Errors, bugs, questions - page 2737

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
In MT4, while debugging indicators, the debugger hangs permanently if you switch to a chart.
Reproduced, for example, during start of debugging of standard CCI indicator.
1. Set a breakpoint;
2. Press F5;
3. Switch to the graph.
Result - the debugger graph hangs.
You can also simply press F5 several times during debugging - the chart hangs.
Build 1260.
Debugger settings:
The search result deletes some of the text.
Here is the original.
Forum on trading, automated trading systems and strategy tester
MetaTrader 5 Strategy Tester: bugs, bugs, suggestions for improvement
fxsaber, 2020.05.11 20:31
It probably doesn't make sense for Tester to create opt-files that have Header.passes_passed== 0.As it turned out, setting the background colour is not possible for an object of OBJ_LABLE type, it is necessary to use OBJ_EDIT.
When using OBJ_EDIT, a new problem has arisen - the need to set the size of OBJPROP_XSIZE and OBJPROP_YSIZE so that all text fits into the corresponding object dimensions.
Question: how to determine the OBJPROP_XSIZE and OBJPROP_YSIZE sizes to fit the whole text?
I considered two options:
1. Creating OBJ_LABLE object, reading its dimensions, deleting OBJ_LABLE object.
Not suitable because dimensioning is only possible after the object has actually been created and is not possible when the object is in the ChartRedraw queue.
2. using TextSetFont followed by TextGetSize.
Not suitable, because the result is radically different from the results of method #1, the difference of 2.5 - 2.9 times, depending on the size of the font.
Probably the reason is 4K monitor and 175% DPI.
Thanks toGeess for thesolution.
It is necessary to multiply by -10 the size of the shuffle when passing it to TextSetFont.
I rarely work with graphical objects, I need to paint the background color for OBJ_LABLE (set OBJPROP_BGCOLOR).
As it turned out, setting the background colour is not possible for an object of OBJ_LABLE type, it is necessary to use OBJ_EDIT.
When using OBJ_EDIT, a new problem has arisen - the need to set the size of OBJPROP_XSIZE and OBJPROP_YSIZE so that all text fits into the corresponding object dimensions.
Question: how to determine the OBJPROP_XSIZE and OBJPROP_YSIZE sizes to fit the whole text?
I have considered two options:
1. Create OBJ_LABLE object, read the dimensions, delete OBJ_LABLE object.
Not suitable because dimensioning is only possible after the object has actually been created and is not possible when the object is in the ChartRedraw queue.
2. using TextSetFont followed by TextGetSize.
Not suitable, because the result is radically different from the results of method #1, the difference of 2.5 - 2.9 times, depending on the size of the font.
Probably the reason is 4K monitor and 175% DPI.
Firstly, text size and object size are not the same thing. At the very least there has to be a border. And therefore these values can't coincide.
Secondly, it is better to use OBJ_BITMAP_LABEL which has no limitations.
And if you do use it, it is better to use CCanvas class.
The result is the same object, only with more possibilities. For example, by adding transparency to the text label.
Firstly, text size and object size are not the same thing. At a minimum, there must be a border. And so these values cannot coincide.
Thank you very much for your help.
You are adapting a ready-made solution to your own needs, so I don't see the need to implement libraries.
The solution you originally proposed can be represented as:
Unfortunately, because of a defect in MT5 - the proposed solution cannot be used normally.
The text size turns out to be 3 times smaller than needed on a 4K monitor with 175% Windows DPI.
I have to multiply font size by DPI / 100% * [1.6 ... 1.8]
ThanksGeessfor thesolution.
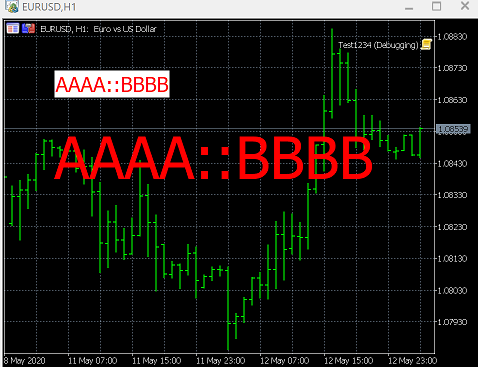
You have to multiply the font size by -10 when passing it toTextSetFont.
Thank you very much for your help.
You are adapting a ready-made solution to your own needs, so I don't see the need to implement libraries.
The solution you originally proposed can be represented as:
Unfortunately, because of a defect in MT5 - the proposed solution cannot be used normally.
The text size turns out to be 3 times smaller than needed on a 4K monitor with 175% Windows DPI.
I have to multiply font size by DPI / 100% * [1.6 ... 1.8].
https://www.mql5.com/ru/docs/objects/textsetfont

you have to do it like this:
I don't understand why you need OBJ_LABEL so much? You have implemented a variant without libraries with OBJ_BITMAP_LABEL. What is its advantage? I see only a limitation.
https://www.mql5.com/ru/docs/objects/textsetfont
I don't understand why you need OBJ_LABEL so much? You have implemented a variant without libraries with OBJ_BITMAP_LABEL. What is its advantage? I see only a limitation.
Thank you very much again.
Didn't know, didn't see, didn't read, about multiplication by -10. Problem solved.
OBJ_LABEL was used to illustrate the problem and be able to reproduce it.
Please tell me what the problem is.
Different indicators, which are working correctly and updating, start to show something different in sync, not based on the price chart displayed in the main window. It happens occasionally, not every day.
At first I blamed on indicators, but after trying different ones, including MT5 native ones, I am suspicious about the terminal. I observe the problem for a long time, since last year, on different versions of the terminal. First it was on Alpari custom version, now it is the same on the original version. Both on demo account and on real ecn.
Alpari broker. MT5 build 2363 from 13.03.2020. I don't remember on other periods, but it definitely happens on M1.
Screenshots:
"Slipped" variant. Except for the zigzag, all indicators are built in. The zigzag with correct work with history. Readings of indicators are convergent among themselves. They do not correspond with prices.
After the update.
MT5 version
Afternoon.
Stumbled across an incomprehensible thing and don't understand what it is.
There are two functions which are used in different strategies. Logically, the code in checks like
It should not be executed if one of the conditions is wrong. But for some reason it is executed if the magic number and the magic number passed into the function are NOT EQUAL.
It seems to be an integer type comparison. I cannot understand why. You can see it in the screenshot below.