I'd suggest to use CopyTo to copy the map content into an array. See CopyTo for IMap. https://www.mql5.com/en/docs/standardlibrary/generic/imap
Once you got the keys in your array you just loop over it.
int count=map.CopyTo(keys,values);
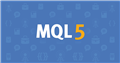
Documentation on MQL5: Standard Library / Generic Data Collections / IMap
- www.mql5.com
Standard Library / Generic Data Collections / IMap - Reference on algorithmic/automated trading language for MetaTrader 5
Another possibility is to use an array of objects instead of CHashMap. (per @nicholi shen)
Anthony Garot:
Another possibility is to use an array of objects instead of CHashMap. (per @nicholi shen)
Definitely this. I have found very little use for dictionaries in MQL, however, if you are using CHashMap and need iterate the items then use the CopyTo method.
CHashMap<string, int> d; d.Add("one", 1); d.Add("two", 2); d.Add("three", 3); string keys[]; int values[]; d.CopyTo(keys, values); for (int i=0; i<d.Count(); i++) Print(keys[i], " ", values[i]);

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
I have populated HashMap with keys and values.
Now I need to iterate over all pair (key, values).
How to do this?
CHashMap don't have a method CHashMap.items().
Thanks.