Existe robot o similar que cierre todas operaciones abiertas cuando beneficio total de la cuenta sea X ?
Buenas tardes a todos.
Estoy buscando que una cuenta MT5 cierre todas las operaciones abiertas (en positivo o negativo) cuando el beneficio total de la cuenta llegue a un objetivo de X dolares.
¿Alguien conoce si esto existe? ¿O creen que se deberia engargar directamente a algun freelance?
Gracias por leerme.
Buenas tardes.
Saludos.
https://www.mql5.com/es/code/23715
¿Quizas esto podria ser la solucion?
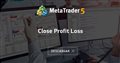
- www.mql5.com
//+------------------------------------------------------------------+ //| Strategy: Cerrador Joaquin.mq5 | //| Copyright 2022, Enrique Enguix | //+------------------------------------------------------------------+ #property copyright "Copyright 2022" #property version "1.00" #property strict #property description "" input double Ganancia = 100;//Ganancia en la moneda de la cuenta int LotDigits; //initialized in OnInit int MagicNumber = 1872290; int MaxSlippage = 3; //slippage, adjusted in OnInit int MaxSlippage_; int OrderRetry = 5; //# of retries if sending order returns error int OrderWait = 5; //# of seconds to wait if sending order returns error double myPoint; //initialized in OnInit //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ void myAlert(string type, string message) { if(type == "print") Print(message); else if(type == "error") { Print(type+" | Cerrador Joaquin @ "+Symbol()+","+IntegerToString(Period())+" | "+message); } else if(type == "order") { } else if(type == "modify") { } } //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ double TotalOpenProfit(int direction) { double result = 0; int total = PositionsTotal(); for(int i = 0; i < total; i++) { if(PositionGetTicket(i) <= 0) continue; if(PositionGetString(POSITION_SYMBOL) != Symbol() || PositionGetInteger(POSITION_MAGIC) != MagicNumber) continue; if((direction < 0 && PositionGetInteger(POSITION_TYPE) == POSITION_TYPE_BUY) || (direction > 0 && PositionGetInteger(POSITION_TYPE) == POSITION_TYPE_SELL)) continue; result += PositionGetDouble(POSITION_PROFIT); } return(result); } //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ void myOrderClose(ENUM_ORDER_TYPE type, double volumepercent, string ordername) //close open orders for current symbol, magic number and "type" (ORDER_TYPE_BUY or ORDER_TYPE_SELL) { if(!TerminalInfoInteger(TERMINAL_TRADE_ALLOWED) || !MQLInfoInteger(MQL_TRADE_ALLOWED)) return; if(type > 1) { myAlert("error", "Invalid type in myOrderClose"); return; } bool success = false; string ordername_ = ordername; if(ordername != "") ordername_ = "("+ordername+")"; int total = PositionsTotal(); int orderList[][2]; int orderCount = 0; for(int i = 0; i < total; i++) { if(PositionGetTicket(i) <= 0) continue; if(PositionGetInteger(POSITION_MAGIC) != MagicNumber || PositionGetString(POSITION_SYMBOL) != Symbol() || PositionGetInteger(POSITION_TYPE) != type) continue; orderCount++; ArrayResize(orderList, orderCount); orderList[orderCount - 1][0] = (int)PositionGetInteger(POSITION_TIME); orderList[orderCount - 1][1] = (int)PositionGetInteger(POSITION_TICKET); } if(orderCount > 0) ArraySort(orderList); for(int i = 0; i < orderCount; i++) { if(!PositionSelectByTicket(orderList[i][1])) continue; MqlTick last_tick; SymbolInfoTick(Symbol(), last_tick); double price = (type == ORDER_TYPE_SELL) ? last_tick.ask : last_tick.bid; MqlTradeRequest request; ZeroMemory(request); request.action = TRADE_ACTION_DEAL; request.position = PositionGetInteger(POSITION_TICKET); //set allowed filling type int filling = (int)SymbolInfoInteger(Symbol(),SYMBOL_FILLING_MODE); if(request.action == TRADE_ACTION_DEAL && (filling & 1) != 1) request.type_filling = ORDER_FILLING_IOC; request.magic = MagicNumber; request.symbol = Symbol(); request.volume = NormalizeDouble(PositionGetDouble(POSITION_VOLUME)*volumepercent * 1.0 / 100, LotDigits); if(NormalizeDouble(request.volume, LotDigits) == 0) return; request.price = NormalizeDouble(price, Digits()); request.sl = 0; request.tp = 0; request.deviation = MaxSlippage_; request.type = (ENUM_ORDER_TYPE)(1-type); //opposite type request.comment = ordername; MqlTradeResult result; ZeroMemory(result); success = OrderSend(request, result) && OrderSuccess(result.retcode); if(!success) { myAlert("error", "OrderClose"+ordername_+" failed; error: "+result.comment); } } string typestr[8] = {"Buy", "Sell", "Buy Limit", "Sell Limit", "Buy Stop", "Sell Stop", "Buy Stop Limit", "Sell Stop Limit"}; if(success) myAlert("order", "Orders closed"+ordername_+": "+typestr[type]+" "+Symbol()+" Magic #"+IntegerToString(MagicNumber)); } //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ bool OrderSuccess(uint retcode) { return(retcode == TRADE_RETCODE_PLACED || retcode == TRADE_RETCODE_DONE || retcode == TRADE_RETCODE_DONE_PARTIAL || retcode == TRADE_RETCODE_NO_CHANGES); } //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { MaxSlippage_ = MaxSlippage; //initialize myPoint myPoint = Point(); if(Digits() == 5 || Digits() == 3) { myPoint *= 10; MaxSlippage_ *= 10; } //initialize LotDigits double LotStep = SymbolInfoDouble(Symbol(), SYMBOL_VOLUME_STEP); if(NormalizeDouble(LotStep, 3) == round(LotStep)) LotDigits = 0; else if(NormalizeDouble(10*LotStep, 3) == round(10*LotStep)) LotDigits = 1; else if(NormalizeDouble(100*LotStep, 3) == round(100*LotStep)) LotDigits = 2; else LotDigits = 3; return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { ulong ticket = 0; double TotalOpenProfitLong = TotalOpenProfit(1); double TotalOpenProfitShort = TotalOpenProfit(-1); //Close Long Positions if((TotalOpenProfitLong + TotalOpenProfitShort) >= Ganancia) { if(TerminalInfoInteger(TERMINAL_TRADE_ALLOWED) && MQLInfoInteger(MQL_TRADE_ALLOWED)) { myOrderClose(ORDER_TYPE_BUY, 100, ""); myOrderClose(ORDER_TYPE_SELL, 100, ""); } else //not autotrading => only send alert myAlert("order", ""); } }
Mi pequeña aportación. No lo he probado, pero debería funcionar
Muchas gracias por tu aportacion Enrique Enguix !!
Lamentablemente, no me ha funcionado.
Puede ser por que es la primera vez que pongo a funcionar algo así, pero tengo activo el "Trading algoritmico", y ha pasado por el nivel definido y no ha cerrado.
Si algun compañero tiene alguna sugerencia que pudiera hacerlo funcionar.
Gracias.
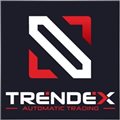
Muchas gracias por tu aportacion Enrique Enguix !!
Lamentablemente, no me ha funcionado.
Puede ser por que es la primera vez que pongo a funcionar algo así, pero tengo activo el "Trading algoritmico", y ha pasado por el nivel definido y no ha cerrado.
Si algun compañero tiene alguna sugerencia que pudiera hacerlo funcionar.
Gracias.
Acabo de activar esta pestaña primera, no la tenia activada, siendo asi, si que deberia de funcionar.
Te confirmo el lunes con el mercado operativo.
Gracias, de nuevo.
Acabo de activar esta pestaña primera ( Permitir comercio algoritmico ), no la tenia activada, siendo asi, si que deberia de funcionar.
Te confirmo el lunes con el mercado operativo.
Gracias, de nuevo.
Espero que te funcione. Y sino le echaré un ojo también al código que mandaste. Un saludo, buen fin de semana
Buenas tardes Enrique.
Gracias de nuevo.
Probando el sistema, no me funciona.
¿Alguna idea a que puede deberse?
Respecto al codigo que mandé ( https://www.mql5.com/es/code/23715 ) , funciona, pero solo si abro y cierro el broker. Delante mia lo veo pasar por el nivel requerido, cierro y abro broker y en ese momento si funciona.
Gracias.
Buena semana.
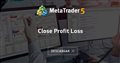
- www.mql5.com
Buenas tardes Enrique.
Gracias de nuevo.
Probando el sistema, no me funciona.
¿Alguna idea a que puede deberse?
Respecto al codigo que mandé ( https://www.mql5.com/es/code/23715 ) , funciona, pero solo si abro y cierro el broker. Delante mia lo veo pasar por el nivel requerido, cierro y abro broker y en ese momento si funciona.
Gracias.
Buena semana.
Joaquín, disculpa es que ahora voy muy liado. Pon número mágico 0. Espera te lo pongo como input. Así tal cuál está debería funcionar
//+------------------------------------------------------------------+ //| Strategy: Cerrador Joaquin.mq5 | //| Copyright 2022, Enrique Enguix | //+------------------------------------------------------------------+ #property copyright "Copyright 2022" #property version "1.00" #property strict #property description "" input double Ganancia = 100;//Ganancia en la moneda de la cuenta int LotDigits; //initialized in OnInit input int MagicNumber = 0;//Numero Mágico int MaxSlippage = 3; //slippage, adjusted in OnInit int MaxSlippage_; int OrderRetry = 5; //# of retries if sending order returns error int OrderWait = 5; //# of seconds to wait if sending order returns error double myPoint; //initialized in OnInit //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ void myAlert(string type, string message) { if(type == "print") Print(message); else if(type == "error") { Print(type+" | Cerrador Joaquin @ "+Symbol()+","+IntegerToString(Period())+" | "+message); } else if(type == "order") { } else if(type == "modify") { } } //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ double TotalOpenProfit(int direction) { double result = 0; int total = PositionsTotal(); for(int i = 0; i < total; i++) { if(PositionGetTicket(i) <= 0) continue; if(PositionGetString(POSITION_SYMBOL) != Symbol() || PositionGetInteger(POSITION_MAGIC) != MagicNumber) continue; if((direction < 0 && PositionGetInteger(POSITION_TYPE) == POSITION_TYPE_BUY) || (direction > 0 && PositionGetInteger(POSITION_TYPE) == POSITION_TYPE_SELL)) continue; result += PositionGetDouble(POSITION_PROFIT); } return(result); } //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ void myOrderClose(ENUM_ORDER_TYPE type, double volumepercent, string ordername) //close open orders for current symbol, magic number and "type" (ORDER_TYPE_BUY or ORDER_TYPE_SELL) { if(!TerminalInfoInteger(TERMINAL_TRADE_ALLOWED) || !MQLInfoInteger(MQL_TRADE_ALLOWED)) return; if(type > 1) { myAlert("error", "Invalid type in myOrderClose"); return; } bool success = false; string ordername_ = ordername; if(ordername != "") ordername_ = "("+ordername+")"; int total = PositionsTotal(); int orderList[][2]; int orderCount = 0; for(int i = 0; i < total; i++) { if(PositionGetTicket(i) <= 0) continue; if(PositionGetInteger(POSITION_MAGIC) != MagicNumber || PositionGetString(POSITION_SYMBOL) != Symbol() || PositionGetInteger(POSITION_TYPE) != type) continue; orderCount++; ArrayResize(orderList, orderCount); orderList[orderCount - 1][0] = (int)PositionGetInteger(POSITION_TIME); orderList[orderCount - 1][1] = (int)PositionGetInteger(POSITION_TICKET); } if(orderCount > 0) ArraySort(orderList); for(int i = 0; i < orderCount; i++) { if(!PositionSelectByTicket(orderList[i][1])) continue; MqlTick last_tick; SymbolInfoTick(Symbol(), last_tick); double price = (type == ORDER_TYPE_SELL) ? last_tick.ask : last_tick.bid; MqlTradeRequest request; ZeroMemory(request); request.action = TRADE_ACTION_DEAL; request.position = PositionGetInteger(POSITION_TICKET); //set allowed filling type int filling = (int)SymbolInfoInteger(Symbol(),SYMBOL_FILLING_MODE); if(request.action == TRADE_ACTION_DEAL && (filling & 1) != 1) request.type_filling = ORDER_FILLING_IOC; request.magic = MagicNumber; request.symbol = Symbol(); request.volume = NormalizeDouble(PositionGetDouble(POSITION_VOLUME)*volumepercent * 1.0 / 100, LotDigits); if(NormalizeDouble(request.volume, LotDigits) == 0) return; request.price = NormalizeDouble(price, Digits()); request.sl = 0; request.tp = 0; request.deviation = MaxSlippage_; request.type = (ENUM_ORDER_TYPE)(1-type); //opposite type request.comment = ordername; MqlTradeResult result; ZeroMemory(result); success = OrderSend(request, result) && OrderSuccess(result.retcode); if(!success) { myAlert("error", "OrderClose"+ordername_+" failed; error: "+result.comment); } } string typestr[8] = {"Buy", "Sell", "Buy Limit", "Sell Limit", "Buy Stop", "Sell Stop", "Buy Stop Limit", "Sell Stop Limit"}; if(success) myAlert("order", "Orders closed"+ordername_+": "+typestr[type]+" "+Symbol()+" Magic #"+IntegerToString(MagicNumber)); } //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ bool OrderSuccess(uint retcode) { return(retcode == TRADE_RETCODE_PLACED || retcode == TRADE_RETCODE_DONE || retcode == TRADE_RETCODE_DONE_PARTIAL || retcode == TRADE_RETCODE_NO_CHANGES); } //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { MaxSlippage_ = MaxSlippage; //initialize myPoint myPoint = Point(); if(Digits() == 5 || Digits() == 3) { myPoint *= 10; MaxSlippage_ *= 10; } //initialize LotDigits double LotStep = SymbolInfoDouble(Symbol(), SYMBOL_VOLUME_STEP); if(NormalizeDouble(LotStep, 3) == round(LotStep)) LotDigits = 0; else if(NormalizeDouble(10*LotStep, 3) == round(10*LotStep)) LotDigits = 1; else if(NormalizeDouble(100*LotStep, 3) == round(100*LotStep)) LotDigits = 2; else LotDigits = 3; return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { ulong ticket = 0; double TotalOpenProfitLong = TotalOpenProfit(1); double TotalOpenProfitShort = TotalOpenProfit(-1); //Close Long Positions if((TotalOpenProfitLong + TotalOpenProfitShort) >= Ganancia) { if(TerminalInfoInteger(TERMINAL_TRADE_ALLOWED) && MQLInfoInteger(MQL_TRADE_ALLOWED)) { myOrderClose(ORDER_TYPE_BUY, 100, ""); myOrderClose(ORDER_TYPE_SELL, 100, ""); } else //not autotrading => only send alert myAlert("order", ""); } }
Joaquín, disculpa es que ahora voy muy liado. Pon número mágico 0. Espera te lo pongo como input. Así tal cuál está debería funcionar
Buenas noches Enrique.
Gracias, de nuevo.
Te cuento: funciona, pero parcialmente. Ejemplo: tengo 4 operaciones abiertas, con los siguientes beneficios: +250, +250, -150, -150, y tras haber seleccionado beneficio general en 500, solo me cierra las dos primeras operaciones, cuando deberia cerrar todo.
Nos vamos acercando, gracias Enrique de nuevo.
Cualquier duda me cuentas.
Buenas tardes a todos.
Estoy buscando que una cuenta MT5 cierre todas las operaciones abiertas (en positivo o negativo) cuando el beneficio total de la cuenta llegue a un objetivo de X dolares.
¿Alguien conoce si esto existe? ¿O creen que se deberia engargar directamente a algun freelance?
Gracias por leerme.
Buenas tardes.
Saludos.
Hola Joaquin,
Acabo de hacer el código pero no lo probé. Espero te sirva. Saludos
//+------------------------------------------------------------------+ //| Ayuda.mq5 | //| Copyright 2022, Antonio Simón Del Vecchio | //+------------------------------------------------------------------+ #property copyright "Copyright 2022, Antonio Simón Del Vecchio" #property version "1.00" //+------------------------------------------------------------------+ //| Include | //+------------------------------------------------------------------+ #include <Trade\Trade.mqh> #include <Trade\PositionInfo.mqh> #include <Trade\AccountInfo.mqh> //+------------------------------------------------------------------+ //| Objetos | //+------------------------------------------------------------------+ CTrade Trade; CPositionInfo Posicion; CAccountInfo Cuenta; //+------------------------------------------------------------------+ //| Inputs | //+------------------------------------------------------------------+ input double Ganancia = 20; //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { if(Cuenta.Profit() >= Ganancia) CerrarPosiciones(); } //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ void CerrarPosiciones() { for(int i = PositionsTotal() - 1; i >= 0; i--) { if(Posicion.SelectByIndex(i)) { Trade.PositionClose(Posicion.Ticket(), -1); } } } //+------------------------------------------------------------------+
hola, me gustaria que algo asi funsione pero para mt4 es posible??

- Aplicaciones de trading gratuitas
- 8 000+ señales para copiar
- Noticias económicas para analizar los mercados financieros
Usted acepta la política del sitio web y las condiciones de uso
Buenas tardes a todos.
Estoy buscando que una cuenta MT5 cierre todas las operaciones abiertas (en positivo o negativo) cuando el beneficio total de la cuenta llegue a un objetivo de X dolares.
¿Alguien conoce si esto existe? ¿O creen que se deberia engargar directamente a algun freelance?
Gracias por leerme.
Buenas tardes.
Saludos.