EDIT: Now it works like above even without the use of ArrayInitialize(). So now the only problem is why indicator buffer values are removed and my candles disappear when I hit refresh?
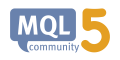
- www.mql5.com
All indicator buffers should have empty values there :(
You are only setting one buffer (buffer 0) to have an initial setting of 0, try setting the initial condition of all buffers to EMPTY_VALUE
PlotIndexSetDouble(0,PLOT_EMPTY_VALUE, EMPTY_VALUE); PlotIndexSetDouble(1,PLOT_EMPTY_VALUE, EMPTY_VALUE); PlotIndexSetDouble(2,PLOT_EMPTY_VALUE, EMPTY_VALUE); PlotIndexSetDouble(3,PLOT_EMPTY_VALUE, EMPTY_VALUE); PlotIndexSetDouble(4,PLOT_EMPTY_VALUE, EMPTY_VALUE);
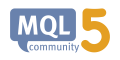
- www.mql5.com
You are only setting one buffer (buffer 0) to have an initial setting of 0, try setting the initial condition of all buffers to EMPTY_VALUE
Hi and thanks for the reply. I tried what you suggested, but I still get unwanted results. Below code seems to work fine some of the time but when I switch between H1 and M30 for a few times the candles are drawn incorrectly:
//+------------------------------------------------------------------+ //| COLOR_CANDLES.mq5 | //| Copyright 2013, MetaQuotes Software Corp. | //| http://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2013, MetaQuotes Software Corp." #property link "http://www.mql5.com" #property version "1.00" #property indicator_chart_window #property indicator_buffers 5 #property indicator_plots 1 #property indicator_type1 DRAW_COLOR_CANDLES #property indicator_label1 "Open;High;Low;Close" #property indicator_width1 3 double o[],h[],l[],c[],clr[]; //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- indicator buffers mapping SetIndexBuffer(0,o,INDICATOR_DATA); SetIndexBuffer(1,h,INDICATOR_DATA); SetIndexBuffer(2,l,INDICATOR_DATA); SetIndexBuffer(3,c,INDICATOR_DATA); SetIndexBuffer(4,clr,INDICATOR_COLOR_INDEX); PlotIndexSetDouble(0,PLOT_EMPTY_VALUE,EMPTY_VALUE); PlotIndexSetDouble(1,PLOT_EMPTY_VALUE,EMPTY_VALUE); PlotIndexSetDouble(2,PLOT_EMPTY_VALUE,EMPTY_VALUE); PlotIndexSetDouble(3,PLOT_EMPTY_VALUE,EMPTY_VALUE); PlotIndexSetDouble(4,PLOT_EMPTY_VALUE,EMPTY_VALUE); PlotIndexSetInteger(0,PLOT_COLOR_INDEXES,1); PlotIndexSetInteger(0,PLOT_LINE_COLOR,0,Blue); //--- return(0); } //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]) { //--- for(int i=prev_calculated;i<rates_total-1;i++) { if(close[i]>open[i]) { o[i]=open[i]; h[i]=high[i]; l[i]=low[i]; c[i]=close[i]; clr[i]=0; } } //--- return value of prev_calculated for next call return(rates_total-1); } //+------------------------------------------------------------------+
Hi my friend
I've tested your code is correct
but If you want to change the color of the candle.
SetIndexBuffer(4,clr,INDICATOR_COLOR_INDEX); //Assign the array with color indexes with the indicator's color indexes buffer PlotIndexSetInteger(0,PLOT_COLOR_INDEXES,4); //Set color for each index PlotIndexSetInteger(0,PLOT_LINE_COLOR,0,clrWhite); // 0th index clrWhite PlotIndexSetInteger(0,PLOT_LINE_COLOR,1,clrAqua); // 1st index clrAqua PlotIndexSetInteger(0,PLOT_LINE_COLOR,2,clrGreen); // 2nd index clrGreen PlotIndexSetInteger(0,PLOT_LINE_COLOR,3,clrRed); // 3th index clrRed
Hi and thanks for the reply. I tried what you suggested, but I still get unwanted results. Below code seems to work fine some of the time but when I switch between H1 and M30 for a few times the candles are drawn incorrectly:
I think the buffers aren't getting cleared when the timeframe is changed . . . no idea why, try adding this, it seems to work quite reliably:
for(int i=prev_calculated;i<rates_total-1;i++) { o[i] = 0.0; h[i] = 0.0; l[i] = 0.0; c[i] = 0.0; // <----- add this line if(close[i]>open[i])
Hope you don't mind, I have taken the opportunity to learn a little about mql5 using your code . . . I have learned that a lot of things are different compared to mql4 and that not having a Book, as there is for mql4, is a pain in the behind.
This is where I got to, it seems to be OK in terms of changing timeframes and it will update the bar to the right of the currently forming bar when a new bar is formed. It does not update the currently forming bar, not sure if you wanted it to or not.
//+------------------------------------------------------------------+ //| COLOR_CANDLES.mq5 | //| Copyright 2013, MetaQuotes Software Corp. | //| http://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2013, MetaQuotes Software Corp." #property link "http://www.mql5.com" #property version "1.00" #property indicator_chart_window #property indicator_buffers 5 #property indicator_plots 1 #property indicator_type1 DRAW_COLOR_CANDLES #property indicator_label1 "Open;High;Low;Close" #property indicator_width1 3 #property indicator_color1 Blue double o[],h[],l[],c[],clr[]; //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- indicator buffers mapping SetIndexBuffer(0,o,INDICATOR_DATA); SetIndexBuffer(1,h,INDICATOR_DATA); SetIndexBuffer(2,l,INDICATOR_DATA); SetIndexBuffer(3,c,INDICATOR_DATA); SetIndexBuffer(4,clr,INDICATOR_COLOR_INDEX); //--- return(0); } //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]) { int FirstBar = 0, i; //--- if(prev_calculated == 0) FirstBar = 0; if(prev_calculated != 0) FirstBar = prev_calculated - 1; for(i = FirstBar; i < rates_total - 1; i++) { o[i] = 0.0; h[i] = 0.0; l[i] = 0.0; c[i] = 0.0; if(close[i]>open[i]) { o[i]=open[i]; h[i]=high[i]; l[i]=low[i]; c[i]=close[i]; clr[i]=0; } } o[i] = 0.0; h[i] = 0.0; l[i] = 0.0; c[i] = 0.0; // clar values for currently forming bar //--- return value of prev_calculated for next call return(rates_total); } //+------------------------------------------------------------------+

- 2012.08.02
- MetaQuotes Software Corp.
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi,
This is a common problem with my indicators. The buffers get strange values like 6.33435 -315 etc. Below is an example. When I open the data window I see that on some bars the four buffers have values. I will attach a screenshot of the phenomenon. Below is the code:
And here is the screen:
All indicator buffers should have empty values there :(
Br, Candles