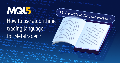
Documentation on MQL5: Custom Indicators / Indicator Styles in Examples / DRAW_COLOR_ARROW
- www.mql5.com
The DRAW_COLOR_ARROW style draws colored arrows (symbols of the set Wingdings ) based on the value of the indicator buffer. In contrast to...
I was going to post this link as well ^
note, from the reference:
The number of buffers required for plotting DRAW_COLOR_ARROW is 2.
- a buffer to store the value of the price which is used to draw the symbol (plus a shift in pixels, given in the PLOT_ARROW_SHIFT property);
- a buffer to store the color index, which is used to draw an arrow (it makes sense to set only non-empty values).
Change your start index so that it removes unnecessary processing overhead
int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]) { if (rates_total<3) return (0); if (prev_calculated<5) { ArrayInitialize(buffer,EMPTY_VALUE); } int start = (prev_calculated == 0) ? 1 : prev_calculated - 1; for (int i=start;i<rates_total-1 && !IsStopped();i++) { if ((high[i]<high[i-1] && low[i]>low[i-1])) { buffer[i]=(high[i]+low[i])/2; } else buffer[i]=EMPTY_VALUE; } return (rates_total); }
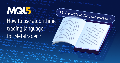
Documentation on MQL5: Custom Indicators / Indicator Styles in Examples / DRAW_COLOR_ARROW
- www.mql5.com
The DRAW_COLOR_ARROW style draws colored arrows (symbols of the set Wingdings ) based on the value of the indicator buffer. In contrast to...
Your topic has been moved to the section: Technical Indicators
Please consider which section is most appropriate — https://www.mql5.com/en/forum/172166/page6#comment_49114893
Please consider which section is most appropriate — https://www.mql5.com/en/forum/172166/page6#comment_49114893
Got it. Thanks guys!

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Hey guys,
I made a small indicator which shows inside candles. Now I want to check if the candle's close is above or below the open price and I want to change the color of the dot accordingly.
I know how to do this with two buffers but I want to know if it's possible with one buffer and how I could change the color of the buffer depending on the candle's close?