Help with error on rectangle for EA - if expression are not allowed on global scale.. where does my curly bracket go?
A curly bracket is missing.
Run the Tools=> Styler of the Editor to find it easier. BTW MT5 prints in the log which { has no }
i think my code is wrong... i dont think i should need that curly bracket
im basically asking how to draw a rectangle where i want it cause when i try its not working and its erroring, and i dont know why
Look for examples: https://www.mql5.com/en/search#!keyword=rectangle%20box
This way is much, much faster!
-
Stop using ChatGPT.
Help needed to debug and fix an AI EA - Trading Systems - MQL5 programming forum #2 (2023) -
double CLow = PRICE_LOW; double CHigh = PRICE_HIGH; double COpen = PRICE_OPEN; double CClose = PRICE_CLOSE;
Those red symbols are constants 3, 2, 1, and zero. Not prices, not doubles.
-
double Bull = PRICE_OPEN<PRICE_CLOSE; double Bear = PRICE_OPEN>PRICE_CLOSE;
One is always more than zero. Therefor Bear will always be true.
-
if(Bear[3] and Bull[2] and Bull[1] and CHigh[3]<CLow[1]){
You declared them as a variable, not an array. Don't post code that will not compile.
-
MT4: Learn to code it.
MT5: Begin learning to code it.If you don't learn MQL4/5, there is no common language for us to communicate. If we tell you what you need, you can't code it. If we give you the code, you don't know how to integrate it into your code.
Look for examples: https://www.mql5.com/en/search#!keyword=rectangle%20box
This way is much, much faster!
i have searched for something specific relating to what im trying to do... theres lots of freelance jobs that are completed... but not useful for me cause i want to learn how to do this haha
My problem is i can follow tutorials relating to drawing a rectangle and drawing parallel lines or trend lines etc..
but when im trying to alter that slightly to suit my own requirements... eg.. using specific candles... i cant seem to figure it out.
Once im shown, im good though... So is there a way you can dumb it down for me
As per this code here....The place i am getting stuck is the defining the bullFVG highs and lows and where to add them for drawing the rectangle?
the syntax is incorrect but i cant find how to fix it
void OnTick(){ //Create an array for prices and sort it from current to oldest candles MqlRates Price[]; ArraySetAsSeries(Price,true); //Copy price data into the array int Data=CopyRates(_Symbol,_Period,0,Bars(_Symbol,_Period),Price); // Create variable bearFVG or bullFVG and highs and lows int bullFVG, bearFVG; // create array for Candle Highs and Lows of candles and sort from current to earliest double High[],Low[],Open[],Close[]; ArraySetAsSeries(High,true); ArraySetAsSeries(Low,true); ArraySetAsSeries(Open,true); ArraySetAsSeries(Close,true); //Fill arrays with data of highs and lows for the last 5 candles CopyHigh(_Symbol,_Period,0,5,High); CopyLow(_Symbol,_Period,0,5,Low); CopyOpen(_Symbol,_Period,0,5,Open); CopyClose(_Symbol,_Period,0,5,Close); // How do i do this part?? // bullFVG is an up candle and high of 3 bigger than low of 1 // How do i define the specific high and low? //Calculate the High and low for the bullFVG bullFVG = Open[2] < Close[2] & High[3] < Low[1]; //create new rectangle for bullFVG using candle3s low, starting at candle 2, //using candle 1s high and ending at candle 0 ObjectCreate(_Symbol,"Rectangle",OBJ_RECTANGLE,0,Price[2].time,Price[bullFVG].High[3],Price[0].time,Price[bullFVG].Low[1]); ObjectSetInteger(0,"Rectangle",OBJPROP_COLOR,clrLime); //set Object line color ObjectSetInteger(0,"Rectangle",OBJPROP_FILL,clrLime); //Set the Object fill color //Calculate the High and low for the bullFVG bearFVG = Price.Open[2] > Price.Close[2] and Price.Low[3] > Price.High[1]; //create new rectangle for bearFVG using candle3s high, starting at candle 2, using candle 1s low and ending at candle 0 ObjectCreate(_Symbol,"Rectangle",OBJ_RECTANGLE,0,Price[2].time,Price[bullFVG].Low[3],Price[0].time,Price[bullFVG].High[1]); ObjectSetInteger(0,"Rectangle",OBJPROP_COLOR,clrRed); //set Object line color ObjectSetInteger(0,"Rectangle",OBJPROP_FILL,clrRed); //Set the Object fill color
-
Stop using ChatGPT.
Help needed to debug and fix an AI EA - Trading Systems - MQL5 programming forum #2 (2023) -
Those red symbols are constants 3, 2, 1, and zero. Not prices, not doubles.
-
One is always more than zero. Therefor Bear will always be true.
-
You declared them as a variable, not an array. Don't post code that will not compile.
-
MT4: Learn to code it.
MT5: Begin learning to code it.If you don't learn MQL4/5, there is no common language for us to communicate. If we tell you what you need, you can't code it. If we give you the code, you don't know how to integrate it into your code.
i didnt use chatGPT... i used this tutorial... https://youtu.be/Y3e1zVROJlY
I am trying to learn... and i am trying to figure it out but nobody actually answers so i can learn
im using MT5.. not MT4 so im using mql5
It wont compile no... but im asking for help.
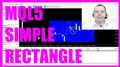
- 2018.02.23
- www.youtube.com
I call BS. A mixture of MT4, MT5, and some other (Pine?) means you didn't try to learn the language.
I call BS. A mixture of MT4, MT5, and some other (Pine?) means you didn't try to learn the language.
ObjectCreate(_Symbol,"Rectangle",OBJ_RECTANGLE,0,Price[2].time,Price[bullFVG].Low[3],Price[0].time,Price[bullFVG].High[1]);Just one example of wrong code.
1. You should check the documentation of ObjectCreate(). What are the parameters ?
2. What is the suppose to mean ?
Price[bullFVG].Low[3]
Price is an array of MqlRates. This structure is well defined in the documentation, is there a "Low[]" array member ?

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Can somebody check my code and tell me what i need to change?
My if statements are within the ontick function so i didnt think they were on the global scope...
but without the closed bracket before the if statements i get alot more errors. 34 as opposed to 3 ha ha
Thought Process based on previous tutorials/doco
Definately could be overthinking...
Define Candles OHLC to be able to use these for working out whether BULL or BEAR
Define BULL or BEAR candles
Create variable for FVGHighs and FVGLows
Create array to store the price of the highs and lows and sort these from candle 0 to previous candles
Fill the arrays with the price data for the last 3 candles
Use those ArrayPrices for our FVGHighs and Lows
Store price data in Price and sort from current candle backwards
Delete any rectangles already present (if i want multiple rectangles... this step shouldn't be here)
Define what constitutes our FVG Gap for the Rectangle
Then create our rectangle with our designated settings
where i want the rectangle to draw (pretend the last 2 candles arent there)