Martingale is not a strategy. It's a betting system.
Hedging, grid trading, same as Martingale.
Martingale, Hedging and Grid : MHG - General - MQL5 programming forum (2016)
Martingale, guaranteed to blow your account eventually. If your strategy is not profitable without, it is definitely not profitable with.
Martingale vs. Non Martingale (Simplified RoR vs Profit and the Illusions) - MQL5 programming forum (2015)
Why it won't work:
Calculate Loss from Lot Pips - MQL5 programming forum (2017)
THIS Trading Strategy is a LIE... I took 100,000 TRADES with the Martingale Strategy - YouTube (2020)
Martingale is not a strategy. It's a betting system.
Hedging, grid trading, same as Martingale.
Martingale, Hedging and Grid : MHG - General - MQL5 programming forum (2016)
Martingale, guaranteed to blow your account eventually. If your strategy is not profitable without, it is definitely not profitable with.
Martingale vs. Non Martingale (Simplified RoR vs Profit and the Illusions) - MQL5 programming forum (2015)
Why it won't work:
Calculate Loss from Lot Pips - MQL5 programming forum (2017)
THIS Trading Strategy is a LIE... I took 100,000 TRADES with the Martingale Strategy - YouTube (2020)
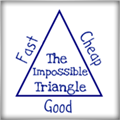
I think that it has something to do with your counting of orders … you are counting ++ or — if OrdersStopLoss() == OrderClosedPrice(). You have 2 doubles which are rarely equal… change that
for (int i = OrdersHistoryTotal() - 1; i >= 0; i--)to this
for (int i = 0; i < OrdersHistoryTotal(); i++)
i think its work now, need to check further more, but thanks for give me a hint, really appreciate it, many thanks
Paste these macros at the top of the mq5 file:
#define nearlyEqual(x, y) MathAbs(x - y) < 1.0e-8 #define EQ(x, y) (x == y || nearlyEqual(x, y)) /* Is Equal */ #define NE(x, y) (x != y && !nearlyEqual(x, y)) /* Is Not Equal */ #define GT(x, y) (x > y && !nearlyEqual(x, y)) /* Is Greater Than */ #define LT(x, y) (x < y && !nearlyEqual(x, y)) /* Is Less Than */ #define GTE(x, y) (x >= y || nearlyEqual(x, y)) /* Is Greater Than or Equal */ #define LTE(x, y) (x <= y || nearlyEqual(x, y)) /* Is Less Than or Equal */
old code that needs to be changed:
if (OrderSelect(i, SELECT_BY_POS, MODE_HISTORY) == true && OrderSymbol() == _Symbol && OrderMagicNumber() == MagicNumber) { if (OrderClosePrice() == OrderStopLoss()) { lossCount++; } else if (OrderClosePrice() == OrderTakeProfit()) { lossCount = 0; } }
to a new code that to handles the comparisons of doubles, nicely:
if (OrderSelect(i, SELECT_BY_POS, MODE_HISTORY) == true && OrderSymbol() == _Symbol && OrderMagicNumber() == MagicNumber) { if ((OrderType == OP_BUY && LTE(OrderClosePrice(), OrderStopLoss())) || (OrderType == OP_SELL && GTE(OrderClosePrice(), OrderStopLoss()))) { lossCount++; } else if ((OrderType == OP_BUY && GTE(OrderClosePrice(), OrderTakeProfit())) || (OrderType == OP_SELL && LTE(OrderClosePrice(), OrderTakeProfit()))) { lossCount = 0; } }
see https://www.mql5.com/en/code/25723
-
else if ((OrderType == OP_BUY && GTE(OrderClosePrice(), OrderTakeProfit()))
TP/SL become market orders when hit. That comparison may not work. Perhaps, try isTP = |OCP-OTP| < |OCP-OSL|
-
Why did you post your MT4 question in the MT5 EA section instead of the MQL4 section, (bottom of the Root page)?
General rules and best pratices of the Forum. - General - MQL5 programming forum? (2017)
Next time, post in the correct place. The moderators will likely move this thread there soon.
-
TP/SL become market orders when hit. That comparison may not work. Perhaps, try isTP = |OCP-OTP| < |OCP-OSL|
Market orders such as TP and SL are subject to slippage. Therefore, a buy order has its OderClosePrice() >= OrderTakeProft(), if it has positive slippage (it can give false results with negative slippage).
isTP = |OCP-OTP| < |OCP-OSL| is also not precise! The latter condition might also be fulfilled for any manually-closed order (i.e. a non-specific test).
NB: on MT4 it is difficult to differentiae between orders closed by TP or SL from manually closed orders.
Edited: I think this will be a better test:
else if ((OrderType() == OP_BUY && GTE(OrderClosePrice(), OrderTakeProfit())) || (OrderType() == OP_SELL && LTE(OrderClosePrice(), OrderTakeProfit())) || (StringFind(OrderComment(), "TP") > 0)) { lossCount = 0; }
Market orders such as TP and SL are subject to slippage. Therefore, a buy order has its OderClosePrice() >= OrderTakeProft(), if it has positive slippage (it can give false results with negative slippage).
isTP = |OCP-OTP| < |OCP-OSL| is also not precise! The latter condition might also be fulfilled for any manually-closed order (i.e. a non-specific test).
NB: on MT4 it is difficult to differentiae between orders closed by TP or SL from manually closed orders.
Edited: I think this will be a better test:
OrderType == OP_BUY && GTE(OrderClosePrice(), OrderTakeProfit())
And what about the slippage you talked about ? The close price can be smaller than the TP.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello guys, nice to meet you, sory for my bad english, i just started to code EA two weeks ago, and i need some help with my martingale strategy, i've been struggling for almost a week now. Short story, my code is execute an order when MA is cross, either up or down, and when order hit stop loss it will open reverse order immediately with increase lot size. below is my code.
it works for the first order with startMarti set to 1 and martiMultiplier set to 2, so the it will open order like 0.01, 0.02, 0.04, 0.08, but if i increase the start marti example 2, it should be like 0.01, 0.01, 0.02, 0.02, 0.04, 0.04, 0.08 and so on, but the results is when it hit take profit for the first time, the first order is right with lot 0.01 for the second order it directly increase to 0.02, below is my results for the EA
as you can see at order #12 it hit stop loss then at order #13 should open with 0.01 lot. can somebody tell me what did i do wrong, or my logic is still wrong, or somebody can teach me more?
thanks before, appreciate you guys answer