Use class instead of struct to gain the benefit of object pointers.
// Include additional header #include <Generic/HashMap.mqh> // User defined struct class MyStruct { public: string symbol; double volume; double rate; }; CHashMap <string, MyStruct *> SymbolMap; //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ void OnStart() { // set MyStruct *st = new MyStruct(); if(SymbolMap.TrySetValue("MyKey",st)) { st.symbol = "EURUSD"; st.volume = 0.1; st.rate = 1.12345; } // get MyStruct *st_out = NULL; if(SymbolMap.TryGetValue("MyKey",st_out)) { Print(st_out.symbol); Print(st_out.volume); Print(st_out.rate); } } //+------------------------------------------------------------------+Edit: you can check this script for more complete usage. https://www.mql5.com/en/code/25667
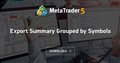
- www.mql5.com
Use class instead of struct to gain the benefit of object pointers.
Edit: you can check this script for more complete usage. https://www.mql5.com/en/code/25667Hi amrali,
Many thanks for your suggestion.
I have already implemented according to your way.
Class is better than Struct in this case.
Thanks everyone for your valuable comments.
While smart (owning) pointers help with proper memory management, raw (non-owning) pointers are still useful for the organizational purposes in data structures (like CHashMap). A smart pointer is automatically deleted when it goes out of scope. But for the HashMap we need to control the lifetime of our pointers, so I see raw pointers are better here.
Edit:
(An alternative solution is a smart container, like CArrayObj. It is auto-cleaning container of pointers. The container's destructor ~CArrayObj() automatically handles the memory cleanup).
However, insertions, deletions and search in CArrayObj are slower than in CHashMap.
My other script for MT4 https://www.mql5.com/en/code/25710 uses CArrayObj (smart container) as MQL4 does not have CHashMap.
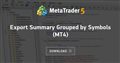
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I would like to use CHashMap to store key/value pair where key will be string and value will be a struct variable.
Trying with below code, but its not compiling.
And below is the error.
How to fix the compile error?
Thanks.