... Can someone say please why it doesn't work? Sometimes it uses up to 3 times the risk as the input. The idea here is to use this file to open trades with the same risk each time (Expert\Money\MoneyFixedRisk.mqh). In this case the Stop Loss is based in ATR...
It looks like you calculate the stop loss wrong. In this line:
double StopLossPips=Factor*ATRArray[1];
you multiply the ATR value by Factor (1 by default) but not by digits_adjust, so that for a 5 digit symbol your stop loss is in points but not in pips.
CheckOpenLong/Short will then return a volume that is too high for the true stop loss.
It looks like you calculate the stop loss wrong. In this line:
you multiply the ATR value by Factor (1 by default) but not by digits_adjust, so that for a 5 digit symbol your stop loss is in points but not in pips.
CheckOpenLong/Short will then return a volume that is too high for the true stop loss.
That work for the Risk Calculation, thanks for the help.
I changed that line to:
double StopLossPips=Factor*ATRArray[1]*_Digits;
However I found another problem. Seems the calculation for the Stop Loss is not correct. The problem is the StopLoss is way higher of what is supposed to be.
For example in the case of the factor being "1", meaning the StopLoss should be only "1 time the ATR value of the period", actually is more than that. I noticed because is way higher, even 6 times the correct value on some trades.
Someone know why this happens? I cannot see the problem.
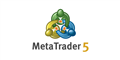
- www.metatrader5.com
That work for the Risk Calculation, thanks for the help.
I changed that line to: ...
However I found another problem. Seems the calculation for the Stop Loss is not correct. The problem is the StopLoss is way higher of what is supposed to be.
This cannot work:
double StopLossPips=Factor*ATRArray[1]*_Digits;
What's the value of ATRArray[1], show us some examples.
This cannot work:
What's the value of ATRArray[1], show us some examples.
You are right. I need to use digits_adjust in order to get it right.
However, I found some problems in some stocks. It doesn't make much sense, I don't think is the digits problem because some stocks of 2 or 4 digits have problems while other don't.
Do you know if there is another step I need to take to correct this.
The problem now seems to be on some stocks, It works correctly on FX and futures.
You need to show more data for the cases where it seems to fail. You've already put some Print statements in, show this output. Looks like either your custom ATR is wrong, or the stop loss. Also check the balance that you set in Tester.
I'm using now the ATR by default in MT5.
One Stock CFD have the problem of [invalid stoploss] and for example send a market order to buy at 122.50 but the stoploss was at 50 and take profit at 195. Clearly the ATR is not that high.
I'm using now the ATR by default in MT5.
One Stock CFD have the problem of [invalid stoploss] and for example send a market order to buy at 122.50 but the stoploss was at 50 and take profit at 195. Clearly the ATR is not that high.
Then leave it as it was before:
double StopLossPips=Factor*ATRArray[1];
And check with the standard iATR if it works now. I assume your custom ATR computes values in pips, iATR does it in point.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello,
Based on another post of a person using the Money Fixed Risk in MQL5 to create a random expert advisor I write the following.
Can someone say please why it doesn't work? Sometimes it uses up to 3 times the risk as the input. The idea here is to use this file to open trades with the same risk each time (Expert\Money\MoneyFixedRisk.mqh). In this case the Stop Loss is based in ATR. I need to use it to test in Cryptos, FX, Stocks, and Futures. All CFD asset classes a broker can have.