Hi All,
I'm sorry if the question is basic but I'm new to coding :)
I have created the below closing script and added the SendNotification line but I would like to add the P&L of the deal to the message. How could I do that ?
Thanks _
Hi phi.nuts !!
Thank you for helping me! I see how these functions can help me get me the data but I'm not too sure how to code.
I tried the below but I get 2 errors:
- Possible loss of data due to type conversion
- 'SendNotification' - wrong parameters count
Not sure what wrong parameters count means ?
void ShortPositionClose() { MqlTradeRequest mrequest; // Will be used for trade requests MqlTradeResult mresult; // Will be used for results of trade requests ZeroMemory(mrequest); ZeroMemory(mresult); double Ask = SymbolInfoDouble(_Symbol,SYMBOL_ASK); // Ask price double Bid = SymbolInfoDouble(_Symbol,SYMBOL_BID); // Bid price if(PositionGetInteger(POSITION_TYPE)==POSITION_TYPE_SELL) { mrequest.action = TRADE_ACTION_DEAL; // Immediate order execution mrequest.price = NormalizeDouble(Ask,_Digits); // Latest ask price mrequest.sl = 0; // Stop Loss mrequest.tp = 0; // Take Profit mrequest.symbol = _Symbol; // Symbol mrequest.volume = Lot; // Number of lots to trade mrequest.magic = 0; // Magic Number mrequest.type = ORDER_TYPE_BUY; // Buy order mrequest.type_filling = ORDER_FILLING_FOK; // Order execution type mrequest.deviation=5; // Deviation from current price OrderSend(mrequest,mresult); // Send order double Ticket = mresult.order; double OutCome = HistoryDealGetDouble(Ticket,DEAL_PROFIT); SendNotification("BBWP Closed a Short position with P&L : ", OutCome); } }
Hi phi.nuts !!
Thank you for helping me! I see how these functions can help me get me the data but I'm not too sure how to code.
I tried the below but I get 2 errors:
- Possible loss of data due to type conversion
- 'SendNotification' - wrong parameters count
Not sure what wrong parameters count means ?
I think you have 1 error ('SendNotification' - wrong parameters count) and 1 warning (Possible loss of data due to type conversion). Don't worry about warnings, they are harmless.
Actually you've made three errors :)
double Ticket = mresult.order; double OutCome = HistoryDealGetDouble(Ticket,DEAL_PROFIT); SendNotification("BBWP Closed a Short position with P&L : ", OutCome);
1. Remember that you send deal order to open position (TRADE_ACTION_DEAL). So, what you need is deal ticket and not order ticket.
2. If you look at MqlTradeResult and click " deal ", you will see that type property of DEAL_POSITION_ID is long and not double . This is the warning of " Possible of of data due to type conversion " from long to double.
3. The parameter needed in SendNotification is only one that is a text of a string type, but you make it two, when you put comma in it. That is the error for " 'SendNotification' - wrong parameter count ".
So your code should looks like this :
long Ticket = mresult.deal; double OutCome = HistoryDealGetDouble(Ticket,DEAL_PROFIT); string text = "BBWP Closed a Short position with P&L : "+ OutCome; SendNotification(text);
Thank you so much for the explanations!!
As a beginner they are more valuable to me than the result. I look forward to put this to the test monday!
Hi phi.nuts,
I hope you'll see this because I can't crack it.
Below is where my closing code is and the problem is that (as you can see from the screenshot) the EA returns the right ticket number but it also returns zero as a profit value. I also tried with the DEAL_PRICE option and it also return zero.
I also tried to using the previous ticket number by doing Ticket-1 but it didn't help : ) A
Any ideas why HistoryDealGetDouble alway returns zero ?
//+------------------------------------------------------------------+ //| Close Short position | //+------------------------------------------------------------------+ void ShortPositionClose() { MqlTradeRequest mrequest; // Will be used for trade requests MqlTradeResult mresult; // Will be used for results of trade requests ZeroMemory(mrequest); ZeroMemory(mresult); double Ask = SymbolInfoDouble(_Symbol,SYMBOL_ASK); // Ask price double Bid = SymbolInfoDouble(_Symbol,SYMBOL_BID); // Bid price if(PositionGetInteger(POSITION_TYPE)==POSITION_TYPE_SELL) { mrequest.action = TRADE_ACTION_DEAL; // Immediate order execution mrequest.price = NormalizeDouble(Ask,_Digits); // Latest ask price mrequest.sl = 0; // Stop Loss mrequest.tp = 0; // Take Profit mrequest.symbol = _Symbol; // Symbol mrequest.volume = Lot; // Number of lots to trade mrequest.magic = 0; // Magic Number mrequest.type = ORDER_TYPE_BUY; // Buy order mrequest.type_filling = ORDER_FILLING_FOK; // Order execution type mrequest.deviation=5; // Deviation from current price OrderSend(mrequest,mresult); // Send order long Ticket = mresult.deal; double OutCome = HistoryDealGetDouble(Ticket,DEAL_PROFIT); string Text = "BBWP Closed a Short position with P&L : "+OutCome; Print(TimeCurrent()," Print Outcome ",OutCome," Ticket ",Ticket); SendNotification(Text); } } //+------------------------------------------------------------------+
Hi phi.nuts,
I hope you'll see this because I can't crack it.
Below is where my closing code is and the problem is that (as you can see from the screenshot) the EA returns the right ticket number but it also returns zero as a profit value. I also tried with the DEAL_PRICE option and it also return zero.
I also tried to using the previous ticket number by doing Ticket-1 but it didn't help : ) A
Any ideas why HistoryDealGetDouble alway returns zero ?
Ups, my bad, we have to select it first using HistoryDealSelect.
I check around, apparently we have to wait until MT5 is updated with trading history, in my case I wait for a sec. Maybe I have lousy connection :(
So the code will be like this.
double OutCome = 0.0; Ticket = mresult.deal; Sleep(1000); // <<============================ wait ! ResetLastError(); if (HistoryDealSelect(Ticket)) { OutCome = HistoryDealGetDouble(Ticket,DEAL_PROFIT); } else { Print("hist deal select fail ",GetLastError()); }
Ups, my bad, we have to select it first using HistoryDealSelect.
I check around, apparently we have to wait until MT5 is updated with trading history, in my case I wait for a sec. Maybe I have lousy connection :(
So the code will be like this.
Still no luck :(( At least in the strategy tester! Could that be the problem ?
Here is the code now. I added "long" before Ticket right ?
Thanks for your help!!
double OutCome = 0.0; long Ticket = mresult.deal; Sleep(1000); // <<============================ wait ! ResetLastError(); if (HistoryDealSelect(Ticket)) { OutCome = HistoryDealGetDouble(Ticket,DEAL_PROFIT); } else { Print("hist deal select fail ",GetLastError()); } string Text = "BBWP Closed a Short position with P&L : "+OutCome; Print(TimeCurrent()," Print 3 Outcome ",OutCome," Ticket ",Ticket); SendNotification(Text);
Outcome in strategy tester:
Still no luck :(( At least in the strategy tester! Could that be the problem ?
Here is the code now. I added "long" before Ticket right ?
Thanks for your help!!
Outcome in strategy tester:
Yes, have to add "long" before "Ticket". Sorry for that ;(.
Well, it does not works on my strategy tester too, and I don't know the reason why :(, but it works on MetaQuotes demo.
Yes, have to add "long" before "Ticket". Sorry for that ;(.
Well, it does not works on my strategy tester too, and I don't know the reason why :(, but it works on MetaQuotes demo.
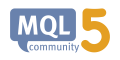
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi All,
I'm sorry if the question is basic but I'm new to coding :)
I have created the below closing script and added the SendNotification line but I would like to add the P&L of the deal to the message. How could I do that ?
Thanks _