I have:
bool MyVar = false;
When I try and display a comment:
Comment ("MyVar: " + MyVar);
I get implicit conversion from 'number' to 'string' warning when compiling.
In MT4 it would compile OK and print a 'one' or 'zero' corresponding to true or false. How do we now display booleans in a Comment?
-Jerry
This is due to more stringent type-checking in MQL5, which has many benefits of picking up coding errors.
If you want to eliminate the warnings, you need to explicitly type-cast the variable, as in
Comment ("MyVar: " + (string)MyVar);
Or use the multiple parameter feature of Comment()
Comment ("MyVar: ",MyVar);
Or perhaps better still
Comment ("MyVar: ",BoolToString(MyVar)); string BoolToString(bool b) { if (b) return("true"); else return("false"); }
Paul
- 2012.06.14
- Paul
- paulsfxrandomwalk.blogspot.com
string BoolToString(bool b) { if (b) return("true"); else return("false"); }
Thanks Paul. That's a great Help.Actually BoolToString would be a useful command to be included in MT5.
-Jerry
Glad you like BoolToString - attached is a stack of string utilities :)
From time to time this will need updating as MetaQuotes adds extra error codes.
Paul
- 2012.06.14
- Paul
- paulsfxrandomwalk.blogspot.com
HELP ME I HAVE THE SAME PROBLEM
//+------------------------------------------------------------------+
//| binaryinvest-lib.mq4 |
//| Copyright 2017, MetaQuotes Software Corp. |
//| https://www.mql5.com |
//+------------------------------------------------------------------+
#property library
#property copyright "Copyright 2017, MetaQuotes Software Corp."
#property link "https://www.mql5.com"
#property version "1.00"
#property strict
//+------------------------------------------------------------------+
//| My function |
//+------------------------------------------------------------------+
// int MyCalculator(int value,int value2) export
// {
// return(value+value2);
// }
//+------------------------------------------------------------------+
#import "shell32.dll"
int ShellExecuteW(
int hwnd,
string Operation,
string File,
string Parameters,
string Directory,
int ShowCmd
);
#import
#import "wininet.dll"
int InternetOpenW(
string sAgent,
int lAccessType,
string sProxyName="",
string sProxyBypass="",
int lFlags=0
);
int InternetOpenUrlW(
int hInternetSession,
string sUrl,
string sHeaders="",
int lHeadersLength=0,
uint lFlags=0,
int lContext=0
);
int InternetReadFile(
int hFile,
uchar & sBuffer[],
int lNumBytesToRead,
int& lNumberOfBytesRead
);
int InternetCloseHandle(
int hInet
);
#import
#define INTERNET_FLAG_RELOAD 0x80000000
#define INTERNET_FLAG_NO_CACHE_WRITE 0x04000000
#define INTERNET_FLAG_PRAGMA_NOCACHE 0x00000100
int hSession_IEType;
int hSession_Direct;
int Internet_Open_Type_Preconfig = 0;
int Internet_Open_Type_Direct = 1;
int hSession(bool Direct)
{
string InternetAgent = "Mozilla/4.0 (compatible; MSIE 6.0; Windows NT 5.1; Q312461)";
if (Direct)
{
if (hSession_Direct == 0)
{
hSession_Direct = InternetOpenW(InternetAgent, Internet_Open_Type_Direct, "0", "0", 0);
}
return(hSession_Direct);
}
else
{
if (hSession_IEType == 0)
{
hSession_IEType = InternetOpenW(InternetAgent, Internet_Open_Type_Preconfig, "0", "0", 0);
}
return(hSession_IEType);
}
}
string httpGET(string strUrl)
{
int handler = hSession(false);
int response = InternetOpenUrlW(handler, strUrl, NULL, 0,
INTERNET_FLAG_NO_CACHE_WRITE |
INTERNET_FLAG_PRAGMA_NOCACHE |
INTERNET_FLAG_RELOAD, 0);
if (response == 0)
return(false);
uchar ch[100]; string toStr=""; int dwBytes, h=-1;
while(InternetReadFile(response, ch, 100, dwBytes))
{
if (dwBytes<=0) break; toStr=toStr+CharArrayToString(ch, 0, dwBytes);
}
InternetCloseHandle(response);
return toStr;
}
void httpOpen(string strUrl)
{
Shell32::ShellExecuteW(0, "open", strUrl, "", "", 3);
}
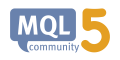
- www.mql5.com
Forum on trading, automated trading systems and testing trading strategies
Hello,
Please EDIT your post and use the SRC button when you post code.
Thank you.
- //+------------------------------------------------------------------+
//| Indicator: BUY.mq5 |
//| Created with EABuilder.com |
//| http://eabuilder.com |
//+------------------------------------------------------------------+
#property copyright "Created with EABuilder.com"
#property link "http://eabuilder.com"
#property version "1.00"
#property description "" - //--- indicator settings
#property indicator_chart_window
#property indicator_buffers 2
#property indicator_plots 2 - #property indicator_type1 DRAW_ARROW
#property indicator_width1 1
#property indicator_color1 0xFFAA00
#property indicator_label1 "'BUY" - #property indicator_type2 DRAW_ARROW
#property indicator_width2 1
#property indicator_color2 0x0000FF
#property indicator_label2 "'BUY" - //--- indicator buffers
double Buffer1[];
double Buffer2[]; - datetime time_alert; //used when sending alert
bool Audible_Alerts = true;
double myPoint; //initialized in OnInit
int BearsPower_handle;
double BearsPower[];
int BullsPower_handle;
double BullsPower[];
int Volumes_handle;
double Volumes[];
double Low[]; - void myAlert(string type, string message)
{
if(type == "print")
Print(message);
else if(type == "error")
{
Print(type+" | BUY @ "+Symbol()+","+Period()+" | "+message);
}
else if(type == "order")
{
}
else if(type == "modify")
{
}
else if(type == "indicator")
{
Print(type+" | BUY @ "+Symbol()+","+Period()+" | "+message);
if(Audible_Alerts) Alert(type+" | BUY @ "+Symbol()+","+Period()+" | "+message);
}
} - //+------------------------------------------------------------------+
//| Custom indicator initialization function |
//+------------------------------------------------------------------+
int OnInit()
{
SetIndexBuffer(0, Buffer1);
PlotIndexSetDouble(0, PLOT_EMPTY_VALUE, 0);
PlotIndexSetInteger(0, PLOT_ARROW, 241);
SetIndexBuffer(1, Buffer2);
PlotIndexSetDouble(1, PLOT_EMPTY_VALUE, 0);
PlotIndexSetInteger(1, PLOT_ARROW, 241);
//initialize myPoint
myPoint = Point();
if(Digits() == 5 || Digits() == 3)
{
myPoint *= 10;
}
BearsPower_handle = iBearsPower(NULL, PERIOD_H1, 27);
if(BearsPower_handle < 0)
{
Print("The creation of iBearsPower has failed: BearsPower_handle=", INVALID_HANDLE);
Print("Runtime error = ", GetLastError());
return(INIT_FAILED);
}
BullsPower_handle = iBullsPower(NULL, PERIOD_M20, 27);
if(BullsPower_handle < 0)
{
Print("The creation of iBullsPower has failed: BullsPower_handle=", INVALID_HANDLE);
Print("Runtime error = ", GetLastError());
return(INIT_FAILED);
}
Volumes_handle = iVolumes(NULL, PERIOD_H1, VOLUME_TICK);
if(Volumes_handle < 0)
{
Print("The creation of iVolumes has failed: Volumes_handle=", INVALID_HANDLE);
Print("Runtime error = ", GetLastError());
return(INIT_FAILED);
}
return(INIT_SUCCEEDED);
} - //+------------------------------------------------------------------+
//| Custom indicator iteration function |
//+------------------------------------------------------------------+
int OnCalculate(const int rates_total,
const int prev_calculated,
const datetime& time[],
const double& open[],
const double& high[],
const double& low[],
const double& close[],
const long& tick_volume[],
const long& volume[],
const int& spread[])
{
int limit = rates_total - prev_calculated;
//--- counting from 0 to rates_total
ArraySetAsSeries(Buffer1, true);
ArraySetAsSeries(Buffer2, true);
//--- initial zero
if(prev_calculated < 1)
{
ArrayInitialize(Buffer1, 0);
ArrayInitialize(Buffer2, 0);
}
else
limit++;
datetime Time[];
if(CopyBuffer(BearsPower_handle, 0, 0, rates_total, BearsPower) <= 0) return(rates_total);
ArraySetAsSeries(BearsPower, true);
if(CopyBuffer(BullsPower_handle, 0, 0, rates_total, BullsPower) <= 0) return(rates_total);
ArraySetAsSeries(BullsPower, true);
if(CopyBuffer(Volumes_handle, 0, 0, rates_total, Volumes) <= 0) return(rates_total);
ArraySetAsSeries(Volumes, true);
if(CopyLow(Symbol(), PERIOD_CURRENT, 0, rates_total, Low) <= 0) return(rates_total);
ArraySetAsSeries(Low, true);
if(CopyTime(Symbol(), Period(), 0, rates_total, Time) <= 0) return(rates_total);
ArraySetAsSeries(Time, true);
//--- main loop
for(int i = limit-1; i >= 0; i--)
{
if (i >= MathMin(5000-1, rates_total-1-50)) continue; //omit some old rates to prevent "Array out of range" or slow calculation
//Indicator Buffer 1
if(BearsPower[i] < 0 //Bears Power < fixed value
&& BullsPower[i] > BullsPower[1+i] //Bulls Power > Bulls Power
&& BullsPower[i] > 0 //Bulls Power > fixed value
&& BullsPower[1+i] > 0 //Bulls Power > fixed value
&& Volumes[i] > Volumes[1+i] //Volumes > Volumes
)
{
Buffer1[i] = Low[i]; //Set indicator value at Candlestick Low
if(i == 0) myAlert("indicator", "'BUY"); //Instant alert, unlimited
}
else
{
Buffer1[i] = 0;
}
//Indicator Buffer 2
if(BearsPower[i] > 0 //Bears Power > fixed value
&& BullsPower[i] > BullsPower[1+i] //Bulls Power > Bulls Power
&& BullsPower[i] > 0 //Bulls Power > fixed value
&& BullsPower[1+i] > 0 //Bulls Power > fixed value
&& BullsPower[2+i] > BullsPower[1+i] //Bulls Power > Bulls Power
&& Volumes[i] > Volumes[1+i] //Volumes > Volumes
)
{
Buffer2[i] = Low[i]; //Set indicator value at Candlestick Low
if(i == 0) myAlert("indicator", "'BUY"); //Instant alert, unlimited
}
else
{
Buffer2[i] = 0;
}
}
return(rates_total);
}
//+------------------------------------------------------------------+
- www.eabuilder.com
Good morning house. please kindly help me, I have an indicator that displayed 33 warnings after compiling it, but I don`t have idea of what to change . I want to ask for permission before pasting it.
Thanks
HELP ME I HAVE THE SAME PROBLEM
You just have to figure out WHAT you want your function to return - a string? or bool?
string httpGET(string strUrl) { int handler = hSession(false); int response = InternetOpenUrlW(handler, strUrl, NULL, 0, INTERNET_FLAG_NO_CACHE_WRITE | INTERNET_FLAG_PRAGMA_NOCACHE | INTERNET_FLAG_RELOAD, 0); if (response == 0) return(false); uchar ch[100]; string toStr=""; int dwBytes, h=-1; while(InternetReadFile(response, ch, 100, dwBytes)) { if (dwBytes<=0) break; toStr=toStr+CharArrayToString(ch, 0, dwBytes); } InternetCloseHandle(response); return toStr; }
Just have to add the highlighted parts:
void myAlert(string type, string message) { if(type == "print") Print(message); else if(type == "error") { Print(type+" | BUY @ "+Symbol()+","+EnumToString(Period())+" | "+message); } else if(type == "order") { } else if(type == "modify") { } else if(type == "indicator") { Print(type+" | BUY @ "+Symbol()+","+EnumToString(Period())+" | "+message); if(Audible_Alerts) Alert(type+" | BUY @ "+Symbol()+","+EnumToString(Period())+" | "+message); } }

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I have:
bool MyVar = false;
When I try and display a comment:
Comment ("MyVar: " + MyVar);
I get implicit conversion from 'number' to 'string' warning when compiling.
In MT4 it would compile OK and print a 'one' or 'zero' corresponding to true or false. How do we now display booleans in a Comment?
-Jerry