Tomq:
2025.02.05 23:14:28.843 2025.01.20 05:51:32 failed modify #2 buy 1 XAUUSD sl: 0.00, tp: 0.00 -> sl: 0.00, tp: 0.00 [Invalid stops]
2025.02.05 23:14:28.843 2025.01.20 05:51:32 failed modify #2 buy 1 XAUUSD sl: 0.00, tp: 0.00 -> sl: 0.00, tp: 0.00 [Invalid stops]
If you're trading a 2 or 3 digit symbol, you might not be respecting your broker-dealer's minimum stop distance. This will cause an invalid stops error.
See SYMBOL_TRADE_STOPS_LEVEL:
Symbol Properties - Environment State - Constants, Enumerations and Structures - MQL5 Reference - Reference on algorithmic/automated trading language for MetaTrader 5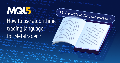
Documentation on MQL5: Constants, Enumerations and Structures / Environment State / Symbol Properties
- www.mql5.com
To obtain the current market information there are several functions: SymbolInfoInteger() , SymbolInfoDouble() and SymbolInfoString() . The first...
#include <Trade\Trade.mqh> CTrade trade; int be_points = 200; void BreakEven() { CPositionInfo pos; double bid = SymbolInfoDouble(pos.Symbol(), SYMBOL_BID); double ask = SymbolInfoDouble(pos.Symbol(), SYMBOL_ASK); for (int i=0; i<PositionsTotal(); i++) { if (pos.SelectByIndex(i) && pos.Symbol()==_Symbol && pos.Magic()==Expert_Magic) { if (pos.PositionType() == POSITION_TYPE_BUY) { double be_target = (pos.PriceOpen() + be_points*_Point)); if (pos.StopLoss()<=pos.PriceOpen() && bid>=be_target) { trade.PositionModify(pos.Ticket(), pos.PriceOpen(), pos.TakeProfit()); } } else if (pos.PositionType() == POSITION_TYPE_SELL) { double be_target = (pos.PriceOpen() - be_points*_Point)); if (pos.StopLoss()>=pos.PriceOpen() && bid<=be_target) { trade.PositionModify(pos.Ticket(), pos.PriceOpen(), pos.TakeProfit()); } } } } }
I use a different approach as yours, this code snippet is easier to read.
Your code's conditional:
sl == 0
Is now this:
pos.StopLoss()>=pos.PriceOpen()
So in the future if you want to add a Stoploss to your strategy it can adapt accordingly.

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Hi all,
I use this code to set Breakeven for my EA, but i got message :
I tried many times to correct it but not work. Please help me to correct it. Thank you so much