given the following two arrays:
If you switch to structs, you can use something like this to sort:
https://www.mql5.com/en/forum/203831#comment_5273405
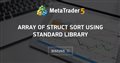
- 2017.06.10
- www.mql5.com
If you switch to structs, you can use something like this to sort:
https://www.mql5.com/en/forum/203831#comment_5273405
Or keep the original arrays and use some existing function :
#include <Math\Stat\Math.mqh> ... int ind[26]; for(int i=0;i<26;i++) ind[i]=i; MathQuickSortDescending(values,ind,0,25); for(int i=0;i<5;i++) { printf("Symbol %s %.2f",symbols[ind[i]],values[i]); } ...
This looks like it is exactly what i want except that the given function is used in MQL5. Or maybe i am not able to include it in my MQL4 code.
These are the errors i get:
can't open "...\Math\Stat\Math.mqh" include file ArrayMinMax.mq4 13 11
'MathQuickSortDescending' - function not defined ArrayMinMax.mq4 40 7
This looks like it is exactly what i want except that the given function is used in MQL5. Or maybe i am not able to include it in my MQL4 code.
These are the errors i get:
can't open "...\Math\Stat\Math.mqh" include file ArrayMinMax.mq4 13 11
'MathQuickSortDescending' - function not defined ArrayMinMax.mq4 40 7
Sorry I missed it was for mql4.
Try it (I didn't check if it works correctly with mql4, but it should).
//+------------------------------------------------------------------+ //| MathQuickSortDescending | //+------------------------------------------------------------------+ //| The function sorts array[] and indices[] simultaneously | //| using QuickSort algorithm. After sorting the initial ordering | //| of the elements is located in the indices[] array. | //| | //| Arguments: | //| array : Array with values to sort | //| indices : Targe array to sort | //| first : First element index | //| last : Last element index | //| | //| Return value: None | //+------------------------------------------------------------------+ void MathQuickSortDescending(double &array[],int &indices[],int first,int last) { int i,j,t_int; double p_double,t_double; //--- check if(first<0 || last<0) return; //--- sort i=first; j=last; while(i<last) { //--- ">>1" is quick division by 2 p_double=array[(first+last)>>1]; while(i<j) { while(array[i]>p_double) { //--- control the output of the array bounds if(i==ArraySize(array)-1) break; i++; } while(array[j]<p_double) { //--- control the output of the array bounds if(j==0) break; j--; } if(i<=j) { //-- swap elements i and j t_double=array[i]; array[i]=array[j]; array[j]=t_double; //-- swap indices i and j t_int=indices[i]; indices[i]=indices[j]; indices[j]=t_int; i++; //--- control the output of the array bounds if(j==0) break; j--; } } if(first<j) MathQuickSortDescending(array,indices,first,j); first=i; j=last; } }
Sorry I missed it was for mql4.
Try it (I didn't check if it works correctly with mql4, but it should).
Wow! It works perfectly!
Thanks a million man!
I see you found your solution, but I agree with Anthony's suggestion. You don't want to reinvent the wheel every time you need to sort some objects, and object sorting happens to be one of the most useful design patterns in MQL programming. That being said, your algo is super easy to implement using inheritance.
#include <Indicators\Oscilators.mqh> #include <Indicators\Indicators.mqh> //+------------------------------------------------------------------+ class MyMACD : public CiMACD { public: virtual int Compare(const CObject *node, const int mode=0) const override { const MyMACD*other=node; if(this.Main(0) > other.Main(0)) return 1; if(this.Main(0) < other.Main(0)) return -1; return 0; } }; //+------------------------------------------------------------------+ class MacdPool : public CIndicators { public: MyMACD *operator[](int i){ return this.At(i);} MacdPool() { for(int i=SymbolsTotal(true)-1; i>=0;i--){ MyMACD *macd = new MyMACD(); this.Add(macd); macd.Create(SymbolName(i, true), _Period, 12, 29, 9, PRICE_CLOSE); } } }; //+------------------------------------------------------------------+ void OnStart() { MacdPool pool; pool.Refresh(); pool.Sort(); int total = pool.Total(); Print("Top 5"); for(int i=total-1; i >= 0 && i > total - 6; i--) printf("%s -> %.2f", pool[i].Symbol(), pool[i].Main(0)); Print("Bottom 5"); for(int i=0; i < total && i < 5; i++) printf("%s -> %.2f", pool[i].Symbol(), pool[i].Main(0)); }

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
given the following two arrays:
string symbols[]=
{
"USDCHF","GBPUSD","USDJPY","EURUSD","AUDUSD","USDCAD","EURCHF","EURGBP",
"EURJPY","EURCAD","EURAUD","GBPCHF","GBPJPY","CHFJPY","NZDUSD","NZDJPY",
"CADCHF","EURNZD","AUDJPY","AUDCHF","AUDCAD","AUDNZD","CADJPY","USDSGD",
"GBPCAD","GBPAUD"
};
double values[26]=
{
51.2, -78.2, 15.6, -22.9, 11.0, 89.4, -44.1, 2.6, -48.6, -45.3, -1.05,
21.2, -8.2, 65.6, 32.9, -71.0, 9.4, -34.1, 52.6, -18.6, 5.3, -51.05,
-94.01,24.3,50.6,-97.5
};
the values in the array values[] correspond to the symbols in the array symbols[]. i would like to send trades in the symbols corresponding to the five highest and five lowest values (in a loop),
that is, [BUY : USDCAD : 89.4],
[BUY : CHFJPY : 65.6],
[BUY : AUDJPY : 52.6],
[BUY : USDCHF : 51.2],
[BUY : GBPCAD : 50.6],
[SELL : GBPAUD : -97.5],
[SELL : CADJPY : -94.01],
[SELL : GBPUSD : -78.2],
[SELL : NZDJPY : -71.0],
[SELL : AUDNZD : -51.05].
thanks in advance...