void calcPolynomialRegression(double &PricesArray[],double &RegressionArray[], int power) { ArrayResize(RegressionArray, ArraySize(PricesArray)); ArraySetAsSeries(RegressionArray,ArrayGetAsSeries(PricesArray)); double summ_x_value[21],summ_y_value[11],constant[11],matrix[11][11]; ArrayInitialize(summ_x_value,0); ArrayInitialize(summ_y_value,0); ArrayInitialize(constant,0); ArrayInitialize(matrix,0); double summ=0,summ_x=0,summ_y=0; int pos=ArraySize(PricesArray)-1; summ_x_value[0]=ArraySize(PricesArray); for(int exp_n=1; exp_n<=2*power; exp_n++) { summ_x=0; summ_y=0; for(int k=1; k<=ArraySize(PricesArray); k++) { summ_x+=MathPow(k,exp_n); if(exp_n==1) summ_y+=PricesArray[pos-k+1]; else if(exp_n<=power+1) summ_y+=PricesArray[pos-k+1]*MathPow(k,exp_n-1); } summ_x_value[exp_n]=summ_x; if(summ_y!=0) summ_y_value[exp_n-1]=summ_y; } for(int row=0; row<=power; row++) for(int col=0; col<=power; col++) matrix[row][col]=summ_x_value[row+col]; int initial_row=1; int initial_col=1; for(int i=1; i<=power; i++) { for(int row=initial_row; row<=power; row++) { summ_y_value[row]=summ_y_value[row]-(matrix[row][i-1]/matrix[i-1][i-1])*summ_y_value[i-1]; for(int col=initial_col; col<=power; col++) matrix[row][col]=matrix[row][col]-(matrix[row][i-1]/matrix[i-1][i-1])*matrix[i-1][col]; } initial_col++; initial_row++; } int j=0; for(int i=power; i>=0; i--) { if(j==0) constant[i]=summ_y_value[i]/matrix[i][i]; else { summ=0; for(int k=j; k>=1; k--) summ+=constant[i+k]*matrix[i][i+k]; constant[i]=(summ_y_value[i]-summ)/matrix[i][i]; } j++; } int k=1; for(int i=ArraySize(PricesArray)-1; i>=0; i--) { summ=0; for(int n=0; n<=power; n++) summ+=constant[n]*MathPow(k,n); RegressionArray[i]=summ; k++; } }
I have implemented regression calculation (not just linear) without cycles at all. More precisely, a cycle is needed only once during initialization.
As a result, calculation speed is a thousand times faster.
And the code is shorter.
But I'm sorry, I won't post the code. It's a secret.
I'm just saying that it's real.
There are two articles that will help
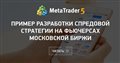
- www.mql5.com
I implemented the calculation of regression (not only linear) without any loops at all. To be more exact, the loop is needed only once at initialization.
As a result, the calculation speed is a thousand times faster.
And the code is shorter.
But I'm sorry, I won't post the code. It's a secret.
I'm just saying that it's real.
The code is very simple. We add the current squares, subtract the squares out of the interval. That's it. That's the whole secret.)
It can be even more interesting, but following different principles.
The code is very simple. We add the current squares, subtract the squares out of the interval. That's it. That's the secret.)
funny ))
To make it even funnier, you could tell not about the channel, but about how to make a polynomial regression line without cycles. But I'm definitely not going to do that. You don't need it.
I have implemented regression calculation (not just linear) without cycles at all. More precisely the cycle is needed only once at initialization.
As a result, the calculation speed is thousands of times faster.
And the code is shorter.
But I'm sorry, I won't post the code. It's a secret.
I'm just saying that it's real.
Thousands of times faster, and without a loop of input values ???
I don't believe it !!!
At the very least, a loop of input parameters is mandatory !
I have implemented regression calculation (not just linear) without cycles at all. More precisely, the loop is needed only once at initialization.
As a result, calculation speed is a thousand times faster.
And the code is shorter.
But I'm sorry, I won't post the code. It's a secret.
I'm just saying that it's real.
And even without an x*y summation loop? And if x and y are not straight lines?
Thousands of times faster, and without a loop of input values ???
I don't believe it !!!
At least a loop over the input parameters is mandatory !
And even without an x*y summation loop ? What if x and y are not straight lines?
Don't believe it for all its worth.
Rashid dropped the articles. Read them carefully. There is a link to another article there:
https://www.mql5.com/ru/articles/270
If you rack your brains at the level of 7th-8th grade maths, you can get the standard deviation to get the channel, not just the sliding average, in a similar way without a cycle. I have this implemented for a polynomial of any degree, not just the first degree (linear regression). You can feel it in the demo version on the marketplace.
HH I wrote that the loop is needed once at initialization.
Thousands of times faster - this includes the calculation of the standard deviation (i.e. channel width)
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I have a linear regression indicator.
How to build its calculation into EA and get data on 0 bar or on 1.
I tried to do it like this:
But it doesn't give out something...