You have no control over the result of a trade request. You have no control over whether a position was opened or not. Therefore, you will often open several positions in a row.
The "&&PC1 == 0" condition isn't enough? because as soon as the position is open PC1 will no longer equal 0
This is not enough. As I say, you do not control the execution of the position at all.
You have sent a trade order - until the trade order is executed, you can enter Ontick several times and issue several more orders. You need to work according to the algorithm:
- issue a trade order
- stop issuing trade orders until you receive confirmation in OnTradeTransaction
This is not enough. As I say, you do not control the execution of the position at all.
You have sent a trade order - until the trade order is executed, you can enter Ontick several times and issue several more orders. You need to work according to the algorithm:
- issue a trade order
- stop issuing trade orders until you receive confirmation in OnTradeTransaction
- Marquese Singfield: As I am not very familiar with OnTradeTransaction. Could you please tell me How would I check for confirmation?
Perhaps you should read the manual. MQL5 Reference → MQL5 programs → Program Running → OnTrade
How To Ask Questions The Smart Way. 2004
How To Interpret Answers.
RTFM and STFW: How To Tell You've Seriously Screwed Up. - Marquese Singfield: I never considered this type of handling!
You are looking at a signal. Act on a change of signal.
MQL4 (in Strategy Tester) - double testing of entry conditions - MQL5 programming forum #1 2017.12.12
-
Perhaps you should read the manual. MQL5 Reference → MQL5 programs → Program Running → OnTrade
How To Ask Questions The Smart Way. 2004
How To Interpret Answers.
RTFM and STFW: How To Tell You've Seriously Screwed Up. -
You are looking at a signal. Act on a change of signal.
MQL4 (in Strategy Tester) - double testing of entry conditions - MQL5 programming forum #1 2017.12.12
This is not enough. As I say, you do not control the execution of the position at all.
You have sent a trade order - until the trade order is executed, you can enter Ontick several times and issue several more orders. You need to work according to the algorithm:
- issue a trade order
- stop issuing trade orders until you receive confirmation in OnTradeTransaction
I realized recently that this actually doesn't relate to the goal I am trying to achieve. "stop issuing trade order until....." The goal is that there will be no more trade orders after the first. the issue is not that I want to wait for confirmation of execution of the order. I do not want to place more than one order......period...confirmation or not I only want to send one request and never another for the same pair. Even though I am entering OnTick during the order being sent, I should not place another request ever again after the first is sent. And unfortunately after hours of reviewing how OnTradeTransaction is working it is only analyzing the process of trade transactions. I can not use this to stop transactions from taking place all together. Unless I am missing something?
Can I somehow implement a waiting period between the first request till confirmation and then call my positionCounter function after this?
I realized recently that this actually doesn't relate to the goal I am trying to achieve. "stop issuing trade order until ....." The goal is that there will be no more trade orders after the first. the issue is not that I want to wait for confirmation of execution of the order. I do not want to place more than one order......period...confirmation or not I only want to send one request and never another for the same pair. Even though I am entering OnTick during the order being sent, I should not place another request ever again after the first is sent. And unfortunately after hours of reviewing how OnTradeTransaction is working it is only analyzing the process of trade transactions. I can not use this to stop transactions from taking place all together. Unless I am missing something?
Can I somehow implement a waiting period between the first request till confirmation and then call my positionCounter function after this?
Example of a blank:
We catch the transaction
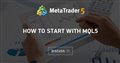
- 2020.09.17
- www.mql5.com
This is not enough. As I say, you do not control the execution of the position at all.
You have sent a trade order - until the trade order is executed, you can enter Ontick several times and issue several more orders. You need to work according to the algorithm:
- issue a trade order
- stop issuing trade orders until you receive confirmation in OnTradeTransaction
int PC1 = 0; void OnTick() { double Lots = 0.01; double Ask1 = NormalizeDouble(SymbolInfoDouble("AUDCAD",SYMBOL_ASK),2); //Grab Ask price from chart double Bid1 = NormalizeDouble(SymbolInfoDouble("AUDCAD",SYMBOL_BID),2); // Grab Bid price string ask1 = DoubleToString(Ask1,2); //convert the double to string string bid1 = DoubleToString(Bid1,2); //convert the double to string int FileHandle = FileOpen("results.txt",FILE_READ|FILE_SHARE_READ|FILE_TXT|FILE_ANSI,"|",CP_ACP); //open file string TextString = FileReadString(FileHandle); //read file string int StringSearchBUY = StringFind(TextString,"BUY"); // search the string from file for the string word "BUY" int StringSearchSELL = StringFind(TextString,"SELL"); // search the string from file for the string word "SELL" int SSBP1 = StringFind(TextString,bid1); // search the string from file for the bid int SSAP1 = StringFind(TextString,ask1); // search the string from file for the ask FileClose(FileHandle); //close the file if(StringSearchBUY >= 0 && (SSBP1 >=0 || SSAP1 >=0) && PC1 == 0) //if the buy string is found and the ask/bid price is found in file string then open buy position if no positions are open { trade.Buy(NormalizeDouble(Lots,2),"AUDCAD",Ask1,NULL,NULL); PC1 = 1; } if(StringSearchSELL >= 0 && (SSBP1 >=0 || SSAP1 >=0) && PC1 == 0) //if the sell string is found and the ask/bid price is found in file string open sell position if no positions are open { trade.Sell(NormalizeDouble(Lots,2),"AUDCAD",Bid1,NULL,NULL); PC1 = 1; } }
But still multiple positions are being opened when the conditions are met. This makes no sense to me if the global variable is set to 1 automatically after the first pass in OnTick() [execution from top to bottom of code in OnTick()]. Any thoughts @William Roeder?

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I created a function that will count the number of positions for any symbol you give it.
My only problem is when I attempt to call this function and store the integer value it returns to a variable and then use this varible to check if multiple positions are open for this pair it doesnt work. Would appreciate any insight:
There are no problems with opening and reading the file but I keep running into the problem of opening multiple positions when the conditions are met.