If the code can be compiled without any error but it doesn't do what you expect use the debugger to find out why - it is exactly made for this.
Code debugging: https://www.metatrader5.com/en/metaeditor/help/development/debug
Error Handling and Logging in MQL5: https://www.mql5.com/en/articles/2041
Tracing, Debugging and Structural Analysis of Source Code, scroll down to: "Launching and Debuggin": https://www.mql5.com/en/articles/272
Code debugging: https://www.metatrader5.com/en/metaeditor/help/development/debug
Error Handling and Logging in MQL5: https://www.mql5.com/en/articles/2041
Tracing, Debugging and Structural Analysis of Source Code, scroll down to: "Launching and Debuggin": https://www.mql5.com/en/articles/272
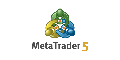
Code debugging - Developing programs - MetaEditor Help
- www.metatrader5.com
MetaEditor has a built-in debugger allowing you to check a program execution step by step (by individual functions). Place breakpoints in the code...
-
Please edit your (original) post and use the CODE button (or Alt+S)! (For large amounts of code, attach it.)
General rules and best pratices of the Forum. - General - MQL5 programming forum #25 (2019)
Messages Editor
Forum rules and recommendations - General - MQL5 programming forum (2023) -
Where do you call initIndicators?

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Hello everyone,
I’ve developed an automated trading bot for MetaTrader 5, but I’m encountering a major issue: the bot is not executing any trades, even during backtesting.
Issue Summary:
• The bot is running, but no trades are being placed.
• There are no errors in the Journal or Experts tab.
• The strategy should be triggering trades, but nothing happens.
• Auto-trading is enabled.
What I’ve Tried So Far:
• Checking for logic errors in my code.
• Ensuring the bot is properly attached to the chart.
• Verifying that the symbol and timeframe match the strategy conditions.
• Testing different input parameters to see if they affect execution.
• Reviewing the Strategy Tester settings to ensure proper backtesting conditions.
Full Code Available
I’m willing to share my full code so that others can review it and help me identify the issue.
Has anyone encountered a similar problem? Any suggestions on how to debug this?
I’d really appreciate any help.
Thanks in advance!