To demonstrate the issue I wrote a EA, which I uploaded to the market (https://www.mql5.com/en/market/product/97058) .
I am not discussion an EA, this is just to demonstrate the issue. I will delete the EA from the market, after the issue has been resovled.
(I assume it is a bug, because I do not see why a demo EA should have such restrictions.)
Here a video demonstrating the issue : Attached the .ex5 Here the complete code: That is a bug right? Thanks for any help.
I understand that this is an issue that you are addressing and I personally will not remove the thread, however I will be removing the ".ex5" file attachment.
Instead, please attach the full ".mq5" file for testing and not the ".ex5".
Can you please clarify the following.
Are you saying that when downloaded as an activated product it does work correctly with the "common" folder, but not when it is downloaded as a "demo" version for running in the tester?
When you run the normal source code in the Strategy Tester, does it work as you expect?
Can you please clarify the following.
Are you saying that when downloaded as an activated product it does work correctly with the "common" folder, but not when it is downloaded as a "demo" version for running in the tester?
When you run the normal source code in the Strategy Tester, does it work as you expect?
Hi,
yes I have an EA on the market, with exactly this issue. Activated product and/or source code in Strategy tester works fine. Only the Demo version provided via the mql5.com market has this issue. I was able to reproduce the issue with the EA provided here (, which I wrote to demonstrate the issue).
So yes, you understood correctly.
Best, Julian.
I can confirm the issue.
I ran a simple optimisation of the provided source code in the Strategy Tester and a file "DemoFile.csv" was produced in the "%APPDATA%\Roaming\MetaQuotes\Terminal\Common\Files" directory.
I then ran the exact same settings with demo version of the product, but no file was produced.
I now wonder if this is a limitation of Market products as some kind of security measure?
I say this, because I have read that "FILE_COMMON" is not supported in MQL5 Cloud testing. So maybe they applied the same condition to demo downloads.
The question remains however, does this also happen with activated products (instead of demo downloads)?
Ok, I have asked a more experienced moderator (he is currently offline) to have a look at this later and give his input.
Hopefully, he will either offer a solution or report it to the devs/admin if he concludes that it is indeed a bug.
However, even if he does report it, it may be some time before MetaQuotes addresses it.
Ok, I have asked a more experienced moderator (he is currently offline) to have a look at this later and give his input.
Hopefully, he will either offer a solution or report it to the devs/admin if he concludes that it is indeed a bug.
However, even if he does report it, it may be some time before MetaQuotes addresses it.
Thanks for the quick answer. I just double checked and it has nothing to do with the common folder.
For testing I uploaded a second EA that does not write in the common folder, but in the mql5/files folder. https://www.mql5.com/en/market/product/97097
The issue persists. (Please be assured that I will delete those EAs after the issue was addressed/resolved).
By the way it is possible to write to the common folder when using the cloud with the offline version (I just checked).
So I can confirm that demo versions cannot write files, independent of using the common flag or not.
I assume it is a bug, because writing files is already in a sandbox.
Thanks for helping! Highly appreciated.
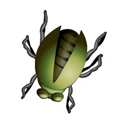
- www.mql5.com
Could you tell me full path for file ?
Show Experts log messages please.
Did this message appear in the experts log ?
printf("Error %i creating tester file",GetLastError());
Please note that the OP has provided full source code for the test in his posts which shows the full path ...
input string filename = "DemoFile";
m_filehandle=FileOpen(filename+".csv" ,FILE_READ|FILE_WRITE|FILE_CSV|FILE_COMMON);
In my own tests, there was no error message in the log (both in normal Tester Journal log and the individual Agent logs).
EDIT: There was also no message in the Experts log either.
EDIT: And the "common" directory I was monitoring was ...
%APPDATA%\Roaming\MetaQuotes\Terminal\Common
EDIT2: And to make extra sure, I also looked at the normal "MQL5\files" and "testing_agent_directory\MQL5\files" too.C:\Users\F.M.I. Carreiro\AppData\Roaming\MetaQuotes\Terminal\Common
Please note that the OP has provided full source code for the test in his posts which shows the full path ...
In my own tests, there was no error message in the log (both in normal Tester Journal log and the individual Agent logs).
EDIT: And the "common" directory I was monitoring was ...
Attached the optimization log.
I just noticed a line that I have overlooked so far:
" Tester OnTesterInit, OnTesterPass and OnTesterDeinit skipped because 'BugNoFilesWritten' is a demo version ".
When OnTesterDeinit is skipped, then obviously the code can not work.
But now I wonder what is the point in limiting demo versions to such a degree?. A potential customer cannot fully test an EA without OnTesterInit, OnTesterPass and OnTesterDeinit.
Regards, Julian.


- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
To whom it may concern,
I am facing the issue that apperently demo versions of market EAs (provided via mql5.com market) are incapable of writing files to the common/agent folder.
To demonstrate the issue I wrote an EA, which I uploaded to the market (https://www.mql5.com/en/market/product/97058).
I am not discussion an EA, this is just to demonstrate the issue. I will delete the EA from the market, after the issue has been resovled.
(I assume it is a bug, because I do not see why a demo EA should have such restrictions.)
Here a video demonstrating the issue :
Here the complete code:
That is a bug right?
Thanks for any help.