Post the code you are running and I'll take a look at it. (and please use the SRC button and then paste the code in)
The only difference with mt5 platform installation that I have done are the junction on other hard disk (not ssd) to decrease the wear.
I post the code I have used to testing with same result:
//+------------------------------------------------------------------+
//| macd.mq5 |
//| Copyright 2016 pietro caporale |
//| http://strategy-trader.net/ |
//+------------------------------------------------------------------+
#property copyright "Copyright 2016 pietro caporale"
#property link "http://strategy-trader.net/"
#property version "1.00"
//+------------------------------------------------------------------+
//| Include |
//+------------------------------------------------------------------+
#include <Expert\Expert.mqh>
//--- available signals
//#include <Expert\Signal\SignalMACD.mqh>
#include "SignalMACD.mqh"
//--- available trailing
#include <Expert\Trailing\TrailingNone.mqh>
//--- available money management
#include <Expert\Money\MoneyFixedLot.mqh>
//#include "sql_manage.mqh"
bool sqlWrite = true; // enable sql updating
//--- inputs for expert
input string Expert_Title ="macd"; // Document name
ulong Expert_MagicNumber =4952; //
bool Expert_EveryTick =false; //
//--- inputs for main signal
input int Signal_ThresholdOpen =10; // Signal threshold value to open [0...100]
input int Signal_ThresholdClose =10; // Signal threshold value to close [0...100]
input double Signal_PriceLevel =0.0; // Price level to execute a deal
input double Signal_StopLevel =50.0; // Stop Loss level (in pips)
input double Signal_TakeLevel =50.0; // Take Profit level (in pips)
input int Signal_Expiration =4; // Expiration of pending orders (in bars)
input int Signal_MACD_PeriodFast =12; // MACD(12,24,9,PRICE_CLOSE) Period of fast EMA
input int Signal_MACD_PeriodSlow =24; // MACD(12,24,9,PRICE_CLOSE) Period of slow EMA
input int Signal_MACD_PeriodSignal=9; // MACD(12,24,9,PRICE_CLOSE) Period of averaging of difference
input ENUM_APPLIED_PRICE Signal_MACD_Applied =PRICE_CLOSE; // MACD(12,24,9,PRICE_CLOSE) Prices series
input double Signal_MACD_Weight =1.0; // MACD(12,24,9,PRICE_CLOSE) Weight [0...1.0]
//--- inputs for money
input double Money_FixLot_Percent =10.0; // Percent
input double Money_FixLot_Lots =0.1; // Fixed volume
//+------------------------------------------------------------------+
//| Global expert object |
//+------------------------------------------------------------------+
CExpert ExtExpert;
//+------------------------------------------------------------------+
//| Expert initialization function |
//+------------------------------------------------------------------+
int OnInit()
{
//--- create timer
EventSetTimer(60);
//--- Initializing expert
if(!ExtExpert.Init(Symbol(),Period(),Expert_EveryTick,Expert_MagicNumber))
{
//--- failed
printf(__FUNCTION__+": error initializing expert");
ExtExpert.Deinit();
return(INIT_FAILED);
}
//--- Creating signal
CExpertSignal *signal=new CExpertSignal;
if(signal==NULL)
{
//--- failed
printf(__FUNCTION__+": error creating signal");
ExtExpert.Deinit();
return(INIT_FAILED);
}
//---
ExtExpert.InitSignal(signal);
signal.ThresholdOpen(Signal_ThresholdOpen);
signal.ThresholdClose(Signal_ThresholdClose);
signal.PriceLevel(Signal_PriceLevel);
signal.StopLevel(Signal_StopLevel);
signal.TakeLevel(Signal_TakeLevel);
signal.Expiration(Signal_Expiration);
//--- Creating filter CSignalMACD
CSignalMACD *filter0=new CSignalMACD;
if(filter0==NULL)
{
//--- failed
printf(__FUNCTION__+": error creating filter0");
ExtExpert.Deinit();
return(INIT_FAILED);
}
signal.AddFilter(filter0);
filter0.PatternsUsage(2);
//filter0.PatternsUsage(4);
//--- Set filter parameters
filter0.PeriodFast(Signal_MACD_PeriodFast);
filter0.PeriodSlow(Signal_MACD_PeriodSlow);
filter0.PeriodSignal(Signal_MACD_PeriodSignal);
filter0.Applied(Signal_MACD_Applied);
filter0.Weight(Signal_MACD_Weight);
//--- Creation of money object
CMoneyFixedLot *money=new CMoneyFixedLot;
if(money==NULL)
{
//--- failed
printf(__FUNCTION__+": error creating money");
ExtExpert.Deinit();
return(INIT_FAILED);
}
//--- Add money to expert (will be deleted automatically))
if(!ExtExpert.InitMoney(money))
{
//--- failed
printf(__FUNCTION__+": error initializing money");
ExtExpert.Deinit();
return(INIT_FAILED);
}
//--- Set money parameters
money.Percent(Money_FixLot_Percent);
money.Lots(Money_FixLot_Lots);
//--- Check all trading objects parameters
if(!ExtExpert.ValidationSettings())
{
//--- failed
ExtExpert.Deinit();
return(INIT_FAILED);
}
//--- Tuning of all necessary indicators
if(!ExtExpert.InitIndicators())
{
//--- failed
printf(__FUNCTION__+": error initializing indicators");
ExtExpert.Deinit();
return(INIT_FAILED);
}
//--- ok
return(INIT_SUCCEEDED);
}
//+------------------------------------------------------------------+
//| Expert deinitialization function |
//+------------------------------------------------------------------+
void OnDeinit(const int reason)
{
//--- destroy timer
EventKillTimer();
ExtExpert.Deinit();
}
//+------------------------------------------------------------------+
//| Expert tick function |
//+------------------------------------------------------------------+
void OnTick()
{
//---
ExtExpert.OnTick();
}
//+------------------------------------------------------------------+
//| Timer function |
//+------------------------------------------------------------------+
void OnTimer()
{
//---
ExtExpert.OnTimer();
}
//+------------------------------------------------------------------+
//| Trade function |
//+------------------------------------------------------------------+
void OnTrade()
{
//---
ExtExpert.OnTrade();
}
//+------------------------------------------------------------------+
//| ChartEvent function |
//+------------------------------------------------------------------+
void OnChartEvent(const int id,
const long &lparam,
const double &dparam,
const string &sparam)
{
//---
}
//+------------------------------------------------------------------+
**************************************************************************************************************************
thanks a lot.
//| macd.mq5 |
//| Copyright 2016 pietro caporale |
//| http://strategy-trader.net/ |
//+------------------------------------------------------------------+
#property copyright "Copyright 2016 pietro caporale"
#property link "http://strategy-trader.net/"
#property version "1.00"
//+------------------------------------------------------------------+
//| Include |
//+------------------------------------------------------------------+
#include <Expert\Expert.mqh>
//--- available signals
//#include <Expert\Signal\SignalMACD.mqh>
#include "SignalMACD.mqh"
//--- available trailing
#include <Expert\Trailing\TrailingNone.mqh>
//--- available money management
#include <Expert\Money\MoneyFixedLot.mqh>
//#include "sql_manage.mqh"
bool sqlWrite = true; // enable sql updating
//--- inputs for expert
input string Expert_Title ="macd"; // Document name
ulong Expert_MagicNumber =4952; //
bool Expert_EveryTick =false; //
//--- inputs for main signal
input int Signal_ThresholdOpen =10; // Signal threshold value to open [0...100]
input int Signal_ThresholdClose =10; // Signal threshold value to close [0...100]
input double Signal_PriceLevel =0.0; // Price level to execute a deal
input double Signal_StopLevel =50.0; // Stop Loss level (in pips)
input double Signal_TakeLevel =50.0; // Take Profit level (in pips)
input int Signal_Expiration =4; // Expiration of pending orders (in bars)
input int Signal_MACD_PeriodFast =12; // MACD(12,24,9,PRICE_CLOSE) Period of fast EMA
input int Signal_MACD_PeriodSlow =24; // MACD(12,24,9,PRICE_CLOSE) Period of slow EMA
input int Signal_MACD_PeriodSignal=9; // MACD(12,24,9,PRICE_CLOSE) Period of averaging of difference
input ENUM_APPLIED_PRICE Signal_MACD_Applied =PRICE_CLOSE; // MACD(12,24,9,PRICE_CLOSE) Prices series
input double Signal_MACD_Weight =1.0; // MACD(12,24,9,PRICE_CLOSE) Weight [0...1.0]
//--- inputs for money
input double Money_FixLot_Percent =10.0; // Percent
input double Money_FixLot_Lots =0.1; // Fixed volume
//+------------------------------------------------------------------+
//| Global expert object |
//+------------------------------------------------------------------+
CExpert ExtExpert;
//+------------------------------------------------------------------+
//| Expert initialization function |
//+------------------------------------------------------------------+
int OnInit()
{
//--- create timer
EventSetTimer(60);
//--- Initializing expert
if(!ExtExpert.Init(Symbol(),Period(),Expert_EveryTick,Expert_MagicNumber))
{
//--- failed
printf(__FUNCTION__+": error initializing expert");
ExtExpert.Deinit();
return(INIT_FAILED);
}
//--- Creating signal
CExpertSignal *signal=new CExpertSignal;
if(signal==NULL)
{
//--- failed
printf(__FUNCTION__+": error creating signal");
ExtExpert.Deinit();
return(INIT_FAILED);
}
//---
ExtExpert.InitSignal(signal);
signal.ThresholdOpen(Signal_ThresholdOpen);
signal.ThresholdClose(Signal_ThresholdClose);
signal.PriceLevel(Signal_PriceLevel);
signal.StopLevel(Signal_StopLevel);
signal.TakeLevel(Signal_TakeLevel);
signal.Expiration(Signal_Expiration);
//--- Creating filter CSignalMACD
CSignalMACD *filter0=new CSignalMACD;
if(filter0==NULL)
{
//--- failed
printf(__FUNCTION__+": error creating filter0");
ExtExpert.Deinit();
return(INIT_FAILED);
}
signal.AddFilter(filter0);
filter0.PatternsUsage(2);
//filter0.PatternsUsage(4);
//--- Set filter parameters
filter0.PeriodFast(Signal_MACD_PeriodFast);
filter0.PeriodSlow(Signal_MACD_PeriodSlow);
filter0.PeriodSignal(Signal_MACD_PeriodSignal);
filter0.Applied(Signal_MACD_Applied);
filter0.Weight(Signal_MACD_Weight);
//--- Creation of money object
CMoneyFixedLot *money=new CMoneyFixedLot;
if(money==NULL)
{
//--- failed
printf(__FUNCTION__+": error creating money");
ExtExpert.Deinit();
return(INIT_FAILED);
}
//--- Add money to expert (will be deleted automatically))
if(!ExtExpert.InitMoney(money))
{
//--- failed
printf(__FUNCTION__+": error initializing money");
ExtExpert.Deinit();
return(INIT_FAILED);
}
//--- Set money parameters
money.Percent(Money_FixLot_Percent);
money.Lots(Money_FixLot_Lots);
//--- Check all trading objects parameters
if(!ExtExpert.ValidationSettings())
{
//--- failed
ExtExpert.Deinit();
return(INIT_FAILED);
}
//--- Tuning of all necessary indicators
if(!ExtExpert.InitIndicators())
{
//--- failed
printf(__FUNCTION__+": error initializing indicators");
ExtExpert.Deinit();
return(INIT_FAILED);
}
//--- ok
return(INIT_SUCCEEDED);
}
//+------------------------------------------------------------------+
//| Expert deinitialization function |
//+------------------------------------------------------------------+
void OnDeinit(const int reason)
{
//--- destroy timer
EventKillTimer();
ExtExpert.Deinit();
}
//+------------------------------------------------------------------+
//| Expert tick function |
//+------------------------------------------------------------------+
void OnTick()
{
//---
ExtExpert.OnTick();
}
//+------------------------------------------------------------------+
//| Timer function |
//+------------------------------------------------------------------+
void OnTimer()
{
//---
ExtExpert.OnTimer();
}
//+------------------------------------------------------------------+
//| Trade function |
//+------------------------------------------------------------------+
void OnTrade()
{
//---
ExtExpert.OnTrade();
}
//+------------------------------------------------------------------+
//| ChartEvent function |
//+------------------------------------------------------------------+
void OnChartEvent(const int id,
const long &lparam,
const double &dparam,
const string &sparam)
{
//---
}
//+------------------------------------------------------------------+
Good question, I had not read well that this time you looked anyway.
No any change ...
I had some very challenging days.
Hi Everyone.
I am experiencing the same problem. I am creating the EA with the wizard "Generate" and the only change I make is by selecting "Moving Average" as the indicator. (Note that the error persist when selecting other indicators)
Error: "2019.11.07 09:24:27.512 Core 1 tester stopped because OnInit returns non-zero code"
The generated code:
//+------------------------------------------------------------------+ //| TestMql5EA.mq5 | //| Copyright 2019, MetaQuotes Software Corp. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2019, MetaQuotes Software Corp." #property link "https://www.mql5.com" #property version "1.00" //+------------------------------------------------------------------+ //| Include | //+------------------------------------------------------------------+ #include <Expert\Expert.mqh> //--- available signals #include <Expert\Signal\SignalMA.mqh> //--- available trailing #include <Expert\Trailing\TrailingNone.mqh> //--- available money management #include <Expert\Money\MoneyFixedLot.mqh> //+------------------------------------------------------------------+ //| Inputs | //+------------------------------------------------------------------+ //--- inputs for expert input string Expert_Title ="TestMql5EA"; // Document name ulong Expert_MagicNumber =1712; // bool Expert_EveryTick =false; // //--- inputs for main signal input int Signal_ThresholdOpen =10; // Signal threshold value to open [0...100] input int Signal_ThresholdClose=10; // Signal threshold value to close [0...100] input double Signal_PriceLevel =0.0; // Price level to execute a deal input double Signal_StopLevel =50.0; // Stop Loss level (in points) input double Signal_TakeLevel =50.0; // Take Profit level (in points) input int Signal_Expiration =4; // Expiration of pending orders (in bars) input int Signal_MA_PeriodMA =12; // Moving Average(12,0,...) Period of averaging input int Signal_MA_Shift =0; // Moving Average(12,0,...) Time shift input ENUM_MA_METHOD Signal_MA_Method =MODE_SMA; // Moving Average(12,0,...) Method of averaging input ENUM_APPLIED_PRICE Signal_MA_Applied =PRICE_CLOSE; // Moving Average(12,0,...) Prices series input double Signal_MA_Weight =1.0; // Moving Average(12,0,...) Weight [0...1.0] //--- inputs for money input double Money_FixLot_Percent =10.0; // Percent input double Money_FixLot_Lots =0.1; // Fixed volume //+------------------------------------------------------------------+ //| Global expert object | //+------------------------------------------------------------------+ CExpert ExtExpert; //+------------------------------------------------------------------+ //| Initialization function of the expert | //+------------------------------------------------------------------+ int OnInit() { //--- Initializing expert if(!ExtExpert.Init(Symbol(),Period(),Expert_EveryTick,Expert_MagicNumber)) { //--- failed printf(__FUNCTION__+": error initializing expert"); ExtExpert.Deinit(); return(INIT_FAILED); } //--- Creating signal CExpertSignal *signal=new CExpertSignal; if(signal==NULL) { //--- failed printf(__FUNCTION__+": error creating signal"); ExtExpert.Deinit(); return(INIT_FAILED); } //--- ExtExpert.InitSignal(signal); signal.ThresholdOpen(Signal_ThresholdOpen); signal.ThresholdClose(Signal_ThresholdClose); signal.PriceLevel(Signal_PriceLevel); signal.StopLevel(Signal_StopLevel); signal.TakeLevel(Signal_TakeLevel); signal.Expiration(Signal_Expiration); //--- Creating filter CSignalMA CSignalMA *filter0=new CSignalMA; if(filter0==NULL) { //--- failed printf(__FUNCTION__+": error creating filter0"); ExtExpert.Deinit(); return(INIT_FAILED); } signal.AddFilter(filter0); //--- Set filter parameters filter0.PeriodMA(Signal_MA_PeriodMA); filter0.Shift(Signal_MA_Shift); filter0.Method(Signal_MA_Method); filter0.Applied(Signal_MA_Applied); filter0.Weight(Signal_MA_Weight); //--- Creation of trailing object CTrailingNone *trailing=new CTrailingNone; if(trailing==NULL) { //--- failed printf(__FUNCTION__+": error creating trailing"); ExtExpert.Deinit(); return(INIT_FAILED); } //--- Add trailing to expert (will be deleted automatically)) if(!ExtExpert.InitTrailing(trailing)) { //--- failed printf(__FUNCTION__+": error initializing trailing"); ExtExpert.Deinit(); return(INIT_FAILED); } //--- Set trailing parameters //--- Creation of money object CMoneyFixedLot *money=new CMoneyFixedLot; if(money==NULL) { //--- failed printf(__FUNCTION__+": error creating money"); ExtExpert.Deinit(); return(INIT_FAILED); } //--- Add money to expert (will be deleted automatically)) if(!ExtExpert.InitMoney(money)) { //--- failed printf(__FUNCTION__+": error initializing money"); ExtExpert.Deinit(); return(INIT_FAILED); } //--- Set money parameters money.Percent(Money_FixLot_Percent); money.Lots(Money_FixLot_Lots); //--- Check all trading objects parameters if(!ExtExpert.ValidationSettings()) { //--- failed ExtExpert.Deinit(); return(INIT_FAILED); } //--- Tuning of all necessary indicators if(!ExtExpert.InitIndicators()) { //--- failed printf(__FUNCTION__+": error initializing indicators"); ExtExpert.Deinit(); return(INIT_FAILED); } //--- ok return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Deinitialization function of the expert | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { ExtExpert.Deinit(); } //+------------------------------------------------------------------+ //| "Tick" event handler function | //+------------------------------------------------------------------+ void OnTick() { ExtExpert.OnTick(); } //+------------------------------------------------------------------+ //| "Trade" event handler function | //+------------------------------------------------------------------+ void OnTrade() { ExtExpert.OnTrade(); } //+------------------------------------------------------------------+ //| "Timer" event handler function | //+------------------------------------------------------------------+ void OnTimer() { ExtExpert.OnTimer(); } //+------------------------------------------------------------------+
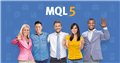
- www.mql5.com
Hi Everyone.
I am experiencing the same problem. I am creating the EA with the wizard "Generate" and the only change I make is by selecting "Moving Average" as the indicator. (Note that the error persist when selecting other indicators)
Error: " 2019.11.07 09:24:27.512 Core 1 tester stopped because OnInit returns non-zero code "
The generated code:
I checked your example on
2019.11.07 09:45:01.487 Terminal MetaTrader 5 x64 build 2201 started (MetaQuotes Software Corp.) 2019.11.07 09:45:01.489 Terminal Windows 10 (build 18362) x64, IE 11, UAC, Intel Core i3-3120M @ 2.50GHz, Memory: 3305 / 8077 Mb, Disk: 110 / 415 Gb, GMT+2 2019.11.07 09:45:01.489 Terminal C:\Users\barab\AppData\Roaming\MetaQuotes\Terminal\D0E8209F77C8CF37AD8BF550E51FF075
- launched a single test -> no errors
- I started testing on the history -> there are no errors.
I wonder what you are doing and on which hardware? How do you get the error? Describe your actions step by step.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I'm updating an EA, I just change my reference site but I get: Validation completed with an errors
test on EURUSD,H1 (netting)
tester stopped because OnInit failed
there are no trading operations
but before I loaded without problems...
I tried to load an Expert Advisor created using MQL5 Wizard and I had the same result.
Can someone help me?
Thank you for the time dedicated to me