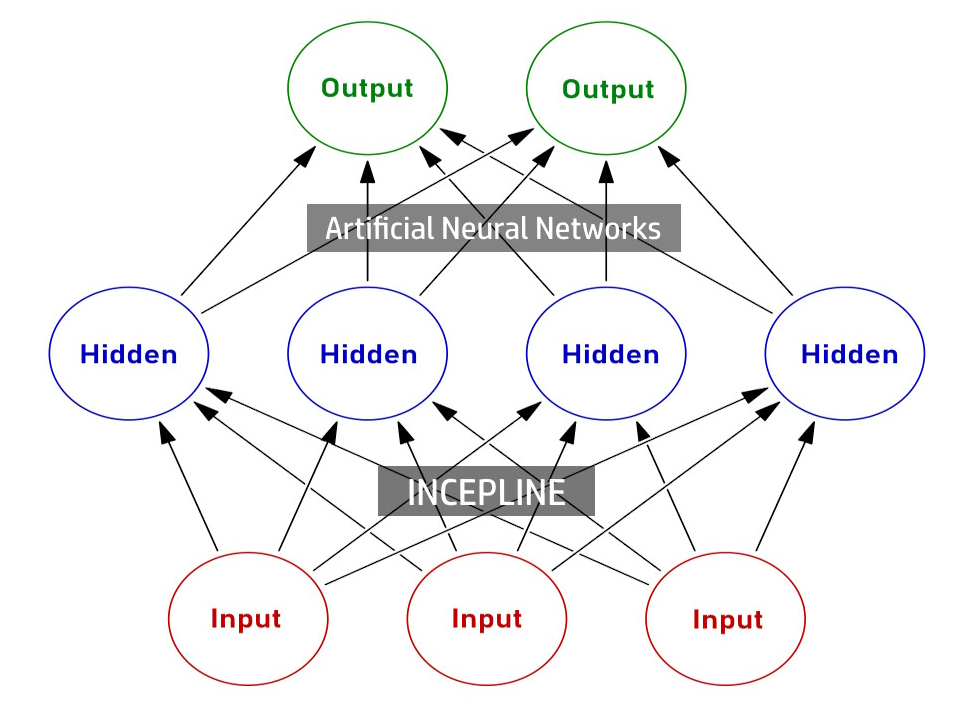
1.1 Operation of neural networks
1.2 Types of neural networks used in finance
1.3 Advantages of neural networks in trading
Adaptability: Neural networks can adapt to new data and market changes, making them relevant to changing financial environments.
Now let's look at some simple examples on the technical side, for example with Python for creating neural networks.
Three possibilities:
1/ Let's use TensorFlow to create a sequential neural network. The model has three layers: an input layer with 10 neurons (as we have 10 features in the example data), a hidden layer with 64 neurons and a ReLU activation function, another hidden layer with 32 neurons and a ReLU activation function, and finally an output layer with 1 neuron and a sigmoid activation function for binary classification.
The model is then compiled with the Adam optimizer and binary_crossentropy loss function, and we train it using the training data.
pip install tensorflow
import tensorflow as tf from tensorflow.keras.models import Sequential from tensorflow.keras.layers import Dense # Génération de données d'exemple from sklearn.datasets import make_classification from sklearn.model_selection import train_test_split # Générer des données d'exemple X, y = make_classification(n_samples=1000, n_features=10, random_state=42) # Diviser les données en ensembles d'entraînement et de test X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Création du modèle du réseau de neurones model = Sequential() model.add(Dense(units=64, activation='relu', input_dim=10)) model.add(Dense(units=32, activation='relu')) model.add(Dense(units=1, activation='sigmoid')) # Compilation du modèle model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy']) # Entraînement du modèle model.fit(X_train, y_train, epochs=10, batch_size=32, validation_data=(X_test, y_test))
Please note that this is a simple example to illustrate the creation of a neural network. In a trading context, you will need to adapt the model's architecture and parameters to your specific needs. Additionally, data preprocessing, time series data management, and other considerations will also need to be taken into account for a more sophisticated business model.
2/ Now let's use PyTorch to create a simple feedforward neural network for binary classification. The model has three fully connected (linear) layers with ReLU activations between them and sigmoid activation at the output layer.
The model is trained using binary cross-entropy loss and the Adam optimizer. We loop through the training data in batches and update the model parameters by backpropagation.
pip install torch
import torch import torch.nn as nn import torch.optim as optim import numpy as np # Generate sample data from sklearn.datasets import make_classification from sklearn.model_selection import train_test_split # Generate example data X, y = make_classification(n_samples=1000, n_features=10, random_state=42) # Convert data to PyTorch tensors X = torch.tensor(X, dtype=torch.float32) y = torch.tensor(y, dtype=torch.float32) # Split data into training and test sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Define the neural network model class NeuralNetwork(nn.Module): def __init__(self): super(NeuralNetwork, self).__init__() self.layer1 = nn.Linear(10, 64) self.layer2 = nn.Linear(64, 32) self.layer3 = nn.Linear(32, 1) self.activation = nn.ReLU() self.output_activation = nn.Sigmoid() def forward(self, x): x = self.activation(self.layer1(x)) x = self.activation(self.layer2(x)) x = self.output_activation(self.layer3(x)) return x # Create the model instance model = NeuralNetwork() # Define the loss function and optimizer criterion = nn.BCELoss() optimizer = optim.Adam(model.parameters(), lr=0.001) # Training loop num_epochs = 10 batch_size = 32 for epoch in range(num_epochs): for i in range(0, len(X_train), batch_size): batch_X = X_train[i:i + batch_size] batch_y = y_train[i:i + batch_size] optimizer.zero_grad() outputs = model(batch_X) loss = criterion(outputs, batch_y.view(-1, 1)) loss.backward() optimizer.step() print(f"Epoch [{epoch+1}/{num_epochs}], Loss: {loss.item():.4f}") # Evaluate the model with torch.no_grad(): predictions = model(X_test) predicted_labels = (predictions >= 0.5).float() accuracy = (predicted_labels == y_test.view(-1, 1)).sum().item() / len(y_test) print(f"Test Accuracy: {accuracy:.4f}")
Finally, we evaluate the accuracy of the model trained on the test data.
Remember that this is still a basic example. In a trading context, you will need to adjust model architecture and parameters, manage financial time series data, and implement risk management strategies to create a reliable trading system.
3/ In this example, we use Keras to create a sequential neural network for regression. The architecture of the model is similar to previous examples, with three layers: an input layer with 10 neurons, two hidden layers with ReLU activations, and an output layer for regression tasks.
The model is compiled with the Adam optimizer and the mean squared error loss function, which is commonly used for regression tasks.
pip install keras
import numpy as np from sklearn.datasets import make_regression from sklearn.model_selection import train_test_split from keras.models import Sequential from keras.layers import Dense # Generate example data X, y = make_regression(n_samples=1000, n_features=10, noise=0.1, random_state=42) # Split data into training and test sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Create the neural network model model = Sequential() model.add(Dense(units=64, activation='relu', input_dim=10)) model.add(Dense(units=32, activation='relu')) model.add(Dense(units=1)) # Compile the model model.compile(optimizer='adam', loss='mean_squared_error') # Train the model model.fit(X_train, y_train, epochs=10, batch_size=32, validation_data=(X_test, y_test))
We then train the model using the training data.
Remember again that this example is simplified for demonstration purposes.
These examples given in raw with Python, are not directly compatible with MQL5, but you can potentially use external libraries to bridge the communication between the two languages. A common approach is to create a dynamic link library (DLL) in a language like C/C++ that can be called from MQL5 to interact with Python code. However, this process can be quite complex for a beginner.
If you are looking for a trading robot including the latest technologies, please visit my profile:
https://www.mql5.com/en/users/incepline
Robot trading BotAGI open source MT4 – MT5
Get your personal metatrader trading robot !
License and rights included : Click here
If you want to learn a bit more about how to pair Python with MQL5, keep reading.
I can provide you with a high-level overview of the steps you could take to create a DLL for this purpose, but keep in mind that this involves more advanced programming concepts:
Create a C/C++ DLL:
You would need to write C/C++ code to create a DLL that acts as an interface between MQL5 and your Python code. This DLL should include functions that can be called from MQL5 and that interact internally with your Python code.
Use the Python C API:
In your C/C++ DLL, you can use the Python C API to embed Python code. This allows you to run Python functions and scripts from your DLL.
Compile the DLL:
Compile the C/C++ code to create the DLL file that MQL5 can use.
Import the DLL into MQL5:
In your MQL5 code, you can use the #import directive to import functions from the DLL. These functions will essentially act as bridges between your MQL5 code and the Python code running in the DLL.
Call the Python code:
You would call functions imported from MQL5, which in turn execute Python code through the DLL.
This is a high level overview of the process. Creating a DLL that communicates effectively between MQL5 and Python requires a solid understanding of both languages, as well as experience with inter-process communication and API usage.
If you are relatively new to programming or it seems daunting, you might want to consider buying a trading robot outright.
Check out my profile below:
https://www.mql5.com/en/users/incepline
Thank you for reading this article.