I'm generating a EA through MetaEditor and I don't know what those words mean. Is it refering to the price when is supposed to close the order or?
I'm really confused.
It is refering to a price that will trigger some action , its calculation formula :
apart from the Open High Low Close basics
- Median = (High + Low )/2
- Typical = (High + Low + Close)/3
- Weighted = (High + Low + Close + Close)/4
It is refering to a price that will trigger some action , its calculation formula :
apart from the Open High Low Close basics
- Median = (High + Low )/2
- Typical = (High + Low + Close)/3
- Weighted = (High + Low + Close + Close)/4
For example, I want the robot to IMMEDIATELY open a buy order when the RSI crosses the 70 level, not when the candle closes or opens. Is that what is refering to? Which one do I choose?
No ,the applied price field refers to the calculation of the RSI .
I dont think the generator can receive such a parameter
Of course, you can. Each tick, read the RSI and compare to 70.
Of course, you can. Each tick, read the RSI and compare to 70.
But which are the lines of code that do that? I've been searching the web to do something like that and I couldn't find anything even related. I just want a simple EA robot to open a buy order when the RSI[14] crosses up the 70 level in the 5-minute chart and once it goes below the 70 level it closes the buy order and opens a sell order. And to do it backward. So, it only does 1 order per time.
If you not a coder, you can place a job in the freelance section
The idea of the strategy it's very simple and I can't waste any money at this time. I developed a very simple code but I'm afraid I got a lot of thing wrong. This it it:
//+------------------------------------------------------------------+ //| roborsi.mq5 | //| Copyright 2021, MetaQuotes Software Corp. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2021, MetaQuotes Software Corp." #proerty link "https://www.mql5.com" #property version "1.00" //+------------------------------------------------------------------+ //| Include | //+------------------------------------------------------------------+ #include <Expert\Expert.mqh> //--- available signals #include <Expert\Signal\SignalRSI.mqh> //--- available trailing #include <Expert\Trailing\TrailingNone.mqh> //--- available money management #include <Expert\Money\MoneyFixedLot.mqh> //+------------------------------------------------------------------+ //| Inputs | //+------------------------------------------------------------------+ //--- inputs for expert input string Expert_Title ="roborsi"; // Document name ulong Expert_MagicNumber =19541; // bool Expert_EveryTick =false; // //--- inputs for main signal input int Signal_ThresholdOpen =10; // Signal threshold value to open [0...100] input int Signal_ThresholdClose=10; // Signal threshold value to close [0...100] input double Signal_PriceLevel =0.0; // Price level to execute a deal input double Signal_StopLevel =50.0; // Stop Loss level (in points) input double Signal_TakeLevel =50.0; // Take Profit level (in points) input int Signal_Expiration =4; // Expiration of pending orders (in bars) input int Signal_RSI_PeriodRSI =14; // Relative Strength Index(14,...) Period of calculation input ENUM_APPLIED_PRICE Signal_RSI_Applied =PRICE_CLOSE; // Relative Strength Index(14,...) Prices series input double Signal_RSI_Weight =1.0; // Relative Strength Index(14,...) Weight [0...1.0] //--- inputs for money input double Money_FixLot_Percent =10.0; // Percent input double Money_FixLot_Lots =0.1; // Fixed volume //+------------------------------------------------------------------+ //| Global expert object | //+------------------------------------------------------------------+ CExpert ExtExpert; //+------------------------------------------------------------------+ //| Initialization function of the expert | //+------------------------------------------------------------------+ int OnInit() { //--- Initializing expert if(!ExtExpert.Init(Symbol(),PERIOD_M5,Expert_EveryTick,Expert_MagicNumber)) { //--- failed printf(__FUNCTION__+": error initializing expert"); ExtExpert.Deinit(); return(INIT_FAILED); } //--- Creating signal CExpertSignal *signal=new CExpertSignal; if(signal==NULL) { //--- failed printf(__FUNCTION__+": error creating signal"); ExtExpert.Deinit(); return(INIT_FAILED); } //--- ExtExpert.InitSignal(signal); signal.ThresholdOpen(Signal_ThresholdOpen); signal.ThresholdClose(Signal_ThresholdClose); signal.PriceLevel(Signal_PriceLevel); signal.StopLevel(Signal_StopLevel); signal.TakeLevel(Signal_TakeLevel); signal.Expiration(Signal_Expiration); //--- Creating filter CSignalRSI CSignalRSI *filter0=new CSignalRSI; if(filter0==NULL) { //--- failed printf(__FUNCTION__+": error creating filter0"); ExtExpert.Deinit(); return(INIT_FAILED); } signal.AddFilter(filter0); //--- Set filter parameters filter0.PeriodRSI(Signal_RSI_PeriodRSI); filter0.Applied(Signal_RSI_Applied); filter0.Weight(Signal_RSI_Weight); //--- Creation of trailing object CTrailingNone *trailing=new CTrailingNone; if(trailing==NULL) { //--- failed printf(__FUNCTION__+": error creating trailing"); ExtExpert.Deinit(); return(INIT_FAILED); } //--- Add trailing to expert (will be deleted automatically)) if(!ExtExpert.InitTrailing(trailing)) { //--- failed printf(__FUNCTION__+": error initializing trailing"); ExtExpert.Deinit(); return(INIT_FAILED); } //--- Set trailing parameters //--- Creation of money object CMoneyFixedLot *money=new CMoneyFixedLot; if(money==NULL) { //--- failed printf(__FUNCTION__+": error creating money"); ExtExpert.Deinit(); return(INIT_FAILED); } //--- Add money to expert (will be deleted automatically)) if(!ExtExpert.InitMoney(money)) { //--- failed printf(__FUNCTION__+": error initializing money"); ExtExpert.Deinit(); return(INIT_FAILED); } //--- Set money parameters money.Percent(Money_FixLot_Percent); money.Lots(Money_FixLot_Lots); //--- Check all trading objects parameters if(!ExtExpert.ValidationSettings()) { //--- failed ExtExpert.Deinit(); return(INIT_FAILED); } //--- Tuning of all necessary indicators if(!ExtExpert.InitIndicators()) { //--- failed printf(__FUNCTION__+": error initializing indicators"); ExtExpert.Deinit(); return(INIT_FAILED); } //--- ok return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Deinitialization function of the expert | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { ExtExpert.Deinit(); } //+------------------------------------------------------------------+ //| "Tick" event handler function | //+------------------------------------------------------------------+ void OnTick() { ExtExpert.OnTick(); } //+------------------------------------------------------------------+ //| "Trade" event handler function | //+------------------------------------------------------------------+ void OnTrade() { ExtExpert.OnTrade(); } //+------------------------------------------------------------------+ //| "Timer" event handler function | //+------------------------------------------------------------------+ void OnTimer() { ExtExpert.OnTimer(); } //+------------------------------------------------------------------+
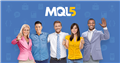
- 2021.02.23
- www.mql5.com
It is refering to a price that will trigger some action , its calculation formula :
apart from the Open High Low Close basics
- Median = (High + Low )/2
- Typical = (High + Low + Close)/3
- Weighted = (High + Low + Close + Close)/4
The documentation for the _AppliedTo variables (where these are defined) has the formula for Weighted as "Weighted price = (Open+High+Low+Close)/4". Yet the Data Type is listed as "Weighted Price (HLCC/4)". The Price Constants section also defines the PRICE_WEIGHTED enum as HLCC (i.e. as mentioned above).
Presumably, the formula documented in the _AppliedTo page is wrong and is a typo? It should be "Weighted = (High + Low + Close + Close)/4"? That's the only correct formula, right?
Thanks.
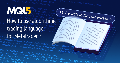
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I'm generating a EA through MetaEditor and I don't know what those words mean. Is it refering to the price when is supposed to close the order or?
I'm really confused.