-
Why did you post your MT4 question in the MT5 General section instead of the MQL4 section, (bottom of the Root page)?
General rules and best pratices of the Forum. - General - MQL5 programming forum? (2017)
Next time, post in the correct place. The moderators will likely move this thread there soon. - Kosei S: How to display RSI for only specified of bars?
Why did you post the RSI indicator? You use iRSI to read its value into your indicator.
-
You have only four choices:
-
Search for it (CodeBase or Market). Do you expect us to do your research for you?
- Try asking at:
- Coding help - MQL4 programming forum
- Make It No Repaint Please! - MQL4 programming forum
- MT4 to MT5 code converter - MQL5 programming forum
- Please fix this indicator or EA - General - MQL5 programming forum
- Requests & Ideas (MQL5 only!) - Expert Advisors and Automated Trading - MQL5 programming forum
- Indicator to EA Free Service - General - MQL5 programming forum
- I will write an advisor free of charge - Expert Advisors and Automated Trading - MQL5 programming forum
-
MT4: Learn to code it.
MT5: Begin learning to code it.If you don't learn MQL4/5, there is no common language for us to communicate. If we tell you what you need, you can't code it. If we give you the code, you don't know how to integrate it into your code.
-
Or pay (Freelance) someone to code it. Top of every page is the link Freelance.
Hiring to write script - General - MQL5 programming forum (2019)
We're not going to code it for you (although it could happen if you are lucky or the problem is interesting.) We are willing to help you when you post your attempt (using CODE button) and state the nature of your problem.
No free help (2017) -
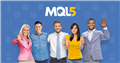
- www.mql5.com
input int Max_Bars = 500; for(i=pos; i<Max_Bars&& !IsStopped(); i++)
An RSI uses an EMAs in its calculations. You can't reduce RSI calculations just to a fixed number of bars without compromising its results.
The following post also applies to your case ...
Forum on trading, automated trading systems and testing trading strategies
Chaikin Volatility in Expert only current bar
Fernando Carreiro, 2023.06.14 00:51
You can't run an exponential moving average in the same way as a simple moving average.
An EMA requires at least 3.45×(Length+1) bars for 99.9% convergence. So for an EMA(30) you require at least 107 bars.
You will actually need much more to get the exact same value as the build in indicator.
Please read the following post ... https://www.mql5.com/en/forum/446533/page2#comment_46578197
An RSI uses an EMAs in its calculations. You can't reduce RSI calculations just to a fixed number of bars without compromising its results.
The following post also applies to your case ...
I see. So the formula for RSI is (A/A+B)*100 A=average uptrend B=average downtrend
can I get approximate results with own calculations?
double diff = 0; double RSI_A = 0; double RSI_B = 0; for(int i=0; i<=13; i++){ diff = Close[i] - Close[i-1]; if(diff>0) RSI_A+=diff; else RSI_B-=diff; RSI[0]= (RSI_A/RSI_A+RSI_B)*100; }
I can caluculate the RSI of the current bar, but I cannot caluculate the RSI of the past. Do you have any good ideas?
for(int i=0; i<Max_Bars; i++)
is it possible?
First ask, yourself — why do you want to do this?
Is it to reduce the "load" when calculating RSI in an EA?
If yes, then the using an EMA is the perfect solution given that it is an incremental calculation. Instead of using the indicator, simply calculate the RSI incrementally in your EA.
Or is there another reason why you want this?

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I would like to display only the specified bars by RSI in order to lighten the indicator, but it is not displayed well. How to display RSI for only specified of bars?