- Market Condition Evaluation based on standard indicators in Metatrader 5
- Problems 2 buffers indicators divide
- How to calculate an indicator for Max number of Bars
Hello, I have an indicator that works on the basis of several timeframes. However, I have the problem that the buffers are not output correctly. I tried to find the problem but it didn't work. Would be grateful for help.
Your 'limit' is based on the chart's timeframe, which cannot be directly used to access candle data of other timeframes.
Take a look at the illustration in this post : https://www.mql5.com/en/forum/315648#comment_12047919 - I was trying to explain the implications of making this mistake.
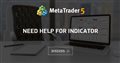
- 2019.06.12
- www.mql5.com
How could I solve the problem?
Simple question, but complex answer... LOL.
I'll need to know more about your requirements before I can answer your question.
But generally, you cannot, meaningfully, display information from smaller timeframes on a chart of larger timeframe. So you cannot expect to show M1, M5, M15, etc. data, bar by bar, on H1 chart. However, displaying larger timeframe information on smaller timeframe chart is ok.
So if your chart displays M1, it is ok to declare buffers for M5, M15, and so on although you'll need to make sure their bar times are synchronized. But if your chart is H1, you cannot declare buffers for M1, M5, etc. because it becomes meaningless since 24 H1 bars cover 1 day, but 24 M1 bars will only cover 24 minutes - so using M1[20] to compute H1[20] is completely wrong. However, nothing stops you from retrieving 24x60 bars of M1 data for internal computation... in which case they should be stored in normal arrays 60 times larger, rather than indicator buffers.
In other words, to solve the problem you're having now, you'll have to think through your requirements first, before designing and implementing your code.
Hi they can help you quickly over here https://www.mql5.com/en/job
If you ask they can tell you how and what they do because it is a lot of code and need some work to fix it.
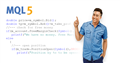
- www.mql5.com
Then code it yourself. If you don't know how to code, you can't possibly know how big it is.
You haven't stated a problem, you stated a want. You have only four choices:
- Search for it,
- Beg at
- Coding help - MQL4 programming forum
- Requests & Ideas (MQL5 only!) - Trade FX - Expert Advisors and Automated Trading - MQL5 programming forum
- Free coding for your trading system.. - Easy Trading Strategy - General - MQL5 programming forum
- I will code & automate your strategy for free - Options Trading Strategies - General - MQL5 programming forum
- Make It No Repaint Please! - MetaTrader 5 - MQL4 programming forum
- learn to code it. If you don't learn MQL4/5, there is no common language for us to communicate. If we tell you what you need, you can't code it. If we give you the code, you don't know how to integrate it into yours.
- or pay (Freelance) someone to code it.
No free help
urgent help.
Yes, but my problem isn't that big.
Your problem is very big.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use