To draw a line you can use this:
bool drawLine( string name, //id datetime t1, double p1, // point 'from' datetime t2, double p2, // point 'to' color clr=clrDimGray, string dscr="", double stl=STYLE_SOLID, double wdt=1, bool asBackground=false) { if(ObjectFind(name) >= 0) ObjectDelete(name); ObjectCreate(name,OBJ_TREND,0,t1,p1, t2, p2); ObjectSet(name,OBJPROP_COLOR,clr); ObjectSet(name,OBJPROP_STYLE,STYLE_SOLID); ObjectSet(name, OBJPROP_RAY, false); ObjectSet(name,OBJPROP_WIDTH,wdt); ObjectSetText(name,dscr,10); if ( asBackground ) ObjectSet(name,OBJPROP_BACK,asBackground); return(true); }
To draw a line you can use this:
Nice function, thanks, but this won't fix my problem. It's not precise enough on the X axis, because no matter what "point 'to' time" I enter, it will always stick to the time of the closest bar, i.e. the "point 'to' time" value '2017.02.28 20:00:10' will always draw the line to '2017.02.28 20:00:00' on a M1 chart. Please check the screenshot I linked to.
What can I do, now?
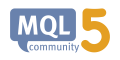
- www.mql5.com
Nice function, thanks, but this won't fix my problem. It's not precise enough on the X axis, because no matter what "point 'to' time" I enter, it will always stick to the time of the closest bar, i.e. the "point 'to' time" value '2017.02.28 20:00:10' will always draw the line to '2017.02.28 20:00:00' on a M1 chart. Please check the screenshot I linked to.
What can I do, now?
You can't use a trend line if you want to work with pixels.
Use Canvas and convert your timestamp to pixels with ChartTimePriceToXY(). You will also have to adjust your line each time the chart is "moved" with OnChartEvent().
Or if you want to keep it easy, forget the pixels and work with time, I don't see why it could be important to work with pixels for a trend line.
perfect

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Basically I want to draw a short horizontal trend line from a specific timestamp to any position X pixels to the left or right.
You might suspect I have problems with the zoom level, so I want to use pixel instead. While this is also the case, I already solved the issue by simply calculating the second timestamp and re-position the trend line every 5 seconds.
In that case I use
double zoom = ChartGetInteger(ChartID(),CHART_WIDTH_IN_BARS);
to get a zoom factor I can work with in further calculations for the second timestamp. Then I can use
ObjectSet("MyTrend",OBJPROP_TIME1,MyTimestamp));
ObjectSet("MyTrend",OBJPROP_TIME2,MyTimestamp + MyCalculatedShift);
It works, but I need a more precise way. When I zoom into my chart and the bars are very thick, the length steps of my trend line are big too, because the times will be oriented on the times of the bars.
I need to be able to draw a trend line at least to a quarter of a bar (15 second preciese min on a 1M period) or a way to draw the trend with a length in pixel.
I was digging into using a canvas (CCanvas object), but this will draw the lines without respecting the horizontal scroll position or the vertical zoom level. However, one of the ends of the trend line has to be fixed on a timestamp and it has to move with horizontal scroll and vertical zoom. Also, the Y position (height) must be on a specific price point.
I'm out of my ideas for now. If you need a practical case for this requirement, see: https://www.dropbox.com/s/kmc9s84x20jzomr/Screenshot%202017-03-10%2001.50.06.png?dl=0
It's a vertical oriented chart inside the chart and it's fixed on a specific timestamp. The vertical chart has to be very precise. At best, there should be 40 different levels of the vertical chart pillars and the maximum length could even be only 1 bar (small window, max zoom in), but anything preciser than now would be appreciated. The image shows different zoom levels from far to close from left to right. As you can see, the more I zoom in, the less precise my chart gets, because the timestamp points are fixed to the bar positions.
Does anybody have a clue what I can go for to fix this issue? Thank you!