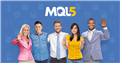
- www.mql5.com
So what is the error or what is the thing it should do that it doesn't?
Just to reinforce, I'm a total beginner in programming in general, after a lot of study I can understand what each thing in the code is doing, but at first I'm lost.
Let's go to the errors (I regenerated chatgpb's response several times and finally got a code that showed the least amount of errors):
Stop using ChatGPT.
Help needed to debug and fix an AI EA - Trading Systems - MQL5 programming forum #2 (2023)
ChatGPT (the worst), “Bots Builder”, “EA builder”, “EA Builder Pro”, EATree, “Etasoft forex generator”, “Forex Strategy Builder”, ForexEAdvisor (aka. ForexEAdvisor STRATEGY BUILDER, and Online Forex Expert Advisor Generator), ForexRobotAcademy.com, forexsb, “FX EA Builder”, fxDreema, Forex Generator, FxPro, Molanis, Octa-FX Meta Editor, Strategy Builder FX, Strategy Quant, “Visual Trader Studio”, “MQL5 Wizard”, etc., are all the same. You will get something quick, but then you will spend a much longer time trying to get it right, than if you learned the language up front, and then just wrote it.
Since you haven't learned MQL4/5, therefor there is no common language for us to communicate. If we tell you what you need, you can't code it. If we give you the code, you don't know how to integrate it into yours.
We are willing to HELP you when you post your attempt (using Code button) and state the nature of your problem, but we are not going to debug your hundreds of lines of code. You are essentially going to be on your own.
ChatGPT |
|
bot builder | Creating two OnInit() functions.* |
EA builder | |
EATree | Uses objects on chart to save values — not persistent storage (files or GV+Flush.) No recovery (crash/power failure.) |
ForexEAdvisor |
|
FX EA Builder |
|
Stop using ChatGPT.
Help needed to debug and fix an AI EA - Trading Systems - MQL5 programming forum #2 (2023)
We are willing to HELP you when you post your attempt (using Code button) and state the nature of your problem, but we are not going to debug your hundreds of lines of code. You are essentially going to be on your own.
Sure william! I'm going to start learning mql5, would you know how to tell me where I can start? I'm a beginner in terms of programming, I know the basics, if, else .....

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I'm trying to create an EA code that does the following task:
--The robot started and randomly selected that it would open a buy order, the market followed and it hit the take. Then he opened another buy order and the market came back and hit the stop. again he opens another buy order and the market comes back and hits the stop. again he opens another buy order and the market comes back and hits the stop. In the next order, as they completed 3 stops, he changes the order and opens a sell order.
Guys, this is an idea I had, it's nothing amazing and maybe some even think it's "idiotic". But it's an idea that I wanted to put into practice to see what happens.I asked chatGPT to create it for me, as I know absolutely nothing about mql5 just the platform itself. However, as expected, chatgpt does not make a perfect code and presents several problems. If anyone can help me solve this code and get it running, I can even buy you a beer :D