Hi Alexandre
I have been using iCustom upto now. However I liked the idea of creating Class(s) and move my calculation into subClasses. Creating indicators always gives me hard time more specially where the averaging is required. Indicators which I download from Market, all uses different ways of calculating averages, that makes my work more complicated, as I found that most of the time I cann't duplicate the same method for another indicator.
All this makes me to think of having Standard Calculations that can be repeated for other indicators too. I found that <MovingAverages.mqh> methods are much simpler to use.
My confusion goes mainly in the loop to fetch values and return calculated values.
Hope this clarifies your question, and also it is just a personal preference too.
In my attempt:
1) able to create CiVolume.mq5 indicator which returns the correct values. https://www.mql5.com/en/articles/10
2) able to create Indicator_cBase.mqh, Indicator_cVolume.mqh and cVolume.mq5. However with this approach I am not getting the correct values. (Tutorials from https://orchardforex.com/)
The code files are attached here, and seeking masters guidance to complete my indicator calculations in the Class
actually, if you take someone's code -- your questions are to be addressed personally to the author... I doubt, that somebody will spend time to look the tutorial video instead of you in order to refactor or check all your project... but knowing this tutorial resource I doubt that the author haven't already explained everything!...
(it's better return to the source! & if questions still remains - try to shorten the questionable point of the whole code - e.g. with a shorter compilable example of the problem reflection - and try to ask the author)
However with this approach I am not getting the correct values.
Maybe I am misreading something but I didn't get an idea about what is the issue here.
Hi Lippmaje
if you look at jpeg file it has same indicator displayed twice.
the first one with green bars uses CiVolume.mq5 file and works correctly without any problem.
I have tried to use the same code logic in a Indicator_cBase.mqh class file and tried to create indicator from the retuned value of the class. This one is second indicator on chart with Silver Bars.
However I am getting different values in indicator from the Class. To my understanding
void CIndicator_cVolume::UpdateValues(int pBars,int pLimit)
seems to be the reason for this, as I am not sure if my for..loop is correct to fetch bars. If someone can help me on that, it will be great support to me.
Thanks
actually, if you take someone's code -- your questions are to be addressed personally to the author... I doubt, that somebody will spend time to look the tutorial video instead of you in order to refactor or check all your project... but knowing this tutorial resource I doubt that the author haven't already explained everything!...
(it's better return to the source! & if questions still remains - try to shorten the questionable point of the whole code - e.g. with a shorter compilable example of the problem reflection - and try to ask the author)
Hi JeeCi
The problem is most of them dont reply. So you are left with no option but to struggle and find yourself the solution 'or' just give up and leave it.
Thanks for your response.
Ok I didn't go through all the code but here's my issues:
- Update() is being called only once during initialisation.
- mBuffer has series flag set, while mTemp has not.
I am not sure if my for..loop is correct to fetch bars.
if you mean this part of your code
if(mPrevCalculated == 0) start = 1; // start filling out mTemp[] from the 1st index else start = mPrevCalculated-1; // set start equal to the last index in the arrays for(int i = start; i < pBars && !IsStopped(); i++) { mTemp[i] = (double)Volume(i);
perhaps try to see this
- I do not see the use of pLimit in your member-method & the declaration of pBars & its source... (at a glance)...
but I still prefer shorter compilable example of the problem or at least attached source files - instead of such listings... of course shorter representation of the problem is better (it might help you even without us))...
p.s. though I use mq4 (therefore not promising to help you)
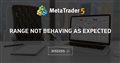
BTW all .mqh-files are header files & should be put in Include directory to be automatically found by .mq4(5)-src:
#include <Indicator_cVolume.mqh>
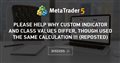
- 2021.05.12
- www.mql5.com
BTW all .mqh-files are header files & should be put in Include directory to be automatically found by .mq4(5)-src:
#include <Indicator_cVolume.mqh>
Hi JeeyCi
thanks for your response. Yes I am aware of this path requirements for automatically locating the files.
However, I am trying to keep my files into single Folder/SubFolder under MQL\Expert. This way it is easy for me to copy all related files in one go. I don't mind to specify path and locations which I take care in my include files.
There is yet another simpler solution was given by Orchard Forex, to create ABCXYZ.mqh file where you define all the include statements and just include this file into to your main EA to pull in all files.
Regards.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Wishing everyone a great weekend.
I am working on an EA with OOP and trying to create indicators for the same.
My object is to standardize calculations of my indicators (in CLASSes) and prefer to use averaging methods from <MovingAverages.mqh> file.
In my attempt:
1) able to create CiVolume.mq5 indicator which returns the correct values. https://www.mql5.com/en/articles/10
2) able to create Indicator_cBase.mqh, Indicator_cVolume.mqh and cVolume.mq5. However with this approach I am not getting the correct values. (Tutorials from https://orchardforex.com/)
The code files are attached here, and seeking masters guidance to complete my indicator calculations in the Class, instead of using iCustom.
Thanks in advance.
CiVolume.mq5 ... The code for working indicator
Indicator Class Code Files
Screen shot of Indicators on the Chart