I am working on this problem for several weeks now and I just can't find a proper way to solve it. I am looking for help.
I want the following to happen:
1) Place 2 orders, n and n+1. n has a TP & SL level, n+1 has only SL level. SL levels are the same for both orders.
2) If SL is hit, fine. Both orders are terminated.
3) If TP on order n is hit, modify order n+1 to have its SL moved to the price where order n hit TP.
4) After that I want to execute trailing stop loss and I have already programmed that successfully.
How is this done? I just can't find a way with CTrade, CHistoryOrderInfo or CDealInfo to work this out. I tried with magic numbers, iterating over HistoryDealsTotal, HistoryOrdersTotal and a few more approaches but I just never get to the point where I am able to track an order inbetween many open orders and check if it just hit TP, then modify the other order that was initially created right after the first order.
I would appreciate any help towards a solution, thanks!
You could try saving the tickets of 'pair' positions, when a position closes, then check the outcome immediately using OnTradeTransaction and make neccessary changes to it's corresponding partner.
If you have two, and only two positions, you don't have to save nothing. Just detect the close as above and make changes to the remaining position.
Thank you for the hint. I did more research yesterday - funnily there is a very similar topic right now in this forum. And I discovered the OnTradeTransaction event handler. I never found it before. I think this is the way to go. It's just the documentation of MQL5 is so all over the place and sometimes just outdated or really hard to find. Thank you for your hint!
Hellos am Martyn by name I want to know how to trigger profit on mql5
Example: run an Expert Advisor ('SL TP Triggered.mq5') on a chart.
//+------------------------------------------------------------------+ //| SL TP Triggered.mq5 | //| Copyright 2020, MetaQuotes Software Corp. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2020, MetaQuotes Software Corp." #property link "https://www.mql5.com" #property version "1.00" /* barabashkakvn Trading engine 3.137 */ #include <Trade\DealInfo.mqh> //--- CDealInfo m_deal; // object of CDealInfo class //--- input parameters //--- //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- } //+------------------------------------------------------------------+ //| TradeTransaction function | //+------------------------------------------------------------------+ void OnTradeTransaction(const MqlTradeTransaction& trans, const MqlTradeRequest& request, const MqlTradeResult& result) { //--- get transaction type as enumeration value ENUM_TRADE_TRANSACTION_TYPE type=trans.type; //--- if transaction is result of addition of the transaction in history if(type==TRADE_TRANSACTION_DEAL_ADD) { if(HistoryDealSelect(trans.deal)) m_deal.Ticket(trans.deal); else { Print(__FILE__," ",__FUNCTION__,", ERROR: HistoryDealSelect(",trans.deal,")"); return; } //--- long reason=-1; if(!m_deal.InfoInteger(DEAL_REASON,reason)) { Print(__FILE__," ",__FUNCTION__,", ERROR: InfoInteger(DEAL_REASON,reason)"); return; } if((ENUM_DEAL_REASON)reason==DEAL_REASON_SL) Alert("Stop Loss activation"); else if((ENUM_DEAL_REASON)reason==DEAL_REASON_TP) Alert("Take Profit activation"); } } //+------------------------------------------------------------------+
Open a position and set Stop Loss and Take Profit for it.
Thanks very much for sharing this @ Vladimir Karputov
Extremely helpful to those getting started. In my case, I am getting annoyed how there are no noises when a TP or SL is hit, so you made exactly what I was looking for, thanks again for sharing, much appreciated.
If the TP was just hit, that is the current market. You want to move the SL to the market.
-
You can't move stops (or pending prices) closer to the market than the minimum: MODE_STOPLEVEL * _Point or SymbolInfoInteger(SYMBOL_TRADE_STOPS_LEVEL).
Requirements and Limitations in Making Trades - Appendixes - MQL4 TutorialOn some ECN type brokers the value might be zero (the broker doesn't know). Use a minimum of two (2) PIPs.
- You second order will likely be closed almost immediately.
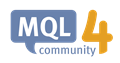
- book.mql4.com
Example: run an Expert Advisor ('SL TP Triggered.mq5') on a chart.
Open a position and set Stop Loss and Take Profit for it.
Example: run an Expert Advisor ('SL TP Triggered.mq5') on a chart.
Open a position and set Stop Loss and Take Profit for it.
Example: run an Expert Advisor ('SL TP Triggered.mq5') on a chart.
Open a position and set Stop Loss and Take Profit for it.
Is there a way to add the order type to the message of the tp activated and sl activated?
It would be great if you guys can provid an example of how to do it :)
Greetings,

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I am working on this problem for several weeks now and I just can't find a proper way to solve it. I am looking for help.
I want the following to happen:
1) Place 2 orders, n and n+1. n has a TP & SL level, n+1 has only SL level. SL levels are the same for both orders.
2) If SL is hit, fine. Both orders are terminated.
3) If TP on order n is hit, modify order n+1 to have its SL moved to the price where order n hit TP.
4) After that I want to execute trailing stop loss and I have already programmed that successfully.
How is this done? I just can't find a way with CTrade, CHistoryOrderInfo or CDealInfo to work this out. I tried with magic numbers, iterating over HistoryDealsTotal, HistoryOrdersTotal and a few more approaches but I just never get to the point where I am able to track an order inbetween many open orders and check if it just hit TP, then modify the other order that was initially created right after the first order.
I would appreciate any help towards a solution, thanks!