
You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Custom time input at MQL4
Is it possible to write a code with MQL4 that can draw a horizontal line starting from a defined bar by the user until the end of the chart
Here is the same code with Metastock
Dy:=Input("Day",1,31,1);
Mn:=Input("Month",1,12,1);
Yr:=Input("Year",2000,2012,2010);
{Time Calculation}
Time:=Dy=DayOfMonth() AND Mn=Month() AND Yr=Year();
{Formula}
Start:= ValueWhen(1,Time,CLOSE);
{Output}
Start;And here is the result
I hope to do the same thing with MQL4
Try like this :
extern string startFrom = "2012.07.06 00:00";
int init() { return(0); }
int deinit() { ObjectDelete("hLine"); return(0); }
int start()
{
string name = "hLine";
int barShift = iBarShift(NULL,0,StrToTime(startFrom));
ObjectCreate(name,OBJ_TREND,0,0,0,0,0);
ObjectSet(name,OBJPROP_PRICE1,Close);
ObjectSet(name,OBJPROP_PRICE2,Close);
ObjectSet(name,OBJPROP_TIME1,Time);
ObjectSet(name,OBJPROP_TIME2,Time[0]);
return (0);
}Just copy and paste it to some indicator (it is an already working indicator) and attach it to chart.
Is it possible to write a code with MQL4 that can draw a horizontal line starting from a defined bar by the user until the end of the chart
Here is the same code with Metastock
Dy:=Input("Day",1,31,1);
Mn:=Input("Month",1,12,1);
Yr:=Input("Year",2000,2012,2010);
{Time Calculation}
Time:=Dy=DayOfMonth() AND Mn=Month() AND Yr=Year();
{Formula}
Start:= ValueWhen(1,Time,CLOSE);
{Output}
Start;And here is the result
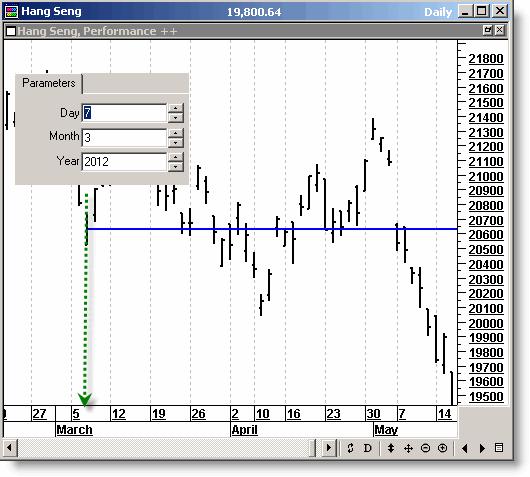
I hope to do the same thing with MQL4Try like this :
extern string startFrom = "2012.07.06 00:00";
int init() { return(0); }
int deinit() { ObjectDelete("hLine"); return(0); }
int start()
{
string name = "hLine";
int barShift = iBarShift(NULL,0,StrToTime(startFrom));
ObjectCreate(name,OBJ_TREND,0,0,0,0,0);
ObjectSet(name,OBJPROP_PRICE1,Close);
ObjectSet(name,OBJPROP_PRICE2,Close);
ObjectSet(name,OBJPROP_TIME1,Time);
ObjectSet(name,OBJPROP_TIME2,Time[0]);
return (0);
}You are the best thanks a lot mladen
Hi Mladen,
Can you tell me more about the rationale of the functions here? I still don't quite get it. Thanks
Terrance
Try this function :
{
int openedOrders = 0;
for(int i=0; i < OrdersTotal(); i++)
{
if(OrderSelect(i, SELECT_BY_POS, MODE_TRADES) == false) break;
if(OrderSymbol() != Symbol()) continue;
if(OrderMagicNumber() != MagicNumber) continue;
if(OrderType() == type) openedOrders++;
}
return(openedOrders);
}
[/PHP]
To count opened buy orders, call it like this :
to count opened sell orders, call it like this :
[PHP]int openedSells = countOpened(OP_SELL);tkuan77
You wanted to limit orders to 1 buy and 1 sell at a time.
So if you call the opened order counter before opening any of the positions, you can control how many opened buys or sells you have.
an example :
if openedBuys uis anything but 0 you do not open a new buy order. Same logic applies to sell (that is the second example in that post)
Hi Mladen,
Can you tell me more about the rationale of the functions here? I still don't quite get it. Thanks
TerranceHi Mladen,
Maybe I might have misrepresent myself. What I am trying to accomplish is when my buy criteria is met, it will trigger a buy and if another buy criteria is met again, it will trigger another buy OR sell if the sell criteria is met. However, the maximum trade that I will have at any point of time will be 2.
I have tried your method previously and I am still encountering the system opening 2 trades at the same time that's why i tried to limit it with the Bars function with the same issue of having the EA opening 2 trades at the same time.
What could be the possible cause here? Is it something to do with my logic?
Regards
Terrance
tkuan77
You wanted to limit orders to 1 buy and 1 sell at a time.
So if you call the opened order counter before opening any of the positions, you can control how many opened buys or sells you have.
an example :
You can add checking if an order of some type have already been opened at a current bar and that way you will prevent opening a new order on a next tick. If yes (there was an order opened on a current bar), then no opening new order. If no, you can open a new order. Here is a function that can count you orders of rewuired type opened at a current bar
{
int openedAtBar = 0;
datetime startTime = Time[0];
datetime endTime = Time[0]+Period()*60;
for(int i=0; i < OrdersTotal(); i++)
{
if(OrderSelect(i, SELECT_BY_POS, MODE_TRADES) == false) break;
if(OrderMagicNumber() != MagicNumber) continue;
if(OrderSymbol() != Symbol()) continue;
if(OrderType() != type) continue;
if(OrderOpenTime()=endTime) continue;
openedAtBar++;
break;
}
return(openedAtBar);
}
Hi Mladen,
Maybe I might have misrepresent myself. What I am trying to accomplish is when my buy criteria is met, it will trigger a buy and if another buy criteria is met again, it will trigger another buy OR sell if the sell criteria is met. However, the maximum trade that I will have at any point of time will be 2.
I have tried your method previously and I am still encountering the system opening 2 trades at the same time that's why i tried to limit it with the Bars function with the same issue of having the EA opening 2 trades at the same time.
What could be the possible cause here? Is it something to do with my logic?
Regards
TerranceHi Mladen,
Maybe I might have misrepresent myself. What I am trying to accomplish is when my buy criteria is met, it will trigger a buy and if another buy criteria is met again, it will trigger another buy OR sell if the sell criteria is met. However, the maximum trade that I will have at any point of time will be 2.
I have tried your method previously and I am still encountering the system opening 2 trades at the same time that's why i tried to limit it with the Bars function with the same issue of having the EA opening 2 trades at the same time.
What could be the possible cause here? Is it something to do with my logic?
Regards
Terrancethat is because you didnt specify how you want the second trade to be different from the first. Differentiation could be in the form of x no. of bars or price away from the FirstBuyPrice.
Thanks Mladen for the help, however I just want to check with you, do I put the code before the my long/short function, in between or somewhere else? Because it seems to keep popping up error and I cant seem to find the source of it. And is MagicNumber suppose to be a integer?
Terrance
total = OrdersTotal();
if(total < 2)
{
int countOpenedOnACurrentBar(int type)
{
int openedAtBar = 0;
datetime startTime = Time[0];
datetime endTime = Time[0]+Period()*60;
for(int i=0; i < OrdersTotal(); i++)
{
if(OrderSelect(i, SELECT_BY_POS, MODE_TRADES) == false) break;
if(OrderMagicNumber() != MagicNumber) continue;
if(OrderSymbol() != Symbol()) continue;
if(OrderType() != type) continue;
if(OrderOpenTime()=endTime) continue;
openedAtBar++;
break;
}
return(openedAtBar);
}
if(isCrossed == 1 && shortEma > mainshortEma && longEma > mainshortEma)
{
ticket=OrderSend(Symbol(),OP_BUY,Lots,Ask,1,Ask-StopLoss*Point,Ask+TakeProfit*Point,
"My EA",12345,0,Green);
if(ticket>0)
{
if(OrderSelect(ticket,SELECT_BY_TICKET,MODE_TRADES))
Print("BUY order opened : ",OrderOpenPrice());
}
else Print("Error opening BUY order : ",GetLastError());
return(0);
}
if(isCrossed == 2 && shortEma < mainshortEma && longEma < mainshortEma)
{
ticket=OrderSend(Symbol(),OP_SELL,Lots,Bid,1,Bid+StopLoss*Point,Bid-TakeProfit*Point,
"My EA",12345,0,Red);
if(ticket>0)
{
if(OrderSelect(ticket,SELECT_BY_TICKET,MODE_TRADES))
Print("SELL order opened : ",OrderOpenPrice());
}
else Print("Error opening SELL order : ",GetLastError());
return(0);
}
return(0);
}
You can add checking if an order of some type have already been opened at a current bar and that way you will prevent opening a new order on a next tick. If yes (there was an order opened on a current bar), then no opening new order. If no, you can open a new order. Here is a function that can count you orders of rewuired type opened at a current bar
{
int openedAtBar = 0;
datetime startTime = Time[0];
datetime endTime = Time[0]+Period()*60;
for(int i=0; i < OrdersTotal(); i++)
{
if(OrderSelect(i, SELECT_BY_POS, MODE_TRADES) == false) break;
if(OrderMagicNumber() != MagicNumber) continue;
if(OrderSymbol() != Symbol()) continue;
if(OrderType() != type) continue;
if(OrderOpenTime()=endTime) continue;
openedAtBar++;
break;
}
return(openedAtBar);
}
...
Terrance
That is a function. Post it at the end of your EA and place calls to it where you think it is appropriate to check if an order is already opened at a current bar
Thanks Mladen for the help, however I just want to check with you, do I put the code before the my long/short function, in between or somewhere else? Because it seems to keep popping up error and I cant seem to find the source of it. And is MagicNumber suppose to be a integer?
Terrance
total = OrdersTotal();
if(total < 2)
{
int countOpenedOnACurrentBar(int type)
{
int openedAtBar = 0;
datetime startTime = Time[0];
datetime endTime = Time[0]+Period()*60;
for(int i=0; i < OrdersTotal(); i++)
{
if(OrderSelect(i, SELECT_BY_POS, MODE_TRADES) == false) break;
if(OrderMagicNumber() != MagicNumber) continue;
if(OrderSymbol() != Symbol()) continue;
if(OrderType() != type) continue;
if(OrderOpenTime()=endTime) continue;
openedAtBar++;
break;
}
return(openedAtBar);
}
if(isCrossed == 1 && shortEma > mainshortEma && longEma > mainshortEma)
{
ticket=OrderSend(Symbol(),OP_BUY,Lots,Ask,1,Ask-StopLoss*Point,Ask+TakeProfit*Point,
"My EA",12345,0,Green);
if(ticket>0)
{
if(OrderSelect(ticket,SELECT_BY_TICKET,MODE_TRADES))
Print("BUY order opened : ",OrderOpenPrice());
}
else Print("Error opening BUY order : ",GetLastError());
return(0);
}
if(isCrossed == 2 && shortEma < mainshortEma && longEma < mainshortEma)
{
ticket=OrderSend(Symbol(),OP_SELL,Lots,Bid,1,Bid+StopLoss*Point,Bid-TakeProfit*Point,
"My EA",12345,0,Red);
if(ticket>0)
{
if(OrderSelect(ticket,SELECT_BY_TICKET,MODE_TRADES))
Print("SELL order opened : ",OrderOpenPrice());
}
else Print("Error opening SELL order : ",GetLastError());
return(0);
}
return(0);
}