- yogureee: it doesn't work at all."Doesn't work" is meaningless - just like saying the car doesn't work. Doesn't start, won't go in gear, no electrical, missing the key, flat tires - meaningless. We can't see your broken code. There are no mind readers here and our crystal balls are cracked.
double Close[]; ArraySetAsSeries(Close, true); CopyClose(_Symbol, PERIOD_CURRENT, TimeCurrent(), 2, Close); datetime Close_time[]; ArraySetAsSeries(Close_time, true); CopyTime(_Symbol, PERIOD_CURRENT, TimeCurrent(), 2, Close_time); double Open[]; ArraySetAsSeries(Open, true); CopyOpen(_Symbol, PERIOD_CURRENT, TimeCurrent(), 2, Open);
Why are you doing this when you already have arrays open[], close[], and time[]?- Use the debugger or print out your variables, including and find out why.
- "Doesn't work" is meaningless - just like saying the car doesn't work. Doesn't start, won't go in gear, no electrical, missing the key, flat tires - meaningless. We can't see your broken code. There are no mind readers here and our crystal balls are cracked.
- Why are you doing this when you already have arrays open[], close[], and time[]?
- Use the debugger or print out your variables, including and find out why.
Thank you for taking time reading my wrong code and for your advice.
I will post here again when I find out the problems and how I should code it.
For now, it is difficult to see whether the code is redundant or not.
I use " Print ("A = ", A); " for both MT4 and MT5, and it is very helpful to see how the code works.
I will try Debug.
If you use the latest build you should be able to use the same MQL4 code thanks to the newly added functions.
Thank you for the information.
I use the latest build, Version: 5.00 build 1816.
I'm afraid I can't find the newly added functions that enable MQL4 code be available in the MT5 MetaEditor.
How can I find them or articles about it?
Thank you for the information.
I use the latest build, Version: 5.00 build 1816.
I'm afraid I can't find the newly added functions that enable MQL4 code be available in the MT5 MetaEditor.
How can I find them or articles about it?
By "latest build" I think he meant 1845, which is in Beta. See the release statement:
Search for "MQL5: New functions: iTime, iOpen, iHigh, iLow, iClose, iVolume, iBars, iBarShift, iLowest, iHighest, iRealVolume, iTickVolume, iSpread."By "latest build" I think he meant 1845, which is in Beta. See the release statement:
Search for "MQL5: New functions: iTime, iOpen, iHigh, iLow, iClose, iVolume, iBars, iBarShift, iLowest, iHighest, iRealVolume, iTickVolume, iSpread."Thank you again for your information.
I will install the latest build on this weekend, and restart my converting when the stable version is released.
I'm looking forward to other new features too.
We already have ".......const double &close[]........ " as formal parameters of "int OnCalculate()" ,
so I don't need "double Close[]......." anymore. These are redundant.
I should use "close", and wrote like this:
ArraySetAsSeries(close, true); CopyClose(_Symbol, PERIOD_CURRENT, TimeCurrent(), 2, close);
But I got an error.
I tried these 3 codes above. Still error.
I read: https://www.mql5.com/en/forum/1316 I still don't know what to do.
Could anyone give me helpful advice?
Converting is too difficult.
Instead, I modified the MQL4 alert indicator to MQL5 one which works but the codes are not good at all.
#property indicator_chart_window input int SoundInterval = 30; input bool Check_HorizontalLine = true; input bool Check_TrendLine = true; bool AlertFlag = false; int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]) { int obj_total = ObjectsTotal(0, 0, -1); //Print("obj_total: ", obj_total); string name; for (int i = 0; i < obj_total; i++) { name = ObjectName(0, i, 0, -1); if(ObjectGetInteger(0, name, OBJPROP_TYPE) != OBJ_HLINE && ObjectGetInteger(0, name, OBJPROP_TYPE) != OBJ_TREND) continue; if (!Check_HorizontalLine && ObjectGetInteger(0, name, OBJPROP_TYPE) == OBJ_HLINE) continue; if (!Check_TrendLine && ObjectGetInteger(0, name, OBJPROP_TYPE) == OBJ_TREND) continue; double HorizontallinePrice, TrendlinePrice; double Close[]; ArraySetAsSeries(Close, true); CopyClose(_Symbol, PERIOD_CURRENT, TimeCurrent(), 2, Close); //Print("Close[0] = ", Close[0]); datetime Close_time[]; ArraySetAsSeries(Close_time, true); CopyTime(_Symbol, PERIOD_CURRENT, TimeCurrent(), 2, Close_time); double Open[]; ArraySetAsSeries(Open, true); CopyOpen(_Symbol, PERIOD_CURRENT, TimeCurrent(), 2, Open); if (ObjectGetInteger(0, name, OBJPROP_TYPE) == OBJ_HLINE) { HorizontallinePrice = ObjectGetDouble(0, name, OBJPROP_PRICE); //Print("horizontalline_price = ", HorizontallinePrice); } if (ObjectGetInteger(0, name, OBJPROP_TYPE) == OBJ_TREND) { TrendlinePrice = ObjectGetValueByTime(0, name, Close_time[0]); //Print("trendline_price = ", TrendlinePrice); } if((HorizontallinePrice > Open[0]) && (HorizontallinePrice <= Close[0])) { if(AlertFlag == false) { Alert(_Symbol+_Period+" UP @" +DoubleToString(Close[0], _Digits)+" over " +DoubleToString(HorizontallinePrice, _Digits)+" ["+name+"]"); AlertFlag = true; } } else if((TrendlinePrice > Open[0]) && (TrendlinePrice <= Close[0])) { if(AlertFlag == false) { Alert(_Symbol+_Period+" UP @" +DoubleToString(Close[0], _Digits)+" over " +DoubleToString(TrendlinePrice, _Digits)+" ["+name+"]"); AlertFlag = true; } } else if((HorizontallinePrice < Open[0]) && (HorizontallinePrice >= Close[0])) { if(AlertFlag == false) { Alert(_Symbol+_Period+" DOWN @" +DoubleToString(Close[0], _Digits)+" under " +DoubleToString(HorizontallinePrice, _Digits)+" ["+name+"]"); AlertFlag = true; } } else if((TrendlinePrice < Open[0]) && (TrendlinePrice >= Close[0])) { if(AlertFlag == false) { Alert(_Symbol+_Period+" DOWN @" +DoubleToString(Close[0], _Digits)+" under " +DoubleToString(TrendlinePrice, _Digits)+" ["+name+"]"); AlertFlag = true; } } else { AlertFlag = false; } } return(rates_total); }
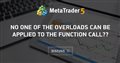
- 2010.07.06
- www.mql5.com
When you open a demo account on MetaQuotes Demo Server then your terminal will shortly update to 1846 and you can omit copyclose and use the regulat iClose iOpen iHigh iLow and etc.
You can see these functions here: https://www.mql5.com/en/forum/254082
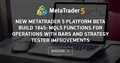
- 2018.06.08
- www.mql5.com
When you open a demo account on MetaQuotes Demo Server then your terminal will shortly update to 1846 and you can omit copyclose and use the regulat iClose iOpen iHigh iLow and etc.
You can see these functions here: https://www.mql5.com/en/forum/254082
Thank you for the information.
I was able to get 1847.
Now I can forget about CopyClose(), and I will try iClose .... later.
ArraySetAsSeries(close, true); iClose(_Symbol, PERIOD_CURRENT, 0); // current bar
My codes got better. I appreciate MT5, MQL5 experts who gave me advice and information here.
#property copyright "Copyright 2018, MetaQuotes Software Corp." #property link "https://www.mql5.com" #property version "1.00" #property indicator_chart_window input int SoundInterval = 30; input bool Check_HorizontalLine = true; input bool Check_TrendLine = true; bool AlertFlag = false; int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]) { int obj_total = ObjectsTotal(0, 0, -1); Print("obj_total = ", obj_total); string name; for (int i = 0; i < obj_total; i++) { name = ObjectName(0, i, 0, -1); if(ObjectGetInteger(0, name, OBJPROP_TYPE) != OBJ_HLINE && ObjectGetInteger(0, name, OBJPROP_TYPE) != OBJ_TREND) continue; if (!Check_HorizontalLine && ObjectGetInteger(0, name, OBJPROP_TYPE) == OBJ_HLINE) continue; if (!Check_TrendLine && ObjectGetInteger(0, name, OBJPROP_TYPE) == OBJ_TREND) continue; double HorizontallinePrice, TrendlinePrice; ArraySetAsSeries(time, true); iTime(_Symbol, PERIOD_CURRENT, 0); Print("time[0] = ", time[0]); ArraySetAsSeries(open, true); iOpen(_Symbol, PERIOD_CURRENT, 0); Print("open[0] = ", open[0]); ArraySetAsSeries(close, true); iClose(_Symbol, PERIOD_CURRENT, 0); Print("close[0] = ", close[0]); if (ObjectGetInteger(0, name, OBJPROP_TYPE) == OBJ_HLINE) { HorizontallinePrice = ObjectGetDouble(0, name, OBJPROP_PRICE); Print("horizontalline_price = ", HorizontallinePrice); } if (ObjectGetInteger(0, name, OBJPROP_TYPE) == OBJ_TREND) { TrendlinePrice = ObjectGetValueByTime(0, name, time[0]); Print("trendline_price = ", TrendlinePrice); } if((HorizontallinePrice > open[0]) && (HorizontallinePrice <= close[0])) { if(AlertFlag == false) { Alert(_Symbol+_Period+" UP @" +DoubleToString(close[0], _Digits)+" over " +DoubleToString(HorizontallinePrice, _Digits)+" ["+name+"]"); AlertFlag = true; } } else if((TrendlinePrice > open[0]) && (TrendlinePrice <= close[0])) { if(AlertFlag == false) { Alert(_Symbol+_Period+" UP @" +DoubleToString(close[0], _Digits)+" over " +DoubleToString(TrendlinePrice, _Digits)+" ["+name+"]"); AlertFlag = true; } } else if((HorizontallinePrice < open[0]) && (HorizontallinePrice >= close[0])) { if(AlertFlag == false) { Alert(_Symbol+_Period+" DOWN @" +DoubleToString(close[0], _Digits)+" under " +DoubleToString(HorizontallinePrice, _Digits)+" ["+name+"]"); AlertFlag = true; } } else if((TrendlinePrice < open[0]) && (TrendlinePrice >= close[0])) { if(AlertFlag == false) { Alert(_Symbol+_Period+" DOWN @" +DoubleToString(close[0], _Digits)+" under " +DoubleToString(TrendlinePrice, _Digits)+" ["+name+"]"); AlertFlag = true; } } else { AlertFlag = false; } } return(rates_total); }

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello.
This MQL4 alert indicator gives alerts everytime the price touches the horizontal lines or trendlines you drew.
I converted it to MQL5, and it doesn't work at all.
Can anyone help me with this problem, please? Thank you in advance.