if (FileIsExist(myFile.csv)) {
Do not post code that will not even compile.
Hi Guys.
Same issue, hence not creating a new thread - but also not the same issue...
Code below gets called from OnInit()
bool FileFunc() { ResetLastError(); if(!FileIsExist("fileName.csv")) { Print("File does not exist. Opening a new file."); int H_File = FileOpen("fileName.csv", FILE_CSV|FILE_UNICODE|FILE_WRITE, ","); if(H_File == INVALID_HANDLE) { Print("Error opening 'fileName.csv' - Error: ", _LastError); return false; } Print("File Opened."); FileClose(H_File); Print("File Closed."); } else { if(!FileDelete("fileName.csv")) { Print("File Delete Failure - Error: ", _LastError); return false; } else Print("File Deleted"); } return true; }
Here's the funny thing. When attaching the EA to a chart, it works as expected.
2023.11.29 13:12:04.274 File does not exist. Opening a new file.
2023.11.29 13:12:04.275 File Opened.
2023.11.29 13:12:04.275 File Closed.
2023.11.29 13:14:19.907 File Deleted.
When running it in tester, it keeps on executing the 'true' section of FileIsExist over and over again.
Therefore, I have the same question: What am I missing?
Am I indeed missing something?
Am I overlooking my own error?
Am I... Am I... Am I...
A pair of fresh eyes will be highly appreciated.
LMC
FileFunc
How many times do you call FileFunc() ?
Looking at the output you shared, the deletion seems to be happening 2 mins later than the creation.
2023.11.29 13:12:04.275 File Closed.
2023.11.29 13:14:19.907 File Deleted.
Thus it is most likely is a another call, made after the file is created in the 1st call.
So in fact the "else" section of the code should indeed be executed because on the subsequent call, the file does exist.
You should set a break point and step through the code to ascertain whether that is what is happening
How many times do you call FileFunc() ?
Looking at the output you shared, the deletion seems to be happening 2 mins later than the creation.
2023.11.29 13:12:04.275 File Closed.
2023.11.29 13:14:19.907 File Deleted.
Thus it is most likely is a another call, made after the file is created in the 1st call.
So in fact the "else" section of the code should indeed be executed because on the subsequent call, the file does exist.
You should set a break point and step through the code to ascertain whether that is what is happening
Sure - can't understand it either.
You may find changing your logic could overcome this - for example use FileOpen() first (which is common whether you create or open an existing file), and then use FileSize() to determine whether the file is empty or not.
From memory the FileSize() of a new file is 2 bytes, so you could use <= 2 as a determining factor (but please do check)
Sure - can't understand it either.
You may find changing your logic could overcome this - for example use FileOpen() first (which is common whether you create or open an existing file), and then use FileSize() to determine whether the file is empty or not.
From memory the FileSize() of a new file is 2 bytes, so you could use <= 2 as a determining factor (but please do check)
The complete EA:
int OnInit() { ResetLastError(); if(FileIsExist("TestFile.CSV")) { Print("FileIsExists() == true"); Print("Last Error: ", _LastError); } else { Print("FileIsExists() == false"); Print("Last Error: ", _LastError); } return INIT_SUCCEEDED; } void OnDeinit(const int reason) { } void OnTick() { }
Stepped trough the code, and as previously, I got the following result:
No wonder FileIsExist() are processing false repeatedly 'cause there is no bloody file!
The complete EA:
Stepped trough the code, and as previously, I got the following result:
No wonder FileIsExist() are processing false repeatedly 'cause there is no bloody file!
Sneaky workarounds are my favourite :)
Anyway - I have noticed there can be a delay in Windows updating, even outside MQL - hitting F5 is necessary.
I do not recall hitting this exact problem in MQL5 but some time back I included the FileFlush() command in my code to force a write to disk, to minimize the chance. Why not give it a try?
https://www.mql5.com/en/docs/files/fileflush
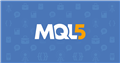
- www.mql5.com
Sneaky workarounds are my favourite :)
Anyway - I have noticed there can be a delay in Windows updating, even outside MQL - hitting F5 is necessary.
I do not recall hitting this exact problem in MQL5 but some time back I included the FileFlush() command in my code to force a write to disk, to minimize the chance. Why not give it a try?
https://www.mql5.com/en/docs/files/fileflush
I am aware of what you are referring to w.r.t. refresh, but as I mentioned in my previous post, I created the file (outside of the IDE); opened and closed it after creation; closed file explorer and re-opened it; went back to the relevant folder to check that the file is still there.
Which it was. So, the file was committed to disk.
FileFlush() would only be relevant of the file was created by FileOpen(). Never the less, I changed the code:
int OnInit() { ResetLastError(); if(FileIsExist("TestFile.CSV")) { if(!FileDelete("TestFile.CSV")) Print("File Delete Error - ", _LastError); } else { int H_File = FileOpen("TestFile.CSV", FILE_CSV|FILE_WRITE|FILE_UNICODE, ","); if(H_File == INVALID_HANDLE) { Print("File Open Error - ", _LastError); return false; } FileFlush(H_File); FileClose(H_File); } return INIT_SUCCEEDED; } void OnDeinit(const int reason) { } void OnTick() { }
First execution, without the file existing in ...\Files, FileIsExist() acts as expected and process the false block.
Check - the file was created successfully.
Second execution with the file existing in ...\Files, FileIsExist() process the false block again.
<sigh>
LMC
I am aware of what you are referring to w.r.t. refresh, but as I mentioned in my previous post, I created the file (outside of the IDE); opened and closed it after creation; closed file explorer and re-opened it; went back to the relevant folder to check that the file is still there.
Which it was. So, the file was committed to disk.
FileFlush() would only be relevant of the file was created by FileOpen(). Never the less, I changed the code:
First execution, without the file existing in ...\Files, FileIsExist() acts as expected and process the false block.
Check - the file was created successfully.
Second execution with the file existing in ...\Files, FileIsExist() process the false block again.
<sigh>
LMC
Yes - some functions may not be 100% reliable (in another thread there was a discussion of conversion functions which seem to be lacking, prompting many to write their own).
I suspect you need to consider FileIsExist() has such quirks and thus the workaround of reading the size could be a more reliable approach
Hi Guys.
Same issue, hence not creating a new thread - but also not the same issue...
Code below gets called from OnInit()
Here's the funny thing. When attaching the EA to a chart, it works as expected.
2023.11.29 13:12:04.274 File does not exist. Opening a new file.
2023.11.29 13:12:04.275 File Opened.
2023.11.29 13:12:04.275 File Closed.
2023.11.29 13:14:19.907 File Deleted.
When running it in tester, it keeps on executing the 'true' section of FileIsExist over and over again.
Therefore, I have the same question: What am I missing?
Am I indeed missing something?
Am I overlooking my own error?
Am I... Am I... Am I...
A pair of fresh eyes will be highly appreciated.
LMC
This is normal behaviour using MQL5/Files with the Strategy Tester. The files there are deleted on each run, so your file never exists. I suppose it's written somewhere in the chaotic documentation.
If you want your file(s) to remain between different tester runs, then use the FILE_COMMON flag to use the common folder.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I am trying to use FileIsExist to search the common directory of MQL, which is, C:\Users\Jim\AppData\Roaming\MetaQuotes\Terminal\9B101088254A9C260A9790D5079A7B11\MQL5\Files.
In the code below, it always defaults to the "else" statement even if the file does not yet exist.
if the file does not exist yet, i want to create it and add a header, then do "something1"
If it already exists, then do not add a header,just do "something2".
appreciate if can point me to the right direction pls.