the retrieved stop loss price. This function is particularly useful for scenarios where tracking or analyzing stop loss levels of recently filled pending orders is required.
Let us begin by declaring a PendingOrderData variable named lastFilledPendingOrderInfo to temporarily hold the details of the last filled pending order. We then use the GetLastFilledPendingOrderData() function to fetch the details of the order.
If the data retrieval is successful, the slPrice field from lastFilledPendingOrderInfo is extracted and saved in the referenced lastFilledPendingOrderSlPrice variable. The function then returns true to indicate success. If the retrieval fails, the function exits without updating the referenced variable and returns false to signal that no data was found.
Here’s the full implementation of the LastFilledPendingOrderSlPrice() function:
bool LastFilledPendingOrderSlPrice(double &lastFilledPendingOrderSlPrice) export { PendingOrderData lastFilledPendingOrderInfo; if(GetLastFilledPendingOrderData(lastFilledPendingOrderInfo)) { lastFilledPendingOrderSlPrice = lastFilledPendingOrderInfo.slPrice; return(true); } return(false); }
Last Filled Pending Order Take Profit Price Function
The LastFilledPendingOrderTpPrice() function retrieves the take profit price of the most recently filled pending order and stores it in the referenced lastFilledPendingOrderTpPrice variable. This function is essential for applications that analyze or manage take profit levels of filled pending orders. It accepts the lastFilledPendingOrderTpPrice variable as input to store the take profit price.
We start by declaring a PendingOrderData variable, lastFilledPendingOrderInfo, to temporarily hold the details of the last filled pending order. We then use the GetLastFilledPendingOrderData() function to retrieve the order details. If the retrieval is successful, we extract the tpPrice from lastFilledPendingOrderInfo, store it in the lastFilledPendingOrderTpPrice variable, and return true. If the retrieval fails, we return false without updating the referenced variable.
Here’s the implementation of the LastFilledPendingOrderTpPrice() function.
bool LastFilledPendingOrderTpPrice(double &lastFilledPendingOrderTpPrice) export { PendingOrderData lastFilledPendingOrderInfo; if(GetLastFilledPendingOrderData(lastFilledPendingOrderInfo)) { lastFilledPendingOrderTpPrice = lastFilledPendingOrderInfo.tpPrice; return(true); } return(false); }
Last Filled Pending Order Stop Loss Pips Function
The LastFilledPendingOrderSlPips() function retrieves the stop-loss pips value of the most recently filled pending order and stores it in the referenced lastFilledPendingOrderSlPips variable. This function is vital for analyzing the risk parameters associated with executed pending orders.
First, we declare a PendingOrderData variable named lastFilledPendingOrderInfo. This variable will temporarily hold the details of the last filled pending order. We then use the GetLastFilledPendingOrderData() function to fetch the order details. If the operation is successful, we extract the slPips field from the lastFilledPendingOrderInfo structure and store it in the referenced lastFilledPendingOrderSlPips variable. The function then returns true to indicate success. If the retrieval process fails, the referenced variable remains unchanged, and the function returns false to signal that no data was found.
Here’s the complete implementation of the LastFilledPendingOrderSlPips() function,
bool LastFilledPendingOrderSlPips(int &lastFilledPendingOrderSlPips) export { PendingOrderData lastFilledPendingOrderInfo; if(GetLastFilledPendingOrderData(lastFilledPendingOrderInfo)) { lastFilledPendingOrderSlPips = lastFilledPendingOrderInfo.slPips; return(true); } return(false); }
Last Filled Pending Order Take Profit Pips Function
The LastFilledPendingOrderTpPips() function retrieves the take profit pips value of the most recently filled pending order and saves it in the referenced lastFilledPendingOrderTpPips variable. This functionality is valuable for tracking the take profit levels of executed pending orders and can be used for performance analysis or strategy adjustments.
To implement this, we first declare a PendingOrderData variable named lastFilledPendingOrderInfo. This variable is used to temporarily hold the details of the most recently filled pending order. We then call the GetLastFilledPendingOrderData() function to retrieve the order's details. If the retrieval is successful, the tpPips value is extracted from lastFilledPendingOrderInfo and stored in the lastFilledPendingOrderTpPips variable. The function then returns true. If the retrieval fails, the referenced variable remains unchanged, and the function returns false to indicate the failure.
Here is the complete implementation of the LastFilledPendingOrderTpPips() function.
bool LastFilledPendingOrderTpPips(int &lastFilledPendingOrderTpPips) export { PendingOrderData lastFilledPendingOrderInfo; if(GetLastFilledPendingOrderData(lastFilledPendingOrderInfo)) { lastFilledPendingOrderTpPips = lastFilledPendingOrderInfo.tpPips; return(true); } return(false); }
Last Filled Pending Order Time Setup Function
The LastFilledPendingOrderTimeSetup() function retrieves the time when the most recently filled pending order was set up. It stores this time in the referenced lastFilledPendingOrderTimeSetup variable. This function is essential for tracking when specific pending orders were initiated, enabling time-based analysis of order activity.
The LastFilledPendingOrderTimeSetup() function accepts a referenced variable, lastFilledPendingOrderTimeSetup, as input. This variable will hold the setup time of the last filled pending order after the function executes. To achieve this, the function begins by declaring a PendingOrderData variable named lastFilledPendingOrderInfo. This variable is used to temporarily store the details of the last filled pending order.
Next, the GetLastFilledPendingOrderData() function is called to retrieve the details of the last filled pending order. If the retrieval is successful, the timeSetup field from the lastFilledPendingOrderInfo structure is extracted and stored in the lastFilledPendingOrderTimeSetup variable. The function then returns true to indicate success. If the retrieval fails, the function returns false without updating the referenced variable.
Here is the full implementation of the LastFilledPendingOrderTimeSetup() function.
bool LastFilledPendingOrderTimeSetup(datetime &lastFilledPendingOrderTimeSetup) export { PendingOrderData lastFilledPendingOrderInfo; if(GetLastFilledPendingOrderData(lastFilledPendingOrderInfo)) { lastFilledPendingOrderTimeSetup = lastFilledPendingOrderInfo.timeSetup; return(true); } return(false); }
Last Filled Pending Order Time Done Function
The LastFilledPendingOrderTimeDone() function retrieves the time at which the most recently filled pending order was triggered (time done) and saves it in the referenced lastFilledPendingOrderTimeDone variable. This function is essential for tracking the execution times of filled pending orders, allowing you to analyze order timing or create detailed reports.
The function takes the referenced lastFilledPendingOrderTimeDone variable as input to store the time done. It begins by declaring a PendingOrderData variable named lastFilledPendingOrderInfo, which is used to temporarily store the details of the most recently filled pending order.
Next, the function calls GetLastFilledPendingOrderData() to retrieve the data for the last filled pending order. If the operation succeeds, the function extracts the timeDone field from the lastFilledPendingOrderInfo structure and saves it in the referenced lastFilledPendingOrderTimeDone variable. It then returns true to indicate success.
If the retrieval fails, the function does not update the referenced variable and instead returns false to signal that no valid data was found.
Here’s the full implementation of the LastFilledPendingOrderTimeDone() function.
bool LastFilledPendingOrderTimeDone(datetime &lastFilledPendingOrderTimeDone) export { PendingOrderData lastFilledPendingOrderInfo; if(GetLastFilledPendingOrderData(lastFilledPendingOrderInfo)) { lastFilledPendingOrderTimeDone = lastFilledPendingOrderInfo.timeDone; return(true); } return(false); }
Last Filled Pending Order Expiration Time Function
The LastFilledPendingOrderExpirationTime() function retrieves the expiration time of the most recently filled pending order and saves it in the referenced lastFilledPendingOrderExpirationTime variable. This function is useful for managing and analyzing the lifespan of pending orders, particularly when monitoring their validity period.
The function accepts the referenced lastFilledPendingOrderExpirationTime variable as input to store the expiration time. It starts by declaring a PendingOrderData variable named lastFilledPendingOrderInfo, which serves as a temporary container for the details of the most recently filled pending order.
The function then invokes GetLastFilledPendingOrderData() to fetch the data for the last filled pending order. If the operation is successful, it extracts the expirationTime field from the lastFilledPendingOrderInfo structure and saves it in the referenced lastFilledPendingOrderExpirationTime variable. It returns true to indicate the successful retrieval of the data.
If the retrieval fails, the function leaves the referenced variable unchanged and returns false to signal the absence of valid data.
Here’s the full implementation of the LastFilledPendingOrderExpirationTime() function.
bool LastFilledPendingOrderExpirationTime(datetime &lastFilledPendingOrderExpirationTime) export { PendingOrderData lastFilledPendingOrderInfo; if(GetLastFilledPendingOrderData(lastFilledPendingOrderInfo)) { lastFilledPendingOrderExpirationTime = lastFilledPendingOrderInfo.expirationTime; return(true); } return(false); }
Last Filled Pending Order Position ID Function
The LastFilledPendingOrderPositionId() function retrieves the position ID of the most recently filled pending order and saves it in the referenced lastFilledPendingOrderPositionId variable. This function is particularly useful for associating pending orders with their corresponding positions, enabling better tracking and management of trading activity.
The function takes the referenced lastFilledPendingOrderPositionId variable as input to store the position ID. It begins by declaring a PendingOrderData variable named lastFilledPendingOrderInfo, which serves as a temporary container for the details of the most recently filled pending order.
Next, the function calls GetLastFilledPendingOrderData() to fetch the data for the last filled pending order. If the data retrieval is successful, it extracts the positionId field from the lastFilledPendingOrderInfo structure and assigns it to the referenced lastFilledPendingOrderPositionId variable. It then returns true to indicate that the operation was successful.
If the data retrieval fails, the function does not modify the referenced variable and returns false to signal the failure.
Here’s the complete implementation of the LastFilledPendingOrderPositionId() function.
bool LastFilledPendingOrderPositionId(ulong &lastFilledPendingOrderPositionId) export { PendingOrderData lastFilledPendingOrderInfo; if(GetLastFilledPendingOrderData(lastFilledPendingOrderInfo)) { lastFilledPendingOrderPositionId = lastFilledPendingOrderInfo.positionId; return(true); } return(false); }
Last Filled Pending Order Magic Function
The LastFilledPendingOrderMagic() function retrieves the magic number of the most recently filled pending order and saves it in the referenced lastFilledPendingOrderMagic variable. This function is essential for identifying the unique identifier associated with a specific order, especially when managing multiple strategies or systems within the same trading account.
The function takes the referenced lastFilledPendingOrderMagic variable as input to store the magic number. It begins by declaring a PendingOrderData variable named lastFilledPendingOrderInfo, which temporarily holds the details of the most recently filled pending order.
Next, the function calls GetLastFilledPendingOrderData() to retrieve the data for the last filled pending order. If the data retrieval is successful, it extracts the magic field from the lastFilledPendingOrderInfo structure and assigns it to the referenced lastFilledPendingOrderMagic variable. It then returns true to indicate that the operation was successful.
If the data retrieval fails, the function does not modify the referenced variable and returns false to indicate the failure.
Here’s the complete implementation of the LastFilledPendingOrderMagic() function.
bool LastFilledPendingOrderMagic(ulong &lastFilledPendingOrderMagic) export { PendingOrderData lastFilledPendingOrderInfo; if(GetLastFilledPendingOrderData(lastFilledPendingOrderInfo)) { lastFilledPendingOrderMagic = lastFilledPendingOrderInfo.magic; return(true); } return(false); }
Last Filled Pending Order Reason Function
The LastFilledPendingOrderReason() function retrieves the reason for the most recently filled pending order and stores it in the referenced lastFilledPendingOrderReason variable. This function is useful for tracking the specific reason behind the execution of an order. It helps identify whether the order was executed from a mobile, web, or desktop application, triggered by an Expert Advisor or script, or activated by a stop loss, take profit, or stop-out event. This information is crucial for analysis and debugging, providing insights into how and why an order was filled.
The function takes the referenced lastFilledPendingOrderReason variable as input to store the reason. It starts by declaring a PendingOrderData variable named lastFilledPendingOrderInfo, which temporarily holds the details of the last filled pending order.
Next, the function calls GetLastFilledPendingOrderData() to retrieve the data for the last filled pending order. If the data retrieval is successful, it extracts the reason field from the lastFilledPendingOrderInfo structure and assigns it to the referenced lastFilledPendingOrderReason variable. The function then returns true to indicate the operation was successful.
If the data retrieval fails, the function does not modify the referenced variable and returns false to indicate the failure.
Here’s the complete implementation of the LastFilledPendingOrderReason() function.
bool LastFilledPendingOrderReason(ENUM_ORDER_REASON &lastFilledPendingOrderReason) export { PendingOrderData lastFilledPendingOrderInfo; if(GetLastFilledPendingOrderData(lastFilledPendingOrderInfo)) { lastFilledPendingOrderReason = lastFilledPendingOrderInfo.reason; return(true); } return(false); }
Last Filled Pending Order Type Filling Function
The LastFilledPendingOrderTypeFilling() function retrieves the filling type of the most recently filled pending order and stores it in the referenced lastFilledPendingOrderTypeFilling variable. This function is important for determining how the pending order was filled, which could be through a Fill or Kill, Immediate or Cancel, Passive (Book or Cancel), or Return policies.
The function takes the referenced lastFilledPendingOrderTypeFilling variable as input to store the filling type. It begins by declaring a PendingOrderData variable, lastFilledPendingOrderInfo, which temporarily holds the details of the last filled pending order.
Next, the function calls GetLastFilledPendingOrderData() to retrieve the data for the last filled pending order. If the data retrieval is successful, it extracts the typeFilling field from the lastFilledPendingOrderInfo structure and assigns it to the referenced lastFilledPendingOrderTypeFilling variable. The function then returns true to indicate the operation was successful.
If the data retrieval fails, the function does not modify the referenced variable and returns false to indicate the failure.
Here’s the complete implementation of the LastFilledPendingOrderTypeFilling() function.
bool LastFilledPendingOrderTypeFilling(ENUM_ORDER_TYPE_FILLING &lastFilledPendingOrderTypeFilling) export { PendingOrderData lastFilledPendingOrderInfo; if(GetLastFilledPendingOrderData(lastFilledPendingOrderInfo)) { lastFilledPendingOrderTypeFilling = lastFilledPendingOrderInfo.typeFilling; return(true); } return(false); }
Last Filled Pending Order Type Time Function
The LastFilledPendingOrderTypeTime() function retrieves the type time of the most recently filled pending order and stores it in the referenced lastFilledPendingOrderTypeTime variable. This function helps determine the lifetime of the pending order.
The function takes the referenced lastFilledPendingOrderTypeTime variable as input to store the type time. It begins by declaring a PendingOrderData variable, lastFilledPendingOrderInfo, to temporarily hold the details of the last filled pending order.
The function then calls GetLastFilledPendingOrderData() to retrieve the data for the last filled pending order. If the data retrieval is successful, it extracts the typeTime field from the lastFilledPendingOrderInfo structure and assigns it to the referenced lastFilledPendingOrderTypeTime variable. The function then returns true to indicate the operation was successful.
If the data retrieval fails, the function does not modify the referenced variable and returns false to indicate the failure.
Here’s the complete implementation of the LastFilledPendingOrderTypeTime() function.
bool LastFilledPendingOrderTypeTime(datetime &lastFilledPendingOrderTypeTime) export { PendingOrderData lastFilledPendingOrderInfo; if(GetLastFilledPendingOrderData(lastFilledPendingOrderInfo)) { lastFilledPendingOrderTypeTime = lastFilledPendingOrderInfo.typeTime; return(true); } return(false); }
Last Filled Pending Order Comment Function
The LastFilledPendingOrderComment() function retrieves the comment associated with the most recently filled pending order and stores it in the referenced lastFilledPendingOrderComment variable. This function is useful for capturing any additional notes or annotations that may have been added to the pending order.
The function accepts the referenced lastFilledPendingOrderComment variable as input, where it will store the comment. It begins by declaring a PendingOrderData variable, lastFilledPendingOrderInfo, to temporarily hold the details of the last filled pending order.
Next, the function calls GetLastFilledPendingOrderData() to retrieve the data for the last filled pending order. If the data retrieval is successful, it extracts the comment field from the lastFilledPendingOrderInfo structure and assigns it to the referenced lastFilledPendingOrderComment variable. The function then returns true to indicate the operation was successful.
If the data retrieval fails, the function does not modify the referenced variable and returns false to indicate the failure.
Here’s the complete implementation of the LastFilledPendingOrderComment() function.
bool LastFilledPendingOrderComment(string &lastFilledPendingOrderComment) export { PendingOrderData lastFilledPendingOrderInfo; if(GetLastFilledPendingOrderData(lastFilledPendingOrderInfo)) { lastFilledPendingOrderComment = lastFilledPendingOrderInfo.comment; return(true); } return(false); }
Conclusion
We have successfully developed the exportable functions to retrieve and store the properties of the most recently filled pending order, enhancing the resourcefulness of the History Management EX5 library. These new capabilities provide seamless access to critical data about filled pending orders, enabling more straightforward and effective trade analysis and strategy optimization, while strengthening the library’s ability to handle essential pending order history operations.
In the next article, we will expand the library further by adding functions to fetch and store the properties of the most recently canceled pending order. This addition will enhance the library’s ability to manage and analyze trade history, offering even more valuable tools for your trading toolkit.
The updated HistoryManager.mq5 library source code, including all the functions created in this and previous articles, is available at the end of this article. Thank you for following along, and I look forward to continuing this journey with you in the next article.





- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use