- Type conversion warning, I can't spot it
- I can't figure out why I can't compile this script.
- Possible loss of data due to type conversion.
-
Why did you post your MT4 question in the MT5 Indicators section instead of the MQL4 section, (bottom of the Root page)?
General rules and best pratices of the Forum. - General - MQL5 programming forum? (2017)
Next time, post in the correct place. The moderators will likely move this thread there soon. -
TL_OpenTime = ObjectGet(sparam,OBJPROP_TIME1);
You have aDatetime = aDouble Perhaps you should cast to the correct data type.
-
Why did you post your MT4 question in the MT5 Indicators section instead of the MQL4 section, (bottom of the Root page)?
General rules and best pratices of the Forum. - General - MQL5 programming forum? (2017)
Next time, post in the correct place. The moderators will likely move this thread there soon. - You have aDatetime = aDouble Perhaps you should cast to the correct data type.
First Let me apologize for posting in the wrong place. By all means place it in the correct form.
Now I realize that there is a data type mismatch. But, I can't seem to work it out. For instance: In the link you posted, there is an ObjectGet example for getting color. I used this very example and I get a warning.
// LINKED EXAMPLE color oldColor=ObjectGet("hline12", OBJPROP_COLOR); // MY CODE color TL_Color, HL_Color; TL_Color = ObjectGet(sparam,OBJPROP_COLOR); // Yields possible loss warning???
TL_Color = ObjectGet(sparam,OBJPROP_COLOR); // Yields possible loss warning???What part of “Perhaps you should cast to the correct data type” was unclear?
Read the following documentation on Typecasting.
ObjectGet returns a "double" data-type. You will need to typecast it into a "datetime" or a "color", or whatever other data-type is expected to be returned depending on your needs.
TL_OpenTime = (datetime) ObjectGet(sparam,OBJPROP_TIME1); TL_Color = (color) ObjectGet(sparam,OBJPROP_COLOR);
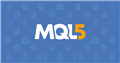
- www.mql5.com
Read the following documentation on Typecasting.
ObjectGet returns a "double" data-type. You will need to typecast it into a "datetime" or a "color", or whatever other data-type is expected to be returned depending on your needs.
Thank you soooooo much! I hated the warnings when compiling. When William told me I needed to cast the data, I found Typecasting. However, I couldn't figure out what to do. I have made the changes and it now works great with no warnings. Thanks again. ---Tom
Read the following documentation on Typecasting.
ObjectGet returns a "double" data-type. You will need to typecast it into a "datetime" or a "color", or whatever other data-type is expected to be returned depending on your needs.
I reread the Typecasting (key being "Type"). I get it now. Thanks for your guidance. Kind Regards ----Tom

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use