Not sure if this is what you want to do, but this script toggles the market watch symbol list.
//+------------------------------------------------------------------+ //| FilterMarketWatch.mq4 | //| Copyright 2017, nicholishen | //| https://www.forexfactory.com/nicholishen | //+------------------------------------------------------------------+ #property copyright "Copyright 2017, nicholishen" #property link "https://www.forexfactory.com/nicholishen" #property version "1.00" #property strict #include <Arrays\ArrayString.mqh> class ToggleWatchList { protected: bool m_mode; CArrayString m_default; CArrayString m_desired; public: ToggleWatchList():m_mode(false){} ~ToggleWatchList() { m_mode = true; Toggle(); } void Init(string &symbol_array[]) { for(int i=0;i<SymbolsTotal(true);i++) m_default.Add(SymbolName(i,true)); m_desired.AddArray(symbol_array); } void Toggle() { if(!m_mode) { for(int i=SymbolsTotal(true)-1;i>=0;i--) SymbolSelect(SymbolName(i,true),false); for(int i=0;i<m_desired.Total();i++) { for(int j=SymbolsTotal(false)-1;j>=0;j--) { if(StringFind(SymbolName(j,false),m_desired[i])>=0) { SymbolSelect(SymbolName(j,false),true); break; } } } } else { for(int i=SymbolsTotal(true)-1;i>=0;i--) SymbolSelect(SymbolName(i,true),false); for(int i=0;i<m_default.Total();i++) SymbolSelect(m_default[i],true); } m_mode= !m_mode; } }; void OnStart() { //--- string symbols[]={"USDJPY","GBPUSD","EURUSD"}; ToggleWatchList t; t.Init(symbols); t.Toggle(); Sleep(10000); t.Toggle(); Sleep(10000); t.Toggle(); Sleep(10000); } //+------------------------------------------------------------------+
Not sure if this is what you want to do, but this script toggles the market watch symbol list.
I tested your script and it worked as you described
I tested the code in my timer void:
void OnTimer() { EventSetTimer(deltaTime); //set and count the symbols data TotalSymbols=FindSymbols(); ArrayResize(Symbols,TotalSymbols); ArrayResize(Descr,TotalSymbols); string var="variable"; //Clean up variables deleteVariables(); //set Headers int posY=0; displayHeaders(var,posY,acceptableMargin,tf); //clean up old display instrumentList list; list.Clear(); //Fill Market Watch - have to or does not have any values //for(int j=0; j<TotalSymbols; j++)//all Instruments // { // if(!IsSymbolInMarketWatch(Symbols[j])) // SymbolSelect(SymbolName(j,true),true); // } //delete instruments that dont match criterion bool isInWatch; for(int j=0; j<TotalSymbols; j++)//all Instruments { isInWatch=IsSymbolInMarketWatch(Symbols[j]); if(isInWatch) list.Add(new instrument(Symbols[j],Descr[j],tf,indPeriod,adxAgo,maxBars,equityRisk,acceptableMargin)); } list.Sort(sortVar); //shortenList list.shortenList(watchFill); //Fill putInWatch[] string putInWatch[1000]; int sizeofPutInWatch=list.createArray(putInWatch); Print("sizeofPutInWatchList: ",sizeofPutInWatch); ArrayResize(putInWatch,sizeofPutInWatch); Print("toggleList"); ToggleWatchList t; t.Init(putInWatch); t.Toggle(); Print("sleep1"); Sleep(10000); t.Toggle(); Print("sleep2"); Sleep(10000); t.Toggle(); Print("sleep3"); Sleep(10000); posY++;//yaxis for display //show top watchfill symbols list.displayData(var,posY,sortVar); //write to file symbols that are of interest list.writeInstruments(); Print("done!!!"); //remove dead wood //for(int i=0; i<TotalSymbols; i++)//all Instruments // { // if(!list.isInTop(Symbols[i])) // { // isInWatch=SymbolSelect(Symbols[i],false); // // Print (Symbols[i]+" "+string(isInWatch)); // } // } }
//+------------------------------------------------------------------+ //|Put in watch | //+------------------------------------------------------------------+ int createArray(string &array[]) { int count=0; for(instrument *j=GetFirstNode();j!=NULL;j=j.Next()) { array[count]=j.symbol; count++; } return count; }
Unfortunately the watch symbols list did not update to reflect the toggles.
I believe the reason is that I do some number crunching on the symbols in the list before it is toggled ... I think that is why the script works but the event above does not
Documentation:
Selects a symbol in the Market Watch window or removes a symbol from the window. bool SymbolSelect( string name, // symbol name bool select // add or remove );
Note
To get symbol data using functions for accessing timeseries and indicators, make sure that the symbol exists in the MarketWatch window. If the symbols is not available in Market watch, enable it using the SymbolSelect(symbol_name, true) function before you request the data.
The symbol can be hidden from MarketWatch after 10 minutes since the last access to the symbol history, i.e. since the call of functions like iOpen(), iHigh(), CopyTime() etc. This is due to the fact that the terminal stores symbol data for 10 minutes since the last access to them; after that unused data are automatically deleted from the terminal memory.
//--
Unless I've missed something I have tried changing the watch list contents after 11 minutes and it didnt work and is not really a solution. Think I need to reset the terminals symbols data. If this is true can someone agree. If I've gone wrong in my thinking please throw a little light
Many Thanks
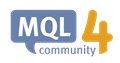
- docs.mql4.com
** Of course if the watch list is not changeable for ten minutes after operation on historical data then I shouldnt be able to change the list manually either and guess what I can't :).
I'll try again with the ten minute delay before updating
tried this in the timer event:
. . . string putInWatch[1000]; int sizeofPutInWatch=list.createArray(putInWatch); Print("sizeofPutInWatchList: ",sizeofPutInWatch); ArrayResize(putInWatch,sizeofPutInWatch); Print("toggleList"); Print ("starting Delay"); Sleep(700000);//Minutes ToggleWatchList t; t.Init(putInWatch); t.Toggle(); Print("sleep1"); Sleep(10000); t.Toggle();.
.
.
didn't work - no change in watch list contents but I was able to delete a symbol manually through mt4 terminal?
Ran the script toggleWatchList on a chart after my event timer had finished (no 11 minute delay) and it also could not toggle the watch list elements. So....Why the 11 minute hold failed programatically I don't know.
How to nullify the terminals storage of symbol data for 10 minutes is also unknown, unsearchable and maybe not a good idea?
https://www.mql5.com/en/forum/124577
Maybe running two terminals one to periodically invoke the sort Timer and write to file. the other to do the trading and read the file generated from the first script.
Maybe this will be an answer but have to say not keen to have two terminal up. Better to code it but I thinks out of ideas unless I can delete the terminal variables some how?
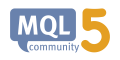
- 2010.03.14
- www.mql5.com
Ok .. I moved on to testing a multi-time frame multi-instrument system which renders a view of the watch list inapplicable for my case.
I leave the post as information for someone else stumbling down this road.
Many thanks
Hello everyone, i need help for coding....
i don't know why my script don't delete any symbol from market watch....
//+------------------------------------------------------------------+ //| test.mq4 | //| Copyright 2019, MetaQuotes Software Corp. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2019, MetaQuotes Software Corp." #property link "https://www.mql5.com" #property version "1.00" #property strict //+------------------------------------------------------------------+ //| Script program start function | //+------------------------------------------------------------------+ void OnStart() { int total=SymbolsTotal(true); for(int i=total-1; i>=0; i--) { string name=SymbolName(i,true); if(iMA(name,PERIOD_M1,50,0,MODE_EMA,PRICE_CLOSE,1)<iMA(name,PERIOD_M1,160,0,MODE_EMA,PRICE_CLOSE,1)) bool deletesymbol=SymbolSelect(name,false); } } //+------------------------------------------------------------------+
Hello everyone, i need help for coding....
i don't know why my script don't delete any symbol from market watch....
Did you read the documentation?
A symbol can't be removed if the symbol chart is open, or there are open orders for this symbol.
The symbol can be hidden from MarketWatch after 10 minutes since the last access to the symbol history, i.e. since the call of functions like iOpen(), iHigh(), CopyTime() etc. This is due to the fact that the terminal stores symbol data for 10 minutes since the last access to them; after that unused data are automatically deleted from the terminal memory.
Did you read the documentation?
A symbol can't be removed if the symbol chart is open, or there are open orders for this symbol.
The symbol can be hidden from MarketWatch after 10 minutes since the last access to the symbol history, i.e. since the call of functions like iOpen(), iHigh(), CopyTime() etc. This is due to the fact that the terminal stores symbol data for 10 minutes since the last access to them; after that unused data are automatically deleted from the terminal memory.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
A timer within a EA that loads all watch symbols and supposed to weeds out the ones I dont want:
Sorts the List and displays the data
the timer void:
All good short output with a few symbols shown!
Effect Required: Now I want to re populate the watch List with the symbols I have chosen : Documentation The symbol can be hidden from MarketWatch after 10 minutes ....
Tried 3 methods:
1/. Loading Templates sequentially
2/. seperate Ea's for creation and filling watch list - documentation I think says no!
2/.stalling for eleven minutes - not nice but still couldn't delete unwanted symbols - last hope!
None worked out
Above is what I want to do - here im trying to remove symbols I dont want - doesnt work for documented reasons
So question might be what am I trying to do - hide them or remove them - or is it possible to perform both of these operations?
What I want to do is avoid polling unwanted symbols in the EA's
..and I want a watch list that is easy on the eye - just the symbols I want to see. If anyone has any ideas or can give me a pointer please comment.