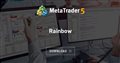
Here is an example that plots 60 indicator lines use it an an example to set the initial part of your indicator: https://www.mql5.com/en/code/13031
int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]){ ⋮ int handleSMA14H = iMA(NULL, 0, MA_Period, 0, MODE_SMA, PRICE_HIGH); int handleSMA14L = iMA(NULL, 0, MA_Period, 0, MODE_SMA, PRICE_LOW);
Perhaps you should read the manual, especially the examples.
How To Ask Questions The Smart Way. (2004)
How To Interpret Answers.
RTFM and STFW: How To Tell You've Seriously Screwed Up.
They all (including iCustom) return a handle (an int). You get that in OnInit. In OnTick/OnCalculate (after the indicator has updated its buffers), you use the handle, shift and count to get the data.
Technical Indicators - Reference on algorithmic/automated trading language for MetaTrader 5
Timeseries and Indicators Access / CopyBuffer - Reference on algorithmic/automated trading language for MetaTrader 5
How to start with MQL5 - General - MQL5 programming forum - Page 3 #22 (2020)
How to start with MQL5 - MetaTrader 5 - General - MQL5 programming forum - Page 7 #61 (2020)
MQL5 for Newbies: Guide to Using Technical Indicators in Expert Advisors - MQL5 Articles (2010)
How to call indicators in MQL5 - MQL5 Articles (2010)
Perhaps you should read the manual, especially the examples.
How To Ask Questions The Smart Way. (2004)
How To Interpret Answers.
RTFM and STFW: How To Tell You've Seriously Screwed Up.
They all (including iCustom) return a handle (an int). You get that in OnInit. In OnTick/OnCalculate (after the indicator has updated its buffers), you use the handle, shift and count to get the data.
Technical Indicators - Reference on algorithmic/automated trading language for MetaTrader 5
Timeseries and Indicators Access / CopyBuffer - Reference on algorithmic/automated trading language for MetaTrader 5
How to start with MQL5 - General - MQL5 programming forum - Page 3 #22 (2020)
How to start with MQL5 - MetaTrader 5 - General - MQL5 programming forum - Page 7 #61 (2020)
MQL5 for Newbies: Guide to Using Technical Indicators in Expert Advisors - MQL5 Articles (2010)
How to call indicators in MQL5 - MQL5 Articles (2010)
Your topic has been moved to the section: Technical Indicators — In the future, please consider which section is most appropriate for your query.
- Aryan Singh #: Can you checkout my code and tell me how to fix it also getting time out in 5sec for my private messages
I already did. What part of “You get that in OnInit” was unclear? Did you move the creation of your handles there?
-
CI_H3Buffer[i] = (SMA14RangeBuffer[i] * 3) + iMA(NULL, 0, bars_CI_H3, 0, MODE_SMA, price_CI_H3);
That is not a value. What part of "you use the handle, shift and count" was unclear?
-
Mixture of MT4 and MT5 code. Stop using ChatGPT.
Help needed to debug and fix an AI EA - Trading Systems - MQL5 programming forum #2 (2023)If you don't learn MQL4/5, there is no common language for us to communicate. If we tell you what you need, you can't code it. If we give you the code, you don't know how to integrate it into your code.
-
I already did. What part of “You get that in OnInit” was unclear? Did you move the creation of your handles there?
-
That is not a value. What part of "you use the handle, shift and count" was unclear?
-
Mixture of MT4 and MT5 code. Stop using ChatGPT.
Help needed to debug and fix an AI EA - Trading Systems - MQL5 programming forum #2 (2023)If you don't learn MQL4/5, there is no common language for us to communicate. If we tell you what you need, you can't code it. If we give you the code, you don't know how to integrate it into your code.
oh, then how can I add another iMA with userinput period and Enum applied price to BandRange

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I need help in one of my project that is in MT5 using MQL5 language. I have started learning MQL5 recently. So, basic summary of task is; I need a custom indicator that incorporates 2 other standard indicators. For example, take the range of the SMA50H & SMA50L, and add/substract the SMA50Range to the SMA14Mid, plot it as an indicator on the chart.
Further Clarification:
Build a custom indicator that uses 2 other standard indicators, and then plots them
on a chart.
MT5
Variables;
BandHigh=SMA.High.14Bars (the number of bars should be defineable as a
parameter)
BandLow=SMA.Low.14Bars (the number of bars should be defineable as a
parameter)
BandRange=BandHigh-BandLow
Custom Indicator;
"TNJK ExtensionBands"
"CI-H3"=((BandRange3)+SMA.High.14Bars). Plot as RedColour
"CI-H2"=((BandRange2)+SMA.High.14Bars). Plot as OrangeColour
"CI-H1"=((BandRange1)+SMA.High.14Bars). Plot as YellowColour
"CI-L1"=((BandRange1)-SMA.Low.14Bars). Plot as YellowColour
"CI-L2"=((BandRange2)-SMA.Low.14Bars). Plot as OrangeColour
"CI-L3"=((BandRange3)-SMA.Low.14Bars). Plot as RedColour
The number of bars in each leg of the indicator should be defineable as a
parameter.
The SMA price point (High, Low, Mid, Weighted) in each leg of the indicators
BandRange =(SMA.14.High-SMA.14.Low), and BandRange1 =(SMA.14.High-
SMA.14.Low)+SMA.14.High, BandRange2 =((SMA.14.High-
SMA.14.Low)*2)+SMA.14.High,BandRange3 =((SMA.14.High-
SMA.14.Low)*3)+SMA.14.High
For the above requirement I have written the code for calculating the BandRange and SMA's but facing diffculty in plotting those 6 legs. Can anyone help me with that also some reading material that would help in long run. Below is my code: