- Needs Help in coding.
- NEED A HELP PLEASE
- Trailing Stop EA
-
//| Profit Maker V3.mq4 |
Why did you post your MT4 question in the MT5 EA section instead of the MQL4 section, (bottom of the Root page)?
General rules and best pratices of the Forum. - General - MQL5 programming forum? (2017)
Next time, post in the correct place. The moderators will likely move this thread there soon. Your code if (MA1 > MA2 || MA2 > MA1) return true; else return false;
Simplified return MA1 > MA2 || MA2 > MA1;
-
double tempTP = Ask + (tp_points * Point()); double tempSL = Ask - (sl_points * Point());
You buy at the Ask and sell at the Bid. Pending Buy Stop orders become market orders when hit by the Ask.
-
Your buy order's TP/SL (or Sell Stop's/Sell Limit's entry) are triggered when the Bid / OrderClosePrice reaches it. Using Ask±n, makes your SL shorter and your TP longer, by the spread. Don't you want the specified amount used in either direction?
-
Your sell order's TP/SL (or Buy Stop's/Buy Limit's entry) will be triggered when the Ask / OrderClosePrice reaches it. To trigger close at a specific Bid price, add the average spread.
MODE_SPREAD (Paul) - MQL4 programming forum - Page 3 #25 -
The charts show Bid prices only. Turn on the Ask line to see how big the spread is (Tools → Options (control+O) → charts → Show ask line.)
Most brokers with variable spreads widen considerably at end of day (5 PM ET) ± 30 minutes.
My GBPJPY shows average spread = 26 points, average maximum spread = 134.
My EURCHF shows average spread = 18 points, average maximum spread = 106.
(your broker will be similar).
Is it reasonable to have such a huge spreads (20 PIP spreads) in EURCHF? - General - MQL5 programming forum (2022)
-
-
ulong lastClosedTradeTicket = OrderSelect(OrdersHistoryTotal() - 1, SELECT_BY_POS, MODE_HISTORY) ? OrderTicket() : 0;
-
Do not assume history has only closed orders.
OrderType() == 6, 7 in the history pool? - MQL4 programming forum #4 and #5 (2017) -
Do not assume history is ordered by date, it's not.
Could EA Really Live By Order_History Alone? (ubzen) - MQL4 programming forum (2012)
Taking the last profit and storing it in a variable | MQL4 - MQL4 programming forum #3 (2020) -
Magic number only allows an EA to identify its trades from all others. Using OrdersTotal/OrdersHistoryTotal (MT4) or PositionsTotal (MT5), directly and/or no Magic number/symbol filtering on your OrderSelect / Position select loop means your code is incompatible with every EA (including itself on other charts and manual trading.)
Symbol Doesn't equal Ordersymbol when another currency is added to another seperate chart . - MQL4 programming forum (2013)
PositionClose is not working - MQL5 programming forum (2020)
MagicNumber: "Magic" Identifier of the Order - MQL4 Articles (2006)
Orders, Positions and Deals in MetaTrader 5 - MQL5 Articles (2011)
Limit one open buy/sell position at a time - General - MQL5 programming forum (2022)You need one Magic Number for each symbol/timeframe/strategy.
Trade current timeframe, one strategy, and filter by symbol requires one MN.
If trading multiple timeframes, and filter by symbol requires use a range of MN (base plus timeframe).
Why are MT5 ENUM_TIMEFRAMES strange? - General - MQL5 programming forum - Page 2 #11 (2020)
-
-
if(is_trade_on_candle_close && Volume[0] <= 1)
For a new bar test, Bars is unreliable (a refresh/reconnect can change number of bars on chart), volume is unreliable (miss ticks), Price is unreliable (duplicate prices and The == operand. - MQL4 programming forum.) Always use time.
MT4: New candle - MQL4 programming forum #3 (2014)
MT5: Accessing variables - MQL4 programming forum #3 (2022)I disagree with making a new bar function, because it can only be called once per tick (second call returns false). A variable can be tested multiple times.
Running EA once at the start of each bar - MQL4 programming forum (2011) -
current_lot = NormalizeDouble(current_risk_amount / ((current_open_price - current_sl_price)/Point()), 2);
Risk depends on your initial stop loss, lot size, and the value of the symbol. It does not depend on margin and leverage. No SL means you have infinite risk (on leveraged symbols). Never risk more than a small percentage of your trading funds, certainly less than 2% per trade, 6% total.
-
You place the stop where it needs to be — where the reason for the trade is no longer valid. E.g. trading a support bounce, the stop goes below the support.
-
AccountBalance * percent/100 = RISK = OrderLots * (|OrderOpenPrice - OrderStopLoss| * DeltaPerLot + CommissionPerLot) (Note OOP-OSL includes the spread, and DeltaPerLot is usually around $10/PIP, but it takes account of the exchange rates of the pair vs. your account currency.)
-
Do NOT use TickValue by itself - DeltaPerLot and verify that MODE_TICKVALUE is returning a value in your deposit currency, as promised by the documentation, or whether it is returning a value in the instrument's base currency.
MODE_TICKVALUE is not reliable on non-fx instruments with many brokers - MQL4 programming forum (2017)
Is there an universal solution for Tick value? - Currency Pairs - General - MQL5 programming forum (2018)
Lot value calculation off by a factor of 100 - MQL5 programming forum (2019) -
You must normalize lots properly and check against min and max.
-
You must also check Free Margin to avoid stop out
-
For MT5, see 'Money Fixed Risk' - MQL5 Code Base (2017)
Most pairs are worth about $10 per PIP. A $5 risk with a (very small) 5 PIP SL is $5/$10/5 or 0.1 Lots maximum.
-
-
if(OrdersTotal() != 0) { for(int i=0; i<=OrdersTotal()+2; i++) { OrderSelect(i,SELECT_BY_POS,MODE_TRADES);
When i i greater or equal to OrdersTotal, the select fails but you don't check. -
In the presence of multiple orders (one EA multiple charts, multiple EAs, manual trading), while you are waiting for the current operation (closing, deleting, modifying) to complete, any number of other operations on other orders could have concurrently happened and changed the position indexing and order count:
-
For non-FIFO (non-US brokers), (or the EA only opens one order per symbol), you can simply count down, in an index loop, and you won't miss orders. Get in the habit of always counting down.
Loops and Closing or Deleting Orders - MQL4 programming forum -
For In First Out (FIFO rules — US brokers), and you (potentially) process multiple orders per symbol, you must find the earliest order (count up), close it, and on a successful operation, reprocess all positions (from zero).
CloseOrders by FIFO Rules - Strategy Tester - MQL4 programming forum - Page 2 #16
MetaTrader 5 platform beta build 2155: MQL5 scope, global Strategy Tester and built-in Virtual Hosting updates - Best Expert Advisors - General - MQL5 programming forum #1.11
and check OrderSelect in case other positions were deleted.
What are Function return values ? How do I use them ? - MQL4 programming forum
Common Errors in MQL4 Programs and How to Avoid Them - MQL4 Articlesand if you (potentially) process multiple orders, must call RefreshRates() after server calls if you want to use, on the next order / server call, the Predefined Variables (Bid/Ask.) Or instead, be direction independent and just use OrderClosePrice().
-
If you want to learn to code, then you need to put in more effort and dedicate some time to researching the information.
If you can't do that, then consider hiring someone to code it for you.
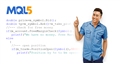
- 2023.05.30
- www.mql5.com
Help you with what? You haven't stated a problem, you stated a want. Show us your attempt (using the CODE button) and state the nature of your difficulty.
No free help (2017)
Or pay someone. Top of every page is the link Freelance.
Hiring to write script - General - MQL5 programming forum (2018)
We're not going to code it for you (although it could happen if you are lucky or the issue is interesting).
No free help (2017)

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use