I'm using a struct for collecting the data which includes spreads and their instances.
The thing needed is a sorting method for these two variables in the struct by either descending or ascending.
If I'm not wrong, you have only two options when it comes to sorting arrays...
(1) Use ArraySort (https://www.mql5.com/en/docs/array/arraysort), which is not useful if you want to select which of the fields in your struct to be sorted.
(2) Use CArray (https://www.mql5.com/en/docs/standardlibrary/datastructures/carray), but you'll have to define your own sort routine.
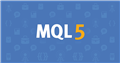
- www.mql5.com
(1) Use ArraySort (https://www.mql5.com/en/docs/array/arraysort), which is not useful if you want to select which of the fields in your struct to be sorted.
(2) Use CArray (https://www.mql5.com/en/docs/standardlibrary/datastructures/carray), but you'll have to define your own sort routine.
- ArraySort only processes n dimensional arrays by the first dimension. It can not process structs at all.
- Here's my generic sort.
Sort multiple arrays - MQL4 and MetaTrader 4 - MQL4 programming forum
Array or not to array - Trading Positions - MQL4 and MetaTrader 4 - MQL4 programming forum - Page 2 - CList can also sort.
pass generic class data field - Swing Trades - MQL4 and MetaTrader 4 - MQL4 programming forum
Forum on trading, automated trading systems and testing trading strategies
How to sort struct variables in MT4?
Brian Lillard, 2019.05.01 05:03
I'm using a struct for collecting the data which includes spreads and their instances.
struct _buffer{int spread; int instances;}; _buffer WellingtonSpread[];
The thing needed is a sorting method for these two variables in the struct by either descending or ascending.
Форум по трейдингу, автоматическим торговым системам и тестированию торговых стратегий
Особенности языка mql5, тонкости и приёмы работы
fxsaber, 2019.05.01 17:30
Удобная сортировка массива структур#property strict // Сортировка массива структур и указателей на объекты по (под-) полю/методу. #define ArraySortStruct(ARRAY, FIELD) \ { \ class SORT \ { \ private: \ template <typename T> \ static void Swap( T &Array[], const int i, const int j ) \ { \ const T Temp = Array[i]; \ \ Array[i] = Array[j]; \ Array[j] = Temp; \ \ return; \ } \ \ template <typename T> \ static int Partition( T &Array[], const int Start, const int End ) \ { \ int Marker = Start; \ \ for (int i = Start; i <= End; i++) \ if (Array[i].##FIELD <= Array[End].##FIELD) \ { \ SORT::Swap(Array, i, Marker); \ \ Marker++; \ } \ \ return(Marker - 1); \ } \ \ template <typename T> \ static void QuickSort( T &Array[], const int Start, const int End ) \ { \ if (Start < End) \ { \ const int Pivot = Partition(Array, Start, End); \ \ SORT::QuickSort(Array, Start, Pivot - 1); \ SORT::QuickSort(Array, Pivot + 1, End); \ } \ \ return; \ } \ \ public: \ template <typename T> \ static void Sort( T &Array[], int Count = WHOLE_ARRAY, const int Start = 0 ) \ { \ if (Count == WHOLE_ARRAY) \ Count = ::ArraySize(Array); \ \ SORT::QuickSort(Array, Start, Start + Count - 1); \ \ return; \ } \ }; \ \ SORT::Sort(ARRAY); \ }
Use
struct _buffer{int spread; int instances;}; _buffer WellingtonSpread[]; void OnStart() { ArraySortStruct(WellingtonSpread, spread); // Sort by spread. ArraySortStruct(WellingtonSpread, instances); // Sort by instances. }
Got a string of errors when just copy, paste and compile, so after some minor debugging efforts assumed it to be for mq5. After your comment tried it on a different machine and it compiled without issues. Will check tomorrow what happened and dive into the code a bit deeper to see what it does.
Thanks for your response, much appreciated.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi
I'm using a struct for collecting the data which includes spreads and their instances.
The thing needed is a sorting method for these two variables in the struct by either descending or ascending.