New coder can anyone see anything obviously wrong with this code, its is opening and closing trades on every candle but does seem to be loading the indicators. Thanks.
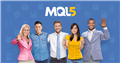
- 2023.02.05
- www.mql5.com
- im newbee here, i ran this code and keep saying "erorr 4051", idk whats wrong with this code, i already google it, and still confuse
- order error 138. code attached help would be appreciated
- ordersend error 138... any advice would be appreciated
-
Why did you post your MT4 question in the MT5 EA section instead of the MQL4 section, (bottom of the Root page)?
General rules and best pratices of the Forum. - General - MQL5 programming forum? (2017)
Next time, post in the correct place. The moderators will likely move this thread there soon. -
Please edit your (original) post and use the CODE button (or Alt+S)! (For large amounts of code, attach it.)
General rules and best pratices of the Forum. - General - MQL5 programming forum (2019)
Messages Editor - Chad Michael:
New coder can anyone see anything obviously wrong with this code, its is opening and closing trades on every candle but does seem to be loading the indicators. Thanks.
A title should be short. Post your question in the post.
-
openOrderID = OrderSend(NULL,OP_SELL,0.01,Bid,10,0,0,NULL,magicNB);
Be careful with NULL.
- On MT4, you can use NULL in place of _Symbol only in those calls that the documentation specially says you can. iHigh does, iCustom does, MarketInfo does not, OrderSend does not.
- Don't use NULL (except for pointers where you explicitly check for it.) Use _Symbol and _Period, that is minimalist as possible and more efficient.
- Zero is the same as PERIOD_CURRENT which means _Period. Don't hard code numbers.
- MT4: No need for a function call with iHigh(NULL,0,s) just use the predefined arrays, i.e. High[].
- Cloud Protector Bug? - MQL4 programming forum (2020)
-
if(OrderSelect(openOrderID,SELECT_BY_TICKET)==true) {
You select by ticket. You don't check if the ticket has already closed.
-
EAs must be coded to recover. If the power fails, OS crashes, terminal or chart is accidentally closed, on the next tick, any static/global ticket variables will have been lost. You will have an open order but don't know it, so the EA will never try to close it, trail SL, etc. How are you going to recover?
Use a OrderSelect / Position select loop on the first tick, or persistent storage (GV+flush or files) of ticket numbers required.
On a network disconnection you might get ERR_NO_RESULT or ERR_TRADE_TIMEOUT. There is nothing to be done, but log the error, return and wait for a reconnection and a new tick. Then reevaluate. Was an order opened or is the condition still valid.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use