You can't run trading code in an Indicator, so you now have to develop an EA which will use your indicator signals to open and/or close trades. In order to get the Indicator data/signals you have to use the iCustom() function.
However, I recommend the following book to help you on your journey. There are probably many other books for the same purpose, but this is the one the helped me when I started off.
Instead of ploughing through the reference documentation and examples in the CodeBase (many of which are incorrectly done, because they were coded by newbies too), the book will follow a more logical approach from start to finish, but you should always reference the documentation for more details.
Forum on trading, automated trading systems and testing trading strategies
Sergey Golubev, 2017.09.16 05:40
Expert Advisor Programming for MetaTrader 4
This book will teach you the following concepts:
- The basic of the MLQ4 language, including variables and data types, operations, conditional and loop operators, functions, classes and objects, event handlers and more.
- Place, modify and close market and pending orders.
- Add a stop loss and/or take profit price to an individual order, or to multiple orders.
- Close orders individually or by order type.
- Get a total of all currently opened orders.
- Work with OHLC bar data and locate basic candlestick patterns.
- Find the highest high and lowest low of recent bars.
- Work with MetaTrader’s built-in indicators, as well as custom indicators.
- Add a trailing stop or break even stop feature to an expert advisor.
- Use money management and lot size verification techniques.
- Add a flexible trading timer to an expert advisor.
- Construct several types of trading systems, including trend, counter-trend and breakout systems.
- Add alert, emails, sounds and other notifications.
- Add and manipulate chart objects.
- Read and write to CSV files.
- Construct basic indicators, scripts and libraries.
- Learn how to effective debug your programs, and use the Strategy Tester to test your strategies.
All of the source code in this book is available for download, including an expert advisor framework that allows you to build robust and fully-featured expert advisors with minimal effort.
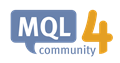
- docs.mql4.com
The easiest thing is for you to leave the indicator as it is and create an EA that uses the signals given by that indicator. To do this, you should use the iCustom function to receive the indicator values in the EA. Obviously, all commercial functions must go within the EA.
For mql4: https://docs.mql4.com/indicators/icustom
For mql5: https://www.mql5.com/en/docs/indicators/icustom
Regards.
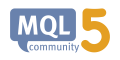
- www.mql5.com
Hello again. I have been playing around with the iCustom function as well as reading as much information as I could on it. It seems to work, but only partly. I'm now having this incredibly annoying thing where only part of my code is working and I cannot for the life of me figure out why. Here is the EA code:
#include <stdlib.mqh> #include <stderror.mqh> //External Inputs for Indicator extern double EntryThreshold=11; extern int MagicNumber=12345; extern double Lots=1; extern double StopLoss=15; extern double TakeProfit=50; extern int Slippage=3; extern int Expiration=14400; //External Input for Trailing Stop extern int TrailingStop=15; //Variable Declaration double PointValue = 0; double Spread = 0; double Trigger = 0; // 0 = None, 1 = Buy, 2 = Sell //+-------------------------+ //| Expert Advisor Function | //+-------------------------+ int start() { PointValue = (Point*10); NormalizeDouble(PointValue,Digits); Spread = (MarketInfo(Symbol(), MODE_SPREAD)/10); NormalizeDouble(Spread,1); Trigger = iCustom(NULL,0,"Turtle",4,0); if (Spread > 0) { if(Trigger == 1) { Print("Buy @ ",Bid); Print("Trigger = ",Trigger); } if(Trigger == 2) { Print("Sell @ ",Bid); Print("Trigger = ",Trigger); } } }
At the moment it's just following the Indicator and printing a message when it gets triggered. The Indicator itself is setting the buffer "Trigger" to either 1 or 2, depending on if it's a buy or sell signal. However something rather weird is happening, in that it's only triggering on the sell signals. I know that the buy signals are being generated, because I made the indicator tell me the value of "Trigger" each time it was generated. So I think something is going wrong somewhere but after staring at it and trying to trace it for a day now I can't seem to track down the problem. Any ideas?
Regards,
Nik
At the moment it's just following the Indicator and printing a message when it gets triggered. The Indicator itself is setting the buffer "Trigger" to either 1 or 2, depending on if it's a buy or sell signal. However something rather weird is happening, in that it's only triggering on the sell signals. I know that the buy signals are being generated, because I made the indicator tell me the value of "Trigger" each time it was generated. So I think something is going wrong somewhere but after staring at it and trying to trace it for a day now I can't seem to track down the problem. Any ideas?
Judging from your code, it seems obvious that you are not reading the book. Can you confirm?
That is correct, I can't afford it at the moment so I decided to try and do it from online help topics instead.
It's also worth mentioning that this is nowhere near the finished code, I'm writing it stepwise to make sure that I spot any mistakes more easily, since I'm not used to looking at code, at least in this language.
I will be honest and blunt here - if you can't afford $20 for an eBook to help develop your skills and knowledge, then you certainly should not be contemplating trading Forex or any other market, both from a "required Capital or Funds" point of view as well as from a "required mindset for a trader" point of view.
Well, I would think that when an experienced programmer and trader, recommends a book, that he himself read, that it should count for something, but obviously, you are free to do as you believe best!

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi guys,
So this is my first time posting on this forum. I have only a very small amount of coding ability, but after a few weeks of reading, trial and error, and trying hard to remember what I learned in school I have manged to write an indicator that gives fairly good entry signals according to the strategy that I want to use. Now I want to automate it, so instead of painting an arrow and sending me an email I want it to just go ahead and place a limit order at a set price. So I assumed that the code that determines when to place the arrow would also work in determining when to place the order. But it just doesn't work. I'm sure this is a fairly n00b question, but what am I missing? I obviously know that the code needs to be run as an EA rather than an indicator (since the MT4 terminal told me that OrderSend cannot be run from an indicator). But I can't figure out why the code won't work, when it works perfectly well elsewhere. So anyway, I am now ready to farm this out to the internet hive mind.
I hope someone will be able to help me.
Regards,
Nik